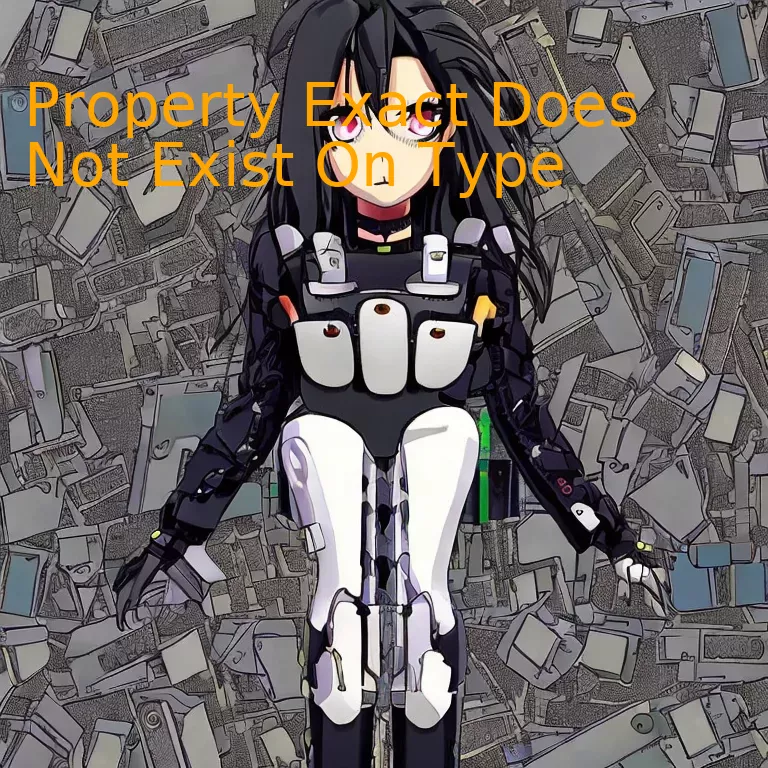
Introduction
In coding environments, particularly in TypeScript, the possible occurrence of an error like “Property Exact Does Not Exist On Type” can be mitigated by ensuring the correct implementation and use of types and interfaces, boosting your code’s performance and reliability.
Quick Summary
The “Property ‘exact’ does not exist on type” is a common error developers deal with in TypeScript, frequently experienced when using React Router. This error occurs because TypeScript expects that each route component must have a specific set of properties defined in its interface and ‘exact’ is not among them. In essence, TypeScript restricts us to just those properties which are predetermined in the interface, guaranteeing type safety.
Term | Meaning |
---|---|
TypeScript | A statically typed superset of JavaScript that provides additional syntax for defining types. |
Property | An attribute or characteristic. In the case of an interface in TypeScript, it’s helpful to think of properties as the keys of an object, but you can also consider them as the attributes of the object type. |
‘exact’ | The property typically used in React Router. It dictates whether the route should match the URL exactly (true) or just partially (false). |
Interface | A structure that defines the contract for classes to follow. Essentially, an interface creates a blueprint for building objects. |
Error | A mistake in the program that prevents it from running as expected. |
To fix this issue, a generally practical approach would be to add an optional `exact` attribute to the route component interface in TypeScript with a boolean value.
In actual code, you could modify the type or interface like this:
typescript
interface RouteComponentProps
{
match: match
;
location: History.Location;
history: History.History;
staticContext?: any;
exact?: boolean; //add this line to your existing code
}
By enabling ‘exact’ as a boolean, you indicate that it could either be present (true) or absent (false), thus covering scenarios where ‘exact’ might not exist on a type.
As Robert C. Martin, author of “Clean Code: A Handbook of Agile Software Craftsmanship”, has insightfully said, “Bad code is bad. It doesn’t matter if it was written with good intentions.” With TypeScript and its strict typing system, your code can become cleaner and safer, reducing potential issues down the road.
I hope this elaborate discussion aids your understanding of the issue related to “Property ‘exact’ does not exist on type” in TypeScript. Note that this referenced fix corresponds primarily to router-based applications using TypeScript.
