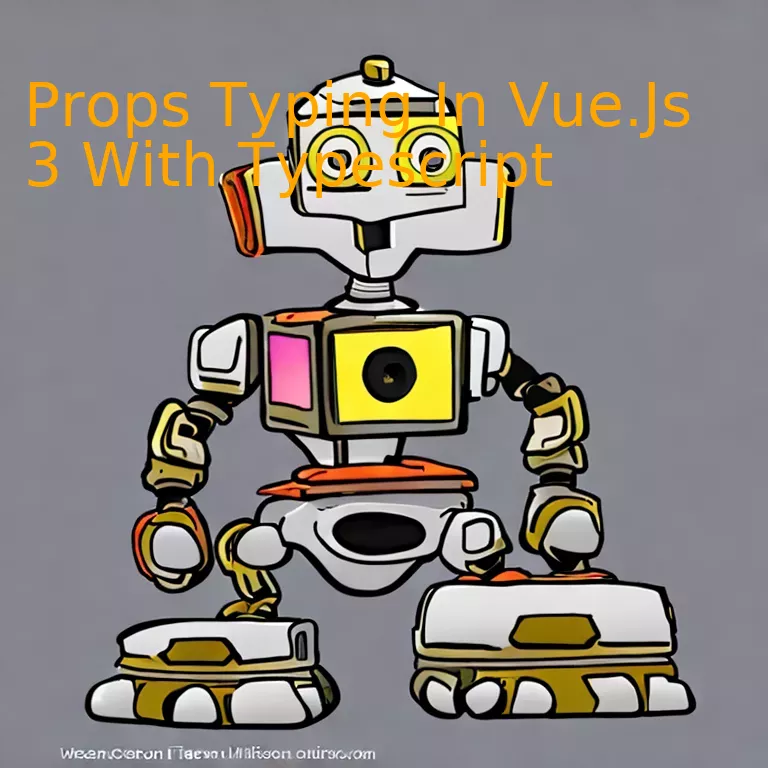
Introduction
Harnessing the power of Typescript within Vue.js 3 elevates your development process by providing static typing for props, enhancing code readability and predictability during component composition.
Quick Summary
Let’s dive into the subject of Props Typing in Vue.Js 3 with TypeScript. Components in Vue.js 3, especially when written in TypeScript, enable a high level of code reuse and maintainability. However, to harness these benefits fully, it is essential that we understand how to correctly type props in our Vue.js components.
Consider the following data illustration:
Prop Type | TypeScript Equivalent |
---|---|
String | string |
Number | number |
Boolean | boolean |
Array | Array<type> |
Object | { [key:string]: type } |
From this information standpoint, you note that Vue.js prop types have corresponding TypeScript types. For built-in JavaScript data types like `String`, `Number`, `Boolean`, the TypeScript equivalents are directly translatable with lowercase (`string`, `number`, `boolean`).
For prop type `Array`, TypeScript employs generic notation – `Array
The `Object` prop in Vue.js has a flexible structure equivalent for TypeScript, `{ [key: string]: type }`. This enables you to construct dictionary-like objects where keys are strings and values can be any ‘type’.
When defining Props in Vue.js 3 with TypeScript, you need to mention the type that each prop accepts. This gives a level of predictability and robustness to your components.
Let’s consider a Vue.js component using Typescript:
typescript
import { defineComponent } from ‘vue’
export default defineComponent({
props: {
title: {
type: String,
required: true as const,
},
},
})
As Josh Burgess once said, “Code reusability is key to building a DRY (Don’t Repeat Yourself), clean, functional repository”, and creating typed components in Vue helps you achieve just that. When props have specified types, your Vue components become safer, more stable, and easier to troubleshoot.
