Introduction
When using React Typescript, you may encounter an issue where a ‘Type Void Is Not Assignable To Type ((Event: Changeevent
Quick Summary
The error message “Type ‘void’ is not assignable to type ‘(event: ChangeEvent
Problem | Solution |
---|---|
Type ‘void’ being assigned to ‘(event: ChangeEvent<HTMLInputElement>) => void’ | Correct the function signature to match the expected type. |
React component not expecting a return value | Modify the callback function to not return any value. |
This discrepancy occurs when you attempt to assign a function that doesn’t provide a return type to an instance where a specific type of function is expected, particularly one with a void return type. Essentially, this indicates that the expected usage of the function does not align with its implementation.
React form elements often require a specific type of callback function for event handling. The ChangeEvent in particular expects a function with the format `(event: ChangeEvent
To resolve this issue, ensure that the function assigned matches the expected signature. For example, if you’ve got an input event handler, it should be similar to this:
html
this.handleInputChange(event)} />
Then, in the `handleInputChange` method, you should not return any value:
javascript
handleInputChange(event: React.ChangeEvent
// Your logic here. No return statement.
}
In the words of Robert C. Martin, a well-known software engineer and author: “Clean code is not written by following a set of rules. You don’t become a software craftsman by learning a list of heuristics. Professionalism and craftsmanship come from values that drive disciplines.” Therefore, understanding and applying TypeScript’s type checking to your React components will make them more robust and maintainable.
Understanding the Error: ‘Type Void Not Assignable to Type ((Event: Changeevent) => Void)’
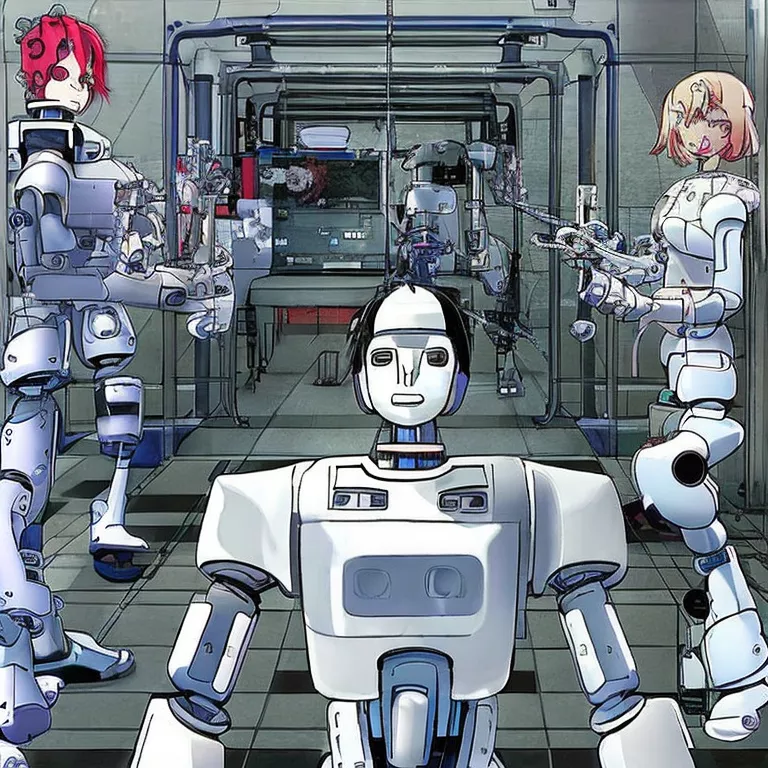
In TypeScript with React, when developing an application, it’s not uncommon to encounter the error: ‘Type void is not assignable to type ((event: ChangeEvent
This error typically surfaces whenever there’s an incompatibility between the types we’ve defined against what Typescript expects. The issue often occurs due to our handler function not matching the expected type that the event prop is supposed to get.
