Introduction
In TypeScript, the ‘as const’ construct is employed to indicate that a variable’s value or an object’s property is a constant and should not be changed, enhancing code stability by offering strict type checking to avoid unintentional modifications.
Quick Summary
The `
as const
` assertion in TypeScript informs the type checker to consider the literal constant value of variables, reducing potential mutations in your code.
A table detailing its usage and effects can be demonstrated as:
Without as const | With as const |
---|---|
typescript let arr = [1, 2, 3]; arr.push(4); |
typescript let arr = [1, 2, 3] as const; // Error! push does not exist on type readonly number[]; arr.push(4); |
Effects | Effects |
Mutability allowed: You can add elements to the array. | Immutability enforced: Trying to modify causes a compile-time error. |
This example demonstrates the enforceability of immutability when using
xxxxxxxxxx
as const
. The declaration `as const` makes the JavaScript Object or Array immutable. This concept is aligned with one of the fundamental principles of functional programming – immutability, making your code more predictable and robust against bugs caused by side effects.
Breaking down the table:
1. Without using the `as const` syntax in TypeScript, the declared variable (in this case an array) can be mutated (by adding in a new element).
2. However, once we use `as const`, this leads to compile-time errors due to the enforcing of immutability, hence restricting any changes to the array.
As quoted by Rob Pike, “Data dominates. If you’ve chosen the right data structures and organized things well, the algorithms will almost always be self-evident. Data structures, not algorithms, are central to programming.” This emphasises how `as const` can help provide a robust structure for your code by enforcing immutability.
Use cases of `as const` in TypeScript include:
– When you want to prevent unexpected changes in your data objects or arrays.
– When you need to efficiently manage state, such as in Redux or NgRx store, where `as const` could be helpful to keep the actions immutable.source
In essence,
xxxxxxxxxx
as const
is a handy tool in a TypeScript developer’s arsenal that increases the predictability and maintainability of their programs by enforcing immutability on their variables.
Understanding the ‘as const’ Syntax in TypeScript
xxxxxxxxxx
as const
syntax uniquely stands out for its practical utility in Type assertion.
The
xxxxxxxxxx
as const
is a key syntax in TypeScript, referred to as a ‘const assertion’. It is a type assertion way that lets TypeScript treat variables as immutable.
Type Script Syntax/Keyword | Description |
---|---|
xxxxxxxxxx as const |
This assertion indicates immutability |
A typical usage of const assertions would often see a literal expression being enshrined with the
xxxxxxxxxx
as const
syntax. The most driving appeal of the
xxxxxxxxxx
as const
syntax is in how TypeScript interprets literals to create the broadest or narrowest types possible. This process alters under the influence of
xxxxxxxxxx
as const
, creating the narrowest type possible for the literal:
xxxxxxxxxx
let ordinaryTuple = ['Hello', 10]; // type of ordinaryTuple becomes (string | number)[]
let constTuple = ['Hello', 10] as const; // the type of constTuple becomes readonly ['Hello', 10]
Notably, with reference to variable mutability, objects and arrays transformed using
xxxxxxxxxx
as const
become read-only. Any attempts made towards modification, deletion, or addition will result in TypeScript throwing an error.
To quote Anders Hejlsberg, the original designer and lead architect of TypeScript, “It’s about describing the shape of what something must look like but not really saying much about what it can do”
Therefore,
xxxxxxxxxx
as const
is effectively used when the programmer wants to ensure a particular structure or value of an object/array in their TypeScript code remains constant.
This novel feature results in safer TypeScript code by injecting immutability and facilitating precise type-checking. Optimal utilization of
xxxxxxxxxx
as const
fosters bug avoidance even before runtime. Therefore, the
xxxxxxxxxx
as const
syntax enjoys its distinguished place in TypeScript for ensuring enhanced code safety and reliability.
The detailed application of
xxxxxxxxxx
as const
may be further explored at the TypeScript’s official documentationhere.
