
Introduction
To select specific columns with the Typeorm Querybuilder, carefully pinpoint your desired columns first and then utilize the ‘select’ method to efficiently tailor your database interaction.
Quick Summary
Typeorm Querybuilder allows developers to perform SQL-like queries in a more programmatic and type-safe manner. One common requirement when building applications or APIs is to fetch specific columns from a table while excluding others for reasons such as performance optimization, data privacy, etc. In Typeorm Querybuilder, one can achieve this by making use of the `select` method which accepts array of column names to be fetched.
Consider we have an entity called User defined with multiple columns.
@Entity('user')
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@Column()
email: string;
@Column()
password: string;
}
Now, say we need to fetch only ‘id’ and ‘name’ fields for a given user. The operation can be written as follows:
xxxxxxxxxx
const user = await getConnection()
.createQueryBuilder(User, 'user')
.select(['user.id', 'user.name'])
.where('user.id = :id', { id: 1 })
.getOne();
Let’s decode:
– `getConnection`: It establishes the connection to the database.
– `createQueryBuilder`: It starts a new query for the User entity. The second parameter ‘user’ is an alias used for the table.
– `select`: This method is used here with an array input of the specific user fields that are required – ‘user.id’ and ‘user.name’. If no selection is made, all columns will be selected by default.
– `where`: The where clause specifies the condition to be met.
– `getOne`: Returns a single user that fulfills the conditions laid out before.
As per Tim Berners-Lee, “Data is a precious thing and will last longer than the systems themselves.” Precise selection of data using Typeorm Querybuilder is a skilled and efficient approach in data management.
You can explore more about the Typeorm QueryBuilder on
Official Typeorm Documentation.
Understanding Typeorm QueryBuilder: A Deep Dive

xxxxxxxxxx
TypeORM QueryBuilder
is a critical component of TypeORM, one of the most popular Object-Relational Mapping (ORM) frameworks for TypeScript and JavaScript. ORM libraries provide easy-to-use database interactions using object-oriented APIs. Among the features offered by TypeORM’s QueryBuilder, the ability to select specific columns in a database query proves invaluable in fine-tuning your data access.
When dealing with a large database with many tables and columns, often it is neither practicable nor desirable to pull all the information at once. Zooming in on only the crucial data not only saves the data bandwidth but also optimizes the overall application performance. This is where TypeORM’s QueryBuilder’s capability comes handy to select specific columns.
Selecting Specific Columns
To select specific columns from your database table in TypeORM, you can employ the
xxxxxxxxxx
select
function provided by QueryBuilder. The
xxxxxxxxxx
select
function accepts an array of strings as input where each string represents a column name that you wish to fetch.
Let’s consider an example. Assume we have a “users” table with columns “id”, “username”, “email”, and “password”. You want to retrieve only the “id” and “username” columns:
xxxxxxxxxx
const userRepository = getRepository(User);
const users = await userRepository
.createQueryBuilder("user")
.select(["user.id", "user.username"])
.getMany();
In the above code, the alias “user” is used to refer to the “users” table. Using this alias, we identify the columns we desire (“user.id”, “user.username”) within our
xxxxxxxxxx
select
method.
The power granted by TypeORM’s QueryBuilder is evident. You can carve out precisely what you require from the expanse of your database table, thereby boosting application performance and efficiency.
Writer Thoughts
“One important trait that separates excellent from mediocre software is creative refinement: polish, simplicity, elegance.1” Just as he suggests, using tools like TypeORM’s QueryBuilder promotes such refinement. By aiding in the efficient extraction of data, we progress towards creating a robust and optimized application.
1 Quote attributed to Dennis Ritchie, the creator of the C programming language.
Working with Specific Columns in Typeorm QueryBuilder
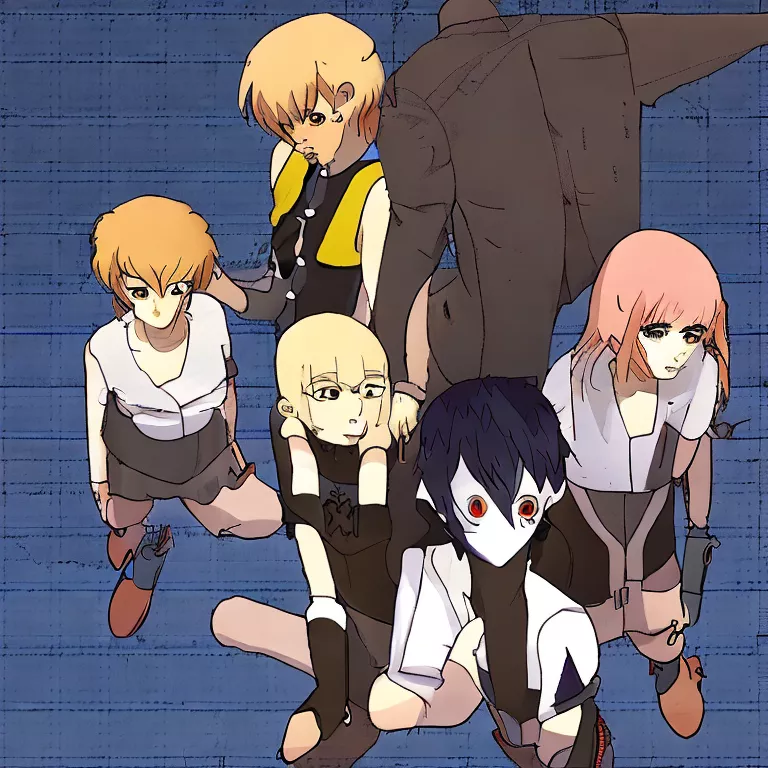
Typeorm, a powerful Object-Relational Mapping (ORM) for Typescript, offers comprehensive tools to interact with databases. One such tool is QueryBuilder, which can help developers fine-tune their queries.