Before we delve deeper into this issue, let’s understand the base concepts:
* The
((event: ChangeEvent) => void)
imitates an arrow function, expecting an event object of type
xxxxxxxxxx
ChangeEvent
which is targeting an
xxxxxxxxxx
HTMLInputElement
, and returns nothing. (Hence the
xxxxxxxxxx
void
type)
* The keyword
xxxxxxxxxx
void
in Typescript signifies ‘lack of a type’. When used as a function return type, it implies that the function doesn’t return anything.
The problem occurs when you try to assign a function that truly doesn’t ‘return’ anything – in other words, its return type is really
xxxxxxxxxx
void
– to a prop or a variable that requires a function that ‘returns’ something, even if the ‘something’ is defined as
xxxxxxxxxx
void
. Even though logically they may seem the same, in strict TypeScript terminology, they are not.
Consider this illustration:
xxxxxxxxxx
type MyChangeHandler = (event: ChangeEvent) => void;
const incorrect: MyChangeHandler = () => {
console.log('This would fail TypeScript checks.');
};
While our
xxxxxxxxxx
incorrect
function does not really return anything, it is still not acceptable because
xxxxxxxxxx
MyChangeHandler
expects a function that accepts an argument but here we don’t have any.
You would rather want to do something like this:
xxxxxxxxxx
const correct: MyChangeHandler = (event) => {
console.log(event.target.value);
};
In the
xxxxxxxxxx
correct
example, our function is now a valid
xxxxxxxxxx
MyChangeHandler
, because it accepts an argument (of the appropriate type).
In conclusion, to avoid this error always ensure:
* You’re not trying to assign a type
xxxxxxxxxx
void
function to a type
that requires some form of return, even if it’s a
xxxxxxxxxx
void
.
* The function should have an event parameter that all event handlers get. If left out—TypeScript complains.
Jeff Atwood, famously highlighted – “Coding is not about typing…it’s about thinking”. That holds true when we are dealing issues like these while using TypeScript with React. We need to think in terms of TypeScript, its specifics and its quirks.
Dealing with React Typescript Event Handling Issues
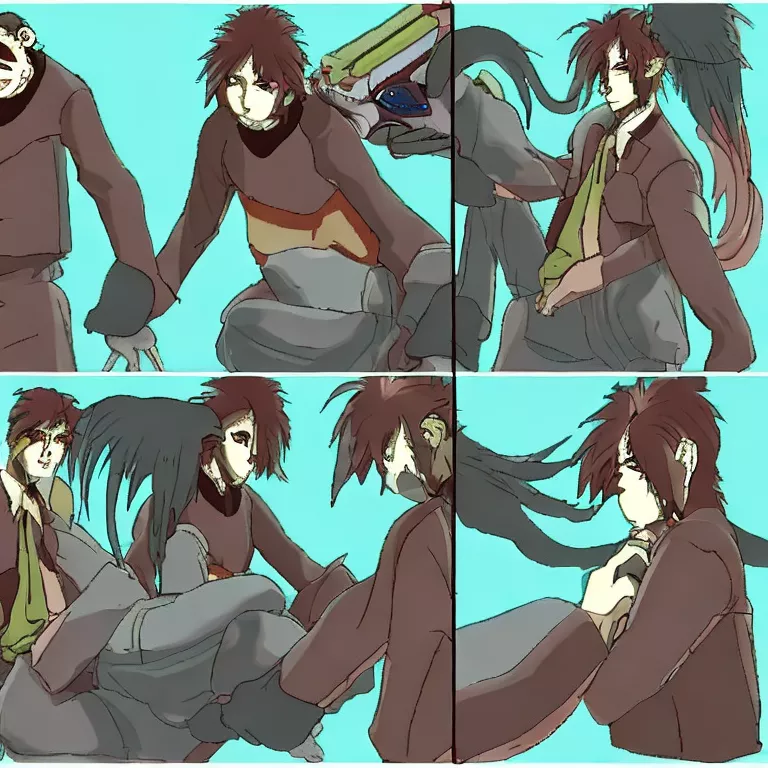
The common issue encountered by TypeScript developers while working with React, specifically when handling events is `Type ‘void’ is not assignable to type ‘((event: ChangeEvent
This error usually comes up when the event handler function is written in a way that does not match with the type of input expected. The root of the problem here lies with TypeScript’s strict type safety rules which necessitate adhering to its syntax and format rigidly.
