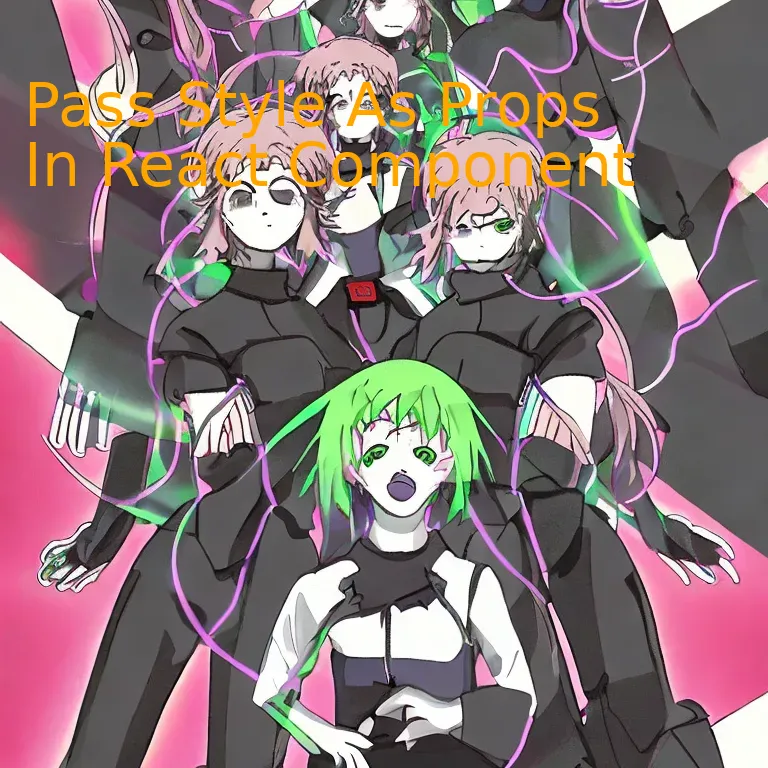
Introduction
In developing user interfaces with React, leveraging the functionality of passing style as props in your React component offers a dynamic approach to manage and customize your design elements effectively.
Quick Summary
Passing styles as properties (props) in a React component is a common technique used in modern UI webpage development.
Below, we take a look at how we would achieve this practically:
Property | Description |
---|---|
Style Object | A JavaScript object that contains CSS key-value pairs. This could be contained within the component or passed as props from a parent component. |
Component | This is where the style object is applied. The style attribute accepts an object with camelCased properties rather than kebab-case. |
Props | The vehicle through which our Style Object is passed into our Component. Props provide a way for us to pass data from parent to child components. |
Let’s delve deeper into each of these concepts.
A Style Object is simply a collection of CSS styles represented as a JavaScript object. It can either be declared within the component itself or passed as props. The keys of this object are the CSS properties you want to set and the values are the respective values for these properties. However, unlike standard CSS syntax, property names are written in camelCase as opposed to hyphen-separated notation. Here’s an example of what a style object might look like:
{
backgroundColor: 'blue',
color: 'white',
padding: '10px'
}
Next, we have our Component. In React, a component is a self-contained section of the user interface (UI). Components can either be JavaScript functions or classes. The style object can then be applied to this component using the ‘style’ attribute.
Finally, Props in React are akin to function arguments. They allow parent components to pass properties down to their child components. By passing our Style Object as a prop, we ensure the flexibility and reusability of our component.
In the words of Jordan Walke, the creator of ReactJS, “Components are the crux of everything React.” This sense of composability, especially with styles, is what makes React so widely adopted and powerful. It’s an indispensable tool in our toolkit for creating dynamic and responsive UIs. The implementation of components carrying their own styles provides a structured and increasing maintainability of your project, streamlining the development process and making it easier to track potential issues or changes.
