Introduction
Ensuring a unique declaration for each component in a single NgModule proves beneficial by eliminating confusion when an unexpected system anomaly named “component is declared by more than one NgModule” appears in Angular applications.
Quick Summary
When working with Angular, an error known as ‘The Component Is Declared By More Than One NgModule’ may occur. This issue arises when the same component is declared in more than one NgModule. It’s a best practice to declare a component within a single NgModule and then import it where it’s required. Let’s take a deeper look:
Error | Cause | Solution |
---|---|---|
The Component Is Declared By More Than One NgModule | A single component is declared in multiple NgModules. | Declare the component in a singular NgModule only and import it where necessary. |
Angular employs a modular structure, encapsulating related elements together within separate modules to improve maintainability, testing, and reusability within applications. However, declaring a component in multiple modules is against the framework’s standards, generating conflicting scenarios, and that’s what inspires this error message.
Components are the primary building blocks of Angular applications, providing templates that are used to magnify views, which are directly displayed in the browser. A component encapsulates functionality for the app, absorbing data into properties that can be bound to specific syntax in view templates. A simple component declaration in a module would look like this:
@NgModule({
declarations: [MyComponent],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent]
})
To resolve this issue, you can adhere to an Angular best practice known as ‘Feature Modules.’ These modules allow you to organize your code around application’s features. You can declare a component in its associated feature module, and later import this module wherever the component’s needed.
As Douglas Crockford, the creator of JSON, once said, “Programming is not about typing… it’s about thinking.” Thus, a well-structured Angular program brings us one step closer to better software development.
Understanding the Concept of NgModule in Angular
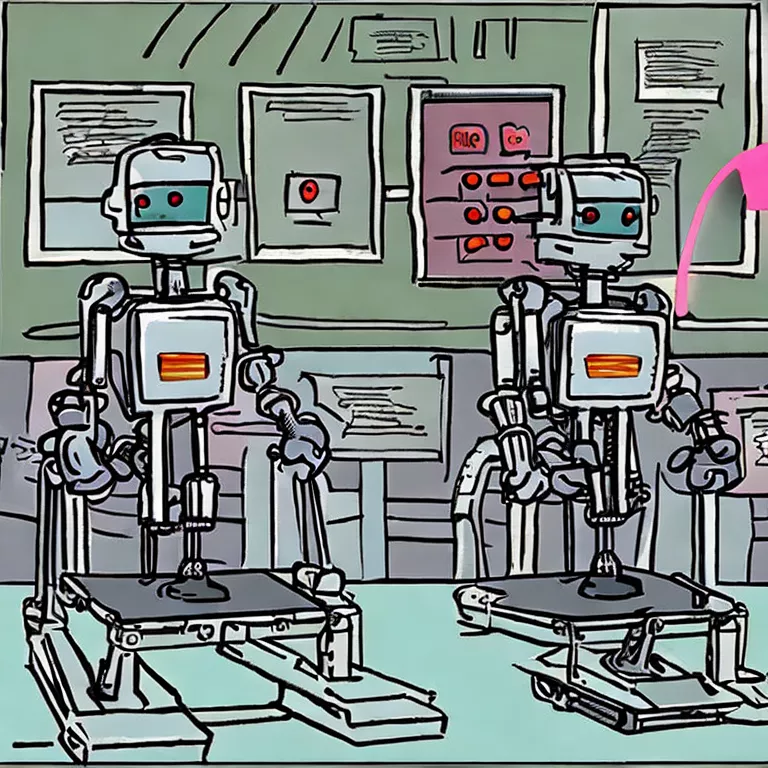
The concept of NgModule in Angular provides a consolidated package of functionality that can be used across multiple components. Notably, each Angular application has at least one NgModule class— the root module— often named AppModule. This module presents a logical way to organize components, directives, pipes and services into cohesive blocks of code with a shared purpose.
