Introduction
In Angular, when an error indicates that a type object is not assignable to type NgIterable
Quick Summary
The `Type Object is not assignable to type NgIterable
Let’s consider this intelligently organized data structure:
Error Component | Description |
Type Error: ‘Object’ | This type represents a non-iterable object value in your application. |
Not Assignable To | A term from the TypeScript language stating that one type cannot be treated or used as another type. |
Type Target: ‘NgIterable<any>’ | Angular uses this type label for iterable objects that can be looped through using Angular directives such as *ngFor. |
The root issue here revolves around what a JavaScript/TypeScript ‘Object’ and an ‘NgIterable
An ‘Object’ represents an instance which contains a set of key-value pairs. It could entail anything you’d like it to: numbers, strings, other objects, functions – even Array. However, despite its flexibility, an Object in itself is not Iterable. This means you cannot directly loop through them like you do with Arrays or Maps, at least not without additional handling.
On the contrary, ‘NgIterable
So quite ostensibly, TypeScript refuses to let an ‘Object’ (non-Iterable) masquerade as an ‘NgIterable
As Tim Berners-Lee, inventor of the World Wide Web, aptly mentioned, “Data is a precious thing and will last longer than the systems themselves.” So it is crucial to ensure that our data types align correctly with the expected output when designing robust systems.
To resolve this error, you need to present your data as an Iterable. If your data originates from an Object, consider using `Object.keys()`, `Object.values()`, or `Object.entries()` methods to turn them into an Array first which is iterable. Then Angular can happily digest it using *ngFor or similar constructs.
An example would be:
let obj = { 'a': 1, 'b': 2, 'c': 3 }; let iterableObj = Object.entries(obj); // Outputs: [['a',1], ['b',2], ['c',3]]
This way, properties of the object can be iterated over since the array follows the ngIterable interface. Understanding such language specific semantics and handling the data accordingly helps in ensuring smooth integration between different parts of our application hence enhancing overall development experience and product quality.
Understanding the “Type Object is Not Assignable to Type NgIterable | Null | Undefined” Error
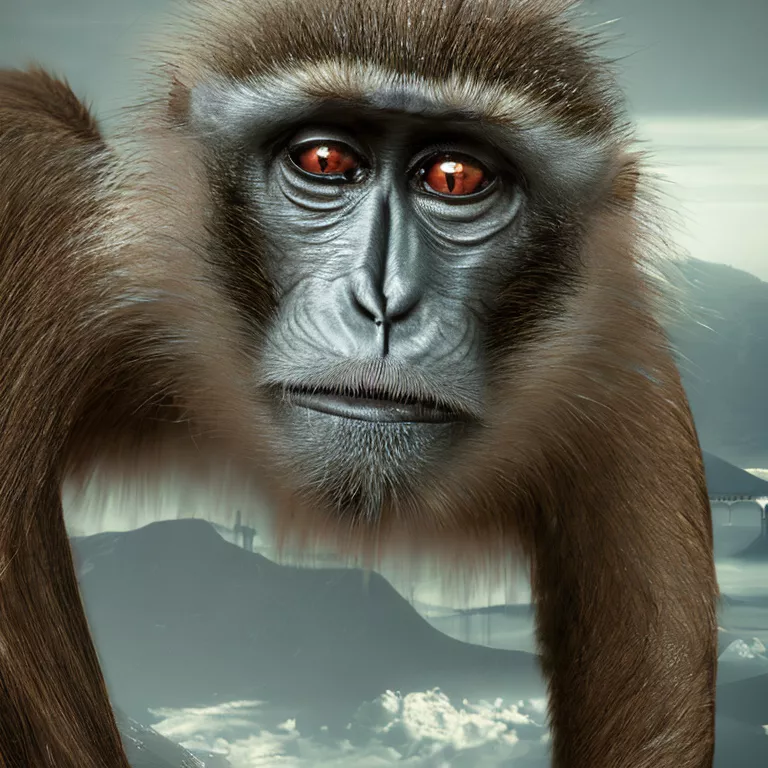
The TypeScript error “Type Object is not assignable to Type `NgIterable
TypeScript
failing to assign a simple JavaScript object to an
Angular
NgIterable object, or cases where it’s trying to handle a non-null and undefined item in an iterable context.”
