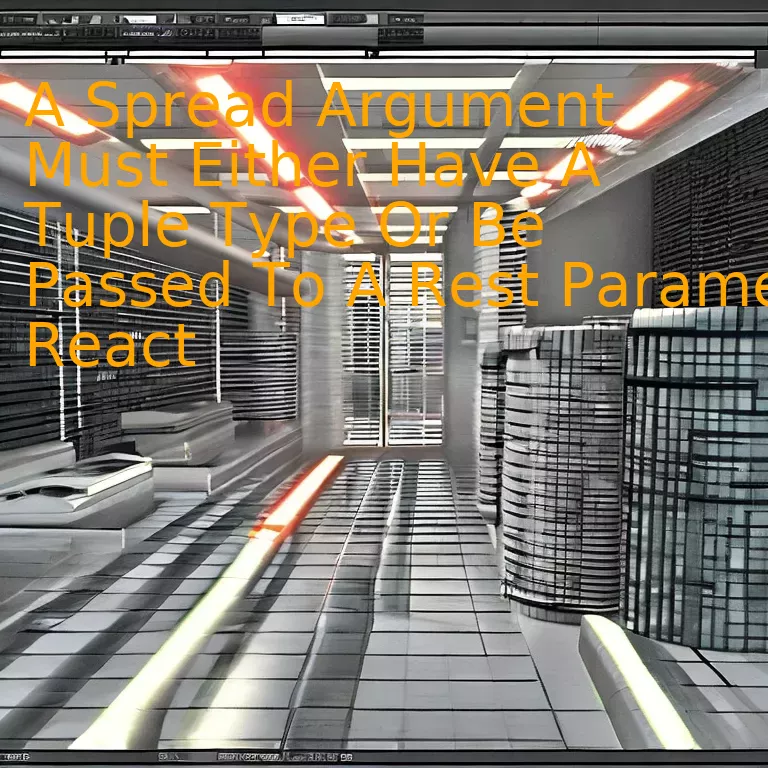
Introduction
In React, a spread argument must meet certain criteria, such as being of a tuple type or passed to a rest parameter, to ensure efficient and effective code execution.
Quick Summary
The Spread operator in React (denoted by …) is a powerful tool that allows developers to expand an iterable such as an array or string into individual elements, or combine multiple objects into a singular one. However, it’s crucial to understand the correct usage to avoid potentially complex errors. One such fundamental rule is that spread arguments in React need to either have a tuple type or must be passed to a rest parameter.
Let’s start by illustrating this concept with a table representation:
Kind | Description |
---|---|
Spread Argument with Tuple Type | In TypeScript, Tuples allow us to express an array with a fixed number of elements whose types are known but need not be the same. When you spread in a tuple, it gets expanded into its respective values. |
Passing Spread Argument to Rest Parameter | Rest parameters function in conjunction with the spread operator to pack or unpack elements or properties into a collection. Passing a spread argument to a rest parameter means utilizing the capability of the spread operator to unpack collected values into distinct arguments. |
Let’s suitably illustrate these principles using TypeScript:
In the first case, let’s see an example of how a tuple type would work with a spread operation,
let tuple: [number, string, boolean] = [1, "test", true];
function handleArgs(a: number, b: string, c: boolean) {
console.log(`Number: ${a}, String: ${b}, Boolean: ${c}`);
}
handleArgs(tuple);
// Outputs: Number: 1, String: test, Boolean: true
In the above TypeScript snippet, we’ve a standard tuple with three different data types. We then use a spread operator in the function call to ‘handleArgs’. The tuple gets expanded, and each element corresponds with the respective parameter in the function.
In the second case, let’s see an example of passing a spread argument to a rest parameter,
xxxxxxxxxx
function handleRestArgs(args: number[]) {
args.forEach((arg) => console.log(arg));
}
let numbers: number[] = [1, 2, 3, 4];
handleRestArgs(numbers);
// Outputs: 1, 2, 3, 4
Here, we’ve an array of numbers that we’d like to pass individually to a function. The function ‘handleRestArgs’ uses a rest parameter (…args) to capture these.
As Bruce Lee once said, “Adapt what is useful, reject what is useless, and add what is specifically your own.” In TypeScript or any programming language, understanding the context where specific syntax or operations are beneficial enables us to write cleaner, more efficient code.
