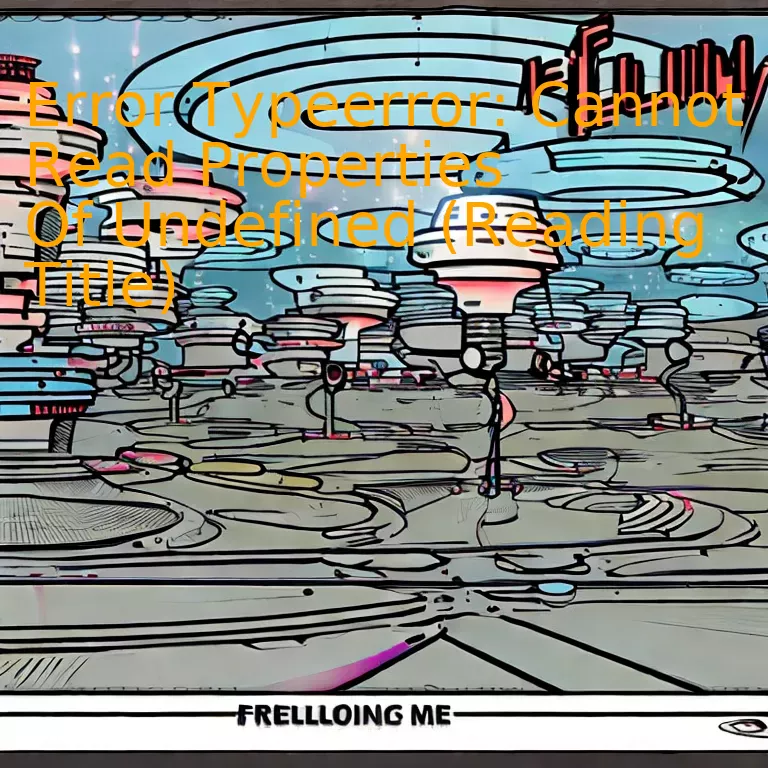
Introduction
An insightful understanding of a common javascript error, TypeError: Cannot read properties of undefined (reading title), is crucial since it typically transpires when we attempt to access an attribute or method on a variable that does not hold and/or is not originally destined to possess a value, thus nourishing an improved web development experience.
Quick Summary
The error message “TypeError: Cannot read properties of undefined (reading ‘title’)” appears during the runtime of JavaScript or TypeScript code when an attempt is made to access a property of an undefined object. To help understand this better, let’s use a tables scenario as means of illustration.
Error Code | Issue Trigger | Potential Solution |
---|---|---|
x 'TypeError: Cannot read properties of undefined (reading \'title\')' |
The code attempts to access a property (in this case, ‘title’) of an object that isn’t defined or is currently undefined. | Ensure the object you’re trying to access exists and has been properly initialized before trying to retrieve any of its properties. |
Let’s delve deeper into the cause of this error. To illustrate, suppose we have the following piece of code:
xxxxxxxxxx
var blogPost;
console.log(blogPost.title);
In this example, the variable `blogPost` has been declared but not assigned a value, meaning it’s undefined by default in JavaScript. When we try to access a property (‘title’) of an undefined object (`blogPost`), we receive the TypeError.
The solution lies in ensuring all objects are initialized before attempting to access their properties. Modifying our previous code snippet:
xxxxxxxxxx
var blogPost = { title: 'Understanding TypeErrors'};
console.log(blogPost.title);
Now that `blogPost` is defined as a JavaScript object complete with a ‘title’ property, the TypeError no longer occurs, proving that proper initialization is essential in preventing such errors.
To put in Steve McConell’s words, “Good code is its own best documentation… When you’re about to add a comment, ask yourself, ‘How can I improve the code so that this comment isn’t needed?’.” Reflecting upon this quote, it’s realised that following correct initialization and checking for undefined variables, we can prevent such errors from occurring in future, reducing the need for extensive debugging and ensuring self-explanatory, well-documented code.
