Introduction
In dealing with JavaScript programming, you may encounter “This expression is not callable. Type number has no call signatures” error, a common issue highlighting your attempt to invoke a numeric data type as a function, which contradicts its inherent nature and format rules defined by the coding language.
Quick Summary
This common error message in TypeScript, “This expression is not callable. Type ‘Number’ has no call signatures,” happens when you try to invoke a value that’s been assigned a type which does not include a call signature, typically caused by trying to invoke or call a number as if it were a function.
The table below provides a quick breakdown:
Error Statement | Problem Causing the Error | Possible Solution |
---|---|---|
This expression is not callable. Type ‘Number’ has no call signatures. | A value is being invoked or called like a function which was declared with a ‘Number’ type | Review the code to ensure functions are correctly identified and number values aren’t inadvertently coded to be executed as functions |
In TypeScript, each type boasts its unique characteristics and properties. The ‘Number’ type, for instance, primarily represents numeric values – not a function’s operational capability. Thus making an attempt to use a numerical value (like invoking a function) can unsurprisingly trigger this error statement.
Consider the example shown below:
let x: number; x = 5; x();
In this scenario, a variable ‘x’ is defined as a number and then assigned the value ‘5’. Next, there’s an attempt to call ‘x’ as if it were a function. Because ‘x’ is not a function (but instead a number), TypeScript flags an issue and throws the error: “This expression is not callable. Type ‘Number’ has no call signatures.”
To fix such issues, ensure that any function invocations are applied to appropriate function names and not variables holding types that have no call signatures. Remember, TypeScript’s static type-checking is there to help you spot potential errors in your code early during development.
As Martin Fowler, a renowned author and international speaker on software development, explained, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” This adage certainly applies to the proper use of types in TypeScript.
If you’ve run into this TypeScript error, understanding what’s causing it allows you to approach the task of debugging more scientifically, and leaves less room for guess work. The underlying principle emphasized here is that an item defined as a number or any other non-function type should be used appropriately and should not get called as if it were a function.
Understanding “Expression Is Not Callable. Type Number Has No Call Signatures” Error
The error “Expression is not callable. Type ‘number’ has no call signatures” usually occurs when you’re trying to invoke something that, in TypeScript language, isn’t invocable or doesn’t have any call signatures. Understanding this involves delving deeper into TypeScript basics.
TypeScript Types:
In TypeScript, everything has a type. The type ‘number’ simply signifies that the variable holds a numerical value – whether integer, float, etc.
Consider this example:
let x: number; x = 5;
Here, `x` is explicitly declared as of type ‘number’, and therefore, it can hold any numerical value.
Call Signatures:
A call signature in TypeScript describes a function type. It entails the types of input the function accepts (parameters) and what it returns (output). However, numerical values and numeric variables do not have a concept of call signatures because they are not functions and cannot be invoked like one.
Problem of “This Expression Is Not Callable”:
Coming back to this error message, if you encounter it, most probably you’re treating a numerical entity as a function, which TypeScript doesn’t allow. Here is an inappropriate way of calling a number that results in aforementioned error:
let x: number; x = 5; x();
In the last line, `x()` implies TypeScript is expecting a function while ‘x’ is clearly a number.
Solution:
Hence, to solve “This expression is not callable. Type ‘number’ has no call signatures.” You need to revise your code so that you’re not attempting to invoke a numeric variable.
Remember, as Steve McConnell underscored in his book “Code Complete”, “Programmers who subconsciously view themselves as artists often feel that they can only work when they’re inspired and that their best work comes from doodling and dreaming, not from the debugging and code reviews”. Thus, it’s okay to get stuck but what’s important is to learn from these errors and refine your TypeScript coding skills.(McConnell) With time and practice, you will become adept at understanding and resolving compilation errors swiftly.
