Introduction
When exploring Typescript, if you are experiencing an issue with Useref not being assignable to type Legacyref
Quick Summary
In the context of TypeScript and React, especially when it comes to leveraging hooks like `useRef`, a common issue or error faced by developers can be something similar to “
Typescript - Not Assignable To Type LegacyRef<HTMLDivElement>
“. This typically means that TypeScript is encountering problems with the type assignment of a ref, specifically concerning an HTMLDivElement.
Consider, for instance, this following table:
html
Terms | Description |
---|---|
TypeScript | A statically typed superset of JavaScript, providing type safety. |
useRef | A built-in hook in React used to access DOM nodes or React elements directly. |
LegacyRef | A type category in TypeScript/React accommodating strings (legacy context) and functions. |
HTMLDivElement | A standard object interface representing HTML <div> element. |
Essentially, TypeScript strives to maintain correct typing in your code. With
xxxxxxxxxx
useRef
, you’re dealing with mutable refs where the
xxxxxxxxxx
.current
property is mutable and can hold a reference to a DOM element, hence requiring well-mapped type definition.
When you see an error “
xxxxxxxxxx
Not Assignable To Type LegacyRef<HTMLDivElement>
“, it indicates that you’re trying to assign a reference to something that does not match LegacyRef category. Here, you might be assigning a string ref or a function in place of what should have been an instance of
xxxxxxxxxx
HTMLDivElement
.
A potential solution to this error is annotating the useRef with the correct type (here: HTMLDivElement). See this TypeScript and React compatible usage of useRef:
html
xxxxxxxxxx
const ref = React.useRef<HTMLDivElement | null>(null);
You can also use `
xxxxxxxxxx
React.createRef<HTMLDivElement>
` to create a mutable ref object. This version is more commonplace in Class Components than in Functional ones where `
xxxxxxxxxx
useRef
` is typically leveraged.
Always remember, as the celebrated computer scientist Edsger W. Dijkstra once said, “If debugging is the process of removing software bugs, then programming must be the process of putting them in.” Being aware of different type-definitions and how useRef works with HTML elements in TypeScript will help you minimize these errors and efficiently debug them when they occur.
Understanding the Basics of Useref in TypeScript
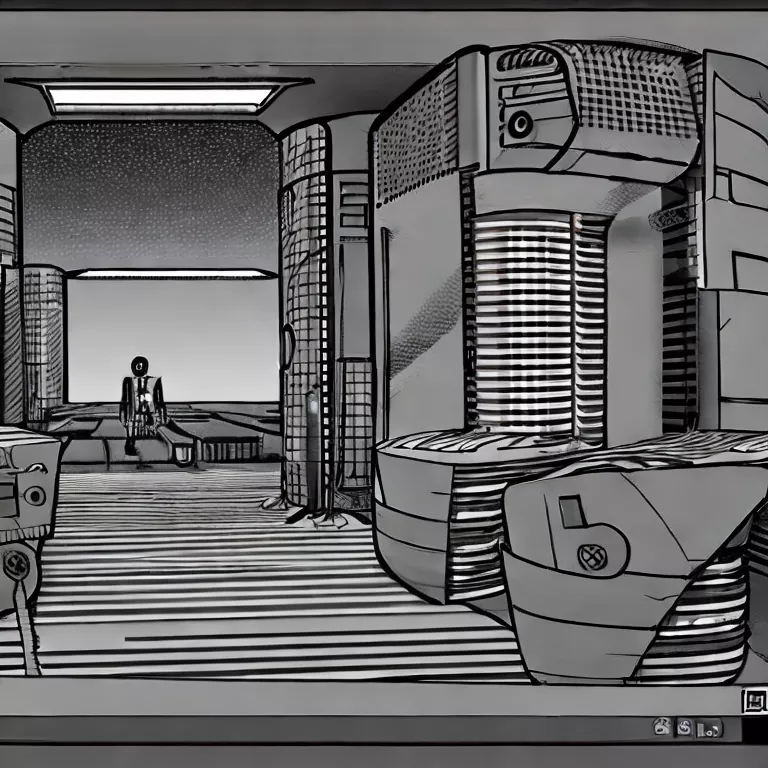
In the land of TypeScript development, hooks provide a powerful and convenient way for managing state and side effects in functional components. Certainly, one of the more intriguing concepts you are going to encounter is
xxxxxxxxxx
useRef
.
The
xxxxxxxxxx
useRef
hook is comparable to
xxxxxxxxxx
createRef
for class components. It typically serves as a way of referencing particular HTML elements. However, it can also hold mutable values in its
xxxxxxxxxx
.current
property.
A usage case where an error “Useref Typescript – Not Assignable To Type Legacyref
xxxxxxxxxx
let myRef = useRef();
In this case, TypeScript doesn’t have a specific HTML element that it’s ref attached to hence isn’t sure of its type.
