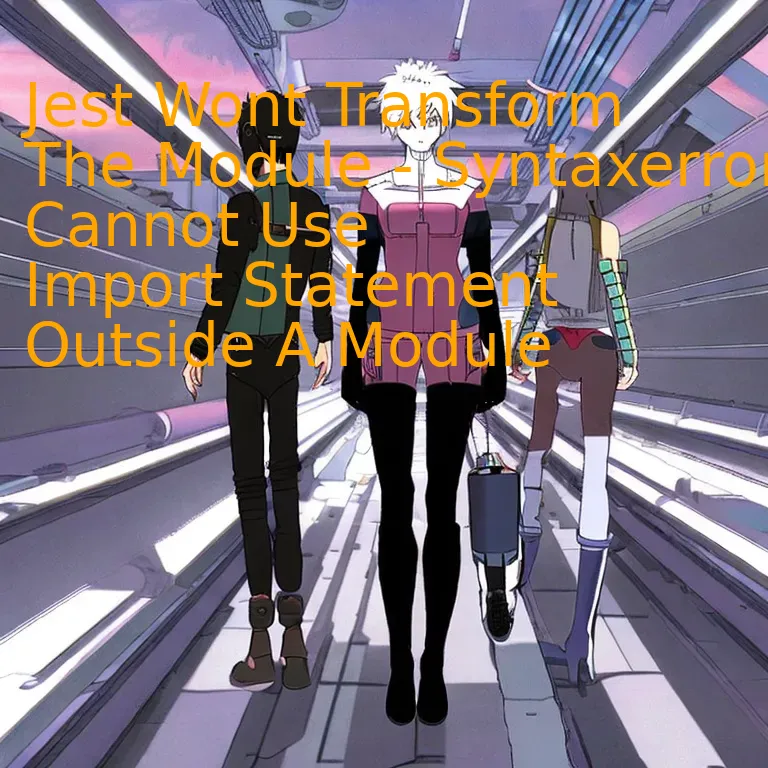
Introduction
When using Jest and you encounter the issue “Syntaxerror: Cannot Use Import Statement Outside A Module”, it’s more than likely that Jest is not transforming your module correctly. This problem occurs when the Jest testing environment does not support ECMAScript modules natively. To overcome this, ensure to update your Babel settings accordingly and use the transformIgnorePatterns in your Jest configuration file, which could help bypass this error.
Quick Summary
The inability of Jest to transform a module, leading to the “SyntaxError: Cannot Use Import Statement Outside A Module”, arises primarily via two channels:
Causes | Explanation |
---|---|
ES Modules | Jest runs in Node.js, and as of now, Node.js does not support ES modules out-of-the-box. ES Modules is the ECMAScript standard for working with modules, which stipulates the use of the ‘import’ statement. |
Babel Configuration | Incorporating Babel can compile ‘import’ statements authors use while writing tests, but if it’s not set up correctly in conjunction with Jest, errors can arise. |
When your code uses ES modules, and you’re running it in a CommonJS compatible environment, consequently, you’ll run into the “SyntaxError: Cannot Use Import Statement Outside A Module” error. This incompatibility occurs because, as Jest runs within a Node.js environment, it doesn’t naturally support ES modules. The support for importing or exporting modules, instead of using Node.js styled `require()` or `module.exports`, isn’t available out-of-the-box.
To solve this problem and add compatibility, we integrate Babel into our project setup. By incorporating Babel, we enable Jest to understand the ‘import’ and ‘export’ keyword by translating them into `require()` and `module.exports`. However, should there be an incorrect setup in Babel in relation to Jest, the SyntaxError will still be prompted.
Updating the Babel configuration would look something like this:
bash
npm install –save-dev @babel/plugin-transform-modules-commonjs
Adding it to the Babel setup would look like this:
{
"presets": ["@babel/preset-env"],
"plugins": ["@babel/plugin-transform-modules-commonjs"]
}
As an aside, while not taking away from the main topic, I’d like to bring your attention to Martin Fowler’s statement: Treat your code as a crime scene.
In a sense, understanding the error messages that your code returns us allows developers to draw the line between what’s expected and what’s actually happening in the codebase – much like how investigators scrutinize every detail at a crime scene.
Properly configured, Babel navigates ES6 syntax smoothly, befitting Jest in a Node.js environment or any other CommonJS compatible environments without prompting the syntax error caused by importing statements outside a module.
