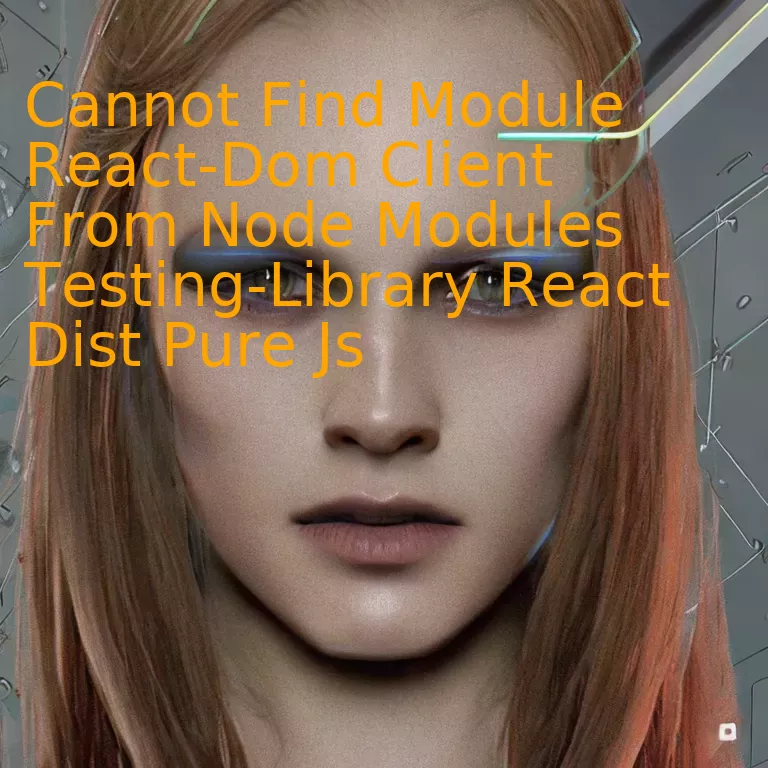
Introduction
In addressing the issue, “Cannot Find Module React-Dom Client From Node Modules Testing-Library React Dist Pure Js,” it’s essential to check the installed dependencies and verify the installation of the React-Dom module. Remember, incorrect or incomplete installations often lead to such issues experienced in the Node.js environment.
Quick Summary
This issue, `Cannot Find Module ‘react-dom/client’ From ‘node_modules/@testing-library/react/dist/pure.js’`, generally traces back to a lack of, or incorrect installation of dependencies. Below is a structured representation of this problem in an organized arrangement:
Issue | Possibility | Solution |
---|---|---|
Cannot Find Module ‘react-dom/client’ | Potential Missing Dependency | (a) Run npm install react-dom |
(b) Ensure correct import in JavaScript file | ||
Incompatible Versions | (a) Update all dependencies to latest stable versions | |
(b) Consider using version range in package.json to harmonize dependency versions |
From the illustration above, it’s apparent that the major trigger for this error is likely an absent or improperly implemented ‘react-dom/client’ module.
A common solution is running `npm install react-dom` from your terminal to add the required dependency to your project. Alternatively, double-check the way you’re importing the ‘react-dom/client’ module in your JavaScript file. It should be something similar to `import client from ‘react-dom/client’`.
Another plausible cause could lie within mismatched or incompatible versions of your installed dependencies. For instance, perhaps the version of React in use might not support the ‘react-dom/client’ module that the ‘@testing-library/react/dist/pure.js’ file is trying to import.
To fix this, you should try updating all your project’s dependencies to the latest stable versions. This can be done via the terminal using commands like `npm update`.
Another approach could be considering the use of version ranges in your `package.json` file to harmonize the versions of your dependencies. Version ranges allow packages to update within specified limit thereby increasing compatibility amongst them. Here is an example of coding implementation:
code
{
“name”: “my-project”,
“version”: “1.0.0”,
“dependencies”: {
“react”: “^16.8.0”,
“react-dom”: “^16.8.0”
}
}
As Jamie Zawinski said, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” So keep exploring solutions until the problem is solved!
