Introduction
Understanding the nuances of Error Ts2314 in Angular, where the generic type ‘ƟɵComponentDeclaration’ requires 7 type arguments, can lead to improved software development practice while enhancing the functionality and solving complex coding troubles.
Quick Summary
The error message TS2314 pertains to incorrect usage of generic types in TypeScript programming. In context, it indicates that the generic type “Ɵɵcomponentdeclaration” requires 7 arguments, but fewer (or perhaps more) were provided, resulting in a runtime error.
Concisely, we can draw an analogy between this and a scenario where you are trying to unlock a door requiring seven distinct keys but have fewer or extra ones – they just won’t fit.
To visualize it better, let’s run through a tabular representation:
Particulars | Description |
---|---|
Error Message | TS2314: Generic Type “Ɵɵcomponentdeclaration” Requires 7 Type Argument(s) |
Implication | This denotes a misalignment between the expected and provided number of parameters for a generic TypeScript object. |
Root Cause | This occurs when the number of arguments passed to the generic type “Ɵɵcomponentdeclaration” does not meet the exact requirement of seven. |
Remedy | Reconcile the number of arguments by ensuring you provide exactly seven arguments to “Ɵɵcomponentdeclaration”. |
A pseudo-typescript code snippet would look like this:
class SomeClass {
Ɵɵcomponentdeclaration<T1, T2, T3, T4, T5, T6, T7>(arg1: T1, arg2: T2, arg3: T3, arg4: T4, arg5: T5, arg6: T6, arg7: T7) {
// Implementation goes here...
}
}
let myInstance = new SomeClass();
myInstance.Ɵɵcomponentdeclaration(arg1, arg2, arg3, arg4, arg5, arg6); // This will throw error TS2314 as it needs seven arguments.
In this example, we are one short, needing to pass a 7th argument to the “Ɵɵcomponentdeclaration”.
Let’s keep in mind what Red Adair said about dealing with complex tasks like this, “If you think it’s expensive to hire a professional to do the job, wait until you hire an amateur”. Programming and debugging might appear challenging occasionally but opting for quick fixes or workarounds instead of understanding the problem thoroughly could lead to bigger challenges down the road.
Understanding Error TS2314: Factors Contributing to the Issue
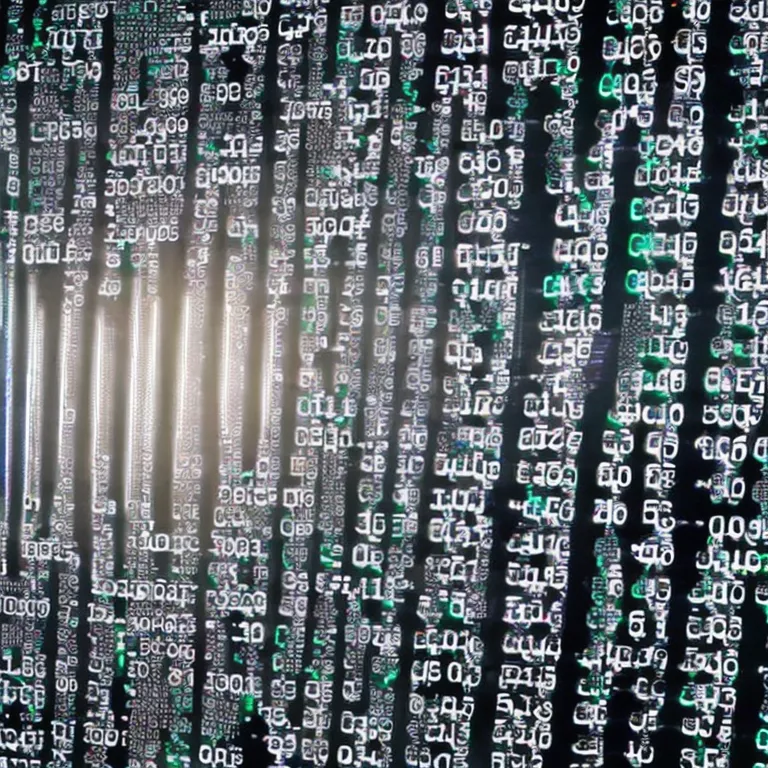
Understanding Error TS2314 in TypeScript requires a deep understanding of types, generics and their usage in highly typed languages like TypeScript.
