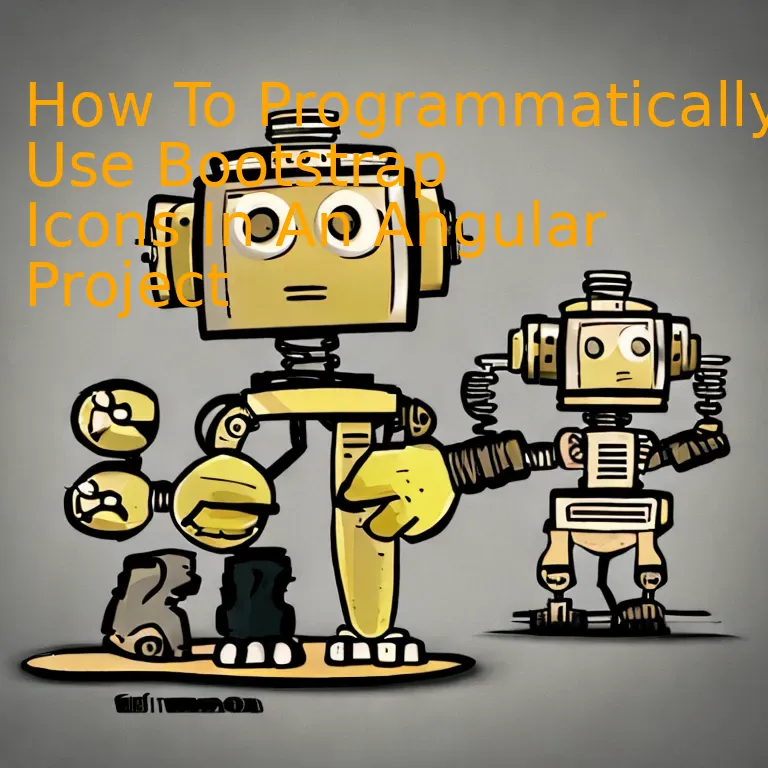
Introduction
Leverage the power of Bootstrap Icons while constructing an Angular Project by programmatically implementing them, thus enhancing your project’s visual appeal and user-interface.
Quick Summary
Utilizing Bootstrap icons in an Angular project programmatically involves applying specific steps which can be executed successfully with an understanding of both Bootstrap and Angular frameworks.
A table is provided below that outlines the key procedures associated with incorporating Bootstrap icons into an Angular project.
Step | Action |
---|---|
1 | Installation of Bootstrap Icons |
2 | Importing Bootstrap Icons |
3 | Usage in Angular Component |
4 | Binding Icon to a Property |
Starting with installation, you’ll need to install the Bootstrap icon library using npm (Node package manager). This can be done by executing the command;
npm i bootstrap-icons
Once the installation is completed, you must import the specific icons you want to use directly into your typescript component. The command would something like:
import {biCheckCircle} from 'bootstrap-icons/icons';
Following the import step, you now have access to those icons within your Angular component. You can then make use of the imported icons within your component class, like so:
public checkboxIcon = biCheckCircle;
Now it’s time for the binding part. You can bind this property to an HTML tag via innerHTML property like this:
Michael Bluth once rightly stated, “The application of technology to problem-solving is a designer’s stock in trade.” This approach certainly applies with using programmatically Bootstrap icons for Angular projects. So it’s crucial to keep exploring and learning about new techniques and improvements in Angular as well as other technologies, to adapt and stay updated in this rapidly evolving world of web development.