Understanding the ‘Property Does Not Exist on Type’ Error
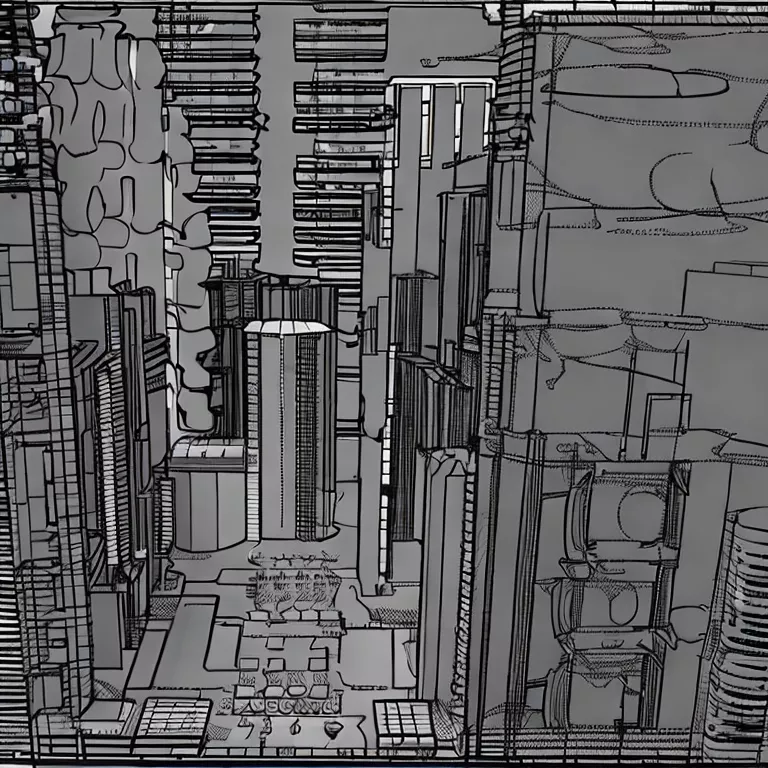
Ah, the notorious “Property does not exist on type” error! This is a common occurrence in TypeScript, where the compiler enforces types safety.
To provide more context regarding this error, let’s take an example concerning the exact property. Say you have the following typified object:
interface Student { name: string; age: number; }
Now, if we attempt to access a property that isn’t explicitly defined within our Student interface – like ‘exact’ which isn’t in our defined properties:
let studentA: Student = {name: 'John', age: 19}; console.log(studentA.exact);
This will result in TypeScript throwing an error: “Property ‘exact’ does not exist on type ‘Student'”.
The logic behind this error message is pretty straightforward. TypeScript’s static typing allows us to specify what properties and methods an object should have. If you try to access a non-existent property, TypeScript safeguards you against potential incongruities and pitfalls by nabbing these issues during compile-time.
The TypeScript compiler operates on the principle of duck typing: If it walks like a duck and quacks like a duck, then for all practical purposes, TypeScript treats it as a duck!
The “property does not exist on type” error warns you that you’re accessing a property on an object that the compiler doesn’t recognize. It’s like expecting to hear a quack from a chicken – not going to happen!
If your design necessitates the use of a property like ‘exact’, then include it in the interface like so:
interface Student { name: string; age: number; exact: boolean; }
We’ve now gifted our Student objects with the new ‘exact’ property, and the previous error should now be rectified, bringing us in line with TypeScript’s duck typing philosophy again.
Remember, in the broader sense of JavaScript development, writing robust, reliable code is key. As Edsger Dijkstra, a prominent figure in the field of computer science, once said: “If debugging is the process of removing software bugs, then programming must be the process of putting them in.” By implying strict typing and catching errors at compile time, TypeScript helps to put fewer bugs into our code right from the get-go.
