Introduction
Through leveraging Typescript generics, it becomes less problematic to handle situations where an object is of an unknown type, ensuring your code remains robust and resilient in the face of uncertain data types.
Quick Summary
The
unknown
in TypeScript is a type-safe counterpart of
any
. Anything is assignable to
unknown
, but you can’t do anything with values of this type unless you do some form of checking to narrow the types. Generic programming in TypeScript involves writing code that can handle different types, defined at runtime.
Below, I create a table that summarizes these concepts:
Type | Description | Applications |
---|---|---|
Unknown |
A type-safe alternative to
any . Nothing can be done without explicit type-checking. |
When we are not sure about the type we will be dealing with. |
Generics |
Supports a variety of types. Promotes reusable and maintainable code. | Useful when we want our functions or classes to support multiple types, but still maintain type safety. |
In relation to the
unknown
type, taking an object as an input where we don’t know its type, we use
unknown
. As for TypeScript Generics, they are used when we want to design functions or classes that can work over several types rather than a single one.
For instance, consider a function that merges two arrays — no matter the type of elements they contain. Here’s a way to write it using generics:
function mergeArray(array1: T[], array2: T[]): T[] { return [...array1, ...array2]; }
Then, we can call
mergeArray([1, 2, 3], [4, 5, 6]);
or
mergeArray(['a', 'b'], ['c', 'd']);
. As you can see, generics help ensure type safety while keeping the code flexible.
As Douglas Crockford once said about JavaScript (which TypeScript is a superset of): “JavaScript is the world’s most misunderstood programming language. But, JavaScript is not a bad language. It is an outstanding, dynamic programming language.” Exploring topics like TypeScript’s unknown types and generics helps peel back that layer of misunderstanding and really explore the potential of this dynamic language.
When it comes to
unknown
, we can safely accept inputs of type
unknown
and perform checks as necessary. If we were using
any
instead, TypeScript would let us make any operations on this value, potentially leading to runtime errors that couldn’t be caught at compile-time.
In effect, both TypeScript’s
unknown
and generics allow us to write more robust and versatile code. They help capture user intent in a way that’s friendly with the type checker enhancing overall language efficiency.
Understanding the Concept of “Object is Of Type Unknown” in TypeScript
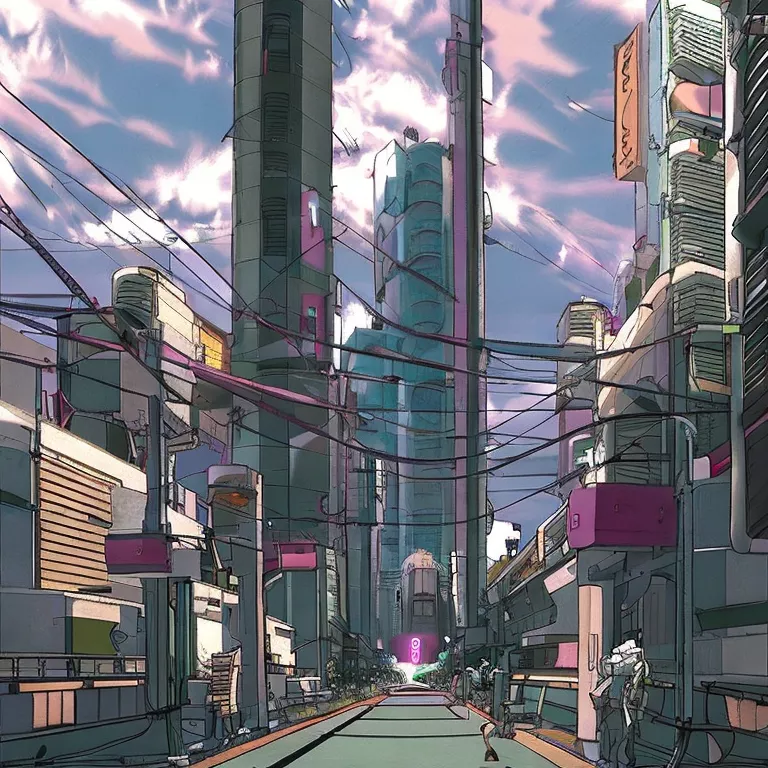
The Type
unknown
is one of the key features in TypeScript, aiding in achieving code safety and readability. By declaring an object to be of type
unknown
, you communicate to fellow developers that the specific nature of the variable remains nebulous, subject to change, or depending on the context where it is used. However, when tied to the concept of TypeScript Generics, it cultivates a bolstering layer of flexibility and reusability.