To solve the issue, we should always ensure proper typing when using
xxxxxxxxxx
useRef
in TypeScript to prevent ambiguities in our code:
xxxxxxxxxx
let myRef = useRef<HTMLDivElement | null>(null);
The above example correctly types the
xxxxxxxxxx
useRef
by explicitly setting its type to either
xxxxxxxxxx
HTMLDivElement
or
xxxxxxxxxx
null
.
As a TypeScript developer, understanding these concepts helps you write clean, efficient, and less buggy code.
Pondering on the words of Steve McConnell, author of Code Complete: “Good code is its own best documentation. As you’re about to add a comment, ask yourself, ‘How can I improve the code so that this comment isn’t needed?'” Therefore, while
xxxxxxxxxx
useRef
might get complicated, remember good code often starts with clarity, and in TypeScript, good typing is part of that story.
Resolving ‘Not Assignable to Type LegacyRef’ Error in TypeScript
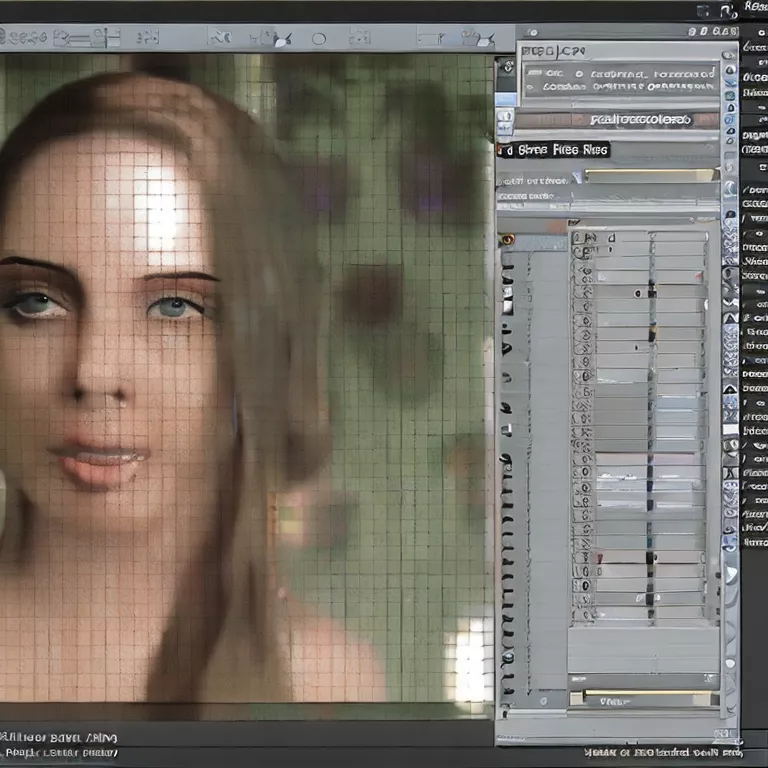
The ‘Not Assignable to Type LegacyRef’ error is a common issue that TypeScript developers come across particularly when they use the `useRef` hook in React. This error essentially surfaces when an attempt is made to assign an object that does not conform to the type declared by the `LegacyRef` interface.
Unpacking this further, it is worth understanding that `useRef` is designed to persist value between re-renders of a component, and often used to maintain a reference to an HTML DOM element.
So, if you are trying to fix this `’Not Assignable to Type LegacyRef
Here is an example where TypeScript complains:
html
const refDiv = React.useRef
return
In above situation, properly initializing the `useRef` should rectify the error. The initial value should be set to null and followed by the check to ensure that the current property of the ref is not null before using it. It allows to signal TypeScript that there is explicit null checking happening. Have a look at the corrected code snippet below:
html
const refDiv = React.useRef
return
Always enforce explicit null check before using the DOM element reference prevents unintentional `null` issues and thus avoiding the ‘Not Assignable to Type LegacyRef’ error.
Eric Elliott, a renowned JavaScript expert, once said, “TypeScript catches several types of basic errors (like not calling functions the right way) so you can avoid lots of pitfalls up front and prevent bad JavaScript from ever being shipped to a browser.” Here, understanding the type system, particularly in relation to `LegacyRef`, is the key for clean and error-free code.
