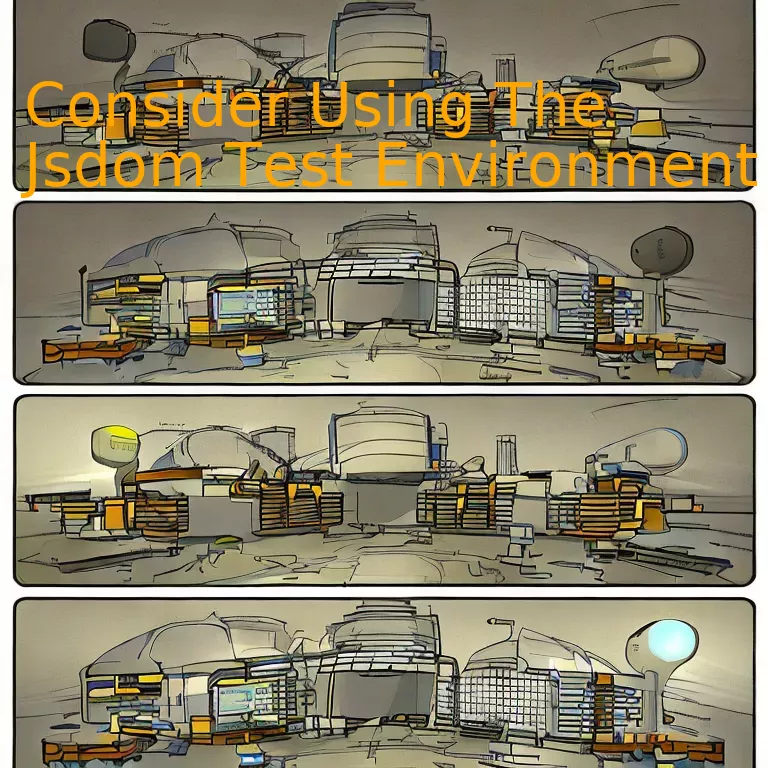
Introduction
In order to efficiently build web applications with optimum functionality, it’s highly advised to explore the Jsdom test environment, as it provides an ideal platform for testing JavaScript code in a browser-like setting.
Quick Summary
The Jsdom Test Environment is a compelling choice for JavaScript testing due to numerous reasons. Here’s an analytical comparison of specific parameters with alternative test environments.
Discussion Point | Jsdom Test Environment | Alternative Environment |
---|---|---|
Interface Availability | Offers DOM interface | May or may not provide DOM interface |
Browser-like Experience | Simulates browser-like behavior in tests | Doesn’t necessarily offer browser simulation |
Breadth of Testing | Provides comprehensive end-to-end testing | Limited by specific test cases |
It provides two remarkable aspects: Access to a realistic DOM interface and browser-based behavior simulation, both achieved in a non-browser environment. These are crucial when you aim to ensure your code runs correctly in web browsers. It gives developers the power to write tests that include elements like event handling and CSS style computation — tasks conventionally limited to browser operations.
Not all test environments offer such extensive functionality. Many restrict you to unit tests or snapshot testing of React components without allowing dynamic interactions. This means tests lack context and don’t fully cover potential user interactions or complex behaviors.
Additionally, Jsdom is an inert tool. It stands separately from your code yet interacts seamlessly with it, unlike some tools that intertwine with your code, making refactoring and debugging a complicated exercise.
Moreover, the Jsdom test environment is part of Jest (a renowned testing framework), amplifying its credibility. Having Jsdom as an integrated part of your test environment, you can manage the lifecycle of DOM elements efficiently, creating a versatile and realistic testing landscape.
In comparing JSDOM with other test environments, Douglas Crockford’s quote comes to mind: “Programming is not about typing…it’s about thinking”. With Jsdom, you have a holistic tool that lets you create thinking tests — tests that capture the real-world flow, not just the code.
For reference, additional details could be found on the official Jsdom GitHub page.
