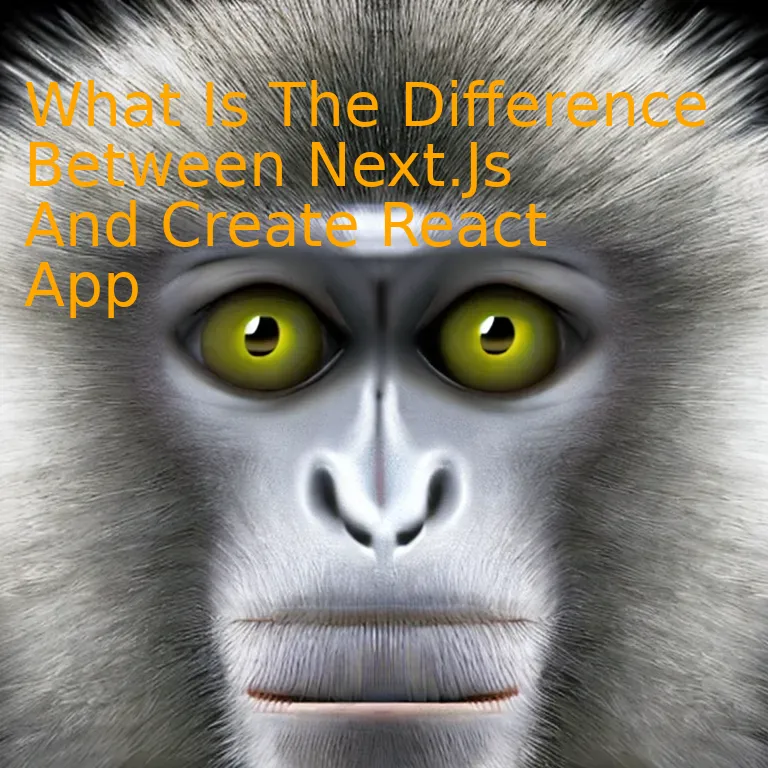
Introduction
The fundamental difference between Next.js and Create React App lies in their rendering capabilities: Next.js offers server-side rendering, greatly improving your app’s SEO performance while Create React App is client-side rendered, focusing more on a seamless user interface.
Quick Summary
One of the key distinction points between Next.js and Create React App (CRA) lies in the framework used, pre-rendering capabilities, data fetching techniques, routing methods, environment setup, and their bundle size optimization mechanisms. An overview of these differences are highlighted in the comparison table below:
html
Feature | Next.js | Create React App |
---|---|---|
Framework Used | Server-side rendered (SSR) applic.plications | For creating single-page applications (SPAs) |
Pre-rendering Capabilities | Supports both static generation (SG) and server-side rendering (SSR) | No pre-rendering. Code runs in the client’s browser |
Data Fetching Techniques | getStaticProps or getServerSideProps functions for data fetching at build time or on each request | Client-side data fetching, not optimized for SEO |
Routing Methods | Built-in file-system based router | Needs third-party libraries like react-router |
Environment Setup | More complex setup intended for larger scale projects | Simplified setup perfect for smaller projects and beginners |
Bundle Size Optimization | Automatic code-splitting to optimize load times | No built-in support for bundle optimization |
Next.js is more of a framework rather than a library, and it’s designed for building server-side rendered applications. Its features such as pre-rendering, automatic code splitting, and optimized data fetching make it stand out significantly when compared with Create React App.
On the other hand, Create React App tends towards single-page applications (SPA). It doesn’t have server-side rendering capabilities and lacks the automatic optimization mechanisms inherent in Next.js. One major strength of CRA over Next.js is its simplicity, making it ideal for small scale projects and beginners.
Finally, it’s important to note that the choice between Next.js and Create React App would largely depend on the scope and needs of your project. The server-side rendering and SEO optimization provided by Next.js might be a decisive advantage for certain projects, while the simplicity and quick setup process offered by Create React App could cater better to simpler, smaller scale applications.
As Dan Abramov, one of the core developers behind React, uncovers: “Next.js offers a lot of functionality out of the box, like routing and server rendering. If you need those, either use Next or be ready to configure them yourself.”
This give insights into understanding that choosing the right tool for a specific need can make all the difference in enhancing productivity and delivering a quality product.