Exploring Utilization of ‘as const’ in TypeScript
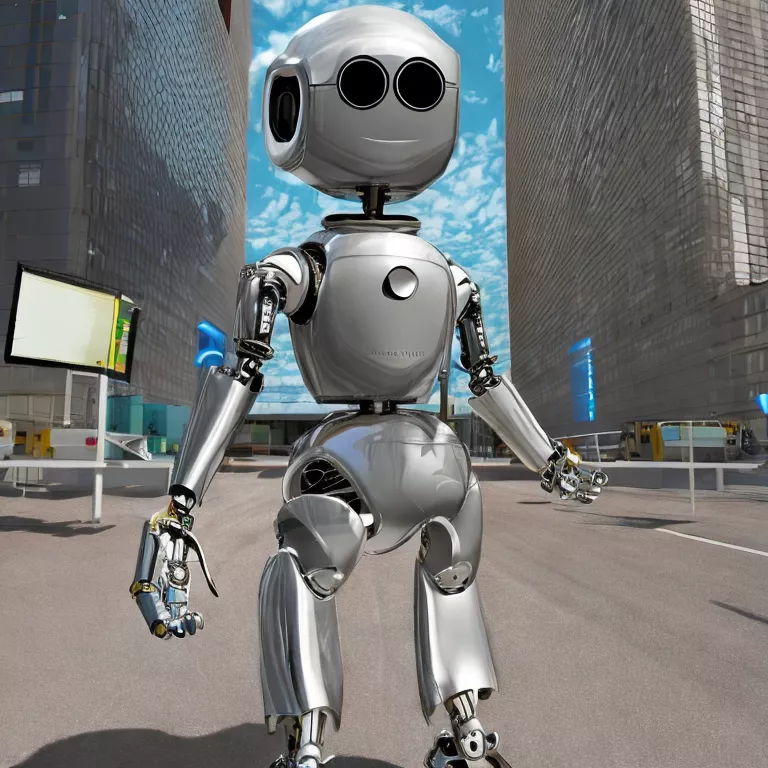
The
xxxxxxxxxx
as const
construct in TypeScript, often referred to as const assertions, is a way to signal to the TypeScript compiler that an object or array should be considered deeply readonly. This will prevent any mutations, even those typically allowed through type widening. The utilization of
xxxxxxxxxx
as const
may apply for various scenarios which we will unpack below.
Data integrity maintenance:
Using
xxxxxxxxxx
as const
can preserve the literal values of variables at runtime, maintaining data integrity.
For instance, consider a scenario where you want to ensure the specific color codes to be used in a project don’t get changed unintentionally somewhere in the codebase:
xxxxxxxxxx
const colorCodes = {
red: '#FF0000',
green: '#008000',
blue: '#0000FF'
} as const;
After this, attempting to change any of these values would result in a compile time error.
Preventing Type Widening:
TypeScript’s “type widening,” behavior expands literal types to their more generic version. For example,
xxxxxxxxxx
let foo = 42;
will give ‘foo’ a type of number, not 42.
Using
xxxxxxxxxx
as const
, programmers can stop this behavior, keeping literal types intact:
xxxxxxxxxx
let foo = 42 as const; // 'foo' is of type 42, not number
Working with Enums:
When leveraging enum-like objects,
xxxxxxxxxx
as const
can provide similar benefits of ‘enum’ without its drawbacks.
Enum can create additional Javascript during compilation but using an object
xxxxxxxxxx
as const
just gives us the key-value pairs we defined:
xxxxxxxxxx
const DaysOfWeek = {
Monday: 1,
Tuesday: 2,
//...
} as const;
References:
- TypeScript 3.4 Release Notes – TypeScript Docs
- Const Assertions in Literal Expressions in TypeScript – Marius Schulz’s Blog
As the renowned developer Tony Hoare once said, “There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies, and the other is to make it so complicated that there are no obvious deficiencies.” The usage of
xxxxxxxxxx
as const
aligns with making software simple, clear and robust by ensuring type integrity and reducing potential for bugs or misunderstandings down the line.
