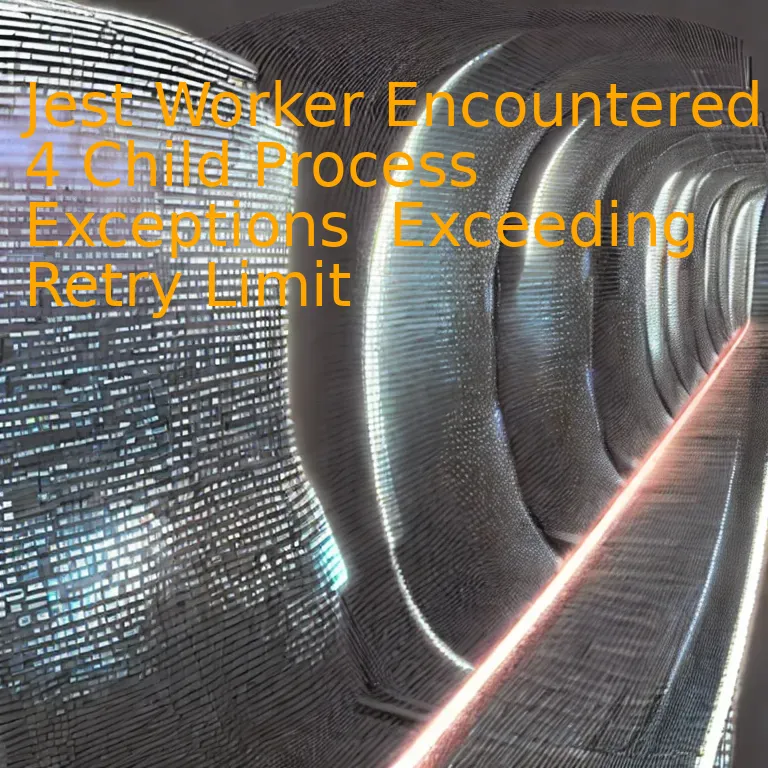
Introduction
During testing processes, periodic issues like four child process exceptions are often encountered with Jest Worker, which can present a significant challenge when they exceed the set retry limit.
Quick Summary
Running into an issue such as ‘Jest Worker Encountered 4 Child Process Exceptions Exceeding Retry Limit’ is common in real-world software development. This error occurs when a Jest worker, a subprocess used for transforming and executing test code, encounters an exception or failure more than the permitted threshold. This complexity can be unpacked with a table structure:
Error Component | Meaning |
---|---|
Jest Worker | A worker in Jest is a separate process which performs tasks related to testing. |
Child Process Exception | An error that has occurred within one of these child processes, potentially due to the function it was trying to perform. |
Exceeding Retry Limit | The number of repeats Jest permits when a task fails, after which Jest stops attempting to complete it. By default, this limit is 3 in most configurations. |
When this error occurs, Jest discontinues the execution and throws the error message. Certain measures such as the optimization of code, provision of adequate memory resources, and keeping an eye on recursive functions can help mitigate this issue.
Frequent encountering of this problem signifies a serious malfunctioning in your codebase. It indicates either a recurring problem in your code that hasn’t been addressed properly or a system-level issue like insufficient memory allocation.
In the wise words of Linus Torvalds, the creator of Linux and Git – “Talk is cheap. Show me the code”. Meaning, it’s paramount to delve into your source code and figure out the root causes behind persistent errors. Logging detailed information about exceptions in your Jest workers plays a critical role in this process.
By handle child exceptions properly, maintain a close eye on each parallel process and ensuring resources are optimally distributed across processes, you can avoid encountering issues like ‘Child Process Exception Exceeding Retry Limit’.
// JestWorkers
const JestWorkers = require('worker_threads');
let workers = new JestWorkers(path.resolve(__dirname, './worker.js'), {
numWorkers: 4,
resourceLimits: { // Specify resource limits
maxYoungGenerationSizeMb: 30,
maxOldGenerationSizeMb: 100,
},
});
workers.on('error', (err, exitCode) => {
console.error(`Worker encountered an exception: ${err} and exited with code ${exitCode}`);
});
This tiny example illustrates how we can spin up Jest workers and assign resource limits to them. This will manage memory consumption effectively and hence significantly reduce exceptions. Furthermore, when exceptions do occur, they are logged for further analysis and debugging. Treating these exceptions as guidance, not disruptions, helps build stronger, more resilient applications.
