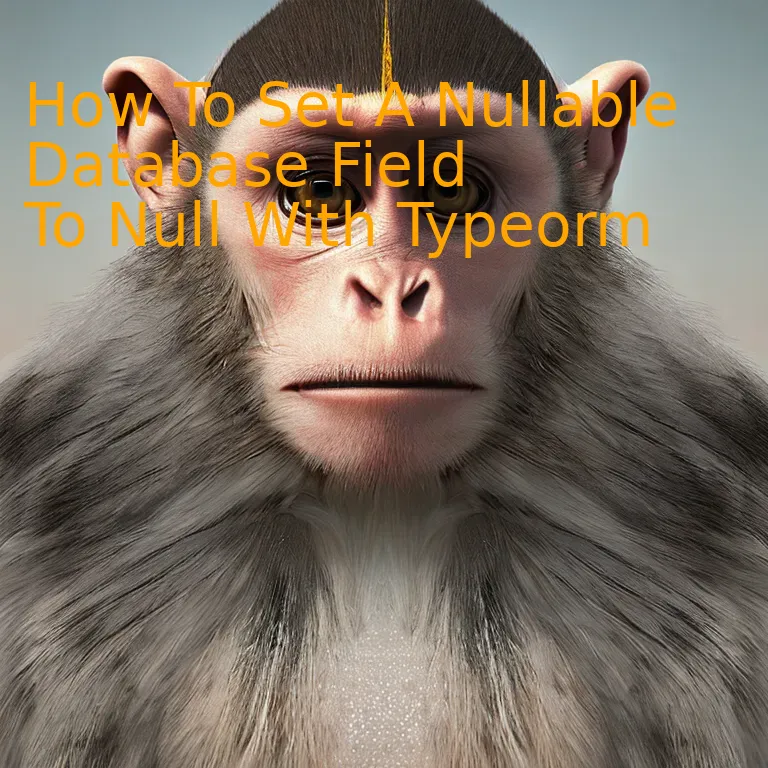
Introduction
When using Typeorm, setting a nullable database field to null is straightforward; simply choose the field you wish to manipulate in your entity and assign the value Null to it. This action makes your data more efficient by reducing unnecessary redundancies.
Quick Summary
When working with TypeORM, one way to set a nullable database field to null is through the use of the `QueryBuilder` method. Consider a hypothetical entity of User where we have nullable fields such as `nickname`.
Field | Type | Nullable |
---|---|---|
username | string | No |
nickname | string | Yes |
string | No |
To set the nickname to null in our case, the usage might look as follows:
repository.createQueryBuilder()
.update(User)
.set({ nickname: null })
.where("username = :username", { username: "sampleUser" })
.execute();
In this snippet above, we employed `createQueryBuilder()` which is a method of Repository and it’s providing a way to write a type-safe queries. The `update(User)` specifies which entity to update. The `set({ nickname: null })` part tells it to set the nullable ‘nickname’ field to ‘null’. The subsequent `where` clause identifies the user whose nickname will be updated by using their username.
This will result in a SQL query similar to: UPDATE User SET nickname=NULL WHERE username=’sampleUser’; This directly sets the nickname field to null in the database for the specified user.
Referencing the guidance of popular programmer Edsger Dijkstra who said, “Simplicity is a prerequisite for reliability”. Keeping the codes simple and clean can increase the readability and maintainability of it. Can avoid possible mistakes, and make debugging easier. The simplicity of TypeORM and its `createQueryBuilder` method allows us to precisely modify our data while maintaining reliable code functionality.