Coding Structure
The general structure of an NgModule class in TypeScript will typically follow this pattern:
xxxxxxxxxx
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
We delve further into the NgModules module on the Angular website.
The Component Is Declared By More Than One NgModule
In relation to ‘the component is declared by more than one NgModule’ issue, it comes up when the same component is recognized as part of multiple NgModules. Angular’s compiler doesn’t fancy it. Within Angular, every component must be exclusive to one NgModule.
Here’s an example that leads to this problem:
xxxxxxxxxx
//First Module
@NgModule({
declarations: [SharedComponent],
exports: [SharedComponent]
})
export class FirstModule {}
xxxxxxxxxx
//Second Module
@NgModule({
declarations: [SharedComponent],
exports: [SharedComponent]
})
export class SecondModule {}
Both modules are trying to declare the SharedComponent, causing Angular’s compiler to raise an error. The solution would be to create a third module, a SharedModule for instance, which declares and exports the SharedComponent. Then you simply have both modules import the SharedModule:
xxxxxxxxxx
//Shared Module
@NgModule({
declarations: [SharedComponent],
exports: [SharedComponent]
})
export class SharedModule {}
xxxxxxxxxx
//First Module
@NgModule({
imports: [SharedModule]
})
export class FirstModule {}
xxxxxxxxxx
//Second Module
@NgModule({
imports: [SharedModule]
})
export class SecondModule {}
“Now, isn’t that a beauty?” as Charles Petzold, author of various programming books said, denoting the elegance and efficiency in this coding structure.
To avoid ‘the component is declared by more than one NgModule’ problem altogether, try to design your NgModules so they align with the feature areas or shared utility classes/components/services within your application. This helps you manage dependencies across different sections of your app. Clear guidelines on how to do this are provided in Angular’s Angular Style Guide.
Common Mistakes: Declaring a Component in Multiple NgModules
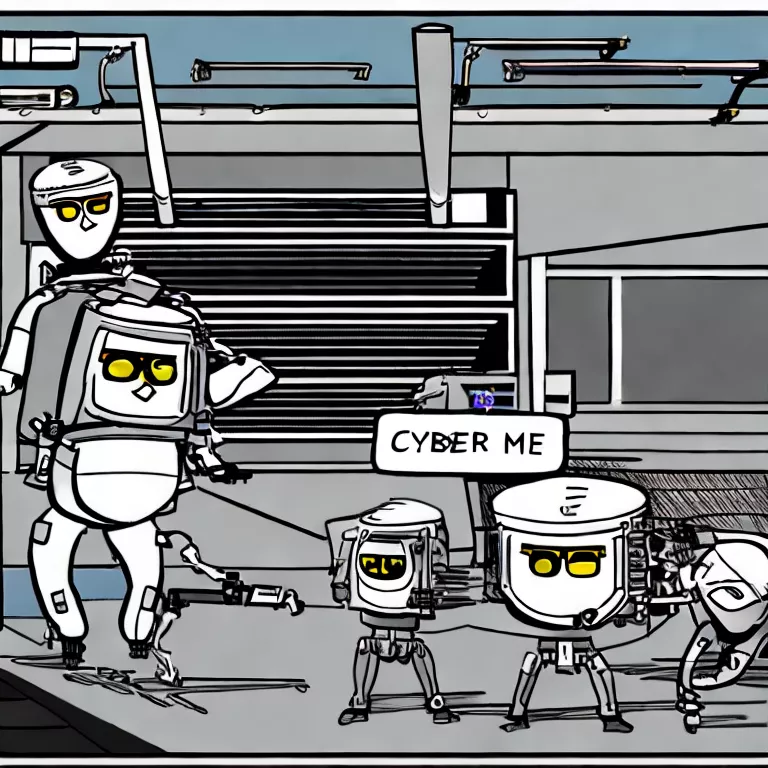
Declaring a component in more than one NgModule can lead to unexpected and difficult-to-debug errors in your Angular application. Such a scenario goes against the modularity principles of Angular, affecting the smooth functioning of your app. Here are some issues you may encounter and ways to solve them:
• Duplicate Declaration Error: Angular will throw an error if a component is declared in more than one NgModule. Although Angular supports shared components through the ‘exports’ array of the NgModule, it restricts declaring the same component in multiple NgModules for maintaining singleton behavior.
