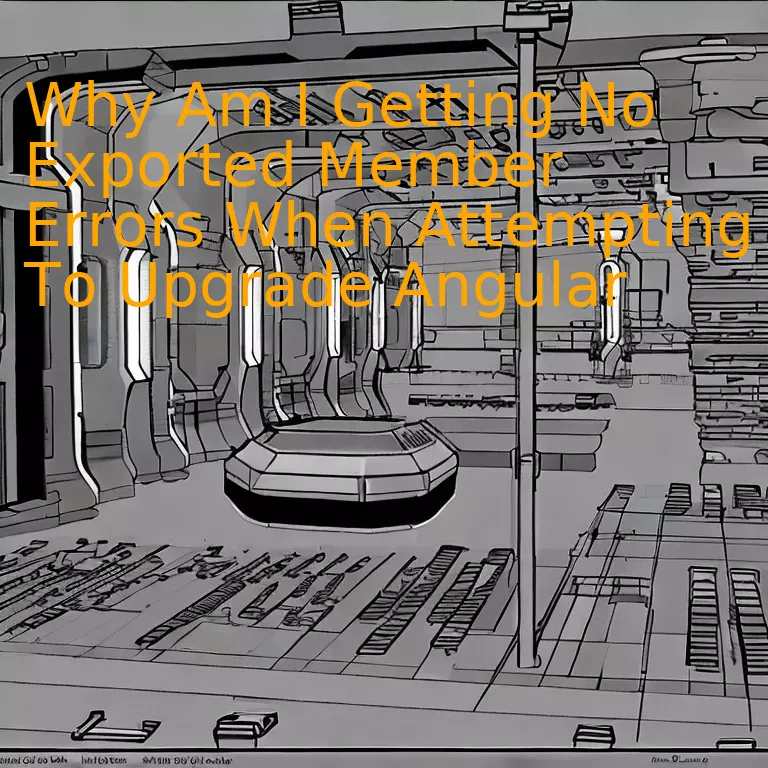
Introduction
While upgrading Angular, the occurrence of ‘No Exported Member’ errors may be due to changes in syntax or the obsoletion of certain functions and features that were present in previous versions, necessitating a review of your codes and ensuring they align with the updated Angular principles and architecture.
Quick Summary
When upgrading Angular, it is not uncommon to encounter issues such as ‘No Exported Member’ errors. This issue often arises due to incompatible versions of different Angular modules, missing exports in your module’s file, or deprecated elements that no longer exist in the newer version of Angular.
Let’s conceptualize this with a hypothetical table:
Causes | Solutions |
---|---|
Incompatible versions of Angular modules | Ensure all Angular modules are updated to the same version that is compatible with the latest Angular. |
Missing exports in your module’s file | Verify that all necessary exports are included in your module’s file. |
Deprecated elements in new Angular version | Check and remove any deprecated features or methods that no longer exist in the updated Angular version. |
As you work on these challenges, here’s a closer look at each scenario:
Incompatible Angular Modules: In this scenario, an “export” could be missing because an update altered its availability or the structure of the Angular module. Consistency between module versions is crucial. For example, if one module is running Angular 7 and another is running Angular 8, inconsistencies that lead to export errors can occur. That’s why aligning all your Angular modules to the same version during an upgrade can help circumnavigate this problem.
Missing Exports: Here, failure to explicitly export components, directives, or pipes in the declarables section of NgModule decorates could trigger no export member issues. Angular modules use these exports to share code with other modules. Ensuring that everything necessary is exported properly is key in resolving these types of issues.
Deprecated Elements: Angular’s ongoing development includes regular updates. Some of these updates may deprecate certain features or functionalities that exist in your code, leading to no export member errors. This is where hashing out deprecated methods and replacing them with their newer counterparts proves helpful.
David Attenborough, a renowned broadcaster, once said, “An understanding of the natural world and what’s in it is a source of not only a great curiosity but also great fulfillment.” Likewise in the world of coding and technology, gaining a deep understanding of the tools and technologies you’re using can help alleviate such issues and lead to more efficient, error-free code.
