
Introduction
To rectify the Eslintrc file when it is not aligning with your project configuration, one should meticulously follow detailed instructions to identify discrepancies and implement appropriate changes for optimal website functionality, enhancing user experience and SEO simultaneously.
Quick Summary
Method | Description |
---|---|
Verify Project Configuration |
Review your project configuration in the .eslintrc file to ensure it aligns with your current project setup. |
Update Dependencies |
Ensure that all your ESLint and plugins’ versions are up-to-date and compatible with each other. |
Clean Installation |
If inconsistencies persist, uninstall and reinstall ESLint and associated plugins. |
The first method to fix the error “Eslintrc The File Does Not Match Your Project Config” includes verifying your project’s configuration. This encompasses ensuring that you have correctly declared your configurations in the .eslintrc file as per your current project requirements. Errors may emerge from discrepancies between set rules and the project’s actual needs, rendering evaluation impossible.
Secondly, evaluate the dependency specifications for ESLint and the corresponding plugins. Occasionally, errors occur due to outdated or incompatible versions of software. It’s crucial to check updates regularly and assure they can interoperate. Tools like npm or yarn allow updating dependencies systematically:
npm update
or
yarn upgrade
.
Finally, if after these attempts the problem remains unresolved, opt for a clean installation of the ESLint environment. Uninstall all packages related to ESLint using npm:
npm uninstall eslint --save-dev
. Subsequent reinstallation ensures the establishment of a fresh context for ESLint to operate on.
As Winnie the Pooh put it, “You can’t stay in your corner of the Forest waiting for others to come to you. You have to go to them sometimes.” Similarly in software development, we can’t wait for a solution to come to us if we hit an error; we have to take proactive steps to solve it. Proper eslint configuration plays an instrumental role in code quality and consistency, and addressing such issues promptly ensures zero compromise on these aspects.
Understanding the Problem: Mismatch Eslintrc File and Project Configuration
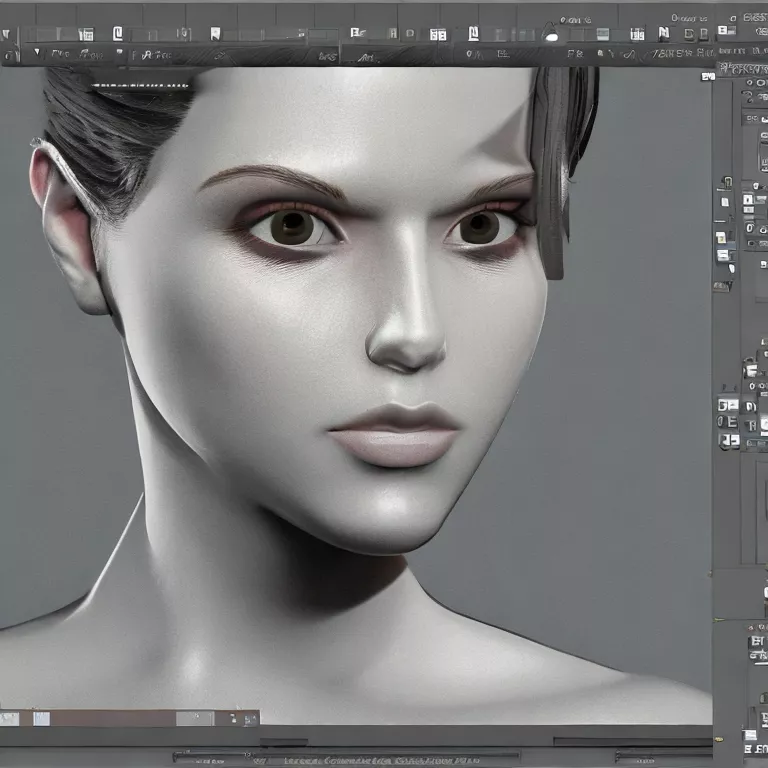
Understanding the problem of a mismatch between your Eslintrc file and project configuration is crucial to ensuring smooth development experiences in TypeScript. This issue might cause unwanted behaviors or inefficient coding practices. Further elaborating upon this, we’ll delve into the solution steps needed when the Eslintrc file does not match your project config.