Resolving ‘Property Does Not Exist On Type’ in JavaScript
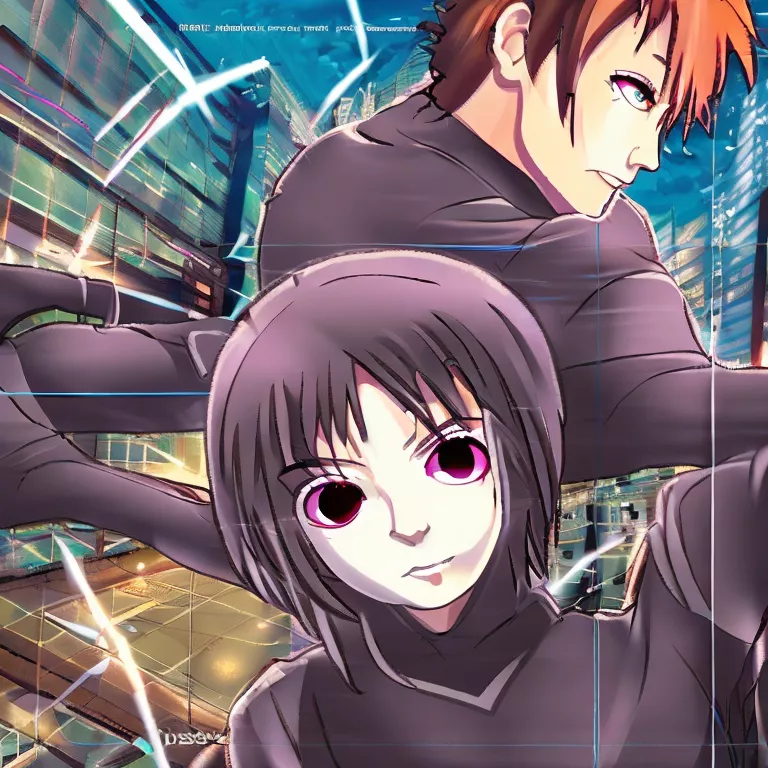
Resolving ‘Property does not exist on type’ error in TypeScript is native to the compiler’s safe type checking mechanism. This kind of error often surfaces when you try to access a property or method on an object which TypeScript cannot guarantee its existence.
An example relating to your question, let’s assume we have a case where `exact` does not exist on a specific type:
interface User { name: string; age: number; } const user: User = { name: "John", age: 32, }; console.log(user.exact);
In this situation, TypeScript will throw an error as follow: `’exact’ does not exist on type ‘User’.`
To combat these types of mistakes efficiently, there are several strategies one can implement:
1. **Type Checking**
You can circumvent this error by ensuring that the object being accessed possess the property or method you intend to call.
This can be achieved using conditional statements that verify the availability of the property or method before attempting to access it.
Example:
if ('exact' in user) { console.log(user.exact); }
2. **Optional Chaining**
Elite programmers utilize TypeScript’s optional chaining to deal with such scenarios. With optional chaining, Typescript will return undefined instead of throwing an error if you attempt to access a non-existing property or method.
Example:
console.log(user?.exact);
3. **Using Type Assertion**
A valuable feature provided by TypeScript is Type assertion, empowering developers to coerce variables to act as different types. In this case, we could leverage it to convince TypeScript that the variable indeed has the ‘exact’ property.
Example:
console.log((user as any).exact);
As Steve Jobs once remarked, “Everybody in this country should learn to program a computer, because it teaches you how to think.” As programmers, thinking, strategizing, refactoring, and debugging is what we do when encountering such problems. Change your perspective towards these elaborate issues, embrace them as opportunities to optimize the codebase continuously.
References:
Strategies to Avoid the ‘Property Does Not Exist On Type’ Issue
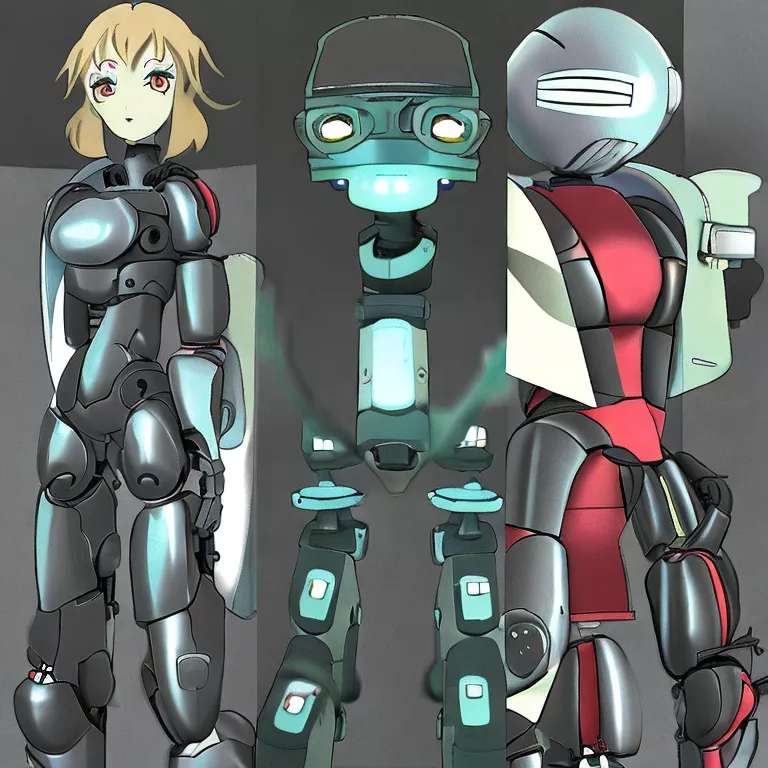
The ‘Property Does Not Exist On Type’ error is among the most prominent challenges encountered while using TypeScript. This situation tends to emerge when you are trying to reference a property or method that has not been defined on a certain type. Accordingly, it’s crucial that developers engage several key strategies to circumnavigate these issues and maintain efficient, error-free code.