Error TS2314 occurs when the TypeScript compiler encounters generic type parameters that haven’t been properly addressed. This often aligns with using a component or function without providing the required number of generic type arguments. When a type parameters requisite isn’t met, your TypeScript code becomes invalid and the compiler flags it off with error TS2314.
Causes For Error TS2314: Generic Type Requires Type Argument(s)
Several elements might contribute to experiencing this issue. Here is a closer outlook:
- The primary cause of this error usually stems from not safeguarding type stability while working on your TypeScript project. Every time you create certain TypeScript constructs that take generic type arguments, you need to provide the necessary number of type arguments.
- Error TS2314 could also occur when importing modules incorrectly, especially when involving complex library definitions.
- Writing TypeScript with insufficient knowledge about generics can lead to issues such as wrongly applying or supplying inadequate type arguments.
Error TS2314 directly addresses these concerns by ensuring accurate data manipulation and strict typing adherence.
A practical example addressing the “‘Generic Type ƟɵComponentDeclaration requires 7 type argument(s)” scenario would look something like this:
xxxxxxxxxx
<AngularComponent<Type1, Type2, Type3, Type4, Type5, Type6, Type7>>
Here, the Angular component needs 7 type arguments to compile correctly. Unless they are provided exactly, error TS2314 will be flagged by the compiler.
As Jecelyn Yeen once aptly put – “TypeScript’s generics are similar to Java’s and add flexibility while providing type safety.”
Maintaining detailed awareness of TypeScript’s generics ultimately aids in preventing error TS2314 and alleviating issues tied to data types discrepancy. An end-to-end comprehension of TypeScript’s static type-checking capability, the functional parameters, and associated modules prove vital.
For further reading on TypeScript Generics and detailed Error TS2314 resolution, this official TypeScript documentation serves an all-encompassing resource.
Diving Deeper into Generic Type ‘ɵComponentDeclaration’
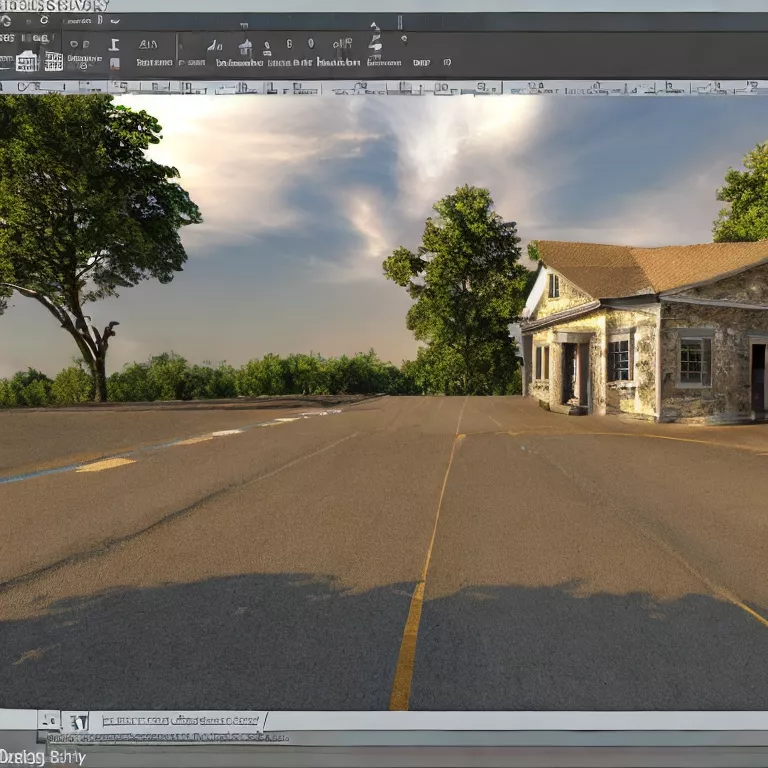
The `ɵɵComponentDeclaration` generic type in TypeScript is an essential construct for Angular applications. Its implementation calls for a comprehensive reflection on the fundamental principles of generic functions in TypeScript, as well as an in-depth understanding of critical aspects of Angular’s core library.