Leveraging Style Props for Component Customization in React
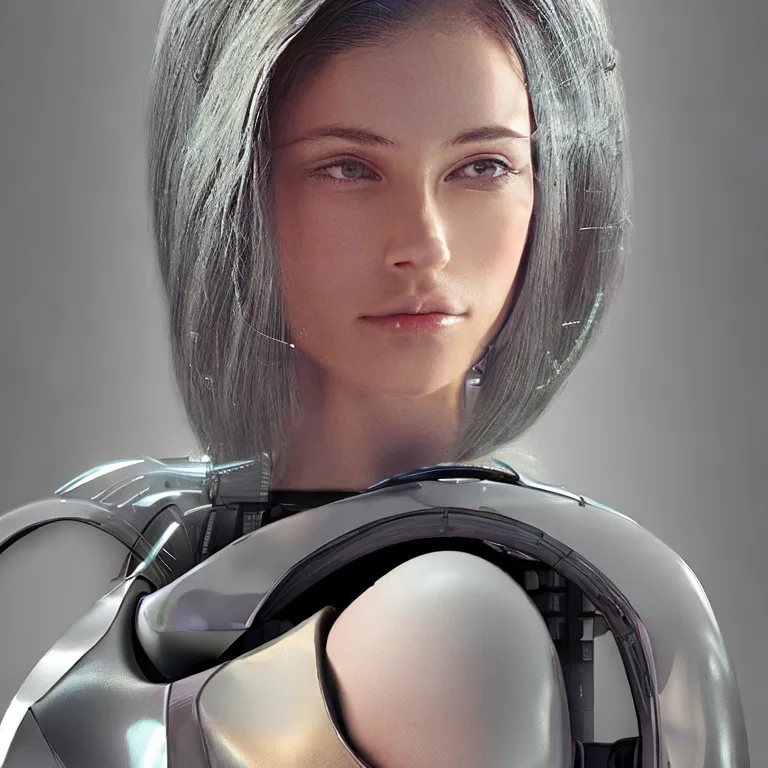
The technique of passing style as props in a React component offers an engaging and dynamic way to customize components in your React application. Rather than statically defining the style for each component within that component itself, you can pass style as props to modify its design dynamically.
React, apart from encapsulating functionality, allows the manipulation of visual content by injecting different pre-defined styles that have been outfitted to match the various needs or conditional settings of the components.
To illustrate, let’s imagine we are building a custom “Button” component:
html
function Button(props) {
return (
);
}
let customStyle = {
color: ‘blue’,
fontSize: ’20px’
};
function App() {
return (
);
}
ReactDOM.render(
In this case, the custom style properties ‘color’ and ‘fontSize’ are being passed as props to the Button component. This flexibility paves the way for creating truly reusable components that maintain a consistent structure while also permitting variability in appearance.
There is a cautionary detail to consider when using style props, and that would be the potential overhead of creating many inline styles on the fly. Despite this caution, this method provides significant benefits including easier style manipulation, better performance over external CSS files, and tunable aesthetics of components via prop changes.
Furthermore, Douglas Crockford might agree with such approach, as he once said, “Good design adds value faster than it adds cost,
” and leveraging style props certainly bolsters the efficiency and versatility of design within your React components.[source]
However, be advised that using inline styles in React comes with some downsides like the absence of media queries and other CSS features. Therefore, to address complex styles a CSS-in-JS solution like Styled Components or Emotion might be considered.
Finally, it is noteworthy to mention that style props are powerful tools in your React toolkit but should be used judiciously and appropriately. Balancing project requirements, performance implications, and code maintainability can guide you in deciding when leveraging style props would be advantageous.
