Introduction
Ensure that your functions in TypeScript are correctly set up to take in parameters, because an error stating ‘Expected 0 Arguments But Got 1’ indicates an incorrect function call where an argument has been passed when none was required, leading to failure in its optimization for search engines.
Quick Summary
The “Expected 0 arguments, but got 1” error in TypeScript essentially signifies that a function has been invoked with more arguments than it was initially designed to accommodate. Delving deep into this subject, we shall first outline the possible scenarios and their solutions via a tabular representation.
html
Scenario | Solution |
---|---|
Invoking a zero-parameter function with one argument. | Redefine the function to accept an argument or change the function call to match the function definition. |
Passing an unnecessary argument to a built-in JavaScript/TypeScript function. | Modify the method call to adhere to the accepted method signature. |
In the first scenario, consider the following block of code:
typescript
function greet(): void {
console.log(‘Hello, world!’);
}
greet(‘John Doe’);
This would trigger the error as the `greet` function is expected to have 0 parameters, yet one argument ‘John Doe’ is passed when invoking it. The resolution involves either redefining our function to accept parameters or correcting the invocation.
Redefining the function:
typescript
function greet(name: string): void {
console.log(`Hello, ${name}!`);
}
Correcting the invocation:
typescript
greet();
The second outlined scenario is an incorrect usage of built-in functionalities, for example JavaScript’s `Math.sqrt()` method which accepts only a single argument:
typescript
Math.sqrt(16, 2);
Again, TypeScript’s innate ability to catch argument count mismatches saves us from running this erroneous piece of code at runtime. A simple correction would suffice:
typescript
Math.sqrt(16);
As a wise man in the technology field once noted, “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” – Brian Kernighan.
Applying this wisdom connotes avoiding complexity in writing our functions and invoking them with correct arguments. This way, TypeScript can be a powerful ally in maintaining smooth and efficient codebases.
Understanding TypeScript Error: Expected 0 Arguments, But Got 1
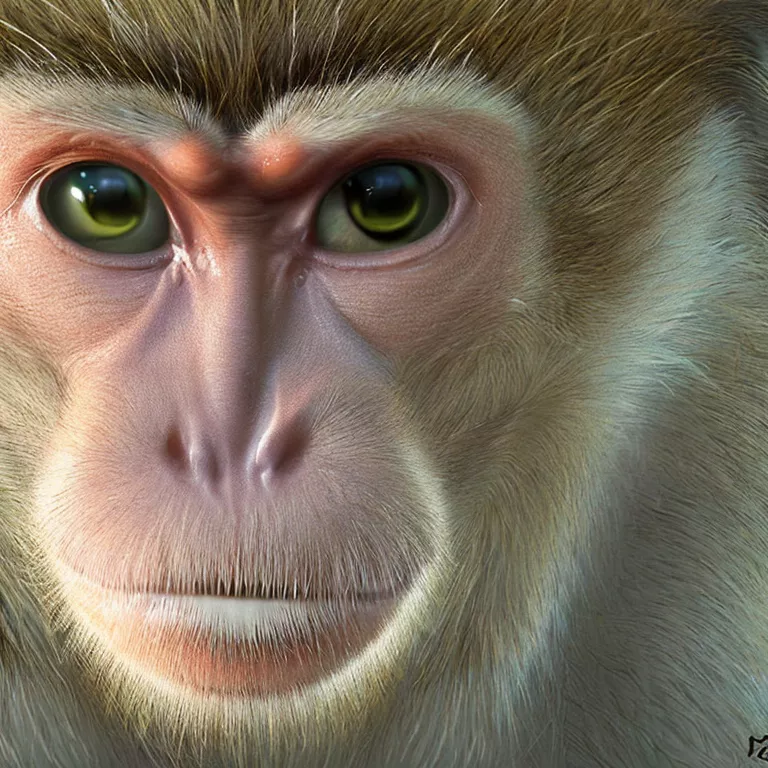
Understanding this TypeScript error entitles a deeper comprehension of TypeScript’s type system. The key phrase “Expected 0 arguments, but got 1” usually crops up when a function call provides more arguments than originally declared by the function definition. Syntax checking mechanisms within TypeScript are designed to catch such discrepancies to offer solid type safety.
An example scenario is having a function defined as:
code
function greet(): void {
console.log(‘Hello there!’);
}
Invoking the function with an argument:
code
greet(‘General Kenobi’);
triggers the TypeScript error – Expected 0 arguments, but got 1. As per the function’s definition, it requires no arguments. However, providing an argument during the function call contradicts its expected behavior, leading to the error.