Now, tackling ‘No Exported Member’ errors when upgrading Angular should be a manageable task with this handy toolkit at your disposal.
Understanding the Issue: ‘No Exported Member’ Errors in Angular
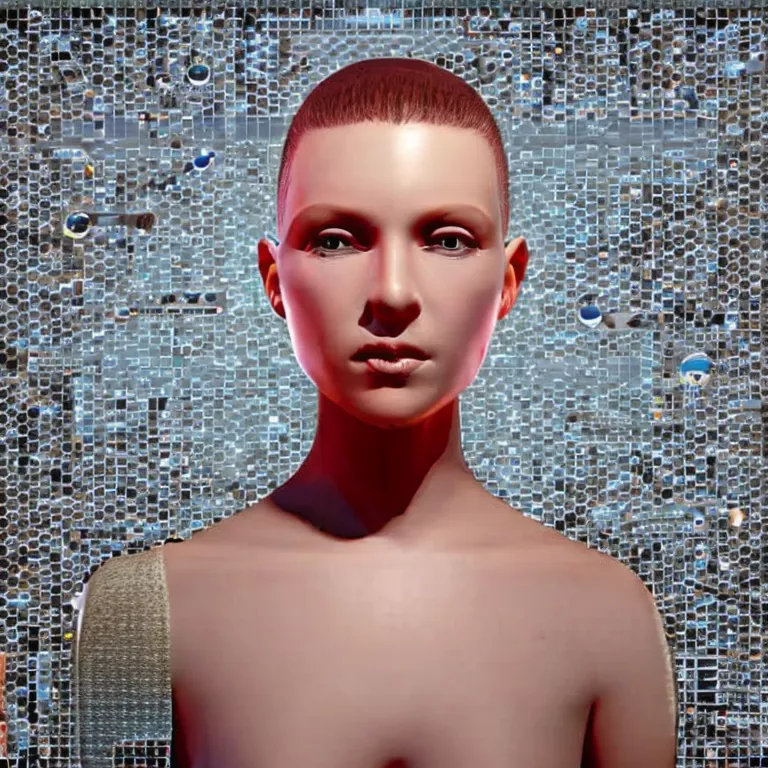
Defining the problem context: ‘No Exported Member’ errors are a common obstacle faced when upgrading an Angular application. Error messages such as
'ERROR in src/app/app.module.ts(5,10): error TS2305: Module '\\"../node_modules/@angular/platform-browser/platform-browser\\"' has no exported member 'BrowserModule'
are indicative of this issue.
The root cause of the ‘No Exported Member’ error is usually related to inconsistencies between Angular modules used in an application and their respective versions. For instance, attempting to use certain members that are available in a later version of Angular in one which doesn’t recognize them results in this particular error.
When you’re working on upgrading your Angular application, there are a number of reasons why you could be seeing these errors:
1. **Deprecated or renamed members**: Some members might have been removed or renamed in newer versions of Angular. If your code is trying to import these deprecated or renamed members, you’ll encounter ‘No Exported Member’ errors.
For example, the `Http` module was replaced by `HttpClient` in Angular version 4.3.0. If your previously built application made extensive use of `Http` and you’ve upgraded without updating these references, it would lead to numerous ‘No Exported Member’ errors.
2. **Mismatches between package versions**: Different versions of Angular packages might not work well together due to changes in exported members. Therefore, if you only upgrade a few Angular packages and not all of them, you might find yourself stuck with ‘No Exported Member’ errors.
3. **Incorrectly structured imports**: In some cases, an import maybe incorrectly addressed. This causes Angular’s compiler to look in the wrong place for an exported member leading to a ‘No exported member’ error.
Addressing these issues will involve revising your codebase:
– You should start by ensuring that you’ve replaced deprecated or renamed members with their current iterations. You may need to consult the official Angular documentation and changelogs to get up-to-speed on these updates.
