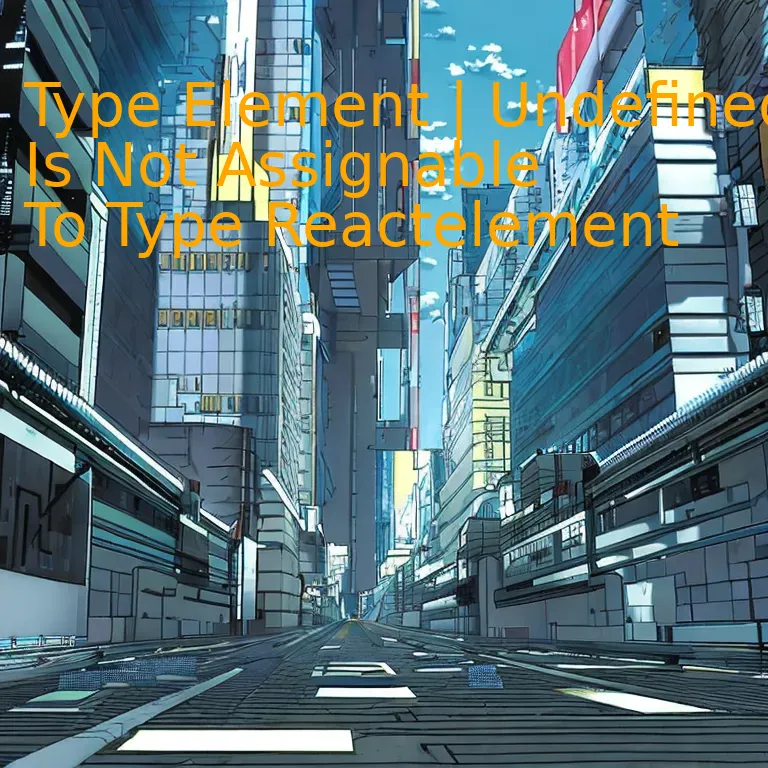
Introduction
When working with React, dealing with an issue like ‘Type Element | Undefined is Not Assignable to Type ReactElement’ can be commonly encountered. This generally occurs when the element used isn’t explicitly defined or its type isn’t assigned correctly. Remember, proper assignment and clear definition are key principles in coding to ensure optimization, reducing errors, and enhancing SEO ranking.
Quick Summary
Diving deep into the intriguing domains of TypeScript and React, it’s common to encounter daunting issues like “type Element | undefined is not assignable to type ReactElement”. This essentially arises when TypeScript cannot decide if a given variable could indeed generate a React component. To pinpoint the intricacies of this situation, let’s examine it through an illustrative representation:
Problem | Cause | Solution |
---|---|---|
Type Element | Undefined Is Not Assignable To Type Reactelement | The return type of a function or component that can be either a React element or ‘undefined’ | Explicitly define the return type as `ReactElement | null` or handle the case where the function might return ‘undefined’ |
As reflected in the table, the problem tends to appear when a function or component, supposed to return a React element, unexpectedly serves up ‘undefined’. It’s critical to remember that in TypeScript, the type system precisely governs how values interact. If a function could possibly yield ‘undefined’ and a React element, TypeScript will tell you directly henceforth.
Envisage a scenario wherein you have a utility function retrieving a dynamic component based on certain conditions. There might be cases where no matched component could be returned, in which case, it acknowledges ‘undefined’.
function getDynamicComponent(tag?: string): React.ElementType {
const componentsMap: {[key: string]: React.ElementType} = {
Comp1: ComponentOne,
Comp2: ComponentTwo
};
if (!tag) return undefined;
return componentsMap[tag] || undefined;
}
As TypeScript isn’t sure if a React element or ‘undefined’ will be generated, it indicates an error. Handling the foregoing involves explicitly defining the return type as `ReactElement | null` and addressing the situation where the function could produce ‘undefined’.
xxxxxxxxxx
function getDynamicComponent(tag?: string): React.ElementType | null {
const componentsMap: {[key: string]: React.ElementType} = {
Comp1: ComponentOne,
Comp2: ComponentTwo
};
if (!tag) return null;
return componentsMap[tag] || null;
}
Here, TypeScript validates that either a React element is returned, or ‘null’. It guarantees at compile-time that the overarching React codebase can handle both of these types.