A common requirement for application performance optimization is selecting specific columns from a database table. Instead of fetching all data, which could be processing-intensive, QueryBuilder in Typeorm allows you to specify exactly what you want.
The “select” method comes handy for this purpose. It takes an array of strings representing the column names that should be selected.
Consider the following code snippet:
xxxxxxxxxx
const qb = getRepository(User)
.createQueryBuilder("user")
.select(["user.id", "user.name"])
.getMany();
In this example, ‘id’ and ‘name’ are the only columns selected from the User table. The keyword ‘user’ represents an alias that gives context to the columns we aim to select from.
To make this task easier, Typeorm supports dot notation. So, if you are dealing with relations and need to select columns from related entities, dot notation becomes quite useful.
Here’s another use case:
xxxxxxxxxx
const qb = getRepository(User)
.createQueryBuilder("user")
.leftJoinAndSelect("user.photos", "photo")
.select(["user.id", "photo.url"]) // Selecting relation's specific columns
.getMany();
In this scenario, we join the Photos entity with the User entity using the leftJoinAndSelect method. Then, we elect the ‘id’ from the User table and the ‘url’ from the Photos table.
As mentioned by Bill Gates, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” With this feature, Typeorm seamlessly streamlines database operations by enabling you to select specific columns effortlessly, thereby making technology an integral part of our coding life.
For more detailed and deep-dived information, refer to the official Typeorm Documentation.
The Art and Science of Selecting Columns in Typeorm QueryBuilder
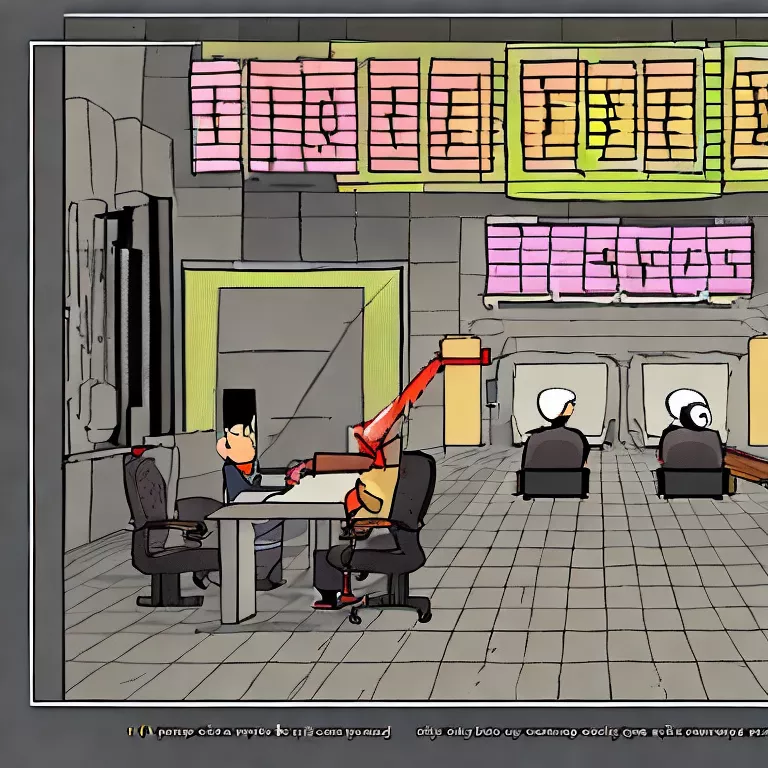
As TypeScript developers, TypeORM’s QueryBuilder provides us with an efficient and secure way to interact with the database in our Node.js applications. One of the most common tasks developers undertake when using this library is retrieving specific columns from a table – a useful feature that enables efficient fetching of data. Let’s delve deeper into how you accomplish this task in a systemic and artful manner.
TypeORM’s QueryBuilder employs the
xxxxxxxxxx
select
method that allows you to specify the precise columns you’re keen on fetching from the database. By defining these columns in your query explicitly, you are able to maintain control over the volume of data pulled from your database and make your application more performant and resource-efficient.
