Introduction
Incorporating TypeScript with Vue.js 3, one may come across an issue where the property $store does not exist on the type ComponentPublicInstance. To resolve this, ensure that your Vuex store is properly set up and accurately imported into your project; a common error stems from incorrect Vuex integration.
Quick Summary
The issue of “Property `$store` Does Not Exist On Type `ComponentPublicInstance`” in Vue.js 3 with Typescript is a common challenge developers face when transitioning from Vue 2 to Vue 3. It typically occurs due to the change in how Vue 3 handles the injection of the $store property into its components.
Concept | Vue.js 2 | Vue.js 3 |
---|---|---|
$store Property Injection | Automatically injects $store into every component | Does not automatically inject $store, requires explicit declaration |
Usage in Components | Accessible using “this.$store” | Options API: still “this.$store”; Composition API: use “useStore()” instead |
In Vue.js 2, the Vuex library would automatically inject the $store property into every component, and it was universally accessible as `this.$store`. Moving to Vue.js 3, however, does not automatically provide this functionality which results in the error mentioned above.
To overcome this, you need to make some adjustments based on whether you’re using the Options API or Composition API for your components.
For those utilizing the Options API, you are required to explicitly declare that your component has access to the $store property:
declare module '@vue/runtime-core' { interface ComponentCustomProperties { $store: Store; } }
However, if you are employing the Composition API, you’ll need to use the `useStore()` function provided by Vuex.
import { useStore } from 'vuex' export default { setup() { const store = useStore() //... } }
Akin to Linus Torvalds’ words, “Talk is cheap. Show me the code,” these examples provide a direct solution to the issue caused by the change in Vue.js’s handling of $store between its second and third versions.
It’s crucial for developers to familiarize themselves with the changes in how libraries like Vuex handle storing and retrieving state data when moving between versions, particularly when using Typescript. Ensuring your components have proper access to resources such as $store can prevent minor headaches during development and make the transition process much smoother.
Remember, as Jamie Zawinski famously said, “Every problem in computer science can be solved by another level of indirection,” this includes managing state in major modern front-end frameworks like Vue.js.
Understanding the Relationship between Vue.js 3 and TypeScript
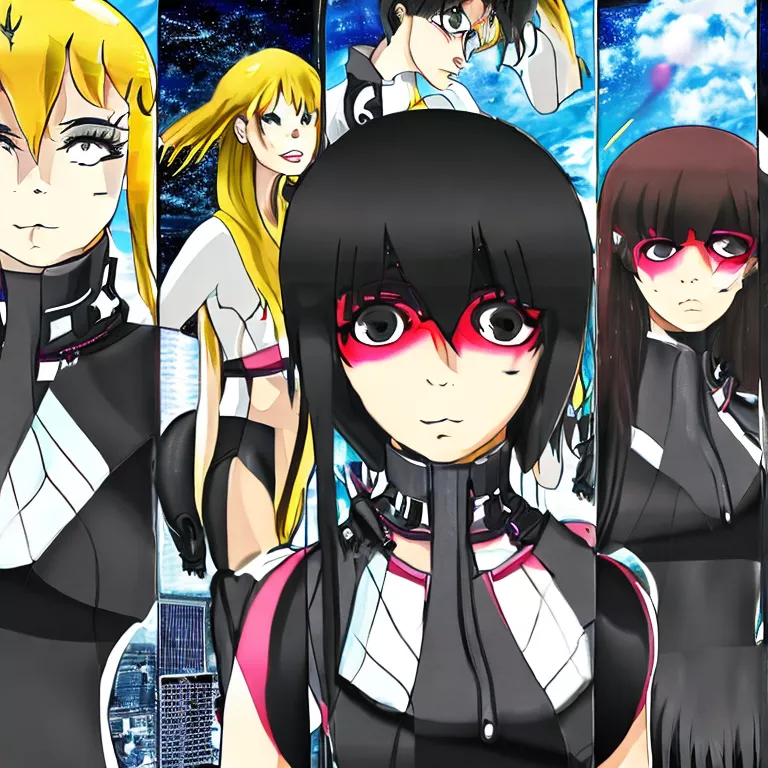
Understanding the relationship between Vue.js 3 and TypeScript, particularly regarding the “`Property $Store Does Not Exist On Type Componentpublicinstance`” error, requires a deep dive into both JavaScript technologies.