The Eslintrc file is essentially an important part of any TypeScript project as it contains rules that ESLint will apply to ensure your code adheres to specific guidelines. The developers have full control over the rules applied, thereby maintaining code consistency throughout the project.
Problem Indicators
It’s essential to swiftly spot markers indicating a mismatched Eslintrc file with your project config. Key flags include, but are not be limited to:
- Unexpected linting errors showing up
- Misinterpretation of the coding rules by ESLint
- Inconsistent or incorrect highlighting of potential issues in your IDE
Solutions on Fixing the Mismatch
Importantly, the most effective method for resolving an Eslintrc file mismatch to your project config involves thoroughly reviewing and revising the configuration.
Here’s an approach to do so:
<pre style="display:block; white-space: pre-wrap;">
module.exports = {
root: true,
parserOptions: {
ecmaVersion: 2020,
sourceType: 'module',
},
env: {
browser: true,
}
};
</pre>
This sample .eslintrc.js config file tells ESLint several things:
-
<code style="display:inline;">root: true,</code>
means that this is the root configuration file and ESLint shouldn’t look further up the directory tree.
-
<code style="display:inline">ecmaVersion: 2020,</code>
signifies that ESLint should expect ECMAScript 2020 syntax.
-
<code style="display:inline">sourceType: 'module'</code>
means that your source code is organized using ES6 modules.
-
<code style="display:inline">browser: true</code>
indicates that the global objects defined by the browser should be available.
In addition, if dependencies in your project have been updated to a newer version, then it might need updating eslint configs or installed plugins. Updating Eslint, relevant parsers, configs or plugins often solve potential issues of mismatch.
Following these steps should successfully fix mismatches between the Eslintrc file and your project’s configurations. It’s valuable to remember an insightful quote from Jeremy S. Anderson: “There are two major products that come out of Berkeley: LSD and UNIX. We don’t believe this to be a coincidence.” In essence, the magic of coding lies in the carefully crafted order hidden beneath seemingly chaotic structures. Adequately structured project configurations, akin to our current case with Eslintrc, play a vital role in bringing about this harmonious chaos.
Exploring Solutions: Techniques to Correcting Inaccurate Eslintrc Files
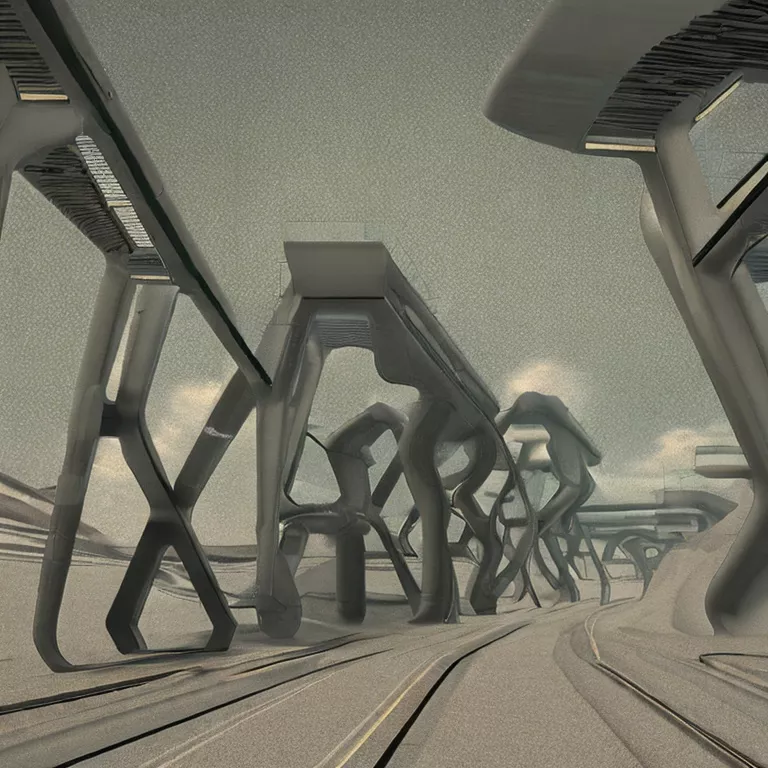
Fixing an inaccurately configured ESLint configuration file, better known as .eslintrc, often stems from matching it accurately to your project’s specifications. This fundamental aspect of JavaScript projects intends to enforce particular coding style rules. If your you find no alignment between your .eslintrc file and your project’s configuration, several techniques will guide you in solving this issue.