To demonstrate this, consider a typical scenario where you have a
xxxxxxxxxx
Users
entity and you want to fetch only the
xxxxxxxxxx
username
and
xxxxxxxxxx
email
columns. In SQL, this would be akin to:
xxxxxxxxxx
SELECT username, email FROM Users;
In TypeORM, using QueryBuilder, you’d write it like this:
xxxxxxxxxx
createQueryBuilder("user")
.select(["user.username", "user.email"])
.getMany();
Take note of the array passed to the
xxxxxxxxxx
select
method, which is populated by the columns we need. For each column, we need to reference it as it belongs to the “alias” that we’ve assigned to the entity i.e.,
xxxxxxxxxx
"user.username"
,
xxxxxxxxxx
"user.email"
. The alias in this instance is “user” and was assigned in the first argument provided to the
xxxxxxxxxx
createQueryBuilder
function.
As Donald Knuth, esteemed computer scientist stated, “Premature optimization is the root of all evil.” However, when considering the optimized usage of database resources and the overall efficiency of your application, thoughtful selection of your data through precise querying in TypeORM cannot be understated as anything but a well-paced refinement.
All in all, it is apparent the select operation in TypeORM QueryBuilder enables you to harness the optimized retrieval of data. Furthermore, it’s both an art and science – balancing efficient code writing with specific column fetching for operational resource optimization.
The official TypeORM documentation, provides an even more comprehensive overview over this feature and others, worth checking out for any keen TypeScript developer looking to reap full benefits from using TypeORM in their applications.
Advanced Techniques for Column Selection in Typeorm QueryBuilder

Typeorm, a powerful ORM (Object-Relational Mapping) for TypeScript and JavaScript, includes a utility called QueryBuilder which exists to facilitate the creation and execution of SQL queries in a systematic and dynamic way. When you’re working with larger databases and require more granularity over your data selection, advanced techniques for column selection in Typeorm’s QueryBuilder can come into play.
One of these techniques involves using Typeorm’s
xxxxxxxxxx
select
method on an individual field in order to query a specific column. To illustrate, let’s assume that you have a user entity and you are interested only in pulling out each user’s username from the database:
xxxxxxxxxx
// Using the select method
repository.createQueryBuilder('user')
.select('user.username')
.getMany();
Here, ‘user’ functions as an alias for the User entity while
xxxxxxxxxx
.select('user.username')
specifies that only the username column should be selected
Another technique makes use of the subquery functionality within the QueryBuilder. This is useful when you want to display certain columns from one table that are related to columns from another table, in a minimally coupled manner. Here’s how you would achieve that:
xxxxxxxxxx
// Subquery within the QueryBuilder
let subQuery = repository.createQueryBuilder('details')
.select('details.userId')
.where('details.age > :age', { age: 20 });
repository.createQueryBuilder('user')
.where('user.id IN (' + subQuery.getSql() + ')')
.getMany();
In this example, the subquery selects users from the details table where their age is greater than 20. The main query then nests this subquery and pulls user entries from the user table whose ids match those returned by the subquery.
As an advice from Martin Fowler, a renowned software developer and author, “An ORM is like a Swiss Army knife – versatile and capable, but a bad substitute for a scalpel when it comes to performing surgery on your data. Use it judiciously.”
To select specific columns or perform complex queries, Typeorm’s QueryBuilder provides several advanced techniques. The key is using these tools correctly and appropriately based on the specific requirements of your application. And as always in development work, continuous learning and practice are essential for mastering these techniques.[source]
Conclusion
Taking into account the core focus which is, how to select specific columns in Typeorm QueryBuilder, it’s essential to shed light on key points. Typeorm QueryBuilder provides a robust and flexible way for developers to create complex SQL queries that can selectively pull information from databases.
By using the select method in Typeorm QueryBuilder, you’re able to specify the columns that should be retrieved from the database, rather than fetching all data. This can greatly increase the efficiency of your database operations, as it reduces unnecessary data transfer and processing. A basic usage example might look like this:
html
xxxxxxxxxx
const userRepository = getRepository(User);
const user = await userRepository
.createQueryBuilder("user")
.select(["user.name", "user.email"])
.getOne();
Invoking the select command with an array argument gives us the ability to retrieve just the fields of interest (in this case, name and email fields from the User table). Additionally, aliasing our entity allows for cleaner, more manageable code.
Considerations while using this approach include ensuring the selected fields are indeed present in your entity and remembering the syntax – strings in the array should contain both the table alias and column name.
As Bill Gates has been quoted, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” Similarly, adept use of tools like Typeorm QueryBuilder can help streamline your development processes and integrate smoothly into your coding routines.
Further exploration and understanding of Typeorm QueryBuilder will open doors to more powerful querying capabilities, like joining tables, applying conditions, or even complex sub-queries. Online Reference. Ultimately, harnessing the power of Typeorm QueryBuilder and leveraging its functions adequately contributes positively towards the development process and enriches the end-product, making it a skill worth mastering for any dedicated TypeScript developer.