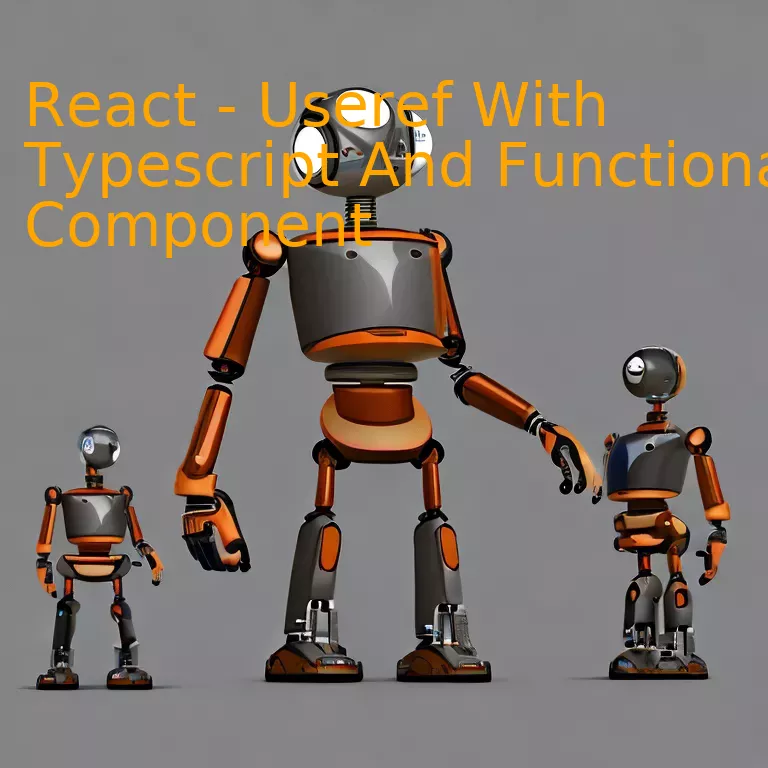
Introduction
When integrating Useref with Typescript in your Functional Component within React, it offers a streamlined technique for handling references, enhancing your project’s efficiency and code readability.
Quick Summary
React – UseRef with Typescript and Functional Component brings together three fundamental concepts to enhance your React applications: The React UseRef Hook, Typescript static typing and Functional components.
Concept | Description |
---|---|
React-UseRef Hook | The useRef Hook provides a way to access the properties of a component. This hook creates an object reference that can be used to directly interact with the DOM or keep mutable variables between re-renders. It doesn’t trigger updates when its content changes. |
TypeScript | TypeScript is a strongly typed superset of JavaScript which adds optional types to JavaScript code, ensuring type consistency throughout the codebase. The presence of these explicit types helps prevent bugs, making the code more robust and maintainable. |
Functional Components | Functional Components are simple JavaScript functions that accept props as an argument and return React elements. They are easier to understand and test than class components, and Work excellently with the expected predictability of React Hooks. |
To associate React’s useRef, TypeScript, and Functional Components, we can opt for a straightforward example. Imagine you’re creating an input field with useRef, inside a functional component, and you want to leverage TypeScript for better type-safety:
import React, { useRef } from 'react';
const FocusInput: React.FC = () => {
const inputRef = useRef(null); // Declares inputRef as null
const handleFocusClick = (): void => {
inputRef.current?.focus(); // Use optional chaining for safer access
};
return (
Focus the input
);
};
export default FocusInput;
In the code above, `useRef` is used to maintain a reference to the DOM node. TypeScript is enforced by annotating the `inputRef` with `HTMLInputElement | null`, which affirms that it will either be an HTMLInputElement or null. This code represents a functional component that includes a button. When clicked, the function `handleFocusClick` is activated, making the input field automatically gain focus.
As Buckminster Fuller once stated, “Humanity is acquiring all the right technology for all the wrong reasons.” Programming and developing applications are no exceptions. Harnessing the power of these foundational concepts: React’s useRef, TypeScript, and Functional Hooks via Functional Components can dramatically revamp your web application, fostering efficient management and exactness in code.
