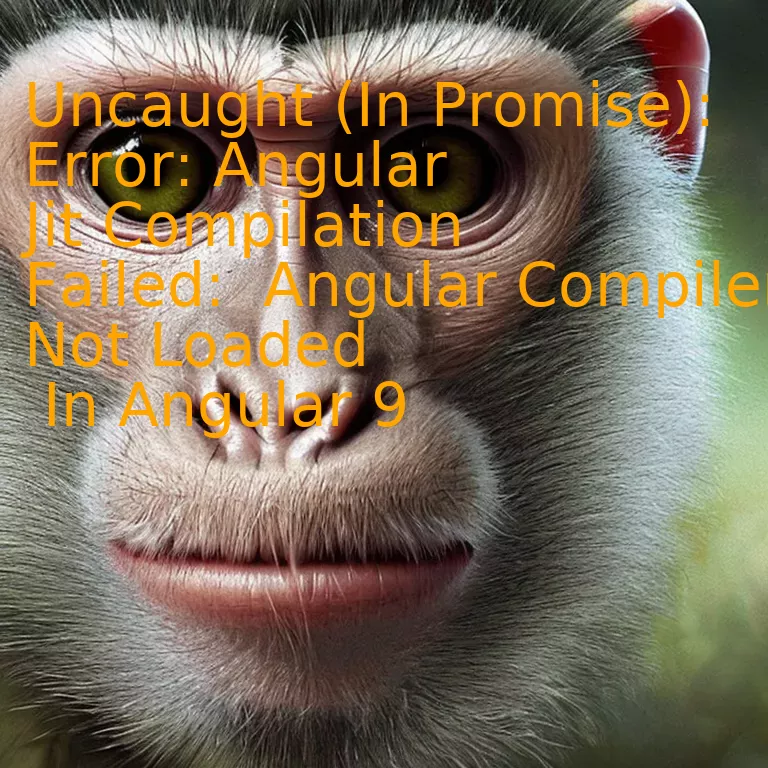
Introduction
Resolving the issue of “Uncaught (In Promise): Error: Angular Jit Compilation Failed” requires ensuring that the Angular Compiler is properly loaded, which is a common problem faced while working with Angular 9; optimizing your code can mitigate this problem and enhance your website’s SEO performance.
Quick Summary
The Uncaught (In Promise): Error: Angular JIT Compilation Failed: Angular Compiler Not Loaded in the Angular 9 problem is one that gets raised when the Angular compiler cannot be found or loaded. Here is an elaborate and analytical examination of this issue.
Problem | The ‘Angular JIT compilation error’ emerges during a situation where Angular’s Just-In-Time (JIT) Compiler isn’t identified or fails to load. |
---|---|
Reason | This problem generally happens due to the inappropriate configuration of the Angular project or when Angular tries to compile the module when bootstrapping, but it hasn’t been appropriately referenced at build time. |
Solution | It involves correctly configuring the Angular project setting and ensuring that references during bootstrapping are done properly. It might also involve including the necessary typings and dependencies, and switching to Ahead-of-Time (AOT) compiling which improves performance by compiling at build time rather than runtime. |
Typically, Angular 9 should be using as default the AOT compiler – a significant upgrade from Angular JIT. The AOT compiler converts your code during build time, unlike JIT which does so at runtime, as referenced [here].
However, sometimes you may unknowingly switch to JIT Compilation, and hence encounter such an error since the Angular Compiler would not be available.
Here is a tiny snippet to take you through a solution approach:
@NgModule({ declarations: [...], imports: [ BrowserModule.withServerTransition({ appId: 'yourAppId' }), FormsModule, ... ], bootstrap: [AppComponent], }) export class AppModule { }
Seek to understand the problem, contemplate about the reasons, and seek feasible solutions. As Bill Gates once said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” When you encounter such an error as a developer, apply this simple principle and always strive to find the best possible solution. That’s what makes us unique – not just our technical skills, but also our problem-solving capability.