Different Root Causes of “Type Number Has No Callable Signatures”
The TypeScript error message “Type ‘number’ has no call signatures” typically occurs when you attempt to invoke or call a variable that’s been assigned with a number. When appropriately addressed in relation to the TypeScript error “This expression is not callable. Type ‘number’ has no call signatures”, this issue can surface from various root causes:
– Accidental Invocation of a Number: The most common root cause is attempting to call or invoke a number as if it were a function. Take this snippet into context:
let a = 5; a()
This code would result in the aforementioned error because ‘a’ is a number, and numbers are not callable in TypeScript.
– Misassignment of Variables: In certain cases, this error might persist due to inappropriate assignment of values to variables. For instance, if a variable was initialized expecting a function but receives a number instead.
let b: () => void = 5; b();
In this scenario, the variable ‘b’ anticipates a function, yet a number is being assigned, causing the subject error when ‘b’ is called. Here, rectifying the assigned value to ‘b’ to be a function would resolve the issue.
– Incorrect Use of Function Returns: On particular occasions, the issue could stem from misusing what a function returns.
function c() { return 5; } c()();
In the above example, the function ‘c’ returns a number and isn’t callable. Thus, attempting to invoke ‘c()’ results in an error.
These are typical root causes for “Type ‘number’ has no callable signatures” relevant to “This expression is not callable. Type ‘number’ has no call signatures”. However, there might be other unique and infrequent reasons that may vary based on the script’s context.
While dealing with such errors, it is good to recollect the wise words of Andrew Hunt and David Thomas, authors of “The Pragmatic Programmer”, who said, “Remember the big picture. Don’t get so engrossed in details that you forget to check what’s happening around you.” [^1^]
[^1^]: The Pragmatic Programmer
Key Solutions to Rectify the ‘Not Callable’ Error in TypeScript
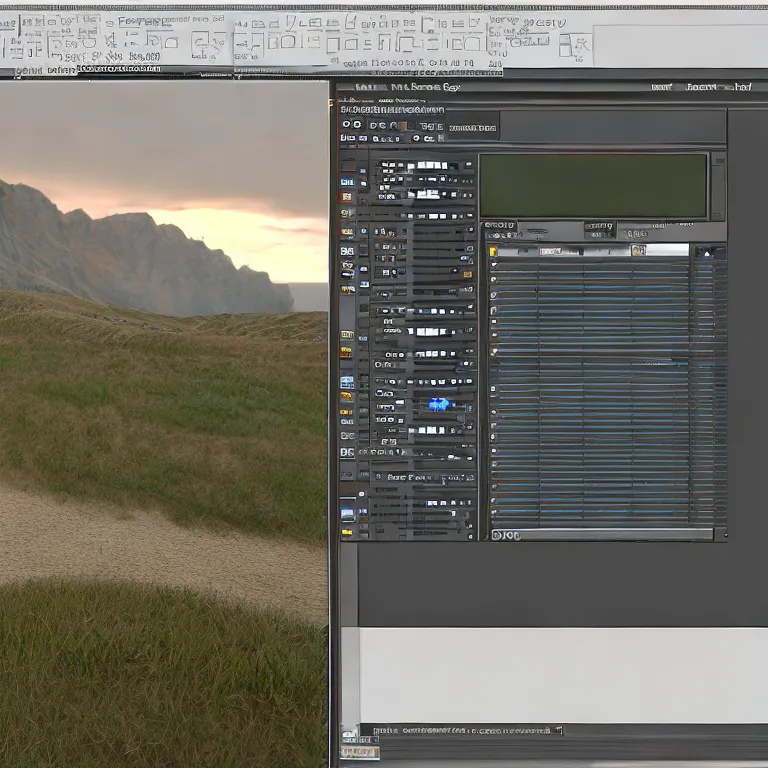
The ‘Not Callable’ error in TypeScript often surfaces when attempting to invoke a function that hasn’t been defined yet. This error is quite common among developers, particularly when dealing with a type mismatch scenario. In your case, the error “This Expression Is Not Callable. Type Number Has No Call Signatures” implies that you are mistakenly trying to call an expression that has been identified as a number.
