Introduction
In comparing Typescript async function return type void with Promise
Quick Summary
In TypeScript, when it comes to asynchronous functions, two commonly used return types are `void` and `Promise
Return Type | Definition | Usage Scenario |
---|---|---|
x Void |
The
xxxxxxxxxx void type in TypeScript indicates the absence of having any type at all. Essentially, xxxxxxxxxx void is a subtype of undefined. |
When an asynchronous function does not return a value, we denote it with
xxxxxxxxxx void . It communicates that the function’s return value does not hold any significant meaning and should not be utilized. |
xxxxxxxxxx Promise<void> |
A
xxxxxxxxxx Promise object represents an eventual completion or failure of an asynchronous operation. xxxxxxxxxx Promise<void> specifically signifies that the promise does not hold a significant return value. |
When our intention is to perform asynchronous operations without caring about the result, we use
xxxxxxxxxx Promise<void> . This enables handling the termination or success of a promise without depending on its value. |
To illustrate these concepts, let’s look at two examples:
1. Async function with `void`:
ts
async function alertUser(): void {
await alert(‘Hello, user!’);
}
In this example, the asynchronous function `alertUser()` uses the `void` return type, declaring that it won’t be returning any meaningful value.
2. Async function with `Promise
ts
function delayedAlert(): Promise
return new Promise
setTimeout(() => {
alert(‘This is a delayed alert!’);
resolve();
}, 3000);
});
}
Here, the function `delayedAlert()` returns a `Promise
Choosing between `void` and `Promise
As famously said by Jeff Atwood, co-founder of Stack Overflow and Discourse: “Any application that can be written in JavaScript, will eventually be written in JavaScript.” Although mentioning JavaScript, it definitely showcases the importance of understanding nuances like function return types also in TypeScript, a superset of JavaScript, to ensure we are writing efficient and readable code.
Understanding Async Functions in TypeScript
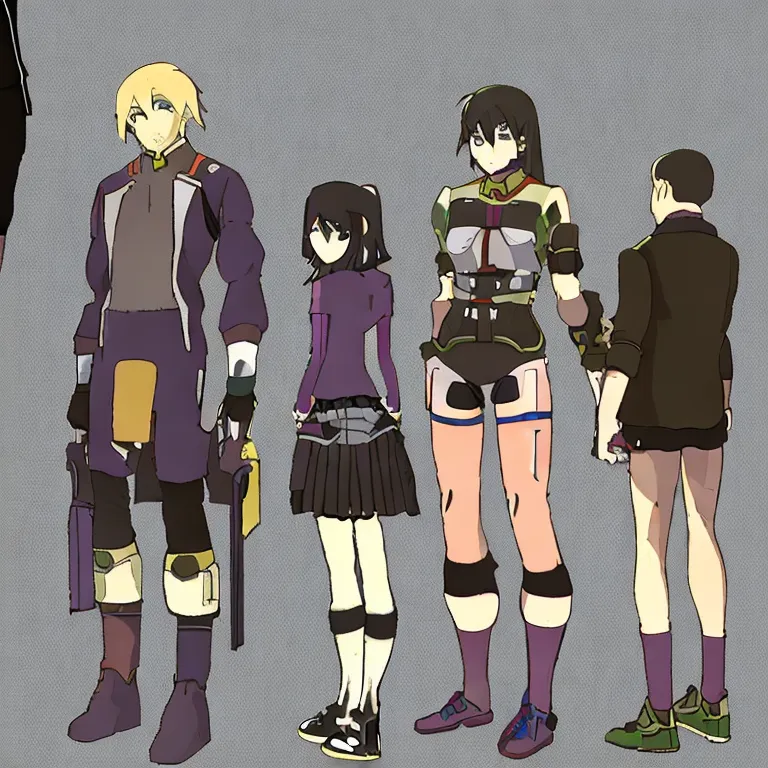
Understanding async functions in TypeScript involves delving into the concepts of asynchronous programming and how TypeScript handles this with its “async” and “await” keywords, particularly concerning return types. Numerous development scenarios require non-blocking operations, which would necessitate a sound understanding of async functions.
When defining an async function in TypeScript, your focus is often on handling Promises more comfortably. You can declare an async function by putting the ‘async’ keyword before a function name. The asynchronous function always returns a Promise, even if you don’t consciously return one from the function body. Now, let’s consider the differences between
xxxxxxxxxx
void
and
xxxxxxxxxx
Promise
as return types for these asynchronous functions.
1) Async Functions with Return Type Void:
When an async function is designed to return ‘void’, it means the Promise doesn’t provide any meaningful value when resolved.
Consider this example:
xxxxxxxxxx
async function fetchData(): void {
const response = await fetch('https://api.example.com/data');
console.log(await response.json());
}
Here, the function ‘fetchData’ retrieves some data using the ‘fetch’ API, logs the returned data to the console but doesn’t return any value.
2) Async Functions with Return Type Promise <Void> :
A function that returns Promise
Consider this slightly modified example of our previous ‘fetchData’ function:
xxxxxxxxxx
async function fetchData(): Promise<void> {
const response = await fetch('https://api.example.com/data');
console.log(await response.json());
}
Once again, ‘fetchData’ doesn’t return any value. However, the declaration now clearly shows that ‘fetchData’ is designed to return a Promise.