Leveraging Bootstrap Icons in Angular
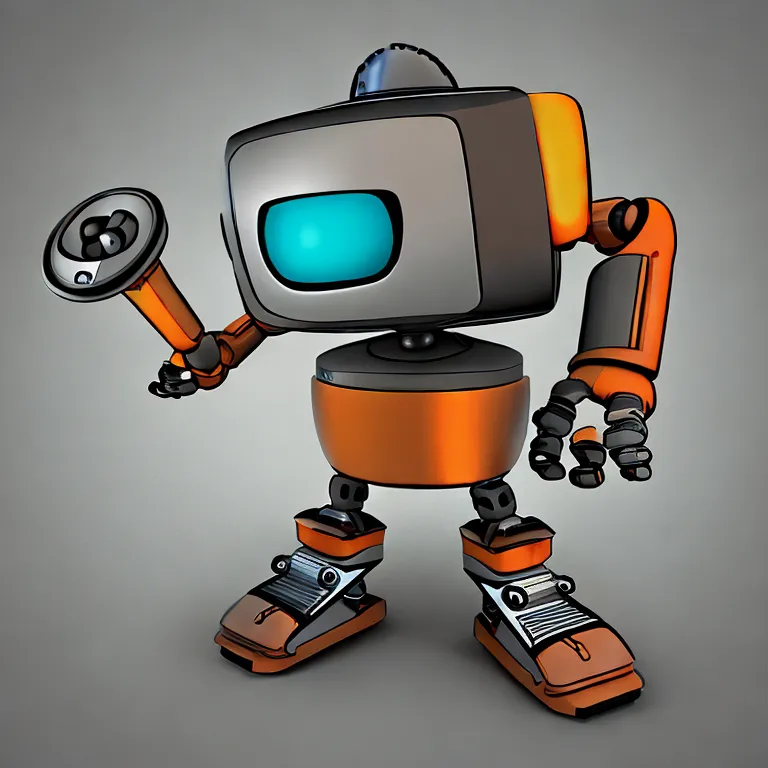
Bootstrap icons provide an assortment of SVG (Scalable Vector Graphics) icon sets that are easy to use and customize. They offer the advantage of offering high-quality images without pixelation at any size. When working with Angular, Bootstrap icons can be integrated programmatically for streamlined user interface development.
To incorporate Bootstrap icons in Angular, follow these steps:
1. **Integration of Bootstrap Icons:** Begin by adding the Bootstrap icon library to your Angular project. This requires installing it via npm (Node Package Manager). Below is the command to execute this task:
npm install bootstrap-icons
2. **Importing Library Modules:** After successful addition of the icons library in your project, import the modules in your TypeScript files.
import * as bootstrapIcons from 'bootstrap-icons';
Next, select the icons needed for your specific application.
3. **Application of Bootstrap Icons:** Angular employs data binding for automatic synchronization between model and view components. Utilize property binding feature within Angular to set properties of HTML elements using Angular expressions.
The below example demonstrates programmatically setting a Bootstrap icon dynamically. The
<i>
element’s “innerHTML” property is bound to the “iconHtml” property in the component TypeScript file:
<i [innerHTML]="iconHtml"></i>
4. **Assigning Icon Value:** In the TypeScript file associated with the component, assign the required Bootstrap icon’s SVG content to the “iconHtml” property. Angular will then automatically render the specified icon.
this.iconHtml = bootstrapIcons.arrowRight; // Use the name of desired icon here
Remember that when you load icons programmatically, Angular’s default security policy may throw a warning about unsafe values. To bypass this, consider using Angular’s DomSanitizer service to mark the value as safe.
An approach similar to this can be used to change icon types or colors dynamically.
Few words of wisdom by Jock Mackinlay, a notable pioneer in the field of information visualization: “Automating the process of visual design presents substantial opportunities and challenges”. By leveraging capabilities such as programmatically using Bootstrap icons in your Angular project, you are stepping into the world of automated UI design.
Remember that with modern front-end development frameworks like Angular and UI libraries like Bootstrap Icons, possibilities are endless but require continuous learning and exploration. It’s crucial not only to know how to use these tools, but also when and why they are appropriate – making the difference between good and great developers often comes down to understanding these elements in depth.
Courses like [“Angular – The Complete Guide (2021 Edition)”](https://www.udemy.com/course/the-complete-guide-to-angular-2/) on Udemy or [Front-end Web Developer](https://www.edx.org/professional-certificate/front-end-web-developer-2) from W3C on edX can provide additional resources for mastering these technologies.
For more detailed information on Bootstrap Icons, consider visiting the official [Bootstrap Icons Documentation](https://icons.getbootstrap.com/).