xxxxxxxxxx
// AppModule
@NgModule({
declarations: [MyComponent],
})
export class AppModule { }
xxxxxxxxxx
// OtherModule
@NgModule({
declarations: [MyComponent], // Duplicate declaration error
})
export class OtherModule { }
The solution here would be to structure your code such that each component belongs to just one NgModule.
• Unanticipated Behaviour: If somehow Angular does not throw error due to some workaround and a component belongs to more than one NgModule, you might face unpredictable consequences like improper change detection, event handling, life cycle discrepancies etc.
To avoid these complications, it’s essential to understand and follow a few best practices:
– Use SharedModule: If you need to use a component in multiple parts of your application, consider using a SharedModule. This module can encapsulate the shared component and other common codes, and then be imported wherever needed.
xxxxxxxxxx
// Shared module
@NgModule({
declarations: [CommonComponent],
exports: [CommonComponent]
})
export class SharedModule { }
– Restructure Your Code: Always restructure your modules and components in a way that promotes component uniqueness in its declaration.
– Use CoreModule for Singleton Services: Sometimes, you might need to provide a service that’s available throughout the app. In such cases, use CoreModule.
xxxxxxxxxx
// Core module
@NgModule({
providers: [SingletonService],
})
export class CoreModule { }
As code developer Jack Dorsey once said, “The greatest technology will be remembered for how it connected people and provided them with simple solutions.”(source) This is true for Angular development as well; by avoiding common mistakes like declaring a component in multiple NgModules, you can more easily write clean, maintainable code.
Resolving Issues with Duplicate Component Declarations
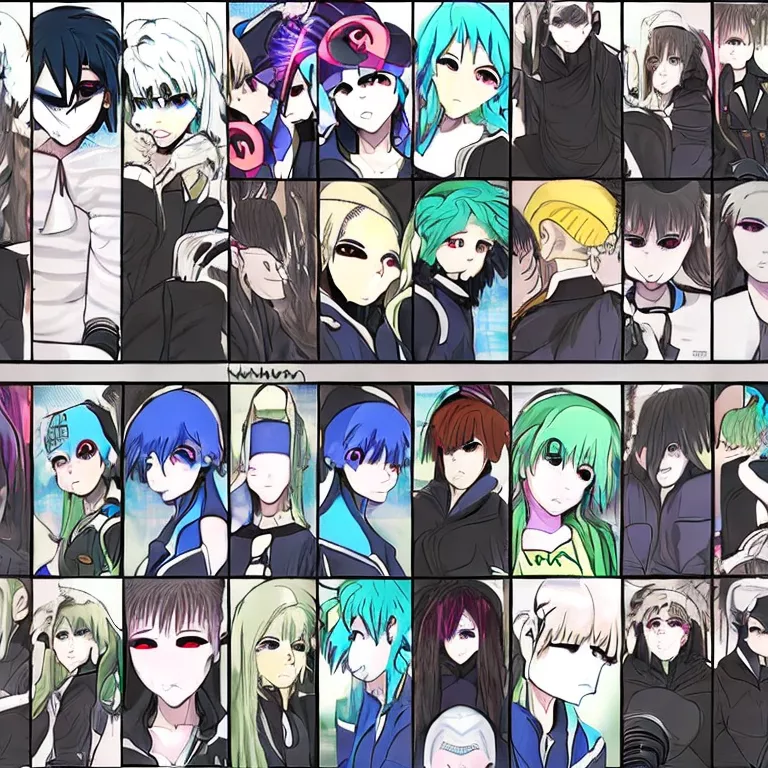
Web developers may frequently encounter an issue while working with Angular, where a component is declared in more than one NgModule. This challenge often materializes specifically when you reuse a component across multiple modules. Though the goal of code reusability is well-intended, it often can lead to complications and errors if not managed properly.