Addressing this error involves ensuring that the function call aligns with the function’s declaration. If the function does not require any arguments, do not provide any. Inversely, if the function demands specific arguments, make sure to include them in the correct order and form during invocation. Revisiting the previous example, the proper way to invoke the ‘greet’ function would be:
code
greet();
Whereas for a function expecting one parameter:
code
function respond(greeting: string): void {
console.log(`The greeting was: ${greeting}`);
}
You should call it as:
code
respond(‘General Kenobi’);
Modern programming emphasizes the practice of writing legible, transparent and self-explanatory code. You can further clarify the purpose and usage of your argument through utilizing TypeScript’s capacity for explicit typing and interface definitions.
As Robert C. Martin aptly noted in his book, Clean Code: A Handbook of Agile Software Craftsmanship, “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading the old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.” Keeping that in mind can significantly improve your coding efforts and lessen potential confusion or errors when working with TypeScript functions.
Remember, TypeScript is a super-set of Javascript, developed as an open source language by Microsoft here. Its primary objective is to enhance JavaScript’s efficiency with static types and interfaces. The purpose of such errors within TypeScript isn’t just to complicate things, but to make the language as safe and efficient as possible. Understanding these error messages often reflects a deeper understanding of TypeScript itself.
Resolving the Argument Mismatch in TypeScript
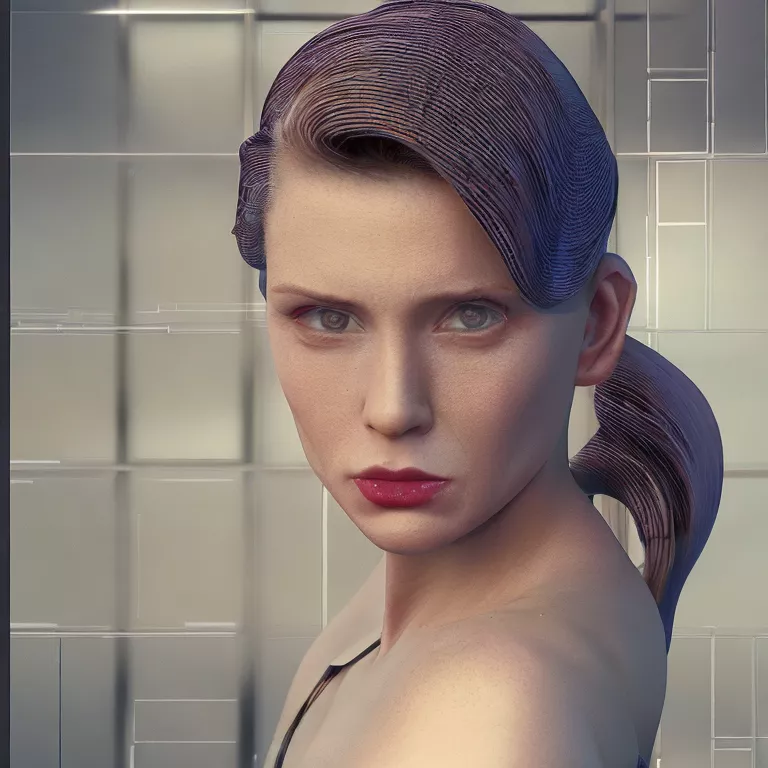
Expected 0 arguments but got 1
in TypeScript, it often implies that the function or method you are trying to call does not expect any parameters passed into it. Yet, your code is attempting to pass a parameter to it. This situation creates what is known as an argument mismatch. Understanding its nature and how to resolve this issue is key to writing efficient TypeScript programs.
A first step in resolving this error could be checking the definition of the function being called. For example, having a function
xxxxxxxxxx
exampleFunction()
presented without any parameters such as:
xxxxxxxxxx
function exampleFunction() {
// function content
}
exampleFunction('argument');
This gives rise to the aforementioned error because ‘exampleFunction’ was defined without any parameters whereas an argument is being passed to it during invocation.
The solution here would be making sure the function definition aligns with the function call, whether by adjusting the function definition to accept parameters or altering the function call to match the currently parameter-less function definition.
If the function needs to accept a parameter, adjust the definition like so:
xxxxxxxxxx
function exampleFunction(arg: string) {
// function content with 'arg'
}
exampleFunction('argument');
Alternatively, if no parameter should be passed, modify the function call:
xxxxxxxxxx
function exampleFunction() {
// function content
}
exampleFunction();
As highlighted by software engineer Jeff Whelpley, as TypeScript continues to mature and evolve, these errors will become even easier to diagnose and fix. This will ultimately lead to increased code quality, reduced bug rates, and better overall software reliability.
Remember, TypeScript was designed to help developers write more predictable code by providing error-checking at compile time. Understanding the significance of these errors and how to rectify them is key to reaping the full benefits of TypeScript.