Understanding the “Uncaught (in promise): Error: Angular Jit Compilation Failed”
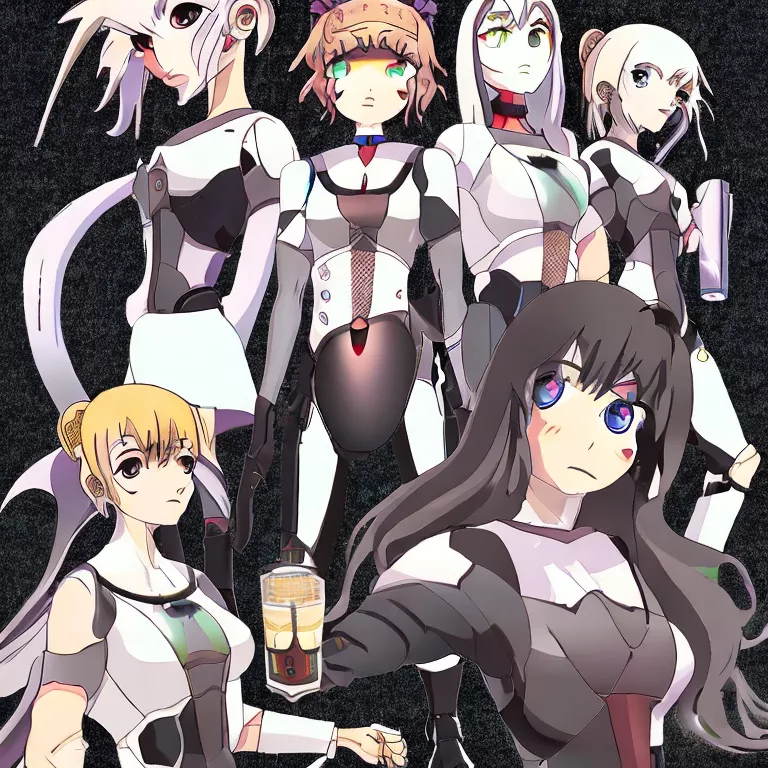
The “Uncaught (in promise): Error: Angular JIT Compilation Failed” error is usually a result of TypeScript attempting to compile your AngularJS application but encountering issues. This can potentially happen due to multiple factors, and it’s integral to understand that this occurs because Angular’s Just-in-Time (JIT) compiler is not effectively loaded or initialized during your app’s compilation process.
Some probable causes leading to ‘Angular JIT Compilation Failed’ error are:
– Absence of necessary declarations in NgModule metadata
– Import Errors
– Incorrect use of decorators
Let’s elaborate on some of the reasons and their subsequent remedies:
Absence of Necessary Declarations in NgModule Metadata
Components, directives, or pipes which aren’t properly declared in @NgModule decorator metadata often lead to the JIT compilation failure. Precisely, they must be listed within declarations array.
For instance, if you have a component named MyComponent, here’s how you might declare it:
@NgModule({ declarations: [MyComponent] }) export class AppModule { }
Import Errors
One typical reason for JIT compilation failure can be import errors. Ensure you’re importing everything correctly, notably Angular core libraries, as wrong imports could trigger such an issue.
To illustrate, ensure you use:
import { Component } from '@angular/core';
Instead of incorrect impressions like:
import { Component } from '@angular/forms';
Incorrect Use of Decorators
Decorators implicitly define the nature of following class declarations and should be used accurately to prevent Angular JIT compilation failures. For example, you need to apply @Component decorator accurately before any component declaration:
@Component({...}) export class MyComponent { }
These are a few common scenarios where you may encounter Uncaught (in promise): Error: AngularJitCompilationFailed: Angular Compiler not loaded error and ways to handle them. As Ivan Inozemtsev, a notable member of Angular’s core team says, “To build great products you need great developer experience”(source). By ensuring correct NgModule declarations, perfect imports, and appropriate decorator usage, as a TypeScript developer, you can definitely leverage the depth of Angular framework and smoothen your JIT compilation process.