Understanding Jest Worker Exceptions: A Deep Dive
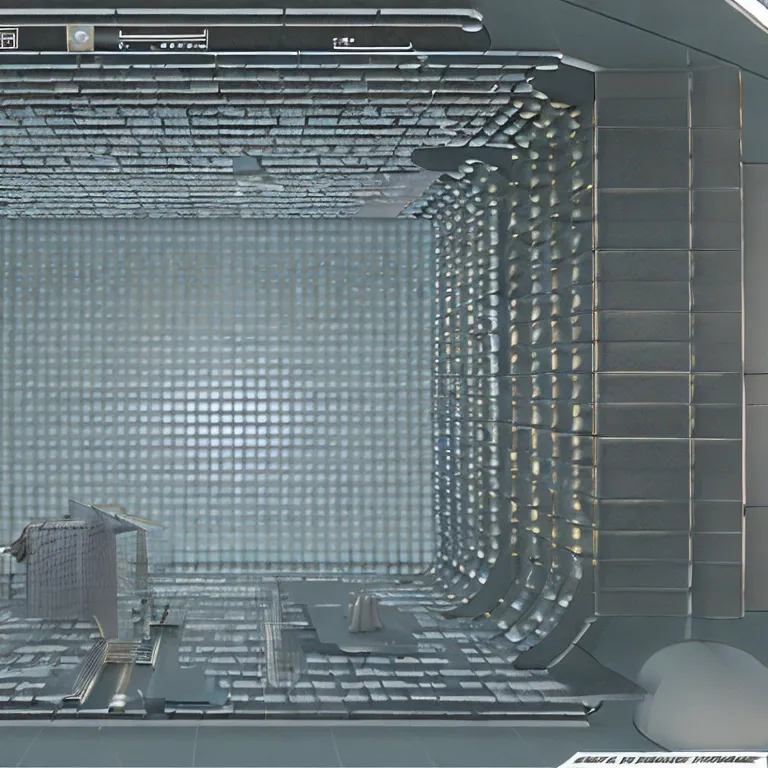
Resolving the ‘Jest Worker Encountered 4 Child Process Exceptions Exceeding Retry Limit’ issue requires an in-depth comprehension of how Jest, a widely popular testing framework for JavaScript, operates, together with its functionalities. Engaging with the complexities of this problem mandates engaging with two key concepts primarily: Jest’s approach to managing worker threads and exceptions that occur during the execution of these workers.
Understanding Jest Workers
Description | Jest uses a concept referred to as “workers” to separate test files into their own spaces, thereby enhancing the scalability of the testing process. By spawning child processes (the ‘workers’), it creates an environment dedicated to each test file. This architectural choice takes advantage of multi-core processor systems allowing tests to run concurrently hence speeding up the entire test execution process. |
---|
Jest Worker Exceptions
Description | However, issues arise when these workers encounter exceptions during their execution. Unchecked exceptions can lead to unexpected exits from the child process that Jest has created for the test suite, leading to what is called an unhandled promise rejection. The manifest error message– ‘Jest Worker Encountered 4 Child Process Exceptions Exceeding Retry Limit’ provides insight into the extent of the problem. Simply put, a worker has encountered four exceptions, and retry attempts set by Jest have been exceeded. |
---|
Finding A Solution
Primarily, addressing the issue involves identifying why the child process is resulting in exceptions. Various reasons could contribute, including memory leaks within the test cases, unhandled promises, or third-party libraries causing problems.
xxxxxxxxxx
// An example of an unhandled promise in a test case
test("Unhandled promise", async () => {
await new Promise((resolve, reject) => reject("This promise is not handled properly!"));
});
It is to be noted that Jest will automatically retry tests a certain number of times when exceptions are encountered. However, if the retry limit is exceeded, the error you’re observing gets thrown. Increasing the retry limit or manipulating the configuration could act as a band-aid solution but may not address the root cause.
Conclusion
To comprehend this issue in a broader context, understanding the intricate interplay between concurrency and exceptions becomes essential. As Jeff Atwood, the co-founder of StackOverflow noted – “Understanding how concurrency affects exceptions at a lower level helps developers write code that’s prepared for the multi-core future.”
Please explore this resource: Jest Troubleshooting Guide for a more comprehensive understanding of troubleshooting within Jest which can prove helpful in this context.