Understanding Spread Arguments in React
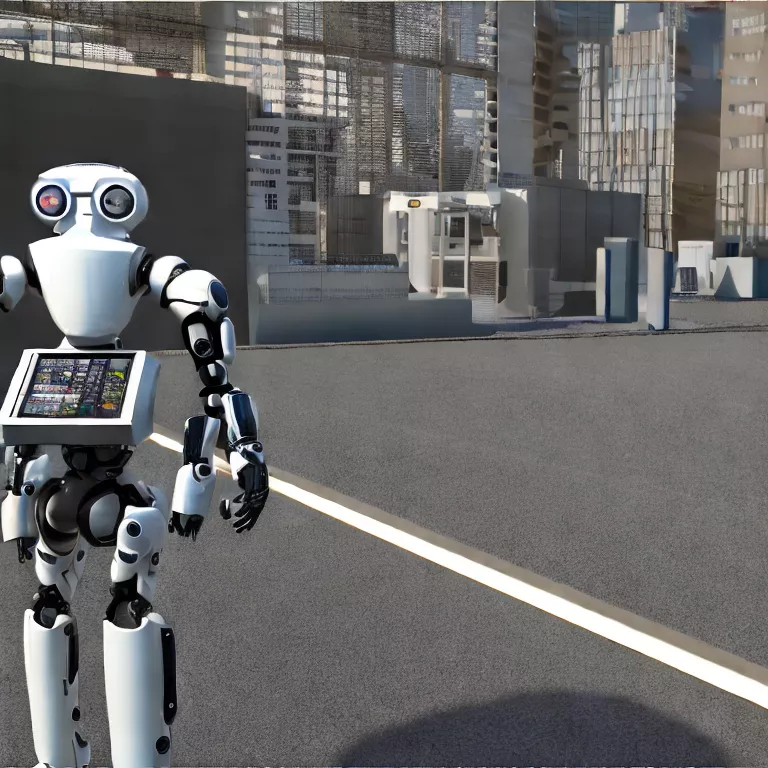
Understanding spread arguments in React is a concept that can be applied to any object, including components. Spread arguments allow an object to explode its properties into discrete elements, which translates to an easier way of passing multitudes of props to components swiftly and efficiently.
Normal Props Pre-Spread Arguments | With Spread Arguments |
---|---|
xxxxxxxxxx <Component propOne={propOne} propTwo={propTwo} propThree={propThree} /> |
xxxxxxxxxx const props = { propOne, propTwo, propThree }; <Component {props} /> |
In TypeScript, a spread argument can either have a tuple type or be passed to a rest parameter. This notion is essential for creating scalable, clean, and maintainable components. Tuple types are used when the number and sequence of elements are known, while rest parameters capture an indefinite number of values.
Tuple Type with Spread Argument as a React Prop:
xxxxxxxxxx
type MyProps = [number,string]; // This is a Tuple Type
const Component = (props:MyProps) => {/* component implementation */}
Rest Parameter with Spread Argument as a React Prop:
xxxxxxxxxx
const Component = (restProps: Array<string>) => {/* component implementation */}
Any attempt to misapply these concepts will immediately get monished by TypeScript compiler, making your code robust and free from bugs caused by incorrectly typed props. It is also important to mention that expand arguments conserve the immutability of objects and arrays, which is crucial in React’s functional programming approach.
Remember, as Ada Lovelace quoted, “Those who know how to anticipate can be considered prepared, but those who know how to improvise are truly ready.” Hence, learning more about TypeScript spread arguments and how they apply to function parameters can set the stage for efficiently propagating properties in your applications. This powerful tool should be inherent in every React developer’s arsenal to streamline components’ props management. You can learn more details from the official TypeScript documentation on function parameters.