Unraveling Use Cases for the ‘as const’ Statement
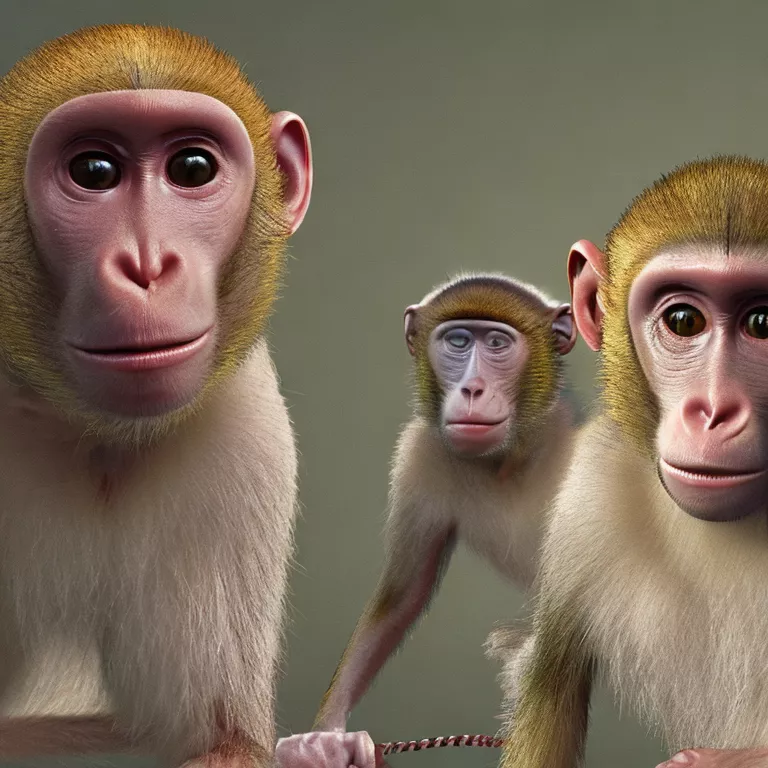
The
xxxxxxxxxx
as const
assertion in TypeScript is a potent tool for developers, providing enhanced control over making specific expressions or variables read-only and immutable. It exists to support scenarios where we aim to restrict the type to the literal type of its value, not its wider generic equivalent. By enabling
xxxxxxxxxx
as const
, TypeScript treats our data as a unique specific localStorage that can result in higher precision while programming.
For example:
xxxxxxxxxx
let arr = ['This', 'is', 'an', 'immutable', 'array'] as const;
Here, TypeScript interprets this array (arr) as an immutable tuple of five strings instead of simple string[]. Each item in the Tuple gets its type from the corresponding position in the Array. This improves code predictability and enhances data security.
But what are some noteworthy use cases?
1. Defining Gravitational Constants: Observe difficulties programmers encounter when working with physical constants. The gravitational constant on earth never changes. Hence, we need it declared strictly once in order not to be changed accidentally within the code.
xxxxxxxxxx
const g = 9.81 as const; // Earth's gravitational constant
2. Enumerations and Configuration Objects: Consider a situation where you have pre-defined strings or sets of values that should remain untouched throughout your application. Here,
xxxxxxxxxx
as const
can be a great help.
xxxxxxxxxx
const CardSuites = {
SPADES: 'spades',
HEARTS: 'hearts',
DIAMONDS: 'diamonds',
CLUBS: 'clubs'
} as const;
3. Color Palettes: Creating object literals with set properties, like a color palette used across an application, would be another potential use case of
xxxxxxxxxx
as const
.
xxxxxxxxxx
const COLOR_PALETTE = {
PRIMARY: '#0000FF',
SECONDARY: '#008000'
} as const;
In the words of Tony Hoare, “Inside every large program, there is a small program trying to get out.” The
xxxxxxxxxx
as const
assertion remarkably simplifies your TypeScript journey by providing precision and control over data immutability. For modern developers aiming to write robust, optimized TypeScript code, understanding and utilizing the power of
xxxxxxxxxx
as const
is therefore essential.
You can find more info in the official [TypeScript documentation](https://www.typescriptlang.org/docs/handbook/release-notes/typescript-3-4.html#const-assertions) on the subject.
In-depth Look at ‘as const’: Enhancing Coding Efficiency in TypeScript
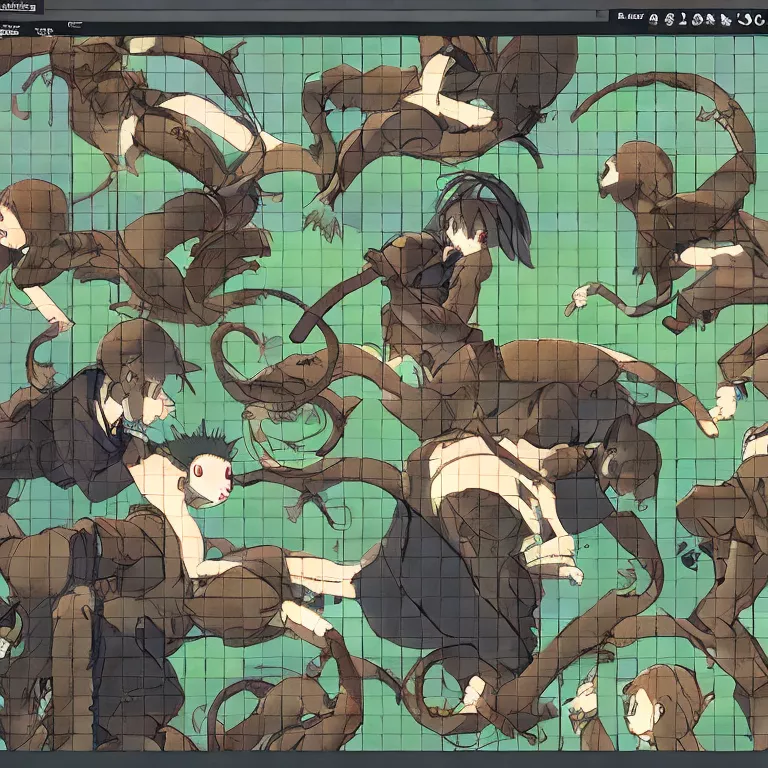
TypeScript offers powerful utilities to developers, one of which is the addition of the `as const` assertion signature. Primarily, it serves two significant tasks in TypeScript that support rendering code more readable, efficient, and effortless to debug.