Vue.js 3, the latest version of the Vue.js framework, has been designed from the ground up to take full advantage of TypeScript. TypeScript, on the other hand, is a statically-typed superset of JavaScript that enhances JavaScript by introducing static types, interfaces, generics, and more advanced language features. If used in tandem with Vue.js 3, TypeScript presents a more robust, maintainable, and scalable codebase.
Despite their complementary nature, there can be occasions when interface mismatches occur. An example includes encountering the “Property $store does not exist on type ‘ComponentPublicInstance'” error. This suggests that there’s an issue with accessing Vuex store inside a Vue component. The central cause lies in how TypeScript interprets Vue.js 3’s Composition API.
Issue | Solution |
---|---|
The Vuex store is not correctly recognized as part of the Vue component’s public instance. | To make sure Vuex’s $store property is recognizable, declaring Vue module augmentation is essential. |
To illustrate, Api composition methods wouldn’t implicitly know about your Vuex store without additional configuration. Applying this configuration helps TypeScript recognize the `$store` property on the Vue’s `ComponentPublicInstance`.
import { ComponentCustomProperties } from 'vue' import { Store } from 'vuex' declare module '@vue/runtime-core' { // Declare your own store states here interface State { count: number } interface ComponentCustomProperties { $store: Store } }
In this example, we’re importing `ComponentCustomProperties` from Vue and `Store` from Vuex. We’ve declared a module augmentation for `@vue/runtime-core`, added a custom state – in this case, `count` – to the state interface, and highlighted `$store` as being of type `Store`. This process adds `$store` to all Vue components under Vuex context.
In coding, there’s an old saying by Martin Fowler that describes this situation aptly: “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Code should not only be functional but also maintainable, legible, and contextualized for everyone else on your team. Integrating TypeScript with Vue.js 3 enables developers to get closer to this ideal, once potential mismatches like the “$store” issue are resolved.
Decoding Error: Property $store Does Not Exist on Type ‘ComponentPublicInstance’
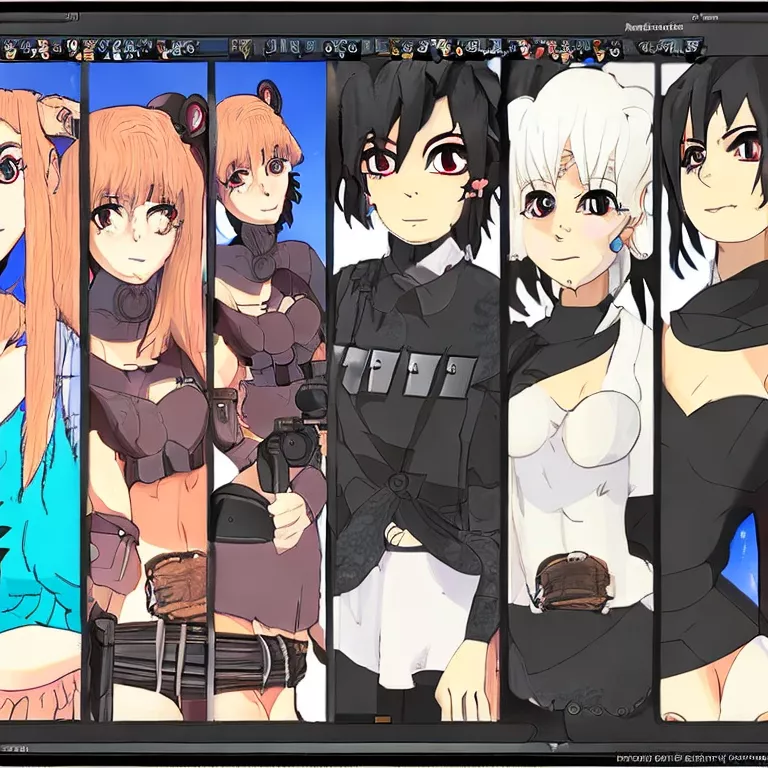
Facing the “Error: Property `$store` does not Exist on Type ‘ComponentPublicInstance'” usually arises when integrating Vuex with Vue.js 3 and Typescript. This typically happens due to type definitions not understanding Vuex and prompting TypeScript to throw a compilation error, hence the error you’re seeing.