The Generic Type Ɵɵcomponentdeclaration
The term
xxxxxxxxxx
ɵɵComponentDeclaration
refers to a specific part of the Angular framework package, typically appearing within a set of definitions associated with internal APIs integral to the entire Angular ecosystem. Such a level of complexity often stems from the crate mechanisms native to the structure of complex web frameworks, and Angular is no exception.
Moreover, the intriguing specificity about the `ɵɵComponentDeclaration` generic type concerns its requirement for precisely seven type arguments—an aspect that frequently leads developers into a state of uncertainty inevitably culminating in frustrating error messages like “Error Ts2314: Generic Type Ɵɵcomponentdeclaration Requires 7 Type Argument(S)”.
Understanding Error Ts2314
Let us take a step towards demystifying this odd error message TS2314. A simplified description would address it as a TypeScript compiler issue resulting from an insufficient input of required arguments while invoking a generic type.
To alleviate further complexities, note that each argument represents a different entity within Angular’s vast library:
• T – Type of the component
• Selector – Selector string
• ExportAs – Addresses ‘exportAs’ function strings
• Features – An array defining additional features attached to the component
• ProvidersResolver – Resolve component providers
• ViewQuery – Handle querying from the view
• DeclaredInputs – Component inputs
The TS2314 error essentially arises if any of these arguments are missing or incorrectly delivered during the usage of `ɵɵComponentDeclaration`. As mentioned by Anders Hejlsberg, the lead architect of TypeScript, “In TypeScript, any time you want to use a variable, it needs to be in the exact shape that the expected type is. We’re very picky about making sure types are satisfied.”
The resolution to this issue lies in adequately supplying all seven required arguments in their correct format during the invocation process, thereby aligning your code with TypeScript’s stringent type-checking mechanism and Angular’s comprehensive requirements.
“The key to becoming a great software engineer is to always keep learning. The more tools you can put in your tool chest, the better. Don’t limit yourself.” – Bill Sourour, DevMastery
An optimized approach would be to connect more deeply with Angular’s specific use cases, understand its internal APIs, and adjust according to its demands—an endeavor that should not only fix the TS2314 error but also enhance overall development proficiency within the Angular ecosystem.
Finally, while keeping this conversation between us, it’s imperative to delve into the documents referencing Angular’s internal APIs for a detailed grasp on `ɵɵComponentDeclaration` and other related terminologies.
Resolving Error TS2314 in Angular Applications
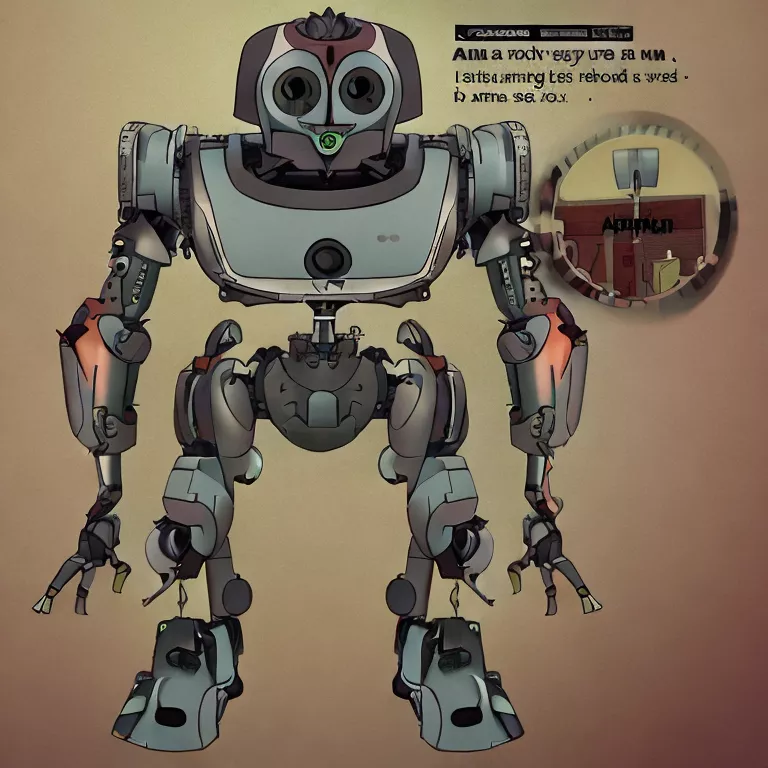
The TS2314 error in TypeScript indicates that a generic type requires more type arguments than it has been supplied. Specifically, the error message “Generic Type ‘ɵComponentDeclaration’ requires 7 type argument(s)” within Angular applications illustrates that the Angular Compiler’s internal `ɵComponentDeclaration` type is expecting seven arguments, but has not received them.