– Next, verify that all your Angular packages are upgraded to compatible versions. Using Angular’s CLI command
xxxxxxxxxx
ng update
might be useful in managing synchronized package updates.
– Lastly, double-check your imports to ensure they’re well-structured and pointing towards the correct directories.
One of the greatest benefits of Angular is its commitment to forward compatibility, which often makes upgrades very straightforward. Despite this, ‘No Exported Member’ errors can still emerge, but understanding their root cause and how to address them efficiently, forms an important step to a successful upgrade.
As Farrell Fritz once said, “Most good programmers do programming not because they expect to get paid or get adulation by the public, but because it is fun to program.” So, consider this challenge as part of the fun and keep learning.
Framework Updates and Their Effect on Exported Members
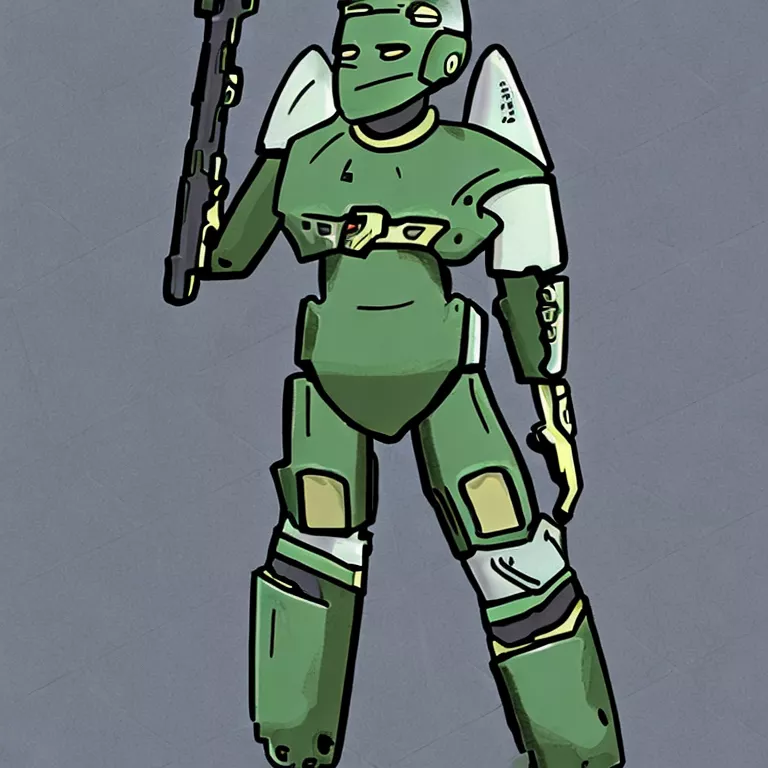
Experiencing No Exported Members errors when attempting to update your Angular app could be a fairly common issue. The problem is often associated with changing codebases as a result of version upgrades, particularly in relation to the migration from one Angular version to another.
Understand that when you upgrade Angular or any JavaScript framework, it tends to affect your codebase’s exported members. To understand why these errors occur, we need to delve deeper into what happens when updating these frameworks:
1. Breaking Changes: Developers consistently need to watch out for breaking changes between versions, which might include deprecated and removed methods or properties. If you have components that depend on now outdated code, upgrading might result in No Exported Member errors.
For instance, when migrating from Angular 8 to Angular 9, the `Renderer` class was replaced by `Renderer2`. Thus, apps using `Renderer` would start showing ‘No Exported member: Renderer’ after the upgradesource.
2. Dependent Libraries: Not all libraries are immediately compatible with new Angular updates. If your application relies on external libraries that haven’t been updated per the latest framework version, you’ll encounter this error.
3. TypeScript Version Compatibility: Angular requires specific TypeScript versions. Using incompatible TypeScript versions leads to various issues, including the No Exported Member Error.
To avoid these errors, consider the following:
• Always review Angular’s change log before initiating an update.
• Update not just your Angular packages but also ensure other dependencies and TypeScript versions are compatible with your target Angular version.