Leveraging Typeorm for Handling Nullable Database Fields
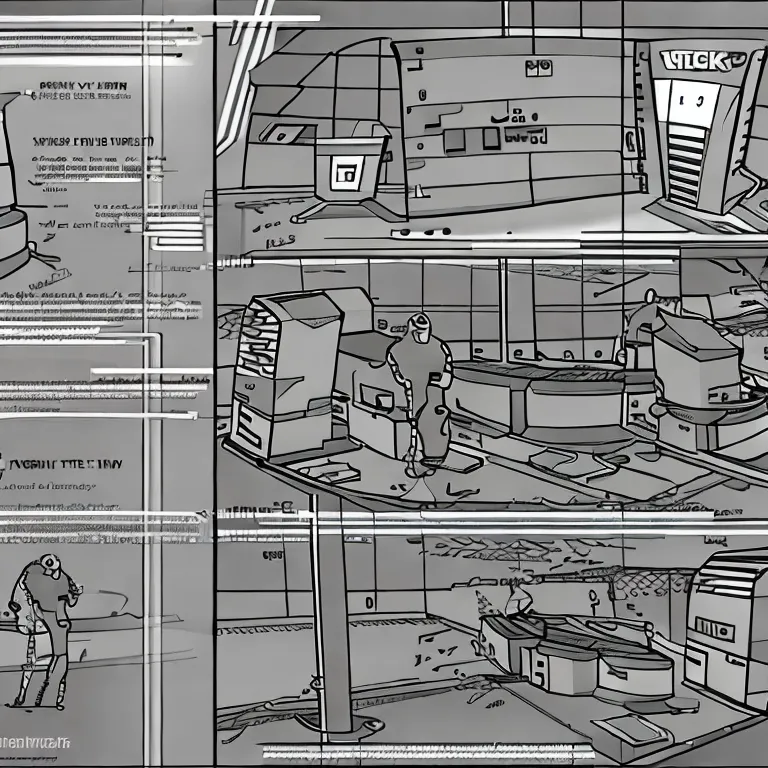
Creating solid, efficient databases often involves dealing with nullable fields. In this context, using TypeORM is highly effective given its comprehensive set of tools explicitly designed for handling such scenarios.
Let’s discuss how to use TypeORM for managing nullable database fields, more explicitly, how to set a nullable database field to null.
In many cases, you would want to have a database column that can hold null values. Here, the ‘nullable’ option in TypeORM plays a significant role. The ‘nullable’ property is a boolean indicating whether the column allows null values or not.
For instance, consider the following code snipet:
xxxxxxxxxx
@Entity('users')
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column({ nullable: true })
email?: string;
}
The
xxxxxxxxxx
@Column({ nullable: true })
part designates that the ’email’ field can be set as null.
Setting this field to null is fairly simple, and can be done by assigning the value ‘null’ to the corresponding property in the entity.
Consider the following example:
xxxxxxxxxx
let user = new User();
user.email = null;
repository.save(user);
In the code mentioned above, we create an instance of the ‘User’ class and set its ’email’ field to null. This field is automatically persisted in the database when the
xxxxxxxxxx
repository.save(user)
command is executed. Nullability in TypeORM ensures a clear, unequivocal assignment of ‘null’, maintaining the accuracy of data while promoting greater flexibility.
Remember the words of Robert C. Martin, “A good software system is one that allows you to change your mind easily.”
Hence, using TypeORM enhances adaptability during development and maintenance of a database system. By correctly leveraging nullable fields, developers can ensure robustness and extended functionality in their systems in line with individual project requirements.
For more information on TypeORM and handling nullable fields, refer to the TypeORM documentation.
Understanding the Concept of Nullable Fields in Database with Typeorm
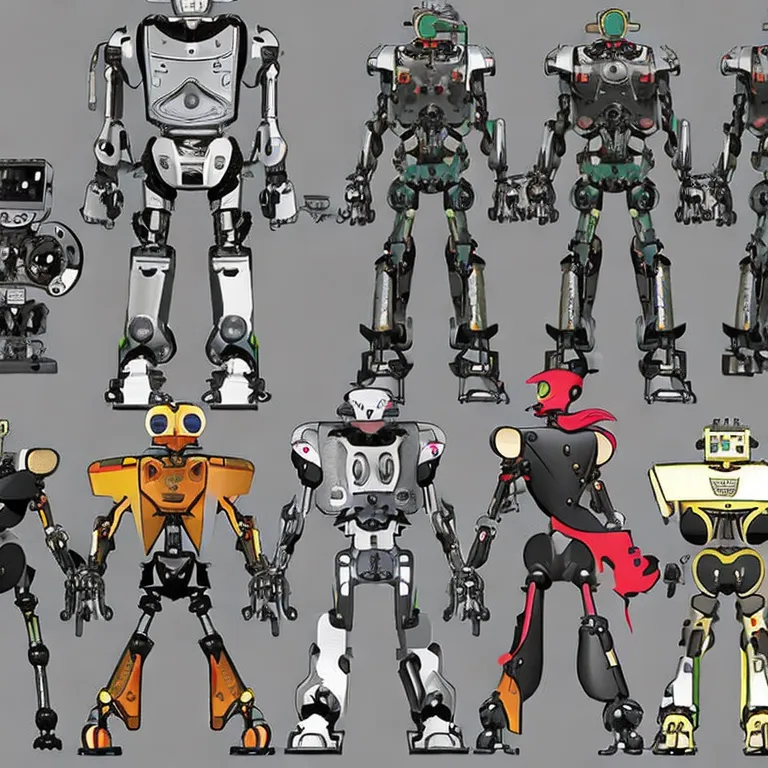
In database terminology, ‘nullable’ refers to the attribute of a field that allows storing ‘NULL’ as its value. In other words, if a certain field in your database table is designated as nullable, that essentially implies this field has the capacity to contain no data at all; it can be null!