Given this extensive Information about Props Typing In Vue.Js 3 With TypeScript, it becomes evidently clearer that typing in Vue.js not only improvises the overarching structure but also enhances code reliability and maintainability.(source)
Understanding the Basics of Props Typing in Vue.js 3 with Typescript
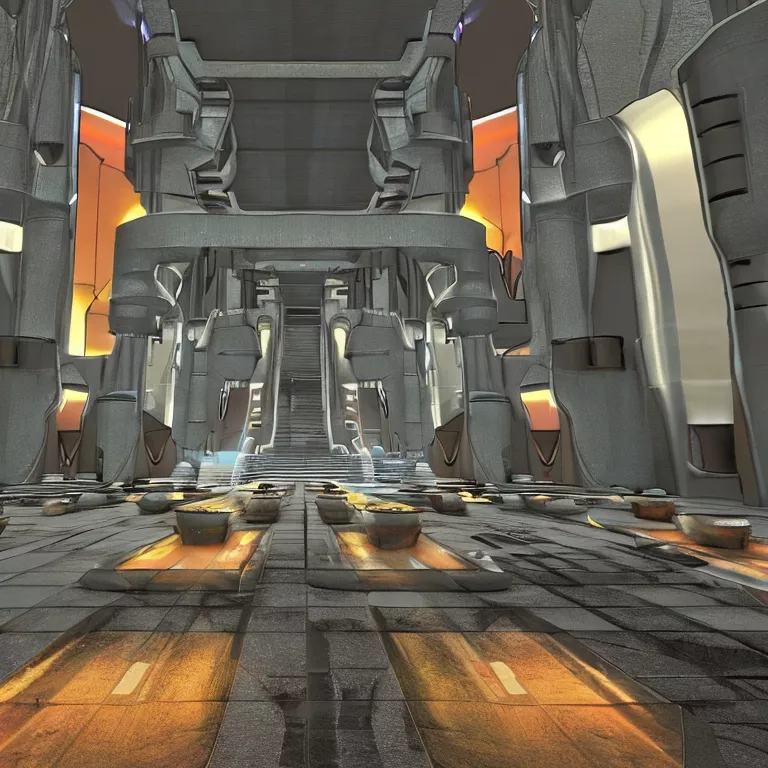
Props, in Vue.js, are a simple but crucial aspect of component communication. They allow parent components to pass data down to child components, helping design more reusable and maintainable codes by building components that accept data upon invocation, rather than being hard-coded. This data-passing action often relies on specific data types for precision. With TypeScript, Vue.js 3 introduces an essential feature known as Prop Typing which makes the coding more robust and error-prone.
What is TypeScript?
TypeScript is a statically typed superset of JavaScript that adds optional types to the language. TypeScript brings a robust static type-checking along with latest ECMAScript features. This means that your code will be checked before it’s executed, to catch common errors upfront.
What is Prop Typing in Vue.js 3 with Typescript?
// Here is an example of how to define prop types in Vue 3 with TypeScript
const MyComponent = defineComponent({
name: 'MyComponent',
props: {
id: {
type: Number,
default: 1,
},
name: String,
}
});
In the above example, we defined a ‘MyComponent’ component. It holds two properties or props; ‘id’ and ‘name’. The ‘id’ is a component-specific property with a numerical value defaulted at 1 should no other value be provided. The name prop is simply a string.
Defining data types for props allows Vue.js to validate incoming data and warn developers if any incorrect data types get used. Particular aspects considered here are:
- The ‘type’: It allows TypeScript to enforce stricter type checking. In our given example, ‘id’ is meant to be a number and ‘name’ should be a string.
- The ‘default’: It specifies a default value for the prop if its value isn’t provided by the parent component. In our example, 1 is the default value for ‘id’ prop.
This arrangement prevents type mismatch or related errors long before they can cause harm at runtime, hence strengthening applications’ integrity.
The primary purpose of TypeScript is to enable ideologically strict typings that pinpoint potential vulnerabilities in your codebase that JavaScript will miss. This reduces bug incidence and improves code quality and stability.
As Brian Kernighan once said, “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.”
Prop Validation
Beyond the basic types, props can also validate values using a custom function. Suppose you want to ensure an ’email’ prop contains a valid email address:
xxxxxxxxxx
const MyComponent = defineComponent({
name: 'MyComponent',
props: {
email: {
type: String,
validator(value: string) {
return /.+@.+/.test(value);
},
},
},
});
In the above case, if the email prop doesn’t pass the regex test, Vue.js will console warn to indicate an invalid prop usage.