Understanding the TypeError: Undefined Properties Issue
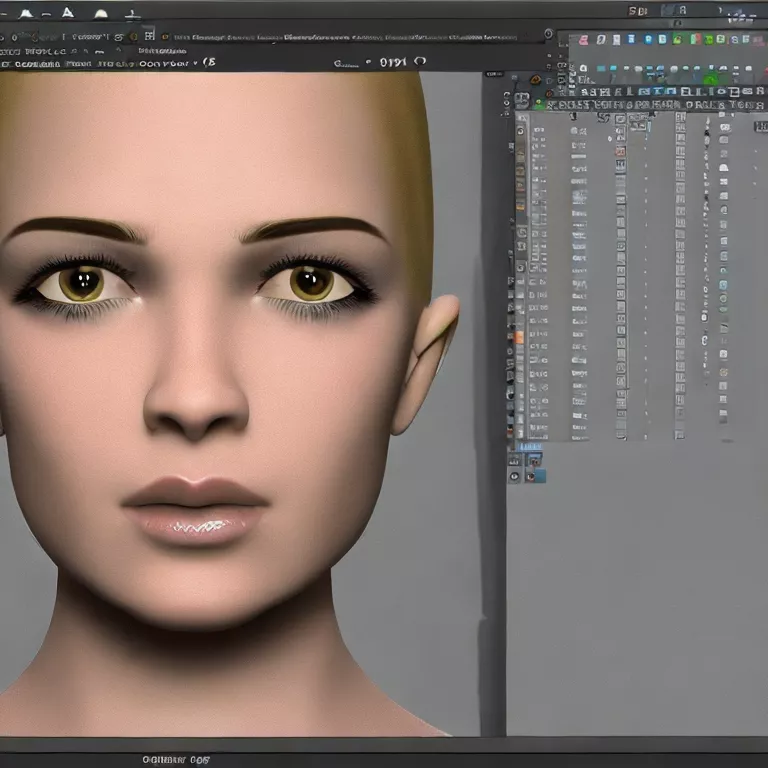
To correctly grasp the “TypeError: Cannot read properties of Undefined (reading ‘title’)” error, it is crucial to comprehend that this particular issue usually emerges due to attempts at accessing properties on an object that has not been defined.
To visualize this, consider the following piece of TypeScript code:
xxxxxxxxxx
let book;
console.log(book.title);
In the code snippet above, the variable “book” has been declared but not defined or initialized. Therefore, when one attempts to access a property named “title” from “book”, TypeError occurs because you are essentially trying to read property from an undefined variable.
To address this problem, we have two key solutions that revolve around checking if the object is defined before utilizing its properties and initializing your variables or objects during declaration respectively. This can again be illustrated as follows:
xxxxxxxxxx
let book = {};
console.log(book.title);
Here, the variable “book” is initialized as an empty object using curly brackets (‘{}’). Even though the “title” attribute does not exist in the book object, attempting to access it will not throw TypeError but will rather return an “undefined” since there’s no value set for the non-existent property.
Alternatively, a common pattern in JavaScript and TypeScript is to use the logical AND (&&) operator when trying to avoid such assertions on potentially undefined reference:
xxxxxxxxxx
let book;
console.log(book && book.title);
In above example, the log statement prints the value of `book.title` only if `book` is defined. If `book` is indeed undefined, the ‘&&’ condition fails up front and `book.title` is never attempted to be accessed, avoiding the aforementioned TypeError.
However, it’s always important to note that preventing errors through proper initializations can truly pay dividends. As Undefined properties issues could lead to unexpected behaviours in TypeScript. It is often beneficial to initialize variables and objects during the declaration, which might lead to cleaner and bug-free code.
In the wise words of C.A.R. Hoare, “There are two ways of constructing a software design: One way is to make it so simple that there are obviously no deficiencies, and the other way is to make it so complicated that there are no obvious deficiencies. The first method is far more difficult.”. Thus, understanding and fixing errors such as “TypeError: Cannot read properties of Undefined (reading ‘title’)” can significantly simplify the coding process and result in a cleaner, more efficient codebase.
For further learning on handling such issues in TypeScript, consider exploring the official TypeScript handbook. This resource provides comprehensive guidelines on how to effectively handle different error types among other helpful coding tips.