Let’s indulge in a deep analysis of this issue and explore possible solutions:
Vue.js 3, Vuex and TypeScript: An Interplay
When working with Vue.js 3, Vuex (the state management library) and TypeScript, one might stumble upon challenges related to type definitions and accessing certain properties within the component.
Take the $store instance property injected by Vuex for example. Any Vue.js component has the capability to access the store instance via `this.$store`. However, default TypeScript typings provided by Vue.js 3 does not cover Vuex’s injected `$store` property which leads to the mentioned error.
Solving the Problem
To circumvent such issues, custom augmentation is required for module.
In Vue.js composition API you would inject the store like this:
<script lang='ts'> import { defineComponent, inject } from 'vue'; import { key, StoreType } from './store/store'; export default defineComponent({ name: 'MyComponent', setup() { const store = inject(key); if (!store) { throw new Error('store is not provided'); } console.log(store.state.count); return {}; } }) </script>
This allows TypeScript to be aware of what’s occurring, thus reducing the chances of running into the aforementioned error when binding your vuex store.
While building larger applications however, adding store interface in all components could become repetitive and cumbersome.
Hence to avoid code duplication, an alternate approach involves declaring global properties in your main.ts file:
import { createApp } from 'vue'; import App from './App.vue'; import store from './store'; const app = createApp(App); app.config.globalProperties.$store = store; app.mount('#app'); declare module '@vue/runtime-core' { interface ComponentCustomProperties { $store: typeof store; } }
Wrap-up with a technology quote for developers, as beautifully put by Steve Jobs: “Technology is nothing. What’s important is that you have a faith in people, that they’re basically good and smart, and if you give them tools, they’ll do wonderful things with them.”
One can refer to the official Vue.js 3 documentation for more on TypeScript support and Vuex.
Integrating Vuex Store with Vue.js Components using TypeScript
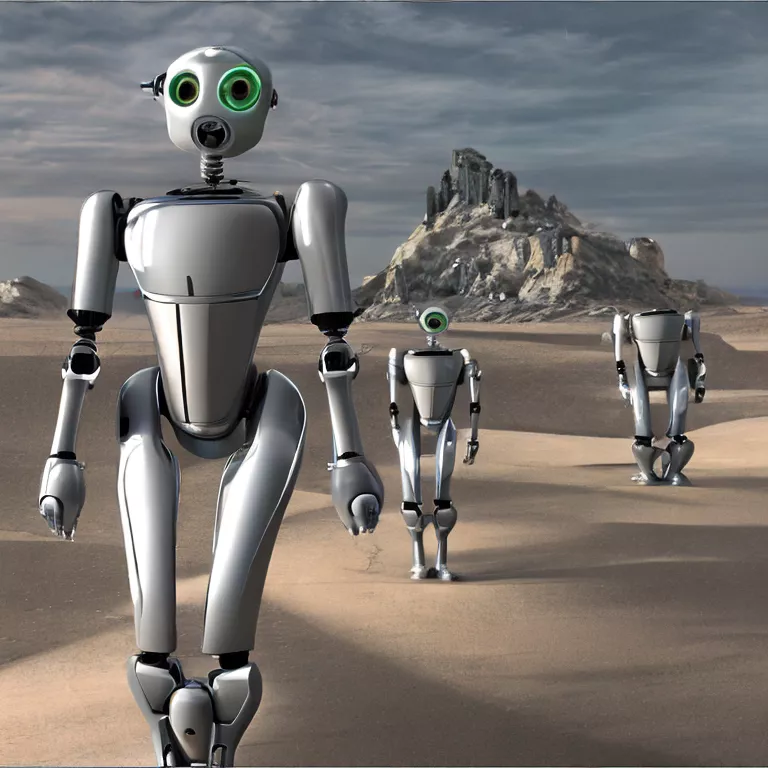
To integrate Vuex store with Vue.js components using TypeScript, it is important to ensure the application architecture aligns with Vue 3. This recent iteration of Vue.js significantly shifts its internal structure compared to its predecessors. A common error that can occur due to this change regards accessing the `$store` property within the `ComponentPublicInstance` type.