Understanding the Jest SyntaxError: Cannot Use Import Statement Outside A Module
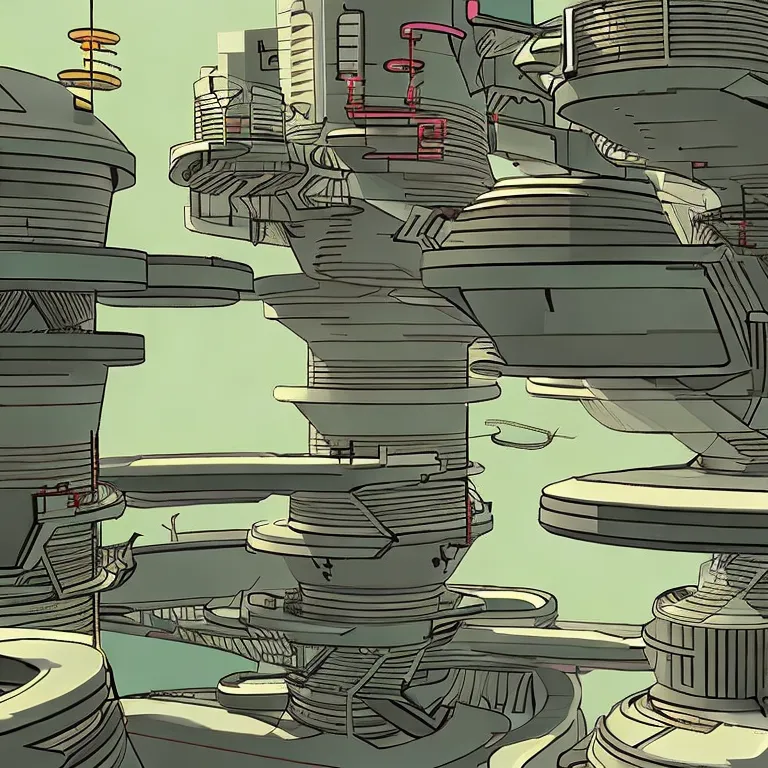
When working with Jest in TypeScript, you may encounter the error “SyntaxError: Cannot use import statement outside a module”. This error typically occurs when Jest tries to parse ES6 modules syntax in your code without proper configuration. Importantly, remember that this challenge directly relates to “Jest won’t transform the module – SyntaxError: Cannot use import statement outside a module”.
Why Does This Error Occur?
The main culprit for this issue lies in JavaScript’s two different types of export/import modules:
– CommonJS (require/module.exports)
– ES6 Modules (import/export)
By default, Jest, when used in a Node.js environment, doesn’t support ES6 import/export syntax natively as Node itself wasn’t supporting ES6 imports until recently.
Fixing The Issue
There are two primary options you can consider when facing this issue.
1. The first involves configuring Babel to transform your code by compiling it down to suitable versions that Jest can understand. Additionally, Babel allows you to utilize ES6 import/export syntax seamlessly. Here’s how you can achieve this solution:
Install Babel and @babel/preset-env:
xxxxxxxxxx
npm install --save-dev @babel/core @babel/preset-env
Define your Babel configuration in a .babelrc file:
xxxxxxxxxx
{
"presets": ["@babel/preset-env"]
}
Next, make sure Jest knows to use Babel by installing a bridge between these two:
xxxxxxxxxx
npm install --save-dev babel-jest
2. The second method would be rewriting your code to use CommonJS syntax. However, this option might not be the best one if you’re working with a larger codebase.
Take into consideration that depending on the framework you’re using, there might be other aspects that need additional configuring, such as TypeScript or React components. Jest configuration can sometimes be tricky and may vary from project to project. This is why providing a universal solution might not be feasible.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler (Martin Fowler). This quote emphasizes the critical point that when configuring environments like this, it’s essential to maintain readability and understandability along with solving the issue at hand.