• If a method or component has been deprecated, look for its replacement and refactor your code before upgrading.
xxxxxxxxxx
// Angular 6
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
// Angular 7 onwards
import { HttpClientJsonpModule, HttpClientModule } from '@angular/common/http';
Alan Turing once said, “Sometimes it is the people no one can imagine anything of who do the things no one can imagine.” Similarly, while resolving errors may seem like a daunting process at first glance, taking systematic steps to understand and correct them can lead you to undiscovered pathways in coding efficiency and accuracy.
Resolving ‘No Exported Member’ Errors During Angular Upgrade
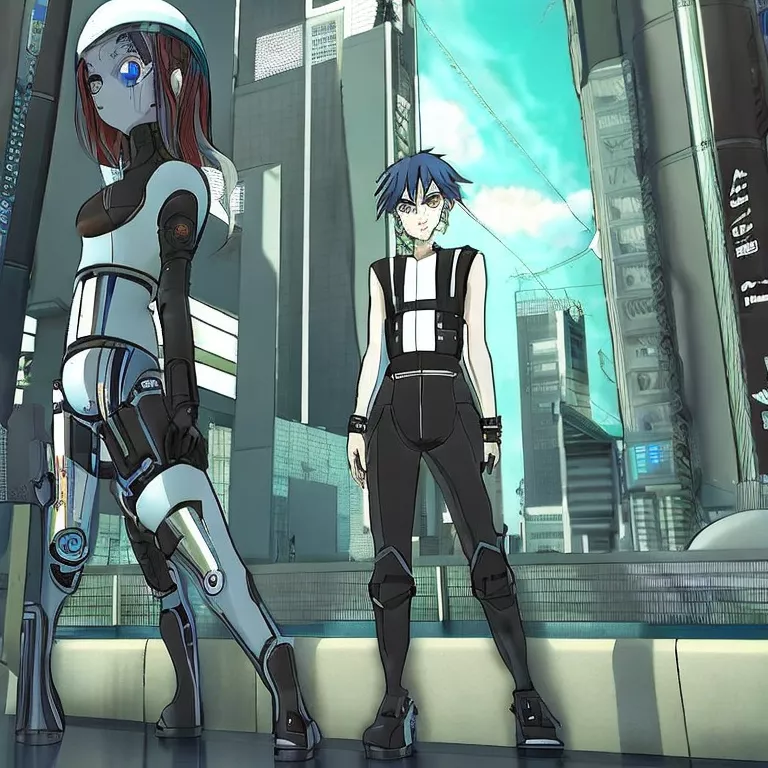
Angular developers might sometimes encounter ‘No Exported Member’ errors during an Angular version upgrade. The ‘No Exported Member’ error usually arises when Angular fails to recognize a module or component that has been exported by the developer. If you are seeing these types of errors while trying to upgrade Angular, there are several key reasons and methods to resolve them.
At the core of this issue is TypeScript’s static type checking mechanism. During the build or compilation phase, TypeScript interprets your code and checks for any inconsistencies between the types you have declared and their use in your code. In certain scenarios, upgrading Angular versions can lead to mismatches in types which TypeScript then flags as ‘No Exported Member’.
Change or Mismatch in Module Types After Upgrade
One major cause of ‘No Exported Member’ errors following an Angular upgrade could be due to changes or mismatches in module types. Certain classes or interfaces that were present in one version of Angular might not be available in the latest iteration, or they might have been replaced with different constructs. This often happens if Angular authors decide to re-optimize the codebase, leading to significant refactoring.
To resolve, developers will need to check the release notes for the specific version upgrade being performed. Angular maintains detailed upgrade guides that developers can use as reference points. These guides highlight breaking changes in each version which should prove invaluable in pinpointing and replacing deprecated modules. Afterwards, it’s just a matter of updating the affected exported members to match those defined in the new Angular version.
Incompatible or Outdated Dependencies
A second common source of this type of error pertains to incompatible or outdated dependencies. The library dependencies specified in your package.json file might not be compatible with the newer Angular version, which can affect how TypeScript identifies and handles modules.