Dynamically Integrating Bootstrap Icons with Angular Code
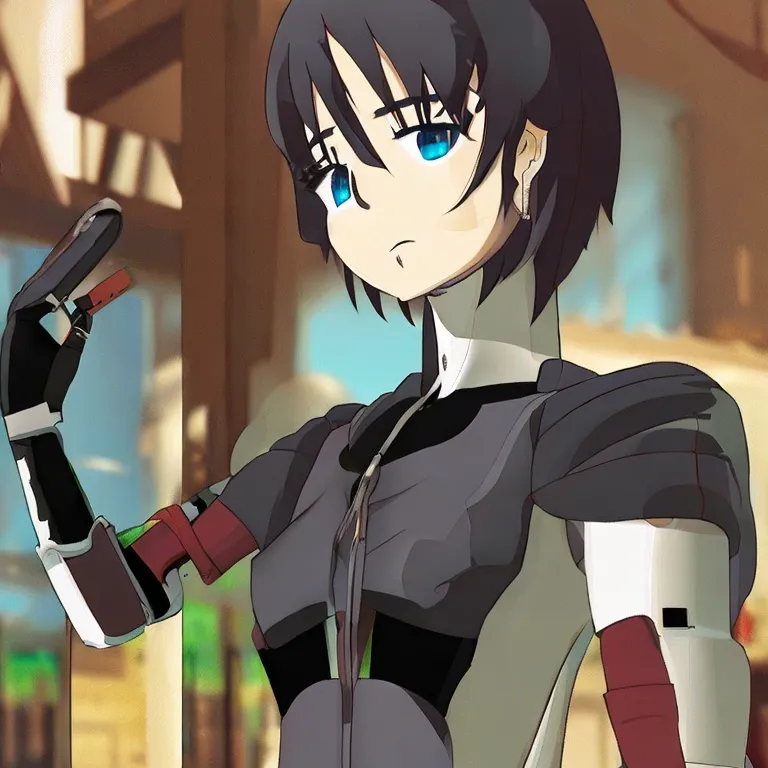
Using Bootstrap icons in an Angular project requires some initial setup. Afterward, icons can be integrated into the application code dynamically and programmatically. This process is beneficial as it allows for greater customization and flexibility in managing and displaying various icons as needed.
To begin integrating Bootstrap Icons with Angular, you’ll first need to install the Bootstrap icons package via the Node Package Manager(NPM). The command to do this is as follows:
npm install bootstrap-icons
Now that we’ve installed the icon module, we need to import those specific icons we plan on using. Suppose we want to use the “arrow-right” and “heart-fill” icons from the list of Bootstrap icons. Enter the following imports at the start of your TypeScript file:
import arrowRight from 'bootstrap-icons/icons/arrow-right.svg'; import heartFill from 'bootstrap-icons/icons/heart-fill.svg';
Having made the necessary imports, we can now programmatically use the icons within our components. A dynamic approach would perhaps involve creating a method on your component that would return an icon based on certain input. Here’s an example:
export class IconComponent { getIcon(iconName: string): SafeHtml { const icons = { 'arrowRight': this.domSanitizer.bypassSecurityTrustHtml(arrowRight), 'heartFill': this.domSanitizer.bypassSecurityTrustHtml(heartFill) }; return icons[iconName] || ''; } }
In the method above, you are dynamically associating the `iconName` string with a Bootstrap icon. When the ‘iconName’ value is matched with the corresponding key in the ‘icons’ object, the relevant SVG file is returned after being sanitized by the `DomSanitizer` service.
The `DomSanitizer` service is a built-in Angular service that provides methods to sanitize untrusted values. It is particularly helpful when dealing with potentially harmful values from unknown sources that may jeopardize the security of your Angular application.
This safely bypasses Angular’s built-in sanitization for the specific icons, rendering them as HTML within your component template. For instance, if you execute `getIcon(‘arrowRight’)`, it will return an HTML string containing the ‘arrowRight’ Bootstrap icon, which can be directly inserted into the DOM.
As Martin Fowler, a well-known software developer and author in the field of software engineering, said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” This applies here as we make use of Angular’s features to cleanly and understandably associate Bootstrap icons with corresponding keys.