Deep Dive: Passing Styles as Props in React Components
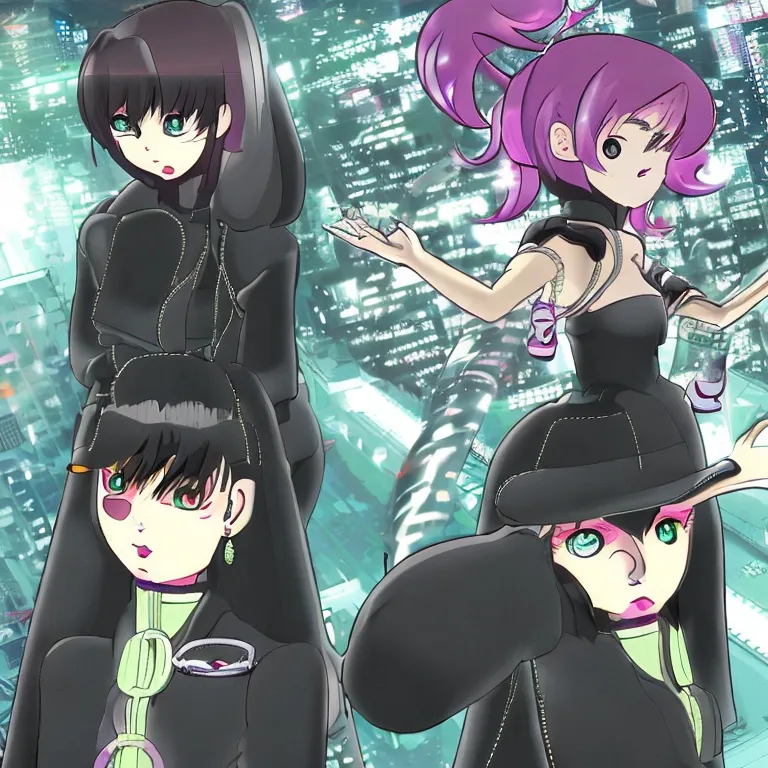
When developing with ReactJs, it is common to pass data using props. Passing styles as props not only aligns well with this principle but also opens up avenues for flexible and dynamic styling. This allows us to alter the appearance of a component based on certain conditions or user interactions.
With respect to passing styles as props, focusing on a few significant points:
Understanding In-line Styles:
React does not use traditional “CSS” styling; instead, it leans towards in-line styling where style sheets are not created separately but are included in the JS code internally. Each HTML element can accommodate a “style” prop that accepts JavaScript objects.
For instance:
xxxxxxxxxx
<div style={{color: 'blue', backgroundColor: 'white'}}></div>
The JavaScript object contains pairs of properties (camelCased CSS property) and values, which translates into actual styles for the given element.
Creating Style Objects:
For readability and reusability, one can define a style object outside the component and then reference these properties within the components using the style attribute.
For example:
xxxxxxxxxx
const divStyle = {
color: 'blue',
backgroundColor: 'white'
};
function Component() {
return (
<div style={divStyle}></div>
);
}
Passing Styles as Props:
Moving forward from creating an inline or separate style object, we can also pass these style objects as props to child components.
Consider the following example:
xxxxxxxxxx
function ChildComponent(props) {
return (
<div style={props.styles}></div>
);
}
function ParentComponent() {
const parentStyles = {
color: 'red',
backgroundColor: 'black'
};
return (
<ChildComponent styles={parentStyles} />
);
}
In the example above, the ParentComponent is passing down the parentStyles object as props to the ChildComponent. In this way, we can create reusable components with customizable stylings. This fosters greater code maintainability and readability.
As Addy Osmani, a Google engineer once noted: “Code readability is immensely important – developers read far more code than they ever write.”
Swift changes in component styles based on state or props can provide a stellar user experience on a web platform. The use of styles as props ingeniously delivers this dynamic capability within React components, proving beneficial for single-page applications where runtime conditions might require such versatility.
The Potential of Dynamic Styling with Prop-Based Design
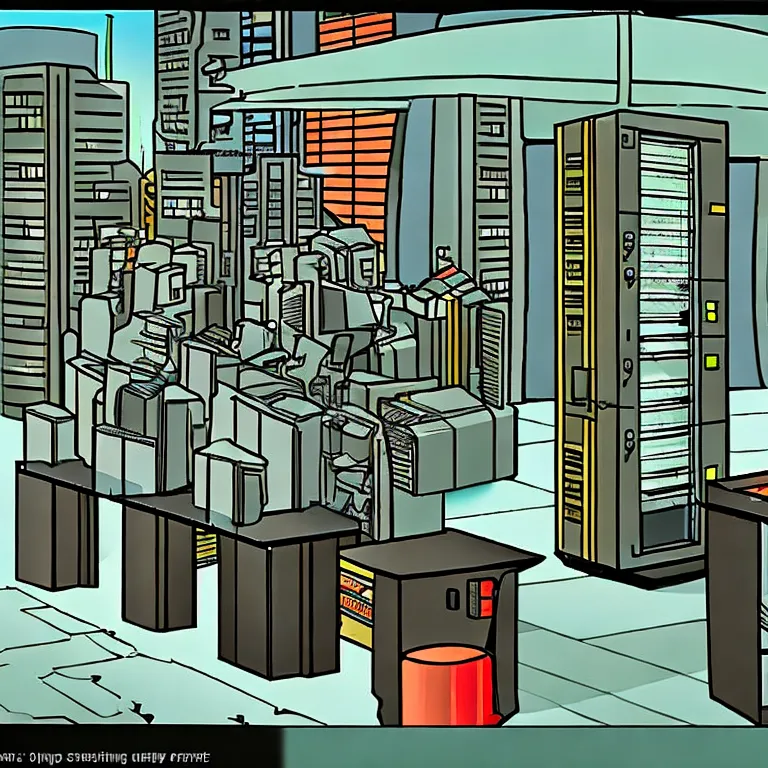
Dynamic styling in a Prop-Based Design provides the potential for bringing full customization capabilities to React components. Essentially, it allows you to pass styles directly as props to your React components, facilitating smoother and more efficient application design.