In order to manage such situation, the npm package manager could be utilized to update all dependencies and ensure they are in sync with the new Angular version. This method typically involves running
xxxxxxxxxx
npm outdated
to list all outdated packages and then proceeding to update each one using
xxxxxxxxxx
ng update [package-name]
.
Inconsistent Compiler Options
At times, inconsistent compiler options might create confusion for TypeScript while it checks your code. Angular uses the tsconfig.json file to define the configuration for a TypeScript project. If this file is misconfigured, it might not signal to TypeScript that certain modules are exported, leading to ‘No Exported Member’ errors.
Correcting these errors can be as simple as adjusting the compilerOptions object in the tsconfig.json file. For example, enabling the
xxxxxxxxxx
esModuleInterop
option will adapt default imports to work with CommonJS modules, potentially resolving issues with unexported members.
It’s worth noting that debugging ‘No Exported Member’ errors demands a precise understanding of the Angular ecosystem and extreme patience. However, through regular practice and deep engagement with Angular resources, overcoming such hurdles becomes much simpler. As Bjarne Stroustrup once said, “Proof by analogy is fraud.” – proving to us that only through practical experience and extensive research can developers truly master various Angular aspects, especially when troubleshooting its upgrade processes.
Preventing Future Issues with ‘No Exported Members’ while Upgrading Angular
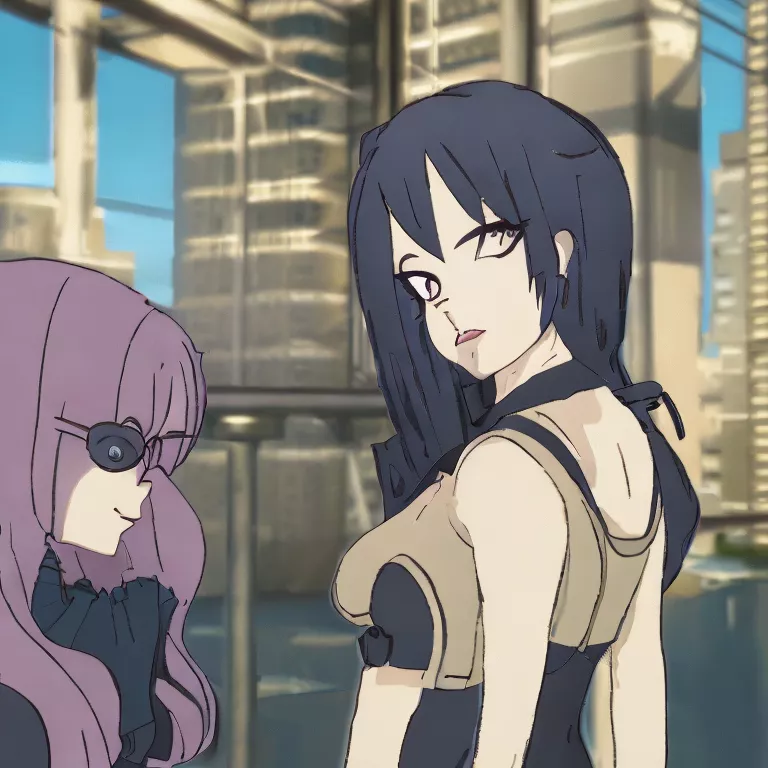
The issue of ‘No Exported Member’ in Angular fundamentally hinges on the way your coding environment is structured and how different elements correspond and interact. It’s particularly common when executing an upgrade, leading many developers to inquire: Why Am I Getting ‘No Exported Member’ Errors When Attempting To Upgrade Angular?
Firstly, it’s necessary to cite what exactly this error depicts. Essentially, when TypeScript looks for a specific module you are importing from and fails to find an exported member there, it will yield a ‘No Exported Member’ error. The error can spring out due to a variety of underlying conditions, which need to be meticulously catered to mitigate any replicated errors over future course.