Understanding the Core Features of Next.js and Create React App
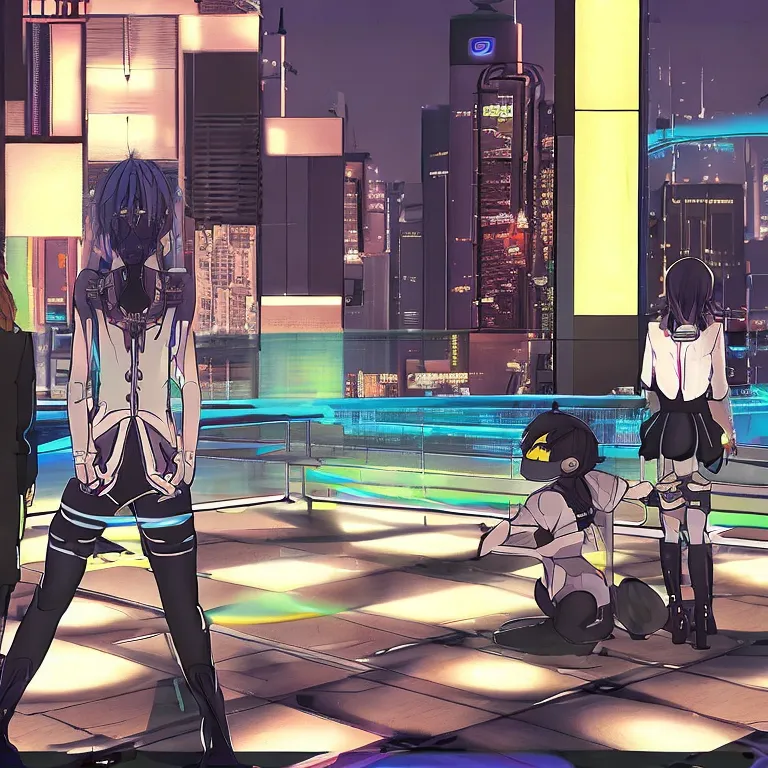
When we delve into the dynamic ecosystem of React, two important frameworks–Next.js and Create React App–often emerge as central players. While both are instrumental in crafting seamless React applications, they have unique attributes that distinguish their operation and deployment.
b. Architecture
Next.js operates on a Universal JavaScript model where rendering takes place at the server-side, enhancing optimization for search engines. Boasting improved performance and faster page loads, it extensively employs automatic server rendering and code-splitting.
// Next.js page example
function HomePage() {
return Welcome to Next.js!;
}
export default HomePage;
In contrast, Create React App deploys a Single Page Application (SPA) architecture where all necessary code is retrieved with a single page load or dynamically loaded as the user navigates around the application. Unlike Next.js, Create React App entirely depends on client-side rendering, resulting in slower initial page loads but enhanced speed on subsequent interactions.
xxxxxxxxxx
// Create React app component example
import React from 'react';
class Welcome extends React.Component {
render() {
return Hello, {this.props.name};
}
}
export default Welcome;
c. SEO Optimization
Given its inherent implementation of server-side rendering(SSR), Next.js pages are indexed by search engines upfront, which improves Search Engine Optimization (SEO). Concurrently, SSR boosts the performance making user experience superior compared to the first-render delay experienced with client-side rendered apps.
However, the client-side rendering employed by Create React App isn’t readily indexed by search engines. To overcome this limitation, SSR would have to be manually configured which can be a complex process.
d. Routing
Contrary to Next.js’s built-in routing mechanism that enables automatic routing based on the file system, Create React App doesn’t present an innate router and necessitates a third-party library such as React Router.
e. Data Fetching
In Next.js, data fetching is managed prior to the render thanks to its inherent functionality, getInitialProps or getServerSideProps. This results in pages that are virtually independent of external data sources at the time of rendering.
On the other hand, Create React App would rely on lifecycle methods for data fetching such as componentDidMount, componentDidUpdate, or the presently emerging `useEffect` hook within functional components.
As Bill Gates once underscored, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” In real-world applications deciding between these two tools will be influenced by the project’s unique requirements regarding aspects such as SEO performance, initial load time, complexity, or need for third-party libraries.