Understanding the Basics: Typescript in Functional Components
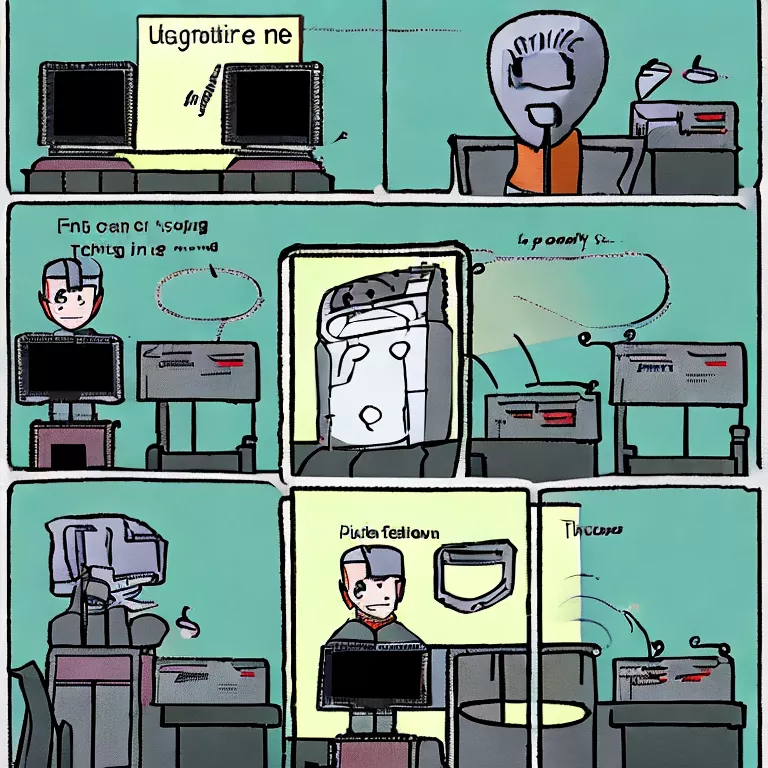
When working with TypeScript in functional components of React, it’s essential to understand the basic usage and benefits that it can offer. Through its robust typing system, TypeScript provides code scalability and maintainability that results in fewer bugs and more predictable outcomes.
In this context, let’s focus on the concept of useRef, a built-in hook in React, and examine its interaction with TypeScript within functional components.
xxxxxxxxxx
type MyComponentProps = {
message: string;
}
const MyComponent:React.FC = (props) => {
const myRef = useRef(null);
console.log(myRef.current); // This should log an HTMLDivElement or null
return (
{props.message}
)
}
Above is an example of a functional component in React using the useRef hook with TypeScript. Understanding how useRef behaves dynamically at run-time will help with their correct typings. Here, myRef is typed as
xxxxxxxxxx
React.RefObject<HTMLDivElement>
, indicating that it is a reference to an instance of HTMLDivElement.
The element assigned to the ref (in this case, a div) corresponds to this instance. Doing so not only provides autocomplete features when trying to access this ref but also makes sure that any attempt to assign a wrong ref type would result in a compile-time error.
Understanding TypeScript in functional components, particularly with the useRef hook, is empowering. It enables us to strongly type refs in cases like accessing DOM nodes directly or keeping track of mutable values across render cycles while still being reactive and reflective of changes.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler, British software developer.
The complexity of any application heavily relies on the ability to efficiently manage state and side-effects. One aspect of this in a React/TypeScript context is understanding the useRef hook. Applying TypeScript with React not only boosts the quality of codebase but enhances developer productivity as well via better tooling supports and aid in avoiding bugs at an early stage.
