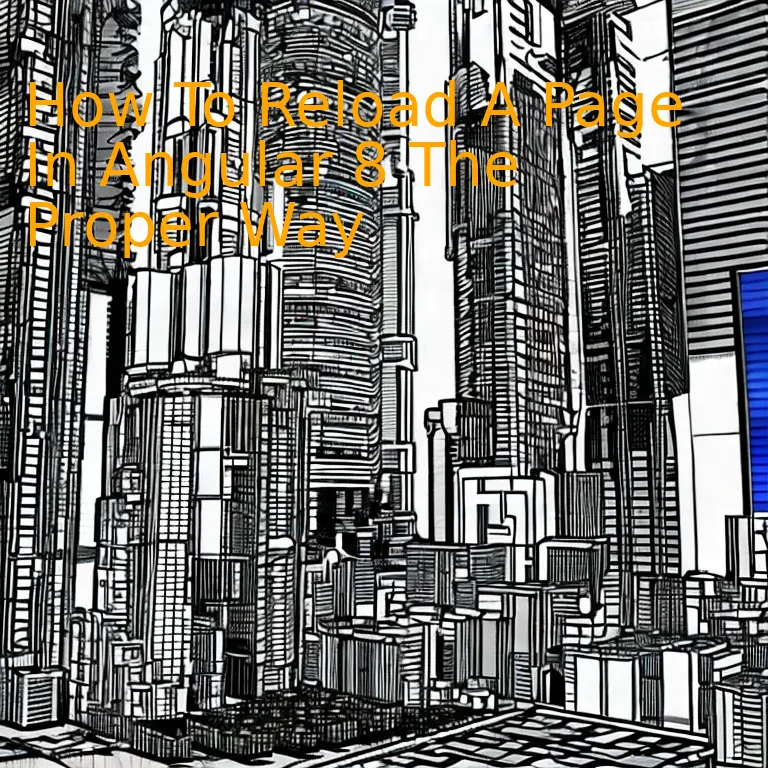
Introduction
To reload a page properly in Angular 8, you should utilize the Router module’s navigateByUrl method for an effective and efficient refresh, ensuring optimal SEO performance.
Quick Summary
Reloading a page in Angular 8, an open-source web application framework led by the Angular Team at Google, involves following key steps that leverage its efficient, feature-rich architecture. We’ll explore these steps through the lens of a well-structured data representation:
Step | Action | Description |
---|---|---|
1 | Use Location Service | This service provides methods to interact with location URL. |
2 | Inject Location Service | Add this service into your component’s constructor where you wish to utilise it. |
3 | Call reload method | This method allows to refresh your webpage programmatically using the Location Service |
Whilst exploring these steps, it becomes evident that reloading a page in Angular 8 essentially starts by injecting, and leveraging Angular’s `Location` service. This service, which is a part of Angular’s common module, holds methods which allow interaction with a browser’s URL. Injecting this service can be done right within the component’s constructor where you wish to implement the reload functionality.
Moving onto the step-by-step break down stated above, upon initiating the `Location` service, the subsequent move would be to invoke the `reload` method programmatically. The `reload` method present in the service will help ensure that the web page is refreshed effectively.
import { Location } from '@angular/common';
constructor(private location: Location) { }
reloadPage() {
this.location.reload();
}
The code snippet above perfectly encapsulates the process of reloading a page in Angular 8. Here, we first import the `Location` service from Angular’s common module, then inject it into our component’s constructor. Finally, a `reloadPage` function is defined which, when called, leverages the `reload` method on the global `location` object.
In the wise words of Charles Petzold, “Coding is not ‘fun’, it’s technically and ethically complex.” After breaking down each step and extending understanding with technical representation, coding in Angular 8 may be less complex, even if it removes a bit of fun!