Comparative Breakdown: Development Experience in Next.js vs Create React App
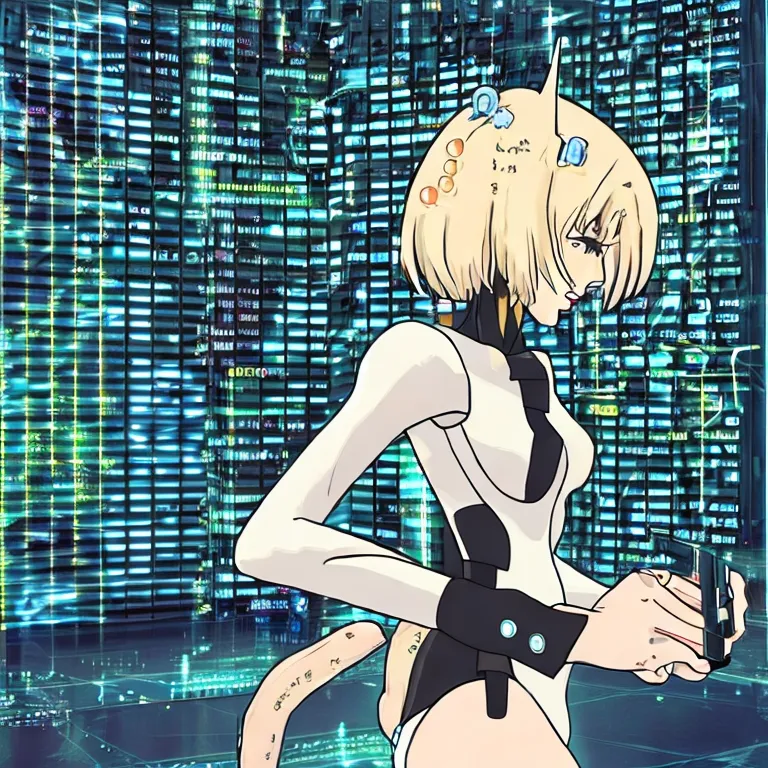
The realm of JavaScript has evolved greatly, with tools like Next.js and Create React App (CRA) marking prominence in fostering development ease. The critical distinction between these platforms revolves around their foundational architecture, aptitude for server-side rendering (SSR), and build optimization features.
Next.js
Next.js is a lightweight framework for static and server-rendered universal JavaScript applications. It’s based on React, Webpack, and Babel.
- Server-side Rendering: Next.js shines in its ability to support Server-side Rendering out of the box. This gives it an edge over CRA when search engine optimization (SEO) is of utmost priority.
- Prefetching: Next.js allows developers to prefetch pages with ease. When links that point to other pages are visible in viewport, those pages get preloaded automatically. This results in a very smooth user experience.
- Static Exporting: You may export your application to static HTML with Next.JS. Great for hosting your website on CDN..
For exmple, a basic feature of Next.js is built-in routing:
xxxxxxxxxx
import Link from 'next/link'
function Home() {
return (
About Us
)
}
export default Home
Create React App
CRA is more straightforward, providing a comfortable environment for learning React, and it’s a viable tool for building a single-page application.
- No setup configuration: With CRA, you can create a new project and start writing code immediately. There’s no need to worry about setting up Babel or Webpack—everything just works.
- Fast Refresh: Another delightful trait of CRA is its hot reloading feature. With Fast Refresh, the app retains the state you’ve built up when tweaking the UI.
For instance, following is a simple use of Create React App:
xxxxxxxxxx
import React from 'react';
import ReactDOM from 'react-dom';
function App() {
return (
Hello World
);
}
ReactDOM.render(, document.getElementById('root'));
Considering their unique features and characteristics, both Next.js and Create React App are important tools in JavaScript development ecosystem. The choice between these two depends largely on the project requirements, team’s expertise, and the specific problems the application aims to solve.
As the pioneering computer scientist Edsger W. Dijkstra rightfully said, “Simplicity is prerequisite for reliability.” Thus, choose the solution that uncomplicates your process while adding maximum value.