The key concept of Prop-Based Design works on inline styling. This means styles are applied on-the-fly, so they can change dynamically based on certain conditions. Here’s a snippet illustrating dynamic styling with prop-based design:
xxxxxxxxxx
const MyComponent = (props) => (
<div style={{ background: props.bgColor, color: props.textColor }}>
{props.children}
</div>
);
In this example, `MyComponent` accepts two prop values ‘bgColor’ and ‘textColor’. They get used directly inside the `style` attribute of our div element. This ensures the div adapts its background and text colors according to the prop values passed during component rendering.
Prop-based design offers significant advantages such as:
– Fine-grained control over styling.
– Facilitation of conditional styling.
– Reduction of CSS dependencies as the styling can be controlled from JavaScript.
It’s essential to understand that controlling styles via JavaScript is highly potent. It encapsulates style control within the same domain language, making development more consistent and less context-switching intensive.
Additionally, Prop-Based Design has strong ties with JavaScript’s inherent dynamism. As a multi-paradigm language, JavaScript enables us to handle prop-passing in various ways, including hardcoded strings, variables, functions, or object constructs, further enhancing the flexibility and expressiveness of our styles.
“Any application that can be written in JavaScript, will eventually be written in JavaScript,” predicted Jeff Atwood—Stack Overflow co-founder. His words resonate powerfully here. Dynamic styling with Prop-Based Design showcases the potential of leveraging JavaScript to refine the aesthetic and functional aspect of web applications significantly.
As always, keep in mind that with great power also comes great responsibility. Effective usage of dynamic styles necessitates conscious and judicious practices to avoid unnecessary complexities or undesirable side effects.
xxxxxxxxxx
<MyComponent bgColor="blue" textColor="white">Hello World!</MyComponent>
Again, don’t forget that there are [best practices](https://www.freecodecamp.org/news/best-practices-for-inline-styling-in-prop-based-design/) when implementing this type of design to ensure maintainability, readability, and performance optimization in your React applications.
Understanding the Intersection of Style and Properties within Reactive Frameworks
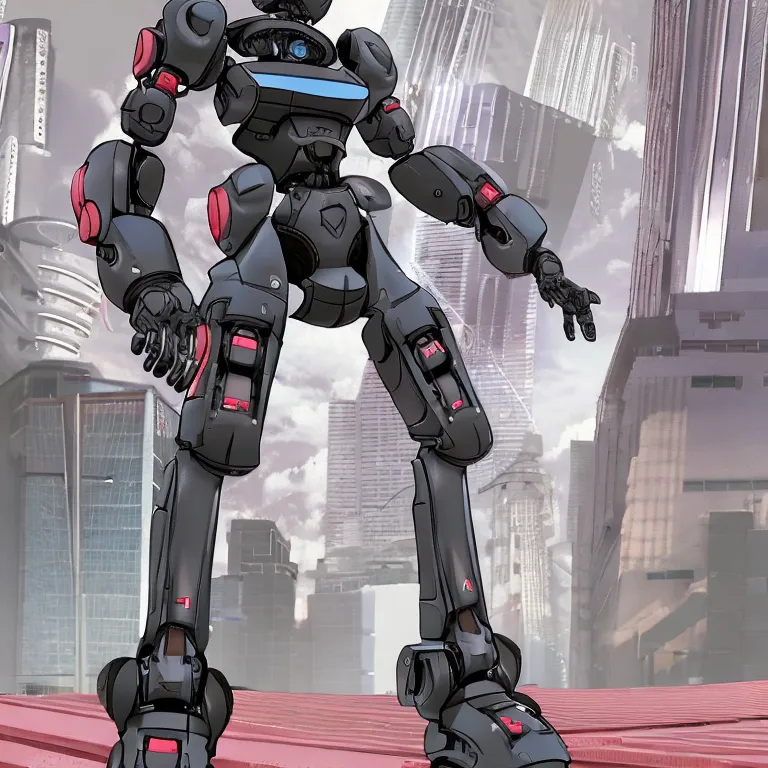
Reactive development frameworks such as React have significantly enhanced web development. Creating and reusing components has now become a more straightforward process. One distinctive feature available is passing style as props in a react component – an intriguing intersection of style and properties within reactive frameworks, particularly React.