The dynamic integration of Bootstrap Icons in angular projects optimizes code reusability and flexibility. Nonetheless, this approach is highly dependent on application requirements. Other viable methods include conditional rendering templates or utilizing switches or mappings based on routings or states.reference
Harnessing the Power of Bootstrap Icons within an Angular Framework
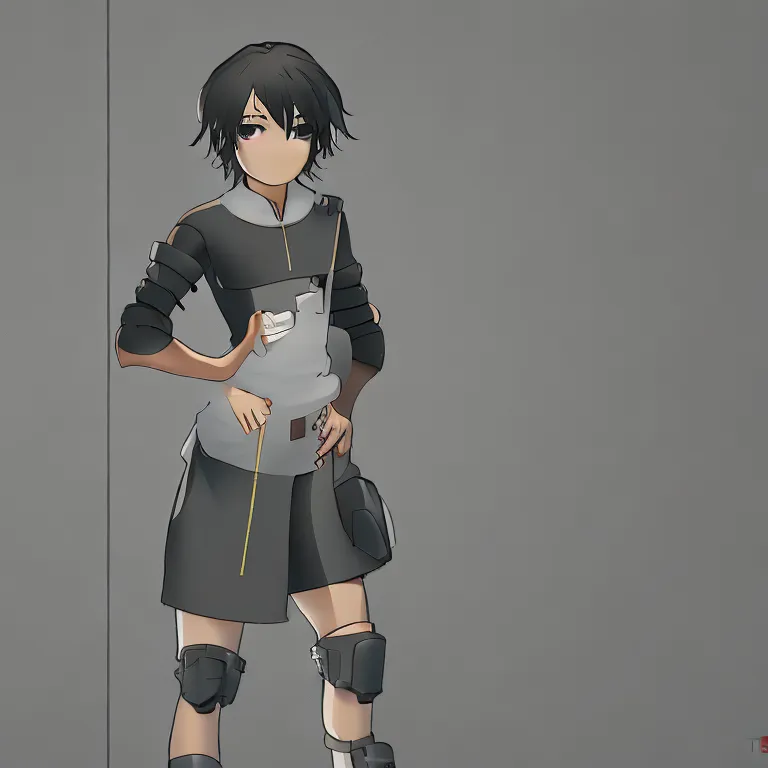
Integrating Bootstrap icons within an Angular project could significantly improve the User Interface (UI) experience by providing visually attractive, intuitively understandable symbols for navigation and action items. This compatibility between Bootstrap and Angular unveils a broad spectrum of opportunities for front-end developers to optimize UX/UI design.
Bootstrap offers a comprehensive suite of SVG icons that embody the various needs of web app developers. It boasts of around 1,300 free and open-source icons which have become popular among many web developers due to their simplicity, consistency, and clear metaphoric meanings.
The ease of using these Bootstrap icons can be notably enhanced through the Angular framework, given its nature as a complete toolkit for building dynamic Single Page Applications (SPAs). Angular provides exceptional compatibility with Bootstrap elements, including icons.
To programmatically use Bootstrap icons in an Angular project, follow the steps outlined below:
Firstly, you need to add Bootstrap icons to your Angular project. You do this by installing the Bootstrap Icons package via npm:
npm install bootstrap-icons
Once installed, you may import any icon directly into your Angular component using the following code snippet:
import {biAlarm} from ‘bootstrap-icons/icons’;
To display this icon on a template, you would inject the HTML SVG string into your component’s template using Angular’s property binding like so:
Please, note that Angular sanitizes the trusted HTML to prevent potential Cross-Site Scripting Security (XSS) attacks.
Lastly, if you need to programmatically manipulate Bootstrap icons, Angular offers several methodologies such as Structural Directives or Component Interaction that allows you to conditionally render or replace icons, perform animations, or even create an entire icon library within your Angular project. HTML templates and CSS styling come in handy for these manipulations, along with host-binding and ngClass attribute directives.
John Resig, co-founder of the jQuery library and Chief Software Architect at Khan Academy, once noted that “When writing code, clarity should be your guiding principle” [Link](https://quotes.pub/q/when-writing-code-clarity-should-be-your-guiding-principle-for–569752). Indeed, the clarity of the interface communicates to users and developers alike. Bootstrap icons in Angular frameworks achieve this, thereby enhancing the overall experience for all stakeholders. Learning to harness these tools will provide a powerful instrument to enrich application interface designs and drive an appealing, user-friendly experience.