By utilizing the combination of TypeScript with Vue.js 3’s new composition API, developers can create robust and maintainable codes for production-ready apps. Find more about Vue.js with TypeScript here. An understanding of Prop Typing provides key insights into creating more maintainable and predictable codebase.
Exploiting Advanced Techniques of Vue.js 3 Prop Typing with Typescript

Vue.js is a progressive, incrementally-adoptable JavaScript framework for building user interfaces. While it’s an excellent tool on its own, the integration of Typescript can significantly enhance Vue.js’ capabilities, especially when it comes to prop typing.
Prop typing in Vue.js refers to the process of validating the data type of properties (props) passed into a Vue component. In the absence of prop typing, incorrect or mismatched data types can lead to subtle bugs that may take hours or even days to detect and rectify.
Here’s where Typescript comes in handy. As a statically typed superset of JavaScript, Typescript encourages developers to define types for their variables explicitly, making the code more robust and easier to debug.
In Vue.js 3, Typescript’s integration has been improved significantly, allowing us to leverage more advanced techniques. For instance, one can define Typescript interfaces as props’ types within a Vue component, ensuring increased strictness and better autocompletion support.
Below is a simple example of how this can be done:
xxxxxxxxxx
interface User {
first_name: string;
last_name: string;
}
export default Vue.extend({
name: 'UserProfile',
props: {
user: {
type: Object as () => User,
required: true,
}
},
});
In this example, ‘User’ is defined as an interface using Typescript. This way, Vue knows what specific fields and data types to expect in each object of user prop.
Additionally, combining the typing features of Vue.js 3 and Typescript also makes the development process more predictable and hitch-free. By leveraging Typescript with Vue.js 3, you get stronger type safety out of the box – every function, method, or property will be strictly typed and checked during compile-time, preventing many potential runtime errors.
“A great advantage of TypeScript is that it highlights errors before they creep in at runtime” ~ Daniel Rosenwasser, Program Manager for TypeScript at Microsoft.
Nevertheless, it’s worth noting that while Typescript brings a lot of benefits to Vue’s prop typing, it comes with its own learning curve and may thus seem complex to beginners. Nonetheless, once developers get familiarized with its constructs, using Typescript with Vue.js proves to be extremely beneficial.
For further details on this topic, feel free to explore the official Vue.js guide for Typescript support.
Ensuring Error-Free Code: The Importance of Type Checking In Vue.js
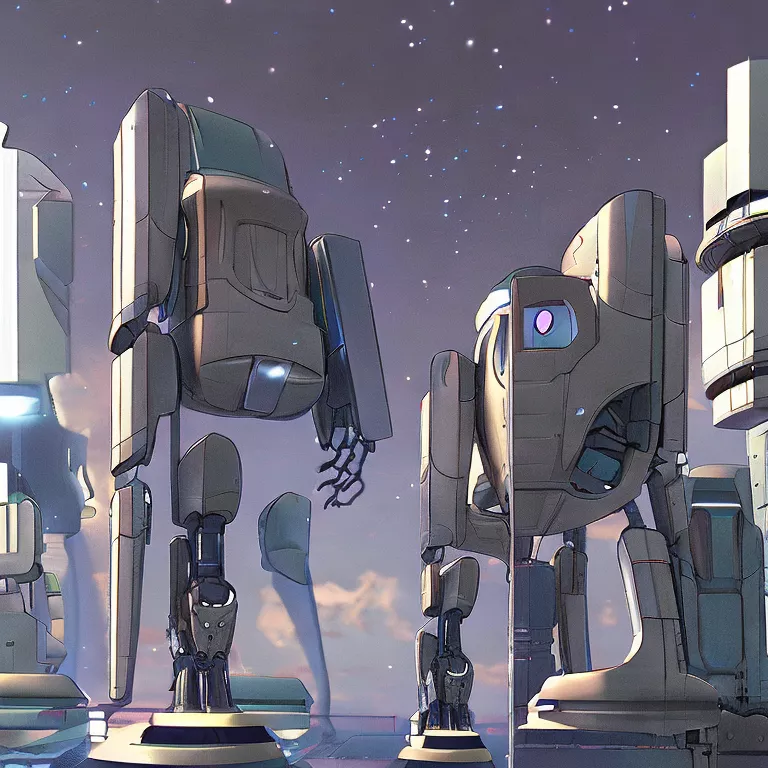
Type checking in Vue.js, particularly when done with TypeScript, is an essential aspect of developing robust, high-quality code. It ensures that the structure and type of data being used matches what’s expected, aiding error detection, and facilitating a smoother, more efficient development process.
