Introduction
In the realm of programming, encountering an issue like ‘Type Null Is Not Assignable To Type T’ is often due to the non-assignment axiom within stricter type checking settings, which requires proper evaluation and understanding for efficient coding practices.
Quick Summary
Element | Description |
---|---|
x Type T |
An arbitrary type identifier in TypeScript, which can represent any valid type, such as string, number, array, object, etc. |
xxxxxxxxxx null |
A special keyword in TypeScript representing the absence of value or an object reference. |
xxxxxxxxxx Type Null is Not Assignable To Type T |
An error that often occurs when a variable of type ‘null’ is attempted to be assigned to another defined type ‘T’. |
This relatively common error frequently surfaces during TypeScript programming. The “Type Null is Not Assignable to Type T” error typically punctuates instances of mismatched data types. TypeScript language uses static types to ensure that no undesirable output or behavior affecting code quality ensues due to incorrect datatype assignments.
Interestingly, this highly accurate checker allows typescript to maintain program structure integrity by avoiding potentially crash-inducing operations like attempting to call nonexistent methods or accessing nonexistent properties within objects, hence its strictness on type null assignments to Type T.
For instance:
xxxxxxxxxx
let myString: string;
myString = null; // This will throw an error - "Type null is not assignable to type string"
In the context of TypeScript, an application runtime environment that checks for type safety, ‘null’ is specified as its own distinct type. Therefore, unless explicitly declared in a union type, you cannot assign null to a variable of another type. If it was necessary to assign null to ‘myString’, the string literal type should have been defined as so:
xxxxxxxxxx
let myString: string | null;
myString = null; // This now is acceptable to TypeScript
This instance demonstrates using a union type to indicate that ‘myString’ can be either a ‘string’ or ‘null’. Hence allowing TypeScript to maintain a strict type-checking mechanism, promoting accuracy and robustness of code.
As Francis Bacon once stated, “Knowledge is power.” Understanding these type assignment principles grants power to leverage TypeScript’s strengths fully, creating an application resilient to bugs introduced by type inconsistencies.
Understanding the Error: “Type Null is Not Assignable to Type T”
The error message “Type Null is Not Assignable to Type T” in TypeScript occurs when you try to assign a null value to a variable that has been declared to be of a certain type. This is a common issue you might face, especially when dealing with optional values or things like API responses where there’s a chance that a certain value might be returned as null. TypeScript does not permit this because of its strict checks for type safety.
For example, if we have a variable declared as follows:
xxxxxxxxxx
let str: string;
If later we attempt to set the variable `str` to null, a “Type Null is Not Assignable to Type String” error will be thrown by TypeScript:
xxxxxxxxxx
str = null;
To understand and solve this error, it’s crucial to delve into some key TypeScript concepts.
## Nullable Types in TypeScript
In TypeScript, every type is implicitly nullable or undefinable which means values can always be assigned with null or undefined. However, by running TypeScript with the –strictNullChecks flag (or “strictNullChecks”: true in tsconfig.json), you tell TypeScript to track nullable information. Enabling strict null checks introduces null and undefined as distinct types. Variable declarations like `let str: string = null;` will lead to an error.
## Solution 1: Union Types
You can use a union type to allow a variable to hold either a specified type or a null. A union type is a type formed from two or more other types, representing values that may be any one of those types. We make a type union by combining multiple types using the | symbol.
xxxxxxxxxx
let str: string | null;
Now, let’s assign null to str:
xxxxxxxxxx
str = null;
This time, your TypeScript code compiles without errors.
## Solution 2: Optional Parameters / Properties
You can use optional parameters or properties in object types. By adding a question mark ? after the parameter or property name, we specify that this is optional.
For instance:
xxxxxxxxxx
function greet(name?: string) {
console.log('Hello, ' + name);
}
In this function, name is an optional parameter.
