Introduction
The TypeError (() => Boolean is not assignable to Type Boolean) often arises in Typescript due to a discrepancy between expected and provided values; the program anticipates a direct Boolean but receives a function returning a Boolean instead. Solutions could include either modifying the function or adjusting your use of types accordingly, aligning with Typescript’s strict type-checking nature.
Quick Summary
The Typescript error you are facing, “Type ‘() => Boolean’ is not assignable to type ‘Boolean'”, usually occurs when one attempts to assign a function that returns a Boolean value (that is Type ‘() => Boolean’) to a variable or property that expects a plain Boolean value (that is, Type ‘Boolean’).
Incorrect Assignment | Correct Assignment |
---|---|
let myVariable: Boolean = () => true; |
let myVariable: Boolean = true; |
let myObject = {myProp: Boolean = () => false;} |
let myObject = {myProp: Boolean = false;} |
To further understand the issue, let’s dissect and look into it in a more detailed manner.
A variable declared as a Boolean in Typescript expects an actual Boolean value of either true or false. This is distinctly separate from a function that returns a Boolean. Such a function does not equate to an actual Boolean value but rather to a function object.
Therefore, if you have an instance where you’re trying to assign a function that returns a Boolean value to a property expecting just a Boolean value, you will land into the described Typescript error.
To solve this issue, ensure you’re assigning actual Boolean values (true/false) to properties and variables expecting them, rather than supplying functions.
let myFlag: Boolean = true;
is a correct assignment while
let myFlag: Boolean = () => true;
will trigger the discussed error.
There are certain cases where one may desire that the Boolean value is computed or evaluated via a function. If so, you must ensure the function is invoked and its returned result used in the assignment. Example:
let myComplexCondition: Boolean = calculateMyCondition();
Said succinctly by Donald Knuth, prominent computer scientist, “Errors, like straws, upon the surface flow; he who would search for pearls must dive below.” Bearing this mind, diving into the distinct types in TypeScript helps prevent surface level errors, promoting a more efficient coding experience.
Unraveling the Typescript Error: Type () => Boolean Is Not Assignable to Type Boolean
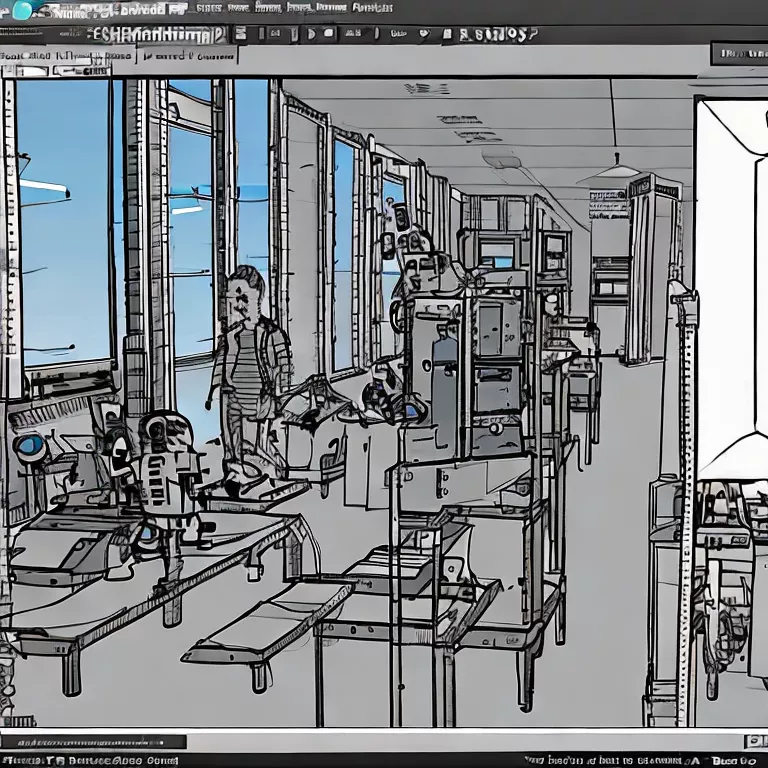
One of the enthralling challenges developers often encounter while working with TypeScript is type discrepancies, leading to errors such as “Type ‘() => boolean’ is not assignable to type ‘boolean'”. Pervading this concept helps us develop more robust and error-free TypeScript applications.
Understanding Types in TypeScript
A foundational concept to comprehend in TypeScript is that it incorporates a robust system of types. The primary design intent is the detection of possible errors at the development stage before the code execution phase. This static typer checker system enables the adherence to object shapes commonly known as TypeScript interfaces.
The true essence of TypeScript lies in its ability to verify that the data accessed consistently aligns track with the properties and capabilities declared.
