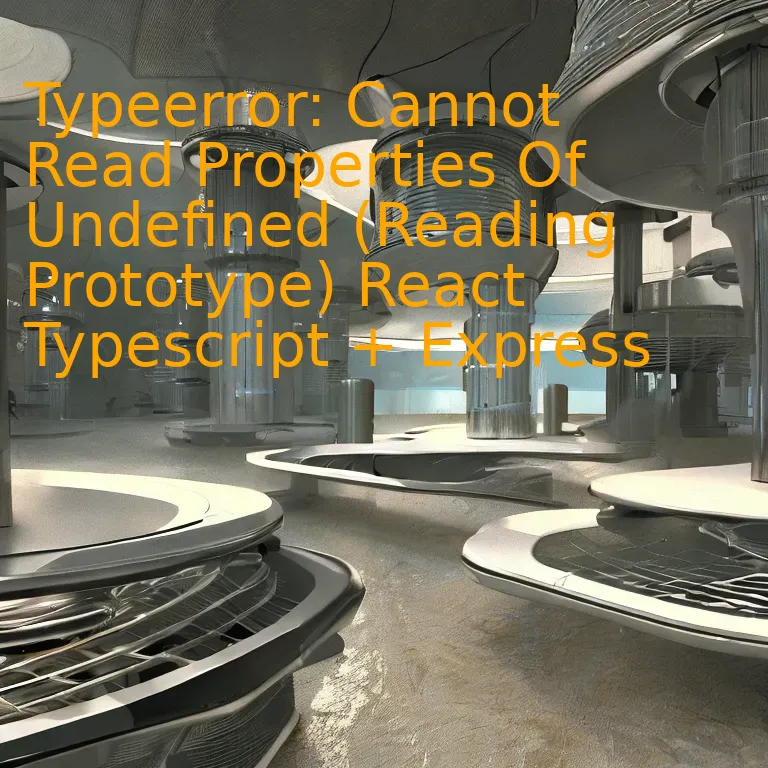
Introduction
Understanding and resolving the TypeError: Cannot Read Properties of Undefined (Reading Prototype) in React TypeScript with Express involves digging into your coding structure. This common issue often stems from an improper reference to variables, objects or function names, causing a disruption in backend operations. The key lies in meticulous debugging and ensuring that all properties are properly defined and initialized within your React TypeScript + Express framework.
Quick Summary
The
Typeerror: Cannot Read Properties Of Undefined (Reading Prototype)
in a React Typescript and Express app is quite common. This error occurs when one attempts to read a property of an object that may not exist at certain points throughout the application. For understanding, let’s consider the following:
Error | Possible Cause | Solution |
---|---|---|
Typeerror: Cannot Read Properties Of Undefined (Reading ‘Prototype’) | This usually emerges from trying to use an object or function which hasn’t been initialized yet or doesn’t exist. | To resolve this issue, always check if an object exists before accessing its properties. This can be achieved effectively using optional chaining. |
An example in TypeScript context would look as follows:
typescript
let user = {
name: ‘John’,
details: {
age: 30,
address: undefined,
},
};
If we try to access `user.details.address.street` directly, it would lead to the same TypeError because ‘street’ is a property of ‘undefined’. Instead, using Optional Chaining like `user?.details?.address?.street` allows us to safely attempt to access nested object keys.
Furthermore, while programming with TypeScript on a React + Express application, following best practices for error handling can help mitigate such issues. Here are some key points:
– Make sure to initialize your variables appropriately.
– Use try-catch blocks wherever necessary.
– Explicitly specify data types where possible.
– Avoid any type modification after initialization.
To quote Anders Hejlsberg, creator of TypeScript: “By allowing you to express what you expect from the API in code, TypeScript makes it easy for others to use your API, and for you to use others’ APIs.”
Hence, keep in mind that TypeScript is not just a language but is also a toolkit for structured coding, helping us avoid common JavaScript errors like
xxxxxxxxxx
TypeError: Cannot read properties of undefined (reading 'Prototype')
.