To recall our quote from Matthias Hertel, “The type system stands between you and the wild jungle of JavaScript — catch errors before runtime!” Ultimately, greater understanding and leveraging TypeScript’s type-checking capabilities aid in cleaner, bug-free codebases.(source)
Exploring the Concepts of Types and Null in Programming
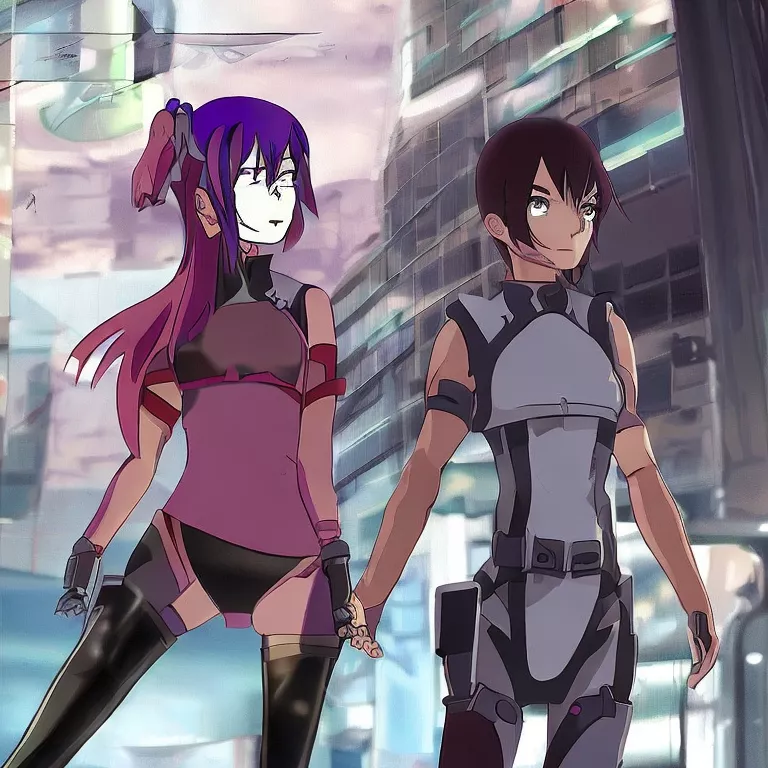
Exploring the reality of types and null in programming requires a deep dive into data handling. Dealing with the nuances of type assignment and variables’ potential to hold a ‘null’ value are key elements that define efficient code.
Understand Types and Null
——–
A type, in the context of programming, refers to a specific kind of data classification. For example,
xxxxxxxxxx
string
,
xxxxxxxxxx
number
, and
xxxxxxxxxx
boolean
are common types you’ll see in most typed languages like TypeScript.[1]
Meanwhile,
xxxxxxxxxx
null
is a special value in the programming world. It represents ‘no value’ or ‘no object’. Essentially, it denotes the intentional absence of any object value.[2]
Type ‘null’ is not Assignable to Type ‘T’
————–
In TypeScript, the phrase “Type ‘null is not assignable to type ‘T'” means the variable’s type does not permit the assignment of a null value. This could occur when the programmer has enabled strict null checking in TypeScript.[3]
For instance, consider the following TypeScript code:
typescript
let userName: string;
userName = null; // Error: Type ‘null’ is not assignable to type ‘string’.
In this snippet, TypeScript prevents assignment of ‘null’ to ‘userName’ because it has explicitly been declared as type
xxxxxxxxxx
string
.
The code needs a little tweaking to make it accept a null value. In cases where a variable might need to hold a null, we could employ Union Types in TypeScript:
typescript
let userName: string | null;
userName = null; // Now allowed
The “| null” makes TypeScript aware that
xxxxxxxxxx
userName
can either host a string value or null.
To quote Ritchie Hoang, a seasoned developer and tech enthusiast:
> “The elegance of programming lies not only in crafting efficient solutions but also in foreseeing and accommodating potential variables – like the unassuming ‘null’.”[4]
Avoiding the Null Assignability issue
———-
There are multiple ways to avoid the “Type ‘null’ is not assignable to type ‘T'” error. This could be curated to the coding style and the project requirements:
* **StrictNullChecks setting**: An obvious first step would be to disable the strictNullChecks TypeScript setting if it’s not vital for your project.[3]
* **Use Union Types**: As outlined above, allowing your variables to hold multiple types, one of which is null (e.g.,
xxxxxxxxxx
string | null
)
* **Optional chaining operator** (
xxxxxxxxxx
?
): Typical in more recent versions of Typescript, this operator allows navigation through potentially undefined variables without throwing an error.[5]
Every strategy has its pros and cons, all influenced by the overall goals of the project and the structure of the existing codebase.
Understanding and handling types and null effectively are critical to successful and bug-free software development practices. Always remember, each line of code could create the next colossal software solution.