Exploring Causes of “Cannot Read Properties of Undefined” Error
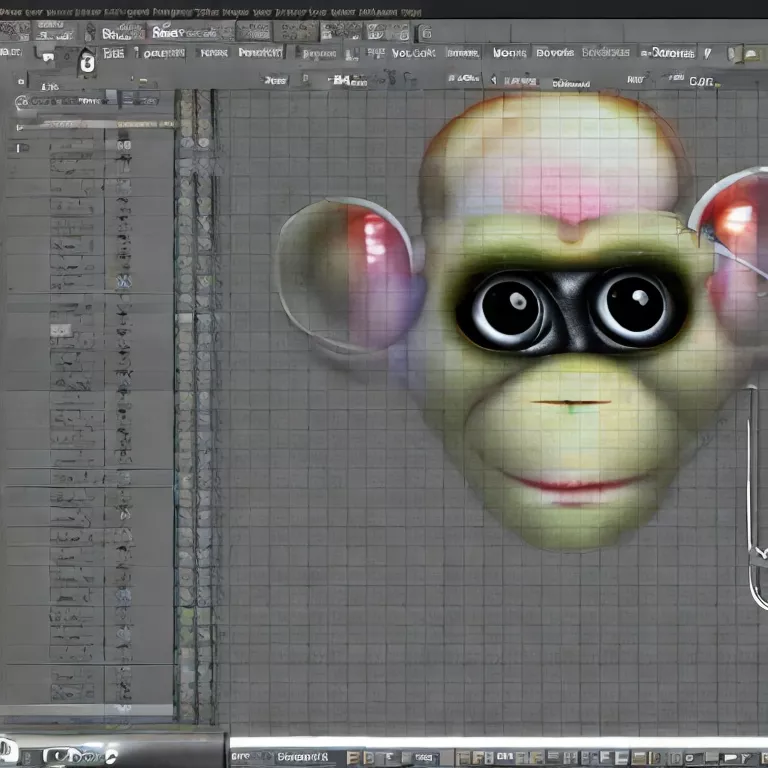
“TypeError: Cannot Read Properties of Undefined (Reading ‘title’)” is an error you might come across while working with JavaScript or TypeScript. This error typically occurs when you try to access a property (‘title’ in this case) on an object that happens to be undefined. Here’s how the problem code might look:
xxxxxxxxxx
let obj;
console.log(obj.title);
In this example, ‘obj’ is undefined, so trying to read the ‘title’ property will throw an error. Let’s analyze potential causes for this error:
- Property Initialization: Ensure that the object and its properties have been initialized before accessing them. If you declare an object but forget to initialize it, or if it is asynchronously set at some point which has not yet occurred, you get this error.
- Non-existent Property: If you are trying to access a property that doesn’t exist on the object, this error can occur. Make sure the property you’re trying to access is defined on your object.
- Synchronous vs Asynchronous Code: As JavaScript is asynchronous, sometimes data expected in an object may not yet be available when the line of code runs. This can result in an undefined error.
- Scope Issues: If the object was declared in a different scope than where you are trying to access it, it could be undefined and cause this error.
To prevent these errors, use conditionals to check if the object and/or property is not undefined before accessing it.
xxxxxxxxxx
if (obj && obj.title) {
console.log(obj.title);
}
“An undefined variable is a reality in coding. Handling it gracefully is art!” – A developer’s wisdom.
Moreover, in TypeScript, there’s a neat way to prevent this error using optional chaining:
xxxxxxxxxx
console.log(obj?.title);
Optional Chaining in TypeScript automatically returns undefined if the object is null or undefined, preventing the run-time error.
For further reading, one might find this Mozilla Developer Network (MDN) documentation about handling these types of errors helpful.
Solutions to Rectify the ‘Reading Title’ TypeError
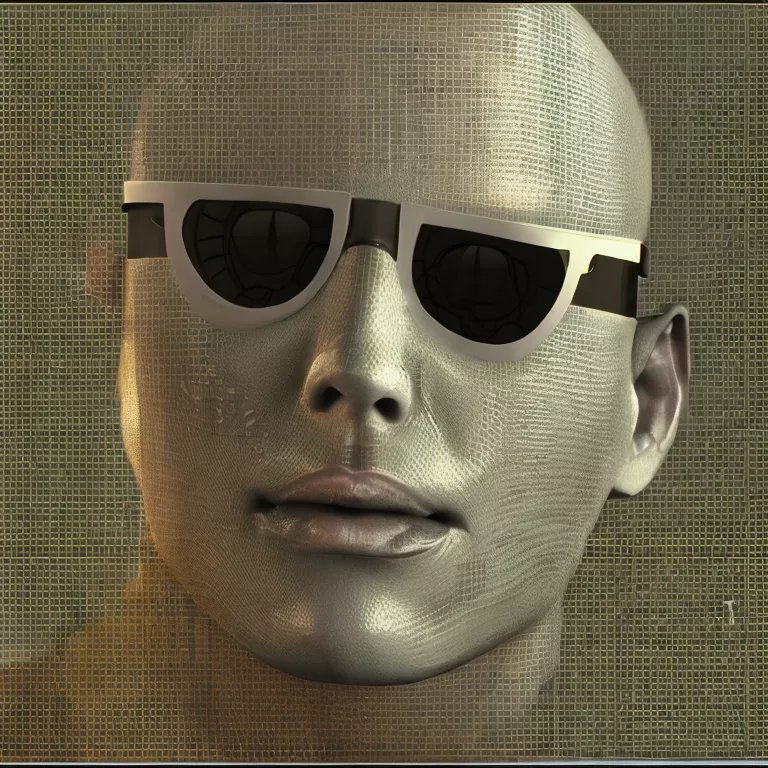
The ‘TypeError: Cannot read properties of Undefined (reading title)’ in Typescript is an error message that you would encounter when you try to access a property or method of an object that is undefined at runtime. This is one common issue in JavaScript and TypeScript programming because these languages are dynamically typed.