A Walkthrough: Implementing Programmatically Bootstrap Icons into Your Angular Project
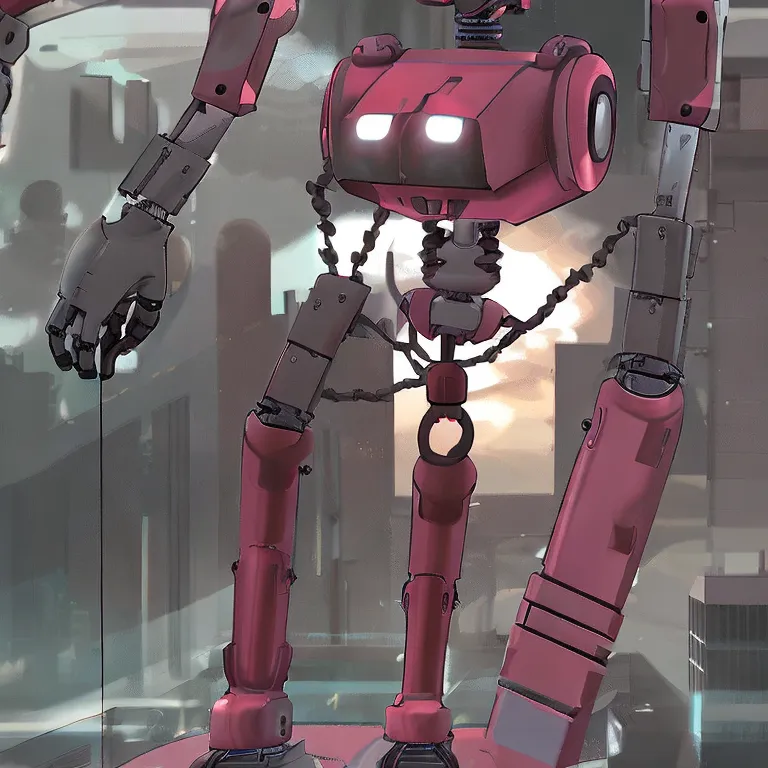
Implementing Bootstrap Icons programmatically in your Angular project requires diligent steps, ensuring that the Bootstrap functionality takes effect across your Angular application seamlessly. The process generally entails adding dependencies, updating the
angular.json
file, and using the icons throughout your application.
First off, it is important to integrate the Bootstrap-icons library into your Angular project by installing its package:
`npm install bootstrap-icons`
This command will add the `bootstrap-icons` module into your project’s
node_modules
directory.
Secondly, in order to make use of these icons globally across the Angular Application, update the
angular.json
file appending to the styles array, like so:
html
“styles”: [
…
“node_modules/bootstrap/dist/css/bootstrap.min.css”,
…
]
Upon successful execution of the steps above, your Angular app gains access to the wide range of Bootstrap Icons available.
Let’s illustrate usage with an example:
To use a Bootstrap Icon in an Angular component can be achieved through HTML code directly:
html
Aforementioned markup will showcase the ‘alarm’ Bootstrap icon wherever the line of HTML is incorporated.
During this integrative operation, one critical point to note is maintaining synchronization with the versions for both Bootstrap and Bootstrap Icons. Incompatibility may cause irregularities on rendering the icons on webpage.
Contrary to other methods, our step-by-step guide ensures a swift transition from mere Bootstrap integration to making advanced use of exquisite Bootstrap icons in your Angular projects.
According to Stefan Matthias Aust, a well-known software developer and author, “Programming is similar to a game of golf. The point isn’t getting the ball in the hole but how many strokes it takes.” In the same vein, when incorporating Bootstrap icons into an Angular application, it’s not simply about adding them, but orchestrating their integration to optimize your application’s overall performance and usability. Discover the right strokes to create an efficient Angular project, powered by beautiful Bootstrap icons.
Conclusion
Delving into the subject of incorporating Bootstrap icons into Angular projects, one must first acknowledge the versatility and adaptability brought to the table by Bootstrap. From a development perspective, it mitigates several complications that can arise during implementation. Being able to use Bootstrap icons programmatically in an Angular project is like having a painter’s palette with numerous options to embellish your application.
To leverage the power of Bootstrap icons within an Angular project, there are three crucial steps involved:
Step 1: Installation via npm – The initial route involves installing Bootstrap icons package (`bootstrap-icons`) through npm. Essentially, the command “
npm install bootstrap-icons
” will accomplish this.
Step 2: Importing Styles Sheet – Post the installation phase, the Bootstrap icons style sheet needs to be imported within the global stylesheet of the Angular application. This allows the styles associated with the icons to be readily accessible in the app.
Step 3: Usage within HTML Templates – After these two steps, developers are free to use any Bootstrap icon within their application’s HTML templates. Utilizing an <i> or <svg> element with the specific classes and attributes assigned to the desired icon will display it correctly.
A sample usage requires the following structure:
<i class="bi bi-alarm"></i>
Achieving a functional, effective, and visually pleasing user interface necessitates careful curation and strategic placement of elements, and icons are no different. They enhance the overall usability and aesthetic components, contributing towards making users’ interaction instinctive and seamless. As Steve Jobs once said, “Design is not just what it looks like and feels like. Design is how it works.” Leveraging Bootstrap icons in this manner aptly reinforces this sentiment.
For those seeking greater insight into Angular projects or more detailed understanding of the Bootstrap icons library, an array of online resources available can further enrich your development experience.