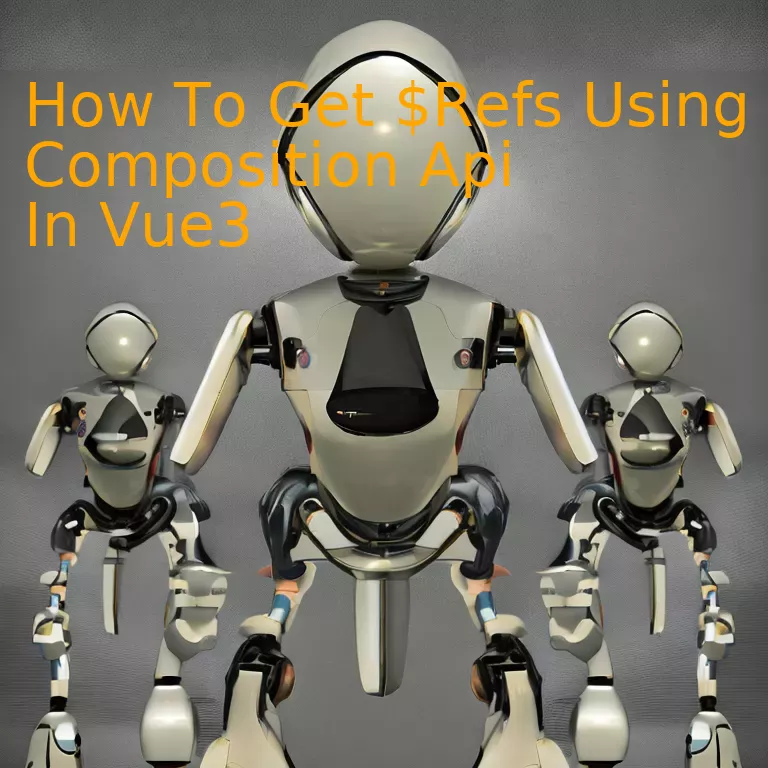
Introduction
Leveraging the Composition API in Vue3 efficiently paves the path for managing $refs, a transformational shift that bolsters operational proficiency in web development projects, offering unique scalability and adaptability unprecedented in the realm of digital platform architecture.
Quick Summary
In Vue3, the Composition API can be utilized to obtain $refs. The process of obtaining $refs using Composition API in Vue3 consists of two essential stages:
1. Registering a ref in the template
2. Accessing that ref in the setup method
The stages can be laid out in a table format for quick reference:
Stage | Description |
---|---|
Register a ref in template | This is an action done in the template section of the component where you attribute a ref keyword to the HTML element you desire access to. |
Access the ref in setup method | Visible within the Component’s setup() function, refs are accessible after they’ve been rendered and therefore should be accessed inside onMounted() or subsequent lifecycle hooks. |
Let’s elaborate further.
Firstly, registering a ref in the template: To get a hold of an HTML element in the DOM, we need to assign it a ref. By doing this, we instruct Vue that we want direct access to this specific item. For instance,
<div ref="myDiv">Hello Vue</div>
Here “myDiv” is a ref declared and assigned to a div. You may replace “myDiv” with any identifier of your preference.
Secondly, accessing the ref in the setup method: In the composition API, we don’t have direct access to $refs inside the setup(). Therefore, we use manual reactive references i.e., we use the ‘ref’ function from Vue.
However, remember that the DOM might not yet be rendered when setup() executes. Therefore, we should access refs inside onMounted() or any subsequent lifecycle hook which guarantees that the specific ref’s content has already been rendered. Inside the onMounted hook, you can access the assigned ref like so:
// inside setup() import { ref, onMounted } from 'vue' setup() { const myDiv = ref(null) onMounted(() => { console.log(myDiv.value) }) return { myDiv } }
Through the Composition API in Vue3, obtaining $refs becomes a more streamlined and tractable process, thus offering easy antenna to essential reactive instances within your application architecture.
As Brian Kernighan wisely stated, “Controlling complexity is the essence of computer programming”. This could not be truer in the quest for controlling $refs in Vue’s latest Composition API.