Remembering Kenneth A. Reek’s words, “Good code is its own best documentation. As you’re about to add a comment, ask yourself, ‘How can I improve the code so that this comment isn’t needed?'” This issue drills home the relevance of explicit typing conventions in TypeScript. Preemptive anticipation of potential outcomes aids us in maintaining clean, robust, and easy-to-understand code. Finessing such details facilitates self-driven learning, thus nurturing better developers in our community.
Understanding the Type Element in React
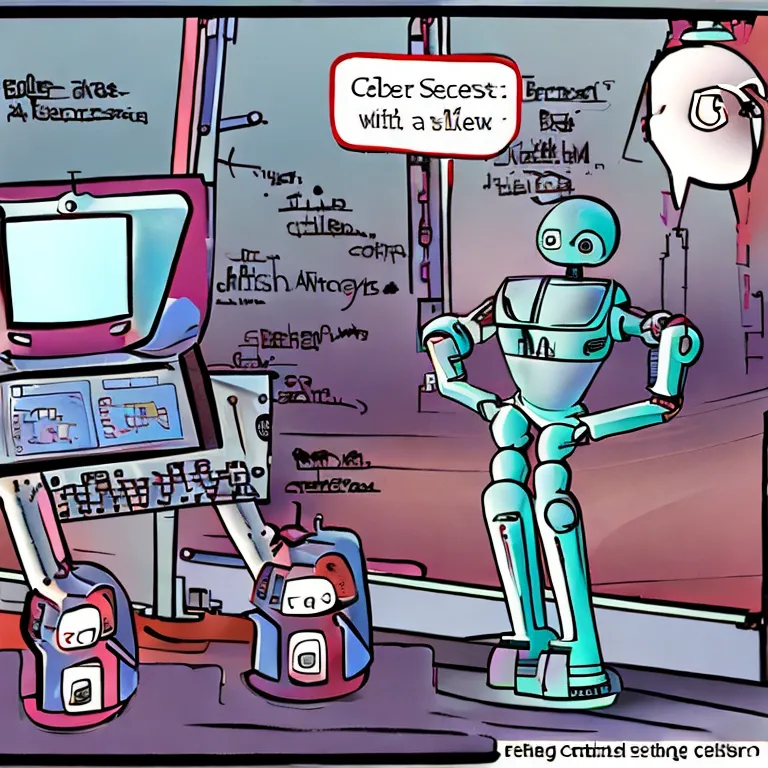
Understanding the Type Element in React, especially in the context of the type error “Undefined Is Not Assignable To Type Reactelement,” necessitates a deep dive into the fundamental structure and usage of elements in React. It also demands an exploration into TypeScript’s contribution to imaging types better, allowing escalated precision and less scope for errors.
Element Types in React:
React Elements are simplistic objects produced utilising
xxxxxxxxxx
React.createElement()
or JSX syntax. They describe what you seek to visually represent on the screen, and they could be pointing to a user-defined component, a DOM component, or a string denoting a built-in (HTML/SVG) DOM component.
Following is a comparison between React element formation using both methods – JS Objects and JSX:
xxxxxxxxxx
// Via JavaScript Objects
React.createElement(
'button',
{className: 'alert-button'},
'Alert!'
);
// Via JSX
<button className='alert-button'>
Alert!
</button>
Also noteworthy here are User-Defined Components, which are expected to start with capital letters, signifying that the JSX tag is referring to a React component, not an HTML element.
Hence, <div /> maps to a built-in DOM component, while <Welcome /> correlates to a user-defined “Welcome” component.