Performance Evaluation: Speed and Efficiency of Next.js Compared to Create React App
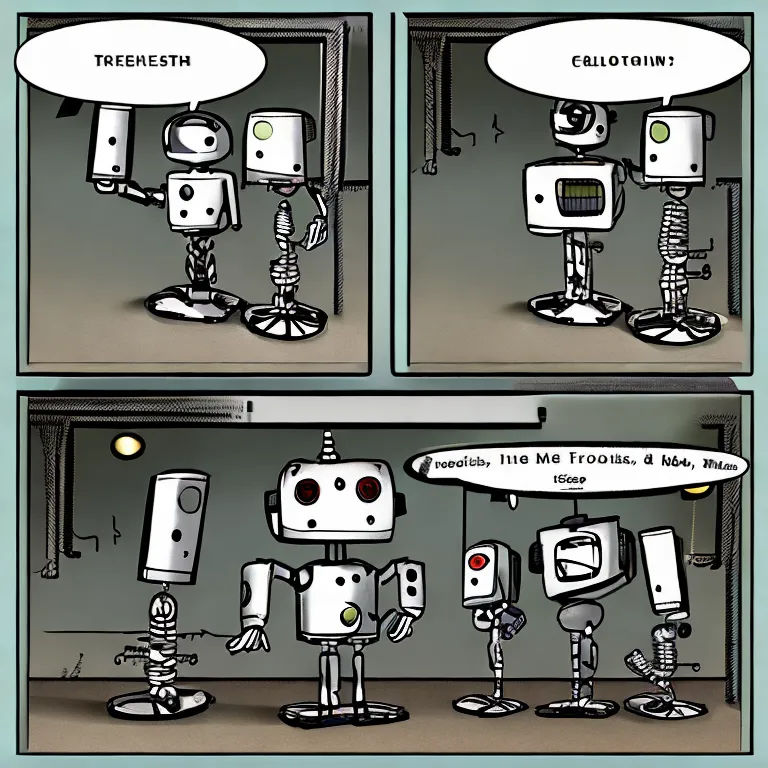
When evaluating the performance, speed, and efficiency of Next.js compared to Create React App (CRA), it’s crucial to keep in mind that these two JavaScript tools have different use cases and feature sets as part of their core design.
Next.js, developed by Vercel, is an open-source framework used for rendering React applications on the server-side by default. This means every page in a Next.js application is rendered from the server initially:
xxxxxxxxxx
export default function HomePage() {
return (
<div>
<p>Hello Next.js</p>
</div>
);
}
This approach improves the overall performance, particularly when dealing with large-scale applications. It significantly enhances Search Engine Optimization (SEO) since server-rendered pages are easier for web crawlers to parse. Additionally, Next.js automatically splits your code into various bundles, so users only load what’s necessary for each page (“Automatic Code-Splitting”).
On the other hand, “Create React App” (CRA) is an out-of-the-box environment for building single-page applications (SPAs) with React. SPAs built with CRA render content client-side:
xxxxxxxxxx
function App() {
return (
<div className="App">
<p>Hello Create React App</p>
</div>
);
}
export default App;
There are significant trade-offs to this method relative to server-side rendering. Client-side rendered apps tend to have longer initial load times because all JavaScript must be loaded and parsed before the app can be interactive. However, once loaded, navigation between pages can be faster due to client-side routing.
It’s important to note that Next.js can also be configured for Static Generation or even hybrid approaches, making it more flexible depending on your application needs. Here is a hyperlinked resource related to this topic.
In the words of Bill Gates, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” Therefore, understanding technological tools like Next.js and Create React App, allows for better development designs that seamlessly integrate into user/developer experiences.
Lastly, when discussing speed and efficiency comparison, one might be faster or more efficient than the other depending on how you use them. But remember both are tools designed for specific scenarios, and their effectiveness can vary greatly depending on how they are utilized.
Exploring Scalability & Flexibility – Can Next.js or Create React App Adapt Better?
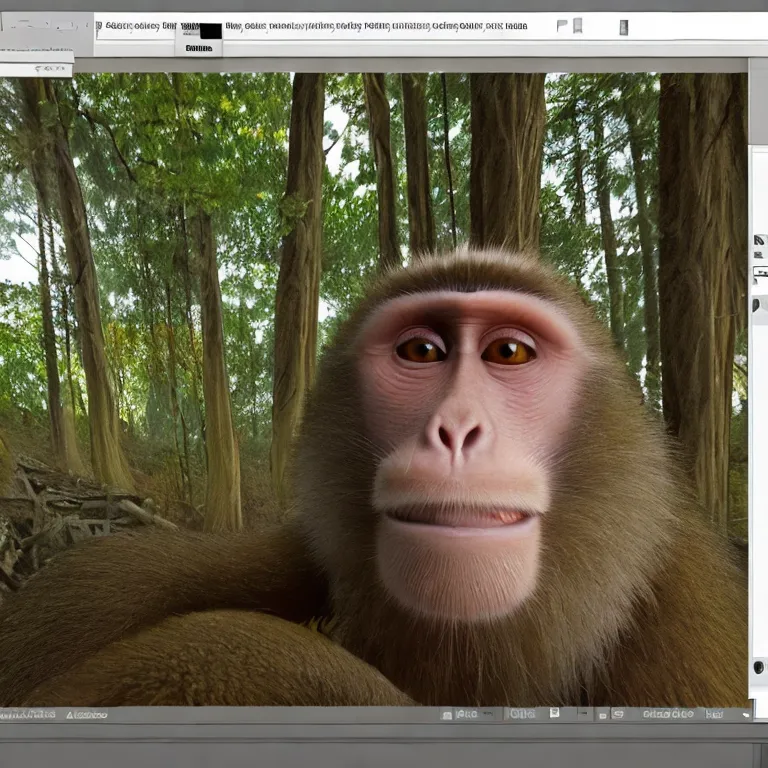
When it comes to exploring scalability and flexibility in web app development, both Next.js and Create React App (CRA) come into play and the choice between them largely pivots around application size and complexity.