Understanding the TypeError: Cannot Read Properties of Undefined in React TypeScript
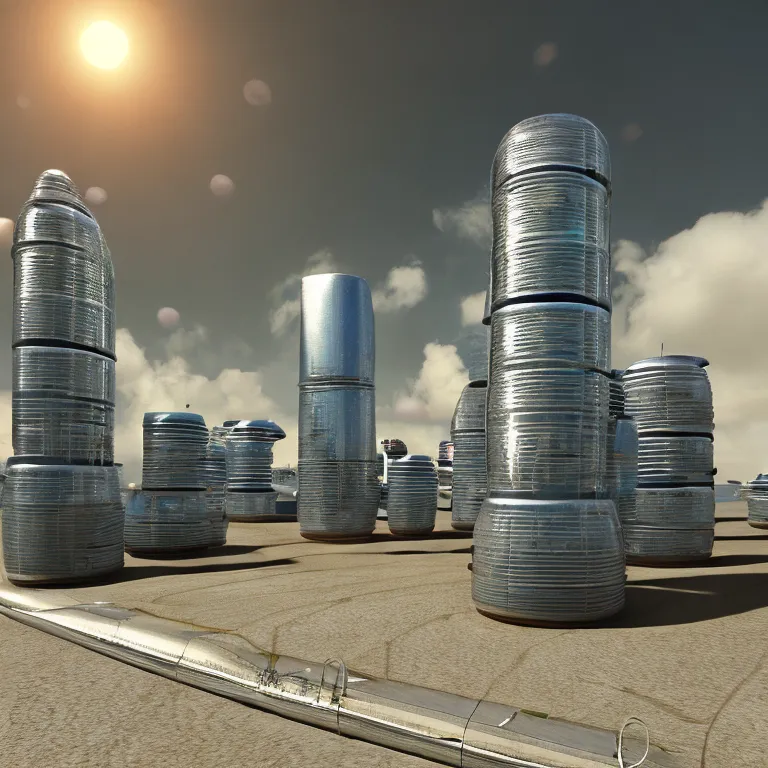
Diving into the error “TypeError: Cannot Read Properties of Undefined (Reading ‘Prototype’)” using TypeScript with React and Express, it’s necessary to grasp the underpinning principles at play. This error is generally surfaced when you try to invoke a method or access a property on an object that is undefined in your TypeScript code.
Before embarking on the debugging process, here are some insights into why this error might occur:
– Either during the runtime or the compile-time, the reference to a particular object is not available or has been mistakenly defined as null or undefined.
– The execution context or scope could have changed unknowingly, causing undefined errors.
– The data fetch operations from Express JS may be asynchronous in nature. Here, a potential race condition could exist where the rendering process in React tries to access properties before they’re loaded.
Understanding these points allows us to delve into the solutions to this issue:
Let’s consider the first point – unavailability or wrong definition. TypeScript attempts to enforce type checking at both compile-time and run-time levels (TypeScript_Type_Checking) . It is prudent to ensure the data types for your objects are correctly defined:
xxxxxxxxxx
// TypeScript
let myObject: MyObjectType | undefined;
In the case of changing contexts, using arrow functions instead of traditional function declarations can help safeguard the context, as arrow functions don’t bind their own “this”:
xxxxxxxxxx
// TypeScript
myFunction = () => {
console.log(this.myObject);
}
When it comes to asynchronous race conditions, it is advisable to handle asynchronous codes using async/await mechanism or utilising Promise then/catch methods:
xxxxxxxxxx
// TypeScript with ExpressJS
app.get('/data', async (req, res) => {
let data = await fetchDatafromDB();
res.send(data)
});
In React, before accessing any object property or method, ensure the object is not null or undefined. You can use conditional (ternary) operators or Logical ‘AND’ (&&) operator for this:
xxxxxxxxxx
// TypeScript with React
let displayData= this.state.myObject && this.state.myObject.property;
Thus, as Benjamin Franklin aptly said: “An ounce of prevention is worth a pound of cure.” Accurate definition of variables, utilizing arrow functions to retain context, and appropriate handling of asynchronous code can prevent the error “TypeError: Cannot Read Properties of Undefined (Reading ‘Prototype’)” while using TypeScript, React, and Express.
