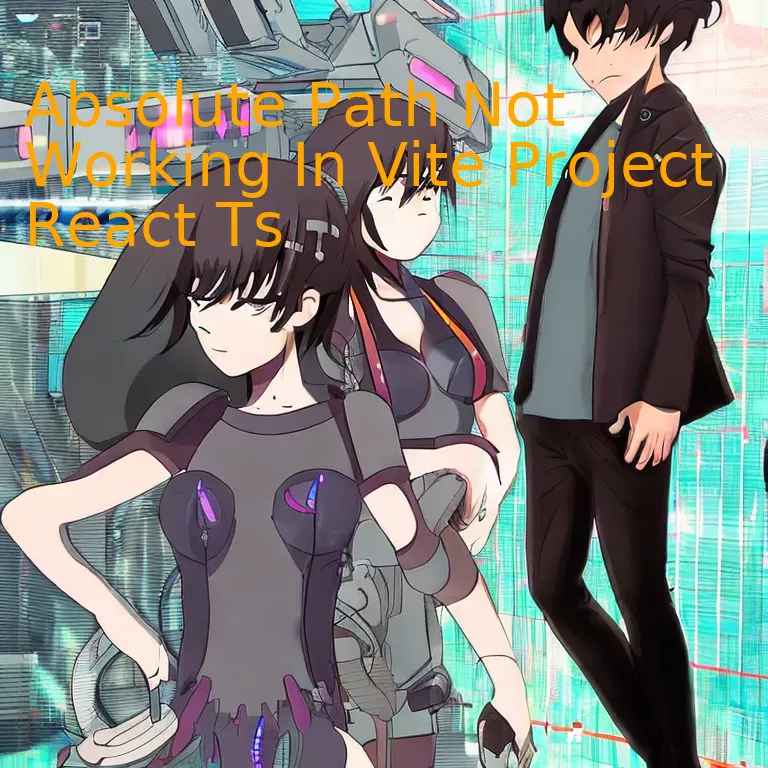
Introduction
In the context of your Vite Project using React TS, encountering issues with the absolute path not functioning could stem from incorrect configuration in your tsconfig.json file, and to resolve it, ensure that the ‘baseUrl’ and ‘paths’ properties are correctly set.
Quick Summary
The issue of an absolute path not working in a Vite project React TS (TypeScript) requires careful analysis. It’s a common issue that developers encounter when using the Vite project with TypeScript and React, resulting from misconfiguration or omission in setting up paths for your project. Now, let’s discuss it through a structured tabular format, representing the primary attributes of this problem alongside their associated potential solutions:
Problem Area | Potential Solution |
---|---|
Project Configuration | If you’re experiencing “Cannot resolve dependency” error, then you need to ensure that the base configuration file ‘vite.config.ts’ is properly set up. Your configurations should include ‘baseUrl’ settings in your tsconfig.json file to indicate where your absolute paths begin. Example:
x "baseUrl": "./src" |
TypeScript Settings | The tsconfig.paths.json file specifies a series of entries which re-map import requests, allowing absolute imports from specified root directories instead of relative ones. Ensure that these are correctly declared, and that they match the corresponding settings in ‘vite.config.ts’. For instance:
xxxxxxxxxx "paths": { "@/*":["src/*"] } |
Vite Resolution | Sometimes, the issue could be Vite failing to recognize the declaration in the ‘vite.config.ts’ during development. In this scenario, utilize Vite’s alias feature in its configuration file to help your system understand your pathing preferences. E.g.:
xxxxxxxxxx { find: '@', replacement: '/src' } |
As we transition to discuss about the table, we note that it centers around three chief areas – project configuration, TypeScript settings, and Vite’s resolution – offering potential solutions to resolve the issue of the absolute path not working.
To quote Edsger W. Dijkstra, “Simplicity is prerequisite for reliability.” Ensuring these simple but effective settings are correctly configured in your Vite project can alleviate the challenges associated with absolute paths and reduce the complexity of file management.
