Introduction
Understanding the issue of ‘TS2786 Component Cannot Be Used As A JSX Component’ relies on grasping how JavaScript and TypeScript interact, as well as JSX’s unique syntax rules; appropriate use of components leads to effective digital construction and smoother web development.
Quick Summary
The TS2786 error in TypeScript sparks when you attempt to utilize an entity as a JSX component, which isn’t constructible into a JSX element. Expanding on this topic, let’s explore more about the issue in the following table.
Issue | Cause | Solution |
---|---|---|
TS2786 Error | Occurs when trying to use an object that cannot be rendered as a JSX component. This could be an object, primitive value or function that doesn’t return a valid JSX element. | Ensure the component is either a valid class extending React.Component or a function returning valid JSX element. Regular functions or objects cannot be used as a JSX component. |
A significant cause of the TS2786 error stems from the incorrect type definition of a component. If your component is defined using a standard function or as an ES6 arrow function and does not return a valid JSX element, it will trigger a TS2786 error.
Let’s illustrate with a typical example where you may encounter such an issue:
//Incorrectly typed JSX component
const App = {};
<App />;
In this scenario, `App` is defined as an empty object, which will not work as a JSX component leading to a TS2786 error. Instead, `App` should be defined as a function that returns a JSX element:
xxxxxxxxxx
//Correctly typed JSX component
const App = () => {
return <div>Welcome to my Application</div>
};
<App />;
Adhering to the right protocols for defining JSX components is essential. Paraphrasing Steve Jobs’ words of wisdom: “Details matter, it’s worth waiting to get it right.”. Thus, eradicating TS2786 error involves ensuring your Javascript elements are rightly constructed as per TypeScript JSX element standards, abiding by the fact that visualizing code isn’t just about typing – it’s also about embracing and navigating the world of logics!
Understanding the Error: TS2786 Component Cannot Be Used As A JSX Component
When dissecting the error message “TS2786: ‘Component’ cannot be used as a JSX component. Its instance type ‘Component’ is not a valid JSX element” we are essentially dealing with Typescript’s complain, particularly when working with React. This issue typically arises due to either one of two problems.
Firstly, the issue might stem from your definition of a functional or class component not being properly recognized by TypeScript as a valid JSX component. In React, a valid functional component should always return a JSX Element, hence TypeScript will also expect this as well.
Here’s an example where Typescript would throw the TS2786 error:
); } }
xxxxxxxxxx
class MyComponent {
render() {
return (
To fix this, you need to ensure the class extends from `React.Component` or `React.PureComponent`.
Correct implementation:
); } }
xxxxxxxxxx
import React from 'react';
class MyComponent extends React.Component {
render() {
return (
Secondly, this error can occur if Typescript doesn’t recognize the type of props being passed to the component. This could be resolved by defining types for our props or making use of TypeScript’s built-in utility types, such as `React.ComponentProps`.[source].
Example:
; }
xxxxxxxxxx
interface MyProps {
name: string;
}
function MyComponent({ name }: MyProps) {
return
Remember, TypeScript and JSX work together in harmony, but proper setup and syntax are essential to avoid issues like the TS2786 error. The overarching goal of validating Typescript usage with JSX isn’t just about nullifying these types of errors, but also to bring about effective type-checking and tooling for our JavaScript code.
“Any application that can be written in JavaScript, will eventually be written in JavaScript.” – Jeff Atwood (Co-Founder StackExchange).