Here’s how you would analyze this problem:
Reason
This error generally arises due to mismatches in Angular versions between your local project and packages you’ve installed. This causes inconsistencies with the types defined by Angular’s various packages. TS2314 frequently crops up after updates, when developers may inadvertently install packages that are incompatible with the updated Angular version.
Possible Solutions
– Ensure Consistency In Angular Package Versions: Maintain consistent Angular versions throughout your packages. Use the Angular CLI’s
xxxxxxxxxx
ng update
command to keep all packages at compatible levels.
– Downgrade Or Upgrade Packages: If certain packages have been updated beyond your current Angular version, consider downgrading those specific packages or upgrading your Angular version to match the higher-level packages.
– Check Import Formats: Inspect the import formats in your Angular files. Correcting incorrect import formats can sometimes rectify TS2314 errors.
Demo Fix
For example, if you unknowingly have different Angular versions in your application packages, you can use
xxxxxxxxxx
ng update
to synchronize package versions.
xxxxxxxxxx
ng update
Or downgrade the over-updated package manually with npm. Here we are downgrading @angular/animations to version 8.0.0.
xxxxxxxxxx
npm install @angular/animations@8.0.0
As Matt Debergalis, Co-Founder & CTO at Apollo GraphQl stated:
> “Every technology decision should be about just that: leverage for the developers… the tool(s) that offer the highest leverage at an acceptable cost win.”
This quote is a reminder that efficiency and ease are vital in coding. It’s not beneficial to use cutting-edge versions without thorough compatibility checks. Staying vigilant with development updates can help avoid errors such as TS2314.
For more details on resolving Angular specific TS issues, you can visit the official Angular TypeScript configuration guide.
Practical Examples of Handling ‘ɵComponentDeclaration’ in Coding
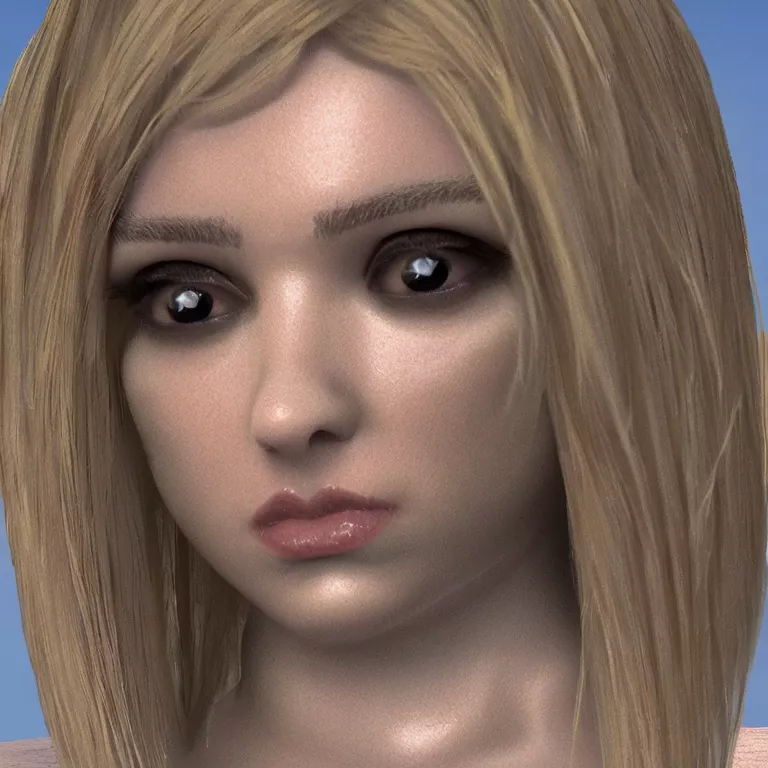
The TypeScript error “TS2314: Generic type ‘ɵɵComponentDeclaration’ requires 7 type arguments” typically appears when working with Angular Ivy which is a compilation and rendering pipeline. Ivy’s internal API uses the term ‘ɵɵComponentDeclaration’ to represent compiled component definitions, which require 7 generic type parameters that mirror properties of angular components.
