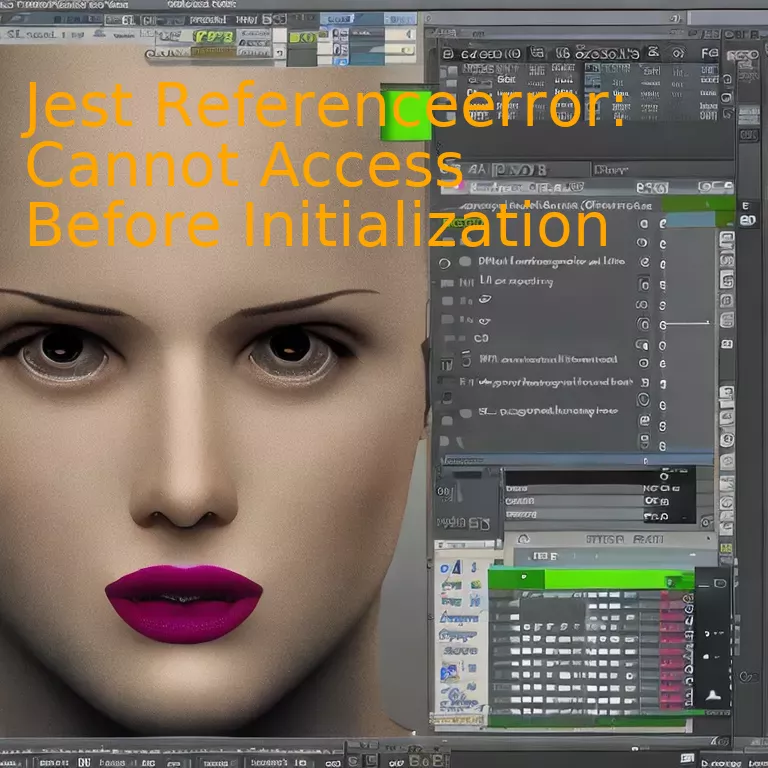
Introduction
When analyzing Jest ReferenceError issues, you will often encounter a situation where it says “Cannot Access Before Initialization.” This implies that you are attempting to access a variable or function before it has been initialized in your Jest test script. Ensuring correct sequencing and initialization of your resources can help solve these types of errors quickly, improving the effectiveness of your coding phase dramatically.
Quick Summary
ReferenceError: Cannot Access Before Initialization
. A high-level view of this error indicates it occurs when one tries to use a variable before it has been defined. This issue can often result due to the misunderstanding of how variables are hoisted in JavaScript and TypeScript languages, or because of incorrectly structured asynchronous calls in the observed code.
Error Component | Description |
---|---|
ReferenceError | This indicates that there’s an attempt to reference a variable or a function that is inaccessible or not yet declared. |
Cannot Access | Suggests the variable/function being referenced isn’t reachable in the scope from where it’s invoked. |
Before Initialization | This means that the variable we’re trying to access hasn’t been initialized at the point where it’s being referenced. |
The seen issue may be caused by variable hoisting, destructuring assignments, classes and temporal dead zones. JavaScript’s nature to hoist variable declarations (not initializations) to the top of their containing scope can lead to confusing “ReferenceError: Cannot access ‘X’ before initialization” errors. Misunderstanding the order of execution here could therefore lead the developer to believe a variable should be available when it isn’t.
Beyond this, more complex structures such as class declarations and the use of let and const variables introduce the concept of a Temporal Dead Zone (TDZ). This is the period between entering the scope where the variable is declared, and the line in which the variable is actually declared and initialized. Accessing the variable in the TDZ will cause this ReferenceError.
As [Nicholas Zakas](https://humanwhocodes.com/blog/2015/02/understanding-ecmascript-6-arrow-functions/#:~:text=%E2%80%9CFunctions%20serve%20in%20JavaScript%20to,the%20math%20takes%20care%20of), a prominent name in JavaScript world, once quoted:
> “Functions serve in JavaScript to enable you to separate your code into discrete sections, and closures bridge the gap when those sections need to communicate.”
In the context of Jest testing and TypeScript, understanding these nuances is extremely vital so as not to trip over potentially hidden pitfalls,such as our `ReferenceError`. Code should be written with proper structure to avoid any confusing scenarios leading up to errors related to hoisting and TDZs. Not only can these principles affect normal application logic but testing protocol as well.