Working with an
unknown
type can seem daunting because it cannot be worked with directly due its broad applicability. You may not call any methods, access any properties, or pass it on to another method without employing some form of additional verification—known as “typeguards.”
Consider an illustrative example:
function processInput(input: unknown) { if (typeof input === "string") { console.log(input.toUpperCase()); } else { console.log(input); } }
Since we started off defining
input
as
unknown
, we had to use a typeguard (
typeof input === "string"
) to check if it’s safe to run a string-specific method on it.
unknown
comes into its own real element when paired with Generics. Generics allows creating reusable components while maintaining a sense of safety. It provides the user inferred types based on how they interact with your component. Being capable to handle diverse data types, it pairs seamlessly with the concept of
unknown
type.
Continuing our previous example involving Generics:
function processInput(input: T): T { if (typeof input === "string") { return input.toUpperCase() as any as T; } else { return input; } }
In this example, the input and output types are guaranteed to be the same through the use of Generic
T
. The function doesn’t specify which properties it expects, nor what type
input
should be. Instead, it utilizes generics, i.e.,
T
, to automatically infer and align the output with the nature of your input.
Echoing Linus Torvalds words—creater of Linux kernel—”Bad [code] is a disease – a contagious one. When you see bad code, run away from it and try to find good, healthy code where function boundaries are respected.” Understanding the use of
unknown
in TypeScript Generics is intrinsically linked to creating healthy, flexible, and reusable code.
Utilizing Generics for Ensuring Type Safety in TypeScript
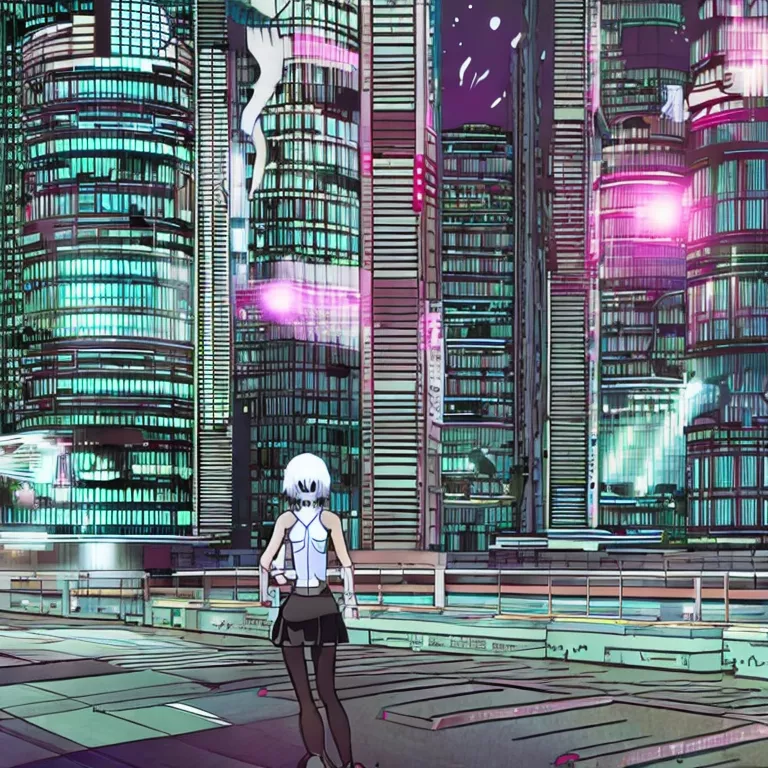
In the pursuit of enhanced code robustness, scalability, and understandability, TypeScript leverages static typing to compile-time error checking through its Generics feature. To fully grasp the importance and practical application of generics in TypeScript, one should also comprehend the “unknown” type – a powerful tool that can help developers uphold type safety.