So, depending upon your coding style or requirements of your TypeScript project, you can use either ‘void’ or ‘Promise
For an extended understanding of working with asynchronous processes in TypeScript, read more on the official TypeScript documentation page.
Examining the Return Type Void in TypeScript

The return type of an asynchronous function in TypeScript could either be a `void` or a `Promise
When we talk about the `void` type in TypeScript:
– Void is generally said to signify ‘no value’ or ‘non-existence of anything’.
– It indicates that a function does not return a value.
– In Typescript, when void is used as the return type of a function, it suggests that the function doesn’t return any usable value.
Let’s look at a simple example:
typescript
function greet(name: string) : void {
console.log(`Hello, ${name}!`);
}
Here, the ‘greet’ function does not return anything, hence the type is void.
In contrast, when dealing with async functions, the type returned is implicitly set to `Promise
– The main distinction between `void` and `Promise
– A Promise is essentially an object that represents the eventual completion or failure of an asynchronous operation.
For an asynchronous function which does not return anything, you could use `Promise
typescript
async function greetAsync(name: string): Promise
// simulated delay
await new Promise(resolve => setTimeout(resolve, 500));
console.log(`Hello, ${name}!`);
}
It’s essential to note that while the async keyword makes a function return a Promise implicitly, the void return type isn’t truly “void” since it implies a Promise.
As Larry Tesler, renowned computer scientist once stated, “Simple things should be simple, complex things should be possible.” This perfectly encapsulates the difference between `void` and `Promise
Understanding the nuances between these two types can be crucial in creating clean, maintainable code. If you’re writing an asynchronous function that isn’t expected to return a value, using `Promise
For learning more about TypeScript types or Async/Promise patterns, TypeScript Handbook would be an excellent resource to start with.
The Role of Promise in Asynchronous Functions
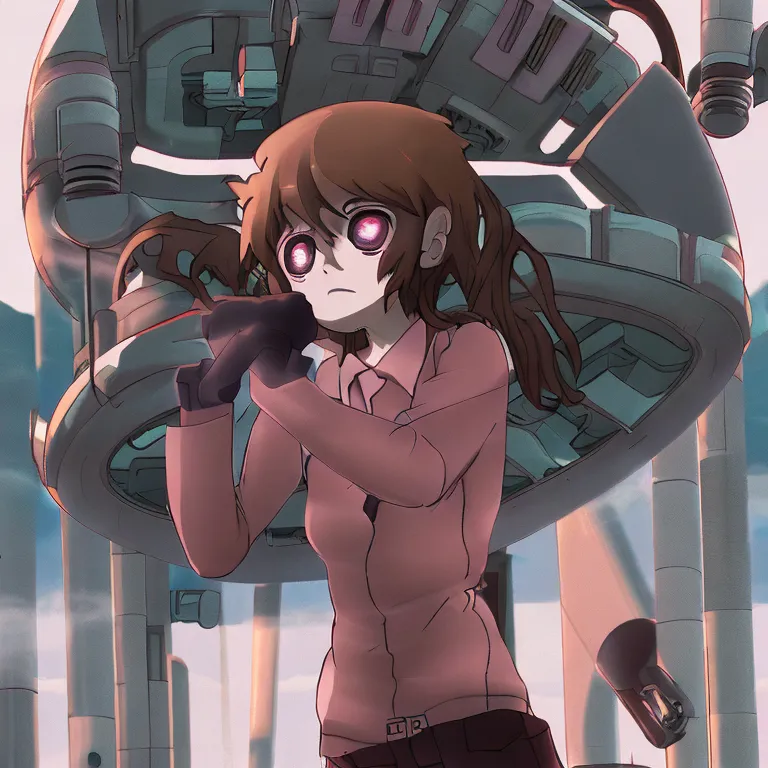
In TypeScript, the role of `Promise
Firstly, understanding what Promise
For instance, consider the following TypeScript async function:
xxxxxxxxxx
async function exampleFunc(): Promise<Void> {
// perform some asynchronous operations
}
Here, `exampleFunc` doesn’t explicitly return a value – what it promises is that it will eventually complete. This is particularly useful when it’s the side effects of the operation you’re interested in, rather than any returned value.
Comparing `void` vs `Promise
xxxxxxxxxx
async function anotherExampleFunc(): void {
// perform some asynchronous operations
}
The function `anotherExampleFunc` automatically wraps its outcome into a `Promise`. Ignoring the `Promise` in the return signature might lead to unexpected results, especially when orchestrating complex asynchronous flows or catching exceptions properly.
The correctness of handling these asynchronous operations can’t be understated. As Tony Hoare, the inventor of the null reference once said, “I call it my billion-dollar mistake…At that time, I was designing the first comprehensive type system for references in an object-oriented language. My goal was to ensure that all use of references should be absolutely safe, with checking performed automatically by the compiler.”