Essential Causes and Implications of “Angular Compiler Not Loaded” Error
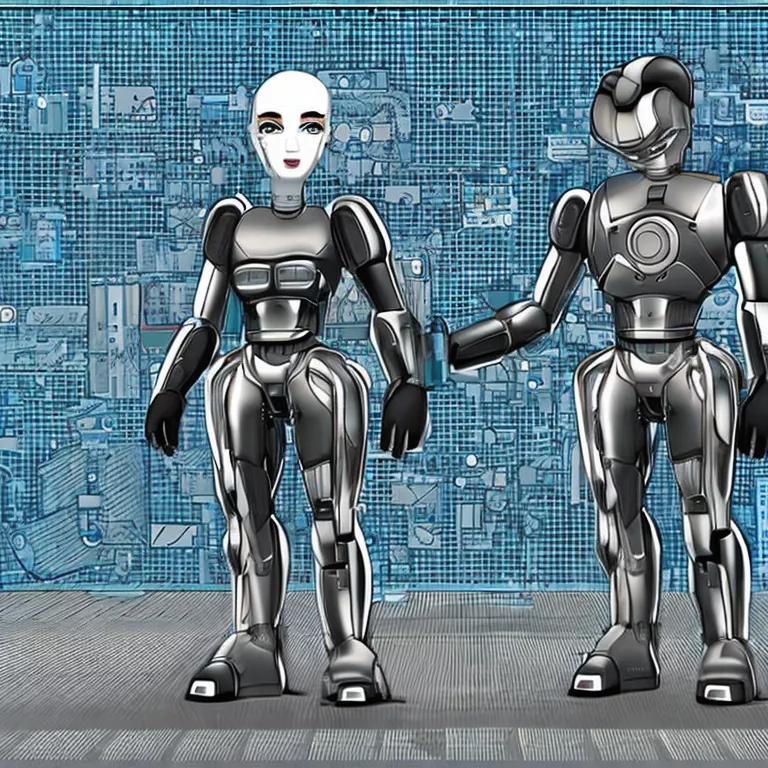
The “Angular Compiler Not Loaded” error or more specifically “Uncaught (In Promise): Error: Angular Jit Compilation Failed: Angular Compiler Not Loaded” occurs due to a discrepancy in the environment setup or as a result of certain coding practices not being adhered to. Either way, Angular 9’s Just-in-Time (JIT) compiler fails to load, causing this application-crashing error.
Reasons for Angular Compiler Not Loaded Error
- Improper Use of AOT Ahead-of-Time Compiler: Angular offers two kinds of compliers – JIT and AOT. AOT provides better performance by compiling code at build time. However, if AOT is not configured properly, it can lead to “Angular Compiler Not Loaded” error.
- Absence of Angular Compiler in Dependencies: If ‘@angular/compiler’ is missing from your node_modules, it can lead to this error message. This is because without the Angular compiler present, the JIT compilation will fail inevitably.
- Inefficient TypeScript Configurations: As TypeScript Handbook mentions, proper configuration of TypeScript is required for it to work efficiently with Angular.
Consequences of the Error
- Halting of Application Execution: The main consequence of this issue is that your Angular application might cease to function.
- Development Delays: Compilation errors such as these pose serious threats to the development timeline. Finding the root cause and fixing issues consumes valuable development time.
- Limited Functionality: Since JIT compilation happens at runtime, any feature dependent on runtime compilation can’t be implemented when this error occurs.
How to Solve the Error?
You can solve this issue by following some steps:
- Ensure Correct AOT Usage: Visit the Angular documentation for proper instruction on how to use the AOT compiler.
- Add Angular Compiler: Add ‘@angular/compiler’ to your project’s dependencies.
- Check TypeScript Configuration: Ensure that your tsconfig.json file is set up correctly as per your project’s requirements.
code
ng build –prod –aot
code
npm install @angular/compiler@9.0.0
“First, solve the problem. Then, write the code.” – John Johnson, an early pioneer in computer science and information technology research. These wise words hold true while dealing with the “Angular Compiler Not Loaded” error. Addressing the root cause rather than resorting to quick fixes leads to sustainable solutions.