Exploring Why the Error Emerges:
In angular, the
*ngFor
directive allows for looping through items in an array-like structure, such as:
<div *ngFor="let item of items"></div>
This works exceptionally well when “items” is indeed an array. However, if “items” is a singular update or is considered an object rather than an array-like structure, the TypeScript compiler would produce an error because it conflicts with the expected data type, expecting NgIterable, null, or undefined.
How to Resolve the Issue:
This problem can be resolved by making sure that the variable used in
*ngFor
is an iterable (i.e., array-like) object instead of a plain object. Convert a plain object into an array before trying to iterate over it. For example:
You can create a method in your typescript file:
getArrayOfObject(obj: any){ return Object.keys(obj).map((key)=>{ return {key:key, value:obj[key]}}); }
And then use this in html file :
<div *ngFor="let item of getArrayOfObject(items)"> Key : {{item.key}} , Value : {{item.value}} </div>
So, instead of trying to directly use “items” as an iterable within the
*ngFor
directive, you’re turning it into an appropriate iterable structure beforehand.
As it said by a well-respected technologist Donald Knuth “Programmers waste enormous amounts of time thinking about, or worrying about, the speed of noncritical parts of their programs, and these attempts at efficiency actually have a strong negative impact when debugging and maintenance are considered. We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil.” This quote is particularly relevant here where one small optimization causes such a TypeScript compiler error. It emphasizes the importance of understanding the underlying mechanisms of Angular’s *ngFor directive and TypeScript data types before attempting to optimize.
Debugging Techniques for “Type Object is Not Assignable to Type NgIterable | Null | Undefined”
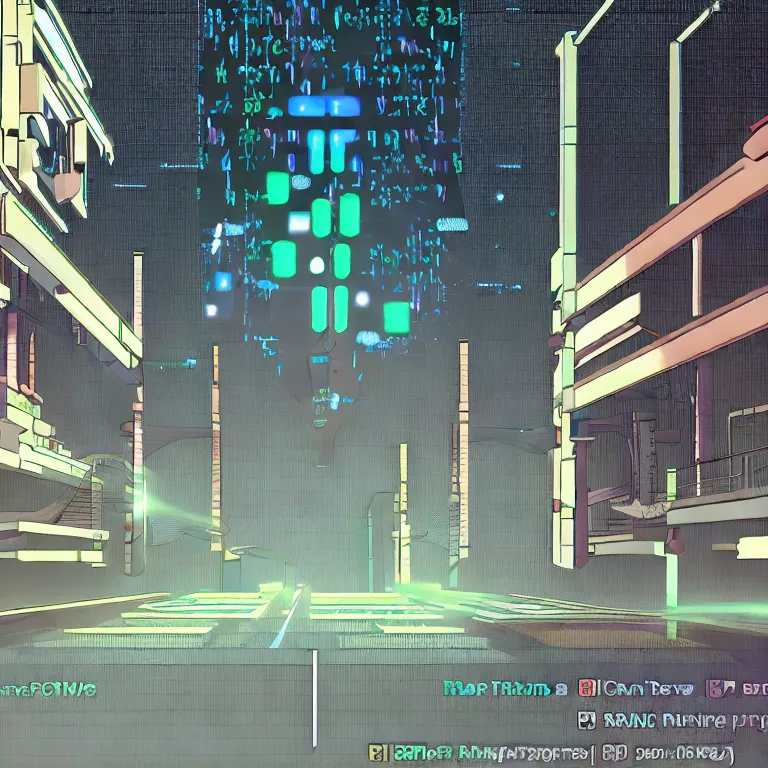
Debugging an error message like “Type Object is not assignable to type NgIterable
Error Message | Cause | Solution |
---|---|---|
Type Object is not assignable to type NgIterable<any> | null | undefined | The variable used with ngFor is an object, but the ngFor requires an array or an iterable. | Convert the object into an array before passing it to the ngFor. |
The first thing to do is Validate Your Data Type. Here are some steps to follow:
1. **Identify the Error Source**: The first step in debugging is knowing exactly where the problem is coming from.
2. **Examine the Variable Data Type**: Use
typeof
to determine if the variable passed to
ngFor
is indeed an object.