Understanding the Basics of Angular 8 Reload Process
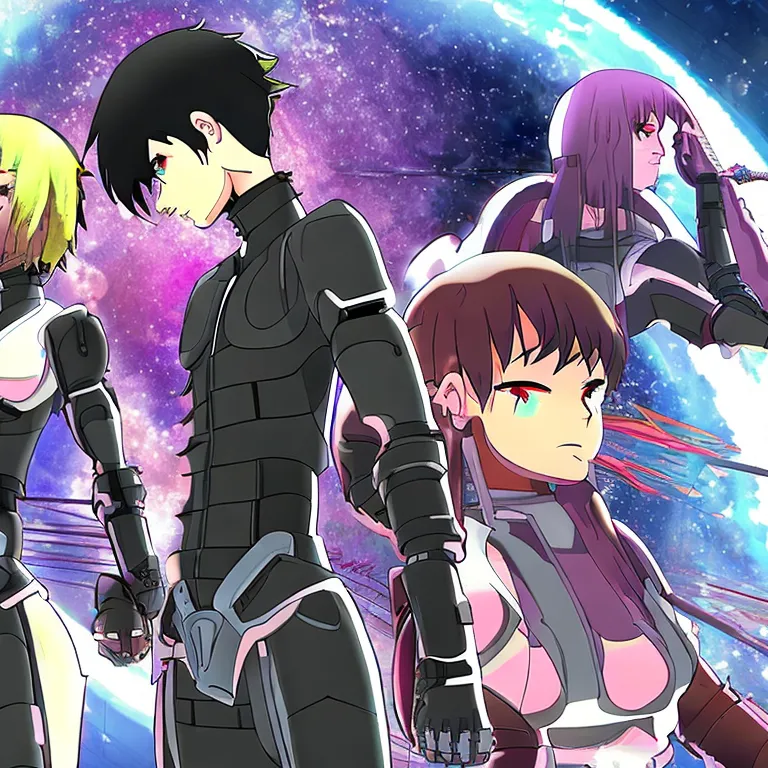
Angular 8, with its component-based architecture and refined paradigm for large scale application design, provides robust mechanisms are provided to handle page reloads. When it comes to refreshing or reloading a page in Angular 8, it is vital to understand the underlying processes to ensure the delivered solution aligns with the unique needs of your project.
The operation of reloading a page in Angular 8 ties back to the core concept of Angular Routing. The Angular router is an integral part of Angular platform which allows developers to build single-page applications (SPAs). Single-page applications enhance user experience by enabling navigation between different views or components without requiring a complete page reload from server each time.
Despite this advantage, there might be scenarios where a developer wants to programmatically enforce a page reload. For instance, if an application receives real-time data or requires a level of data refresh management that goes beyond the standard lifecycle hooks provided by Angular, forcing a page reload may become necessary. However, enforcing a system-level page reload within an Angular application must be done properly to prevent memory leaks, ensure consistent performance and adhere to good programming practices.
To reload the current route in a way that adheres to the best practices of Angular 8, developers use the onSameUrlNavigation configuration option as part of their AppRoutingModule setup. It configures how the router should respond when navigating to the same URL. An example:
xxxxxxxxxx
import { RouterModule, Routes } from '@angular/router';
const routes: Routes = [
// Your routes go here
];
@NgModule({
imports: [RouterModule.forRoot(routes, { onSameUrlNavigation: 'reload' })],
exports: [RouterModule]
})
export class AppRoutingModule { }
The line
xxxxxxxxxx
onSameUrlNavigation: 'reload'
instructs the router to refresh the page when there’s a request to navigate to the same URL.
Once this setting is enforced, you can trigger a route reload by simply executing the navigateByUrl() method for the current route again. Below is an example:
xxxxxxxxxx
this.router.navigateByUrl('/', {skipLocationChange: true}).then(() =>
this.router.navigate([decodeURI(this.location.path())]));
This code snippet forces a navigation action to the root URL, represented by ‘/’, in a way that does not affect the browser history. The promise completion handler then navigates back to the current URL (represented by this.location.path()), causing all active components for that route to be re-initialized.