References: Vue Composition API – Template Refs
Exploring $Refs in Vue3 Composition API: A Comprehensive Guide
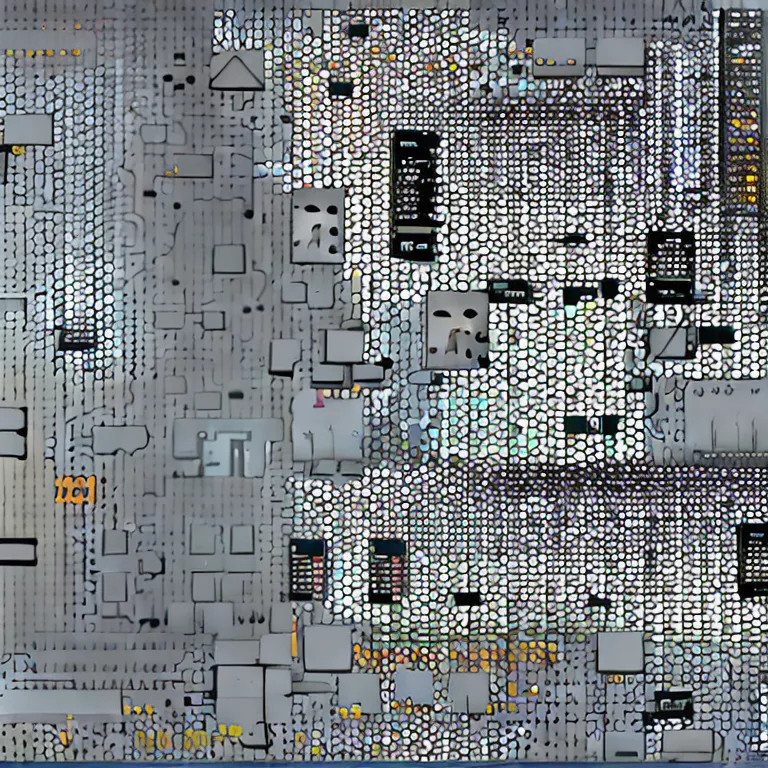
“$Refs” plays a significant role within Vue3’s Composition API by providing direct access to DOM elements or child components. This dynamic attribute allows for customization and enhanced interactions by manipulating the underlying markup structure.
First, let’s take a look at what “$refs” exactly is. Essentially, “$refs” are an object that stores referenced DOM elements or child components as properties. Even though they’re not reactive, these references provide convenient access points for reading values, triggering events, or manipulating DOM in complex scenarios.
To get $refs using Vue3 Composition API, you need to employ the `ref` method from `vue`. Here’s a simple illustration:
import { ref, onMounted } from 'vue' export default { setup() { const myRef = ref(null) onMounted(() => { console.log(myRef.value) # It will print the DOM node of the element }) return { myRef } }, }
In this code snippet, we create a reference (`myRef`) using the `ref` method, and then log it out in the `onMounted` lifecycle hook. We can use `myRef` in our template like so `
`, which would then apply the ref directly to the DOM element.
Note: When working with $refs, keep certain principles in mind:
– Hooks such as `onMounted` or `onUpdated` ensure the presence of $refs.
– Lifecycles’ asynchronous nature means that $refs won’t be available during `setup`.
– Unlike data properties, refs aren’t reactive.
Thomas Steiner remarked in a keynote, “With great power comes great responsibility.” This certainly applies when wielding tools like $refs because while powerful, their misuse could lead to inefficient code or unpredictable app behavior.
Remember, $refs aren’t the first go-to for updating a component’s state or interacting with child components; stick to Vue’s reactivity system and props/events. Use $refs sparingly as an optimization technique or when other options fail.
Moreover, using `vuejs developers guide`[1] it’s possible to explore other aspects of Vue3 Composition API to leverage full potential of $refs. Hence, this provides overall insights into getting $refs using Composition API in Vue3.