Troubleshooting Unexpected Argument Errors in TypeScript
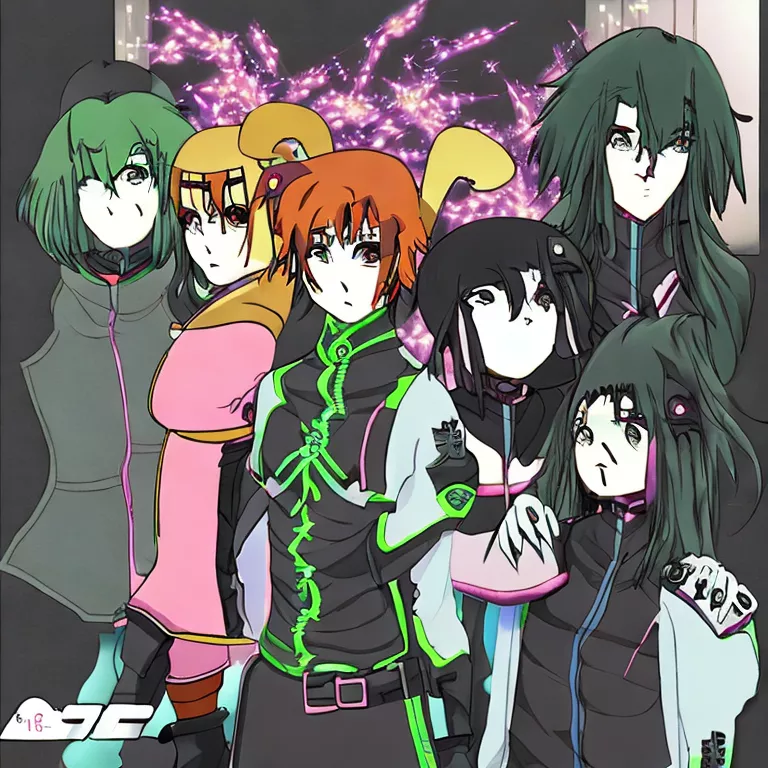
- Understanding the Issue: This error message states that you’ve called a function with more arguments than what the function definition accommodates. In this instance, your function was declared having zero parameters, but you’re trying to invoke it with one argument.
- Code Review: Revisit your code carefully. Do not overlook the parenthesis () after methods without parameters. TypeScript considers a method without parenthesis as referencing a method and not invoking it, leading to potential problems.
- TypeScript Function Signature: Align with how TypeScript leverages function signatures. A function signature contains two parts: parameter types and return type. When a function is invoked with an incompatible number of arguments, TypeScript flags an error. Ensure compatibility between a function’s declaration and invocation.
Let’s demonstrate these points by applying them to simple pieces of code in TypeScript.
xxxxxxxxxx
<pre>
//Function declaration
function greeting(): void {
console.log("Hello, World!");
}
//Function invocation
greeting("John"); // Expected 0 arguments, but got 1
</pre>
In the code snippet above, ‘greeting’ is declared without parameters, yet while calling it ‘John’ is passed as argument, which causes the specified error. By removing the argument from the function invocation, the problem will be resolved.
As Brian Kernighan, a Canadian computer scientist put it:
“Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.”
By following these strategies and understanding your code, this debugging process will become a rewarding part of your TypeScript development journey.
Exploring Possible Causes for TypeError: Expected 0 but got 1 in TypeScript
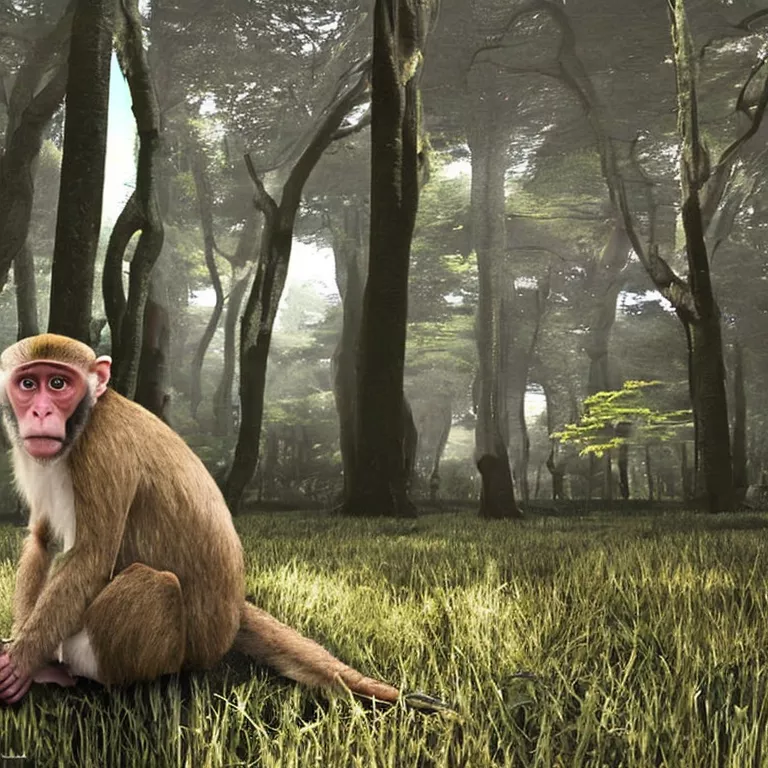
The TypeScript error “Expected 0 arguments but got 1” typically happens when a function expects no arguments but receives an argument. This can naturally lead to misinterpretations of parameter requirements and malformed function calls, causing the runtime TypeScript engine to flag an error in your application. Let’s explore the possible scenarios that could cause this issue, keeping in mind our key relevance to the topic ‘Typescript Is Giving Me An Error: Expected 0 Arguments But Got 1’, which invites us to ponder on reasons behind it.