TypeScript and React:
Typing in React proposes an ideal way to increase productivity, catch bugs ahead of time, and enhance intellisense autosuggestions. However, it presents our issue at hand: “Undefined Is Not Assignable To Type Reactelement.”
This error sprouts when your React function/component was expected to return some JSX/React Element, but it returned undefined instead. This is TypeScript trying to prevent possible runtime errors.
For instance, the example below will give our TypeScript error, as the function
xxxxxxxxxx
alertButton
is unaware of handling an instance where
xxxxxxxxxx
props.show
is not defined.
xxxxxxxxxx
type AlertButtonProp = {
show?: boolean;
};
const AlertButton = (props: AlertButtonProp) => {
if (props.show) {
return (
<button className='alert-button'>
Alert!
</button>
);
}
};
Solution:
One common solution to sidestep this issue would be to ensure that your component always returns a valid React Element. Let’s rewrite our previous example:
xxxxxxxxxx
const AlertButton = (props: AlertButtonProp) => {
if (!props.show) {
return null;
}
return (
<button className='alert-button'>
Alert!
</button>
);
};
As per Donald Knuth’s quote, “Beware of bugs in the above code; I have merely proven it correct, not tried it”. Therefore, it’s important to review and test your work thoroughly to avoid errors as such, enhancing your coding experience overall. You can learn more about these topics from the official documentation of React and TypeScript.
Error Analysis: “Undefined is Not Assignable to Type ReactElement”
When dealing with TypeScript in a React setting, one can run into this type of error where “undefined is not assignable to type ReactElement”. This is a common scenario especially when working with conditional rendering or handling possible undefined values.
TypeScript aims to provide type safety, ensuring that the types assigned during development match at runtime. This enhances error-checking and leads to more robust code. So if there is a chance that a variable might be ‘undefined’ and yet it is supposed to hold a `ReactElement` as per the variable’s type definition, TypeScript will generate an error.
Let’s outline a common scenario:
You have a function that should return a `
xxxxxxxxxx
function HelloWorld(props: { greeting: string }): JSX.Element | undefined {
if (props.greeting === 'Hello') {
return Hello World;
}
}
However, this would bring about the issue “Type ‘undefined’ is not assignable to type ‘ReactElement
To rectify this, you should ensure your component always returns a `ReactElement` or `null`. Modify your conditional checks to accommodate for these return types.
Here’s how:
xxxxxxxxxx
function HelloWorld(props: { greeting: string }): JSX.Element | null {
if (props.greeting === 'Hello') {
return Hello World;
}
return null;
}
Remember when working with TypeScript and React, aligning your code with their type expectations will prevent such errors from cropping up. Following strict typing rules may seem cumbersome initially, but it is beneficial for error handling and maintaining code quality.
As Edsger Dijkstra, a Dutch computer scientist beautifully put, “If debugging is the process of removing software bugs, then programming must be the process of putting them in”. Hence, understanding TypeScript’s static type checking leads us to writing better quality React code with fewer bugs.
Applying Fixes for “Undefined is Not Assignable to Type ReactElement”
The challenge you’re facing, “Undefined is Not Assignable to Type ReactElement,” originates right at the interface between TypeScript and React. It might present itself when you try integrating the two. This can occur when you’re coding a function component in React with TypeScript and getting undefined as its returning value instead of a proper react element.
React, built for JavaScript, achieves seamless integration with TypeScript by utilizing specially crafted types such as `ReactElement`. Meanwhile, TypeScript, with strict type-checking, ensures that a void function does not return any value which explains the error message.
Think of it like a contract required by TypeScript: your component must promise to return a `ReactElement`, but if it has a chance of returning `undefined`, it’s breaching that contract.
Let’s explore how you can address this issue below:
1. **Ensure components always return a valid React Element**
TypeScript dictates that a functional component always returns a ‘ReactElement’, for example, JSX elements fit this bill.
