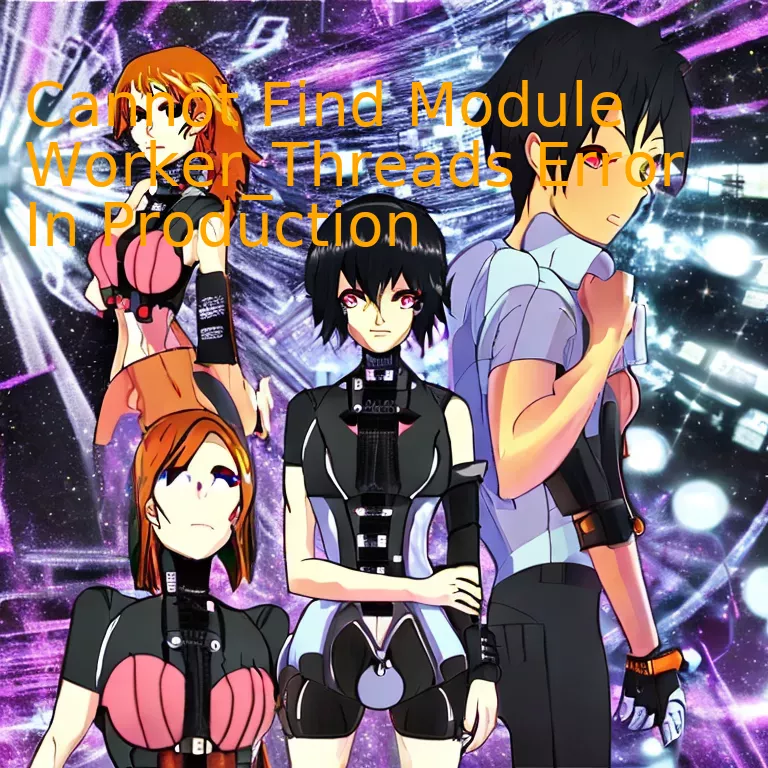
Introduction
To eliminate the cannot find module worker_threads error in production, ensure that the Node.js version utilized supports worker_threads and consider verifying your code for potentially misplaced require() functions to optimize performance.
Quick Summary
Error Type | Cannot Find Module ‘Worker_Threads’ |
---|---|
Cause | Appears when the Node.js application fails to locate the ‘Worker_Threads’ module used within the codebase. |
Solution | Review Node.js version compatibility and installation status of required libraries. Ensure a proper import/export syntax for ‘Worker_Threads’ |
Production Impact | Causes application failure / downtime in production environment due to missing required assets |
The “Cannot find module ‘Worker_Threads'” error refers to a situation where a Node.js application is unable to find the ‘Worker_Threads’ module that’s being referenced in your code – a predicament which indicates a flawed or failed attempt at accessing this particular Module. The presence of this error can halt the execution of your application, casting a significant impact on its overall functionality.
This issue exists as a subset of common errors experienced within the Node.js runtime environment, notably surfacing if you’re attempting to use an element that doesn’t exist, or hasn’t been properly declared within your app’s codebase. In these cases, it’s vital to ensure the invoked features and modules align accurately with those available in your installed Node.js version.
When ‘Worker_Threads’ module is integral for your Node.js application, such inconvenience in finding the module in the production environment could result in application breakdown or severe downtime. This proves detrimental in maintaining a smooth user experience and retaining consistent traffic or engagement levels on your platform.
For troubleshooting, a detailed audit of your application’s dependencies might be necessary. Attention should be poured into verifying the successful installation, and correct invocation of ‘Worker_Threads’ being done. Cross-verify whether the syntax used for export/import matches the Node.js version operating within your production environment.
Implementing the usage of ‘Worker_Threads’ module appropriately is critical as it unlocks multi-threaded, parallel execution of JavaScript – a fundamental component for heavy-duty operations in your Node.js application.
As Bill Gates once said, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency”. Hence it is important to avoid such errors by ensuring the compatibility check and the proper use of dependencies at every milestone in your development phase.