It is crucial to refer to respective Vite , TypeScript Module Resolution and React documentation for more details and improvements which arise over time due to continuous development and upgrades in these technologies.
Remember to frequently test your application during the configuration process to catch any problems early. In doing so, you greatly increase the chance of catching irregularities or issues that could cause your absolute paths to break or not function as expected.
Understanding the Role of Absolute Paths in Vite React TS Projects
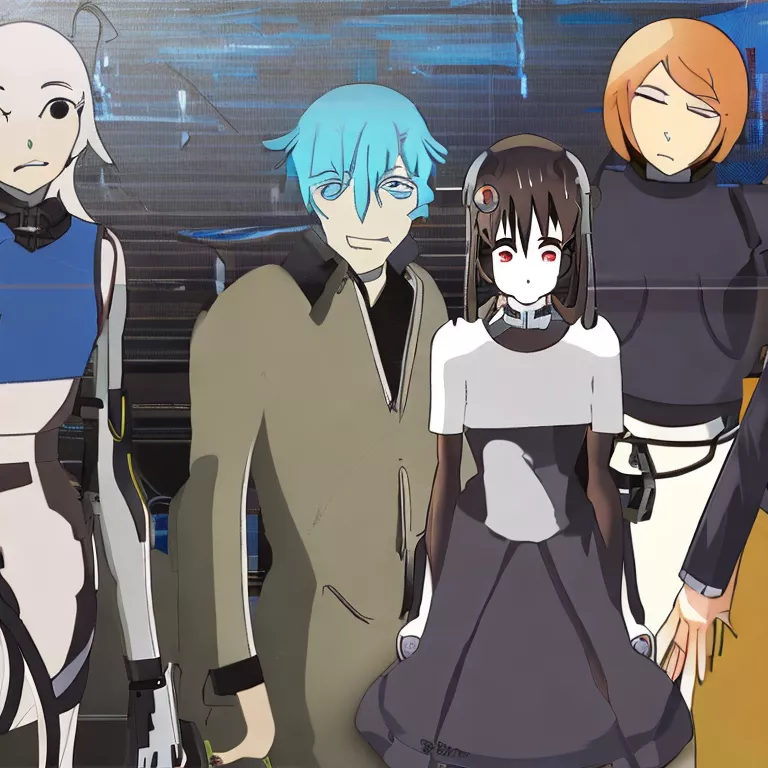
When it comes to managing a Vite React TypeScript (TS) project, understanding the role of absolute paths is crucial to ensure smooth and efficient file management. Using absolute paths for importing modules or resources can make your code cleaner, easier to understand, and more maintainable.
Let us focus on the potential scenario of when an absolute path is not working in your Vite Project React TS.
An instance where an absolute path might not function as expected could be caused by several factors:
1. Incorrect tsconfig.json Configuration
Your `tsconfig.json` file might not be configured correctly. The “paths” and “baseUrl” properties are essential for setting up absolute paths. It may look like this:
xxxxxxxxxx
{
"compilerOptions": {
"baseUrl": "./src",
"paths": {
"@components/*": ["components/*"]
}
}
}
In the above example, `@components` is an alias for the ‘components’ directory in the source (‘src’) folder.
2. Misalignment Between Vite and TypeScript Path Aliases
The alias configurations between TypeScript and Vite may not correspond. Ensure these match within vite.config.ts and tsconfig.json respectively.
Configuration in your `vite.config.ts` file might appear as follows:
xxxxxxxxxx
export default defineConfig({
resolve: {
alias: {
'@components': '/src/components'
}
}
})
Here again, `@components` serves as an alias pointing to the ‘components’ directory in the ‘src’ folder. This configuration should resonate with the one in `tsconfig.json`.
3. Paths Not Resolving Properly
In some cases, the issue might lie with the paths not being resolved correctly during the build time. To mitigate this, make sure you have appropriately installed and configured plugins, like vite-tsconfig-paths.
In your `vite.config.ts`:
xxxxxxxxxx
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
import tsconfigPaths from 'vite-tsconfig-paths'
export default defineConfig({
plugins: [react(), tsconfigPaths()]
})
This plugin helps Vite to shape up the TypeScript path aliases from tsconfig.json.