Diving Deep: How to Minimize or Eliminate Type Mismatch Errors
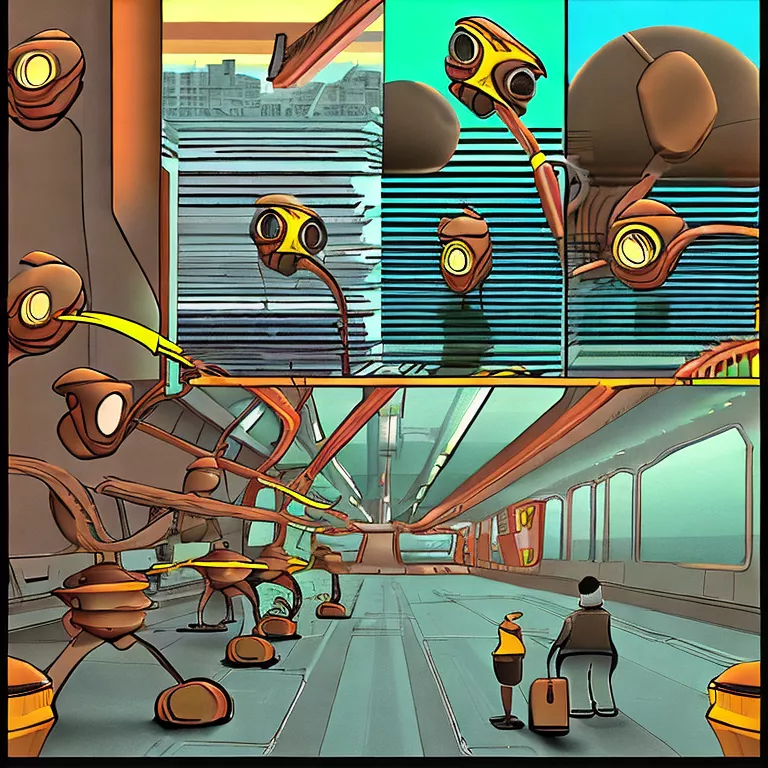
Type Mismatch errors, particularly involving the null type, are a common issue that TypeScript developers face. This error generally occurs when you try to assign a value of null or undefined to an object that does not expect such value. The beauty of TypeScript is that it provides compile-time type checking which prevents many JavaScript runtime errors. However, this advantage becomes a caveat when dealing with values assigned to objects that may be null or undefined unexpectedly.
In TypeScript, attempting to use a null or undefined object when TypeScript expects otherwise, results in the following error: `Type ‘null’ is not assignable to type ‘T’`. It’s crucial to remember that TypeScript includes two special types – Null and Undefined – which have their respective values `null` and `undefined`. By default, these types are subtype to all other types.
Ways to Minimize Type Mismatch Errors
The good news is there several techniques to prevent these kind of Type mismatch errors in TypeScript:
- Type Checking: Ensuring only aligned types are worked together can reduce such issues. TypeScript provides a robust type-checking system that helps developers in enforcing type safety during development. For example, if we define:
xxxxxxxxxx
let notNullObject: any;
notNullObject = null; // Will cause Error.
- Type Assertion: When you’re positive that an object will not be null, you could use a type assertion technique to tell the TypeScript compiler as much. This situation often occurs when interacting with third-party libraries.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” — Martin Fowler, author and speaker on software development
- Strict Null Checks: To specifically prevent null and undefined from being assignable to other types, there’s a unique compiler flag that TypeScript offers – the `–strictNullChecks` flag. While it makes type-checking stricter, it will guard against many run-time errors in JavaScript.
xxxxxxxxxx
// With --strictNullChecks
let x: number;
x = 1; // OK
x = undefined; // Error
x = null; // Error
- Optional Properties: On occasions, certain object properties may not always be available. Using an optional property will permit the value to be undefined, thereby preventing these type mismatch errors.
xxxxxxxxxx
interface OptionalProp {
someProp?: number;
}
let obj: OptionalProp = {};
Minimizing or eliminating Type Mismatch errors is undeniably a critical aspect of crafting sturdy code, especially when deploying TypeScript in production. Adhering to strong type checking as much as possible not only augments readability but also makes sure your codebase remains manageable, scalable and bug-free over time.
For more detailed knowledge about handling Null and Undefined in TypeScript, refer to this official TypeScript documentation on Null and Undefined.
Case Study Analysis: Common Scenarios of “Type Null Is Not Assignable To Type T” Error
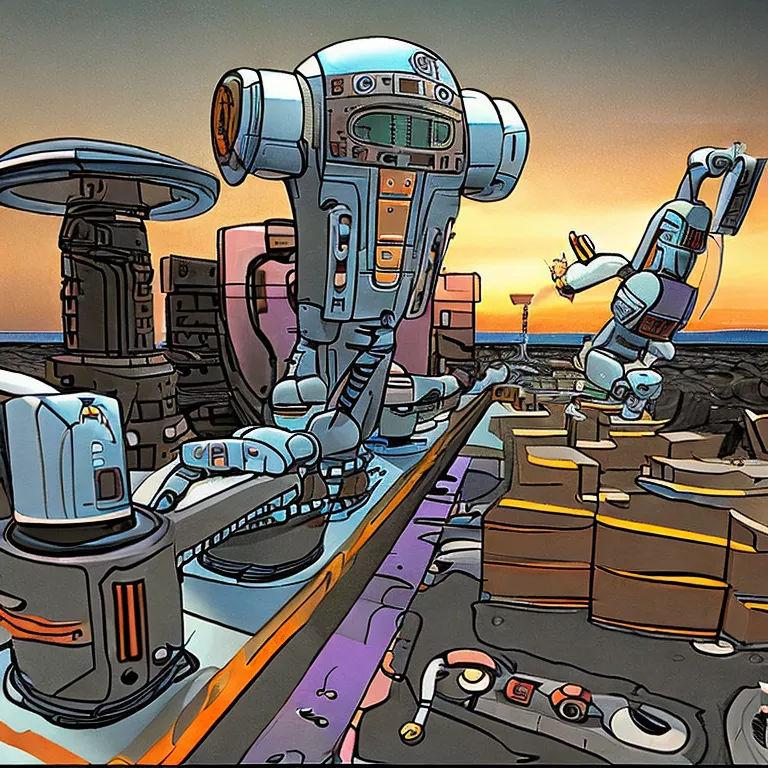
When delving into the specifics of TypeScript, one of the common scenarios a developer might encounter is the “Type ‘null’ is not assignable to type ‘T'” error. Analyzing some cases where this can be encountered will clarify the issue and how best to handle it.