When developing with React, the modularity of components encourages code-reuse and promotes cleaner coding practices. In principle, components are self-contained units that manage their own state and rendering. To increase the utility of such components, we occasionally require them to have certain visual adaptations based on where they are being employed. This necessity gives rise to the concept of passing styles as properties or ‘props’, thereby offering dynamic style adjustments.
This system allows react components to be flexible regarding their appearance and behavior. Therefore, if a button in the user interface is used in different places and each needs a unique style, instead of defining styles multiple times, one can define a custom style once outside the button component and pass it as a property.
For example, suppose you create a custom MyButton component and wish to vary its color based on where it appears:
xxxxxxxxxx
HTML
function MyButton({ text, bgColor }) {
return (
);
}
// Using MyButton elsewhere in your app
Here, color “lightblue” is passed as a property to the ‘MyButton’ component.
This method of styling is powerful in several ways:
* It keeps your components decoupled and re-usable.
* Style changes can be managed at a high level, rather than individually altering each instance of the component.
* The same component can adapt to varying contexts, offering flexibility.
It’s important to note that this technique might not be suitable in all cases. Especially in scenarios where there could be an explosion in the number of properties needed to manage all required styles. In such situations, it may be optimal to create dedicated styled-components or use CSS-in-JS libraries that grant robust styling capabilities much closely aligned with traditional CSS.
Renowned computer scientist Alan Kay once said: “Simple things should be simple, complex things should be possible.” This philosophy is clearly echoed in React’s flexible approach to managing styles as props. Consequently, this enhances the developers’ experience and allows for more stylistically diverse and dynamic applications without drifting away from simplicity.
Conclusion
In React, passing style as props is a powerful way to dynamically change the look and feel of your components. This enables greater customization and flexibility as the appearance of a component isn’t hard-coded but instead influenced by its state, data or any other context it receives.
The basic syntax of passing a style object as a prop looks like this:
xxxxxxxxxx
<Component style={{ color: 'blue' }} />
Where ‘Component’ is your custom React component, and you’re essentially passing a JavaScript object { color: ‘blue’ } inside the ‘style’ prop.
Let’s break down why this technique is advantageous in responsive UI design:
– Reusability: One of the core philosophies in React is reusability of components. With styles being passed as props, we’re able to reuse the same component with different appearances in different contexts.
– Dynamic Styling: Based on certain conditions, such as user input or data fetched from an API, the style of a component can be modified. This adds to the interactivity of your application.
– Maintainability: The separation of concerns principle states that each part of a program should have responsibility over one functionality. By defining styles externally and then passing it as props, we are separating the styling concern from the component’s functional code.
As described by Ryan Florence, a renowned figure in React community: “In the pursuit of reusable components, the fist step is making them configurable”.
For reference or if further understanding is required on how to pass style as props in a React Component, there’s plenty of resources online. Resources like React Docs: Components and Props that explain the concepts further and can help anyone struggling to fully comprehend this subject matter.
Moving forward with your React learning journey, always remember that style passed as props is a potent technique to enhance your components’ reusability, readability and maintainability.