Exploring Tuple Types & Rest Parameters in React
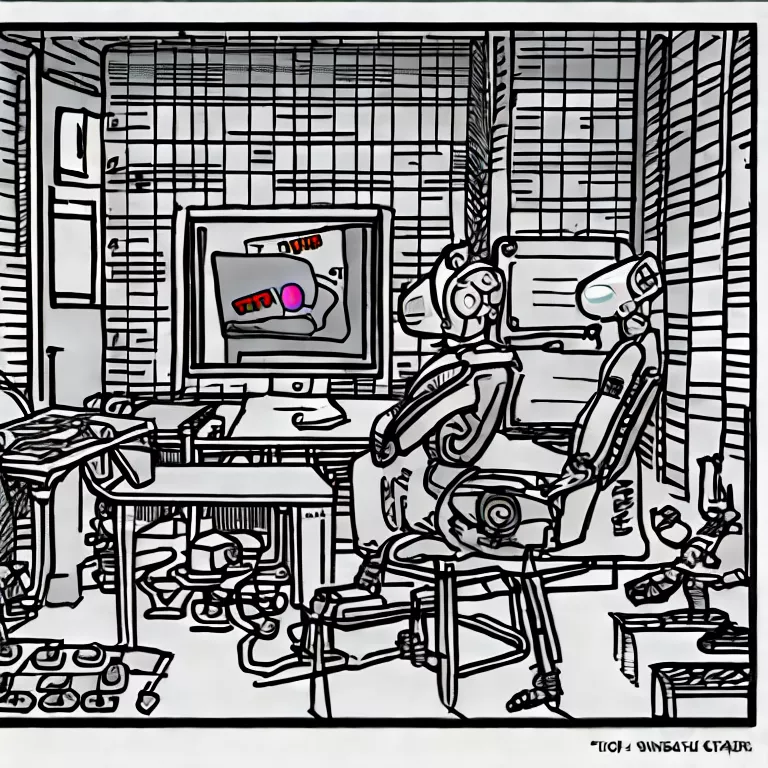
The Typescript language has empowered developers with various types, and among them, Tuple Types and Rest Parameters play vital roles, especially when dealing with functional components in React. These typing structures enable developers to pass multiple parameters of different types, both as function arguments and component props.
Tuple Types:
Tuple types are an advanced form in TypeScript to describe arrays where the type of a fixed number of elements is known but not necessary the same. They’re incredibly useful for functions that return multiple values or components expecting a prop array with certain types in specific positions.
For instance, consider a component that takes a tuple prop:
xxxxxxxxxx
interface MyComponentProps {
myTuple: [string, number, boolean];
}
const MyComponent: React.FC<MyComponentProps> = ({ myTuple }) => (
// The component logic...
);
In the above code snippet, the tuple enforces the order of parameter types.
Rest Parameters:
While rest parameter syntax allows us to represent an indefinite number of arguments as an array, it must be handled carefully in TypeScript owing to its strict nature.
Rest parameters can either take multiple arguments of the same type or have a tuple type, resolving the issue mentioned in the question – “A Spread Argument Must Either Have a Tuple Type Or Be Passed To A Rest Parameter”.
Here’s how we can utilize rest parameters within a functional component:
xxxxxxxxxx
interface MyComponentProps {
someArgs: [string, number];
}
const MyComponent: React.FC<MyComponentProps> = (someArgs: [string, number]) => (
// The component logic...
);
As observed, the rest parameter takes the shape of a Tuple Type, incorporating all required argument types. Effectively, rest parameters have enriched the functional components of React by enhancing flexibility and readability.
Insightfully, Ryan Dahl, the creator of Node.js, once said, “Almost everything we write is essentially a form of user interface.” Therefore, TypeScript introduces a strongly-typed UI language designed to scale. Tuple Types and Rest Parameters are just two examples of this advancement, providing a robust way of managing complex data structures in React.