Practical Solutions for ‘Not Assignable to Type Legacyref‘ Issue
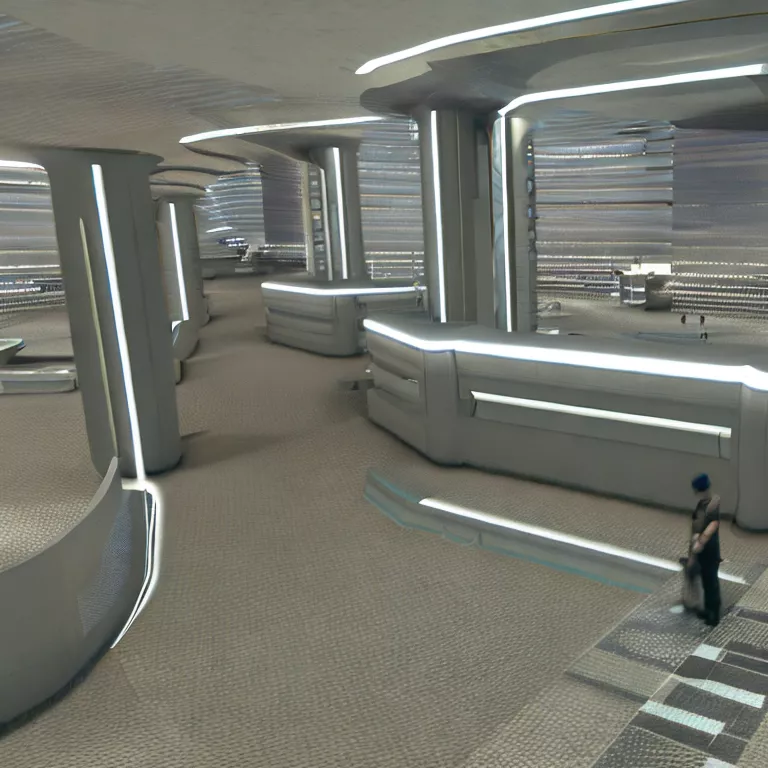
Diving into the TypeScript error relating to “‘Not Assignable to Type Legacyref
At a basic level, the `LegacyRef` type error can occur when React’s `useRef` hook is used without specifying the correct type. This could lead to potential issues where TypeScript cannot accurately infer the element type.
Highlighting the issue
Consider a typical scenario where you want to use a ref on a div tag:
const ref = useRef();
This would trigger the error since TypeScript isn’t sure which type of HTML element you’re referencing. The solution is to inform TypeScript of the type of the element directly:
const ref = useRef
Here, `HTMLDivElement` tells TypeScript that the ref refers to a `
Moving forward: Deeper Insight
However, while defining `HTMLDivElement` seems pretty straightforward, there are situation where using `null` might not be a viable option.
For instance, when applied to class-based components with types defined, setting initial null value may yield the same error message as TypeScript expects values of the same type. In such scenario, typing the ref with `LegacyRef` to accommodate the various possibilities should help resolve this issue:
interface Props {
innerRef: LegacyRef
}
function MyComponent(props: Props) {
return
;
}
This way, the code explicitly states that `innerRef` attribute can either be a string, a function, or a RefObject, allowing TypeScript to allocate space for an HTMLDivElement.
Code elegance and best practices
Explicit typing is the soul of programming languages such as TypeScript. When applied buttoned-down with best practices, `LegacyRef` can be navigated without any hurdle.
In particular, Tim Berners-Lee once quoted, “Anyone who slaps a ‘this page is best viewed with Browser X’ label on a Web page appears to be yearning for the bad old days, before the web, when you had very little chance of reading a document written on another computer, another word processor, or another network.” Translated to our context, always code with various scenarios in mind – consider different type possibilities and account for them in your initial setup to reduce refactoring down the line.
Once these practical solutions are comprehensively understood and seamlessly implemented, TypeScript can bring about greater efficiency, scalability and organization to your overall coding ecosystem. For further understanding, you may refer to the TypeScript Handbook
xxxxxxxxxx
TypeScript Handbook Functions
.