By keeping an eye on these aspects, you’ll gain a better understanding of how absolute paths function in a Vite React TS project and how to troubleshoot when things don’t go as expected.
As Bill Gates once said, “It’s fine to celebrate success but it is more important to heed the lessons of failure.” In the case of this issue, understanding the cause and learning how to rectify it would help turn this stumbling block into a stepping stone towards building better and more dynamic projects with Vite and React-TypeScript.
Troubleshooting Common Errors with Absolute Paths in Vite React TS
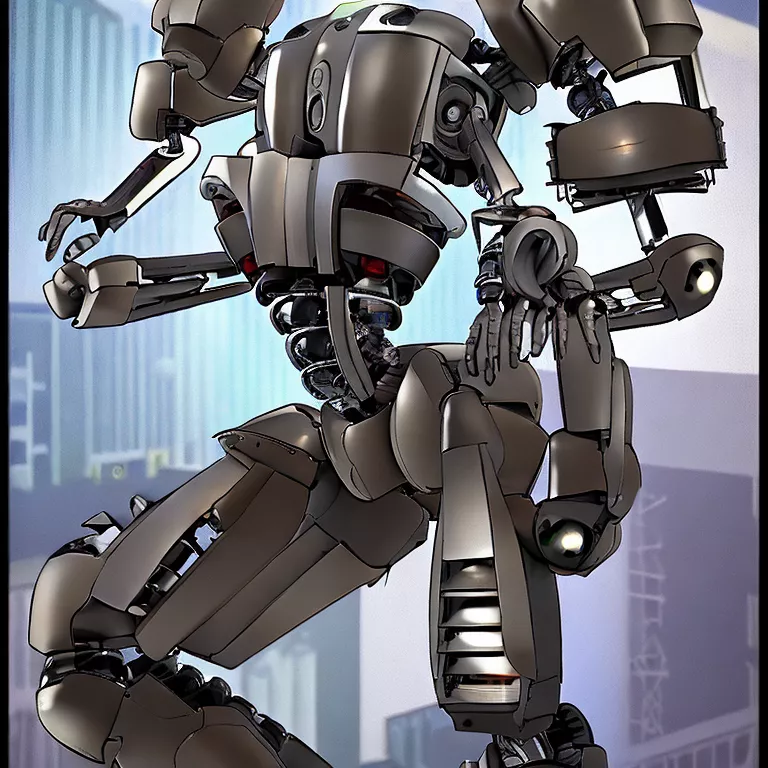
Troubleshooting common errors in using absolute paths with Vite React TS primarily revolves around understanding the dynamics of Vite’s internal structure and TypeScript’s path configuration. One common error faced by developers is when the absolute path does not work as expected in a Vite project powered by React and TypeScript.
The leading causes of this issue could stem from various reasons such as:
– Misconfiguration of `tsconfig.json`.
– Improper resolution of import paths.
– Incorrect definition or usage of the ‘baseUrl’ property in `tsconfig.json`.
Let‘s delve into these problems more in-depth to understand how to troubleshoot them.
Misconfiguration of tsconfig.json
TypeScript leverages the file `tsconfig.json` (TypeScript Documentation) as its main source of structural information about your project. Misconfiguration of this file can cause absolute path issues in your Vite React TS applications. To resolve this, cross-check your `tsconfig.json` file for any syntactical errors or misconfigurations.
xxxxxxxxxx
//Example of tsconfig.json file
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"*": ["src/*"],
"components/*": ["src/components/*"],
"utils/*": ["src/utils/*"]
}
}
}
When correctly set, the TypeScript compiler will interpret and direct the necessary paths accordingly based on this information, resolving absolute path issues.
Improper Resolution of Import Paths
Another common problem relates to the way import paths are resolved, which may differ depending on the specific circumstances of one’s project configuration. Consider a situation where developer uses an incorrect alias which does not conform to those outlined in the `tsconfig.json`. This will lead to the compiler being unable to accurately resolve the import paths. Endeavor to align your aliases consistent to your project configuration.