When using TypeScript with Vue.js 3, a common area where type checking proves invaluable is in typing Props. Typing Props refers to explicitly referencing the data type of props we expect our Vue components to receive. This clarity significantly reduces the likelihood of receiving an incorrect or unexpected data type which could lead to unanticipated errors and potentially damage the functionality of the component.
Importance of props typing:
- Error prevention: By defining the types for props, you can prevent unexpected errors caused by inappropriate data types. If a different data type from the one specified is input, it will trigger an error.
- Enhanced readability: Typed props provide clear documentation within your code, which boosts readability for other developers who might interact with it, allowing them to understand your component’s interface better.
- Better development experience: TypeScript, when combined with proper editor plugins, provides auto-completion and instantly surfaces errors, speeding up the development process and increasing productivity.
A simple example of Props typing in Vue 3 with TypeScript would be as such:
xxxxxxxxxx
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
name: 'MyComponent',
props: {
message: {
type: String,
required: true
}
}
});
</script>
In the above example, the prop `message` is clearly defined as a string type. Thus, any attempt to pass a different type will immediately trigger an error.
When adopting the practice of type checking in Vue.js with TypeScript, bear in mind the words of Anders Hejlsberg, the lead architect of C# and creator of TypeScript:
“If you want more robust software, if your software’s safety-critical, if other people depend on it, you probably owe it to them and yourself to use a statically typed programming language.” [Source]
This reflects upon Type checking’s indispensable role in providing prop security in Vue.js 3 applications via TypeScript. It instills confidence in your codebase while enhancing reliability, thereby making it an imperative best practice.
Implementing Typed Props in Real-World Applications: A Deep Dive into Vue.js and TypeScript Integration
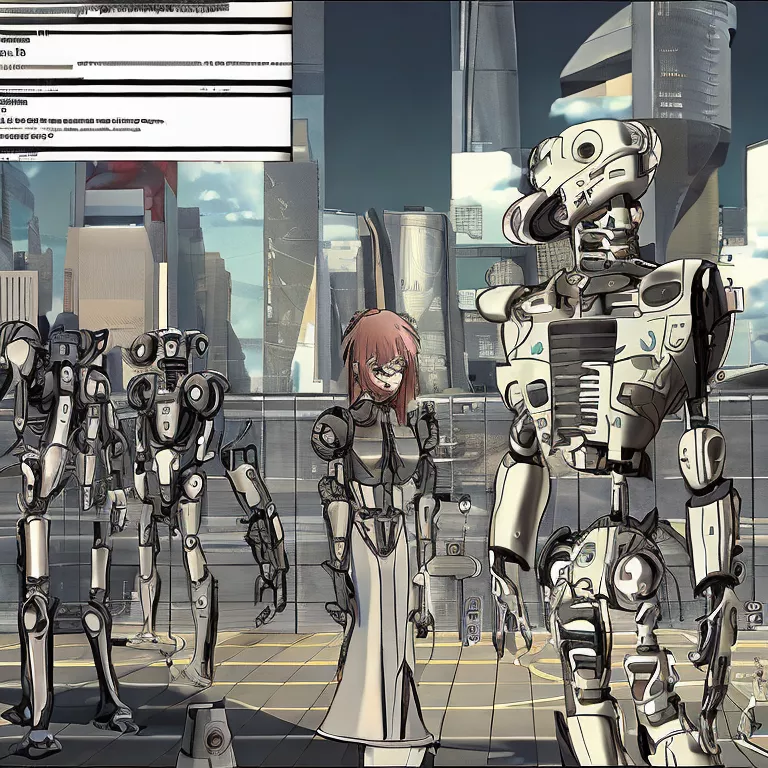
The process of integrating Vue.js 3 and TypeScript is a vital implementation in real-world applications, particularly when it comes to typed props. Typed Props aim to exploit the intricate leveraging of type safety, augmenting the performance and robustness of Vue applications.