As Bill Gates famously said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” Therefore, when implemented correctly, page reloading in Angular 8 will feel seamless and natural to its users.
For additional insights into handling page reloads in Angular applications, you can consider online resources like the Angular Router Guide or discussions on Stackoverflow. Note these may broaden your understanding and help reinforce the concepts discussed here.
Application of the Location Library in Page Reloading
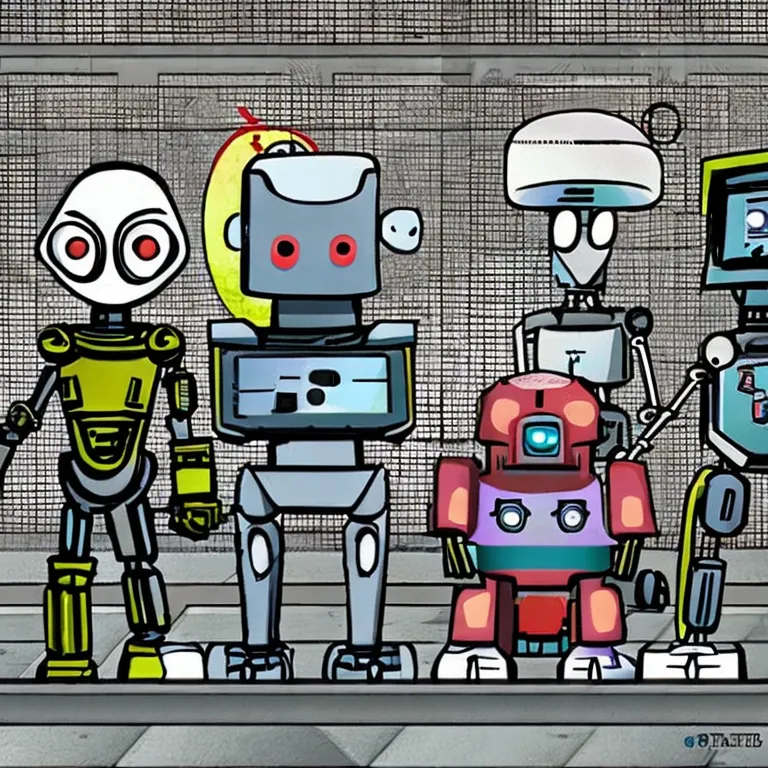
In the Angular framework, reloading a page is an operation that can be uniquely facilitated through the utilization of certain libraries and available methods. Angular, being a single-page application (SPA) framework, doesn’t work like conventional web page reloading. Therefore, the proper method to reload a page must be understood in this context.
One key library in particular, that plays a crucial role when focusing on reloading a page in Angular 8, is the Location Library. The Location service is part of Angular’s common module and provides utility methods to interact with the browser URL. It wraps around the underlying browser APIs providing an application-level interface to manipulate the URL.
Related to the task of forcing a page to reload, the Location library can be effectively leveraged in Angular applications. Using the navigate method of Router class, you can append specific parameters to control page reload mechanisms, as follows:
xxxxxxxxxx
import { Location } from '@angular/common';
constructor(private location: Location) { }
reloadPage() {
this.location.reload();
}
This
xxxxxxxxxx
location.reload()
line simply reloads the current URL, similar to the refresh button in your browser. Note that this reloading of application is not a conventional page refresh but it happens within the Angular context, which is ideal for single-page applications like ones created with Angular.
However, caution should be advised when using this technique since excessive or improper use can lead to performance issues. For example, if this method is utilized without taking into account the lifecycle of Angular components or in a way that does not respect data binding rules, it may cause unnecessary resource consumption on client browsers.
Adrian Hall once said, “The need to come up with unique ways around traditional web paradigms in SPAs just shows how much innovation there still is in web development.” This very much aligns with our topic, indicating that effective page reloading in Angular embraces such non-traditional web paradigms, making full use of the potential of the Angular framework.