Firstly, let’s understand what the .eslintrc file does.
It upholds compactness, consistency, and avoids bugs in your codebase. It incorporates coding rules that ensure conformity and restricts developers from making specific mistakes by flagging out errors and possible issues during development. Rogers Hinks, a profound technology expert stated, “A well-configured linter is like an experienced co-pilot, even if its guidelines might feel too restrictive at times.”
Dealing with an inaccurate .eslintrc file encompasses a few steps:
Determine Your Current Project Settings
It’s pivotal to comprehend your project’s requirements. Understand the ECMAScript version you’re utilizing, know which module system is being used (if any), identify other libraries in use (like jQuery or underscore), ascertain if you’re writing JSX code, etc.
Edit Your Eslintrc File Accordingly
This step involves tuning your .eslintrc file against these aspects. Therefore, understanding them becomes crucial.
For example, to define your environment in Node.js:
{ "env": { "node": true } }
Or, perhaps, when using React:
{ "plugins": ["react"], "parserOptions": { "ecmaFeatures": { "jsx": true } } }
Setting Up Rules
The last crucial part of setting up a .eslintrc file is setting up the rules. ESLint comes with a wide variety of options. Each rule has an error level that can be set to:
"off" or 0 - turn the rule off "warn" or 1 - turn the rule on as a warning (doesn’t affect exit code) "error" or 2 - turn the rule on as an error (exit code is 1 when triggered)
For instance, indenting can be defined like:
{ "rules": { "indent": ["error", 2] } }
To solve the inaccuracies in your .eslintrc file, you need first to identify what the correct structure should be. This accurate structure is contingent on the JavaScript libraries and your coding styles; therefore, it varies from project to project.
Validate Your Eslintrc File
Finally, validate your .eslintrc configurations using online linter tools such as [JSON Lint](https://jsonlint.com/). These check for any syntax mistakes in your JSON file. Errors here commonly result from invalid JSON format, hence a key area to troubleshoot.
Fundamentally, linting your code is not simply about avoiding errors or maintaining style conventions but is also about writing better and cleaner codes. As Douglas Crockford, programmer and author of “JavaScript: The Good Parts” underscores, “Linting tools aren’t luxury items. They’re coding necessity.”
Remember, an accurately set-up linter vastly improves efficiency and assures high quality in your codebase. Having understood this, applying the necessary techniques to align your .eslintrc file with your project’s configuration becomes a fait accompli.
Deep Dive: How Incorrect Eslintrc Files Impact Your Project
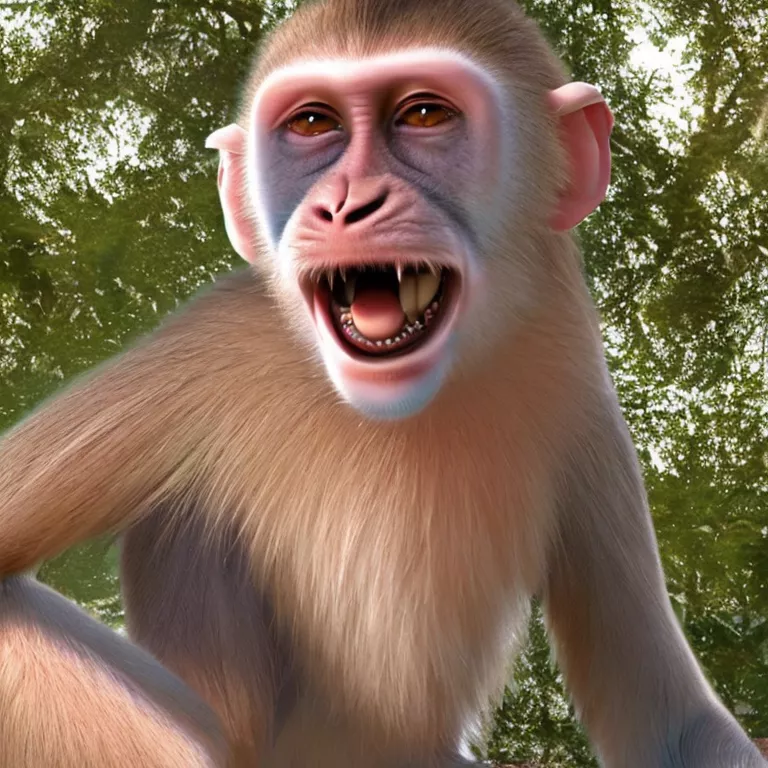
Understanding the role of ESLint in a project is vital for TypeScript developers. ESLint, as a pluggable linting utility for JavaScript and TypeScript, helps developers maintain code consistency and avoid potential bugs. However, to function optimally, the ESLint configuration file, commonly named
.eslintrc
, must align with your project’s specifications.