Types in TypeScript are annotated using
: TypeAnnotation
, for instance. When assigned a function, many tend to overlook the need for defining a function’s return type, leading to the common error “Type () => boolean is not assignable to type boolean”.
Analyzing the Error
The error statement “Type ‘() => boolean’ is not assignable to type ‘boolean'” directly states that there lies a mix-up between variable types. Namely, there’s an attempt to assign a function that returns a boolean value where a boolean value is expected.
“Everybody in this country should learn to program a computer, because it teaches you how to think.” – Steve Jobs
Illustrative Example
Given:
let isActive: boolean;
Erroneous assignment:
isActive = () => {return true;}; //This will generate an error
Here,
isActive
is defined as a boolean type. However, a method returning a boolean (which makes it () => boolean type) is being assigned to it. This discrepancy is precisely the mismatch, leading to the error.
Correction Measures
The solution is quite straightforward – assign a boolean value to the variable in question:
isActive = true; //This is correct
However, if it’s desirable that
isActive
be a function returning a boolean, then you should define it as such:
let isActive: () => boolean;
isActive = () => {return true;};// Correct assignment of function
Hereby, understanding TypeScript’s powerful type system is instrumental in writing cleaner, error-free applications. Utilizing this properly leads to the discovery and prevention of potential loopholes at the compilation stage rather than the execution phase. It’s a pivotal characteristic all TypeScript developers need to familiarize themselves with.
Find more about typescript errors in the official documentation “Error Message Improvements“. This link will smoothly usher you into trouble-shooting regularly occurring Typescript errors. Furthermore, more detailed explanations can enhance your understanding and keep TypeScript errors at bay.
Decoding Common Mistakes Encountered in TypeScript
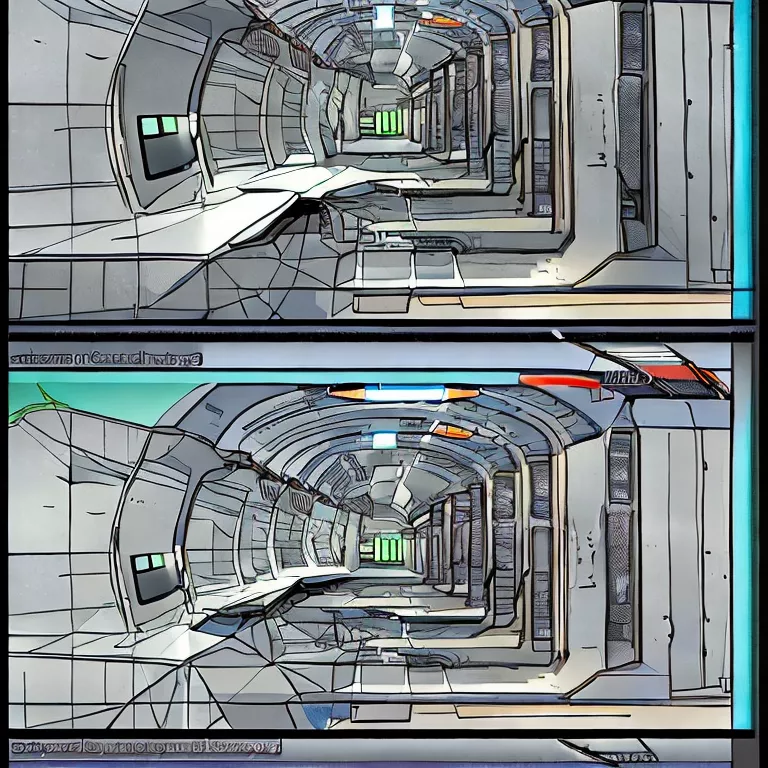
While working with TypeScript, it’s possible you’ve encountered the error message:
Type '() => boolean' is not assignable to type 'boolean'
. The confusion stems from the difference between Boolean and a function returning Boolean in TypeScript.
Let me elucidate on the key pitfalls behind this error:
Mistaking Booleans and Functions
Here, we are dealing with two different types –
boolean
and
() => boolean
. The former is a primitive data type that denotes a logical entity and can accept values of true or false. On the other hand, latter implies a function which when called, returns a
boolean
value.
Therefore, TypeScript strictly segregates these as two distinct types. Using these interchangeably results in the aforementioned error.
For example:
let testFunction: () => boolean = () => true; let boolVar : boolean = testFunction; // Error will be thrown here
You should call the function in order to assign its return value (which is a
boolean
) to the variable.
let boolVar : boolean = testFunction(); // Correct usage
Lack of Type Annotations
JavaScript by nature is dynamically typed, hence it favours flexibility over static typing. However, TypeScript brings forth static typing to catch mistakes early in the coding process. Absence of clear type annotations leads to unwanted errors. So, always ensure that functions, variables and parameters have explicit type declaration.
