Introduction
When encountering the TS2531 error in Angular reactive forms, it implies that a specified object might be null. This prevalent issue is typically resolved by using optional chaining or ensuring form controls and data bindings are correctly initialized to prevent potential null values.
Quick Summary
An error TS2531 occurs in Angular Reactive Forms when you try to invoke a method or access a property of an object that is possibly null or undefined. Codable insights of Angular Reactive forms indicate that the form control instance can possibly be null while we are trying to access its property or method.
Error Type | Reason | Solution |
---|---|---|
TS2531: Object is possibly ‘null’. | The TypeScript compiler has detected that there may be a path through your code in which this object might not have been initialized, and so it may be null or undefined when you try to use it. | To remove this error, check if the object is defined and not null before proceeding with any operations on it. |
Bowles insists on thoughtful handling of Errors in TypeScript language. As he mentioned, “One of the core principles of TypeScript is to allow developers to write JavaScript that is as close to the intended runtime semantics as possible.” This underlines why meticulous scrutiny of errors like TS2531 is significant for the vitality of a typescript application.
Key elements of Angular Reactive Forms contributing heavily to this error are FormControl, FormGroup, and FormBuilder. If these constructs are mismanaged or overlooked, it reflects upon giving rise to such errors. Situations may arise where these elements mistakenly assume a null state, hence paving the path for TS2531 to occur.
For instance, consider a FormControl:
this.profileForm = new FormGroup({
firstName: new FormControl(''),
});
If you attempt to reference a control that does not exist or is not defined, you would encounter the TS2531 error:
xxxxxxxxxx
console.log(this.profileForm.get('lastName').value); // Error TS2531: Object is possibly 'null'.
To prevent this from happening, you should always ensure the control exists before accessing its value:
xxxxxxxxxx
if(this.profileForm.get('lastName')) {
console.log(this.profileForm.get('lastName').value);
} else {
console.error('Control does not exist');
}
Understanding the common grounds of error generation becomes paramount in efficiently building applications with Angular Reactive Forms. Tailoring techniques through gained intelligence around possible pitfalls helps bridge gaps between TypeScript and runtime semantics. Hence enabling to fully leverage the potential benefits of adopting Angular’s Reactive forms.
Addressing Error Ts2531 in Angular Reactive Forms: Exploring the Root Cause
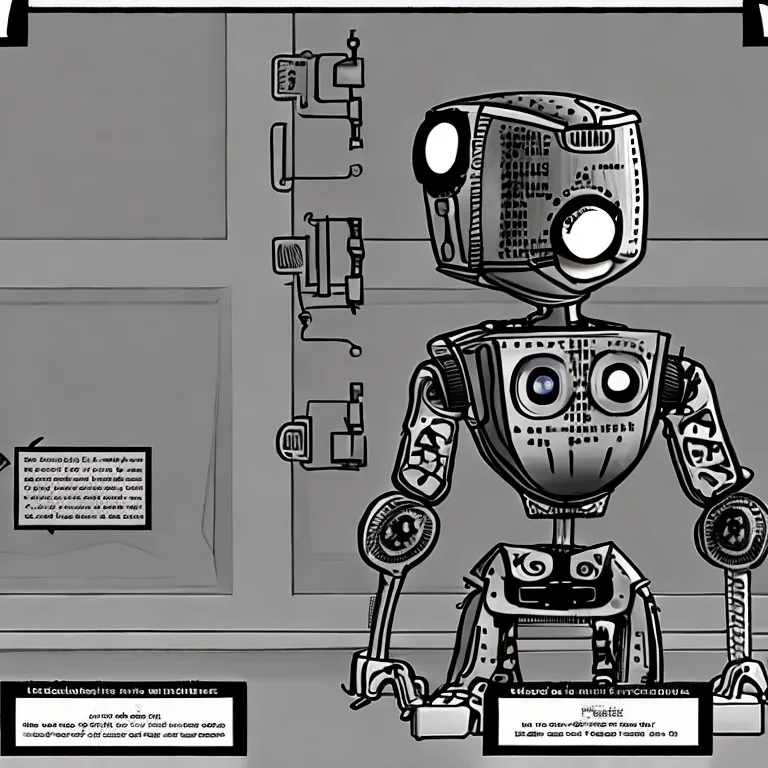
The issue of TS2531 – object is possibly ‘null’ is a prevalent one encountered in Angular’s Reactive Forms. When developing with TypeScript, it’s not uncommon to run into this specific error. This tends to happen because Typescript adheres strictly to object usage and cautions against possible null or undefined value scenarios.