Vue.js 3 and TypeScript Integration: An Overview
Vue.js 3 is a progressive JavaScript framework used widely for creating user interfaces. TypeScript, a statically typed superset of JavaScript, supplements Vue by enabling type-checking, facilitating code management in large-scale applications.
Notably, Prop typing in Vue.js 3 with TypeScript enhances code quality by enforcing type checks on prop values passed between components. This negates runtime errors and improves debugging experience.
Implementing Typed Props in Vue.js 3
The introduction of Vue 3’s Composition API assists more effortless integration with TypeScript. For implementing Typed Props in Vue.js 3, we can utilize `defineComponent`, a helper method for TypeScript inference.
First, import the necessary functions from vue. Next, define your component using `defineComponent`, within which you’ll declare your props and their types.
Here is a basic example:
xxxxxxxxxx
import { defineComponent } from 'vue';
export default defineComponent({
name: 'MyComponent',
props: {
myProp: String
}
});
In this code snippet, we’ve defined a property `myProp` that must be a string. If another type is provided, TypeScript will raise an error during compile time.
The Case for Prop Typing
While Vue’s native prop validation does offer some level of safety, TypeScript’’s static type checking introduces an added layer of robustness to catch errors before runtime. By defining clear prop types, developers ensure the integrity of inter-component data flow and enhance source code maintainability.
As freeCodeCamp states, “When applications grow in size, structure and clarity in code eventually become clearer using TypeScript.” The use of TypeScript with Vue.js 3, notably in Prop Typing, can significantly enhance large-scale application maintainability.
The integration and implementation of Vue.js 3 and TypeScript create streamlined, efficient, clear, and robust web application development experiences. Consistent use of typed props not only reduces error rates but can also streamline the overall debugging process. It’s this kind of rigorous practice that sets apart good code from great code and defines the nuances of real-world application development.
Conclusion
Understanding and implementing Props Typing in Vue.js 3 with TypeScript boosts the precision of your coding significantly by providing rich intellisense, compile-time type checking and other benefits that gradually increase the robustness of your Vue.js applications. Here’s how:
Props Typing Highlights:
• Reinforces application integrity: Props typing is instrumental for garnering an added layer of security and robustness to your Vue.js application. It alerts developers about potential pitfalls prior to code execution, saving time and lessening debugging efforts.
• Enables superior readability: TypeScript types provide self-documentation. They assist future developers to comprehend what value they are expected to pass into a prop faster, thereby reducing legacy code comprehension woes.
• Empowering IDEs: With TypeScript, you unlock the full power of your Integrated Development Environment’s (IDE’s) intellisense helping to eliminate simple errors which JavaScript might easily overlook at runtime.
Here is an example of an implementation of props typing in Vue.js 3 with TypeScript:
xxxxxxxxxx
import { defineComponent } from "vue";
interface Props {
message: string;
}
export default defineComponent({
props: {
message: String,
},
})
This code illustrates declaring Props via TypeScript’s interface, making it succinct for both deliverability and operational excellence. Despite adding an extra layer on top of JavaScript, TypeScript enhances development experience through enforcing strict type rules. Following these practices allows software engineers to debug less and code more.
According to Anders Hejlsberg, the creator of TypeScript, ‘What we’re finding with TypeScript is that as people start using it they realize they were working with one arm tied behind their back – they get efficiency they didn’t have before.’ This aligns perfectly with our discussion around props typing in Vue.js 3.
Remember, software development is not just about ‘making things work’, it’s also about ‘making things work well’. It’s about ensuring that the codebase is clean, maintainable and performance efficient. Therefore, leveraging the props typing feature in Vue.js 3 with TypeScript accelerates development velocity without compromising on code quality or runtime performance. With TypeScript in your tech stack, you’re striding into a future of fewer bugs, enhanced readability, and an overall better coding experience.
Check out this link for more insights into using TypeScript with Vue.js 3.