Case 1: Passing Null to A Function Expecting Type T
Using null as an argument in a function that expects any other type will clearly trigger the error. Consider the function below with parameter ‘value’ of type T:
xxxxxxxxxx
function exampleFunction(value: T) {
//Some logic
}
Calling
xxxxxxxxxx
exampleFunction(null)
would generate the error.
One possible solution is to define the function to take null values explicitly:
xxxxxxxxxx
function exampleFunction(value: T | null) {
//Some logic
}
Case 2: Assigning Null to A Variable of Type T
This is another straightforward case:
xxxxxxxxxx
let variable: T;
variable = null;
Again, allowing null assignment by way of declaring the variable to be either Type T or null is a potential resolution:
xxxxxxxxxx
let variable: T | null;
variable = null;
Case 3: Array Values
Consider an array:
xxxxxxxxxx
let array: T[] = [null];
This code attempts assigning null as one of the elements of an array whose elements should only be of Type T—resulting in the predictable error. Similar to previous scenarios, a solution lies in defining the array to support both Type T and null:
xxxxxxxxxx
let array: (T | null)[] = [null];
The crux of the problem boils down to TypeScript’s strict null check feature. It enforces that values can’t be null unless specifically stated, guarding against null reference exceptions. This requires a shift in mindset from JavaScript where variables can start out as null or undefined but then be assigned a value of any type later.
As Edsger W. Dijkstra, a renowned computer scientist, once said, “The question of whether machines can think is about as relevant as the question of whether submarines can swim.” Equally so, when thinking about TypeScript and its relation to JavaScript, it’s more critical to understand how it behaves differently than worrying if it is just like its parent language.
TypeScript’s official documentation also delves deeply into this topic, offering great insights on handling null and undefined types.
Conclusion
Delving deeper into TypeScript’s type system, the nuance behind the message ‘Type null is not assignable to Type T’ emerges as an intriguing aspect of TypeScript programming. This error typically manifests when we try to assign a `null` value to a variable of a non-nullable type.
Let’s take an illustrative example in TypeScript to bring this into perspective:
xxxxxxxxxx
interface IExample {
field: string;
}
let myVar: IExample = null;
The above code will, as expected, throw an error – “Type null is not assignable to Type IExample”. TypeScript infers that the variable `myVar` should be an object that fits within the scheme of the `IExample` interface. However, by assigning `null` to it, we break this rule.
Now, why has TypeScript deemed it crucial to apprise developers of these violations? As Anders Hejlsberg, lead architect and designer of TypeScript, eloquently puts here, TypeScript’s protective measures against `undefined` and `null` types were introduced as part of the language’s mission to aid programmers steer clear from common pitfalls in JavaScript and improve robustness of code.
Confronting the ‘Type null is not assignable to Type T’ error may seem inconvenient at first, but is ultimately empowering. The TypeScript compiler nudges developers towards safer coding practices, compelling them to ensure that variables of a specific type always hold values conforming to that particular type. Certainly, initiating a variable with a `null` or `undefined` value if its type isn’t explicitly known can lead to unforeseen anomalies down the line.
Unhandled `null` or `undefined` assignments are notorious for causing headaches across programming languages, famously quoted here as the “billion dollar mistake”. Typified languages like TypeScript, rectify this flaw and aid programmers to catch such potential landmines early on during the development process.
Remember, TypeName cannot be assigned a `null` value unless it has been defined as nullable. This can be achieved using the union operator to indicate that the type could either be `null` or of a specific type like such:
xxxxxxxxxx
type NullableExampleType = IExample | null;
let myVar: NullableExampleType = null;
Here, TypeName is declared as either type `IExample` or `null`, making this assignment valid in TypeScript.
Preferably, one should avoid assigning `null` unless absolutely necessary. If a variable doesn’t have a valid value yet, consider if there’s a more suitable default value than `null`. Null checks in TypeScript help us become better developers by teaching us to efficiently handle ‘non-existent values’, ultimately leading to a codebase that is robust and easy to maintain.