Defining the Issue
Typescript’s TS2531 error occurs when you try to access a property or call a method on an object that might be undefined or null.
When dealing with Angular Reactive forms, you will commonly see the error in instances where form controls are accessed using syntax such as
xxxxxxxxxx
this.myForm.controls['controlName']
or when you directly try accessing controls like so:
xxxxxxxxxx
this.myForm.controlName.value
. According to Typescript, these objects may potentially be null.
Finding the Root Cause
Reactive forms utilize FormGroup, FormControl, and the FormArray classes. In essence, a reactive form comprises a tree of Angular form control objects, which can be nested to any level. A common misconception causing confusion and leading to errors like TS2531 is considering form groups or form controls as Observables because they have value changes as an Observable property. However, they aren’t.
As such, value changes being handled via asynchronous events cause uncertainty in control initialization timing, leading TypeScript to warn you about a possibility of null value assignment, hence the TS2531 error.
Solutions
1. Using The Get() Method:
Instead of directly calling the formControl, using the
xxxxxxxxxx
get()
method is safer and avoids the TS2531 error:
xxxxxxxxxx
this.myForm.get('controlName').value
2. Type Assertion:
Another solution could be TypeScript `non-null assertion operator` post-fixed to an expression, like shown:
xxxxxxxxxx
this.myForm.controls['controlName'].value!
3. The Optional Chaining:
With TypeScript 3.7 and above, optional chaining can handle undefined and null cases straightforwardly:
xxxxxxxxxx
this.myForm?.controls['controlName']?.value
Depending on your development preferences and the complexity of the forms being handled, you can choose any method that best fulfills the requirements without raising any TS2531 errors.
It is crucial to remember what Anders Hejlsberg, the lead architect of C#, once said,
“The key to performance is elegance, not battalions of special cases.”
By identifying the root cause and understanding its implications, it becomes easier to create elegant solutions for TS2531 error handling in Angular Reactive Forms. It improves your code’s robustness and reliability without needing unusual workarounds or excessive exception handling blocks.
Reference:[TypeScript 2.0: Null, Undefined, and Strict Null Checks ](https://blog.mariusschulz.com/2016/09/27/typescript-2-0-null-undefined-and-strict-null-checks)
Cracking Down on Null Objects: Tips for Troubleshooting Error Ts2531
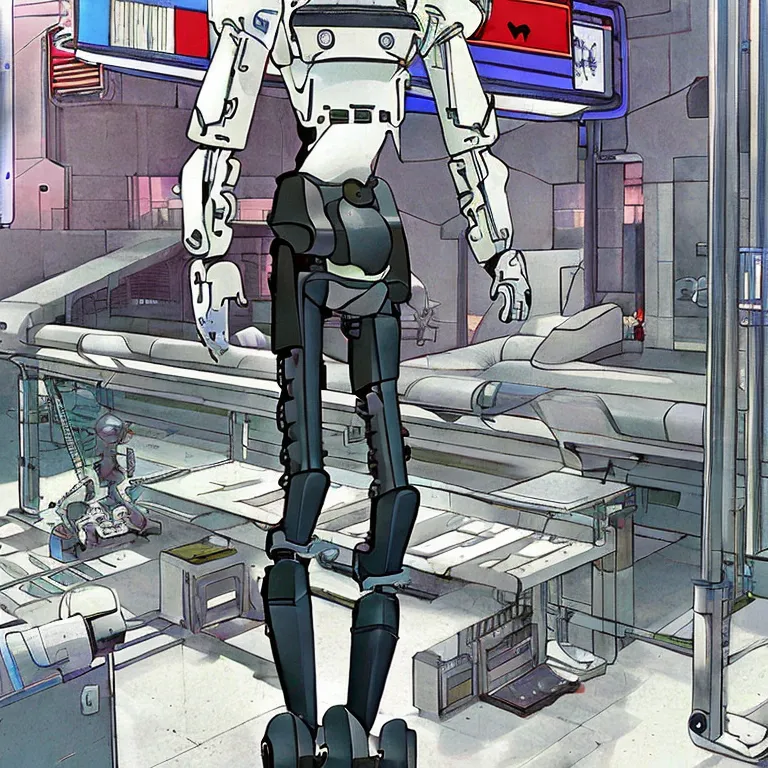
The TypeScript error TS2531, which denotes “Object is possibly ‘null'”, often occurs when an object hasn’t been instantiated or has been set to null and you are trying to access its properties or methods from it, lacking a sufficient null safety check. This error is a common occurrence in Angular Reactive Forms.