To understand this issue better, let’s consider a simple situation. We have `ComponentA`, which is declared in both `Module1` and `Module2`. Here’s how that would look:
xxxxxxxxxx
@NgModule({
declarations: [ComponentA],
imports: [],
})
export class Module1 {}
@NgModule({
declarations: [ComponentA],
imports: [],
})
export class Module2 {}
Angular does not allow a single component to be declared in more than one module. So in this instance, Angular will throw an error stating ‘ComponentA is part of the declarations of 2 modules’.
Solution: Instead of declaring ‘ComponentA’ in both `Module1` and `Module2`, create a shared module (let’s call it `SharedModule`) and declare ‘ComponentA’ only in the `SharedModule`.
Here is how the code declaration should look:
xxxxxxxxxx
@NgModule({
declarations: [ComponentA],
exports: [ComponentA]
})
export class SharedModule {}
@NgModule({
imports: [SharedModule],
})
export class Module1 {}
@NgModule({
imports: [SharedModule],
})
export class Module2 {}
In the example above, I’ve created the SharedModule and declared ComponentA here. The ‘exports’ array then makes ComponentA available for other modules via the import statement. Both `Module1` and `Module2` now import this `SharedModule`, thus gaining access to the declarations within it. This prevents the development pitfall of defining a component in multiple modules, overcoming the challenge and adhering to Angular’s best coding practices.
It is crucial to remember the wisdom shared by computer scientist Robert Biddle: “Programs must be written for people to read, and only incidentally for machines to execute”. Following this ideology not only ensures unambiguous coding but allows you to tackle problems like these much more efficiently.
The `SharedModule` mechanism optimizes code reusability while circumventing declaration issues effectively. Now that we’ve isolated ComponentA within its dedicated SharedModule, you can now seamlessly reuse it across multiple modules without the risk of redundant declarations. So, through this methodology, you’re able to reduce redundancy, streamline your codebase, and simultaneously improve code readability.
Best Practices for Declaring Components in Angular’s NgModule
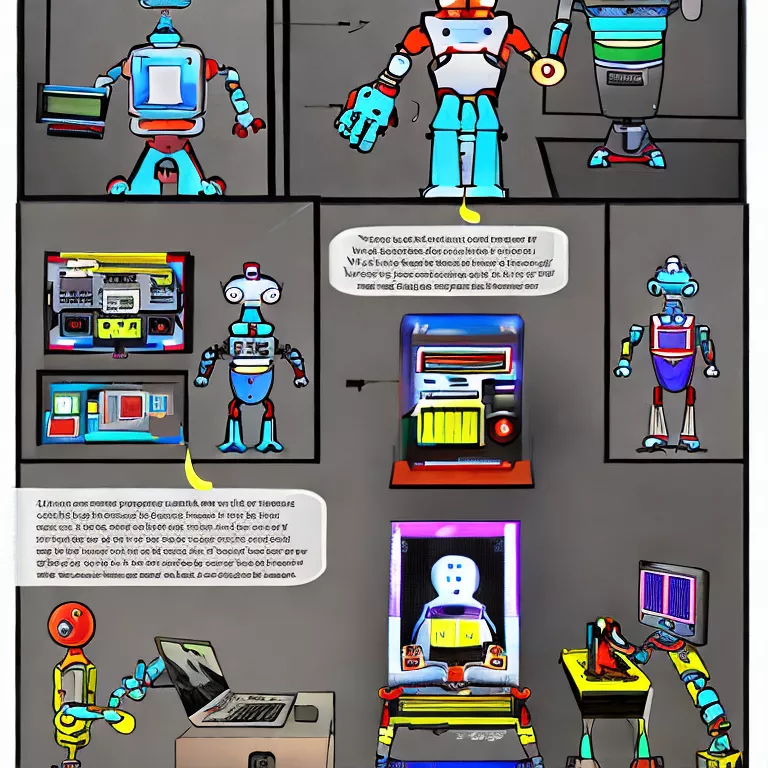
Declaring components in Angular’s NgModule can sometimes become a point of contention when working with larger applications or collaborative teams. It is crucial to follow best practices to prevent issues such as multiple declarations of the same component. Such scenarios arise when a particular Component is Declared by More Than One NgModule, leading to complexities and errors.