Embracing the Unknown
Introduced in TypeScript 3.0, the
unknown
type essentially means that a variable could be anything. It obligates the developer to perform some type of checking before manipulating the value stored. By preventing operations on values of type
unknown
without a type assertion or a control flow based type narrowing, it supports extensive type safety in potentially tricky scenarios.
For instance, this code will fail at compilation:
let value: unknown; value = "hello world"; console.log(value.toUpperCase());
The compiler flags an error because it can’t ensure that
value
is a string when
toUpperCase()
is called. The compiler has ensured type safety here by not letting potential run-time errors pass unchecked.
TypeScript Generics Ensure Type Safety
The potency of generics lies in their ability to handle multiple types, while still maintaining type safety. Ultimately, generics enable you to write dynamic and reusable code pieces without losing the benefits of type checks.
Here’s how you might use a generic:
function getValue(arg: T): T { return arg; }
<T>
is a placeholder for any type. When calling the function, you replace
T
with an actual type. If you attempt to pass an argument that doesn’t match the expected type, TypeScript flags this as an error. This demonstrates a simple way to avoid potential execution pitfalls and maintain type safety.
Combining Unknown and Generics for Maximum Type Safety
The tandem use of
unknown
and Generics in TypeScript could further reinforce the application’s type safety. Upon declaration, an
unknown
variable is empty. The user can initialize this variable with any type using generics, which validates the variable’s new value:
function setValue(value: T): void { let myValue: unknown = value; // Further code working on myValue... }
In the above function,
T
specifies the type
value
should have. As you initialize
myValue
with that input value, TypeScript assumes that this
unknown
typed variable has the specific
T
type. Consequently, this bolsters the type safety enabled by TypeScript.
As Bill Gates put it, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency. The second is that automation applied to an inefficient operation will magnify the inefficiency.” Indeed, maximizing the utilization of TypeScript’s features like Generics and the unknown type, will certainly help increase the efficiency of your TypeScript projects.
Exploring Real-world Scenarios where “Object is Of Type Unknown” Applies
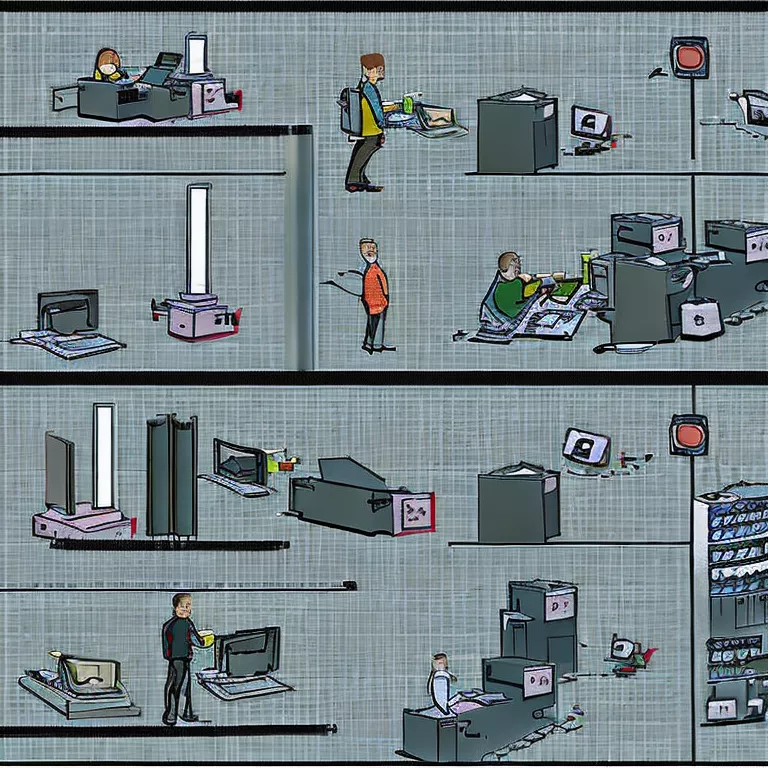
The exploration of real-world scenarios where “Object is Of Type Unknown” applies is fascinating especially considering its relevance to TypeScript generics. This is one of TypeScript’s myriad features that greatly enhance the Belt of JavaScript with the powers of static typing, leading to safer, more comprehensible code.