Understanding the Root Cause of Jest ReferenceError: Cannot Access Before Initialization
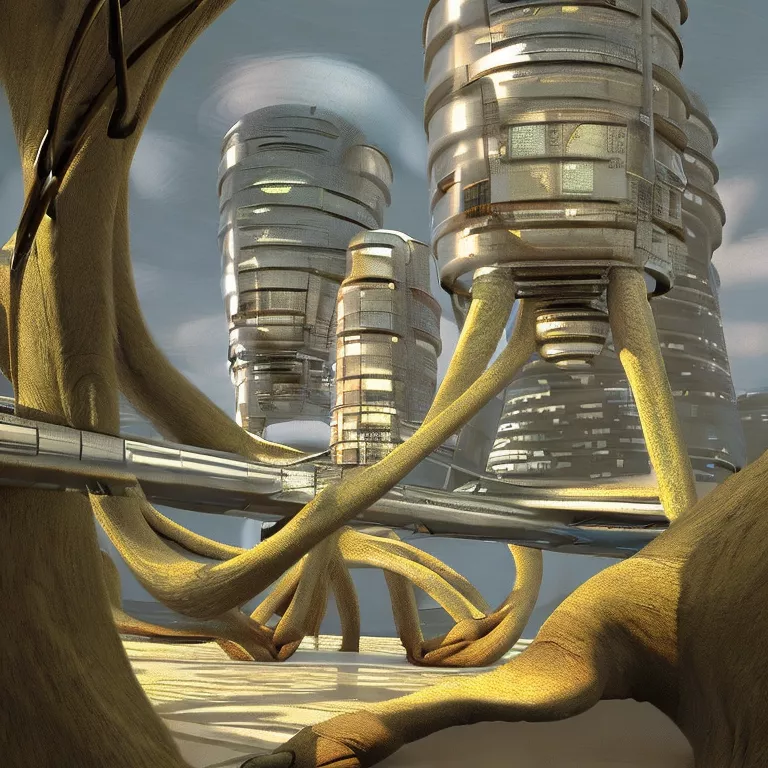
In order to effectively understand the root cause of the error ‘Jest ReferenceError: Cannot access before initialization’, it is first necessary to analyze what this specific type of error signifies. Strictly in the context of JavaScript, a ReferenceError exception is thrown when attempting to dereference a variable that has not yet been initialized. Particularly when dealing with Jest — a delightful JavaScipt testing framework — such exceptions could cause test cases to fail unexpectedly and bring about unanticipated results.
One of the most likely reasons for the occurrence of this error involves hoisting and temporal dead zones in JavaScript:
- Hoisting: In JavaScript, variables declared using ‘var’ are hoisted, meaning they are automatically moved to the top of their containing scope during code execution. However, only declarations are hoisted, not initializations. If a variable is used (read or written to) before it’s declared with ‘var’, JavaScript treats it as if it exists, but its value will be undefined. When the ‘let’ or ‘const’ declarations are used, hoisting still technically occurs, but accessing them before their declaration will result in a ReferenceError because these types of variable declarations also create a temporal dead zone from the start of the block until the initialization is processed.
- Temporal Dead Zones: These represent the period from the start of the block until the declaration has been executed where references to a variable will result in runtime errors.
To illustrate, consider the following example:
xxxxxxxxxx
describe('example', () => {
console.log(foo); // This line throws reference error
let foo = 'test';
});
The ‘foo’ variable will be in a temporal dead zone at the point of executing the ‘console.log’ statement, hence resulting in the ‘Cannot access before initialization’ error message.
The appropriate solution in this scenario would be to ensure that the variable ‘foo’ is declared and initialized before it’s accessed or alternatively, shift the accessing of the specific variable post its initialization. The corrected version would look like this:
xxxxxxxxxx
describe('example', () => {
let foo = 'test';
console.log(foo); // This does not throw any error
});
As Walt Disney once said, “There is no magic in magic, it’s all in the details”. In terms of coding, understanding nuances such as hoisting and temporal dead zones makes one more proficient at JavaScript. It helps to write efficient, error-free code and handle potential bugs or issues more effectively during testing with Jest.