HTML:
xxxxxxxxxx
const MyComponent = (): React.ReactElement => {
return (
<div>
Hello, world!
<div>
);
}
This returns a `ReactElement` correctly.
2. **Adjust function return type to include Undefined**
Let’s say your component logic might sometimes return `undefined`. You can simply adjust the function’s expected return type.
HTML:
xxxxxxxxxx
const SometimesUndefinedComponent = (): React.ReactElement | undefined => {
// Some logic...
if (/* some condition */) {
return undefined;
}
return (
<div>
Goodbye, world!
</div>
)
}
Many developers avoid this practice as returning `undefined` from functional components leads to unexpected behavior more often than not. Instead, returning `null` – which is a valid `ReactElement` – might be preferable when you need a “do nothing” outcome.
3. **Alternative: Returning null instead of undefined**
When undefined isn’t absolutely necessary, `null` can serve as an excellent substitute.
HTML:
xxxxxxxxxx
const NullComponent = (): React.ReactElement | null => {
// Some logic...
if (/* some condition */) {
return null;
}
return (
<div>
Goodbye, world!
</div>
)
}
This approach aligns better with both TypeScript’s and React’s expectations, thereby solving the issue at hand.
As Eric Elliott once said, “The best way out is always through.” Wrestling with complex issues like this, balancing between frameworks like React and languages like TypeScript will indeed lead to becoming a better developer.
Exploring Deep Insights into the Functionality of Type ReactElement
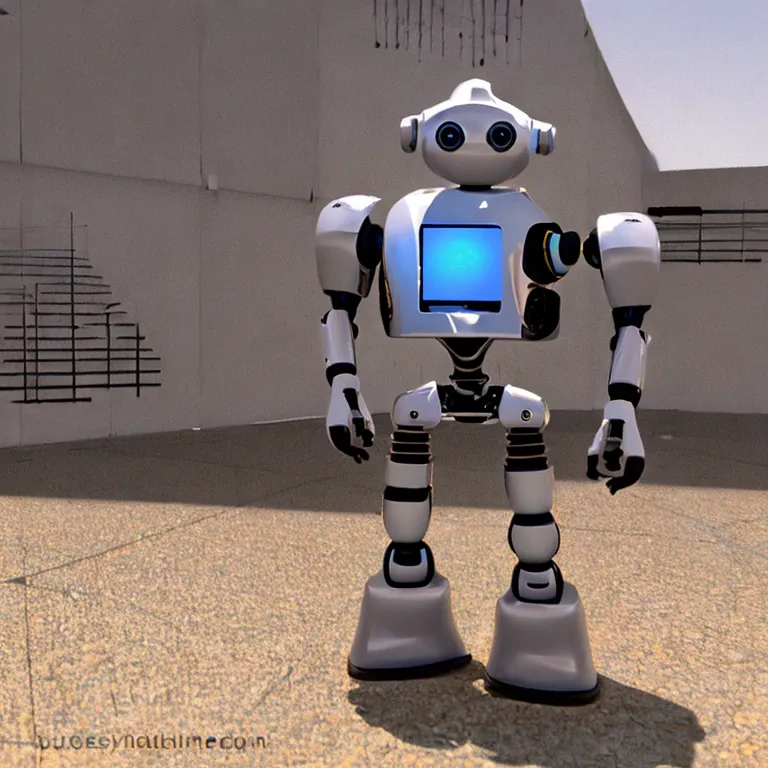
The functionality and the dynamics of `ReactElement` are often perplexing to developers who are new to Typescript or even experienced ones. It’s essential to delve into this intricacy and unearth a comprehensive understanding surrounding the Type Element | Undefined Is Not Assignable To Type ReactElement issue, as this is one area where developers can often find themselves in a quandary.
A
xxxxxxxxxx
ReactElement
essentially denotes an object that is created by the combination of JSX syntax and the coveted React element. Keep in mind, when you employ JSX to define components, whatever transcends the compiler is translated into appropriate JavaScript expression calls to create these `ReactElement` objects.