Consider an example in a function that accepts any kind of input and returns an object of unknown type:
typescript
function getJsonObject(jsonString: string): unknown {
return JSON.parse(jsonString);
}
In the above code snippet, the
getJsonObject
function uses the
unknown
type as it does not know what object will be parsed from the given JSON string. Using
unknown
here is safer than using
any
because TypeScript requires us to do some sort of type checking before performing operations on the result.
You may wonder, where does TypeScript generics come into play? Well, consider a situation where you want to ensure that the object you are parsing have certain properties. Here’s how you might improve the function by adding TypeScript Generics:
typescript
function getJsonObject<T>(jsonString: string): T {
return JSON.parse(jsonString);
}
interface User {
name: string;
age: number;
}
const user = getJsonObject<User>(‘{“name”:”John”,”age”:30}’);
console.log(user.name); // John
In this improved version, we use TypeScript Generics to let the function caller specify what shape they expect the resulting object to take. This can prevent potential runtime errors as TypeScript compel checks for property existence when generics are in use.
“<a href=’https://www.typescriptlang.org/docs/handbook/generics.html’>Generics</a>” provide a way to make components work with any data type and not restrict to one data type. In this way, the type variable captures the user-provided type argument (e.g., number), so that we can use that information later. Thus, generics give us the advantage of abstracting types yet keeping type safety intact.
This interesting observation can be lined up with a quote from Addy Osmani, an Engineering Manager at Google working on Chrome, who says “*Code is read more than it’s written.*” With TypeScript, that reading becomes even more effortless and intuitive, especially when combined with powerful features like TypeScript Generics and the
unknown
type.
Leveraging the Power of TypeScript Generics to Handle Unknown Types
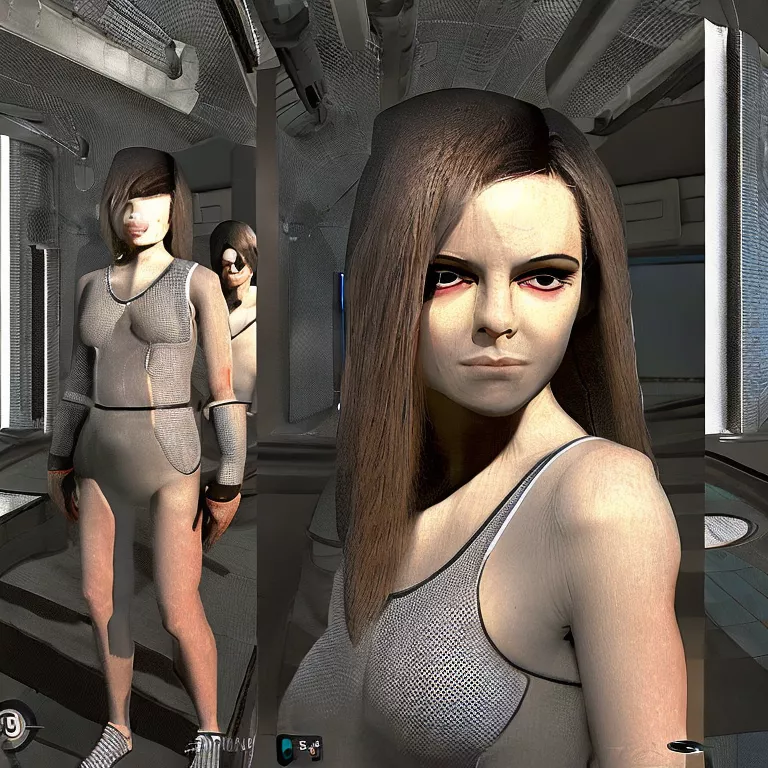
Leveraging TypeScript’s powerful Generics can significantly help in handling unknown types. In TypeScript, when we are uncertain about the type of a variable, we may opt to use the `unknown` type.