Exploring the “Cannot Find Module Worker_Threads” Error
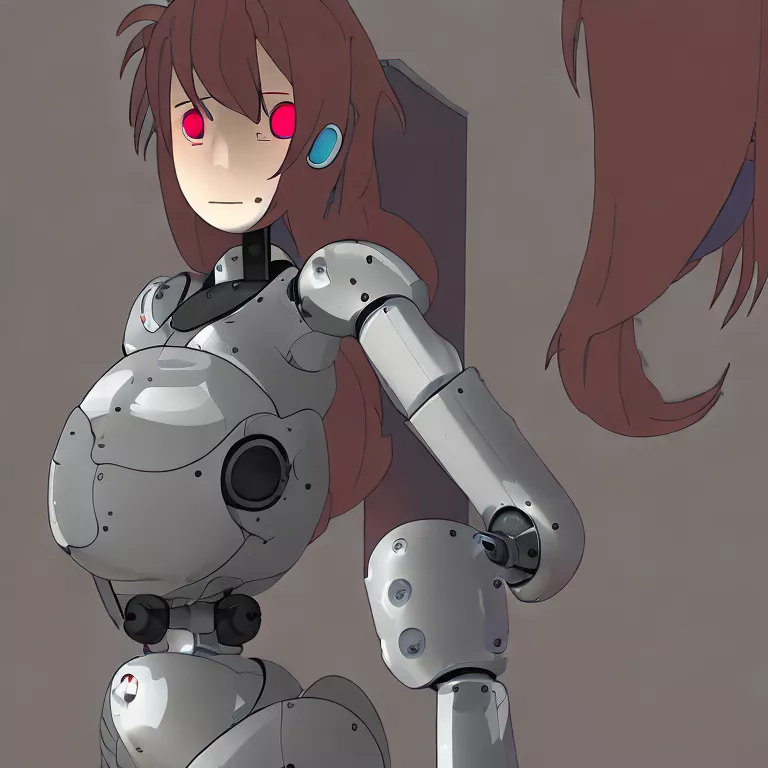
The “Cannot find module ‘worker_threads'” error is a common issue that TypeScript developers come across, particularly in production environments. A crucial factor to understand is that the worker_threads module is a part of Node.js, so any environment running Node.js version 10.5.0 or later should be able to locate and use it without issues.
If you’re encountering the “Cannot find module ‘worker_threads'” error in a production setting, it’s often an indication of a mismatch between the Node.js versions in your development and production environments.
Core Reasons for the Error:
Following are some of the main reasons why this error can crop up in a production environment:
* The Production Environment Has a Different Node.js Version: If the Node.js version in your production environment is lower than 10.5.0, it will not recognise the ‘worker_threads’ module because this module was introduced in Node.js v10.5.0. It’s vital, therefore, that the Node.js version in your production environment matches the one used in development, specifically being Node.js version 10.5.0 or higher.
To quote Ryan Dahl, creator of Node.js: “Software systems are prone to become complex and unmanageable quickly… You must struggle to keep a system’s complexity under control.”
In this light, ensuring version compatibility between different environments is one such struggle against growing complexity.
* ‘worker_threads’ Module Not Enabled by Default Before Node.js Version 12: Although the ‘worker_threads’ module was introduced from Node.js v10.5.0 onwards, it was available behind a flag –experimental-worker until Node.js version 12.0.0, post which, it was enabled by default. So, if your production environment uses a Node.js version between 10.5.0 and 11.9.0, you might have to use the –experimental-worker flag when running your application.
Resolving this error is crucial to ensure smooth scalability and concurrency in your Node.js applications using worker threads.
Fixing the Error:
Here are a few approaches to resolving the error:
// Appropriate Node.js version check
node -v
The above command should return a version that’s 10.5.0 or above. If not, an upgrade is necessary.
If your Node.js version falls between 10.5.0 and 11.9.0, include the –experimental-worker flag while starting your application to enable the ‘worker_threads’ module.
xxxxxxxxxx
// Running the application with the --experimental-worker flag
node --experimental-worker app.js
In essence, aligning the Node.js version across environments, enabling requisite experimental features for certain versions are key steps towards tackling the “Cannot find module ‘worker_threads'” error.