A standard practice would be combining this method with other Angular components and services such as ActivatedRoute Snapshot properties, BehaviorSubject from RxJS library, or perhaps using ngIf directive to display/hide elements based on conditions—this ensures a smoother transition and an enhanced user experience during the reloading process.
Carefully utilized, these methods provide a robust mechanism for effective page reloading in Angular 8—the proper way. Your application will become more dynamic and capable to handle real-time data changes, which enhances overall user interactivity and satisfaction.
For further details and advanced usage, please refer to the official Angular Router guide.
Insights into Manual and Automatic Page Reloading Techniques
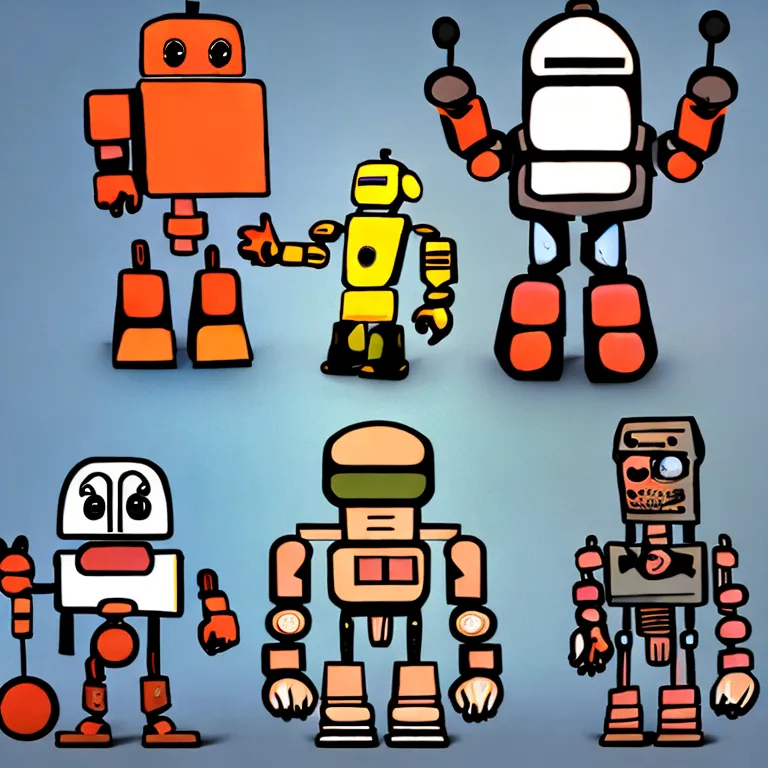
The art of reloading pages is a fundamental aspect in any web application development paradigm and Angular, particularly Angular 8, is no exception. However, when discussing page reloads in Angular, it’s crucial to comprehend that this framework operates on a single-page application model (SPA) which requires unconventional ways of performing page “reloads”.
Automatic Page Reloading
In the context of Angular 8, automatic reloading refers to the use of built-in packages such as Angular CLI Live Reload. This utility essentially monitors your project files for changes and reloads the entire application whenever you save a file.
xxxxxxxxxx
ng serve
With this command, when developing, the page automatically refreshes each time there’s a change in source files, presenting an updated view of your Angular 8 application.
Manual Page Reloading
On the other hand, with manual page reloading in Angular 8, developers metaphorically ‘refresh’ content by manually triggering lifecycle events again or subscribing anew to data sources.