TypeScript Documentation
Potential Errors with Non-Tuple Types in Spread Argument: A Deep Dive into React
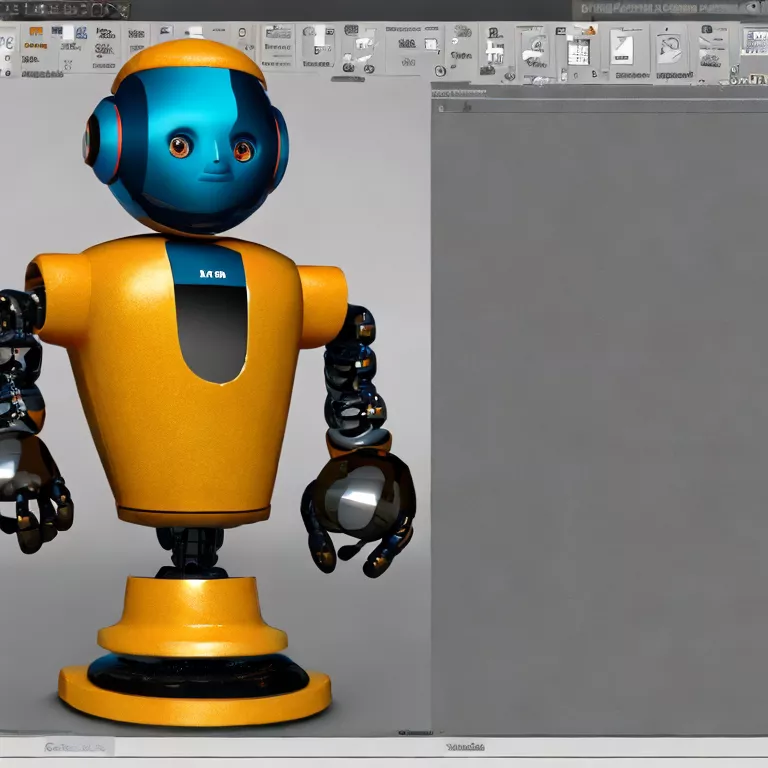
A close examination of React and TypeScript interaction reveals the inherent challenges associated with incorrectly employing non-tuple types in spread arguments. Predominantly, it emphasizes the criticality to abide by the rule that a spread argument must either utilize a tuple type or be transferred to a rest parameter.
Recognizing this vital requirement helps in curtailing potential errors in coding structure and ensures streamlined operation of your application.
HTML:
“React components implement a render() method that takes input data and returns what to display.” — Facebook’s React Documentation
The aforementioned quote draws attention to the fact that appropriate data handling is crucial to effective rendering in React. Applying non-tuple types as spread arguments could misguide this process, potentially leading to complications.
Firstly, let’s probe into one primary problem when non-tuples are used inappropriately in spread arguments in TypeScript with React.
HTML:
xxxxxxxxxx
type T = [string?, number[]];
const arr: T = [];
arr.push("Hello");
arr.push(123);
function acceptsTuple(t: T) {
console.log(t[0] + " " + t[1]);
}
acceptsTuple(arr); // Hello 123
In the above code, an optional string followed by a series of numbers forms a tuple type (T). However, if we replace ‘T’ with a non-tuple such as a simple array, TypeScript is unable to maintain the inferred order. Consequently, JavaScript causes an error since it fails to differentiate between the string and numbers upon second usage.
Hackernoon highlights this issue clearly in their article on React.js and TypeScript.
This raises the importance of embracing practical dynamics in code structure, especially concerning spread argument implementation. TypeScript offers a reliable approach in using tuple types for arguments, which assures consistency and accuracy.
To summarize, inappropriate usage of non-tuple types as spread arguments in React with TypeScript can cause potential errors due to misidentified sequence. Employing a tuple type or passing it as a rest parameter presents an effective solution while implementing spread arguments for seamless application operation.
Therefore, refining your understanding of React and TypeScript compatibility and using tuple types for passing arguments will significantly enhance your coding efficacy and functional output.
Best Practices for Using Spread Arguments with Tuples and Rest Parameters in React
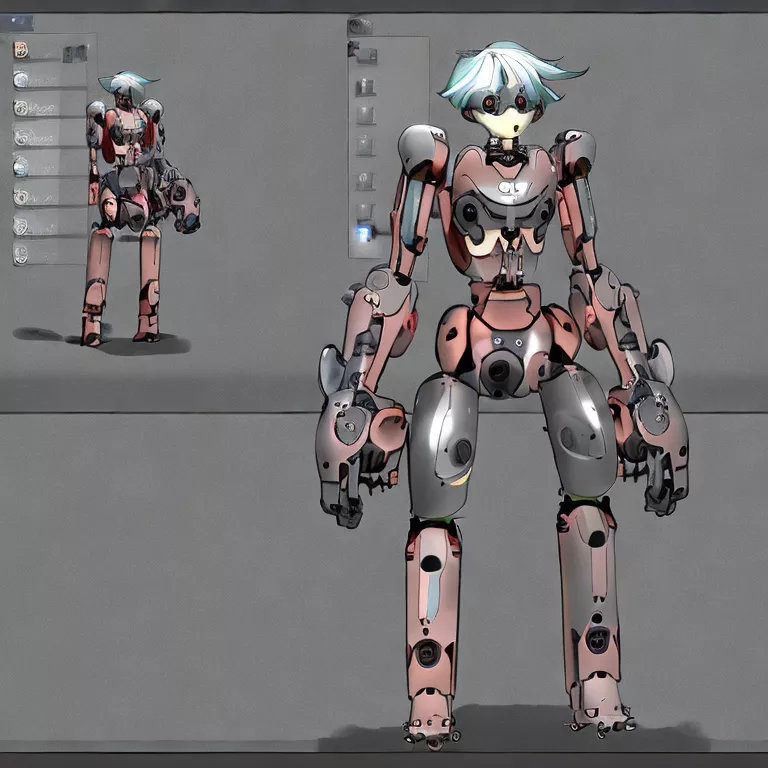
It’s a known fact that utilizing the spread operator in conjunction with tuples and rest parameters can be done very efficiently in React but it comes with a caveat. Spreads arguments must conform to a certain standard: they ought to either have a tuple type or be in sync with a rest parameter.