The primary cause of this error in Angular Reactive Form is attempting to access form controls property that may not be instantiated at the time of accessing. When dealing with reactive forms in Angular, form control instances (FormControl, FormGroup, etc.) are attached to HTML elements by defining them in your component classes.
One of the most typical examples is when you try to access a FormControl value before the FormControl itself is set or initialized causing TypeScript to throw Error TS2531.
For instance, consider the following snippet:
xxxxxxxxxx
formGroup!: FormGroup;
ngOnInit() {
this.createForm();
}
createForm() {
this.formGroup = this.formBuilder.group({
name: [''],
});
}
And let’s say we have HTML tying to this TS like so:
xxxxxxxxxx
<input [formControl]="formGroup.controls.name" placeholder="Name">
The error message TS2531 will be thrown if Angular attempts to resolve
xxxxxxxxxx
formGroup.controls.name
while
xxxxxxxxxx
formGroup
is potentially null. This is because
xxxxxxxxxx
formGroup
gets created after the view has been initialized owing to how Angular life cycle runs.
Luckily, TypeScript provides ways to affirm the non-nullability of an expression. To resolve the issue, you can employ one of the following strategies:
1. **Use the Safe Navigation Operator (?.)**
The Safe Navigation operator allows you to navigate through the uncertain type. You can compactly express a chain of navigation steps for properties of objects which might possibly be undefined or null.
xxxxxxxxxx
<input [formControl]="formGroup?.controls?.name" placeholder="Name">
2. **Use Non-null Assertion Operator (!)**
In cases where you are certain the value is not null, you can use Non-null assertion operator (postfix !), which tells TypeScript to remove null and undefined from the type of what it operates on. However, you need to be sure that the value will not be null when accessed.
xxxxxxxxxx
this.formGroup!.controls.name
These methods ensure you have safe referencing of objects that could potentially be null at some point in their existence leading to compiling code without TS2531 error.
Angular Reactive Forms are powerful tools for building forms with complex validation and transformation logic. Understanding the potential pitfalls and how to handle types appropriately is key to avoid frustrating runtime errors.
As quoted by Joshua Bloch: “APIs, like UIs, should be designed based upon a deep understanding of the needs and wants of its users”. This underscores the importance of handling these kinds of callback assurances properly. As developers, it’s vital we put ourselves in the shoes of our users or rather ‘think in code’ to write robust applications leading to greater user satisfaction and least potential runtime exceptions.
Advanced Strategies for Resolving Object-Null Errors in Angular Reactive Forms
Angular Reactive forms provide a way to handle form inputs and determine their validness. However, developers may encounter the TS2531 error with the message: “Object is possibly ‘null'”. This error surfaces when TypeScript’s strict null checks feature is enabled, which prohibits undefined or null from being assigned to a variable unless explicitly declared.