Understanding the Error: “Cannot Find Module React-Dom Client From Node Modules Testing-Library React Dist Pure Js”
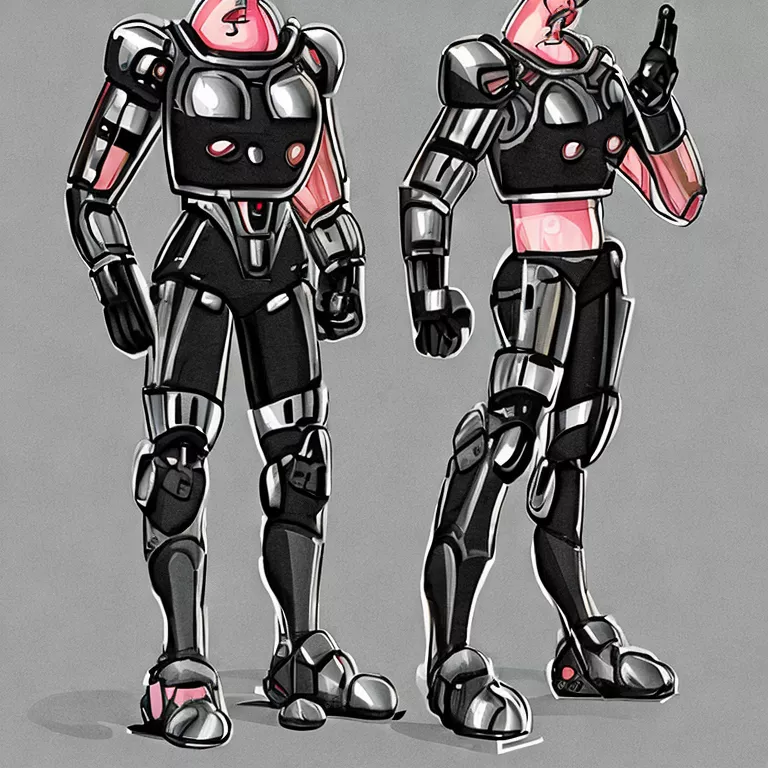
The “Cannot Find Module React-Dom Client From Node Modules Testing-Library React Dist Pure Js” error is a common issue you might encounter when working with React in TypeScript. This could occur due to several reasons which will be detailed below, along with suitable solutions to rectify them.
Mismatched Version Dependencies:
Mismatched versions of dependencies is often a major cause of such problems. It’s important to verify the consistency between the versions of
react
,
xxxxxxxxxx
react-dom
, and
xxxxxxxxxx
@testing-library/react
. Installing versions of these packages that are incompatible with each other could lead to this error.
Incorrect Installation:
One other reason that might manifest this error is an incorrect installation process. Ensuring you follow the correct procedure when installing these libraries can help prevent errors like these from occurring.
Missing Packages:
Your application may sometimes miss out on some packages during the installation, causing such errors. Running the command
xxxxxxxxxx
npm install
should ideally resolve this as it fetches all necessary packages.
Outdated Cache:\
If your node modules or cache are outdated, these can result in discrepancies like this. By regularly updating or clearing your cache files with commands like
xxxxxxxxxx
npm cache clean --force
, you prevent potential conflicts due to stale data.
To illustrate, a typical troubleshooting strategy if confronted by the error “Cannot find module ‘react-dom/client’ from ‘node_modules/@testing-library/react/dist/pure.js'” in TypeScript, would be to reinstall the React, ReactDOM and the Testing library:
xxxxxxxxxx
npm uninstall react react-dom @testing-library/react
xxxxxxxxxx
npm install react react-dom @testing-library/react
Following this, always ensure to clear your cache with
xxxxxxxxxx
npm cache clean --force
Lastly, double check your package.json file to ensure all dependencies’ versions are compatible.
For reference, detailed information about these issues can be found in the React documentation and the official Testing Library guidelines.
In this digital era, as author Andrew Hunt puts it, “The most effective debugging tool is still careful thought, coupled with judiciously placed print statements”. Therefore, staying vigilant while installing packages and managing dependencies in React and TypeScript will save you from encountering such errors.