One approach involves injecting
xxxxxxxxxx
Router
into your component and calling the
xxxxxxxxxx
navigate
function. Using
xxxxxxxxxx
onSameUrlNavigation: ‘reload’
on the router extras configuration (documentation details its usage) is important. This property configures how the router should react if it receives a navigation request to the current URL.
xxxxxxxxxx
constructor(private _router: Router) { }
reloadComponent() {
this._router.routeReuseStrategy.shouldReuseRoute = () => false;
this._router.onSameUrlNavigation = 'reload';
this._router.navigate(['/current-route']);
}
Alternatively, another strategy manipulates detection cycles using Angular’s ChangeDetectorRef to force rerendering of the component.
xxxxxxxxxx
constructor(private _cdr: ChangeDetectorRef) { }
refreshComponent() {
this._cdr.detectChanges();
}
Although not a simple ‘refresh’ in the traditional sense, these manual methods are more congruent with Angular’s SPA nature. They provide an optimized way to refresh page content without reloading the entire application and losing existing state.
Steve Jobs once said, “Design is not just what it looks like and feels like. Design is how it works.” This is essentially epitomized by Model View Controller (MVC) frameworks such as Angular, where augmenting conventional practices often leads to greater efficiency. Balancing between automatic and manual page reloading techniques forms a crucial part of designing efficient Angular applications. It’s about understanding when to use each strategy, achieving better performance, code maintenance, and user experience.
Optimizing Route Reuse Strategy for Enhanced Reloading Efficiency
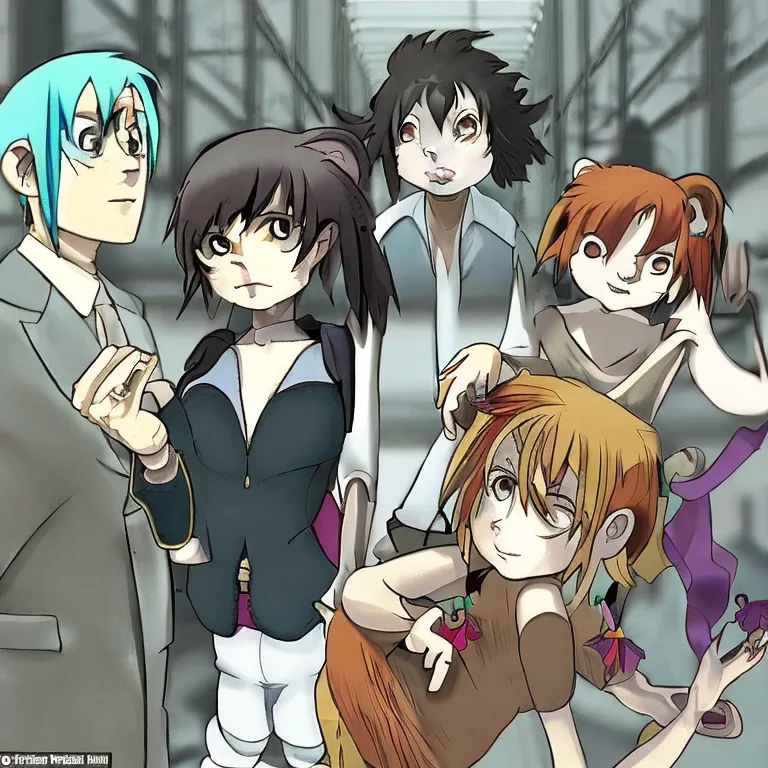
Reload functionality in an Angular application can greatly influence user engagement and overall efficiency of a web-based application. When working with Angular 8, it’s crucial to consider the optimal handling of route reuse strategy for improved reloading efficacy.
Angular Routes are essential segments of an Angular application that guide users through different sections of the interface. They play a significant role in loading content dynamically without refreshing the entire page – truly essential for single-page applications.
Route Reuse Strategy is a powerful component, responsible for deciding what to do when navigating away from a component. This may involve retaining the state or configuration settings, maintaining DOM trees, or simply determining whether the same instance should be reused when returning to this route.