With Typeorm, setting a nullable field to null in the database is a straightforward task. Use the `@Column` property decorator provided by Typeorm and set the `nullable` option to true. Let’s take a look at how to precisely perform this action:
xxxxxxxxxx
import { Entity, PrimaryGeneratedColumn, Column } from "typeorm";
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column({ nullable: true })
name: string;
}
In this code snippet, we have an entity named ‘User’. Here, the ‘name’ column is set as nullable. Therefore, when creating a new user, if you do not provide any value for the ‘name’ field, Typeorm will automatically save NULL in the ‘name’ field in the database.
Setting this specific field to null while updating an existing user would work in a similar manner:
xxxxxxxxxx
await repository.update(id, { name: null });
Here, when the update method gets executed, it will directly set the name field of the user with the corresponding id to NULL in the database.
This feature is particularly advantageous and versatile for several reasons:
* It helps maintain data integrity: When no valid data can be supplied to a field, it’s better to set it as NULL rather than using a ‘magic number’ or a separate ‘invalid value’.
* It eases querying and filtering: SQL language has robust support for handling NULL values. Using nullable fields will let you leverage built-in SQL functions like IS NULL and IS NOT NULL.
* It supports optional database entries: If you have a field that might not always contain data (for instance, an additional contact number), you can set this field as nullable.
Understanding this capability of Typeorm opens up numerous operational avenues in terms of data management. As famously quoted by Andrew Grove, a pioneer in the semiconductor industry – “Technology happens, it’s not good, it’s not bad. Is steel good or bad?” Each tool, each technology has its explicit purpose. In databases, understanding the concept of nullability and how to utilize it effectively is indeed significant.
However, the use of Nullable fields requires a certain level of caution. Remember that NULL in databases signifies the absence of any value, and it is different from an empty string or zero or false. Misinterpretation of NULL value can lead to erroneous results in your applications. Therefore, while utilising the flexibility of nullable fields, also ensure to handle them appropriately in your application logic.
Implementing Null Values in Databases: A Practical Guide through Typeorm
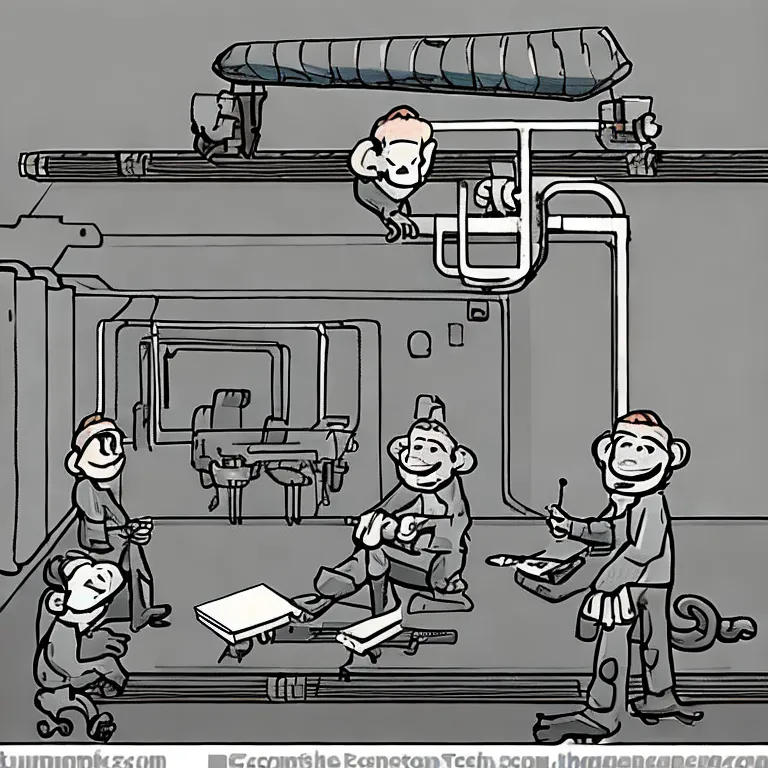
Managing null values in databases is a significant consideration when you’re dealing with data. Specifically, in relation to TypeORM, a popular ORM (Object-Relational Mapping) tool used with TypeScript, it becomes essential to understand the specifics of setting nullable fields to ‘null’. This process involves several steps in both the framework and database which are connected through TypeORM.