The ‘automatic checking’ Hoare mentions is an important element, and `Promise
For developers intent on avoiding common pitfalls associated with asynchronous operations in TypeScript, adhering to this practice can make a significant difference. Adopting explicit promises in function signatures not only leads to clean, readable code but ensures peace of mind when applications scale and grow complex.
References:
1. [TypeScript Promise](https://www.typescriptlang.org/docs/handbook/release-notes/typescript-1-7.html#async-functions)
2. [Tony Hoare – Null References: The Billion Dollar Mistake](https://www.infoq.com/presentations/Null-References-The-Billion-Dollar-Mistake-Tony-Hoare/)
Comparing and Contrasting: Void Vs. Promise In TypeScript
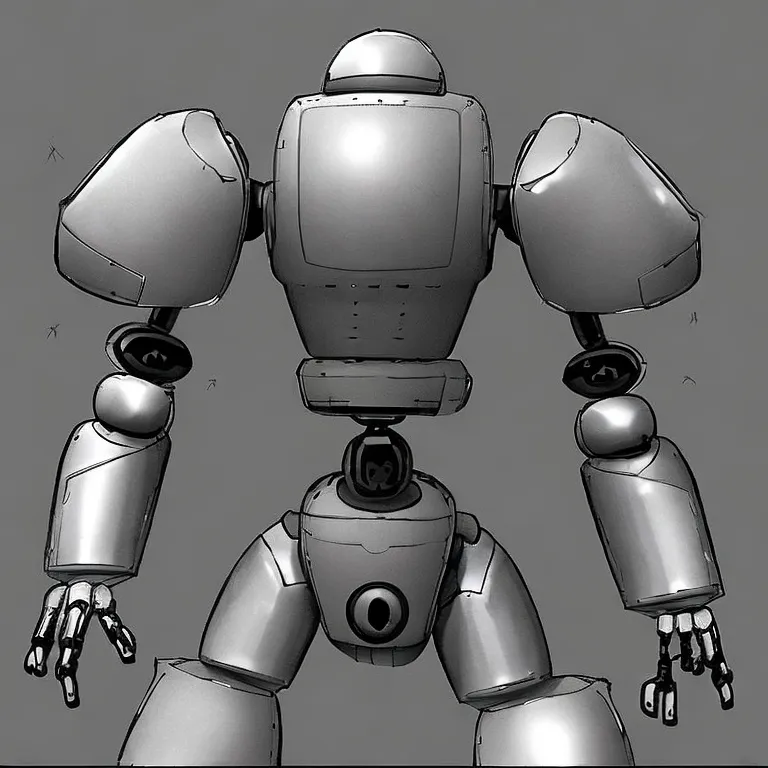
The comparison and contrast of different return types, specifically ‘void’ and ‘Promise
Emphasizing first on ‘void’ type, it is generally associated with a function that does not return a value. The underlying idea while using ‘void’ as a return type is to convey that the primary concern is the side effects of executing the function rather than the yield of it.
On the other hand, ‘Promise
An Example:
Consider an async function that doesn’t return anything. It might look something like this:
xxxxxxxxxx
async function exampleFunction(): Promise {
// Your performative code here
}
In this code snippet, the function ‘exampleFunction’ is denoted as an async function, which returns ‘Promise
Contrasting this with a void return type,
xxxxxxxxxx
function exampleFunction2(): void{
// Your performative code here
}
Here, ‘exampleFunction2’ plainly executes some code without returning anything (no explicit value or promise).
Why Is This Important?
The difference between ‘void’ and ‘Promise
• With ‘Promise
• However, with ‘void’, error handling requires explicit code within the function body to manage possible errors or exceptions.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler
Final Thoughts
As TypeScript provides strict typing, understanding these nuances between ‘void’ and ‘Promise
Source:
TypeScript Handbook – Functions
Conclusion
Understanding the distinction between the return types “void” and “Promise
Referencing
xxxxxxxxxx
void
as a return type means that a function, whether synchronous or asynchronous, isn’t expected to return a value at all. It essentially tell TypeScript developers that they should not use or expect a returned value from this function.
The usage would look like this:
xxxxxxxxxx
async function demoFunction(): void {
await action();
}
However, when you employ
xxxxxxxxxx
Promise
as your async function’s return type, it specifies the same absence of a concrete return value but adds a promise interface that resolves without returning data. In essence, it acknowledges the Promise-based nature of async operations (returning a Promise) while also stipulating that this Promise doesn’t resolve with any useful data.
This could look like:
xxxxxxxxxx
async function demoFunction(): Promise {
await action();
}
Using
xxxxxxxxxx
Promise
over
xxxxxxxxxx
void
offers a clear hint in terms of expectations to other TypeScript developers who may call your async functions. It tells them they can ‘await’ the function and also makes the function then-able. Therefore, if there’s a need to indicate an async function won’t provide meaningful output data,
xxxxxxxxxx
Promise
is especially useful within the context of chained promises and inside async/await syntax structures.
As Martin Fowler expressed, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” The choice between using
xxxxxxxxxx
void
and
xxxxxxxxxx
Promise<void>
comes down to clarity and readability for your fellow developers.