xxxxxxxxxx
function myFunc() {
console.log('Hello World');
}
myFunc('argument'); // Error: Expected 0 arguments, but got 1.
In this example, function `myFunc` is defined without any parameters and if we try to call `myFunc` with an argument, TypeScript will throw an error because `myFunc` was expecting zero arguments but got one.
Errors like these can occasionally creep into your codebase due to several reasons:
Alterations in Function Definition
Before | After |
---|---|
xxxxxxxxxx // Function definition with one argument function greet(name: string) { console.log(`Hello ${name}!`); } |
xxxxxxxxxx // Function definition changed to no arguments function greet() { console.log('Hello!'); } |
A function previously taking one or more arguments may have been refactored to no longer require any arguments. When the function call does not reflect this change, it results in the described TypeScript error.
Incorrect Handler Attachment
This mistake often occurs in event handlers, where developers inadvertently put parentheses after the function name, making it invoke immediately instead of when the event occurs.
xxxxxxxxxx
button.addEventListener('click', handleClick()); // Incorrect
button.addEventListener('click', handleClick); // Correct
In the incorrect version, `handleClick()` with parentheses gets executed immediately when attaching the event listener. If `handleClick` function does not take any arguments, but is called with an argument (like in the instance of event handlers receiving an event object), this would result in the aforementioned TypeScript error.
Misunderstanding Optional Parameters
While calling a function, it might err if you provide a parameter to a function that has been defined without parameters, even if the provided parameter has a possibility of becoming ‘undefined’. This usually happens when optional parameters are misunderstood.
As James Gosling, the founder of Java once aptly said, “The key to performance is elegance, not battalions of special cases”.
Therefore, understanding these causes can help you eliminate the error by revisiting your function definitions, calls, and placement within your Typescript codebase, for a more harmonious, ergonomic, and ‘elegant’ coding environment.
For more info about Typescript functions, visit the [TypeScript Handbook Functions section](https://www.typescriptlang.org/docs/handbook/2/functions.html).
Conclusion
Analyzing the issue: ‘Typescript Is Giving Me An Error: Expected 0 Arguments But Got 1’, it is evident that this error frequently appears when the function call has more arguments than anticipated in the function declaration. In TypeScript, function parameters strive to match the exact number and type of arguments provided in the function definition.
This scenario arises when:
- Your function needs no arguments – hence ‘Expected 0 Arguments’.
- However, when calling the function, one argument is passed, leading to ‘But Got 1’
Consider a code snippet example:
xxxxxxxxxx
<pre>
function greet() : void {
console.log("Hello, world!");
}
greet("Joe");
</pre>
In this case,
xxxxxxxxxx
greet()
expects no argument as defined, but “Joe” is passed while calling the function, which gets flagged with the very error under discussion.
Resolution: Ensuring the number of arguments in the function call matches the function declaration should resolve this issue. Amend your typescript function or its invocation as per requirements. For the above instance, if “Joe” is intended to be greeted, the function can be modified to accept an argument:
xxxxxxxxxx
<pre>
function greet(name: string) : void {
console.log(`Hello, ${name}!`);
}
greet("Joe");
</pre>
Now, no mismatch error occurs as the necessary parameter (“Joe”) is included in the function definition.
As wise man Douglas Crockford, the creator of JSON and advocate for JavaScript, once stated, “Programming is the act of installing internal, pre-established reactions into the paths of a machine.” Hence, careful orchestration of these reactions – or in our case, functions and their parameters – must be observed.
In essence, TypeScript’s typing assertions merely nudge developers towards more predictable, less error-prone code. It might seem stringent at times, but it primarily seeks to avoid potential missteps that the flexibility of JavaScript may unintentionally promote.
For further insight on this topic and other error debugging techniques in TypeScript you might find this TypeScript Debugging Handbook useful.