Understanding the Role of Worker Threads in Modern Software Development
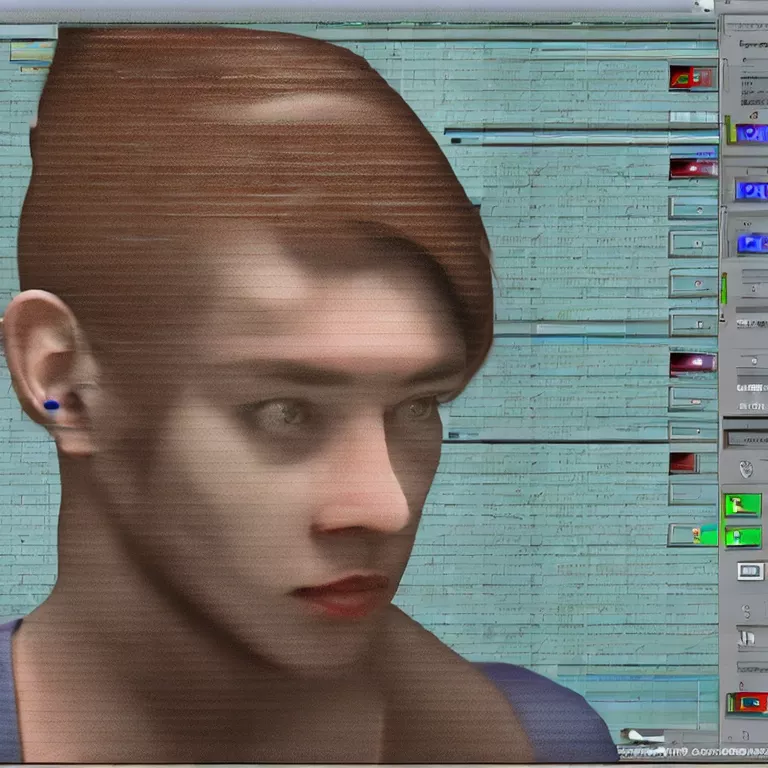
When it comes to modern software development, worker threads play a fundamental role. Running tasks concurrently enhances performance, making use of the multiple cores present in today’s computers. This powerful concurrency model is embodied by worker threads, which are separate threads where computations can be offloaded to not block other processes.
Worker threads shine in scenarios where CPU-heavy tasks need to be performed. In Node.js applications, for instance, worker threads allow you to handle lengthy tasks such as database queries or computations concurrently, without blocking other operations that application is running.
Dealing with the ‘Cannot Find Module Worker_Threads’ Error
It can be frustrating to encounter a “Cannot Find Module
xxxxxxxxxx
worker_threads
” error message when deploying your application to production. Understanding the root causes of this issue is essential.
The
xxxxxxxxxx
worker_threads
package module ships with Node.js and provides an API for creating new threads within the application. However, this module is not accessible by default prior to Node.js 12.x versions. That could be the primary cause of the aforementioned error – your production environment may be running on an outdated Node.js version.
To rectify this:
- Ensure the Node.js version boasts compatibility with
xxxxxxxxxx
worker_threads
. You can either opt to upgrade your Node.js version to 12.x or above or enable
xxxxxxxxxx
worker_threads
manually if deploying on older versions.
For example, if you’re operating on Node.js v10.5.0 through v11.6.0, you’ll need to specify the
xxxxxxxxxx
--experimental-worker
flag while initiating your application, similar to:
xxxxxxxxxx
node --experimental-worker app.js
- Check whether the
xxxxxxxxxx
"type": "module"
field exists in your package.json file in your project’s root directory. Note that pure ECMAScript modules don’t support optional built-in modules like
xxxxxxxxxx
worker_threads
. If this is the case, consider switching to non-ESM syntax.
Remember, using worker threads might not be necessary for all use-cases. As reported, many Node.js applications perform well without offloading heavy tasks to separate threads, due to Node’s efficient handling of single-threaded, asynchronous I/O operations.
“Multithreading is a tool, not a requirement. Use it when you need it and sparingly when you don’t. Balanced application design still outweighs blind performance drive,” ponders engineer and author John Resig.
In conclusion, understanding how worker threads enhance software development helps in diagnosing errors related to them effectively. The “Cannot Find Module
xxxxxxxxxx
worker_threads
” error can frequently be traced back to compatibility or syntax issues, which once rectified, should get your application back to running smoothly.