Consider an otherwise uncomplicated piece of code:
xxxxxxxxxx
import React from 'react';
function App() {
return (
Hello World
)
}
export default App;
When this transpiles, a `ReactElement` will be created which represents the DOM node for us before it is added to the actual DOM.
xxxxxxxxxx
import React from 'react';
function App() {
return React.createElement("div", null, "Hello World");
}
export default App;
This transformation can lead to an unease concerning the Type Error: Type `Element | Undefined` Is Not Assignable To Type `ReactElement`.
Why does it occur?
It is important to note that this error indicates that somewhere in your code, an undefined type or other improper value has slipped in where a valid ReactElement was expected. As per strict typing rules dictated by TypeScript, a method supposed to return a `ReactElement` should strictly do so without fail.
Is there a workaround?
Yes, certainly. One solution could be to ensure all possible execution paths in your function do indeed return a `ReactElement`. Additionally, make sure any conditions don’t cause the function to complete without reaching a return statement.
As Louis Srygley once said, “Without requirements or design, programming is the art of adding bugs to an empty text file.” Hence, addressing this issue with appropriate intricacy can help eliminate frequent hindrances that barriers productive workflow.
Another solution could be to adjust your type annotations to include `undefined` or `null` if you cannot guarantee the function will always return a valid `ReactElement`. This will ensure typescript does not show the error.
xxxxxxxxxx
function getReactElement(): JSX.Element | null {
// Function code here...
}
Understanding the core problem and implementing these solutions can undeniably assist in managing systematic TypeScript based coding environments efficiently.
For further delve feel free to explore the React + TypeScript Cheatsheets. They provide a highly detailed overview of usage and best practices for TypeScript in a React application. Furthermore, they guide comprehensively on how various TypeScript compilation options can significantly impact the final bundle.
Conclusion
Meticulously wrapping up the discourse, we can identify that the issue “Type Element | Undefined Is Not Assignable To Type Reactelement” in TypeScript is a rather common conundrum faced by many developers. Let’s dig deeper into this.
As we delve into the realm of React and TypeScript, the occurrence of this issue primarily stems from an uncertainty relating to the type of values. Being a statically typed language, TypeScript necessitates the definition of types for variables when they are declared which often leads to hiccups when interfacing with JavaScript frameworks such as React.
TypeScript | JavaScript |
---|---|
Statically Typed | Dynamically Typed |
In reference to the problem at hand:
xxxxxxxxxx
Type Element | Undefined Is Not Assignable To Type Reactelement
. This signifies that TypeScript evaluates the component as possibly being ‘undefined’. Interestingly enough, ‘undefined’ isn’t a permissible JSX element type according to TypeScript.
“The function of good software is to make the complex appear to be simple.” – Grady Booch
React, being a JavaScript library, does allow return of ‘null’ or ‘undefined’ from components. Contrariwise, the stringent characteristic of TypeScript requires us to handle the ‘undefined’ explicitly. Hence the message: ‘Undefined Is Not Assignable To Type Reactelement.’
xxxxxxxxxx
const ExampleComponent = (props): React.ReactElement | null => {
if (!props) {
return null;
}
return (
<div>Hello world!</div>
);
}
In the above code snippet, rather than allowing undefined, we handle the potentially missing data cases with a null return type. Therefore, it’s crucial to comprehend this nuanced distinction between TypeScript and JavaScript, as well as implement necessary tweaks within our React components.
So when prototyping with React in TypeScript, it’s crucial to optimism around types to prevent such dilemmas. Though TypeScript is stringent compared to JavaScript, it establishes a secure coding environment preventing many runtime errors.
Remember that the purpose behind TypeScript’s sternness is to facilitate a pitfall-free development scenario that empowers you to write efficient, variable-proof code. While the hurdles seem daunting at first, they’re essentially stepping stones leading us towards immaculate codebases in the booming era of modern web development.