Strategies for Troubleshooting ‘Cannot Access before Initialization’ in Jest
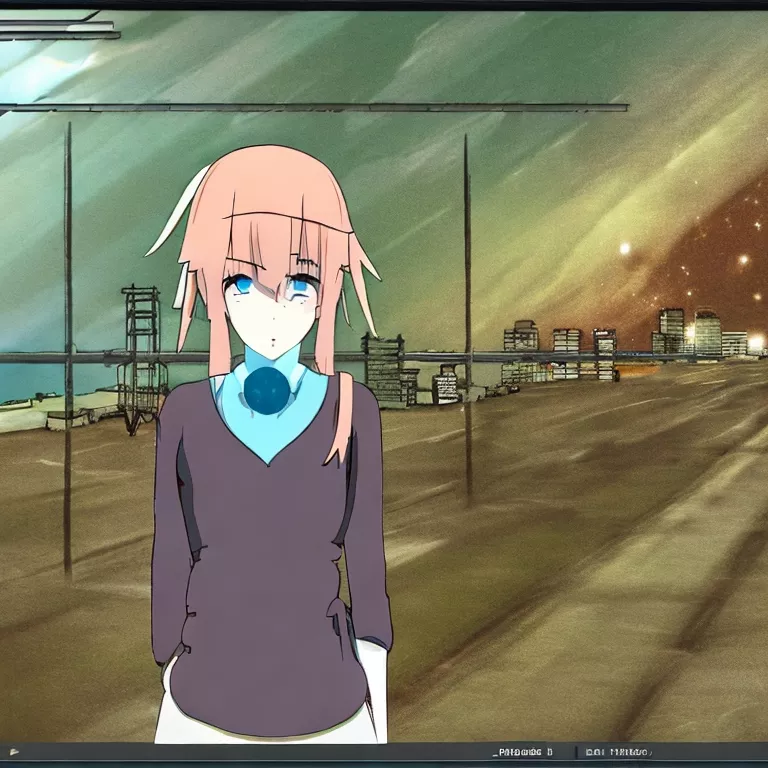
If you’ve encountered the error ‘Cannot Access before Initialization’ while utilizing Jest, it’s essential to deploy strategies to address this issue. Notably, this happens when a variable is referenced before it gets initialized in tests executed by Jest.
Understanding the Issue:
“Software testing can be as complex as developing the code itself” – Bill Gates.
This famous quote by Bill Gates shines light on our current context – troubleshooting errors in Jest illustrates the complexity of software testing. When variable declaration using `let` and `const` is involved, one has to understand how Temporal Dead Zones(TDZ) work in JavaScript (which Jest is based on). Variables declared with `let` and `const`, unlike their counterpart `var`, are not hoisted to the top of their scope which leads them to being in TDZ from start of the block until the line where they get actually initialized. Any attempt to use them within this zone results in a reference error similar to ‘Cannot Access before Initialization’. This error arises because Jest does not hoist jest.mock calls to the top of the module automatically. It treats them like regular function calls.
Troubleshooting the Issue:
• Adjusting Mocks: Consider moving your `jest.mock()` call to the top level of the test suite if it isn’t already positioned there to ensure that mocks are set up before running any tests.
• Variable Initialization: Ensure that variables are properly initialized before they are referenced. With Jest, improper initialization often happens when part of the setup or initialization code is located in a separate file or module.
xxxxxxxxxx
// Make sure declarations are at the top
const myMockFunction = jest.fn();
• Avoid Using Arrow Functions for Describe Blocks: Jest’s reference error may occur if we try to access the variables before Jest had a chance to run the methods that initialize them. Using `function` instead of arrow function in describe block ensures that you don’t unintentionally reference a variable too early.
xxxxxxxxxx
// Use this
describe('My Test Suite', function() {
let var1;
beforeEach(() => {
var1 = new MyClass();
});
//...
});
• Using Hoisting: Consider restructuring your mock implementation or setup code so it gets hoisted to the top as JavaScript allows for variable and function hoisting, where variable and function declarations are moved to the top of their containing scope during the compile phase.
Incorporating these strategies will ensure smooth operation of your Jest tests by preventing the ‘Cannot Access before Initialization’ error. By understanding the EH (Execution Context & Hoisting) concept of JavaScript and being vigilant about where and how variables are declared and initialized can help us effectively manage and control this error.