TypeScript Generics
TypeScript Generics act as a tool for creating reusable components while preserving the integrity of the components.
Consider having an operation that works on a variety of types instead of a single one. To achieve this, you can employ TypeScript Generics:
function genericFunction<T>(arg: T): T { return arg; }
The “ here is known as the type variable, and it is a stand-in for any type. You’re free to replace `T` with any valid identifier.
The Unknown Type
TypeScript provides us with a specialty type known as `unknown`. This type indicates the absence of type checking and guarantees. Essentially, `unknown` represents all possible types hence providing assistance where you are uncertain about a variable’s type. An example in action looks like so:
let notSure: unknown = 4; notSure = 'maybe a string instead';
Leveraging Generics for Unknown Types
Now, how do we correlate Generics with the `unknown` type then? Here, the power of generics supplements the unknown type, allowing for greater flexibility and safety.
Consider the following example:
function handleUnknown<T>(arg: T | unknown) { // handle arg }
In this function, `arg` can be of any type. We’ve accomplished that using the `unknown` type and generics `T`, which makes our function more versatile without compromising type safety.
As Joel Spolsky once said – “The great thing about object-oriented programming is that it allows stupid code to interface with smart code.” This statement indeed echoes in the TypeScript world, where generics along with the unknown type act as the bridge allowing any typed variable to be handled safely and effectively within a function.
Reflecting on this, we can perceive how valuable the combination of Generics and Unknown Types is when dealing with varying types. It gives us the toolset to manipulate data while ensuring strong typing, thus improving the readability, maintainability, and integrity of the TypeScript codebase.
Conclusion
The relevance between “Object is of type unknown” and TypeScript Generics can be appreciated when one talks about dealing with variable types in the TypeScript language. Leveraging TypeScript generics, a component of TypeScript’s type system, allows developers to create reusable code without compromising type safety. Create components that can operate across a range of types rather than a single type can satisfy broader use cases.
“Object is of type unknown” is a common issue many face while working with TypeScript. This problem arises when TypeScript isn’t able to determine the type of an object. An understanding of this concept is crucial for developers who want to have stricter type-checking and compile-time checks.
Key insights into Object Is Of Type Unknown
TypeScript encapsulates JavaScript and also introduces strict type-checking to your codebase via static types. However, during transpilation, TypeScript removes type annotations converting it back to plain JavaScript.
Every value in TypeScript has a type, even if TypeScript doesn’t know what it is. The ‘unknown’ type represents the superset of every possible type in TypeScript. If a variable is of type ‘unknown’, we must perform type checking before performing operations on it
. (let notSure: unknown; notSure = 4; console.log(notSure); // prints "4" )
Perspective on TypeScript Generics
Generics promote code reusability while maintaining the flexibility of the type system. With generics, you don’t have to write duplicated codes for different data types. It gives us the advantage of creating components that are capable of handling a variety of data types securely
. function genericFunction(arg: T): T { return arg; } )
As per Robert C. Martin’s book “Clean Code”, “Duplication is the primary enemy of a well-designed system.”
These insights into “Object Is Of Type Unknown TypeScript Generics” highlight the importance of understanding TypeScript’s unique features to code more effectively and efficiently. The effective use of TypeScript generics plays a significant role in extending the application’s scalability, making it resilient to future changes or additions. Additionally, recognizing ‘unknown’ and assuring type-checking can assist in avoiding unexpected errors during runtime. Data type checking along with reusable components allow developers to build applications that are not only robust but also easy to maintain in the long run.