The `as const` assertion helps to create constant literals in TypeScript:
typescript
let exampleAsConst = { name: ‘John’, age: 25 } as const;
Its first utilization occurs in creating deeply read-only literal types. In TypeScript, the `readonly` label anticipates alterations on an object’s properties. However, this characteristic does not extend to nested objects, potentially leading to inadvertent modifications. Fortunately, using `as const` transforms nested objects into immutable objects, enhancing data integrity across your application.
typescript
let exampleNestedConst = {
personalDetails: {
name: ‘John’,
age: 25
}
} as const;
Given its ability to provide deep-level immutability, `as const` bolsters and secures TypeScript applications against unintended side-effects from nested property changes.
Secondly, `as const` promotes tuple usage over arrays. Arrays bring flexibility but may lead to unexpected results since TypeScript accommodates mixed array types by assigning common values (often, the ‘any’ type) and inferring it to all elements.
typescript
let arr = [‘John’, 25]; // TypeScript considers this string[] | number[]
Rather, with `as const`, TypeScript recognizes each unique value, forming a tuple.
typescript
let tup = [‘John’, 25] as const; // This becomes [“John”, 25]
This enables static type checking for each element in the tuple, providing improved control over data structures. Consequently, adopting `as const` prompts proactive adjustments when data structure changes are required, keeping codebase fidelity intact.
Combining these functionalities amplifies development efficiency, making `as const` a powerful extension to TypeScript’s toolbelt. This phrase reflects a sentiment from the popular programming author, Robert C. Martin: “The only way to make the deadline—the only way to go fast—is to keep the code as clean as possible at all times.”(source) And indeed, using `as const` is one strategy towards achieving this clean-code goal in TypeScript.
Conclusion
Immersing deeply into TypeScript’s rich feature set, we come across the intriguing and powerful ‘
xxxxxxxxxx
as const
‘ assertion. This particular construct is employed when we desire to enforce that literals are taken ‘as is’ in their most immovable state. Fundamentally, it shouts out that our variables are readonly, indisputably non-alterable, thus preserving the original form of values during runtime.
Here’s an illustration:
xxxxxxxxxx
let myObject = {
name: "Coding Guru",
age: 30
} as const;
Attempting to modify ‘
xxxxxxxxxx
myObject.name = "Code Master";
‘ tosses an error because
xxxxxxxxxx
'as const'
has reinforced
xxxxxxxxxx
'myObject'
to be a readonly entity.
Utilizing ‘
xxxxxxxxxx
as const'
‘ exploits TypeScript’s functional prowess. It permits programmers to design code structures with definitive types and enhances maintainability since any unauthorized modification is literally barricaded. As a result, bugs triggered by unintended type changes can be circumvented successfully.
Furthermore, consider arrays. An array declared with ‘
xxxxxxxxxx
as const
‘ won’t tolerate any content transformation. Visualize this scenario:
xxxxxxxxxx
let superheroNames = ["Batman", "Superman", "Iron Man"] as const;
superheroNames[1] = "Spiderman"; // Throws an error - the array is immutable!
TypeScript shines in such circumstances, fortifying your code against accidental mutations.
Table showcasing difference between ordinary variables and those implemented with ‘
xxxxxxxxxx
as const
‘:
Type of variable | Can be altered? |
---|---|
Normal Variable | Yes |
Variable with ‘as const’ | No |
As a final point, recall Robert C. Martin (Uncle Bob) stating that “The only way to go fast is to go well”. The ‘
xxxxxxxxxx
as const
‘ sytnax in TypeScript achieves exactly this: a well-structured and bug-resistant codebase. Therefore, understanding ‘
xxxxxxxxxx
as const
‘ unleashes a robust protective layer for our TypeScript applications.