3. **Switch to Array Data Structure**: If it turns out the data structure isn’t as needed, consider transforming the objected type to an Iterable type.
let originalObject = {}; let iterableArray = Object.entries(originalObject);
All these steps can help rectify the errors related to “Type Object is Not Assignable to Type: NgIterable
It’s essential to note as Chris Heilmann, a veteran developer and technologist once pointed out, “It’s okay to make mistakes. What’s important is that you learn from them.” As such dealing with complex TypeScript issues like this helps us to learn more and grow as developers.
Implications of Misusing NgIterable in Angular
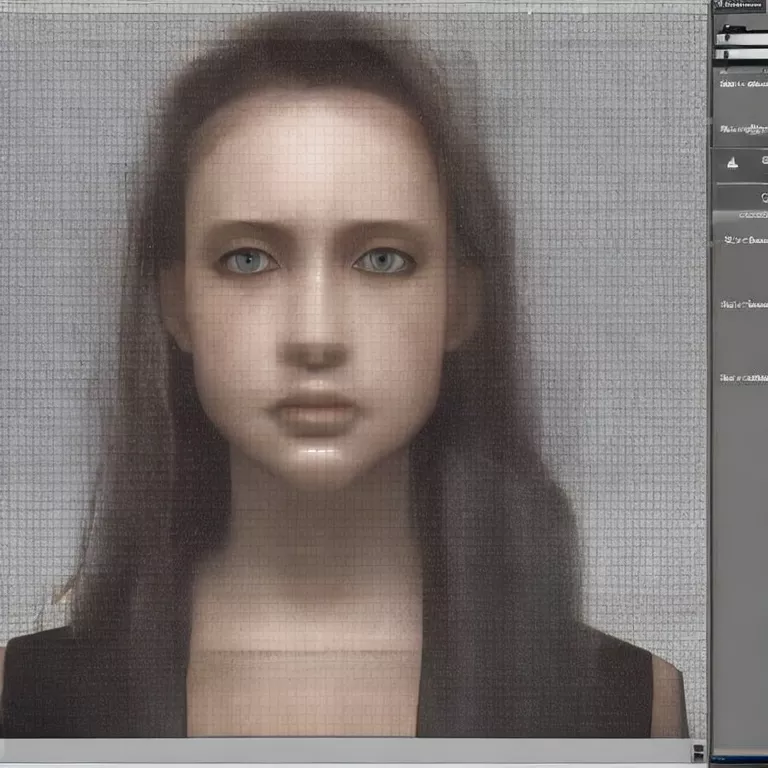
The `NgIterable` interface, along with the type assertion `${type} is not assignable to type \`NgIterable
When encountering this message, it signals that an object is being used where an iterable (an array or similar collection of items) is expected by Angular’s template rendering engine.
Let’s create more clarity around how Angular leverages NgIterable:
•
NgForOf
, one of Angular’s pre-built structural directives works explicitly with NgIterable. It is utilized to iterate over a collection, creating or destroying template instances.
• When providing data to NgForOf, it should be compatible with NgIterable, meaning objects will not execute properly and trigger this error message.
Here is a basic example:
<div *ngFor="let item of items"></div>
Where ‘items’ should be an iterable array, e.g., [1,2,3]. A mistake commonly made is trying to assign an object as shown below:
this.items = { key1: 'value1', key2: 'value2'};
This would result in “type Object is not assignable to type NgIterable
this.items = Object.values({ key1: 'value1', key2: 'value2'});
Improper usage of NgIterable not only interrupts smooth application flow but also impedes development efficiency. Developers spend valuable time troubleshooting problems that could be
avoided with an in-depth understanding of Angular’s template syntax and structures.
As Douglas Crockford, the creator of JSON, stated:
“Programming is the most complicated thing that humans do. Good programming requires an extraordinarily rare combination of talents and a particular kind of psychological profile.”
Achieving mastery over NgIterable and the broader Angular environment is part of this extraordinary talent pool he speaks about, allowing control over your codebase flow and reducing cognitive overhead for Angular developers. Misuse or misunderstanding of NgIterable has far-reaching implications, including unstable application behavior, inefficient debugging, and ultimately impacts developer productivity.