Resolving TS2786 Error in Your React Typescript Project
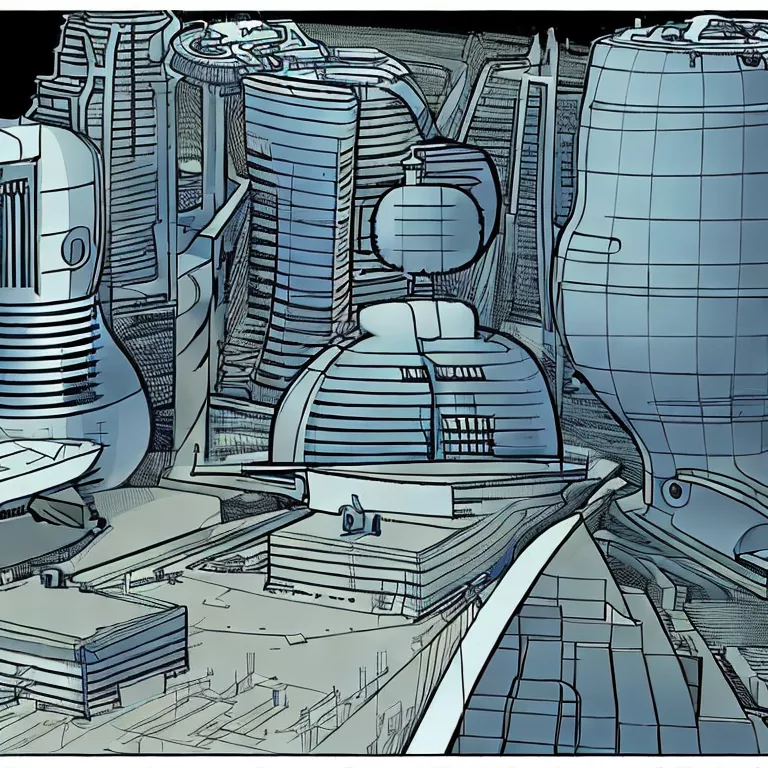
xxxxxxxxxx
TS2786
error in a React TypeScript project is typically encountered when trying to use a component that has not been properly defined or imported. This error appears as “TS2786: ‘ComponentName’ cannot be used as a JSX component. Its instance type ‘ComponentName’ is not a valid JSX element.” Here are several potential methods for resolving this issue:
Verify Component Export and Import
Ensure the component you’re trying to use is exported from its origin file correctly, and imported into the file where it’s being used. For example:
“Avoid named exports for the components return type and props, because Typescript can’t infer them properly.”
– Kent C. Dodds, JavaScript teacher and author
Your code may look like this:
xxxxxxxxxx
// MyComponent.tsx
export default function MyComponent(): JSX.Element { /*... */ }
xxxxxxxxxx
// App.tsx
import MyComponent from './MyComponent';
Make Sure You’re Using the Right Syntax
TypeScript uses unique syntax that is stricter than normal JS, so make sure you are using TypeScript-compatible syntax in your component.
Check For TypeScript Compatibility
Ensure that the libraries you’re using in your project are compatible with TypeScript. If they are not, look for TypeScript-friendly alternatives or consider creating custom types for missing definitions.
Installing @types/* Packages
If you’re using a library that doesn’t natively support TypeScript, installing the respective @types/* package might solve the problem.
xxxxxxxxxx
npm install @types/libraryname
Remember to replace “libraryname” with the actual name of the library.
Adding Type to Component
If the problem persists, consider explicitly adding type to your component. For instance:
xxxxxxxxxx
const Component: React.FunctionComponent=<{ /* component props here */ }>
Where ‘React.FunctionComponent’ denotes the return type of the react component function.
Official TypeScript documentation offers insightful references concerning JSX and React.
Case Studies of Common TS2786 Errors and Solutions
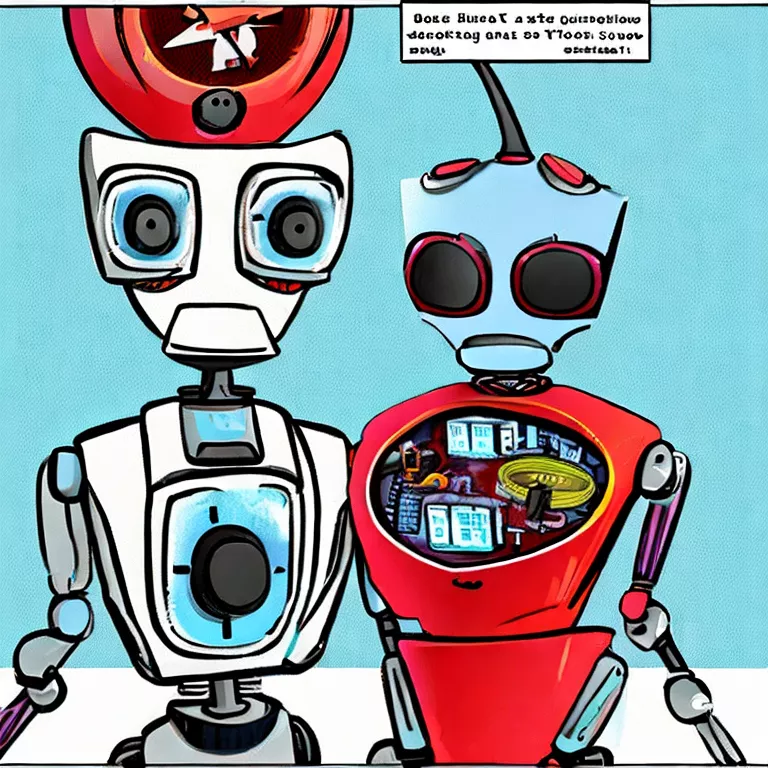
The TS2786 is an error that is likely to occur when working with JSX components in TypeScript. The error message typically reads as “TS2786: ‘Component’ cannot be used as a JSX component.”