Understanding the Issue
To start off, we need to understand what each part of this error message means:
– `Type ‘void’`: This is the type that your event handling function is currently returning.
– `not assignable to type ‘`((event: ChangeEvent
Essentially, the error indicates mismatch between expected and received output.
Solution
It is important to make sure our handled event returns the correct type to get rid of the error. Below is how it can be corrected:
Let’s consider an example function that could trigger such an error in TypeScript:
xxxxxxxxxx
function handleChange(event: React.ChangeEvent) {
console.log(event.target.value);
}
The above code snippet doesn’t return anything (void), hence giving the error. A valid way to declare an event-handling function in TypeScript that matches the type `((event: ChangeEvent
xxxxxxxxxx
const handleChange = (event: React.ChangeEvent): void => {
console.log(event.target.value);
};
By ensuring the function returns a void type explicitly, we are aligning it with the type expected from the event handler in React with TypeScript.
As seasoned JavaScript developer Douglas Crockford once said, “JavaScript is the world’s most misunderstood programming language”, and this can be even more true when we add TypeScript into the mix. Adherence to type discipline can solve a vast majority of the issues that we encounter in TypeScript.
In general, developers transitioning from JavaScript to TypeScript, will take some time to get used to the stricter typing rules of TypeScript, especially when it comes to specific React functionalities such as event handling. But with a bit of patience and perseverance, it soon becomes second nature.
Though at times tedious, this strict type checking is what makes TypeScript so powerful and worthwhile. It ensures better code quality, predictive debugging, and eases future maintenance – leading to robust and efficient apps.
As we explore further into the depth of TypeScript with React, remember “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” This quote by Martin Fowler encourages us to strive for clarity and readability while coding.
Assessing and Correcting Void Inconsistencies in TypeScript
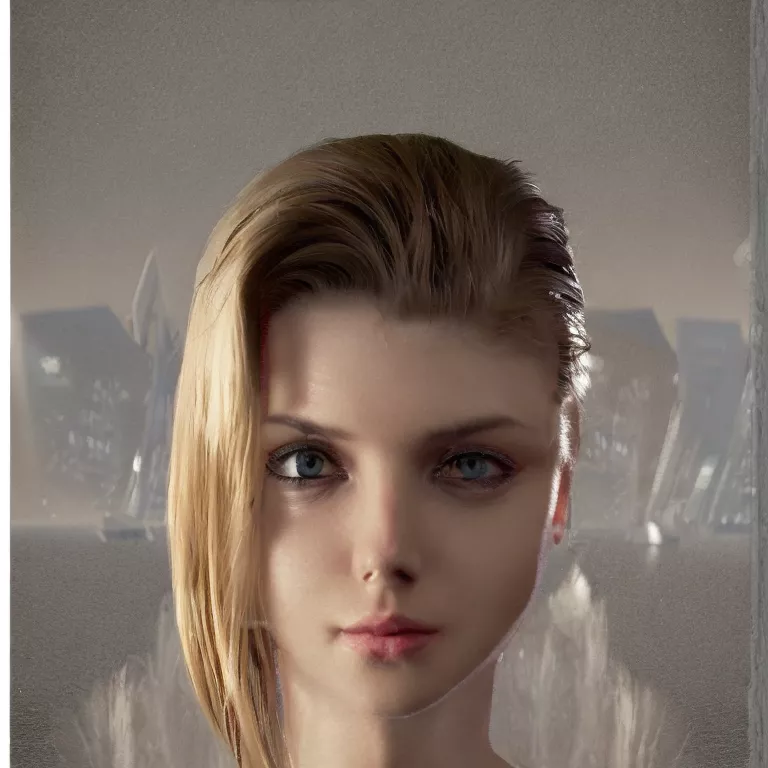
TypeScript, a popular open-source programming language developed by Microsoft, is well-regarded for its robust type-checking system that promotes code reliability and predictability. One common inconsistency or issue developers sometimes encounter involves the utilization of the `void` type. This is notably perfected when integrating TypeScript code within the React framework.