Source
Best Practices for Using NgIterable Effectively
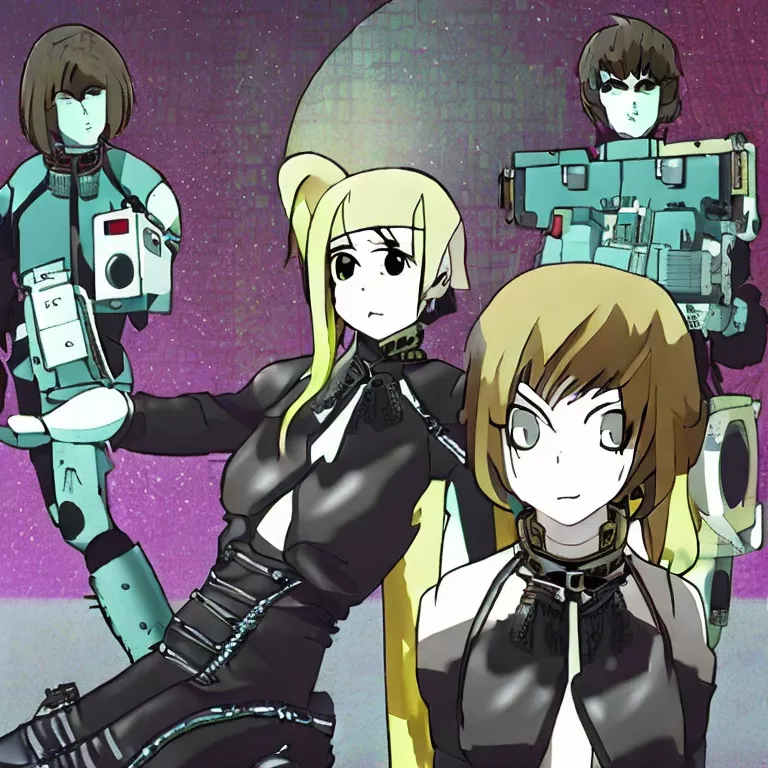
NgIterable
Ensure Proper Data Types are Being Used
If your project throws the error “Type Object is not assignable…”, it means that somewhere within the code base, there’s an attempt to assign an object to an NgIterable variable. Make sure that the variables assigned to NgIterable are indeed arrays or iterable data structures.
For instance, if you have something like:
let myVariable: NgIterable<any> = {};
This would throw an error because myVariable is assigned an object. Instead, it should be something iterable like an array (even if it’s empty):
let myVariable: NgIterable<any> = [];
Strictly Observe Null and Undefined Values
When declaring an NgIterable
Consider this command:
let example: NgIterable<any> | null | undefined;
The command makes ‘example’ perfectly acceptable to Angular, because it constrains the expected type appropriately.
Use TypeScript’s Strict Type Checking Functionality
It’s advisable that TypeScript’s strict type checking functionality is used during the development process. It will significantly save you from unexpected null or undefined errors as it ensures that null and undefined values aren’t used where they’re not supposed to be.
Robust Error Handling & Debugging
Debug the TypeScript code step-by-step to help understand exactly what is being assigned, and throw a customized error when assignment fails. This will make issues easier to identify and fix.
As Steve McConnell famously observed in his book Code Complete: “Good code is its own best documentation.” Consider this investment in error handling as constructing good ‘documentation’ for everyone who might maintain or use your code later on.
Above all, patience and commitment to best practices are key when navigating Type Object Is Not Assignable To Type NgIterable
Conclusion
Understanding the intricacy of the scenario where TypeScript alerts: “Type Object Is Not Assignable To Type NgIterable
let nonIterableObject = {prop1: 'value1', prop2: 'value2'};
Angular’s ngFor directive, only accepts values that are iterable (arrays or sets), null or undefined. Any attempt to bind a non-Iterable object will result in displaying this error.
To resolve this, there are two possible solutions:
– Convert the non-iterable object to an iterable objects such as Array or Set.
– Define a custom iterable for your object.
The step-by-step procedures for these tasks are quite straightforward and can be found in relevant Angular documentation.
A profound quote from Robert C. Martin reminds us that “The only way to go fast is to go well!”. Hence, when using TypeScript with Angular, it’s important to grasp the core principles and potentials, and follow best practices to avoid common pitfalls like the one discussed here. Remember, TypeScript is not just JavaScript with types, but a robust language designed for scalable JavaScript application development that brings static-type checking along with other powerful tools to the table.