Let’s delve into some advanced strategies that can help rectify this issue in Angular Reactive Forms:
- Use Optional Chaining: The optional chaining operator ‘?’ can be used to access deeply nested property paths without having to validate each intermediate step against null and undefined values. Here’s an example for illustrating how it works:
xxxxxxxxxx
// Without optional chaining
let length = a && a.b && a.b.length;
// With optional chaining
let length = a?.b?.length;
- Leverage the Non-Null Assertion Operator (!): If you have affirmed that a value will never be undefined or null, consider using the non-null assertion operator(!). It informs TypeScript compiler that the expression cannot evaluate to null or undefined. Bear in mind that overuse can lead to undetected runtime errors. Let’s exemplify its usage:
xxxxxxxxxx
//Initial Value possibly null or undefined
let exampleValue = document.getElementById('exampleId');
//After applying Non-Null Assertion Operator - TypeScript Compiler assumes it's never null or undefined.
let element = exampleValue!.Value;
- Type Assertion: Another suitable strategy is Type Assertion. It comes into play when you know more about a certain value than TypeScript itself. TypeScript allows us to assert the value to a more specific type to bypass the error. An example of its usage would be:
xxxxxxxxxx
let formValue = <HTMLInputElement>document.getElementById('exampleId');
console.log(formValue.value);
As Bill Gates once said, “Error messages punish people for not behaving like machines. It is time we let people behave like people. When a problem arises, we should call it a bug, not a human error.” Therefore, understand the nature of these errors and apply appropriate solutions instead of getting frustrated.
For detailed insights on handling null or undefined in Angular Reactive forms, consider checking this official documentation on TypeScript Configuration: Strict Null Checks.
A Deep Dive into TS2531 Object Nullity Issues within Angular’s Reactive Forms
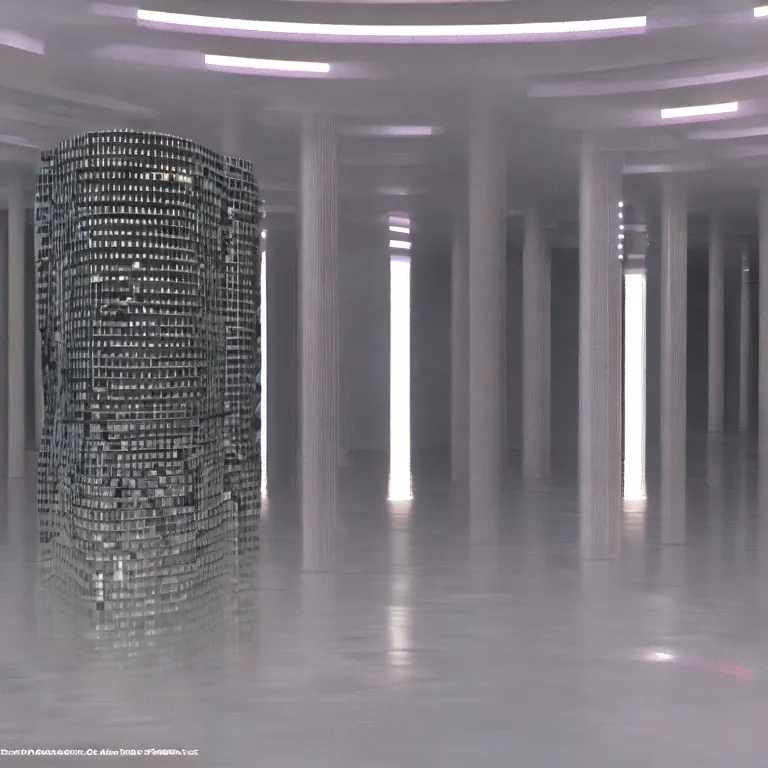
When working with Angular’s Reactive Forms there may occur an error: TS2531 – Object is possibly `null`. This issue stems from TypeScript’s strict null checks which prevents potentially nullable values (like `undefined` or `null`) being assigned to non-nullable types.