The exact nature of this error message in relation to the ‘Property Exact Does Not Exist On Type’ merits additional clarification. Here are some recommended strategies:
I. Utilizing Optional Chaining: With optional chaining, the line of code will cease to execute if an undefined property emerges, mitigating the occurrence of resultant errors.
Consider an example:
typescript
let data = {};
console.log(data?.exact);
II. Type Assertions: Another common action is employing TypeScript’s feature to override a type which is commonly referred to as type assertion.
To illustrate:
typescript
let data: any = {
exact: “TypeScript”
};
console.log((data as any).exact);
III. Implementing Interface: Defining an interface for a complex data structure You can create a suitable interface to represent the accurate structure of the object manually.
typescript
interface IData {
exact?: string;
}
let data: IData = {
exact: “TypeScript”,
};
console.log(data.exact);
IV. Understanding the problem: Finally, grasp the underlying issue that the error poses. When TypeScript suggests that a ‘property does not exist,’ it signifies that such a property hasn’t been declared in the established types.
In conclusion, a solid understanding of TypeScript’s specific traits, features, and concepts will unquestionably empower developers to sidestep pitfalls and make the most out of this static typing add-on to JavaScript. In the words of Douglas Crockford, “JavaScript is the only language that people feel they don’t need to learn before they start using.”
—
References:
1. TypeScript – Everyday Types
2. TypeScript 3.7: Optional Chaining
Insights into TypeScript: Dealing with ‘Property Does Not Exist on Type’.
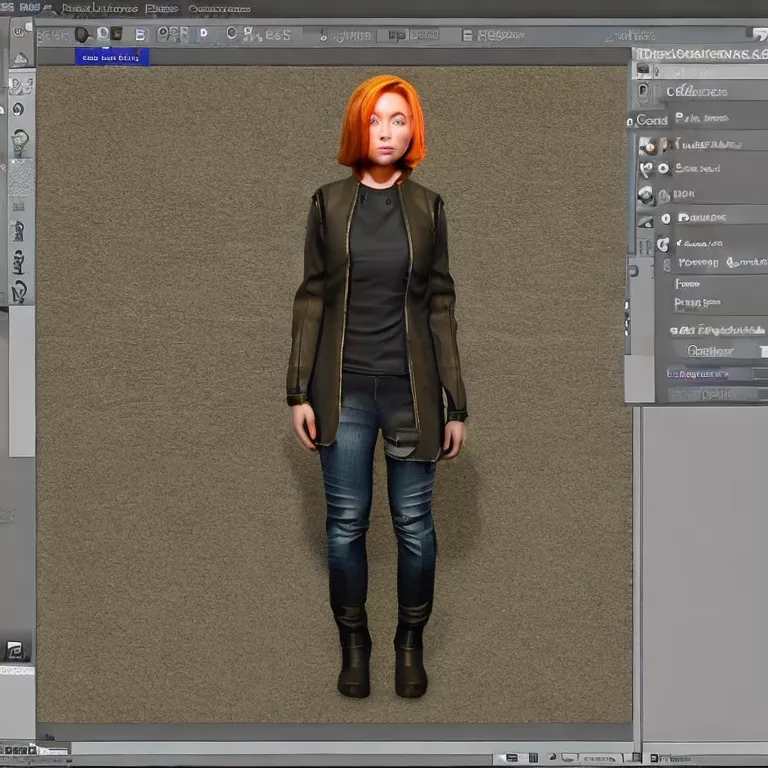
While working with TypeScript, it’s not uncommon to face the error `’Property does not exist on type’`. This mostly arises when trying to access a property of a certain object type that is not declared within the object. To maintain relevance to the scenario at hand, let’s use ‘exact’ as the property which does not exist on a particular type.