Incorrect definition or usage of ‘baseUrl’
In TypeScript’s path mapping, the baseUrl represents a base directory used for resolving relative paths. However, if incorrectly defined, TypeScript source code files may fail to translate to their respective JavaScript files correctly, leading to absolute path problems. To mitigate this issue, ensure your ‘baseUrl’ adequately points out to your source code directory such as `src/`.
Michael Dawson, a renowned Node.js technical lead at Red Hat and an active contributor in the tech community, emphasizes the importance of diligent debugging:
“Debugging helps you learn. There is sometimes no better way to understand how things work than by debugging. Seeing the paths code takes through a program during different scenarios can help you understand that program.”
Recognizing potential issues with how paths are handled and managed in your Vite React TS applications is helpful for developers seeking to optimize their coding experience. Developing effective troubleshooting skills supported by an understanding of Vite’s internal structure and TypeScript’s path configuration serves as a critical competence for technologists navigating these environments.
Effective Strategies for Implementing Absolute Path in Your Vite Project
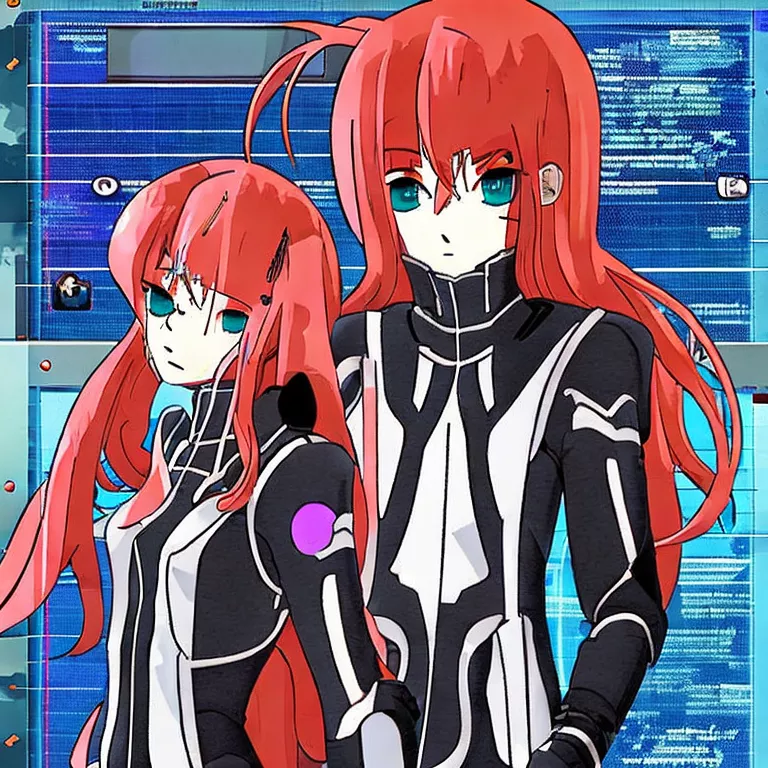
Implementing an absolute path in a Vite project, particularly when using React TS, can entail several strategies. This approach helps eliminate any issues pertaining to the path not working correctly, thus ensuring more seamless app development.
Initially, let’s take a peek at the root cause of this issue. Potentially, if you are attempting to use an absolute path in your React TypeScript (TS) Vite project, then you’re looking to import a module without having to specify its relative path every time. For instance, instead of writing
xxxxxxxxxx
import MyComponent from '../../../MyComponent'
, you may prefer to write
xxxxxxxxxx
import MyComponent from 'components/MyComponent'
. However, because Vite does not natively support this feature, it can result in the path not working as expected.
To effectively rectify this, below are some feasible strategies:
Using jsconfig.json Configuration
One strategy is to use jsconfig.json or tsconfig.json files to set the baseUrl to src. For example, consider the code snippet:
xxxxxxxxxx
{
"compilerOptions": {
"baseUrl": "./src"
},
"include": ["./src/**/*.ts", "./src/**/*.tsx"]
}
With this configuration, imports can be defined from the src directory, allowing for cleaner and more readable code.