Exploring Express as a Solution for “Cannot Read Properties Of Undefined”
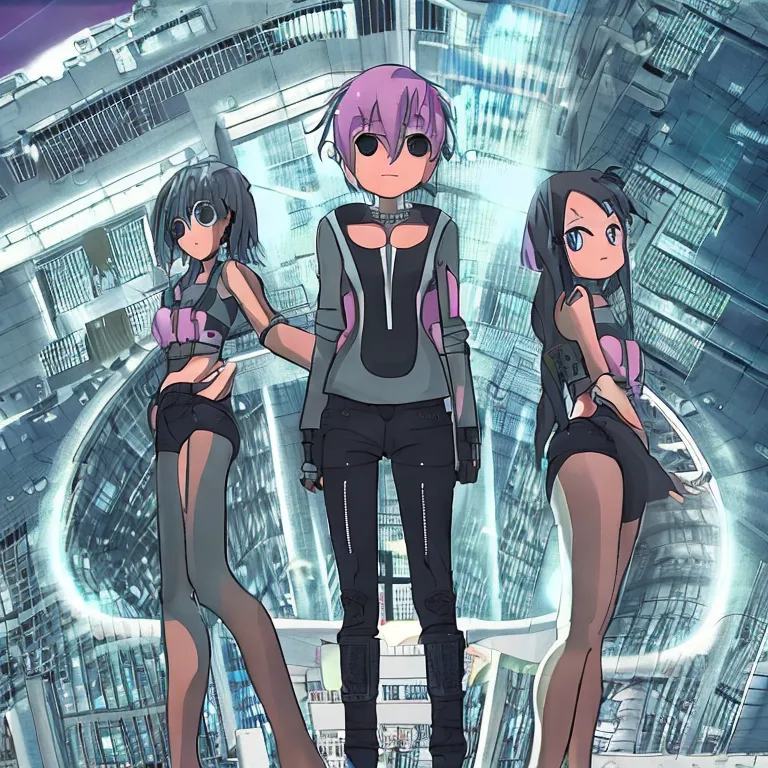
Getting to know Express and how it can help resolve the “TypeError: Cannot Read Properties Of Undefined (Reading Prototype)” error in React TypeScript can be invigorating. It acts as a backbone that manages HTTP requests holistically, streamlines routing, simplifies API creation, and forms middleware.
Express is built on Google’s V8 engine – essentially, the heartbeat of Node.js. In turn, it seamlessly integrates with several JavaScript frameworks like Angular, Vue, and yes, React. Leveraging Express with the diverse ecosystem of Node.js allows for an unparalleled app development experience.
Let’s go over the salient features of Express:
-
xxxxxxxxxx
Routing
: It helps manage the groundwork for receiving and responding to certain types of HTTP requests at precise URLs.
-
xxxxxxxxxx
Middlewares
: With these building blocks, we can perform actions on request and response objects efficiently.
-
xxxxxxxxxx
Templating
: This feature enables injecting dynamic data into HTML templates before sending them back to the client.
Now, regarding this specific TypeError thrown in React with TypeScript and Express, it occurs when we’re trying to access properties of an object that hasn’t been defined yet. In other words,
xxxxxxxxxx
undefined
doesn’t have any properties, hence, trying to read it results in a TypeError.
An effective solution for ‘Cannot read properties of undefined’ involves adding checks before accessing any property. Let’s look at an example in the context of React and Express:
Assume you have an Express middleware function:
xxxxxxxxxx
app.use((req, res, next) => {
req.customProperty = "My custom Express prop!";
next();
});
And then, in your main server file or routing handlers, perhaps you are using it in a manner similar to this:
xxxxxxxxxx
app.get("/", (req, res) => {
console.log(req.customProperty.propName);
res.send("Home Page");
});
In this case, TypeScript would throw
xxxxxxxxxx
'TypeError: Cannot read properties of undefined (reading prototype)'
due to
xxxxxxxxxx
propName
being undefined.
To fix this issue, you should add specific checks before accessing the nested property:
xxxxxxxxxx
if (req.customProperty && req.customProperty.propName) {
console.log(req.customProperty.propName);
}
else {
console.log("The property or object is not defined!");
}
As the iconic creator of JavaScript, Brendan Eich once said, “Always bet on JavaScript.” The synergy between Express, React, and TypeScript serves as a testament to this statement. They ensure flexibility, strong typing, superior development experience while bolstering capacity to solve common runtime errors like ‘Cannot read properties of undefined’.
How to Handle ‘Undefined’ Reading Prototype Errors – A Comprehensive Strategy
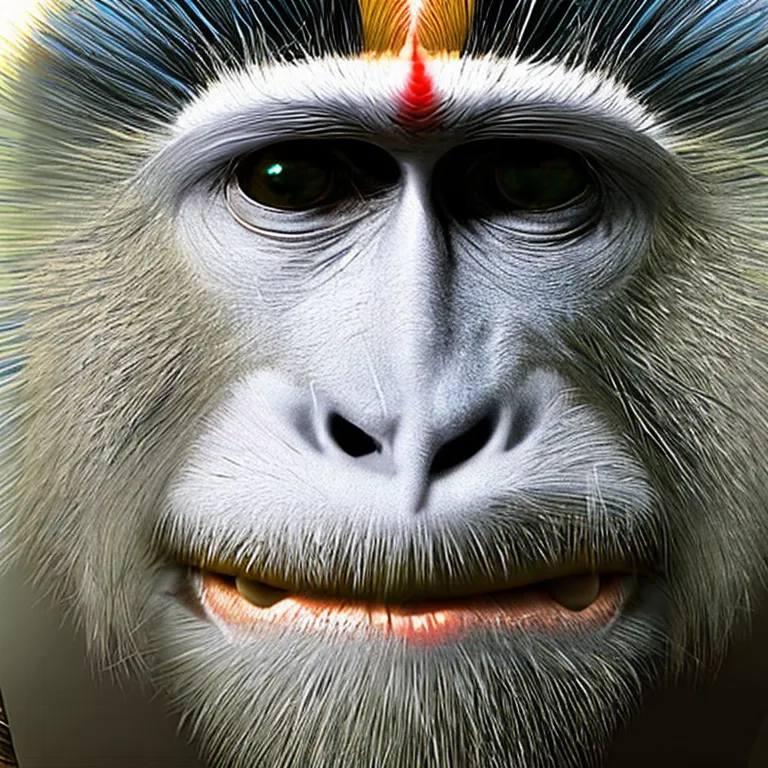
When developing in TypeScript with React and Express, the error “TypeError: Cannot read properties of Undefined (reading prototype)” can be a common roadblock. This is usually encountered when trying to access a property or method that belongs to an undefined variable object.