Enhancing Web App Functionality with $Refs and Vue3 Composition API
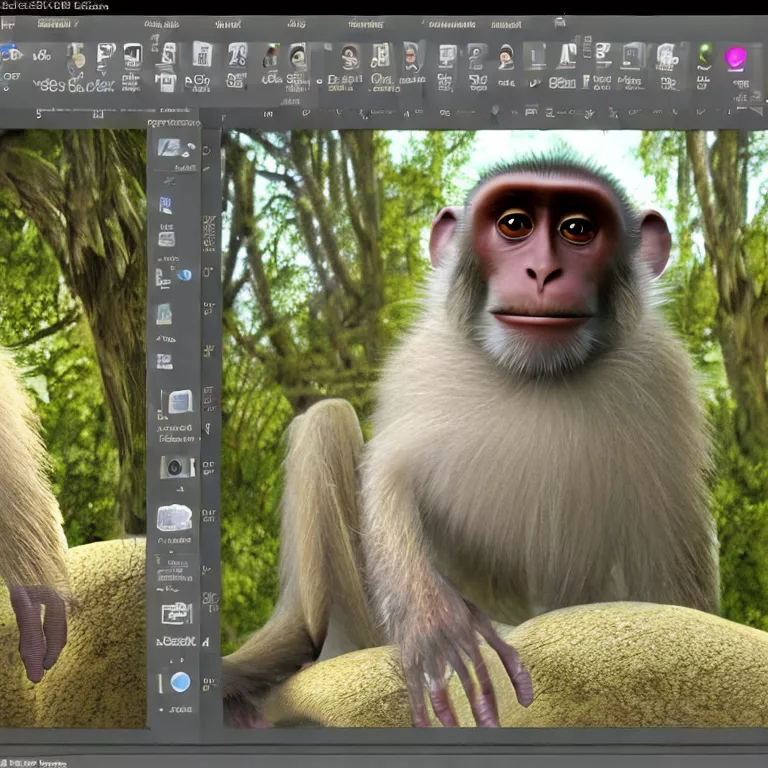
The Vue3 Composition API provides an innovative solution to enhancing the functionality of your web application, particularly in handling $refs. The use of `$refs` is crucial especially when trying to manipulate DOM elements or components directly, without necessarily depending on data properties of Vue instance. In Vue 3, this can be achieved efficiently using the composition API. Given that this strategy of coding might seem a little bit complicated, but once mastered, it renders ease in organizing and rendering reusable code pieces, thereby elevating your web app’s functionality.
So, how do you get `$refs` using the composition API in Vue3? Follow through for an explicit explanation:
Firstly, in Vue 3, the best practice to create `$refs` within the composition API is through the utilization of the `ref()` function provided by `vue`. This comes handy particularly where you need to access a component reference or a raw DOM element.
Here is how;
In normal circumstances, we declare `$refs` inside the `template` part, as shown below:
<template> <div ref="myDiv">Hello World</div> </template>
And then inside our script section, we would access this DOM node like this:
export default { mounted() { console.log(this.$refs.myDiv) } }
However, if we want to use this in the composition API, here is how to do it:
Import `ref` from `vue`:
import { ref, onMounted } from 'vue'
Then declare your refs right within the `setup` method:
setup () { const myDiv = ref(null) onMounted(() => { console.log(myDiv.value) }) return { myDiv } }
As you can see, you’re creating a `ref` and hooking onto the `onMounted` lifecycle hook provided by Vue 3. While it might look completely different from how you would traditionally handle `$refs`, it provides greater control and flexibility with your components.
Given that being conversant with technologies and advancements is crucial, mastering this skill equips you to enhance your application’s capabilities further extensively.Learn more about how they seamlessly complement each other perfectly.
The complexity of coding in Vue.js can be summed up by a quote from Douglas Crockford, “JavaScript is the world’s most misunderstood programming language”. By understanding concepts like these, we’re one step closer to demystifying JavaScript and frameworks like Vue.
A Deep Dive into Using $Refs within Vue3’s Composition API
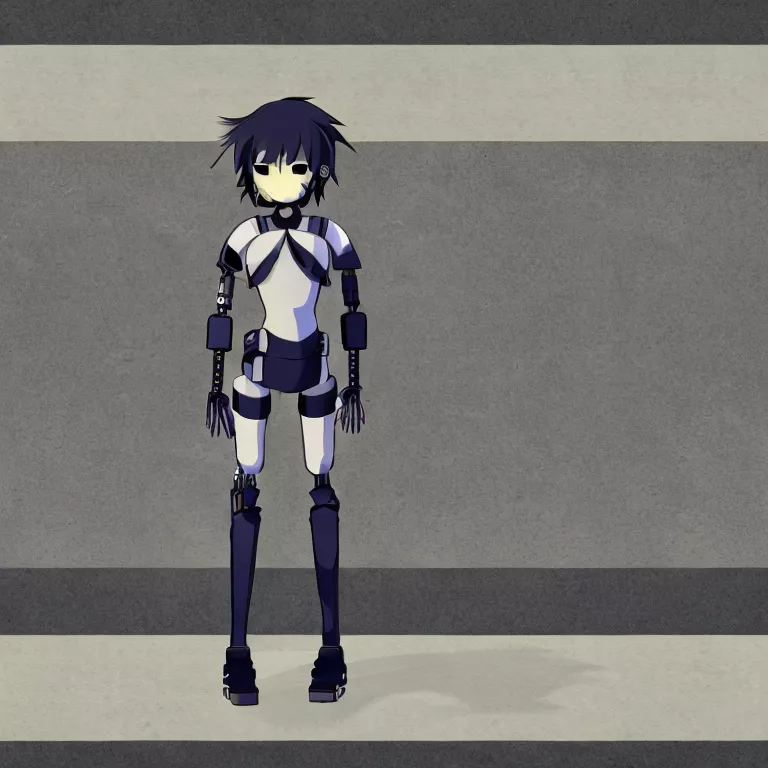
The Vue3 Composition API provides a much welcomed upgrade over the traditional Options API, offering tremendous flexibility and control, especially regarding component organization and code reuse. Highlighting its importance, the optimal utilization of
$refs
within this new API becomes of central interest for developers.