Next.js integrates server-rendering and static-exporting features by default and encompasses a better structure for large-scale projects providing scalability right out of the box. The reason why Next.js is oftentimes chosen over CRA is because in addition to facilitating pages to be rendered on the server side, it also permits Static Site Generation(SSG) where HTML pages can be pre-rendered at build time instead of run time, and Incremental Static Regeneration (ISR), where static pages are updated periodically post-deployment. This implicitly provides superior performance as page content is ready even before a request is made. It also suits well for SEO purposes, as pre-rendered pages ensure there’s content on the page when the search engine crawlers do their job.
Let’s consider this small example showing how an application or a particular page can render server-side with Next.js:
xxxxxxxxxx
export async function getServerSideProps() {
// Fetch data from external API
const res = await fetch(`https://...`)
const data = await res.json()
// Pass data to the page via props
return { props: { data } }
}
On the other hand, Create React App optimally serves smaller scale applications with minimum configuration. Built for simplicity and lesser complexity, it doesn’t support server-side rendering or static site generation by default. It’s built over a single HTML document that pulls in styles and scripts, so it may not rank as high in search engines that expect to crawl HTML content because its JS loads only after the whole file is fetched by the browser. However, a CRA rendered app can become more performant by implementing techniques such as code splitting and lazy loading where bundles are created while building for deployment, then portions of the application are only served when they are needed. Even though it requires added steps and external libraries to implement these techniques, it renders CRA flexible according to the needs of the project.
As Bob Martin (Uncle Bob), one of the founding fathers of Agile Software Development says, “The best architecture is the simplest one that solves the problem at hand.” Thus, selecting between Next.js and Create React App has to be carefully weighed based on specific project requirements including scalability, flexibility, SEO requirements, and application complexity.source
Conclusion
Digging deep into the JavaScript ecosystem, one can observe two prominent tools extensively leveraged in web application development: Next.js and Create React App (CRA). Both offer their unique perks with subtle distinctions that set them apart.
Next.js embodies a robust framework for server-side rendering and generating static websites for applications based on React. It curtails the complexities of setting up a full-stack React application, offering favorable scalability and productivity. With an inherent support for server-side rendering (SSR), it allows the application to load faster, enhancing the user experience and search engine visibility.
Next.js offers automatic code-splitting, hot code reloading, built-in CSS, Zero Configuration, and the ability to handle static exports, among other features. The code snippet below demonstrates a basic Next.js setup:
xxxxxxxxxx
import Head from 'next/head'
import Image from 'next/image'
import style from './style.module.css'
export default function Home() {
return (
)
}
Feature | Next.js |
---|---|
Server-Side Rendering | Yes |
Zero Configuration | Yes |
Hot Code Reloading | Yes |
On the other hand, Create React App is a command-line tool devised by Facebook to realize the swift creation of new React.js projects. It automates the process of setting up and configuring your development environment, circumventing issues by bundling all configurations in a single, well-working package. However, unlike Next.js, it is not server-rendered, meaning CRA apps render on the client-side.
Create React App helps developers focus on writing code rather than wrestling with tedious setup procedures, much like Next.Js but falls short in SSR and static-exporting capabilities.
A basic set up using Create React App can look something like this:
xxxxxxxxxx
import React from 'react';
import logo from './logo.svg';
import './App.css';
function App() {
return (
);
}
export default App;
Feature | Create React App (CRA) |
---|---|
Server-Side Rendering | No |
Zero Configuration | Yes |
Hot Code Reloading | Yes |
As per the renowned programmer Jamshid Asadzadeh, “With technology, it’s not about which is universally superior. It’s about understanding the purposes, benefits, and shortcomings to decide what’s the right fit for your specific project.”
Your selection between Next.js or CRA should be determined by the needs of your project. You might lean towards Next.js if you require good SEO results, server-side rendering or Static Site Generation. If your project doesn’t have strong SEO requirements and is more about creating a fast Single Page Application (SPA) without the need for server-side rendering, CRA would be well-suited.