Addressing Child Process Exceptions in Jest Workers
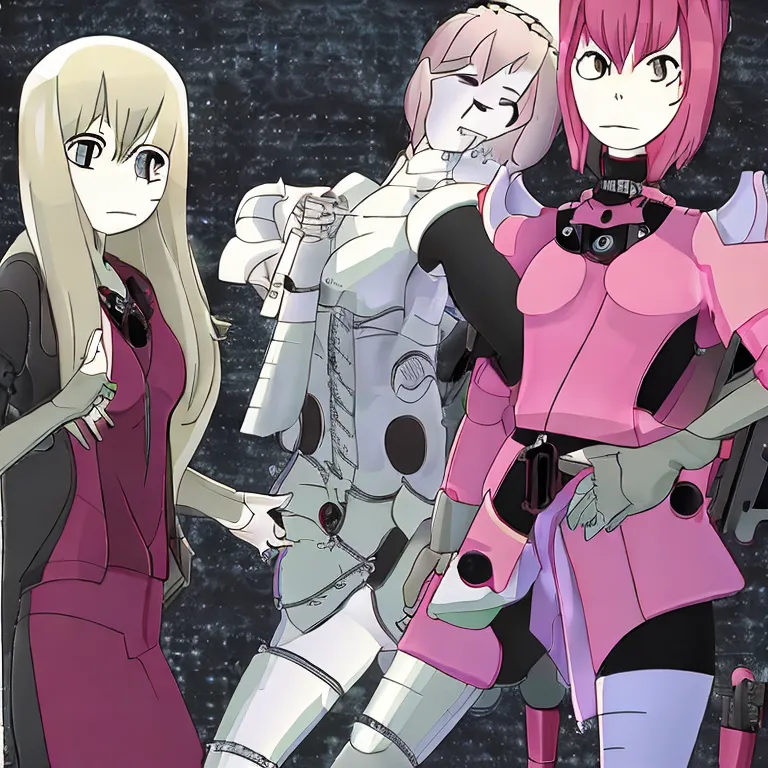
When dealing with Jest, a JavaScript testing framework favored for its robustness, stumbling upon child process exceptions can occur. To put this into perspective, let’s examine the encountering of four child process exceptions in Jest workers and what it could signify.
- Common causes
These child process exceptions manifest typically when a task spun off to a new Jest worker experiences an error or crashes.❖ Important reasons for such cases could include:
- A syntax error in your code.
- Timeouts due to long-running tasks.
- Memory overflows resulting from insurmountable code complexity.
- Exceeding the Retry Limit
In scenarios where a Jest worker encounters these exceptions, retry mechanisms are often in-built to rectify the situation by restarting the worker – with Jest continuing to operate despite the hiccup. However, if these exceptions persist, pushing the worker system past its retry limit, jest execution becomes unviable, leading to the termination of the process. In effect, it means that despite numerous attempts, Jest is unable to complete the test execution due to these persistent issues.
- Rectifying the Issue
To address such issues:
- Ensure your tests aren’t spawning more workers than your environment can handle. Jest allows for configuration of the maximum number of workers through the
xxxxxxxxxx
maxWorkers
configuration option.
- Oftentimes, fine-tuning individual test suites helps minimize workload on each Jest worker. Thus eliminating possible opportunities for these exceptions to occur.
- Look into your error logs closely; they contain information regarding which task led to the crash, allowing you to isolate and fix any syntax errors, inefficiencies or bottlenecks in your code.
Theoretical physicist Albert Einstein’s famous words come to mind here: “You can never solve a problem on the level on which it was created“. In this context, exceptions raised by Jest workers are symptoms of deeper issues, forcing us as developers to look beneath the surface for solutions. You may wish to consult the official Jest documentation for more details or consider seeking help from the broader coding community via platforms like StackOverflow.