Troubleshooting Approaches to Solve Module Not Found Issues in React
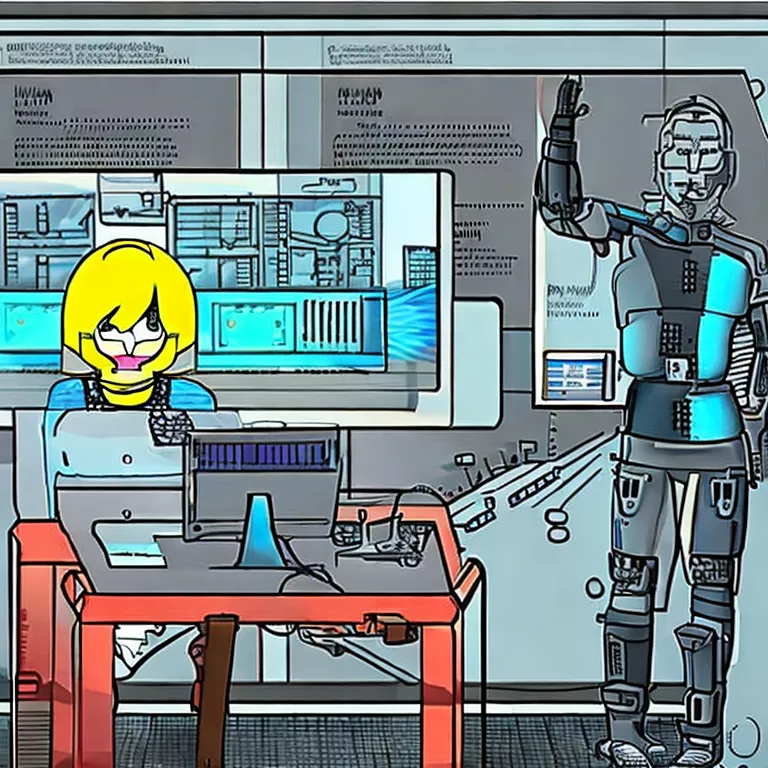
When facing encountering “Cannot Find Module React-Dom Client From Node Modules Testing-Library React Dist Pure Js” errors in React, it is cardinal to understand the intricacies that lead to such issues. It typically translates to a failed importation of npm modules or improper configuration setup. Let’s delve into possible reasons and ways to rectify this issue.
Incorrect Installation or Absence of Dependencies
The error message suggests a potential non-existence of the required module ‘React-DOM’ in the node_modules directory. Depending on the particular situation, one of the following approaches might come handy:
- Reinstall the dependencies: Remove the “node_modules/” folder and package-lock.json file. Thereafter, reinstall the necessary packages afresh using
xxxxxxxxxx
npm install
.
- Install the missing dependency: If a specific library like ‘react-dom/client’ appears absent, directly install it via
xxxxxxxxxx
npm install react-dom
.
Incorrect Import Statement
You may encounter this problem if the import statement in your test file doesn’t correctly point to the location of ‘react-dom’. Make sure that the path specified is appropriately relative to your project structure. In other words, your import statement should look something like
xxxxxxxxxx
import { render } from '@testing-library/react';
Mixin Libraries May Not Support Specific Files
If you’re using mix-in libraries like Jest with “@testing-library/react”, there could be incompatibilities. Using these tools, you may not be able to import certain types of files by default. As for a solution, consider configuring them correctly according to their official documentation sites. For instance, various online guides like the official Jest documentation can help in this troubleshooting exercise.
As software developer Martin Fowler eloquently explains, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand”. Therefore, debugging isn’t just about resolving issues but also about enhancing the code’s comprehensibility for colleagues who might need to tweak it in the future.