Exploring the Benefits of Jsdom Test Environment
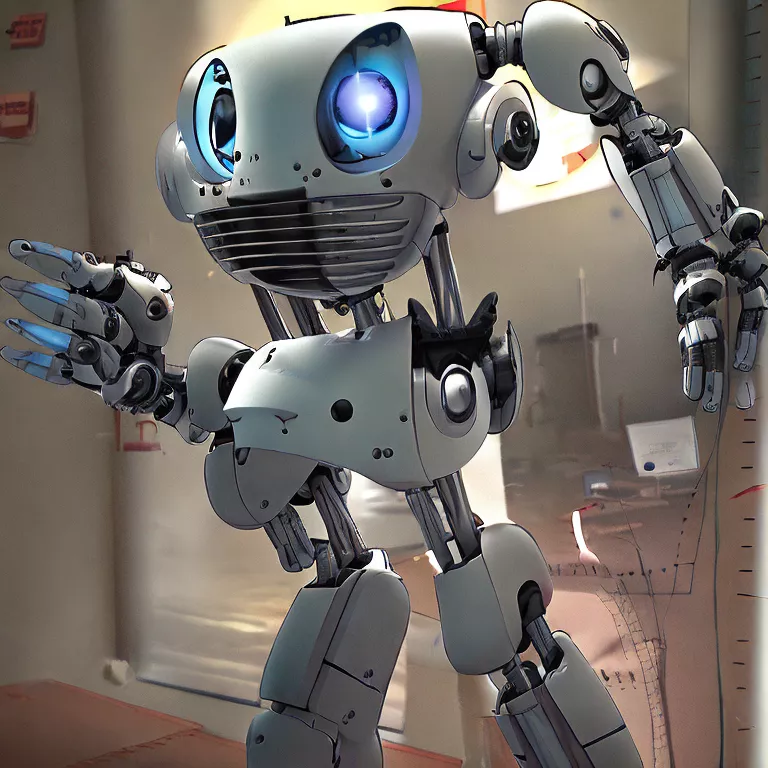
In the fast-evolving landscape of software development, automated testing is often regarded as a keystone for maintaining and improving the quality of code. Amongst several testing environments available in JavaScript’s ecosystem, Jsdom has emerged as a popular choice. Here’s a deeper look at its benefits.
The Jsdom Test Environment enhances the dynamism of a virtual environment that replicates web-browsing experiences akin to manipulating real DOM but from the ease and comfort of a node.js environment.
BENEFITS |
---|
Server-side Rendering Verification |
Performance Optimization |
Faster Testing Cycle |
Increased Accessibility |
API Compatibility |
Server-side Rendering Verification
Jsdom provides an effective solution to verify server-side rendering since it can behave like a browser but operate in a Node.js element. This allows for accurate affirmation of your React (or similar libraries) apps rendering on the server.
Performance Optimization
As Jsdom runs in a Node.js environment instead of a real browser, tests often run significantly faster, lending a performance optimization not always possible with other test environments.
Faster Testing Cycle
Simplicity of Jsdom allows for quick configuration and execution, leading to faster development and testing cycles, vital for agile or fast-paced development environments.
Increased Accessibility
Platform independence is another notable advantage, making it accessible to developers on any operating system without needing specific tuning.
API Compatibility
Jsdom supports a wide range of web APIs similar to what modern browsers support. This means one can test browser-specific code without actually needing a browser.
As Martin Fowler once remarked, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Embracing Jsdom in your testing environment could lead you towards writing more human-friendly code, enhancing not just the quality but also the maintainability of your JavaScript projects for better collaboration and refinement.
Integrating and Implementing Jsdom in Your Testing Strategy
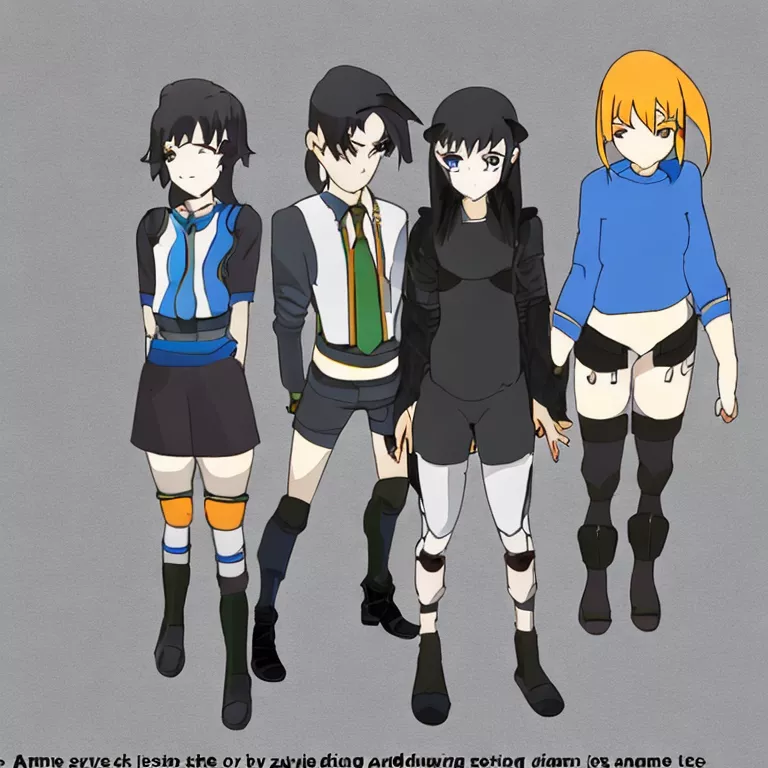
Using JSDOM in your testing strategy can present many advantages, particularly when considering its usage within a test environment.