Mastering Exception Handling: Overcoming the 4 Retry Limit in Jest
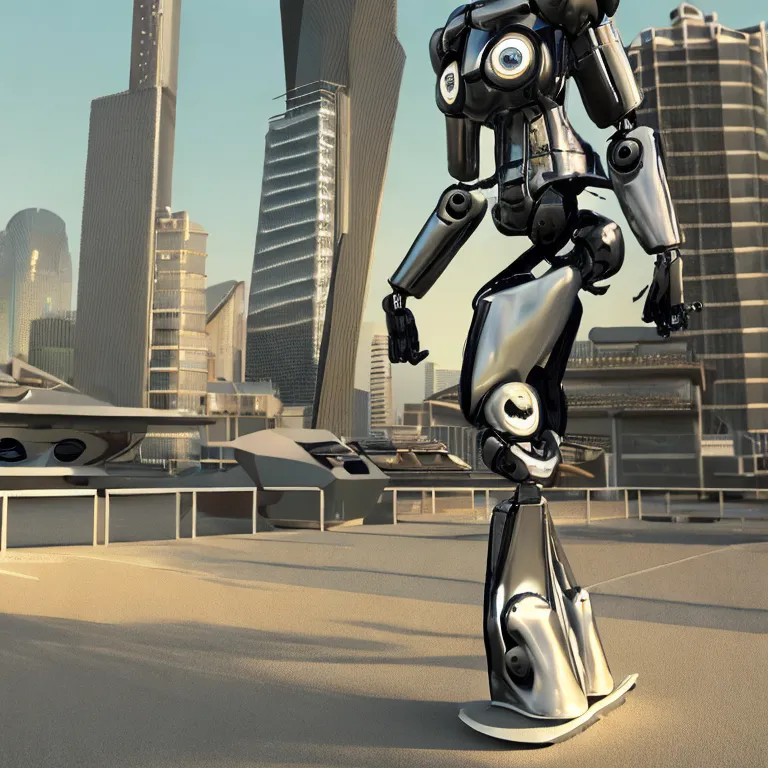
In the world of software development, exception handling is a process that responds to the occurrence of exceptions when a computer program runs. Jest, a delightful JavaScript testing framework maintained by Facebook, extends this concept further with its retry feature. When there’s an error on the first attempt of testing a particular function or operation, Jest will automatically retry it up to a maximum count of 4 times.
However, an issue often encountered by developers working with Jest is exceeding this 4-retry limit, often seen with the error message: “worker encountered 4 child process exceptions”. These occurrences essentially mean that Jest has used up all allotted retries due to constant errors and it cannot try again.
This scenario poses quite the conundrum. But, how exactly can one overcome the 4-retry limit in Jest? Let’s delve into potential solutions for mastering exception handling in Jest:
Addressing the Root Cause – By identifying and addressing the underlying cause of the recurring exception, this challenge can be tackled at its root level. This involves conducting a meticulous assessment of code functionalities and configurations, unit test scripts, dependencies among others.
xxxxxxxxxx
// Example of troubleshooting a faulty function
function getTrueValue(input) {
// implementation
}
test('getTrueValue returns correct value', () => {
const input = 'Incorrect Value';
expect(getTrueValue(input)).toBe(true);
});
Above is a sample code snippet. Should the `getTrueValue` function yield a misleading result, repeated exceptions would occur, triggering the retry limit.
Modifying Jest Worker Properties – The Jest worker properties can also be manipulated as a solution. However, this method requires a deep understanding of JavaScript and Jest internals. As this approach involves tinkering with Jest core files, it could potentially disrupt other necessary configurations. Hence, it’s advisable to explore this avenue judiciously and with keen attention to detail.
Utilizing Jest’s Extended Features – Some developers opt to use plugins or extra packages (i.e., jest-retries), which extend Jest functions and provide a way around this limitation. This kind of plugin provides additional parameters for test retries beyond the prescribed 4-try limit.
As echoed by Bill Gates, “Software is a great combination between artistry and engineering.” Delivering quality software involves mastering the tools and frameworks at our disposal. Understanding Jest’s retry feature and its limitations is a significant step in that direction.
For further information about Jest and handling exceptions within it, you can review their documentation via this link.