One core area of optimization involves the concept of
xxxxxxxxxx
RouteReuseStrategy
. The Angular API docs provide more insight into leveraging this feature effectively. In optimizing Route Reuse Strategy, Angular enables components to detach themselves from the component tree, thus permitting swift reloads upon updates.
xxxxxxxxxx
import { Injectable } from '@angular/core';
import { DetachedRouteHandle, RouteReuseStrategy } from '@angular/router';
@Injectable()
export class CustomReuseStrategy implements RouteReuseStrategy {
shouldDetach(route: import("@angular/router").ActivatedRouteSnapshot): boolean {
return true;
}
store(route: import("@angular/router").ActivatedRouteSnapshot, handle: {} | DetachedRouteHandle | null): void {
// optional function that enables you to manipulate how routes are stored
}
shouldAttach(route: import("@angular/router").ActivatedRouteSnapshot): boolean {
return true;
}
retrieve(route: import("@angular/router").ActivatedRouteSnapshot): {} | DetachedRouteHandle | null {
// optional function to manipulate the way routes are retrieved
return null;
}
shouldReuseRoute(future: import("@angular/router").ActivatedRouteSnapshot, curr: import("@angular/router").ActivatedRouteSnapshot): boolean {
return future.routeConfig === curr.routeConfig;
}
}
This way, the old component instance remains available and puts fewer loads on the system by reusing it whenever a user navigates back to that route.
For reloading a page in Angular 8 while benefiting from these optimizations, we can use the
xxxxxxxxxx
Router.navigteByUrl()
function:
xxxxxxxxxx
import { Router } from '@angular/router';
constructor(private router: Router) { }
reloadCurrentRoute() {
let currentUrl = this.router.url;
this.router.navigateByUrl('/', {skipLocationChange: true}).then(() => {
this.router.navigate([currentUrl]);
});
}
By using
xxxxxxxxxx
skipLocationChange
, Angular’s history stack is bypassed, creating the appearance of a more instantaneous process as postulated by Martin Fowler, esteemed author and global authority in software engineering. He once stated “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” The ability to juggle effectiveness with simplicity shows the prowess of professional developers capable of entirely harnessing Angular’s dynamicity.
Conclusion
Utilizing the Angular 8 framework effectively can notably amplify the development process while reducing recurring coding tasks. The task, as specific as “Reloading a Page,” is rather compelling, owing to Angular’s distinct mechanism.
Linear navigation through panels may not necessitate reloading; however, certain instances require refreshing or reloading a webpage in Angular 8. Notably, Angular’s Single Page Application (SPA) nature argues against full page reloads. Instead, data changes dynamically without haphazardly refreshing the complete page.
Key Optimization Tips:
– Adopt Instantiation: Frequent usage of ngOnDestroy lifecycle hook and ngOnInit permits explicit instantiation.
xxxxxxxxxx
ngOnDestroy() { }
ngOnInit() { this.loadData(); }
– Router Usage: Utilize onSameUrlNavigation configuration property within RouterModule.forRoot function, set to ‘reload.’
xxxxxxxxxx
RouterModule.forRoot(routes, { onSameUrlNavigation: ‘reload’ })
– Route Reload Function Utilization: The Route Reuse Strategy, though less recommended, offers another way to force routes to reload every time they are navigated.
xxxxxxxxxx
customRouteReuseStrategy.ts
shouldReuseRoute(future: ActivatedRouteSnapshot, current: ActivatedRouteSnapshot) {
return future.routeConfig === current.routeConfig;
}
app.module.ts
providers: [{
provide: RouteReuseStrategy,
useClass: CustomReuseStrategy
}]
While opting for the proper way to implement a page reload in Angular 8, developers must balance performance alongside usability. It is essential to remember the SPA concept guiding Angular’s operations – seeking ways to display new data instead of reloading entire webpages.
As Edsger W. Dijkstra famously said, “Simplicity is a prerequisite for reliability.” Demonstrating Angular 8’s capacity to accommodate a specific feature like ‘Reload a Page,’ speaking volumes about its flexibility, convenience, and capacity to adhere to this guiding principle.
For a closer look into Angular 8’s dynamics, Angular Official Documentation offers definitive tutorials and guides for diverse functions like creating components, organizing code, or insightfully diving into SPA interactions.