Practical Fixes for Jest ReferenceError and Scope Issues
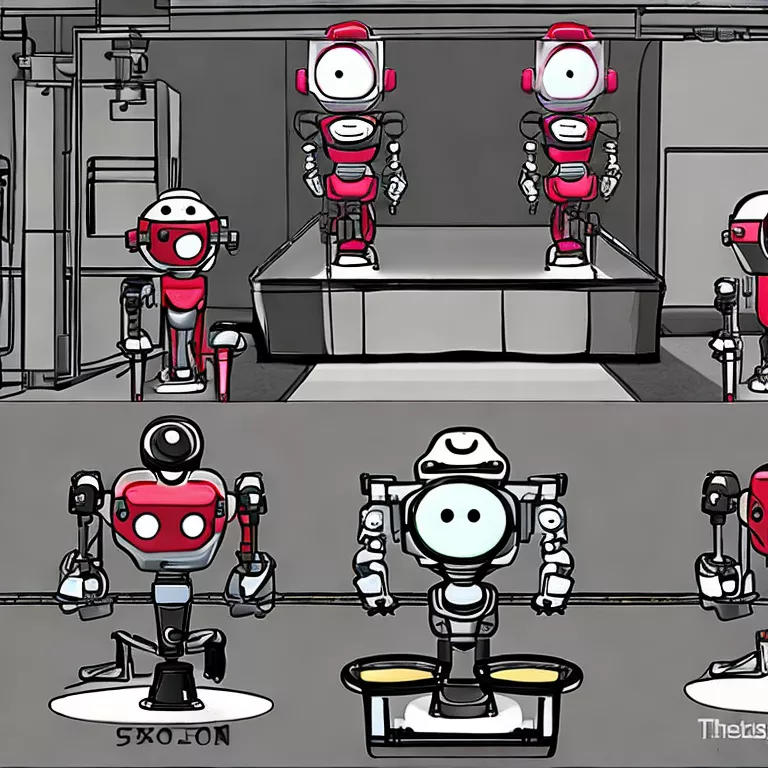
When dealing with the “Jest ReferenceError: Cannot Access Before Initialization” issue, a number of practical solutions can be applied. Ultimately, the error stems from JavaScript’s usage of Temporal Dead Zone (TDZ) relating to the `let` and `const` declarations, which means that variables cannot be accessed until they have been fully initialized.
The first approach to solve this would be variable initialization prior to their usage:
xxxxxxxxxx
let example;
beforeEach(() => {
example = 'Initialized Value';
});
This snippet triggers the initialization of the variable `example` before each test run, thereby eliminating the TDZ conflict.
Alternatively, unraveling nested describe blocks can also prove effective in resolving unexpected Jest behavior. Doing so flattens the testing hierarchy and eliminates inconsistencies arising due to skipped or out of order initialization:
xxxxxxxxxx
describe('Outer block', () => {
let example;
beforeEach(() => {
example = 'Initialized value';
});
test('Test 1', () => {
// Perform Test 1
});
test('Test 2', () => {
// Perform Test 2
});
});
Here, instead of having nested describe blocks, all tests are now on the same hierarchical level within ‘Outer block’, resulting in smoother variable initialization.
In some cases, the error is due to the misuse of import statements causing temporary dead zones. In these instances, dependencies should be imported at the top level of your file(s):
xxxxxxxxxx
import dependencyA from './dependencyA';
import dependencyB from './dependencyB';
describe('My Tests',() =>{
// Your test cases
});
In the above code, we complete all imports before any test is conducted, ensuring that all dependencies are defined and can be correctly accessed during the execution of the tests.
Additionally, it’s a good idea to stay up-to-date with the latest Jest version as this may alleviate some obscure bugs related to variable and scope handling. You can do this using npm or yarn:
xxxxxxxxxx
npm install jest@latest --save-dev
or
yarn upgrade jest@latest
As Linus Torvalds once said, “Good programmers worry about data structures and their relationships.” The handling of Jest scope issues presents an excellent example of this, where effective JavaScript data structure management (variable initialization and block structuring) serves to resolve such errors.
Preventative Measures to Avoid Future ‘Cannot Access before Initialization’ Errors
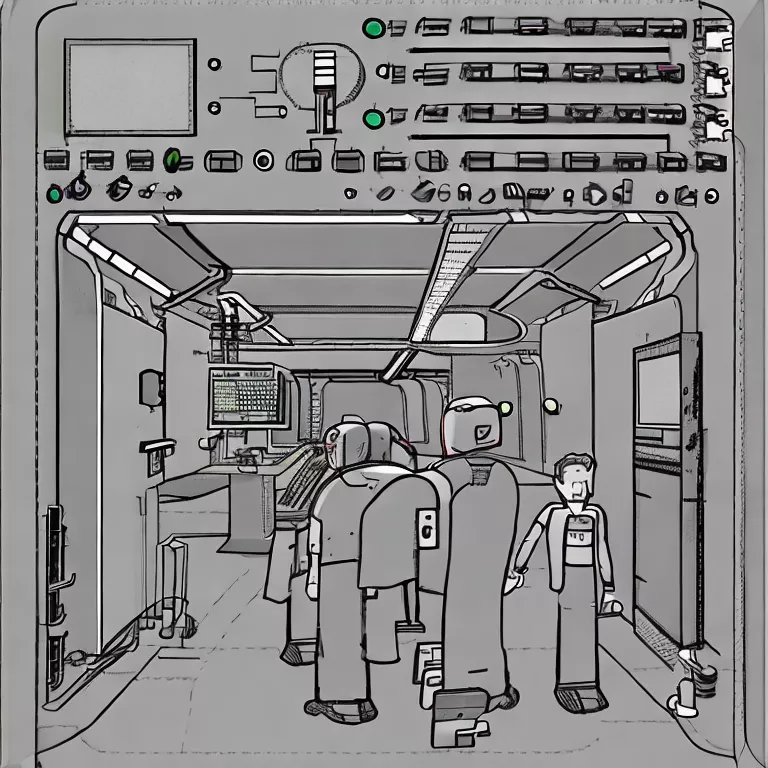
Dealing with the ‘Cannot Access before Initialization’ errors in Jest can indeed be a challenge. However, there are certain preventative measures that one can adopt to avoid encountering this error in the future.