To rectify this issue, it’s fundamental to comprehend the cause behind it. This particular error is thrown when:
– You’re trying to access a property from an uninitialized variable.
– Trying to invoke a function or method from an undefined object.
Redefining Error Example:
xxxxxxxxxx
let profile: any;
console.log(profile.title);
In this snippet, the variable profile has been declared but not initialized, thus triggering the TypeError when trying to access the ‘title’ property.
Solutions:
1) Type Checking: To mitigate this issue, remember to always conduct a type check before accessing properties or methods.
xxxxxxxxxx
if (profile) {
console.log(profile.title);
}
What we’ve added here is a simple conditional statement to check whether the object exists before accessing its properties. This prevents an attempt to read properties off an undefined object.
2) Initialize default object structure: Another approach to solving this problem involves initializing your object with default values to retain the structure.
xxxxxxxxxx
let profile:any = {title: ''};
console.log(profile.title);
In this instance, even if the ‘title’ doesn’t contain any value, it would not trigger a TypeError as we have defined a default structure for the profile object.
3) The use of Optional Chaining: The release of newer ECMAScript versions incorporated optional chaining which aids to defend against TypeErrors. If the variable is null or undefined, the expression short-circuits, returning undefined, preventing TypeErrors from triggering.
xxxxxxxxxx
console.log(profile?.title);
‘Optional Chaining’ was a wonderful addition to the language – it allows you to navigate through nested object properties without having to check each level for null or undefined values. As Justin Searls, the co-founder of Test Double once quoted, “Optional chaining and Nullish coalescing are game-changers when it comes to dealing with nullable objects!”
When dealing with such errors in TypeScript, remember that static type checking could be an invaluable tool. Adhering to best practices like strict null checks (tsconfig: strictNullChecks) can help detect these issues during compile-time, significantly reducing runtime exceptions. Use them diligently to craft robust and error-free applications.
Preventative Measures to Avoid ‘Undefined Properties’ Errors
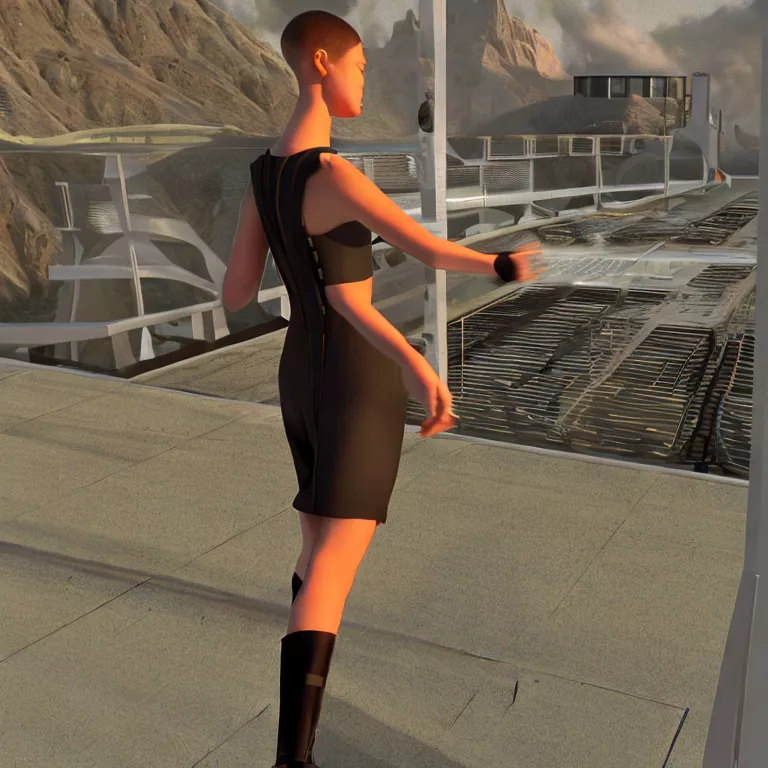
The penchant to encounter ‘Undefined Properties’ Errors, especially within the context of “Error Typeerror: Cannot read properties of Undefined (Reading Title)” frequently plagues developers, undermining the efficiency of their code structures and application functionality. These errors often stem from attempts at accessing properties or methods on variables that essentially define no value. Acknowledging the necessity of eloquent solutions, several preventative measures can be put into effect to combat these setbacks.