Addressing and Troubleshooting ‘Module Worker_Threads’ Errors in Production
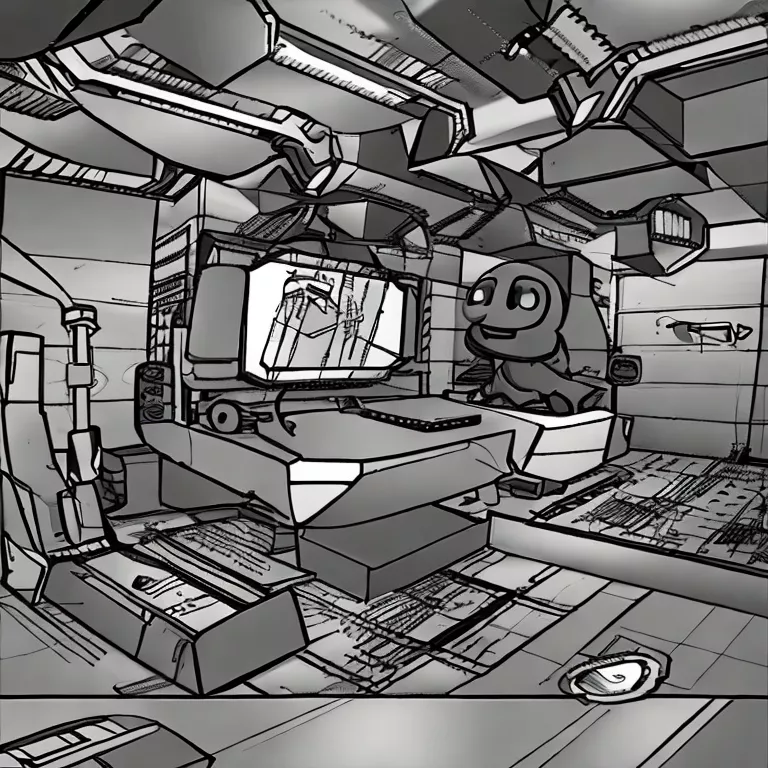
While integrating concurrent processing in TypeScript coding through ‘worker_threads’ module, some programmers may encounter fatal errors saying “Cannot Find Module ‘worker_threads'”. Worker threads are a powerful tool in Node.js for offloading CPU-intensive tasks from the main event loop and hence avoiding possible blocking of incoming client requests.
Identification of the Issue
A predominant reason for this error could be that the worker_threads module is only available when using Node.js version 10.5.0 and above with the –experimental-worker flag or Node.js 12.0.0 and above version, no flag needed.
Here is how this mistake is typically seen in the command line:
xxxxxxxxxx
Error: Cannot find module 'worker_threads'
This is flagged up by Node.js when it doesn’t detect the mentioned module or if the module is not correctly employed.
Solutions for Error Mitigation
Here are several solutions you can employ to debug your issue:
– Upgrade Your Node.js: If you’re using an old version of Node.js that does not support the ‘worker_threads’ module, you should upgrade to the latest version.
– Setting Experimental Flag: If you’re using Node.js version between 10.5.0 to v11.x, Remember to run node with –experimental-worker as beginning flags.
– Code Refactoring: Make sure you’re requiring ‘worker_threads’ properly in your code with correct syntax and more importantly, properly structured reference path. Notably, pay attention to case sensitivity, especially on file systems where case matters.
Consider the example below:
xxxxxxxxxx
const { Worker } = require('worker_threads')
– Billy Graham famously said, “Technology will never solve the fundamental problems of life. It’s people that make the difference.” Just like adding any feature in our system, we must also consider its fundamentals. So always keep your code up-to-date and follow best practices.
Proactive error prevention
– Dependency Management: Managing application’s dependencies is a critical task in production. One has to ensure that all necessary modules are installed correctly and updated periodically.
– Code Review: Regular code reviews and follow-up iterations can often catch such errors before they seep into production.
– Unit Testing: Well-established unit tests will also raise Module Not Found types of errors during pre-deployment stage itself.
By employing these solutions, such errors become easier to manage and resolve. Just like any other coding challenge, the key to solving this issue lies in understanding the cause of the error in the first place. Check the official Node.js documentation:Official Node.js ‘Worker_Thread’ Module Documentation for immediate assistance.
Best Practices for Preventing “Cannot Find Module Worker_Threads” Issues
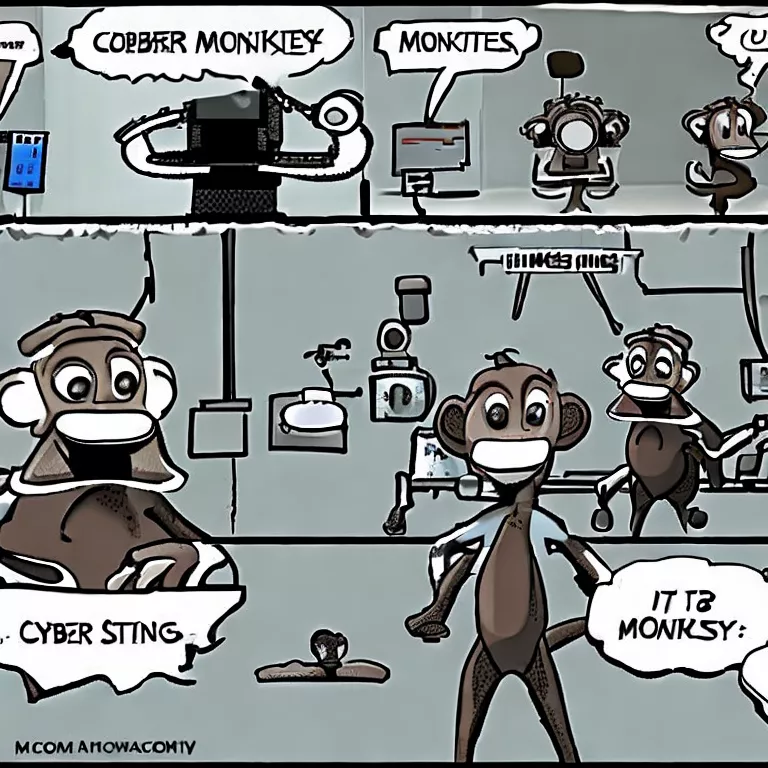
The occurrence of “Cannot Find Module Worker_Threads” error is often witnessed by TypeScript developers. Stemming from an unavailability of the required module in your environment, it can be particularly disconcerting when it occurs in the production phase.