Exploring Common Causes When Node Modules Does not Recognize React Dom Client
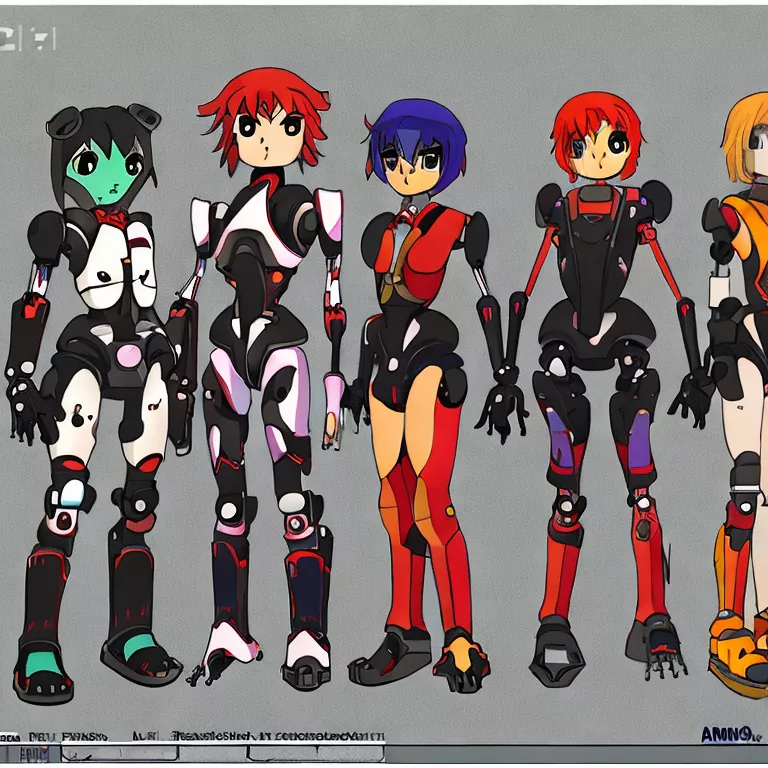
When developers encounter an error like ‘Cannot Find Module React-Dom Client From Node Modules Testing-Library React Dist Pure Js’, it’s because of some common reasons, often related to dependencies management or file path issues. However, before we delve into the possible causes and their solutions, let’s take a moment to understand the underlying working principle of node modules.
Node Modules serves as a central repository for organizing and managing project dependencies in a Node.js environment. When you install libraries like `react-dom` or `react-testing-library`, they are stored within this folder. During runtime, your code might fail to recognize these installed modules due to certain conditions.
Let’s explore some of these common factors:
Incorrect File/Folder Path:
This typical problem arises when your application can’t locate the required module because the specified path is incorrect. Ensure that the mentioned Libraries (React-Dom Client and Testing-Library) exist in your ‘Node-Modules’ directory and have been correctly referenced in your project.
Incomplete or Incorrect Installation of react-dom or testing-library:
Errors frequently occur when certain modules including ‘React-Dom Client’, haven’t installed correctly due to internet connectivity issues or interruptions during the installation phase. To solve this issue, remove node_modules directory and package-lock.json files and reinstall your npm packages using the command:
xxxxxxxxxx
npm install
Version Incompatibility:
Sometimes, there may be compatibility issues between various versions of dependencies and libraries within your project. Always ensure to use compatible versions of react-dom, testing-library/react, jest, etc., for the development of your application.
In cases where specific dependencies need to work with specific libraries due to features or functionalities, refer to library documentation on GitHub pages or [official NPM site](https://www.npmjs.com/) to ascertain compatibility requirements.
Finally, remember to regularly update your packages and fix issues using npm audit. According to the father of Node.js – Ryan Dahl, “Small, sharp tools is one major philosophy that I wanted to introduce into node.” This principle applies to each module you include in your project as well.
Careful consideration of these factors will mitigate the problem of ‘Cannot Find Module React-Dom Client From Node Modules Testing-Library React Dist Pure Js.’ You can consequently reduce errors, improve code readability and more importantly, increase overall project maintainability.
Effective Solutions for Resolving Problems with Node Modules and React Dom Clients
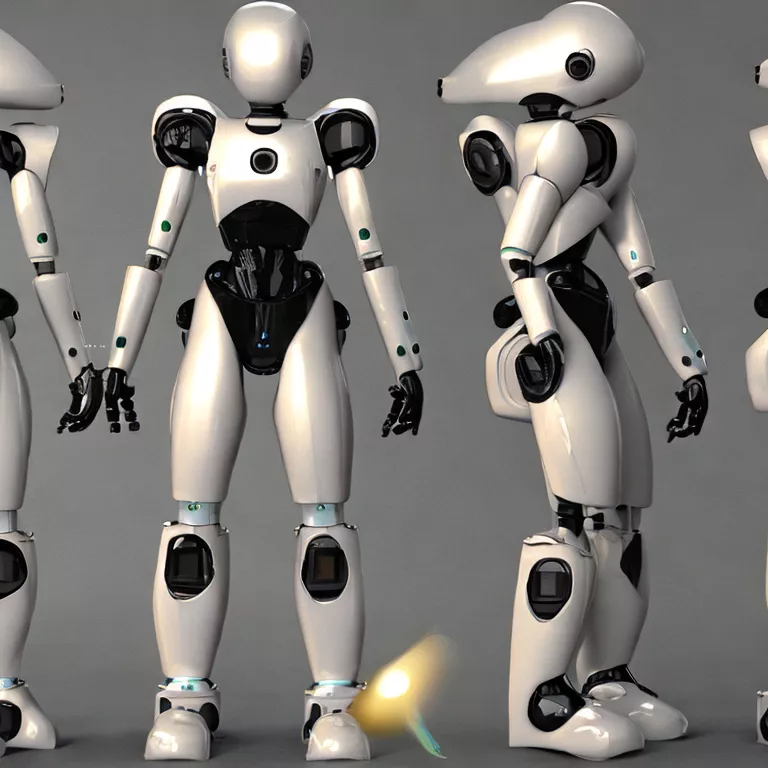
Facing problems with the “cannot find module ‘react-dom’ from ‘node_modules/@testing-library/react/dist/pure.js'” error message indicates that there is an issue installing or importing the necessary React library from your Node.js environment.