To rectify this issue, consider employing these following strategies:
Ensure Correct Function Definition
Verify Variable Types
Check the Scope of Variables and Functions
1. Ensure Correct Function Definition
This might seem self-evident, but it’s essential to ensure your function has been defined correctly, and where necessary, exported suitably from its respective module. TypeScript is unable to call an undefined function or one defined in a scope not within reach.
Let’s illustrate with an example. Let’s say we have the following piece of code:
let foo = 123; foo();
This will generate the error:
This expression is not callable. Type 'Number' has no call signatures.
The reason for this error is clear: a number cannot be invoked like a function. To solve this problem, always ensure a function is defined before calling it.
2. Verify Variable Types
TypeScript, as a statically typed superset of JavaScript, brings along strict typing. Hence, while assigning values to variables, it’s crucial to ascertain that the variable data type matches the value being assigned to it.
For instance:
let isDone: boolean = true; isDone();
This will throw the same “expression not callable” error because we declared ‘isDone’ to be of type boolean but are incorrectly trying to use it as a function. Always verify that variables used in the code match their defined types.
3. Check the Scope of Variables and Functions
Make sure your variables or functions exist within the scope from where you’re trying to call them. If they are defined outside the current scope, the TypeScript compiler won’t recognize them.
function outerScope() { let x = 'outer'; function innerScope() { console.log(x); } } innerScope();
In this scenario, attempting to call innerScope() would result in an error because it’s defined within the scope of outerScope(). To fix this issue, make sure you’re calling functions from a valid scope.
As Frederick P. Brooks Jr., an American computer architect, once said, “The programmer, like the poet, works only slightly removed from pure thought-stuff.” One can conclude that rectifying the ‘Not Callable’ Error requires careful attention to detail and examination of your coding logic with the aim of ensuring the proper usage of variable types and scopes.
You could also look further into TypeScript official documentation for more comprehensive guidance on how TypeScript handles functions and variables.
Enhancing Code Efficiency: Actionable Tips to Avoid Type Errors
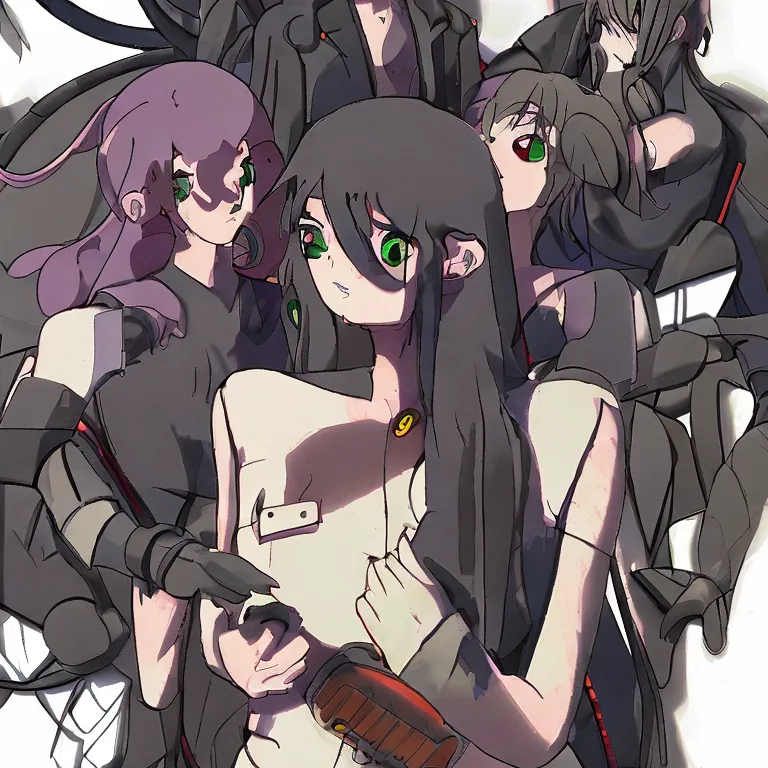
Delving into the world of TypeScript, a statically typed superset of JavaScript that compiles down to vanilla JavaScript, we often come across a common type error: `This expression is not callable. Type ‘number’ has no call signatures.` This error arises when we inadvertently treat a variable of numeric type as a function and try to invoke it. The essence of this issue boils down to understanding Typescript’s precise type checking system and how we can enhance our handling practices to ensure code efficiency.