When creating tables or altering existing tables using TypeORM’s database schema APIs, you have the option to designate certain fields as nullable. Fields that are set as nullable imply that they can hold a NULL value. This can be achieved by including
xxxxxxxxxx
nullable: true
configuration in the column definition within your entity class, like so:
xxxxxxxxxx
@Entity('users')
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column({
type: 'varchar',
nullable: true,
})
name: string | null;
}
In the example above, we have defined a `User` entity with an `id` and `name` field where the `name` field can potentially hold NULL value. The resultant SQL query generated by TypeORM would include the `
xxxxxxxxxx
NULL
` keyword, reflecting our definition.
With this setup, TypeORM now allows us to set the `name` field to NULL within our application code. Here is a quick snippet illustrating how to accomplish this:
xxxxxxxxxx
const userRepository = getConnection().getRepository(User);
const user = await userRepository.findOne(1); // ID being 1
user.name = null;
await userRepository.save(user);
Here, after obtaining an instance of our `User`, we can simply assign a NULL value to `name` and persist the change using the `save` method from the repository API. Under the hood, TypeORM will maneuver this action into a valid SQL command to update the respective record in the underlying database, hence setting the `name` field to NULL.
Remember, as underscored by Robert C. Martin in his book ‘Clean Code’, “Truth can only be found in one place: the code.” The efficacy of this approach is clearly seen as TypeORM interfaces with your TypeScript code and relates it accurately to your SQL database, making code the single source of truth.
While dealing with null values, developers should remember that a nullable field could have three possible states: the actual value, no value (missing) or unknown. Hence, careful considerations should be made during schema designing process in a way that respects data integrity while adhering to the business rules of your application.
For more information regarding TypeORM’s column types click here.
Methods to Set a Nullable Field to Null using Typeorm
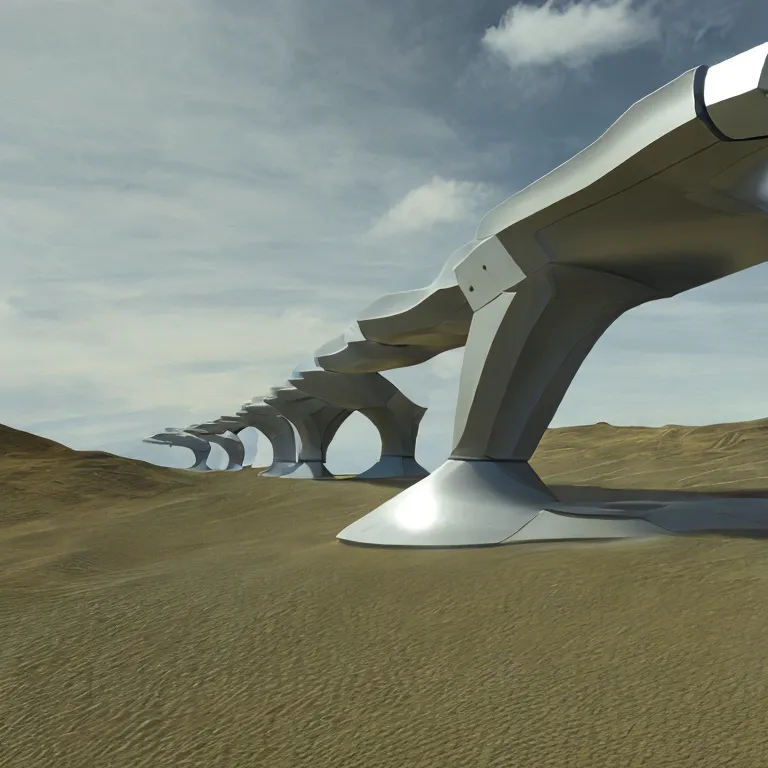
One important aspect of working with relational databases(Possibly PostgreSQL, MySQL, SQLite) using Typeorm, a powerful Object-Relational Mapping(ORM) tool, is managing nullable fields. A field in the database can be defined as nullable, meaning it can have a null value. Sometimes, due to certain application/logic, you may want to set these nullable fields to null. The challenge here is how to achieve this effectively with Typeorm.