Resolving Module Transformation Issues in Jest
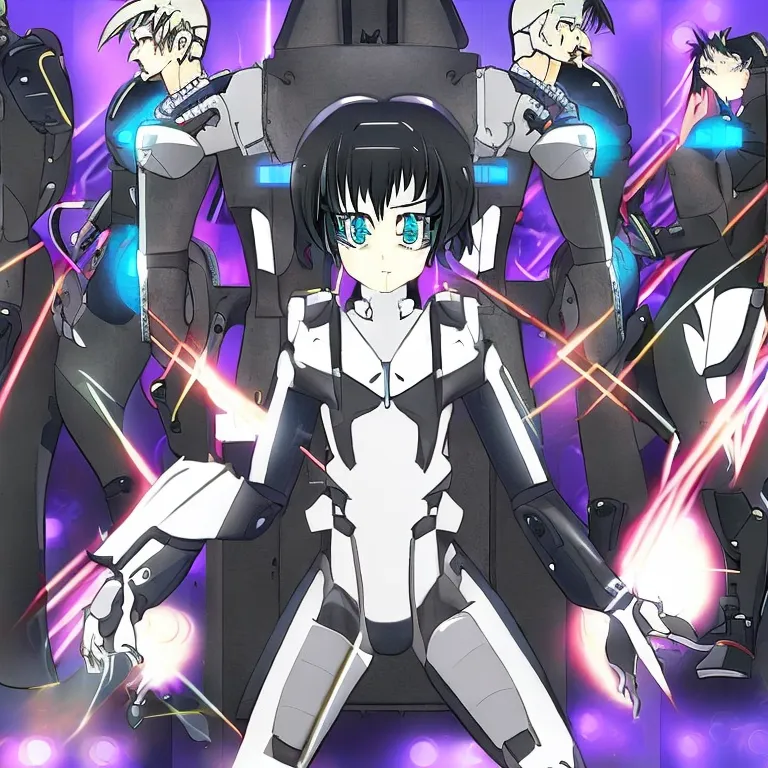
The detection of an error such as
xxxxxxxxxx
SyntaxError: Cannot use import statement outside a module
typically signifies that Jest is not transforming your modules prior to testing. This is a common issue often faced when JavaScript ES6 import/export statements are implemented. To resolve the problem, considerations must orbit around making sure Jest transforms your ES6-style JavaScript before tests are conducted.
1. Configuration of Babel
The utilisation of Babel goes a long way in solving these issues. Babel is a toolchain that transforms code from one source type to another for better compatibility. In most cases, transforming your projects with Babel should solve these problems. To achieve this, ensure {jsx=true} is included in
xxxxxxxxxx
.babelrc
. Here’s an example:
{
“presets”: [“@babel/preset-env”, “@babel/preset-react”]
}
2. Jest Configuration
At times, an improper or incomplete Jest configuration could lead to issues. Ideally, you want Jest to compile ES6 import/exports on the fly so dynamic imports and exports can occur at runtime. Your Jest configuration should look like this:
module.exports = {
transform: {
“^.+\\.(t|j)sx?$”: “babel-jest”
},
};
3. Package Dependencies
Furthermore, assuring all relevant dependencies are properly installed is vital. You’ll need the core `jest` package and `babel-jest`, alongside presets such as `@babel/preset-env` for compiling modern JavaScript down to ES5 and `@babel/preset-react` for React components if used.
As Bill Joy, a pioneering figure in computer science once said, “Innovation is not about saying yes to everything. It’s about saying NO to all but the most crucial features.” This piece of wisdom reflects in resolving the SyntaxError. It entails simplifying your project’s structure, slicing through the overcrowded tech-stack, and focusing on the necessary transformations for proper Jest implementation.
Remember, resolving module transformation issues in Jest requires careful examination of your Babel configuration, Jest setup, and installed dependencies. By ensuring these are all aligned with the overall objectives of your project, you’ll be well-placed to eliminate this error. For further reading regarding the topic check out [Jest Documentation](https://jestjs.io/docs/en/getting-started).