This often happens when you try to instantiate a class or function component that doesn’t meet the required behavior for a functional (stateless) component or a class (stateful) component. This typically occurs when the entire lifecycle of a React component and the render function aren’t implemented correctly.
Error Case Study 1: You may encounter this error when you try to use a component which doesn’t return either JSX or null.
Consider the following example where we’ve created a component that doesn’t return anything:
xxxxxxxxxx
class MyComponent extends React.Component {
render() {}
}
In the above snippet, the render function has no return statement. This lack of proper return value triggers the TS2786 error because a component’s render method must return some JSX for the rendering phase to work properly.
To fix this problem, ensure that the render method returns something – either JSX or `null`:
); } }
xxxxxxxxxx
class MyComponent extends React.Component {
render() {
return (
Error Case Study 2: Another instance of the TS2786 error might occur when you declare a custom component using `typeof` and then try to insert it as an element into your JSX code.
For example:
Hello, world!
; } const Component = typeof HelloWorld; export function App() { return ( ); }
xxxxxxxxxx
function HelloWorld() {
return
In the above case, the `typeof` keyword was used. The correct approach would be directly assigning the `HelloWorld` function to the `Component` constant, like so:
xxxxxxxxxx
const Component = HelloWorld;
While TypeScript brings many benefits to JavaScript developers, it does come with its own unique set of errors that can at times be frustrating. Remembering these examples and best practices mentioned above can help when troubleshooting ‘TS2786 Component Cannot Be Used As A JSX Component’ error.
As the Dutch programmer, Wietse Venema, once said: “Programming is understanding”. Understanding what triggers these types of errors in a TypeScript context is vital for productive and pain-free development.
You can also check the official TypeScript JSX Documentation to understand more about how TypeScript interacts with JSX. Understanding these intricacies could really help you in the long run in avoiding other similar TypeScript errors.
Best Practices to Avoid “Component Cannot Be Used as a JavaScript XML (JSX) Component” Errors
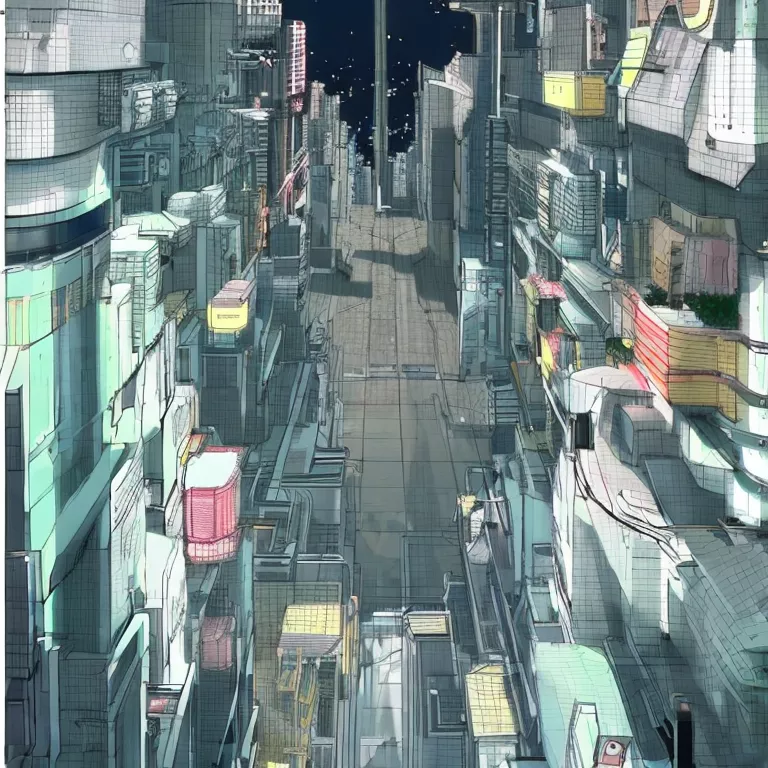
As a TypeScript developer, addressing the “TS2786: Component cannot be used as a JSX component” issue implies understanding the root cause of the matter, and putting forward effective solutions in line with best practices. This specific error often arises when your TypeScript compiler cannot find the function or class you’re trying to use as a JSX component, or fails to identify it as a valid JSX component due to different issues.