Let’s delve deeper in understanding, diagnosing, and strategizing a comprehensive solution for this problem:
Understanding the Error: The root cause of the issue emerges from the JavaScript’s inherent property of behaving loosely with variables. This means attempting to call a method on something that doesn’t exist would result in an undefined error. As Bill Gates said, “Understanding the error makes half of the solution.”
Diagnosing the Problem: Using development tools such as React dev tools and Chrome dev tools becomes instrumental in identifying ‘where’ it all goes wrong. Look for where the variable starts becoming undefined – this is typically where the fault lies.
Avoiding Direct Access: Since reading properties of undefined throws an error, ensure checking if the object you’re referencing actually exists. The use of JavaScript’s optional chaining (`?.`) can provide a safeguard by returning `undefined` instead of throwing an error.
xxxxxxxxxx
const value = data?.property;
Initializing Objects: Always remember to assign initial values to your objects. Keep in mind, TypeScript operates on strict mode which assumes variables are null or undefined unless otherwise declared.
xxxxxxxxxx
let data: DataType = {
property: initialValue,
};
Use Nullish Coalescing: It becomes crucial to guard against null or undefined values. With TypeScript, utilizing nullish coalescing (`??`) will provide a default value when encountering null or undefined.
xxxxxxxxxx
const value = data?.property ?? 'default value';
Implementing Type Guards: Use TypeScript’s type guards, functions that perform runtime checks and return a boolean indicating whether the expected type is correct.
xxxxxxxxxx
function isDataType(obj: any): obj is DataType {
// Check if object meets criteria for DataType
return typeof obj.property !== 'undefined';
}
if(isDataType(data)) {
console.log(data.property);
}
Promise Chaining: When dealing with asynchronous codes, remember to return your promises and properly handle them using promise chaining or async/await syntax. Failing to return a promise will lead to an undefined output.
Practicing these strategies allows you to debug efficiently to prevent or rectify “TypeError: Cannot Read Properties of Undefined (Reading Prototype)”. TypeScript, when harnessed correctly, becomes a powerful tool providing insights into JavaScript’s dynamic nature, nudging towards better code discipline.
Keep in mind what Donald Knuth, a legendary figure in the programming community, said: “We should forget about small efficiencies, say about 97% of the time: premature optimization is the root of all evil.” There’s always room to improve our code but identifying and fixing real errors takes precedence over attempts to prematurely optimize it.