Exploring Solutions to the ‘Cannot Use Import Statement Outside a Module’ Error in Jest
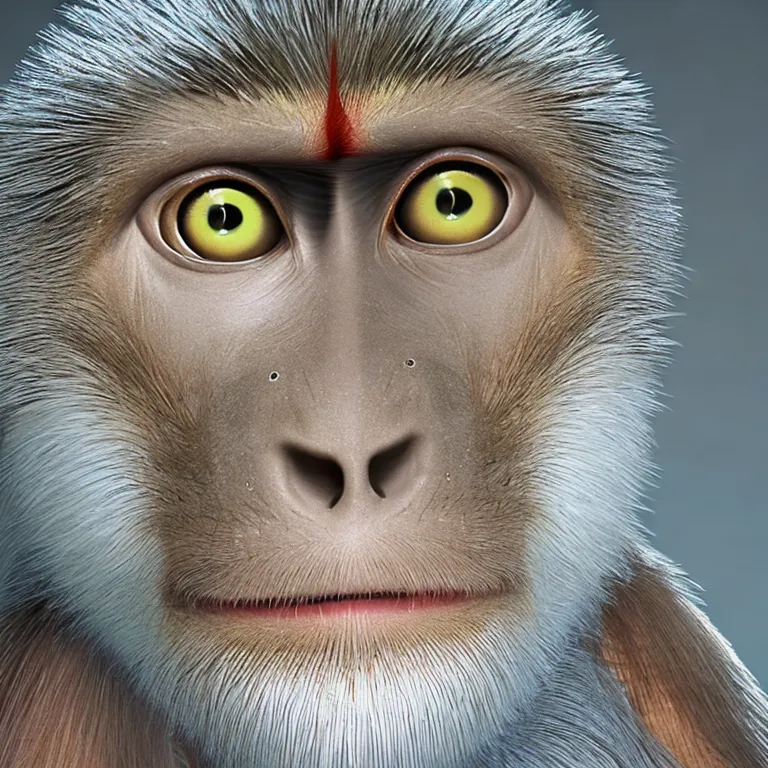
The error “Cannot Use Import Statement Outside a Module” typically arises due to the fact that Node.js does not support ES6 import/export syntax yet, by default. Jest, a delightful testing framework primarily aimed at simplifying and enhancing JavaScript testing workflows, runs in a Node environment, therefore it follows this limitation.
At the core of the mentioned issue in relation to Jest refusing to transform the module is the requirement for appropriate configuration settings. Essentially, you need to instruct Jest as well as Babel (a JavaScript compiler frequently used with Jest) on how they should handle ES6 syntax.
Here’s a walkthrough of the solution:
Step 1: Install Babel and its presets
Ensure Babel is integrated into your project. This can be done using npm, the standard package manager for Node.js as shown below:
xxxxxxxxxx
npm install --save-dev @babel/core @babel/preset-env
Also, create a file called babel.config.js in your project root folder and add the following lines of codes to the file:
xxxxxxxxxx
module.exports = {
presets: [
[
"@babel/preset-env",
{
targets: {
node: "current"
}
}
]
]
};
The code above includes “preset-env”, which allows Babel to transform ES6+ and attach polyfills automatically based on the target browsers or platforms specified. The “node: current” setting here ensures Babel compiles JavaScript to match the version of Node.js currently running.
Step 2: Configure Jest
Thereafter, configure Jest via jest.config.js. If it doesn’t exist, create one at the root of your project. Add the “transform” attribute to define how Jest should transform code from one format to another – in this case, from ES6 to CommonJS.
xxxxxxxxxx
module.exports = {
transform: {
'^.+\\.jsx?$': 'babel-jest'
}
};
This setup tells Jest to use Babel for any files it sees ending in .js or .jsx.
Step 3: Avoid transpiling node_modules
In some instances, the error might still persist. One common reason is that Jest attempts to transpile files in the node_modules directory, which doesn’t need to happen. To resolve this, you can add a “transformIgnorePatterns” attribute to jest.config.js:
xxxxxxxxxx
module.exports = {
transform: {
'^.+\\.jsx?$': 'babel-jest'
},
transformIgnorePatterns: ['/node_modules/']
};
This tells Jest not to apply Babel transformations to anything in the node_modules directory.
As Ken Thompson, co-inventor of Unix and a prominent figure in computer science aptly said, “When in doubt, use brute force.” In context, sometimes the straightforward solution like configuring Babel and Jest properly could solve what seems to be a complex problem.