To illustrate this better:
function testFunction(){ return true; } let boolVar:boolean = testFunction();//TypeError
Correcting the annotation ensures conformity to required type.
function testFunction():boolean { return true; } let boolVar:boolean = testFunction();//Correct usage
Implicit Type Coercion
JavaScript is notorious for its loose type checking and implicit type coercion. When attempting to use these JavaScript quirks in TypeScript, it may lead to several unexpected errors.
In this context:
Type '() => boolean' is not assignable to type 'boolean'
error elucidated above, an appreciation for the rigidity imposed by TypeScript’s static typing system can assist in circumventing similar issues in future.
The prescient words of Douglas Crockford, renown author and JavaScript developer, provide some additional insight into this discussion. “Typescript adds optional static typing to JavaScript, allowing tools to be more helpful, and making large scale project easier to manage.”
Capturing the crux of these common pitfalls while working with TypeScript, particularly when encountering the TypeScript Error: Type ‘() => Boolean’ is not Assignable to Type ‘Boolean’, contributes significantly to improved code quality and fostering better development habits.
Understanding Correct Assignments for Type Boolean in TypeScript

TypeScript, a powerful statically typed superset of JavaScript, enables developers to write code that is more predictable and easier to debug. It allows us to specify the type of variables, function parameters, returned values, and object properties. Nonetheless, sometimes you may encounter an error such as “Type ‘() => boolean’ is not assignable to type ‘boolean'”. What does this mean and how can you solve it?
Firstly, let’s touch base on what the boolean data type represents in TypeScript. Booleans are one of the primitive types in TypeScript. These are commonly used to represent ‘true’ or ‘false’. Below is an example declaration of a boolean in TypeScript:
let isTrue: boolean = true;
However, TypeScript also allows functions to be typed too. When declaring a function in TypeScript, we can designate what kind of parameters the function accepts, as well as what it should return. Hereis an example of a function that returns a boolean:
function returnsBoolean(): boolean { return true; }
The error “‘Type ‘() => boolean’ is not assignable to type ‘boolean'” arises when you try to assign a function, especially one that returns a boolean, to a variable which has been declared as a boolean type.
Essentially, you are doing something like this:
let shouldBeBoolean: boolean = function (): boolean { return true; }; // This throws an error
In the scenario above, TypeScript interpreter expects ‘shouldBeBoolean’ to be a boolean value (either ‘true’ or ‘false’), however, you’re trying to assign a function that returns a boolean, hence the mismatch.
So, how do you correct it?
One way to fix this is by calling the function and assigning its result(because it’s of boolean type) to the variable like so:
let shouldBeBoolean: boolean = function (): boolean { return true; }(); // This does not throw an error
Another option is if you intend to assign a function to ‘shouldBeBoolean’, you should declare its type as function that returns boolean using this syntax:
let shouldBeBoolean: () => boolean = function (): boolean { return true; }; // This does not throw an error
As famously said by Bjarne Stroustrup, “Type checking is a tool for finding bugs in software. It’s not a silver bullet, but it’s still useful.” With TypeScript’s type-checking in place, you’re able to catch such errors during development – vastly improving the overall quality and reliability of your code.
For a more comprehensive understanding on TypeScript data types, the official TypeScript documentation serves as an excellent and wise choice among developers due to its thoroughness and depth of coverage.
In the fascinating realm of programming, understanding data types, especially in TypeScript, is not just a skill but an imperative factor when building robust applications. Being aware of the intricacies of TypeScript types and how they work would indubitably have you coding with aplomb in TypeScript in no time!