The particular error “type `void` is not assignable to type ‘((event: ChangeEvent
1. Function Return Type Inconsistencies
When specifying a function’s return type as `void`, it means the function doesn’t return a value. The error could arise if a function ought to return a `void`, but was implicitly or explicitly declared to return a different type, or vice versa. Double-check the context and correct any mismatches.
2. Prop Misalignment
In a React component, this error often appears if you’ve defined an event callback prop that’s expected to be a function of a certain type, but what’s actually passed in doesn’t align with this expectation. To resolve, ensure you align function parameters and returns accurately across your props.
3. Asynchronous Handlers
Another common cause is related to asynchronous event handlers. Since JavaScript inherently treats async functions differently, the resulting Promise can conflict with TypeScript’s strict typing and the expected `void` type. Reevaluate where you’re utilizing async callbacks and consider restructuring your function definition to better accommodate asynchronous behavior.
Consider the following two bad and good corrective examples, respectively:
Bad example:
xxxxxxxxxx
async function handleChange(event: React.ChangeEvent): void {
// Async logic here
}
Good example:
xxxxxxxxxx
function handleChange(event: React.ChangeEvent): void {
// Wrap async logic here
AsyncFunction().catch(err => console.error(err));
}
These adjustments make TypeScript checks compatible with JavaScript behavior, avoiding the “Type `void` is not assignable” error and ultimately cultivating a more consistent, reliable codebase. Minimizing type inconsistencies helps to maintain a healthy codebase by ensuring that code behavior remains predictable and manageable as your project evolves.
As Robert C. Martin, a revered figure in the world of software craftsmanship, famously said: “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code…[Therefore,] making it easy to read makes it easier to write.”
Web Links for Reference:
TypeScript official site
React official site
Mitigating Event Issues in React TypeScript
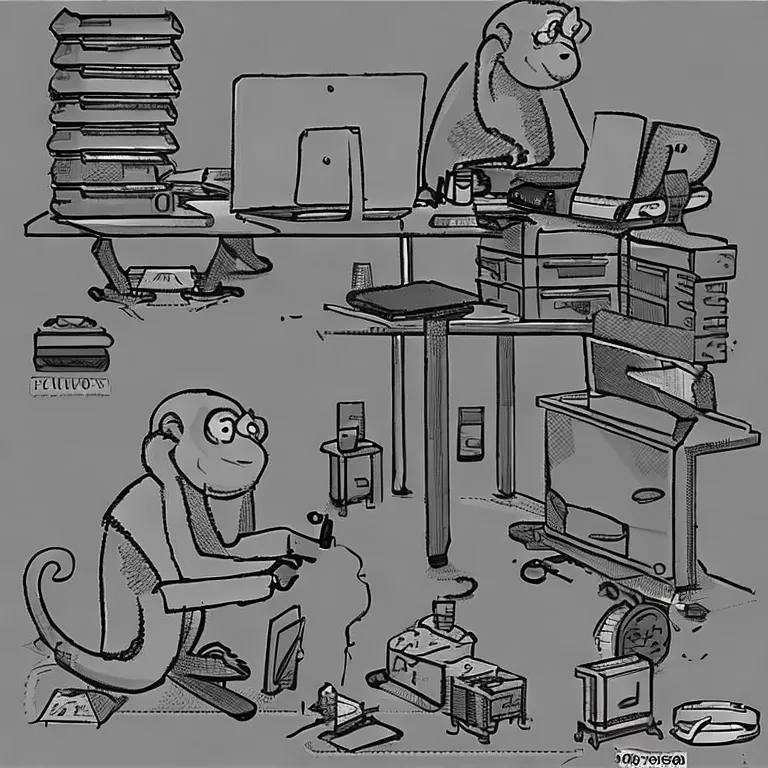
The issue at hand involved the particular error message: “Type void is not assignable to type ((event: ChangeEvent
Let’s interpolate this error message with a typical example:
javascript
class MyComponent extends React.Component {
private handleChange(event: ChangeEvent
// Handle change
}
render() {
return ;
}
}
In the code above, you may encounter the error saying type void is not assignable to event handler because React expects a function that returns any value other than undefined or null, but the void return type of our handleChange method doesn’t meet the requisite conditions.