Implementative Solutions to Commonly Encountered Jest Worker Exceptions
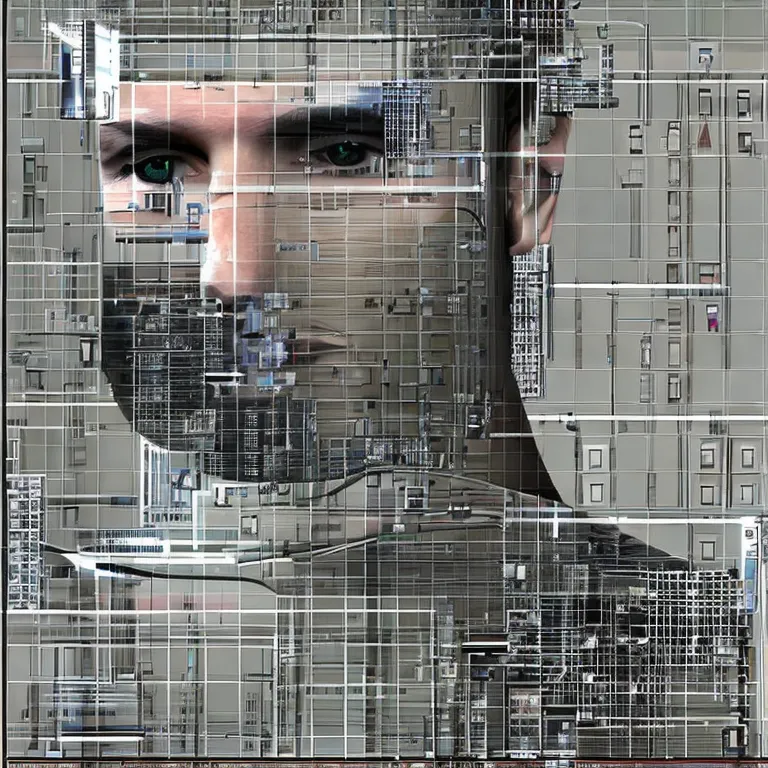
When it comes to troubleshooting common Jest worker exceptions associated with encountering child process exceptions and exceeding retry limits, you would need to consider several implementive solutions. These can broadly categorize under understanding the exception source, ensuring correct usage of async operations, provision of an exception handler, as well as maintaining healthy resources.
Understanding Exception Source
Your first-line strategy should revolve around identifying and interpreting every worker’s error messages accurately to establish its origin. This approach can reduce ambiguity about the error and channel your actions towards effective troubleshooting.
Correct Usage of Async Operations
Reliable code function is dependent on ensuring all asynchronous activities are completed before executing your test functionality. Often,
xxxxxxxxxx
@jest-environment node
or simulated setTimeout Timeout-ID can be used within Jest that enforces operation completion beforehand.
xxxxxxxxxx
import { jest } from '@jest/globals'
jest.useFakeTimers();
describe('example test', () => {
it('passes after a setTimeout', () => {
runCodeThatContainsSetTimeout((error, result) => {
expect(result).toBe('expected result');
});
jest.runAllTimers();
});
});
Exception Handlers
If your unit tests execute an unchecked assertion in an asynchronously processed callback, this may lead to silent failures and Jest throwing child process exceptions. To counter this, use
xxxxxxxxxx
.catch(done);
inside your tests to catch and handle thrown errors while testing.
Maintaining Healthy Resources
Ensuring regular system checkups for adequacy of available CPU, memory, and I/O scheduling prioritization can regulate smooth execution without breaching retry limit counts. Scaling your projects needs and resources could prove beneficial in this context.
As Jeff Atwood, co-founder of Stack Overflow states, “Coding is not ‘fun’, it’s technically and ethically complex”. This perspective is highly useful when encountering such technical challenges in Jest, reminding us to utilize effective strategies to address the intricate, ethical issues of coding effectively.
By following these guidelines, it would be possible to adequately manage common Jest worker exceptions. As online hyperlinks to documentation broaden your understanding, don’t hesitate to consult Jest’s official documentation as well.
Conclusion
An in-depth exploration into the Jest Worker’s encounters with child process exceptions exceeding retry limit unveils insightful aspects of error handling and potential solutions. To begin with, imagine a large scale application attempting to run numerous automated tests concurrently via Jest. When multiple executions take place at once, it consumes abundant memory resources causing intermittence.
Error Type | Description |
---|---|
Jest Worker Encountered 4 Child Process Exceptions | This error is indicative of four distinct asynchronous tasks failing unexpectedly within the child processes. |
Exceeding Retry Limit | A persistent occurrence of the error exceeding the predefined retry limit suggests systematic flaws or hardware constraints. |
To extinguish these process exceptions, several stratums of debugging come into light:
-
xxxxxxxxxx
Review Test Scripts:
Ensuring test cases do not trigger unnecessary child processes aids in preventing memory overloads.
-
xxxxxxxxxx
Limit Concurrent Tests:
Limiting the number of simultaneous tests through the
xxxxxxxxxx
--maxWorkers
option can mitigate resource overutilization.
-
xxxxxxxxxx
Enhance Hardware Capabilities:
Upgrading server-memory allocation could also serve as a more scalable solution.
A deep dive into Node.js child_process documentation may further illuminate understanding on managing child processes effectively. Additionally, exploring community forums for real-world solutions such as Stack Overflow threads could offer unexpected insights.
As famously said by Linus Torvalds, “Talk is cheap. Show me the code.” Bringing this ethos into play, observance and hands-on experimentation assists developers in mastering processes; providing them the prowess to efficiently debug such errors.