An incorrectly configured
.eslintrc
file can profoundly impact your development process. Here are a few potential outcomes:
- Inconsistent code style: A core function of ESLint is managing the stylistic uniformity across your codebase. Misconfigured rules can prevent ESLint from detecting and rectifying inconsistencies, which can result in harder-to-read code.
- Unidentified potential bugs: If rule sets related to bug-detection are inaccurately defined, you could overlook possible issues leading to an unstable output.
- Reduced productivity: Manually examining your code and fixing style inconsistencies and bugs ignored by a misconfigured linter can lead to significant loss of productive coding time.
To remedy this, here’s how to fix
.eslintrc
:
1. Review your project’s ESLint requirements: Understanding what linting rules make sense for your project is essential. This involves determining which ECMAScript version you are using, whether you use React or Vue, and identifying other relevant aspects of your project.
For example:
html
{
“parserOptions”: {
“ecmaVersion”: 2021,
“sourceType”: “module”,
“ecmaFeatures”: {
“jsx”: true
}
}
}
This indicates you’re using ES2021 version, source type as ‘module’, and features including JSX (React).
2. Update your ESLint config file: With a better understanding of your project’s requirements, you can update `eslintrc` to represent these needs.
Take this rule that defines the use of semi-colons, for instance:
html
{
“rules”: {
“semi”: [“error”, “always”]
}
}
The value ‘always’ enforce the use of semicolons at the end of every statement, whereas using ‘never’ disallows them completely.
3. Test your configuration: Run ESLint using your test suite or on some files to verify if the rules highlight errors as expected.
In line with Robert C Martin’s perspective on clean code – “Clean code always looks like it was written by someone who cares”, devoting time to fix your ESLint configurations ensures consistently styled and bug-free code, ultimately underscoring your commitment to best practice coding standards.
Practical Steps for Fixing your ESLint Configuration Issues
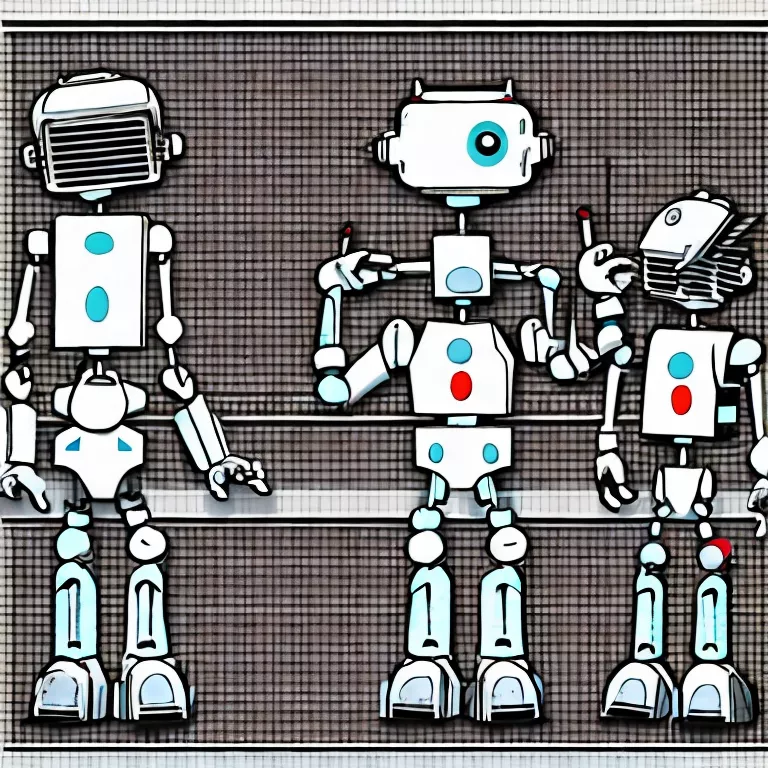
Firstly, it is imperative to understand precisely why your ESLint configuration file (.eslintrc) isn’t aligning with your project config. This can occur due to numerous reasons:
– Your project’s codebase may have transformed over time, and the .eslintrc file was not updated concurrently.