Custom Plugin Strategy
The creation and implementation of a custom plugin is another effective solution for handling absolute paths. The plugin should generally set the resolve.alias property of vite.config.js file to the source path, where the component files exist.
xxxxxxxxxx
// vite.config.js
export default {
plugins: myVitePlugin(),
resolve: {
alias: {
'/@/': `${process.cwd()}/src`
}
}
}
This technique will efficiently route all requests that start with /@/ to the src folder, thereby circumventing the absolute path issue.
setBase: ‘src’ Strategy
Another approach to sidestep this problem in Vite would be by informing it to treat src as the base URL within the tsconfig.json file.
xxxxxxxxxx
{
"compilerOptions": {
"baseUrl": "src"
}
}
Notably, success with these strategies can hinge on whether all aspects of your Vite project are correctly configured. Furthermore, remember there’s no one-size-fits-all solution for every situation, implying that a tailored examination of each unique scenario is essential for a workable solution.
According to Joel Spolsky, co-founder of Stack Overflow, he asserts that “It’s harder to read code than to write it.” As such, using an absolute path not just makes your codes more readable, neater, and easier to manage but it also contributes greatly to the team effort especially during the debugging process when everyone is trying to figure out what went wrong in a sea of codes. This encapsulates why it’s crucial for this issue to be handled appropriately and strategically.
Optimizing Performance through the Use of Absolute Path in Vite React TS
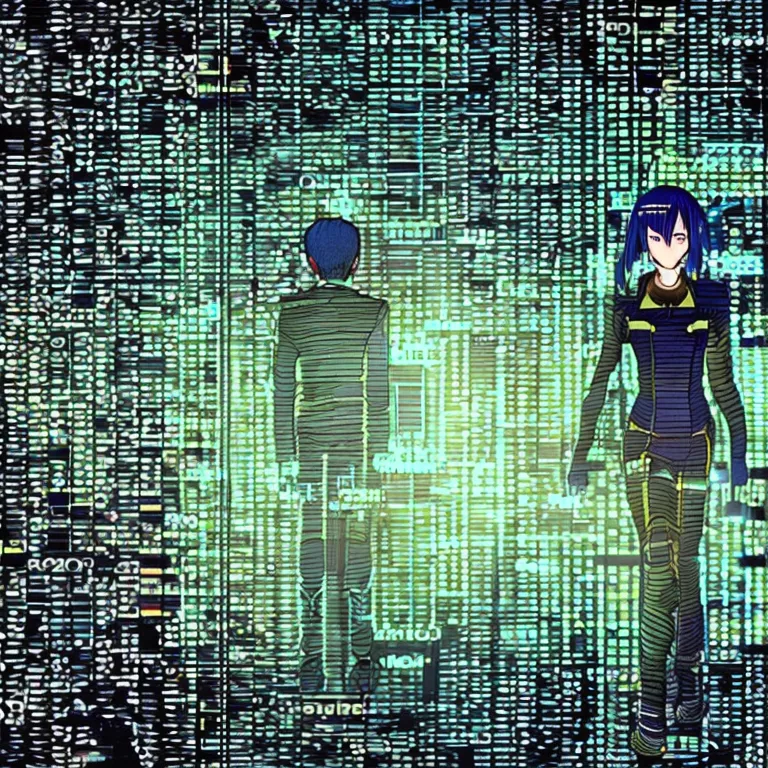
Understanding the importance of optimizing performance in Vite using absolute paths instead of relative paths is crucial. This strategy of optimization can offer multiple advantages as long as it’s employed appropriately. However, issues may arise in certain situations where an absolute path seems not to work in a Vite project with React and TypeScript (TS).
Firstly, let us delve into why absolute paths promises more efficiency than relative ones:
Benefits of Absolute Path Optimization for Performance
• They keep your codebase tidy and navigable: Each reference will point to an explicit location which does not change regardless of the user’s viewing location. Thus, organizing your folders and finding resources become significantly less strenuous.