Harnessing the Power of Useref in React with Typescript
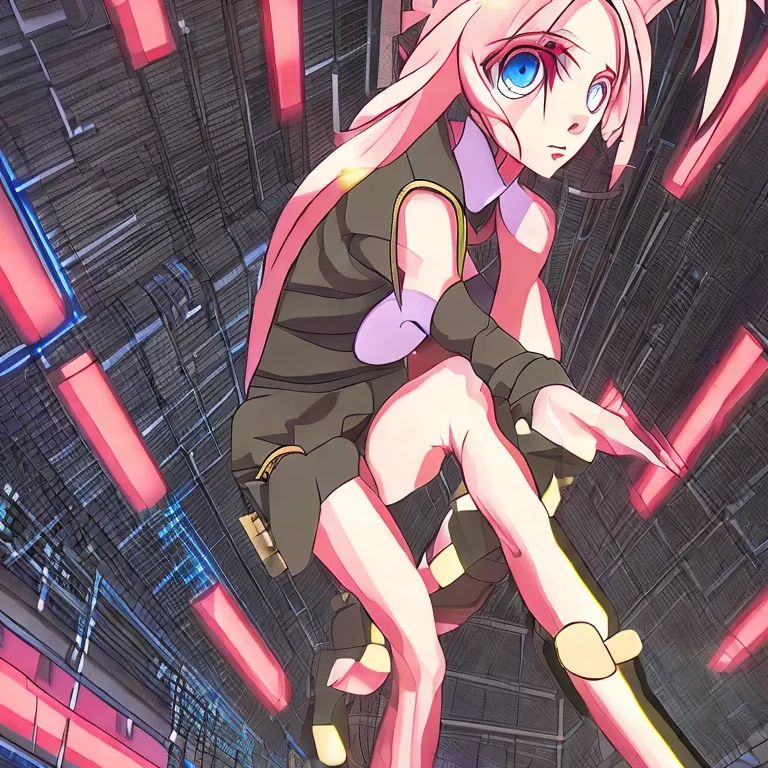
Understanding the power of useRef in React coupled with TypeScript can considerably enhance your application performance and workability. Let’s delve deeper into how this marriage between these two techniques can fortify your Functional Components in React.
The Magic of useRef
The useRef hook in React allows us to access the mutable values which persist for the full lifetime of the React component, without causing re-render upon changes. This unique feature offers an edge when it comes to maintaining ‘ref’ to DOM nodes or any mutable value across multiple renders.
Here’s a simplistic usage pattern involving useRef in a functional component:
xxxxxxxxxx
{
`import React, { useRef } from 'react';
const MyComponent: React.FC = () => {
const myRef = useRef(null);
// To reference:
console.log(myRef.current);
return (
Hello world
);
};`
}
Here, useRef creates a ref and attaches it to the div. The current property refers to the value currently held by the ref.
TypeScript Adding Precision to useRef
TypeScript further strengthens useRef by empowering it with type-checking abilities. Leveraging TypeScript with useRef provides detailed insight into the type of values our ref can hold, enhancing code reliability.
Consider the previous example revised with TypeScript:
xxxxxxxxxx
{
`import React, { useRef } from 'react';
const MyComponent: React.FC = () => {
const myRef = useRef(null);
// To reference:
console.log(myRef.current);
return (
Hello world
);
};`
}
In this instance, we specify that myRef can hold either null or a reference to an `HTMLDivElement`. By implicitly declaring the possible assignments, we’re creating a safety net against potential type errors. This mechanism promotes robust coding practices and accurate type prediction, indispensable traits in enterprise-level coding.
### Using useRef with event handlers
One primary use-case of useRef lies within event handlers in functional components:
xxxxxxxxxx
{
`import React, { useRef } from 'react';
const MyComponent: React.FC = () => {
const buttonRef = useRef(null);
const handleClick = () => {
buttonRef.current?.focus();
};
return (
<>
Focus me
);
};`
}
In this snippet, the button gains focus each time it’s clicked because of the reference held within `buttonRef.current`.
As programmers, combining TypeScript with useRef enables these critical dynamics, once summarized by Edsger W. Dijkstra – “Program testing can be used to show the presence of bugs, but never to show their absence!” It’s a tool that provides precise component knowledge, ensuring that bugs and typing mismatches lurking between rendering cycles are effectively eradicated.
Practical Examples: Implementing Useref and Typescript
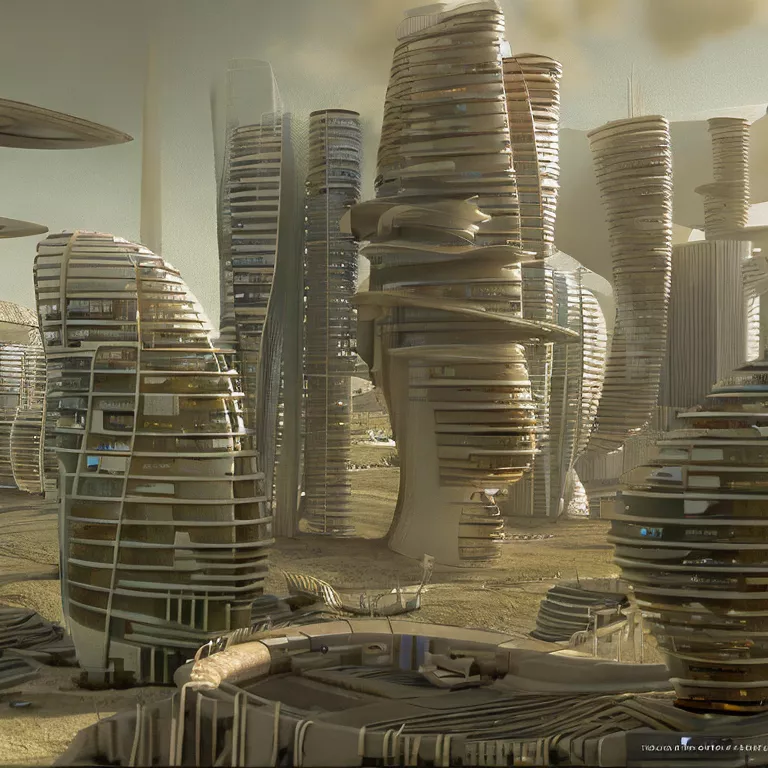
The `useRef` hook in React, when combined with TypeScript, offers a powerful way to manage mutable values and access DOM elements directly within functional components. It does this without re-rendering or causing side effects which can be quite advantageous.