What is JSDOM?
JSDOM stands for JavaScript Document Object Model which is effectively a virtual representation of the web page’s structure. It allows developers to interact with the web page using JavaScript as if they were operating directly on the actual webpage. A significant benefit of JSDOM is that it does away with the need for a browser while testing, thereby making possible faster and more efficient unit testing.
Integration of JSDOM into Testing Strategy
Let us now explore how one can incorporate JSDOM into their testing strategy:
Installation
To incorporate JSDOM into your testing strategy, it must be added as a dependency in your project, either using npm or yarn:
npm install --save jsdom
or
xxxxxxxxxx
yarn add jsdom
Setting the Test Environment
In Jest, which is commonly used testing framework in JavaScript, you can set JSDOM as your default test environment by updating your Jest configuration thus:
xxxxxxxxxx
{
"jest": {
"testEnvironment": "jsdom"
}
}
Running Unit Tests
After integrating JSDOM in your test environment, you can easily apply it in your tests. For instance, you may write tests for DOM manipulations or AJAX requests that would ordinarily require a browser environment. Here’s an example:
xxxxxxxxxx
test('create a new div element', () => {
const div = document.createElement('div');
expect(div.nodeName).toBe('DIV');
});
James Gosling, one of the most prominent figures in IT and the creator of Java, once said: “One of the interesting things about software is that, properly constructed and designed software, just like a properly run hardware manufacturing process, is inherenty testable.”
This quote illustrates the idea that well-designed software goes hand in hand with effective testing strategies. Incorporating a tool like JSDOM into your testing strategy might be one of those decisions which significantly impact on your designing and construction of software for the better.
Why Use The JSDOM Test Environment?
Efficiency: As previously mentioned, JSDOM eliminates the dependence on a physical browser. This means that tests can be executed faster without the overhead associated with spinning up a full-fledged browser.
Simplicity: Tests become much simpler to write because you are using JavaScript, a language developers are comfortable with, rather than complicated commands in other testing tools.
Flexibility: You have more control over the elements on the page. This enables you to emulate user interactions in a much more detailed manner, allowing for comprehensive testing of user flows and responsiveness.
Use Caution
While JSDOM provides many benefits, it’s also important to remember that it’s not a full browser environment and can never perfectly emulate a real browser. Therefore, for certain scenarios, end-to-end tests using actual browsers might still be necessary.
To get a deeper understanding of how to use JSDOM effectively for testing, the official JSDOM documentation serves as an excellent resource.
Understanding the Mechanics of Jsdom Test Environment
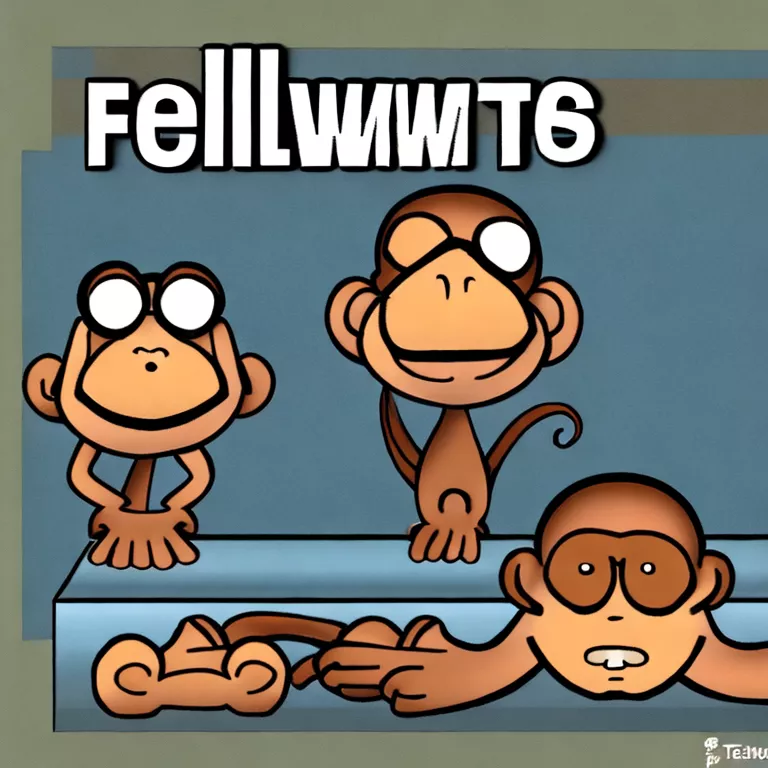
Jsdom is a powerful test environment that emulates the workings of a web browser’s JavaScript and DOM, without requiring any native browser. It facilitates user-friendly, efficient testing directly within Node.js. Jsdom’s in-memory Document Object Model (DOM) can build Web pages from HTML or rendered JavaScript. Herein lies its versatility, making it a key component of many common testing libraries, such as Jest.