1. Integrate a Bespoke Check Prior to Property or Method Access
Within TypeScript, it’s essential to have a safeguard in place that ensures a variable is not undefined before proceeding to access its properties or methods. Implementing straightforward condition checks can help prevent TypeError occurrences related to undefined variables.
For example:
xxxxxxxxxx
if (variable != null) {
console.log(variable.title);
}
In the above code snippet, an attempt would only be made to log ‘variable.title’ on the condition that ‘variable’ is not undefined or null, effectively preventing possible TypeError instances.
2. Leverage Optional Chaining (?)
Optional chaining in TypeScript represents yet another compelling approach for handling ‘undefined’ issues, providing a more concise approach to values checking. By appending a question mark (?) before the complete access of any property or method, we establish that should the variable derived be defined, then the property is accessed. Otherwise, the chain breaks, and ‘undefined’ is returned, minimizing risk of TypeError encounters.
Here is how you might implement this technique:
xxxxxxxxxx
console.log(variable?.title);
This will ensure that ‘title’ is only accessed if ‘variable’ is not undefined, operating similarly to the prior described conditional check but with an added tincture of brevity.
3. Apply Nullish Coalescing Operator (??)
Our third solution criteria emphasize the incorporation of the Nullish Coalescing Operator (??). This operator helps to deal with ‘undefined’ or `null` values by providing a default value when the variable seen is undefined.
Here’s how you can utilize this operator in practice:
xxxxxxxxxx
console.log(variable?.title ?? 'Default title');
In this code snippet, if ‘variable’ or ‘variable.title’ are either undefined or null, the script prints ‘Default title’, promoting a vital fallback and thereby precluding potential TypeError situations.
As programmers, our imperative lies not just in cultivating potent solutions, but also in prophylaxis against probable errors. By infusing these techniques into your Typescript development practices, you may well insulate your applications from TypeError issues and boost their overall performance efficacy.
As Giorgio Ascoli, an expert on Neuroscience Information Technology once remarked, “The greatest challenge to any thinker is stating a problem in a way that will allow a solution.” – an aphorism that resonates deeply in the programming arena.
For further reading, check out the official TypeScript Handbook.
Conclusion
The “TypeError: Cannot read properties of Undefined (reading title)” is a common error plaguing many novice and intermediate TypeScript developers alike. Often, this error occurs when attempting to access the properties of an undefined variable in a TypeScript codebase.
To understand the nature of this error, let’s consider the object access notation in TypeScript:
xxxxxxxxxx
let hero = {
name: 'Superman',
title: 'Justice',
}
console.log(hero.nickname);
In the above code, the TypeError would flare up because we try to access the nickname property, which does not exist on the hero object. The property is undefined, hence leading to the TypeError.
Overcoming this error requires keen adherence to TypeScript best practices:
* Always check if an object is defined before reading its properties.
* Use optional chaining with ‘?.’ to prevent errors when reading undefined properties. This nullish coalescing operator comes in handy when dealing with data that may not follow the structure expected.
* Implement exception handling measures like
xxxxxxxxxx
trycatch
blocks in your code.
Remember, robust testing is essential for identifying potential points of failure in your TypeScript applications, thereby mitigating TypeErrors even before they occur. By leveraging Unit Tests, Integration Tests, and End-To-End tests, you could ensure maximum application stability.
As Google’s Jim Armstrong said: “Code without tests is bad code. It doesn’t matter how well written it is; it doesn’t matter how pretty or object-oriented or well-encapsulated it is. With tests, we can change the behavior of our code quickly and verifiably. Without them, we really don’t know if our code is getting better or worse.”
Links for further reading:
[Understanding TypeScript Error Handling](https://www.typescripttutorial.net/typescript-tutorial/typescript-error-handling/)
[Optional Chaining in TypeScript](https://www.typescriptlang.org/docs/handbook/release-notes/typescript-3-7.html#optional-chaining)