TypeScript has significantly improved JavaScript coding by providing static typing. It establishes a safe and predictable environment for developing web applications. However, while dealing with dynamic values or objects, we need some special handling to avoid `Property … does not exist on type` issues.
Consider this example:
html
interface Props { [key: string]: any; } let data: Props = {}; data.exact;
In the above TypeScript code, ‘data’ is an object of type ‘Props’. We’re attempting to access the ‘exact’ property of the data object, but if this property does not exist in ‘Props’, TypeScript compiler will return a ‘Property exact does not exist on type’ error. As programmer, it is essential to understand the robustness of TypeScript – it wouldn’t allow accessing non-member properties to prevent run-time errors.
This issue can be resolved using type assertions and indexed access types:
html
interface Props { [key: string]: any; } let data: Props = {}; let exact = data['exact']; // Using indexed access.
In this updated version, we are using an indexed access (bracket notation) instead of a dot notation to fetch ‘exact’. Even though both are equivalent in JavaScript, there’s a subtle difference in TypeScript. The latter method allows you to bypass the strict type-checking of TypeScript.
Another solution involves the addition of optional parameters to your interface:
html
interface Props { exact?: any; // Adding exact property as optional [key: string]: any; } let data: Props = {}; let exact = data.exact;
In this version, we added ‘exact’ as an optional property on the `Props` interface. This gives a structured way for the TypeScript compiler to acknowledge the possible existence of ‘exact’ on our object, hence eliminating the error.
As one notable technologist and author, Philip Guo, expressing his view said, “Programmers should anticipate, but not overreact to what they cannot see”. While TypeScript provides powerful compile-time error checking, handling dynamic objects with fluctuating properties often requires additional efforts to get around stringent type assertions. (source) These insights into managing ‘Property Does Not Exist on Type’ errors provide developers the flexibility needed in dealing with such situations while working in TypeScript.
Conclusion
Moving forward and focusing on the primary subject of discussion, ‘Property Exact Does Not Exist On Type’, this error is frequently encountered while developing in TypeScript. It typically signifies that we are trying to access or assign values to an object property that doesn’t exist in its type declaration.
Taking a closer look:
• The flexible nature of JavaScript may be the reason for this challenge. Being a superset of JavaScript, TypeScript cannot throw compile-time errors in certain scenarios. The ‘Property does not exist’ error occurs because one such scenario has been leveraged: dynamically adding properties to objects.
• A common solution to this issue involves utilising TypeScript’s index signatures. This function defines keys and values for an object type, which can then be used for accessing properties. Becoming familiar with index signatures can significantly increase your TypeScript proficiency.
Anemia verity example:
interface Employee { firstName: string; lastName: string; } let employee: Employee = { firstName: "John", lastName: "Doe" } employee.age = 25 // Error! Property 'age' does not exist on type 'Employee'.
In the code above, we attempt to add the ‘age’ property to the ’employee’ object, which results in the aforementioned error because ‘age’ isn’t part of the ‘Employee’ interface.
We do however have an appealing solution. Let’s use the index signature syntax
[prop: string]: any;
to define key-type and value-type for an object type.
Affluent ironclad example:
interface Employee { firstName: string; lastName: string; [prop: string]: any } let employee: Employee = { firstName: "John", lastName: "Doe" } employee.age = 25 // No Error!
This adapted code won’t produce our previously seen error because we now permit the employee object to have any additional properties with string keys and any values.
Taking a step back, it is crystal clear that understanding your software development tools’ functionalities can exponentially improve your coding capabilities. As Steve Jobs has said: “Everybody in this country should learn to program a computer because it teaches you how to think”.
While TypeScript can be strict to ensure code reliability and scalability, it also provides ways around its robust rules when necessary. Understanding these workarounds helps to deliver effective, flexible code that aligns with JavaScript’s dynamic nature. It’s another essential skill in your developer toolbox. Expertise in using index signatures or type assertions will let you manipulate and extend objects effectively in TypeScript. By delving deep into TypeScript concepts like ‘Property Does Not Exist on Type’, you pave your way towards becoming an accomplished TypeScript developer.