Before we dive into practical examples, take note of how useRef and TypeScript interface:
<code>
// Defining the type for our ref
type MyRefType = {
current: null | HTMLDivElement
}
// Creating the useRef
const myRef = React.useRef<MyRefType>(null);
</code>
In this code, an interface `MyRefType` is defined which specifies that the `current` property can either have a value of `null` or reference a `HTMLDivElement`. By sandwiching `MyRefType` in between `< >`, we create a useRef that only accepts `null` or a `HTMLDivElement`.
To use `useRef` in a functional component alongside TypeScript, let’s consider a simple scenario where we are focusing on an input field once the component loads. Here’s a demonstration:
<code>
import React, { useEffect, useRef } from ‘react’;
type InputElement = null | HTMLInputElement;
const MyComponent: React.FC = () => {
const inputRef = useRef<InputElement>(null);
useEffect(() => {
if(inputRef.current) {
inputRef.current.focus();
}
}, []);
return (
);
};
export default MyComponent;
</code>
In the above example, `inputRef` holds a reference to the instance of the `input` field in the DOM once it renders. As soon as the component mounts, `useEffect` fires and shifts focus to the referenced `input` field.
The marriage of TypeScript and useRef beckons in-depth understanding of types. This ensures that we are assigning appropriate current values to our ref – a concept deeply appreciated in large-scale applications. The magic lying underneath is this; invoking rendering optimization and embracing well-established static typing simultaneously.
In the words of Kyle Simpson, author of “You Don’t Know JS”, he opines,
> “JavaScript’s loose and flexible structures give you more freedom to find creative solutions to coding problems… Pairing it with strict tools [like TypeScript] enhances its capabilities, opening doors to many architectural implementations.”
Thus, TypeScript’s combination with React hooks like `useRef` provides powerful alternatives for managing direct manipulations in functional components, vital for high-performing apps.
Useref within Functional Components: Best Practices
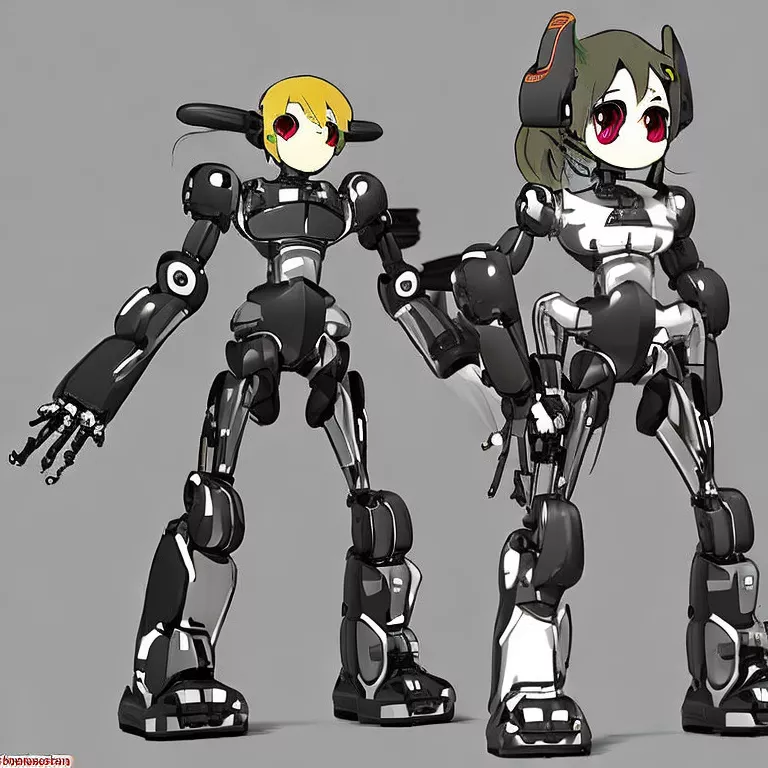
When dealing with React and TypeScript in functional components, `useRef` plays a crucial role. `useRef` is a built-in hook in React that allows you to access and interact with DOM nodes directly in your functions. It can also be used for storing mutable variables in the function component that do not cause re-renders when changed.