Exploring Real-World Cases: Useref Typescript and Legacyref Not Assignability
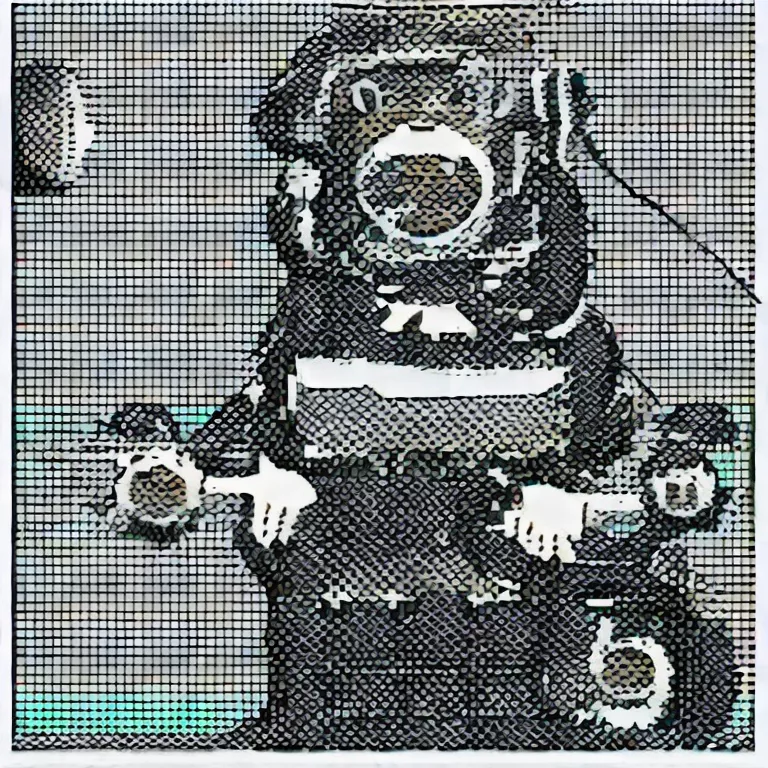
Adventuring into the intriguing realm of TypeScript, we often bump into an interesting case of ‘useRef’ not being assignable to type ‘LegacyRef’. This issue commonly comes to the surface when using React with TypeScript, where a warning is thrown stating ‘Useref Typescript – Not Assignable To Type Legacyref
TypeScript’s `useRef` hook, which creates a persistent reference object that possesses a `.current` property, is frequently utilized for handling DOM elements in functional components. A handy way of preserving mutable values across component re-renders indeed! A typical usage scenario might be:
typescript
let myRef = React.useRef
Meanwhile, `LegacyRef`, another concept from the React and TypeScript world, is a special kind of reference that can be either a callback or a string, besides the standard ref object we’re familiar with.
Through further analysis, problems arise in TypeScript due to strict type checking, which disallows you to assign a `useRef` to a type `LegacyRef`. It is primarily because `LegacyRef` is a union type that combines `string | ((instance: HTMLDivElement | null) => void) | RefObject
Consequently, when you attempt to employ the `useRef` hook in place of a `LegacyRef` type, TypeScript fails to compile the code and churns out an error stating that ‘UseRef Typescript – Not Assignable To Type Legacyref’. Bottom line, this points out a mismatch between the expected type and assigned value based on our TypeScript configuration settings.
Dissecting the issue doesn’t mean we’re left without solutions. A prominent solution to this conflict could be adjusting the type of the `LegacyRef` variable to `MutableRefObject`, which matches the type returned by `useRef`. Here’s a code snippet for clarity:
typescript
let myRef: React.MutableRefObject
Alternatively, you can resort to downcasting the `useRef` value to any before assigning it to the `LegacyRef` variable or disabling the TypeScript checker for that specific line. Both methods may not sound perfect, but they’ll get the job done in most cases.
In relation to [Douglas Crockford](https://en.wikipedia.org/wiki/Douglas_Crockford), the American programmer known for his contributions to JavaScript, and quoting him: “JavaScript is the world’s most misunderstood programming language”, we can add that TypeScript, as a superset of this commonly used language, needs an understanding no less deep and precise.
Conclusion
In the dynamic realm of TypeScript, useRef and its association with the non-assignable issue of ‘LegacyRef
Harnessing the power that runs behind TypeScript technologies such as useRef, it is quintessential to dissect the root cause of LegacyRef issues and devise innovative solutions.
Title: useEffect Hook and LegacyRef Type Non-assinability in TypeScript
Understanding TypeScript’s useRef hook presents its own set of challenges. This difficulty escalates when one stumbles upon the obstacle of the LegacyRef type error where you can’t assign useRef to a LegacyRef HTMLDivElement.
The crux of the matter lies in the mismatch between the useRef returned object and the expected LegacyRef. useRef returns a mutable ref object with current property while LegacyRef could potentially be a string. Moreover, strings are no longer valid as refs in modern versions of React (source). This discrepancy is the root cause that pushes the “\”is not assignable to type ‘LegacyRef
xxxxxxxxxx
// Example
const refObj = React.useRef(null);
let element:React.LegacyRef = refObj; //TypeScript error
To tackle this, a plausible solution is changing the type from LegacyRef to MutableRefObject, which aligns with the return type of useRef hook:
xxxxxxxxxx
// Solution
let element:React.MutableRefObject = refObj; // No error
By impeccable integration of correct types, one can resolve useRef complications thereby paving the way for smooth, error-free code development.
As Bjarne Stroustrup, inventor of C++ programming language pointed out – “There are only two kinds of languages: the ones people complain about and the ones nobody uses”. In essence, every language or technology has its quirks and challenges, it is how developers navigate through these hiccups that determine successful execution.
The LegacyRef issue in TypeScript is a fascinating topic necessitating deeper understanding of useRef, TypeScript types, and their correct utilization. Overcoming such hurdles is paramount to leveraging useRef and TypeScript to create potent, scalable solutions.