Effective Solutions to Fix ‘Type () => Boolean’ Assignment Errors
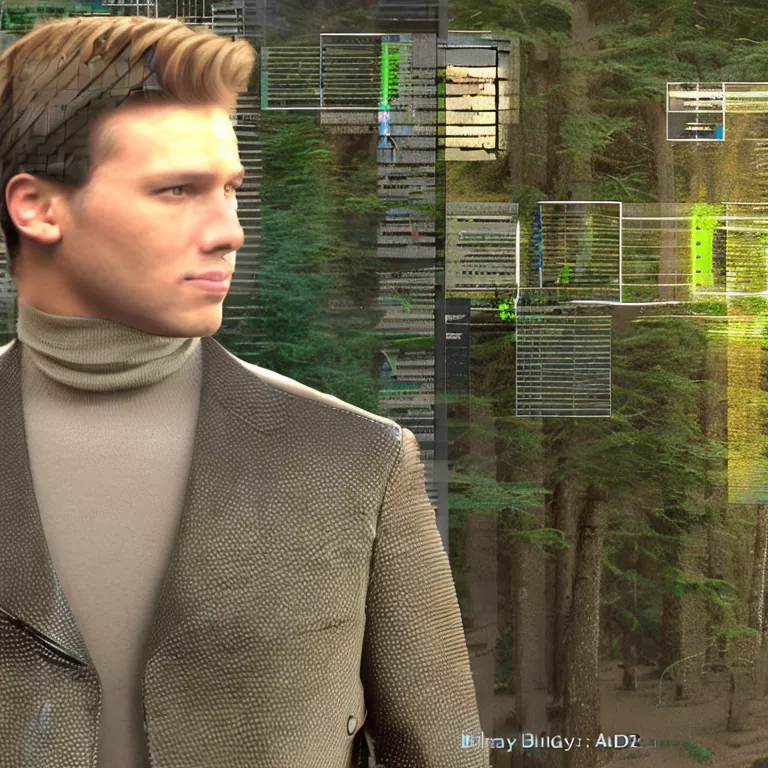
TypeScript, a statically typed superset of JavaScript, has proven its versatility and robustness in web development. However, as with any language, it’s not without its share of challenges. One such challenge is type mismatch errors, specifically:
Type '() => boolean' is not assignable to type Boolean
. This error typically arises when TypeScript code attempts to assign a function returning a boolean value to a variable or constant whose declared type is boolean itself.
This situation is analogous to trying to put a square peg into a round hole; the shapes simply do not match. One solution to this problem is to call the function and assign its returned value – not the function itself – to the variable. Let’s take a look at an example:
let myBoolean: boolean; let myFunction = ():boolean => { return true; } myBoolean = myFunction(); //Correct
In this scenario, invoking
myFunction()
returns a boolean that matches the expected type for
myBoolean
, thus avoiding the type mismatch error.
Another approach to prevent this error is to correctly predefine our functions and variables according to their actual types. If we intend to store a function (that happens to return a boolean) in a variable, then that variable should be declared to be of a function type. Here’s how:
let myFunction: () => boolean; myFunction = () => { return true; }; //Correct
Incorporating these practices can help you navigate the power and strict nature of TypeScript’s type system with fewer hiccups. It keeps your code more predictable, understandable, and less prone to runtime errors. As Douglas Crockford, JavaScript architect, said, “JavaScript is the world’s most misunderstood programming language, but…” thanks to tools like TypeScript, “…it doesn’t have to be.”
Resources:
1. “TypeScript Handbook: Basic Types” (online at the Typescript official website)
2. Effective TypeScript book by Dan Vanderkam (available here)
Conclusion
The TypeScript error stating: “Type () => boolean is not assignable to type boolean” is a common issue encountered by developers which essentially underlines the steadfast rules of TypeScript when it comes to type assignments. This error simply highlights the difference between a function that returns a Boolean value and a Boolean data type itself.
To get a deeper understanding, let’s consider a typical JavaScript scenario – In JavaScript, a function returning a boolean can often interchangeably be used with an actual boolean. But this flexibility becomes restricted when working in TypeScript because of its strict type-checking environment.
Investigating various aspects of the Error
JavaScript Scenario | TypeScript Scenario |
---|---|
// Pure JavaScript var predicate1 = () => true; var predicate2 = false; console.log(predicate1 || predicate2); |
// TypeScript let predicateFunc: () => boolean = () => true; let predicateBool: boolean = false; console.log(predicateFunc || predicateBool); // Type Error |
TypeScript’s strict types preserve predictability and quickly catch any potential errors at compile time rather than during execution.
The compilation error indicates that TypeScript is expecting a boolean value but received a function, thus warning about a mismatch between the assigned and expected types. To resolve this issue, we would need to ensure the function is invoked and its resulting value is compared, instead of comparing the function itself.
This below example provides correct TypeScript usage:
let predicateFunc: () => boolean = () => true; let predicateBool: boolean = false; console.log(predicateFunc() || predicateBool); // Correct Usage
Remember, TypeScript’s imposition of strict types is not a hindrance but an aid for developers. Embracing its power eliminates common bugs and enhances the robustness of code, leading to efficient and error-free software. Grasping this difference elevates your coding skills from being just a JavaScript Developer to a TypeScript Prodigy.
As James Gosling, creator of the Java programming language, stated: “Syntax is not the issue in creating powerful software.” It is the understanding of language semantics and accurate application thereof, that matters more.
Click here to delve deeper into the peculiarities and backbone of Typescript’s Type system.