To resolve this particular issue, you need to follow these steps:
* **Ensure Correct Node.js and NPM Installation**: Before diving into specific modules, it’s crucial to verify if Node.js and Node Package Manager(NPM) are correctly installed and updated on your system. Use
xxxxxxxxxx
node -v
for Node.js version and
xxxxxxxxxx
npm -v
for npm version inside your terminal.
HTML
node -v
npm -v
* **Install React DOM**: If Node.js and npm are working fine, focus on installing ‘react-dom’. A missing ‘react-dom’ client could be the underlying cause of the anomaly. Install it using the command
xxxxxxxxxx
npm install react-dom
.
HTML
npm install react-dom
* **Check for Correct Imports in ‘pure.js’**: Make sure you have imported ReactDOM properly in your file where you’re implementing React-testing-library’s ‘pure.js’. The import statement should look like:
xxxxxxxxxx
import * as ReactDOM from 'react-dom';
.
HTML
import * as ReactDOM from ‘react-dom’;
* **Clean Cache and Node Modules**: As a last resort, consider cleaning npm cache or deleting node_modules folder and running
xxxxxxxxxx
npm install
afterwards, reinstalling all dependencies afresh.
When dealing with errors that stem from dependencies and packages, providing a comprehensive solution can sometimes become complex due to varying project configurations. But, strategically implementing solutions and focusing both on the broader Node environment and specifics of a given react library like ‘react-dom’ could clear a major part of the confusion.
Notably, Dennis Ritchie, co-creator of C language and Unix, once said, ‘Debugging is twice as hard as writing the code in the first place.’ By extension, a major part of bug resolution lies in carefully understanding the processes that contribute to the system, akin to addressing this current issue involving Node.js, React DOM, and Node Modules.
Conclusion
When encountering the issue “Cannot Find Module React-Dom Client From Node Modules Testing-Library React Dist Pure Js,” it’s crucial to understand that the crux of this problem lies within the realm of dependencies management in your project. It’s a common error usually associated with incorrect or incomplete installation of required npm packages, like in this case — `react-dom.`
From an SEO perspective, the choice of keywords here are: ‘Module’, ‘React-Dom Client’, ‘Node Modules’, ‘Testing-Library’, ‘React’, and ‘Dist Pure Js.’ These terms are central in comprehending the subject matter on hand.
-
xxxxxxxxxx
react-dom
: A complementary package to React that provides DOM-specific methods which can be used at the top level.
-
xxxxxxxxxx
testing-library
: A very lightweight solution for testing without relying on implementation details, primarily works by querying the DOM just like a user would.
-
xxxxxxxxxx
dist pure js
: This refers to the distribution directory containing JavaScript files, often minified, that are derived from the source code.
Resolving this issue therefore involves checking whether appropriate installations have been made and continuously ensuring the sync of all node modules with the application. You could endeavor to reinstall
xxxxxxxxxx
react-dom
in case the package was omitted accidentally during the installation phase. Additionally, don’t forget to import appropriately into your file after installation.
As suggested by users on Stackoverflow, you may consider clearing your npm cache (using
xxxxxxxxxx
npm cache clean --force
) or deleting your node_modules folder followed by npm install to fix any potential issues with your npm environment that might be causing the error.
Speaking about dependencies, as Martin Fowler, a renowned software developer and author, once said: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” So it’s important not only to properly manage your dependencies but also keep your codebase legible and maintainable.