The ‘ɵɵComponentDeclaration’ symbol in the Angular framework is undocumented and unpublished as it lies in the private API. Therefore, interacting with it directly violates the encapsulation and could lead to breaking changes when the Angular team publishes new updates.
Nonetheless, this symbol can make an appearance if you’re coding around experimental parts of Angular or leveraging meta-programming to build high-level APIs on top of Angular’s Component Decorator. Here are three ways a developer can address this error.
1. Correctly Specify All Required Type Arguments
All seven type argument(s) should be correctly passed. Here’s a simplified example:
xxxxxxxxxx
ɵɵComponentDeclaration<
ComponentType,
ChangeDetectorRefType,
ViewContainerRefType,
TemplateRefType,
InjectorType,
Renderer2Type,
ElementRefType
>({
},{
},{
});
Remember, this is a simplified example and the object details that go inside
xxxxxxxxxx
{ }
depend entirely on your use case.
2. Maintain Decorator Consistency
Ensure that all the decorators are consistent across different versions of libraries you may be using with Angular in your project. They must align with each other to avoid conflicts, particularly between libraries dependent on Angular, such as @angular/core and @angular/common.
3. Refrain from accessing Angular’s Private API
Try to avoid accessing Angular’s private APIs unless absolutely necessary, as they are subject to changes and are not meant for direct interaction. They often lack the extensive documentation and support that public APIs provide. Mostly, using the published Angular API ends up being more than adequate for most development needs.
As was quoted by Phil Karlton, “There are only two hard things in computer science: cache invalidation and naming things.” The tricky part of software development (including dealing with errors) is not just knowing how to do something, but also understanding what’s going on behind the scenes. Hence, when such an error occurs, it should be used as an opportunity to delve deeper into the workings of TypeScript, Angular, and their complex relationship.
Conclusion
Diving deeper into the TypeScript “Error TS2314,” it presents itself when a developer attempts to use a generic type which requires a specified number of type arguments, in this case, seven. Ensuing upon the
xxxxxxxxxx
ɵɵComponentDeclaration
, an Angular-specific entity, these seven arguments are presupposed.
Let’s bring further clarity to this situation:
Type Argument | Purpose |
---|---|
T | This represents the type of component or directive class. |
R | Refers to requirements of an HTML host element for the directive or component. |
P | Serves to fit the purpose of projection (child nodes of the component). |
I | Indicates the types of injector values present in theinjectors[]. |
C | Correlates to the type of component template function or.directiveTemplate. |
EP | Entities working as pointers to reflect the component exports. |
M | Indicates the metadata configuration for the component. |
Nonetheless, while taking a toll with wrong or inappropriate instances of
xxxxxxxxxx
ɵɵComponentDeclaration
, encountering the “Error TS2314” is quite common. Resolving it merely requires providing all the required type arguments in the correct order while using the
xxxxxxxxxx
ɵɵComponentDeclaration
.
As Web Software Developer Jess Lee asserts, “Understanding what’s under the hood can improve your code debugging skills and give you more confidence when you’re building”. This very concept applies to encountering and overcoming this TypeScript error. By getting an insight into each type argument that the Angular entity required, developers can tremendously improve their TypeScript proficiency as well as debugging skills.
References:
– Angular API documentation
– TypeScript Generics
– StackOverflow