Practical Tips for Dealing with Jest’s Inability to Transform Modules
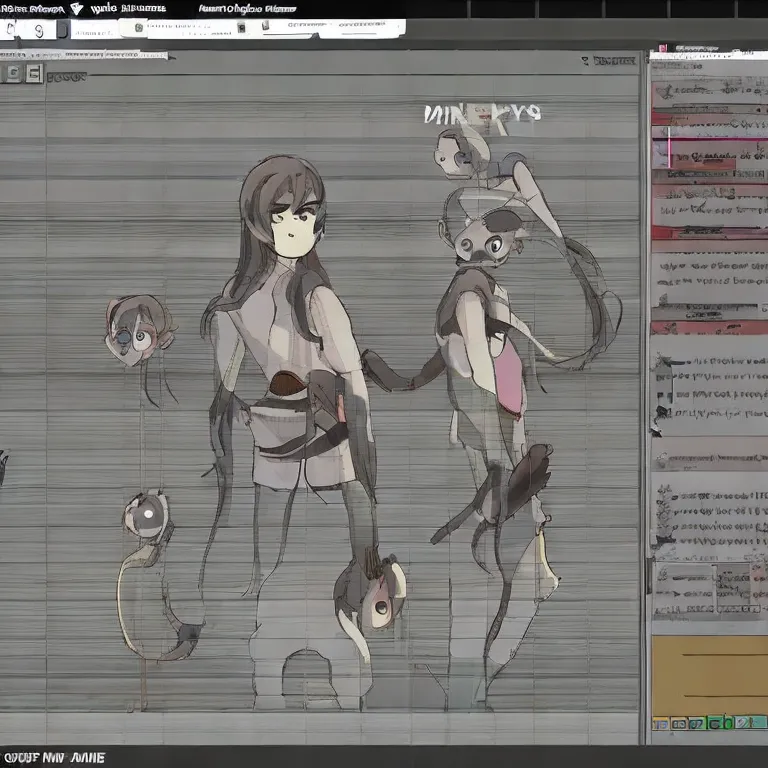
If you’re experiencing issues with Jest and encounter the error message “SyntaxError: Cannot use import statement outside a module”, it’s likely due to one of two probable reasons:
1. Improper Babel Configuration:
Babel is an essential tool for any TypeScript-based project, providing transpilation capabilities which allow developers to utilize new JavaScript features earlier than browser or Node.js support. However, if not correctly configured, Babel may not transform your import statements as needed, hence causing Jest to throw the syntax error.
To rectify this issue, ensure your ‘babel.config.js’ file is equipped to handle ES modules:
xxxxxxxxxx
module.exports = {
presets: [
['@babel/preset-env', { targets: { node: 'current' } }],
'@babel/preset-typescript',
],
};
If such babel configuration doesn’t already exist in your project, add it.
2. Untransformed Node Modules:
Jest has a default behavior where it does not transform anything in the ‘node_modules’ directory. So, when Jest encounters an import statement in a node_module, it throws the SyntaxError. If you have ESM modules in your dependencies, Jest will fail to run these tests [(Source)](https://stackoverflow.com/questions/58613492/how-to-fix-jest-syntaxerror-cannot-use-import-statement-outside-a-module).
To fix this behaviour, you can modify your ‘jest.config.js’ file and specifically include those dependencies that need to be transformed using ‘transformIgnorePatterns’:
xxxxxxxxxx
module.exports = {
transformIgnorePatterns: [
'/node_modules/(?!your-esm-package)',
],
};
Replace ‘your-esm-package’ with the package which contains ESM imports. This tells Jest to transform the ESM packages while leaving the rest of ‘node_modules’ alone. Multiple modules can be included by separating them with a ‘|’, for example ‘(?!module1|module2)’.
“Understanding your tools can make a world of difference when encountering issues, never stop digging until you’re comfortable with every bit and piece of the stack you work with.” -Brian Chan
By understanding your tools such as Jest and Babel, and their configurations properly, it is much easier to avoid or resolve such errors and improve your overall TypeScript-based development experience.
Conclusion
The issue you’re encountering relates to the notorious “Syntaxerror: Cannot Use Import Statement Outside A Module” when utilizing Jest. The crux of such a predicament is fundamentally rooted in how Jest orchestrates module transformations, or more specifically, the occasional lapses therein.
Inherently, when employing ES6 import syntax in Node.js—where by and large depends on the commonJS module system—misalignments are destined to occur. Node.js needs us to harness
xxxxxxxxxx
require()
statements over
xxxxxxxxxx
import
, a tenet not rigidly applied by some codes along their transpilation journey.
Now, assistive as it aims to be, Babel steps in anticipating to seamlessly commute your ES6 syntax into a consolidated version. However, this can further trigger the lamenting “Cannot Use Import Statement Outside A Module” error with a mock file configured for Jest testing. To put it succinctly, Babel may orchestrate an insufficient transformation of modules for Jest admittance.
One plausible solution might involve a direct intervention in your Jest configuration:
xxxxxxxxxx
module.exports = {
transformIgnorePatterns: ["node_modules/(?!(your-node-module)/)"],
}
Essentially, this snippet aids in explicitly instructing Jest which modules require transformation, protecting against inadvertent bypassing.
Additionally, a comprehensive revision and optimization of your Babel configuration settings may streamline future operations.
Remember what Roy Osherove, author of “The Art of Unit Testing,” once said, “Tests are stories we tell the next generation of programmers on a project. A good test is one that tells a story.” Consequently, engage Jest with patience and precision, ensuring each script has its narrative, contributing to your application’s broader saga.