In Vue 3, both Options API and Composition API are used for component organization. However, issue of ‘$store’ not existing manifests differently in these two APIs.
Options API:
This message might appear when developing with TypeScript without declaring module augmentation.
Vuex offers a way to “type” your store by augmenting the Vue types:
html
declare module '@vue/runtime-core' { interface ComponentCustomProperties { $store: Store; } }
This tells Vue that `$store` is available on every instance of our component, and its type is a Vuex Store with state as defined in our main Vuex Store.
Composition API:
With the Composition API, getting a hold of your Vuex store instance is accomplished somewhat differently.
import { computed } from 'vue' import { useStore } from 'vuex' export default { setup() { const store = useStore() return { increaseCounter: () => store.commit('increaseCounter'), } } }
In the example above, we import `useStore` to get our Vuex Store instance. This instance will not be attached to the component directly, making it unavailable as `this.$store`.
As an illuminating note regarding coding practices, renowned developer Edsger Dijkstra remarked:
“Simplicity is prerequisite for reliability.”
Reminding us that while Vue.js 3 and TypeScript might introduce new complexities to our applications, it’s crucial that we maintain understanding and control over these tools. In the case of integrating Vuex Store with Vue components, this translates to properly configuring and accessing our store’s state.
Resolving Component Instance Issues in Vue.js 3 and TypeScript
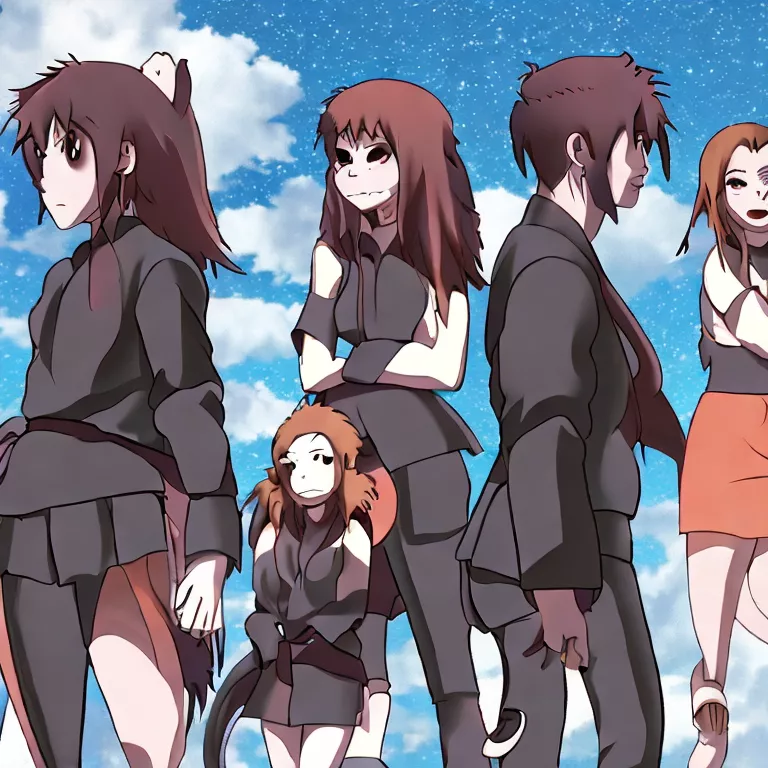
An error like “Property ‘$store’ does not exist on type ‘ComponentPublicInstance{}'” while using Vue.js 3 along with TypeScript typically indicates an instance where TypeScript is unable to find a particular property that you’re endeavoring to utilize. In this specific situation, TypeScript is having difficulty recognizing the `$store` property. This can occur due to an assortment of reasons as outlined:
-A potential concern arises if we are missing the necessary module augmentation that allows TypeScript to identify the additional properties we’ve added onto Vue.