Core causatives causing such an error while upgrading Angular can range from:
• Robustness of Angular versions: Upgrading to a different version sometimes put us in a hole where compatibility issues lie. Certain features may not exist or have been drastically changed in the new version.
• Miscommunication between components: Directives using components that aren’t appropriately declared or imported can cause unidentified exports error.
Vaguely, we could resolve these problems by adhering to a number of operational norms like:
Ensure Consistency with Angular Version
Angular’s regular upgrades roll out numerous feature modifications and enhancements. Hence, if you choose Angular version A for one product and then version B for another without considering consistency, this can easily lead to ‘No Exported Member’ errors. Ensure there is no disparity between the Angular versions used across projects.
Validate Component Communications
Broken communication lines between components could be a source of errors as well. Checking if directives correctly import and use components they depend on is critical. Angular provides handy ways to delineate component communication for object sharing and interaction.
Correct Declaration of Components
Every component, directive, or pipe should be rightfully declared in an Angular module, otherwise, it might become unrecognized leading to ‘No Exported Member’ issue. Use
xxxxxxxxxx
@NgModule
decorator for declarations of components.
For instance:
html
xxxxxxxxxx
NgModule({
declarations: [
AppComponent,
AnotherComponent,
],
})
Check Interface Visibility
Interfaces should be explicitly exported to make them visible to other parts of the application; a ‘No Exported Member’ error could result if they are hidden. For example:
html
xxxxxxxxxx
export interface SomeInterface {
prop1: string;
prop2: number;
}
In essence, understanding why this error occurs and how to preemptively tackle it involves inspecting your codebase thoroughly. Follow best practices, such as adhering to style guides or adopting Test-Driven Development (TDD), to keep your code clean and efficient. As Robert C. Martin, an influential figure in software engineering once said, “It’s not at all important that you understand what you’re doing the first time you do it, but it’s critically important that you understand it when you’re finished.”
Dealing with these errors doesn’t need to be a nightmare, they can be resolved and prevented by laying a concrete foundation of robust development habits, picking up Angular versioning protocols and cross-checking component level interactions. The idea remains clear – prevention rather than comprehensive debugging post occurrence of the issue.
Conclusion
The primary course of the “No Exported Member” error, during Angular upgrade process, is due to a mismatch in the application’s existing code and the upgraded Angular version. This can be attributed mainly to Angular’s robust nature of enhancing its versions with more strict type accuracy rules and other feature enhancements.
To eliminate these errors, pay close attention to the following factors:
The compatibility issue: Angular provides newer versions which establish stricter type checking policies. Therefore, upgrading your Angular application may give birth to compatibility issues between the older code and new syntax rules. In such a scenario, updating the codes become inevitable by adhering to new rules and syntaxes that new angular version brings in.
xxxxxxxxxx
// Here is an example of how to handle such syntax updates:
// Old version Angular
import { Response } from '@angular/http';
// After upgrading Angular
import { HttpResponse } from '@angular/common/http';
The imports misplacement: Often ‘No exported member’ errors arise when Angular components, directives or modules are incorrectly imported. Hence, it becomes imperative to inspect each import statement, ensuring that they are aligned with the updated Angular Library.
xxxxxxxxxx
// An example of incorrect and correct imports:
// Incorrect import
import { Observable } from 'rxjs/Observable';
// Correct import after upgrade
import { Observable } from 'rxjs';
Third-party libraries: A common pitfall while upgrading Angular applications is to overlook the possible upgrade requirements of third-party libraries. These libraries, not being directly under the control of the Angular team, need individual attention to validate their compatibility with the upgraded Angular version.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler
Therefore, clever housekeeping, strict adherence to enforced regulations, regular upgrading of third-party libraries and keen eye for correct semantics can lead to a clean and smooth Angular application upgrade. Be mindful and dedicated in maintaining the technical health of your Angular applications, and remember, code updates are not a one-time effort but a constant journey.
For more details, you can refer to Angular’s official guide on upgrading between major versions.