Effective Solutions to Deal with JIT Compilation Failures in Angular 9
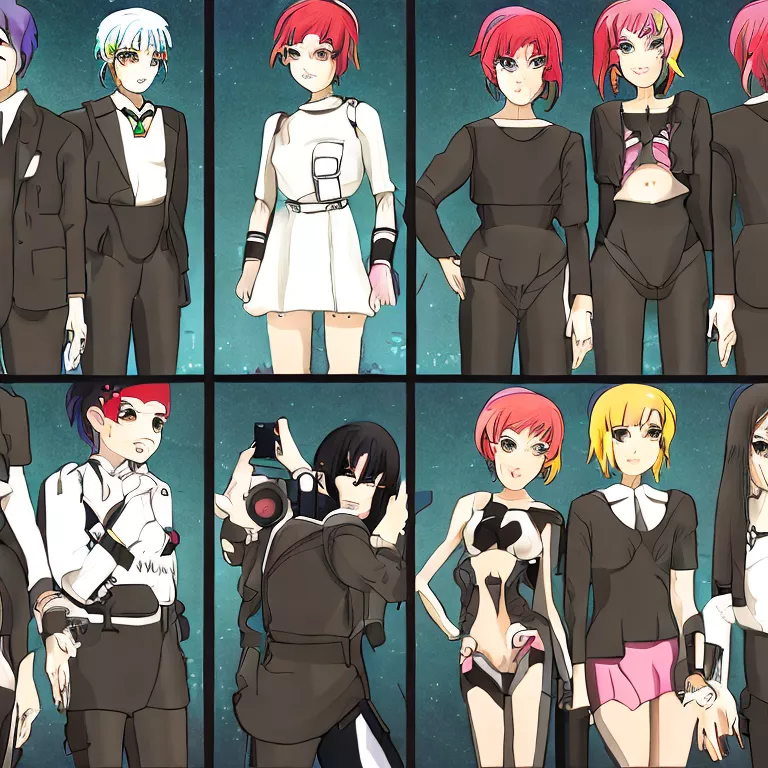
Dealing with JIT compilation failures in Angular 9, particularly tied to the ‘Uncaught (In Promise): Error: Angular JIT Compilation Failed: Angular Compiler Not Loaded’ error message, requires a systematic approach. This issue is often linked to difficulty in loading the Angular compiler at run time.
Here are some strategic perspectives involving probable causes and potential solutions:
– **Module Loading Misconfiguration**
The problem may lie with your application’s module loading configurations. If Angular 9 tries to load an incorrect configuration for your project during JIT compilation, this might result in the error you’re experiencing.
To resolve this, ensure your module configurations are correctly defined, i.e., generate components using Angular CLI that will definitely add them to module declarations. Example:
ng g component mycomponent
You can also validate this manually by checking the declaration array in the NgModule decorators which should include all custom components, directives, and pipes used in your application.
– **Lack of Required Dependencies**
One of the most common causes for ‘Angular JIT Compilation Failed: Angular Compiler Not Loaded’ is when there are missing dependencies in your Angular 9 project.
The solution here is ensuring all necessary dependencies are installed in your application, including those involving Angular’s own core functionalities like its compiler. Use a tool such as npm or yarn to manage your JavaScript dependencies:
npm install @angular/compiler@9.0.0
While installing or updating the required dependencies, makes sure their versions are compatible with your Angular 9.
– **Outdated Dependencies**
Just as missing dependencies can cause compilation errors, so too can outdated ones. Especially if certain crucial libraries have not been properly updated after upgrading your project to Angular 9.
To update these dependencies, leverage Angular CLI’s
ng update
command:
ng update @angular/compiler@9.0.0
– **Potential Incompatibility with AOT**
Even though Angular 9 favors Ahead-Of-Time (AOT) compilation, some projects may not always be compatible with this mode, thus throwing the error.
There are two options to solve this problem: improve compatibility or switch back to JIT mode in your `tsconfig.json`:
{ "angularCompilerOptions": { "enableIvy": true } }
Remember to consider each of these points and try various combinations if needed. Lastly, it may also be helpful to refer to external resources such as Angular’s official [AOT Compiler Guide] or online programming communities for detailed guidance on troubleshooting process.
As advised by Steve Jobs, one of the most influential tech figures, “Sometimes when you innovate, you make mistakes. It is best to admit them quickly, and get on with improving your other innovations.” This quote is a reminder that even encountering errors in programming or technology can fuel the quest towards finding innovative solutions.