-The Vue configuration and setup process may have been incorrectly implemented leading to this issue.
-Vue version compatibility posing problems when integrating with TypeScript could be another cause of this issue.
Resolving Component Instance Issues
Given below are indicative pointers towards potential solutions for remedying the problem encountered:
1. Vue-Configuration Settings: Ensure you’ve included the pertinent store (Vuex) in the creation instance. Vuex holds a central state management library for Vue.js applications. Configuring this properly will allow the $store property to resolve accurately.
import { createStore } from 'vuex' const store = createStore({ // options }) const app = createApp(App) app.use(store).mount('#app')
2. Declaration of Module Augmentation: Declare Vuex in a `.d.ts` file, enabling TypeScript to recognize the Vuex-related additions.
declare module '@vue/runtime-core' { import { Store } from 'vuex' interface ComponentCustomProperties { $store: Store } }
This enables TypeScript to understand that a `$store` property is present within Vue’s global properties, allowing it to link correctly.
3. Ensuring compatibility: Validate that all packages viz., Vue, Vuex and their related TypeScript definitions are upgraded and compatible.
When these steps are performed correctly, TypeScript should now be able to identify `$store` as part of Vue component instances without presenting errors.
As Kent Beck, a prominent computer programmer and the creator of extreme programming, eloquently phrased it – “I’m not a great programmer; I’m just a good programmer with great habits”. Hence, inculcating robust coding practices, embracing the habit of understanding error messages deeply, and developing an analytical approach towards problem-solving can unquestionably guide us towards being better programmers.
For additional understanding, one may refer to helpful online resources like Vue.js Documentation and Vuex Guide.
Conclusion
The issue of `Property $store does not exist on type ‘ComponentPublicInstance’` in Vue.js 3 when using Typescript appears to be a common stumbling block.
Most issues stem from the update to Vue.js 3, where it no longer automatically recognizes global properties such as `$store`. Instead, one has to manually declare these properties by extending the `ComponentPublicInstance` interface.
import { ComponentPublicInstance } from 'vue'; declare module '@vue/runtime-core' { interface ComponentPublicInstance { $store: any; } }
This enables Typechecking and Intellisense support for the `$store` property. However, using `any` as the store type may be too broad. For more specific types, consider defining your own custom Store Type.
interface MyStore { state: { count: number }; dispatch: (actionName: string, ...args: unknown[]) => void; commit: (mutationName: string, ...args: unknown[]) => void; } declare module '@vue/runtime-core' { interface ComponentPublicInstance { $store: MyStore; } }
It’s essential to understand that while updates often serve to improve functionality or offer new features, they might also introduce unforeseen errors, particularly when dealing with vast and diverse systems such as Vuex.
As Richard Branson concisely put it, “Every success story is a tale of constant adaption, revision, and change” – a principle quite applicable to the persistent paradigm of software development.
For those facing challenges with Vue.js and Typescript adaptation, remember the wisdom behind this statement. Update your knowledge and adapt your code accordingly; consider these necessities for thriving in a dynamic industry characterized by rapidly evolving technology.