Let’s comprehend these best practices:
-
- Declare Components Only Once: In an ideal Angular application, each component should be declared only once in a specific NgModule. Subsequently, this module can then be imported into other modules where that component is required.
For example, suppose ‘ExampleComponent’ needs to be used across the application. Declare it first in the ‘SharedModule’ as:
xxxxxxxxxx
@NgModule({
declarations: [ExampleComponent],
exports: [ExampleComponent]
})
export class SharedModule { }
Then, import the SharedModule wherever ‘ExampleComponent’ is required instead of re-declaring the component.
xxxxxxxxxx
@NgModule({
imports: [SharedModule]
})
export class SomeOtherModule { }
-
- Maintain Modularity: Plan the application structure around clear, distinct features encapsulated in their respective modules. This practice reduces the risk of accidental multiple declarations.
- Utilize Angular CLI: Angular CLI tooling is designed to automate & simplify many tasks, including generating new components and automatically adding them to a module’s declaration array.
xxxxxxxxxx
ng generate component example
This command creates the ‘example’ component and automatically declares it in the NgModule.
- Avoid Lazy Loading Ambiguity: Ensure that any component declared within a module that’s meant for lazy loading isn’t declared elsewhere. Failing to do so may lead to duplicate component errors.
Avoiding multiple declarations of the same component in different NgModules is fundamental to adhere by Angular’s design philosophy. As Brendan Eich, creator of JavaScript, once said, “Always bet on JavaScript.” Trusting the system and conventions set up by Angular will lead to a cleaner, more efficient application.
To learn more about Angular NgModule, visit the official Angular NgModule guide.
Conclusion
In providing solutions to this issue of component declaration within multiple NgModules, it is essential to analyze the implications firstly. Angular operates in such a way that every component should be declared within one module for clear context and code compartmentalization. However, when a component is declared in more than one NgModule, confusion arises, leading to difficulties in determining which module to load. Furthermore, any modifications made to the component within one module will not reflect in another.
Understanding and observing the concept of a single responsibility is vital in these situations. It allows developers to ascertain that a specific component has its exclusive NgModule. This approach provides tangible benefits:
- Enhancement of code readability
- Promotion of code adaptability and maintainability
- Elimination of the possibility of component duplication
In cases where more than one NgModule requires a particular component, you can share the said component across different modules. To accomplish this, declare the desired component in a SharedModule to make it available. Here is a succinct TypeScript example for illustration:
xxxxxxxxxx
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { SharedComponent } from './shared.component';
@NgModule({
declarations: [SharedComponent],
imports: [
CommonModule
],
exports: [SharedComponent]
})
export class SharedModule { }
In your desired NgModule, import the SharedModule:
xxxxxxxxxx
import { SharedModule } from './shared.module';
@NgModule({
imports: [
SharedModule
],
})
export class DesiredModule { }
Consequently, adherence to Angular’s recognized practices eliminates the occurrence of issues related to components being declared by more than one NgModule. Hone your development skills by familiarizing yourself with Angular architecture best practices, thereby offering quality solutions for any arising complexities.
The words of Addy Osmani, Google’s Engineering Manager for Chrome – “First do it, then do it right, then do it better” – is pertinent in this context. Improving your TypeScript skills necessitates understanding the basics first before refining your technical know-how and writing durable code.