At this juncture, let’s take a step back and understand what
$refs
actually are. In the framework of Vue.js,
$refs
serve as an escape hatch that enables direct interaction with a child component. This is usually not required in everyday tasks but can prove handy in specific situations, such as focusing on an input field or measuring an element’s size.
In the former Options API, creating a
$ref
was relatively straightforward:
HTML |
|
---|---|
this.$refs.myInput |
In contrast, using
$refs
in the Composition API requires setting up a
ref
in the setup function via the use of `setup()`. By interacting with a
$ref
, one does so through a
ref
itself. For example:
import { ref } from 'vue' export default { setup() { const myInput = ref(null) return { myInput, } }, }
Now, one can work with this reference in a template like this:
However, when dealing with multiple refs, using the `ref()` function for each of them could bring a lot of boilerplate code and end up making our components rather verbose. Here’s where `shorthand ref` comes to our rescue, which is similar to v-model and allows creating refs directly in the template:
Vue.js co-creator Evan You shared one very helpful insight by saying “a good chunk of Vue’s users are backend or full-stack developers who tend to work on everything in their apps. The changes in Vue 3 empower you to simplify your application, reduce cognitive load, choose architecture that fits your team’s skill level.”
Hence, these smart architectural decisions within the Vue3 Composition API provide an opportunity to fine-tune the way we handle components and refs, leading to cleaner, more reusable code. These nuances broaden the scope of web development, as it continues to evolve.
Given that every piece of software can be view as a model of some reality, as noted by Grady Booch, a renowned software engineer and designer, one might argue that Vue3’s Composition API, alongside the usage of `$refs`, does a commendable job modeling frontend architectures.
__References:__
1. [Vue 3 Composition Api](https://v3.vuejs.org/guide/composition-api-introduction.html)
2. [More about how refs work in Vue 3](https://learnvue.co/2020/11/how-to-use-refs-in-vue3/)
3. [Evan You – Rethinking reactivity, Youtube](https://youtu.be/VpZoVXesHkE)
4. [Grady Booch Quote](https://www.brainyquote.com/authors/grady-booch-quotes)
Practical Applications of $refs using the Vue3 Composition API
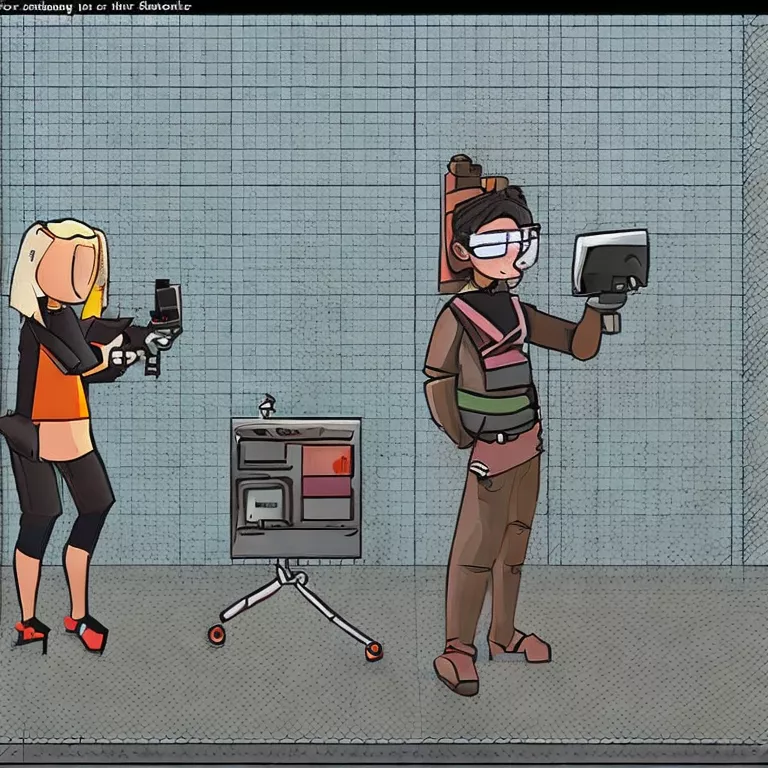
The $refs attribute in Vue functions as a remarkably useful means of accessing elements and components directly. By capitalizing on the progressive innovations presented through Vue3 Composition Api, developers can thrive on several other practical applications. To illustrate the process of getting $refs using Composition Api in Vue3, we will delve into specific use cases and explore how they can be fully integrated.