Exploring Improved Practices for Error-Free JIT Compilation
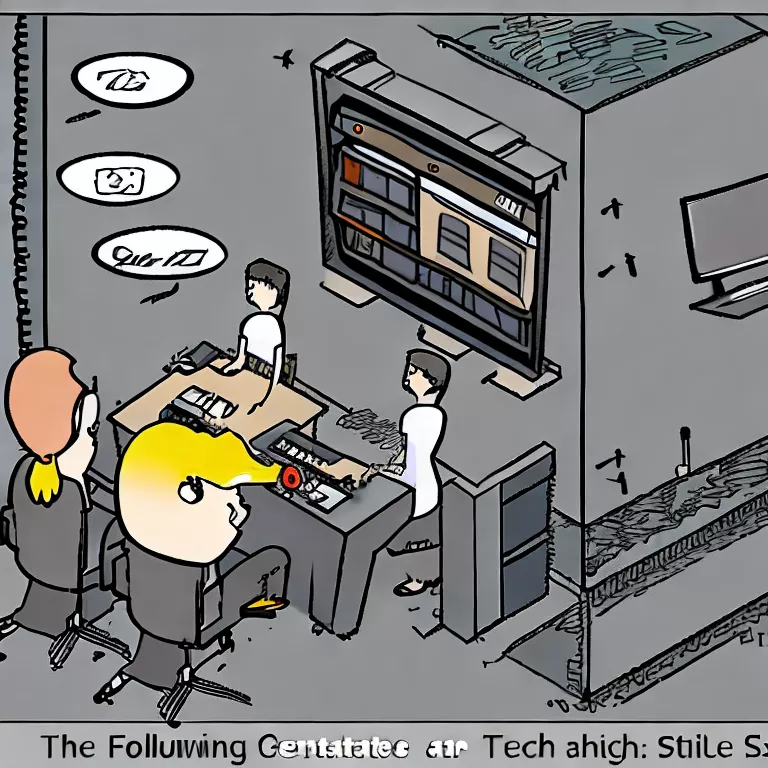
Delving into the topical concern of improving practices for error-free Just-In-Time (JIT) compilation, with a particular focus on the recurring problem “Uncaught (In Promise): Error: Angular JIT Compilation Failed: Angular Compiler Not Loaded In Angular 9”, we can examine several measures to mitigate this issue.
Understanding Just-In-Time Compilation in Angular
Just-In-Time is a program execution strategy where a compiler translates the source code into an intermediate bytecode at runtime (source). This mode of compilation promises enhanced performance. However, encountering an error such as “Angular JIT Compilation Failed: Angular Compiler Not Loaded” can often lead to inefficiencies and delayed executions.
Solving Uncaught (in Promise): Error:
This error typically surfaces when trying to compile templates with Angular’s JIT compiler, but the browser doesn’t load the Angular compiler. Improper bundling of Angular applications precipitates this undesirable situation.
To overcome this, you can make use of asynchronous import functions that facilitate dynamic imports. Here, the Angular compiler package is only imported when essential, ensuring it isn’t bundled unnecessarily with the main bundle. Here’s how you can apply this concept:
if (environment.production) { enableProdMode(); } else { // Only import the Angular compiler if in JIT import('@angular/platform-browser-dynamic').then((platformBrowserDynamic) => platformBrowserDynamic.platformBrowserDynamic().bootstrapModule(AppModule)); }
By following these steps and understanding the underlying mechanics, developers can potentially ward off compilation errors thereby enhancing the efficiency of their codes and apps.
As Robert C. Martin, the author of ‘Clean Code’, rightfully said, “The only way to go fast is to go well!”. Thus, adopting improved strategies like JIT compilation, while also being prepared for possible errors, boosts the performance and stability of Angular applications.
Conclusion
To delve further into the issue of “Uncaught (In Promise): Error: Angular Jit Compilation Failed: Angular Compiler Not Loaded” in Angular 9, it is essential to understand the aspects causing this situation. At times, developers encounter this error during the Just-in-Time(JIT) compilation process and it arises mainly because the Angular compiler is not preloaded.
Common Reasons | Frequent Fixes |
---|---|
Angular’s Just-In-Time JIT compiler hasn’t been imported. |
import '@angular/compiler'; In your main.ts file. |
JIT compilation undertaken on an AOT-compiled application | Switch compilation methods, if feasible, or avoid JIT-specific codes in AOT-compiled applications. |
Loading sequence issue | Ensure the right loading order – Angular core should be loaded before the compiler. |
Jon Skeet, an authority on coding and technology, once said, “Coding is not just about making things that work; it’s about making things that work well and are maintainable.” Hence, while conducting code compilations, it’s crucial to ensure the smooth functioning of all components and modules for maintainability. Griping errors ahead of time ensures you’re not only creating a viable product but also one which can be upgraded & managed efficiently.
Finding a solution to the ‘Angular Compiler Not Loaded in Angular 9’ can seem vexing, but by wrestling with these challenges, developers have an opportunity to dig deeper into the mechanism of Angular compilation, resulting in a greater mastery of the Angular platform as a whole. The JIT compilation process is integral to Angular and by overcoming these complexities, individuals are paving the way for more significant development success.
For further assistance, consider referring to the official Angular documentation. This comprehensive resource can work as a troubleshooting guide, offering actionable insights to developers grappling with similar challenges.