To understand this, let’s create a scenario. Consider the following code snippet:
html
typescript
export class UserComponent implements OnInit {
userForm : FormGroup;
ngOnInit() {
this.userForm = this.formBuilder.group({
username: [”]
})
const username = this.userForm.controls[‘username’].value; // Error: TS2531
}
}
In the above example, it’s trying to access the value of a form control (‘username’) before the form group has been initialized, hence TypeScript triggering TS2531 error.
There are a couple approaches for troubleshooting and resolving such issues within Angular’s Reactive Forms context:
Use a Null-safe Navigation Operator: In Angular templates, you can use the safe navigation operator (`?`) to guard against null and undefined values in property paths i.e., modify the code as follows:
html
Direct Assignment via new FormControl: Assigning an empty string to a form control during its declaration, allowing it to be non-null at runtime:
typescript
export class UserComponent implements OnInit {
userForm : FormGroup = this.formBuilder.group({
username: new FormControl(”),
});
ngOnInit() {
const username = this.userForm.controls[‘username’].value; // No TS2531
}
}
By initializing the formGroup and FormControl immediately upon declaration, we can prevent the TS2531 error as the object is definitively non-null.
As an Angular developer, resolving TS2531 nullity issues is essential. It not only means creating more robust forms, but also aligns with TypeScript’s philosophy of preventing runtime errors during compile time itself. As Robert C. Martin, one of the leading authors on software development and coding principles, once said, “The best way to troubleshoot code is not to create bugs“.
For more in-depth knowledge about Angular forms and their usage, visit the official Angular Reactive Forms documentation.
Conclusion
Diving deep into the realm of Angular Reactive Forms, it becomes apparent that developers often face a common error: TS2531 Object is possibly ‘null’. This issue arises typically when TypeScript cannot guarantee at compile-time that a property or method of an object isn’t null or undefined.
The power and strict type checking of TypeScript, which is what allows developers to catch errors at compile-time, can sometimes lead to this complication. Within Angular Reactive forms, this is frequently seen when accessing form control properties.
Let’s put some light on an example of how to encounter this error:
xxxxxxxxxx
this.formGroup.controls['controlName'].value
Here, TypeScript may complain stating that Object is possibly ‘null’. The typescript compiler issues this warning because there might be a case where the `formGroup` or the `controls` object within it may not have been initialized yet, leading to a potentially null or undefined reference.
To appease the TypeScript compiler and avoid this error, you could use the optional chaining operator, introduced in TypeScript 3.7:
xxxxxxxxxx
this.formGroup?.controls['controlName']?.value
This makes sure that if formGroup or controls indeed turn out to be null or undefined, accessing the ‘value’ property will not lead to runtime errors. A null value would, instead, be returned.
Alternatively, a non-null assertion operator can be leveraged to tell TypeScript that the specific object will not be null or undefined:
xxxxxxxxxx
this.formGroup!.controls['controlName']!.value
A quote by Douglas Crockford, an influential figure in JavaScript and JSON development, puts the essence of this approach quite aptly in perspective: “JavaScript has more in common with functional languages like Lisp or Scheme than with C or Java.” This flexibility and adaptability of JavaScript (and TypeScript, by extension), as highlighted in this quotation, allows for innovative problem-solving paradigms like optional chaining and non-null assertion operators.
This look into the TS2531 error in Angular Reactive Forms and the potential workarounds to resolve it emphasizes the importance of nuanced understanding of TypeScript’s strict type checking and how to turn these checks to one’s advantage when programming with Angular. Armed with this knowledge, developers can navigate through the intricacies of Angular Reactive forms with greater confidence and effectiveness. [Learn more about this from the official TypeScript Documentation](https://www.typescriptlang.org/docs/handbook/2/everyday-types.html#strict-types).
Remember, there’s no substitute for understanding what your code is doing and ensuring it aligns with TypeScript’s standards. With the fundamentals in place, we empower ourselves to build robust, error-free applications that stand the test of time. After all, as computer scientist Alan Kay once said, “The most effective way to cope with change is to help shape it.”