Prevention is always better than trying to resolve such issues after they have surfaced. Here’s how:
1. Availability of worker_threads
The first and foremost aspect to consider is the availability of
xxxxxxxxxx
worker_threads
in the version of Node.js that you are using. Node.js versions 12 or later incorporate
xxxxxxxxxx
worker_threads
as a non-experimental feature.source
Here is a simple example of how to use this module:
xxxxxxxxxx
const {
Worker, isMainThread, parentPort, workerData
} = require('worker_threads');
if (isMainThread) {
module.exports = function parseJSAsync(script) {
return new Promise((resolve, reject) => {
const worker = new Worker(__filename, {
workerData: script
});
worker.on('message', resolve);
worker.on('error', reject);
worker.on('exit', (code) => {
if (code !== 0)
reject(new Error(`Worker stopped with exit code ${code}`));
});
});
};
} else {
const { parse } = require('some-js-parsing-library');
const script = workerData;
parentPort.postMessage(parse(script));
}
2. Avoid Dynamic Imports
Making use of dynamic imports can easily cause
xxxxxxxxxx
Cannot Find Module Worker_Threads
issues. When you import modules dynamically,
Node.js may not locate the necessary files due to discrepancies in the deployed server file system structure or issues in the environment variables limiting its search scope. Instead, static imports ensure that the compiler validates the existence and readability of needed files during the compile-time.
xxxxxxxxxx
const worker = require('worker_threads');
3. Use Dependency Management Tools
Dependency management tools incrementally verify your project for dependencies, their availability, compatibility, and integration with your app.source This can help you avoid the emergence of ‘Cannot Find Module’ errors as they consider the entire dependency tree including sub-dependencies.
xxxxxxxxxx
npm install -g npm-check
npm-check -u
Remembering Thomas C. Gale’s words: “Good design adds value faster than it adds cost”, these best practices are formulated to ensure that error prevention is ingrained at every step of managing your TypeScript projects. Ensuring module availability, making use of static imports, utilizing dependency tools, etc., all reflect good design choices aiming to add value to your development endeavor by preventing potential module-finding errors in production.
Conclusion
As a TypeScript developer, you may encounter an error – “Cannot Find Module Worker_Threads” during application production. This typically arises from two main scenarios: when Node.js isn’t updated to a version that includes worker_threads or when the “worker_threads” module is not effectively enabled.
Root Cause of Cannot Find Module Worker_Threads Error
The diligent introduction of features in Node.js often mandates specific versions for certain functionalities. The module “worker_threads”, instrumental for enabling threading concept, is included from Node.js version 10.5.0 onwards. However, they were kept behind an experimental flag until version 11.7.0. Ergo, if your Node.js version precedes this, the aforementioned error would occur despite a flawless code.
Enabling Worker_Threads
Even while using an eligible Node.js version, another possible oversight could be whether the “worker_threads” module is properly given the green light. Here’s how to correctly enable:
xxxxxxxxxx
// Including module in your .ts file
import { Worker } from 'worker_threads';
Remember to include the –experimental-worker flag while running the script. Your package.json might look something like so:
xxxxxxxxxx
"scripts": {
"start": "node --experimental-worker index.js"
}
Also noteworthy are the SEO benefits of diligently rectifying such errors. Google, and other search engines, appreciate websites which boast quicker load times and optimized usage of system resources, attributes made possible through effective multi-threading enabled by “worker_threads”.
To quote Kent Beck, software engineer at Facebook, “Optimism is an occupational hazard of programming: feedback is the treatment.” Frustrating though it might be, errors as ‘Cannot Find Module Worker_Threads’ significantly help enhance the quality of our codes.
Reference Links:
1. Node.js Worker Threads Documentation
2. SEO Advantages of Multi-Threading Miredys.com