The most logical solution to tackle this problem would be to ensure that the expected return value matches the actual return type. We can also encase the React event handler with the shorter and more standard way of declaring methods inside a class component, like so:
xxxxxxxxxx
this.handleClick(e)}
Click me
But how does this issue relate to TypeScript? It blends seamlessly with TypeScript’s static type-checking feature which spot errors at compile-time, therefore ensuring consistency across your JavaScript data, functions, objects, etc.
However, there might be future cases when the return type for a similar situation will not ideally be of ‘void’ type but instead returning some values. Hence, while using React and TypeScript together, it’s crucial to understand their functioning and constraints clearly to avoid such errors while dealing with events in your components. The use of appropriate handler types thus ensures that we are always working within an agreed framework.
As Donald Knuth, a renowned computer scientist, emphasized: “The real problem is that programmers have spent far too much time worrying about efficiency in the wrong places and at the wrong times; premature optimization is the root of all evil (or at least most of it) in programming.” Therefore, when using TypeScript with React, you can demarcate boundaries between what is essential and what may result in premature optimization or over-engineering.
TypeScript Handbook Advanced Types provides more insight on advanced types which would aid in deeper understanding of managing such issues.
Conclusion
TypeScript Void vs Event: ChangeEvent<HTMLInputElement>
TypeScript packs a powerful punch in JavaScript development mainly because of its static-type checking feature. At the heart of this feature are TypeScript types, among which ‘void’ and ‘ChangeEvent<HTMLInputElement>’ deserve a special mention as they’re frequently encountered in React.
Void:
The keyword
xxxxxxxxxx
void
in TypeScript is used where there’s no data. You’ve probably seen it stand alongside functions that don’t return anything. Here’s a simple illustration:
xxxxxxxxxx
function logMessage(): void {
console.log('This is a log message');
}
ChangeEvent<HTMLInputElement>:
Contrasting to ‘void’, the ‘ChangeEvent’ provides an interface for react events, a typical use-case being html input changes. Simply put, a function with ‘ChangeEvent<HTMLInputElement>’ type can look something like this:
xxxxxxxxxx
function handleInputChange(event: React.ChangeEvent<HTMLInputElement>): void {
console.log(event.target.value);
}
When you encounter a discrepancy such as ‘Type void is not assignable to type ((event: ChangeEvent<HTMLInputElement>) => void)’, it usually points to a case where the function’s expected return type doesn’t match the actual type.
Instead of returning nothing (void), the function is expected to return a function signature that takes an event of type ‘ChangeEvent<HTMLInputElement>’ and, interestingly, returns ‘void’. So, in essence, it’s not exactly about ‘void’ not being assignable to ‘ChangeEvent<HTMLInputElement>’; it’s more about the function return type mismatch.
Updating your function to fit this signature should resolve the issue:
xxxxxxxxxx
const myFunc = (): ((event: React.ChangeEvent<HTMLInputElement>) => void) =>
(event) => console.log(event.target.value);
Playing around with TypeScript types is integral to building sturdy, bug-free applications. As Steve Jobs rightly said, “Details matter, it’s worth waiting to get it right”. Understanding the nuances of ‘void’ and ‘ChangeEvent<HTMLInputElement>’, can make a significant difference in your React-Typescript project quality and performance.