Spread arguments enable the elements of an array or object representation to be expanded in locations where one or more arguments (for function calls) or elements (for array literals) are expected.
The Nuance of Using Spread Operator with Tuples
Being acquainted with tuple types can essentially further optimize the process of leveraging spread syntax. A tuple type facilitates extension and invocation of values based on the array-like structure- encapsulating multiple fields, thereby augmenting code efficiency with respect to static type-checking and control flow-based type analysis.
html
xxxxxxxxxx
// Tuple type in TypeScript
let tupleType: [string, number];
tupleType = ["Hello", 10]; // Initialising
A major advantage of using tuples in TypeScript is their perfect tendency to combine with the spread operator inside function calls. Developers often leverage this feature for diffusing arrays as arguments while working on a React project.
Spread Operator with Rest Parameters
Rest parameters simplify the interaction with multiple arguments of a function by acting as placeholders for any number of arguments. When a spread argument operates within a rest parameter, it dynamically takes up the corresponding value and type from the declared tuple.
html
xxxxxxxxxx
function sampleFunction(args: [string, number]) {
let [text, num] = args;
console.log(text, num);
}
sampleFunction("Hello", 10);
In the above example, each array element after destructuring accepts data type and behavior according to the assigned tuple.
Remember, “Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it” – Brian Kernighan . So leveraging spread arguments judiciously with tuples and rest parameters will save you debugging time in your TypeScript development journey with React.
Conclusion
The “JavaScript Spread Operator” in TypeScript and its usage in React is among the pivotal concepts utilized by developers. Delving deeper into this concept, we can appreciate its multifaceted applicability, specifically its role with Tuple and Rest parameter.
Understanding key functions like passing arguments to a Rest parameter or having a Tuple type prime us in resolving potential errors involving spread arguments during our code journey in React. To optimize comprehension, let’s break down these implementation strategies:
• When working in TypeScript, types must be explicitly defined for objects or functions. A Tuple enforces the sequence and the type of elements an array can hold. This helps avert potential argument-related issues when using the Spread operator.
• Conversionary table depicting typical error vs resolution:
Error/Issue | Solution |
---|---|
Argument of type ‘unknown’ is not assignable to parameter of type ‘[]’ | Explicitly specify Types |
The RHS of an ‘&’ expression is a type which could be instantiated with a ‘null’ type. | Use Optional Chaining (?) for null check |
Consider this example where a developer uses a Tuple – The function expects a Tuple type.
xxxxxxxxxx
type MyTuple = [number, string];
let x: MyTuple = [10, "hello"];
function tupleFunction(a: number, b: string){
console.log(a+b);
}
tupleFunction(x);
React also provides immense flexibility when parsing rest parameters using spread operators. This functionality allows the collection of multiple elements dispersed in iterable objects into a single one, thus ensuring proficiency and neatness in your code.
Elon Musk once said, “You should take the approach that you’re wrong. Your goal is to be less wrong.” Consequently, understanding these practices better is a smart move towards minimizing errors and enhancing efficiency when working with TypeScript in React. This knowledge equips us to effectively use the spread operator, thus ensuring our code’s robustness and sustainability.
References:
1. TypeScript 2.0
2. React Docs – JSX in Depth