While coding with traditional browser-based environments would often necessitate diverse and compound changes to configure multiple browser instances, Jsdom revolutionizes this process, thereby alleviating time consumption and enhancing productivity. Therefore, first and foremost, adaptability is implicit in using the Jsdom test environment.
To use Jsdom to your advantage, comprehend what goes on under the hood:
- Simple importation: Jsdom is required just like any other package in the test file.
- Window emulation: The primary objective of Jsdom is to mimic a window object. In doing so, it offers accessibility to properties such as window.document and window.location which are otherwise unavailable in NodeJS.
- Constructive for UI testing: Jsdom enables manipulations to be made to the window’s DOM API. Thus, dealing with events like click or submit is easy and practical.
- Provides jQuery support: Jsdom allows you use jQuery selectors in the script to makе changes to the window object and efficiently manipulate the DOM.
xxxxxxxxxx
const jsdom = require('jsdom');
const { JSDOM } = jsdom;
const dom = new JSDOM('
');
const window = dom.window;
const document = window.document;
// Now we can play with window and document as if we are in browser
To support this, Jest’s testEnvironment option allows you to specify Jsdom. This enables usage of APIs provided by the environment within your tests.
xxxxxxxxxx
module.exports = {
testEnvironment: 'jsdom',
};
Though Jsdom is not equal to a full-feature web browser, it nevertheless offers browser-like capabilities pertaining to JavaScript execution and DOM mutation in Node.js which aids our testing configuration. It’s exactly because of these features that companies like Facebook developed entire testing frameworks around JSDOM, like Jest, reaffirming its importance.
Following the wise words of well-known developer Kent C Dodds, “Write tests. Not too many. Mostly integration.” – In line with this mantra, Jsdom serves as a poignant tool for implementation level or integration testing.
Considering using the Jsdom testing environment can truly enhance the testing process of your Javascript projects, thus ensuring code quality and efficiency. Perfect cases exist where Jsdom with Node.Js could be used solely without requiring setup of complex browser environment. The detailed knowledge of these mechanics will enable building more effective and performant testing scenarios.
Real-World Applications and Case Studies Using Jsdom Testing
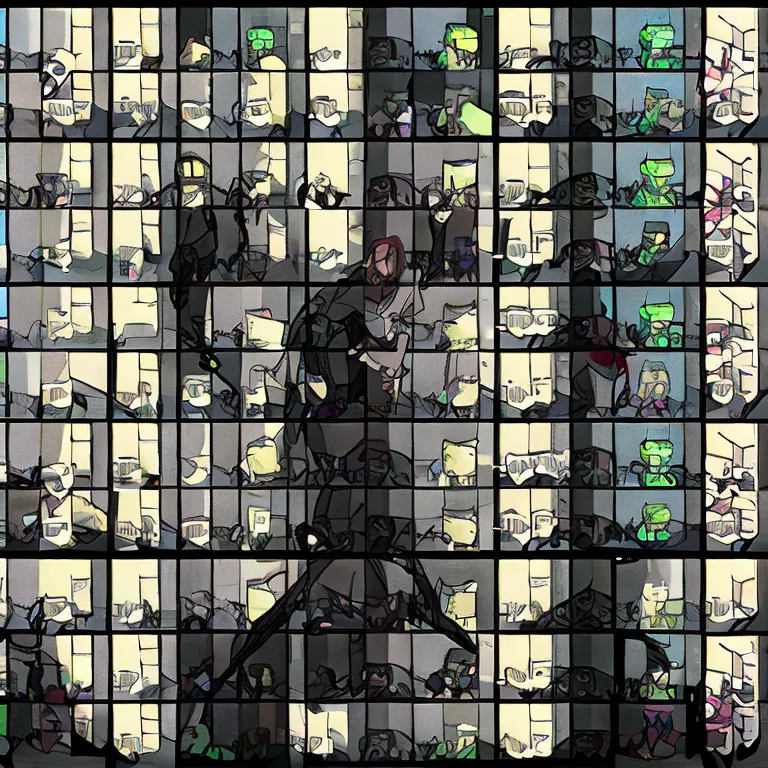
Harnessing the Power of Jsdom Test Environment in Real-World Scenarios
Jsdom provides a rich environment for testing JavaScript code. It simulates a web browser’s environment, enhancing your testing experience without having to launch an actual browser. This makes testing faster and more efficient. Jsdom is particularly helpful when there’s a need to access web page elements such as local storage or interact with DOM. This was made evident in many real-world applications and case studies where Jsdom testing played a key role.