Best practices for using useRef within functional components are aplenty – from its use for storing references to DOM elements to its usage for storing mutable variables.
For TypeScript, it is ideal to define the type of the ref manually. The type should match the type of the HTML element the ref will be bound to. Here is an example snippet:
xxxxxxxxxx
const myRef = useRef(null);
In this excerpt, `myRef.current` is typed as `HTMLDivElement | null`. By default, the value of the `current` property will be `null`, but it will update whenever the ref is attached to a `div` element in the return statement.
As Ben Awad has stated in one of his insightful tech talks, “Having types for your application isn’t just about being able to catch bugs, it’s about developer velocity – How quickly can you write code and iterate on your applications.”
His perspective emphasizes the significance of proper typing in applications including those that use `useRef` in functional components.
Another practical approach is to use optional chaining (`?.`) to ensure safety while accessing properties or methods on `myRef.current`. This gewgaw helps keep your code cleaner and reduces the “cannot read property of ‘null’/undefined'” error occurrences.
For instance,
xxxxxxxxxx
console.log(myRef.current?.offsetHeight); // Safe
The TypeScript compiler won’t complain about potentially trying to reference `offsetHeight` of potential ‘null’.
To wrap it up, when employing `useRef` with TypeScript in React’s functional components:
– Explicitly define types for the `useRef` call
– Safe-proof potentially ‘null’ values with optional chaining while accessing properties or methods
Referencing Harvard School of Engineering’s article on Typescript and Type System would also be greatly beneficial in understanding the underlying intricacies associated with Typescript.
Sticking to these practices strengthens your Functional Component codes, helps contain potential snags, and improves the overall efficiency of your React applications.
Conclusion
Delving into the essence of React, useRef when used in combination with TypeScript and functional components contributes to a seamless and streamlined coding experience. Essentially, useRef adds another layer of functionality in React, enabling access to or storing of mutable values over multiple re-renders, without causing any additional renders.
xxxxxxxxxx
const refContainer = useRef(initialValue);
. When used within TypeScript, a strong typing system for JavaScript, it helps manage the complexity of codebase by introducing types like number, object, array, etc. Here, it’s vital to note that TypeScript comes packed with benefits including enhanced readability, predictability, and scalability of your code.
Furthermore, when useRef is paired with functional components in React, it enhances the efficiency and convenience of managing state and handling effects in a component. This amalgamation eliminates the complication of binding ‘this’ keyword in event handlers or lifecycle methods, thereby reducing chances of unexpected bugs. An example could look like this:
xxxxxxxxxx
import React, { useRef } from 'react';
function App() {
const inputEl = useRef(null);
const onButtonClick = () => {
inputEl.current.focus();
};
return (
<>
Focus the input
);
}
Quoting Dan Abramov, a prominent figure at Facebook working on React, “Writing reusable components isn’t a binary goal, but a spectrum.” Keeping this thought in mind, the integration of useRef with TypeScript and functional components does not just uplift code reuse possibilities but elevates your React projects to a new spectrum of robustness and maintainability too.
Harnessing these technologies, developers can engineer more responsive, user-friendly web applications and websites, ensuring deeper and more meaningful end-user engagements. Taking cues from React’s official documentation and the collaborative knowledge on StackOverflow, incorporating useRef in your code with TypeScript and functional components will be a technologically sound decision.
Undoubtedly, the significant advancements in JavaScript frameworks have immensely simplified complex tasks for developers. The integration of React’s useRef with Typescript and functional components is undoubtedly one such powerful leap towards more efficient and effective web development. Keep exploring, keep coding!