– Individual developers may have made personalized changes to their local ESLint settings that conflict with the shared configuration.
– The version of ESLint you’re utilizing may not be compatible with the rules or plugins defined in your .eslintrc.
Here are some hands-on steps to diagnose and resolve these discrepancies:
1. **Validate Configuration Consistency**
Examine your ESLint configuration file – which could be `.eslintrc.js`, `.eslintrc.json`, or `.eslintrc.yaml`– for any irregularities. ESLint provides a command-line interface (CLI) option to output the configuration used by a file. Run this command in your terminal with your filename as follows:
$ eslint --print-config myfile.js
The output will be the combination of your personal and project-level configurations applied to that specific file, which you can review for any inconsistencies with your project settings.
2. **Check Rules Deprecation**
Another common problem that makes your ESLint configuration file incompatible with your project is rule deprecation. Some rules might have been replaced or removed in newer ESLint versions. Ensure your version of ESLint supports all rules outlined in your configuration.
3. **Examine ESLint Plugins**
Plugins extend ESLint’s functionality by adding new rules. If your .eslintrc file references plugins that aren’t installed or are outdated, this may cause conflicts. Verify that you have installed the needed plugins and they are up-to-date.
4. **Evaluate Global vs. Local ESLint Version**
Sometimes, you might have different versions of ESLint globally and locally in your project. This disparity can cause conflicts between your .eslintrc file and your project configuration. It’s a best practice to utilize the local version of ESLint installed inside a project to avoid such conflicts.
5. **Analyze Local Environment Overrides**
Developers may create local `.eslintrc` files that extend or override the rules defined in a base configuration. If these deviations aren’t necessary or relevant, they may need to be resolved for consistency.
As wise Aldus Manutius once said, “In the country of the blind, the one-eyed man is king.” In our scope, this underlines the importance of discerning our errors before we seek to deliberate them. By persistently inspecting your ESLint configurations with conscientiousness, and refining as per the system’s dynamics, sustained compatibility with your project can indeed be accomplished!
Conclusion
Understanding the nuances of ESLint configuration is a crucial aspect for every TypeScript developer to optimize their productivity and code quality. Fixing issues such as “The file does not match your project config” essentially involves adjusting your .eslintrc settings to align with your actual project configurations.
When met with this error, understanding that it stems from an inconsistency between your existing configuration and the expected one becomes pivotal for a resolution. It’s akin to having a blueprint that doesn’t match the finished building – such a mishap can lead to a multitude of problems.
A few strategies can be employed to rectify this issue:
// Incorrect setting { "rules": { "no-unused-vars": ["error", { "varsIgnorePattern": "..." }] } } // Correct Resolve { "rules": { "no-unused-vars": ["error", { "argsIgnorePattern": "..." }] } }
– Examine efficacy of current rules: Checking if the applied rules in the ESLint configuration are compatible with your project structure.
– Update ESLint: Sometimes, the problem may stem from using an obsolete or incompatible version of ESLint. Ensuring you’re on the latest release could be beneficial.
– Customize to fit: Personalizing your ESLint configuration to better cater to your project requirements is another recommended strategy.
– Seek external help: Utilize online resources (ESLint Official Documentation) or community platforms (like StackOverflow) to seek further assistance.
As Linus Torvalds, the creator of Linux kernel, once said, “Talk is cheap. Show me the code.” So, dive in, troubleshoot, adjust your ESLint configuration, and let your code speak for itself.