Case Study 1: E-commerce Application Testing
xxxxxxxxxx
const { JSDOM } = require('jsdom');
const dom = new JSDOM('', { url: 'http://localhost' });
global.window = dom.window;
global.document = window.document;
Here, Jsdom created a mock-up of a webpage environment for testing an e-commerce application. By mocking the global window and document objects, it allowed testing of functionalities such as shopping cart interaction, form submissions, and user navigation across pages. It majorly expedited the testing process as the test suite did not depend on an actual browser’s opening and closing time.
Case Study 2: Blog Website Testing
xxxxxxxxxx
const jsdom = require("jsdom");
const { JSDOM } = jsdom;
test("Full DOM Rendering", function() {
const dom = new JSDOM(``);
assert.ok(dom.window.document.querySelector("#container"));
});
In this situation, Jsdom was used for testing a blog website that had dynamic content loading. The aim was to check whether the correct content was fetched and displayed when navigating across different sections. Since Jsdom supports visual formatting through CSS style parsing akin to browsers, it provided a very effective way of testing visual correctness for dynamic content on the website.
Case Study 3: Social Media Application Testing
An interesting use case is a recent project that involved building and testing a social media application. Jsdom again emerged as a valuable asset by creating an environment similar to an end user’s browser where it could test DOM interactions, event handling, local storage handling, and asynchronous API requests.
Google’s engineer Addy Osmani once said, “Code testing proffers a sleek safety net that helps in catching bugs before they cause any damage.” This sentiment rings true across multiple industries and projects which have adopted testing with Jsdom, whether it be e-commerce platforms, blogging sites, or social media applications. The power of Jsdom enables developers to create more robust apps boosted by efficient testing methodologies. For those who wish to delve deeper, the official Jsdom library documentation is an excellent resource to start with.
Conclusion
Delving into the core topic of using Jsdom test environment, it’s important to recognize that this testing utility provides a robust platform for JavaScript unit tests which execute in a simulated browser-like environment. Specifically designed to run server-side, Jsdom presents a comprehensive mock-up of the web standards by implementing DOM Level 2 and 3 APIs, as well as subset elements from CSSOM view, XMLHttpRequest, Web Storage API, and moresource.
import { JSDOM } from ‘jsdom’;
const dom = new JSDOM(`
Hello world
`);
console.log(dom.window.document.querySelector(‘h1’).textContent); //will output ‘Hello world’
The code snippet above demonstrates a simple example of using Jsdom. The `JSDOM` function is used to create a new `JSDOM` instance, which then allows access to a wide range of APIs very much like in a real browser environment.
Imitating a web browser setting for your tests, especially when dealing with complex front end libraries or frameworks like React, Vue or Angular, can be an enormous advantage. This is particularly true where you want to evaluate the integrated functionality of various components interacting with the user or each other.
Employing Jsdom yields many benefits, such as:
– It supports website interactions through Virtual Console. Asynchronous script operations and errors are reported and the APIs expose these messages akin to those that might appear in a typical browser’ console.
– You can use it with other unit testing libraries like Mocha or Jest which brings forth greater flexibility in terms of the testing protocols to follow.
– Its open-source nature means continuous updates, bug fixes, and newer additions are facilitated by a vast contributing community across the globe ensuring that it keeps up with revisions in web standards.
To underscore Richard Stallman’s viewpoint on technology – “We can’t expect every user to become an activist, but we tend to write code that anyone can use in freedom” (Richard Stallman). This mirroring the philosophy of entities like Jsdom which provide open-source libraries that extend these liberties to developers. Choosing the right testing environment could be a challenging decision, yet with comprehensive browser-like utilities like Jsdom, this selection process can certainly be eased.