Understanding the Root Cause
– The titular “TS2786:Component cannot be used as a JSX component” is an error thrown by the TypeScript compiler when it unable to classify the intent of the object being rendered as a JSX element. Essentially, this means that TypeScript couldn’t validate your function or class as a valid React component.
– Generally, the typescript compiler specifies a certain format for what it considers a valid component. It checks if the prototype of your declared object extends from ‘React.Component’ or ‘React.PureComponent’. If not, it examines whether your object fits into the parameters of a “React.FunctionComponent” or a “React.VoidFunctionComponent”. In case neither condition is met, the error will surface.
Best Practices to Avoid TS2786 Error
Ensure the Correct Syntax
One common reason for encountering the TS2786 error includes wrongly declaring the component. Each component must abide by the following rules:
- Your component name should begin with an uppercase letter (as per JSX conventions)
- The structure of your components should either extend from
xxxxxxxxxx
React.Component
or
xxxxxxxxxx
React.PureComponent
for class components, and for functional components, they should be arrow functions or function declarations which return a JSX element.
Here’s an example of a correct function component:
xxxxxxxxxx
<Script>
const MyComponent: React.FC = () => {
return (<div>This is a test component</div>);
};
</Script>
Install and Import react Type Declarations
Another common mistake is failing to install or import the @types/react, which supply TypeScript with necessary context about React’s primitives. Here’s how to set it up:
xxxxxxxxxx
npm install --save @types/react
import *as React from 'react';
Properly Set Up Your tsconfig.json
Ensure that your TypeScript configuration file tsconfig.json has its compilerOptions properly set. For instance:
xxxxxxxxxx
<Script>
{
"compilerOptions": {
"jsx": "react"
}
}
</Script>
This directs TypeScript on how to handle JSX syntax. The ‘jsx’ options can be set to ‘preserve’, ‘react-native’, or ‘react’.
To quote Linus Torvalds, the creator of Linux, “Talk is cheap. Show me the code.” Indeed, understanding these best practices is a key step for effectively eliminating such errors in the future. Each of these practices contributes to a more predictable and manageable TypeScript environment, while aligning strongly with overall industry standards.
Conclusion
From a development standpoint, the TS2786 error can be a stumbling block when working with Typescript in the React ecosystem. This error essentially communicates that a particular component cannot be used as a JSX component. It is typically encountered when there’s a mismatch between what Typescript expects and what you’re actually putting forth in your JSX syntax.
The root cause of this issue leans towards how Typescript determines whether or not an entity can legitimately be used as a JSX component. This process heavily depends on if it passes the ‘IntrinsicComponent’/’ExtrinsicComponent’ test.
Definition | Description |
---|---|
xxxxxxxxxx IntrinsicComponent |
A component that is directly built into the framework, like a metaphorical built-in keyword – for example, in React, these would be tags like
xxxxxxxxxx div , xxxxxxxxxx span , etc. |
xxxxxxxxxx ExtrinsicComponent |
Sometimes referred to as ‘user components’, these are components explicitly defined by the user in their codebase – like App or Sidebar component we create in React |
When dealing with such issues, ensuring that the necessary components match up with Typescript’s expectations regarding their typing definitions proves to be the backroad leading to resolution of the problem.
It’s always important to keep in mind that comprehensive troubleshooting necessitates an understanding of both the basic and more nuanced aspects of Typescript’s interaction with JSX. Layers of complexity added by custom typings, variables, and function usage pose botheration, yet thorough comprehension holidayates developers from incessantly facing run-time and compile-time errors similar to TS2786.
As Dan Abramov, a core team member of the React project put perfectly,
“Code is like humor. When you have to explain it, it’s bad.”
Evidently, the TS2786 error underlines the principle that clear, succinct, and well-structured code not only aids in seamless development but also diminishes the need for verbose explanations or clarifications.