A key step towards that direction involves more robust type checking habits. To illustrate, assume we have the following TypeScript code:
html
let testNum = 0; testNum();
Executing this would raise the `This expression is not callable. Type ‘number’ has no call signatures` error due to the fact that `testNum` is a number and numbers are not functions, hence they cannot be called like one. Therefore, to avoid such complications, the inclusion of strict typing is an essential strategy.
Implementing strict typing allows TypeScript to prevent any unintentional type coercion. Compile-time checks, such as ensuring that the types are matching before assignment, can significantly reduce the chances of runtime errors, further enhancing reliability. Hence, for the above scenario, initially asserting that the type of `testNum` should be a function can preclude any probable mix-ups.
html
let testNum: Function = 0; // Error! Type '0' is not assignable to type 'Function'
Interfaces in TypeScript are an excellent tool to effectively define custom types structure. They offer a neat way of defining function types which can be strictly adhered to.
html
interface Callable { (): void; } let testNum: Callable = 0; // Error! Type '0' is not assignable to type 'Callable'.
Furthermore, conditional checks can help vet an object before attempting to call it. Such redundancies are efficient strategies ensuring only callable objects proceed further.
html
if (typeof testNum === 'function') { testNum(); }
Dovetailing this, try-catch blocks can also serve as robust constructs in trapping such errors and supplying a meaningful context to the issue.
html
try { testNum(); } catch (error) { console.error("Invalid function call attempted", error); }
As Donald Knuth, a renowned computer scientist once quoted – “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil.” It is evident that focusing on code correctness first and then slowly moving towards optimizations aids in avoiding runtime headaches like type errors thereby making development more streamlined and efficient.
Conclusion
The “Expression not Callable: Type Number has no call signatures” error arises when an attempt is made in TypeScript to execute a number as if it was a function. This antipattern is often the outcome of a misconception or misinterpretation of data types. It’s essential to comprehend that numbers, in contrast to functions, are not ‘callable’ in most programming languages, and TypeScript strictly enforces these type declarations.
Key pointers for understanding involve:
- Understanding data types: Each value in TypeScript must have a specific type, such as string, number, boolean, and others. Numbers aren’t typically “callable”, meaning they cannot be used like functions.
- Type-safe language: TypeScript, being a type-safe language, enforces strict adherence to proper typing and will show errors if a certain action does not match the assigned data type. When you try to invoke a variable that has been identified as a number, TypeScript rightfully indicates this as an error.
- Misconceptions: A common mistake is misunderstanding the flexibility of JavaScript and thinking that any action permissible in JS will also apply in TypeScript. This usually leads to the misconception that seemingly flexible typings would allow actions such as invoking a number.
So how to fix it? Code components could be tweaked with attention given on type definition. Here is what a faulty line of code may look like:
let num: number = 5; num(); // Error - num is not a function.
In order to avoid this error, we shouldn’t attempt to invoke
num
since it isn’t a function. If
num
was intended to be a function that returns a number, the type should instead be specified as:
let num: () => number = () => 5; num(); // This is now valid.
This informs TypeScript that
num
isnt’ a number but a function returning a number.
It’s pertinent to quote Jeff Atwood here, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Bearing in mind the need for both accuracy and understanding can be central to avoiding such errors. After all, our goal as developers should always lean towards creating efficient, error-free, and easy-to-understand code regardless of which language we’re using. [Check more detail](https://www.typescriptlang.org/docs/handbook/basic-types.html).