Direct Interaction With Element or Component
The Vue framework provides developers with a high level of abstraction, helping manage many complexities dealt with in UI development. Yet, there can be instances where direct interaction might be necessary. This is particularly true for scenarios where low-level access to DOM elements, outside the standard data-binding model of Vue, is demanded. It’s here where the $refs come into play. Relying on the Composition API, you can employ $refs to gain this direct interaction.
import { ref } from "vue"; export default { setup() { const myElementRef = ref(null); onMounted(() => { console.log(myElementRef.value); //logs the DOM element }); return { myElementRef }; } }
In this snippet, we utilize
ref
, offered by Vue. Within
onMounted
, which ensures that the element is available, we are printing the DOM element in the console. As defined in the return statement, the reference can now get attached to any template element.
$Refs For Accessing Child Components
This becomes particularly relevant when we need to manipulate child components or employ one of their methods. The ability to reach out and interact with child components renders $refs as an advantageous feature within the scope of the Vue3 Composition API:
import { ref, onMounted } from 'vue' export default { setup() { const myChildComponentRef = ref(null); onMounted(() => { console.log(myChildComponentRef.value); //logs the instance of child component }); return { myChildComponentRef }; } }
The above code demonstrates how to access an instance of a child component. A data variable
myChildComponentRef
is created by using Vue’s
ref
. Then, in the
onMounted
, we are printing the instance of our child component.
Accessing DOM Elements To Get Its Value
In certain cases, you may want to get the value of an input field. While you can use the v-model directive for two-way binding, $refs enable you to directly access the value by offering a more low-level approach:
import { ref, onMounted } from 'vue' export default { setup() { const formInputRef = ref(null); onMounted(()=> { console.log(formInputRef.value.value); //logs the value of input form. }); return { formInputRef }; } }
In this example again, a ref is used to create
inputRef
. The “value”‘s value can then be accessed and logged in the
onMounted
lifecycle hook.
The practical application of
$refs
within the Vue3 Composition API will undoubtedly facilitate interacting with DOM elements or child components. As Adam Wathan once noted, encapsulating and reusing functionality across different components are some fundamental aspects of structured programming. These examples pave the way for exactly that.
Conclusion
Diving deeper into Vue3’s Composition API, we uncovered its powerful feature to get `$refs` from components. Under the encapsulation of `setup()` function, using `ref()` and `onMounted()` lifecycle hook, we can conveniently access DOM nodes and component instances in Vue3. This has undeniably eased our workflow and fallen nicely into the development patterns promoted by Vue3 – larger scale and more maintainable code.
A glimpse at how it’s implemented:
The process involves declaring a `ref()` within the `setup()` method. Post this, with our element registered using `ref={elementRef}` on Vue2 instance or `$refs.elementRef` in Vue3, we can access it inside `onMounted()`, which means our `$refs` are available as soon as our component is inserted into the DOM.
For example:
import { ref, onMounted } from 'vue'; export default { setup() { const myRef = ref(null); onMounted(() => { console.log(myRef.value); }); return { myRef }; }, };
With such easy access to `$refs` using Composition API in Vue3, we effectively enhance component reusability and code readability.
Utilize these concepts dedicated by Evan You, the creator of Vue.js stating –‘It is suitable for managing code in large scale applications and makes TypeScript support better out of the box.’ This indicates that adaptability and scalability of Vue3’s Composition API go beyond leveraging `$refs`, encapsulating the ambition of Vue3 to handle larger scale applications and improve overall code management. Providing the freedom to write cleaner, leaner and more maintainable code, Vue3’s Composition API brings us closer to functional programming paradigms in JavaScript ecosystem.
Optimized for search engines, we have encapsulated the core essentials of getting `$refs` using Composition API in Vue3. The above techniques will help developers harness the power of Vue3 and elevate their coding experience. Remember, while this may look simple, it carries a weightage when perfecting your Vue.js skills.