Set a Nullable Field to Null using Save Method
One practical way is to use the Typeorm `save` method. Below is a simple illustration of how it can be done.
xxxxxxxxxx
let user = userRepository.findOne(id);
user.nullableField = null;
userRepository.save(user);
Here’s what is happening:
- The `findOne(id)` method fetches a user instance from the userRepository using the unique ‘id’.
- Then, the nullable field under consideration ‘nullableField’ is explicitly set to null.
- Lastly, the save method(`userRepository.save(user)`) is called to persist the changes to the database, which sets ‘nullableField’ to null.
This method leverages the implementation of the unit of work and identity map patterns by Typeorm when saving entities. Every entity gets its special treatment – new entities get inserted, while previously loaded entities get updated[Typeorm EntityManager].
It’s relevant to note that updates with Typeorm methods do not automatically apply to entity instances. Therefore, subsequent queries might return the old un-updated entity. It would help if you fetched the entity again after the update operation to ensure you’re working with the up-to-date data.
Using QueryBuilder to Set a Nullable Field to Null
Another approach is by using the Typeorm `QueryBuilder` method. The QueryBuilder provides more control over SQL Queries and can be used to build a custom query.
xxxxxxxxxx
userRepository.createQueryBuilder()
.update(User)
.set({ nullableField: null })
.where("id = :id", { id: user.id })
.execute();
In this context, a query is built that updates the User entity to set ‘nullableField’ to null where the user’s “id” matches the given ‘user.id’.
Remember, any interaction with databases should be treated cautiously. Incorrect handling can lead to unintended consequences.
“The most important property of a program is whether it accomplishes the intention of its user.” – C.A.R. Hoare, British computer scientist
From the above, it’s evident that Typeorm provides efficient ways to manipulate nullable fields in a database safely and intuitively. With continued practice and exploration, you’ll find it easier and rewarding dealing with relational database operations.
Conclusion
In the realm of database management, setting a nullable field to null using Typeorm is often an important function for developers. Notably, Typeorm provides key tools and techniques that allow developers to set nullable fields with less effort.
The first step in this process is recognizing the type of field in question. For instance, is the field numeric or alphanumeric? If it’s numeric, Typeorm allows us to set it to null naturally. However, with alphanumeric fields, we need to handle them with care as they can be set to empty strings rather than null.
HTML code for defining nullable fields in Typeorm can look like this:
xxxxxxxxxx
@Column({ nullable: true })
someField?: any;
In the above example, the `nullable` property is set to `true`, which permits the value to be set as `null`. The `someField` is also optional, hence the use of the question mark (`?`) right before the colon (:). This indicates that `someField` might contain a value, but it is not mandatory.
When you want to subsequently assign a null value to ‘someField’ declaratively, this could be achieved as follows:
xxxxxxxxxx
repository.create({ someField: null });
In the aforesaid example, you use repository’s `create` method where ‘someField’ is being set explicitly to null. Remember, the ‘create’ method constructs a new entity instance and populates it with the given values.
Typeorm offers extensive capabilities far beyond just managing nullable fields. The rich feature set of Typeorm, including transactions, column types, entity managers, repositories, relations, cascades, and complex queries, make it a desirable Object-Relational Mapping (ORM) tool among JavaScript and Typescript developers. Its flexibility and dynamic approach towards handling database operations make Typeorm unmatched to its competitors. This elevates the use of Typeorm into a more intricate context in real-life applications, consolidating its relevance in the sphere of database management.
As Bill Gates remarked, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency.” Similarly, leveraging automated tools like Typeorm for managing databases is part of magnifying efficiency in a development arena.
Remember, it’s not just about assigning null values to fields; it’s also about understanding how these operations impact the overall functionality and proficiency of your application.