• They decrease potential downtime and speed up the website’s rendering process: This is because they preclude the necessity for further searches for required assets.
Replacing relative paths with absolute paths might aid your Vite application’s performance optimization efforts, provided it is implemented accurately. Regrettably, you may face some challenges while implementing this in a Vite React TS project, and the following could be some factors causing such issues:
Possible Causes of ‘Absolute Path Not Working’
1. Vite Configuration: Inaccurate configuration in the
xxxxxxxxxx
vite.config.ts
file may cause issues with absolute paths. It’s necessary to properly configure
xxxxxxxxxx
alias
and
xxxxxxxxxx
base
options in the config file.
2. TypeScript Configuration: When combining Vite and TypeScript with React, your
xxxxxxxxxx
tsconfig.json
should be properly set up, specifically the
xxxxxxxxxx
"baseUrl"
and
xxxxxxxxxx
"paths"
options. If these settings are incorrect, problems with absolute paths might occur.
To further illustrate, if you are facing challenges with absolute paths not working, the solution might be as simple as appropriately setting up your vite config and tsconfig files. Below is an example of how to do this:
Code Example
xxxxxxxxxx
// vite.config.ts
import { defineConfig } from 'vite';
import reactRefresh from '@vitejs/plugin-react-refresh';
export default defineConfig({
plugins: [reactRefresh()],
resolve: {
alias: {
'@': '/src'
},
},
});
xxxxxxxxxx
// tsconfig.json
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"@/*": ["src/*"]
}
}
}
With these configurations, you can then import components in your code like so:
xxxxxxxxxx
import ComponentName from '@/ComponentFolder/ComponentName';
The “@” here represents the preset absolute path leading to the source directory.
In the words of Linus Torvalds, “Talk is cheap. Show me the code.” This quote implies that proper code implementation is key to solving problems and optimizing performance. Optimization using absolute paths is no exception. The adoption of absolute paths in Vite React TS projects might pose some difficulties initially. But once correctly executed, it’s a sure way to enhance performance and improve the project structure.[1]
Conclusion
Vite, a next-generation front-end build tool, brings both speed and simplicity to modern web development. However, one issue that developers can stumble upon is the “Absolute Path Not Working” in their React TypeScript (TS) Vite project.
Understanding the anatomy of file paths is crucial to navigate this hurdle. Absolute paths are initiations from the root directory, providing an explicit route to a particular file or directory within the system source. Intended for dependability, absolute paths can sometimes create issues in a Vite project with React TS.
The possible causes for this could be:
-
xxxxxxxxxx
tsconfig.json
: The settings and configurations in your TypeScript configuration file play a significant role. Using the baseUrl and paths properties correctly is imperative for successful absolute imports.
- Package Dependencies: Node.js uses CommonJS to handle modules by default. If you’re experiencing troubles with absolute addressing, ensure all dependencies are installed correctly.
- Vite Configuration: Vite itself may require additional configuration settings like configuring the ‘alias’ property inside the
xxxxxxxxxx
vite.config.ts
file.
Solving this usually involves ensuring all configurations are set correctly. Here’s an example of how the `vite.config.ts` and `tsconfig.json` files should look like:
xxxxxxxxxx
// vite.config.ts
export default defineConfig({
resolve: {
alias: {
'@': resolve(__dirname, 'src')
}
}
})
xxxxxxxxxx
// tsconfig.json
{
"compilerOptions": {
"baseUrl": "./",
"paths": {
"@/*": ["src/*"]
}
}
}
Withstanding challenges such as these is part and parcel of a developer journey. As Donald Knuth, a prominent figure in the computer science and programming world cited, “The process of preparing programs for a digital computer is especially attractive, not only because it can economically and scientifically rewarding but also because it can be an aesthetic experience much like composing poetry or music.”source Therefore, continue to engage with TypeScript in Vite and React, master absolute paths usage, and enhance project efficiency.
Do remember that each project’s specific requirements will dictate tweaks and changes to how these paths are managed; identify your needs and fine-tune accordingly.