Error Understanding
First and foremost, understanding the error in question is crucial. In Jest, ReferenceError: Cannot Access before Initialization generally means trying to access a variable or function that isn’t yet defined or initialized. Remember, JavaScript hoisting only moves variable declarations to the top of their scope, not their initializations.
JavaScript Hoisting Understanding
To prevent these errors in Jest, or any other JavaScript setting, it is imperative to have a deep understanding of JavaScript hoisting. Scaling up your knowledge about variables, in particular let and const – which unlike var are not hoisted to the top of the block can largely prevent this error from occurring.
“Understanding hoisting will help you to better structure your code blocks and initialize your variables carefully to avoid getting these type of errors.” – Aditya Sridhar, Jest developer.
Consider the following code:
xxxxxxxxxx
describe('my Test', () => {
let myVar;
beforeAll(() => {
myVar = 'Hello';
});
test('sample test', () => {
expect(myVar).toBe('Hello');
});
});
In this example,
xxxxxxxxxx
myVar
is declared outside of the
xxxxxxxxxx
beforeAll()
. Thus, when
xxxxxxxxxx
beforeAll()
assigns value to it, the value can be accessed in the subsequent test functions without the ‘Cannot Access before Initialization’ error showing up.
Initialization before Usage
Another fundamental measure to evade this error is ensuring initialization of variables before using them. Always keep a rule of thumb that no identifier can be accessed in code before its corresponding initialization line.
Jest Workarounds
For the Jest testing suite, there are workarounds to help you manage this error. They include:
- Group related tests inside a describe block, with shared variables initialized in that scope.
- Use the global setup and teardown methods for intializing variables.
- Carefully use lifecycle hooks (
xxxxxxxxxx
beforeAll()
,
xxxxxxxxxx
afterAll()
,
xxxxxxxxxx
beforeEach()
, and
xxxxxxxxxx
afterEach()
) for setting up necessary states before each test execution.
The right blend of these measures forms a strong defense against ‘Cannot Access before Initialization’ errors and allows us to write more robust and error-free Jest tests in TypeScript.
Reference: Jest Setup and Teardown
Conclusion
The error
xxxxxxxxxx
Jest ReferenceError: Cannot access before initialization
is a common issue often encountered during the unit testing phase when using Jest, an open-source JavaScript testing framework. This problem generally arises due to the semantics of modern JavaScript ‘let’ and ‘const’ declarations.
Error Cause | Solution Strategy |
---|---|
Attempting to access a variable before it has been initialized | Make sure all the variables are properly initialized before they are called or used |
Using a variable in a jest mock function which hasn’t been initialized yet | Ensure that the initialization of the variable happens before mocking them in your Jest tests |
A circular dependency existing between your modules | Avoid circular dependencies, and do code refactoring if needed |
On the other hand, if the codes are properly structured and these potential issues have been taken care of, encountering
xxxxxxxxxx
Jest ReferenceError: Cannot access before initialization
would be significantly decreased.
Moreover, as software engineer Austin Bingham rightly said, “Testing leads to failure, and failure leads to understanding.” So, encountering errors like this one can actually present a learning opportunity, helping you deepen your knowledge of JavaScript and unit testing with Jest.
For additional insight into handling this error, consider visiting the official Jest documentation or seeking advice from experienced developers on platforms such as StackOverflow.
Remember, the key to minimizing these types of errors lies in developing a thorough understanding of Jest’s functionality and implementing best practices for JavaScript testing along with good coding habits.