An In-Depth look at Problem Solving with React TypeScript & Express
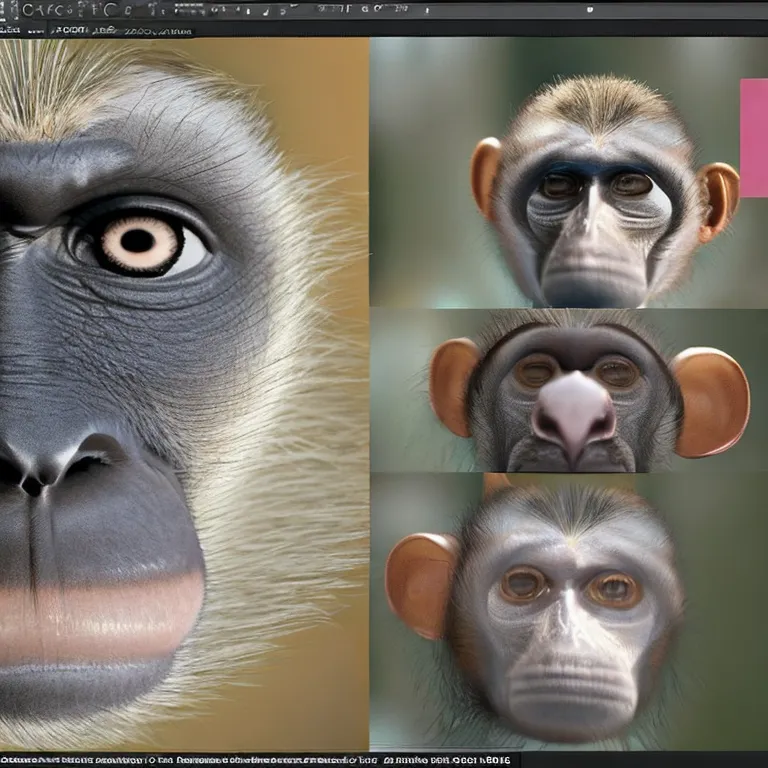
When working with React TypeScript and Express, encountering the TypeError: Cannot read properties of Undefined (Reading prototype) can often be a daunting experience. This notorious error typically arises when trying to read or manipulate an object that has been declared but remains undefined.
In the world of stateful applications written in React TypeScript and interconnected with express.js back-ends, this issue may present in two core areas:
1. When fetching data from your Express server and trying to render it or modify it in your React component.
2. The declaration and initial use of class objects that leverage prototypes for multiple instances.
Debugging and rectifying this error essentially requires attention to patterns of implementation and follows various avenues such as:
Ensuring Correct Fetching and Parsing of JSON Data
React components thrive on data passed from express servers as props or states. Miscommunication in this data passage often results in undefined cases, thereby triggering the undefined TypeError.
Outlined here:
React TypeScript Cheatsheet, you can find strategies on how to correctly pass state and props in TypeScript.
A typical fetch method might resemble:
xxxxxxxxxx
fetchData() {
fetch('/api/data')
.then(response => response.json())
.then(data => this.setState({ data }));
}
Here, the fetched data will only be set as state after the promise is resolved, eliminating possible async issues leading to ‘undefined’ situations.
Safe Navigation through Optional Chaining
TypeScript 3.7 introduced a neat solution towards handling possibly `undefined` or `null` object references—the optional chaining `?.`. Once an attempt to access a property of an undefined object instance is made, instead of a runtime error, `undefined` is returned.
For example:
xxxxxxxxxx
let x = foo?.bar.baz();
This will prevent any TypeErrors from occurring.
Initializing Class Objects
When trying to access a method from a class object that has not been initialized, the result would be an undefined TypeError. Initialising this object properly when using prototypes can avoid this error.
xxxxxxxxxx
class MyClass {
constructor() {}
}
const MyObject = new MyClass();
In conclusion—as David J. Barnes and Michael Kolling, renowned authors of “Objects First with Java,” put it—”Null references can easily cause havoc in systems if they are not handled correctly.” Thus, paying keen attention to possible null reference points can save TypeScript developers lots of debugging nightmares.
Conclusion
The error “TypeError: Cannot read properties of Undefined (Reading Prototype)” in React TypeScript coupled with Express commonly arises due to tentative attempts to access methods or attributes on an object that hasn’t been instantiated amply. When a variable is declared but not assigned a value, it is set as undefined in JavaScript and Typescript. Thus, when you try to access attributes or methods on an undefined object, it leads to the notorious ‘TypeError.’
Delving deep into the root cause of this issue, it could be due to varied reasons:
– Incorrect architecture design in your React application leading to state components not being initialized at runtime.
– Predicament during data fetching from Express where data might not have loaded correctly before attempting to use it.
– Use of ‘this’ keyword outside a class in typescript which doesn’t recognize ‘this’ as an instance of the class.
As far as rectifying this error is concerned, conditional rendering of components in your React App can prove advantageous to ensure your app doesn’t crash due to undefined variables. Plus, error handling should also be put in place while fetching data from your Express server, enabling graceful degradation rather than throwing errors.
Consideration must also be given to understanding the proper usage and limitations of the ‘this’ keyword in Typescript to prevent such errors. As per Fielding & TaylorFielding1999, well-known computer scientists, “Interfaces, objects, classes, inheritance etc are merely abstractions plastered over bits, bytes, and words.”
Embrace this adage and focus on developing your understanding of underlying principles to diminish the occurrence of such issues.
For code snippets check the StackOverflow link.
There’s always a way to correct code errors, the recipe lies in understanding the reason for its occurrence, combined with an analytical approach to rectifying it. Remember, as the rule of thumb goes in the world of coding, every error is a step closer to making your code better and robust.