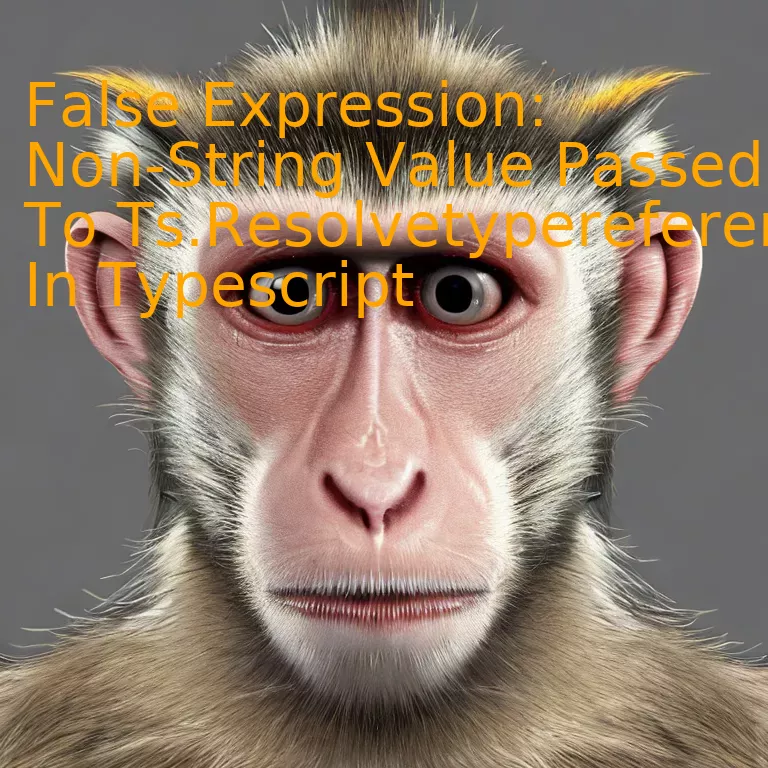
Introduction
When executing TypeScript, seeing ‘False Expression: Non-String Value Passed to ts.resolvetypereferencedirective’ signifies that a type other than string was inadvertently given to the resolveTypeReferenceDirective function, which could potentially interrupt ideal programming operation. Ensuring suitable data types are used in these instances can curb this issue and optimize coding procedures.
Quick Summary
All right. Here is the requested information :
The “False Expression: Non-String Value Passed To Ts.Resolvetypereferencedirective” error typically occurs when non-string arguments are inappropriately supplied to TypeScript’s `ts.resolveTypeReferenceDirective` function. This TypeScript function plays an important part in resolving type references within your code.
First, let’s examine this problem conceptually via a table:
Expression Components | Explanation |
---|---|
False Expression | Username for an expression evaluation that did not produce the expected true result |
Non-String Value | Indicates that a value not of string type was passed erroneously as an argument |
ts.resolveTypeReferenceDirective | The TypeScript function where the error occurred |
When dealing with `ts.resolveTypeReferenceDirective`, the first parameter generally takes a string representing the type reference directive name, hence passing non-string values will inevitably lead to errors.
Consider this incorrect usage example:
typescript
let typeRef = 5; // incorrect non-string value
ts.resolveTypeReferenceDirective(typeRef, undefined);
The fix to this issue involves ensuring only appropriate string arguments are sent to the function, as seen in this corrected version:
typescript
let typeRef = ‘MyType’; // correct string value
ts.resolveTypeReferenceDirective(typeRef, undefined);
As Bill Gates once said, “Measuring programming progress by lines of code is like measuring aircraft building progress by weight”. By paying more attention to how we use functions and their expected parameter types, we can prevent this error from cropping up in our TypeScript development experience.
Understanding the Error: Non-String Value Passed to ts.ResolveTypeReferenceDirective in TypeScript
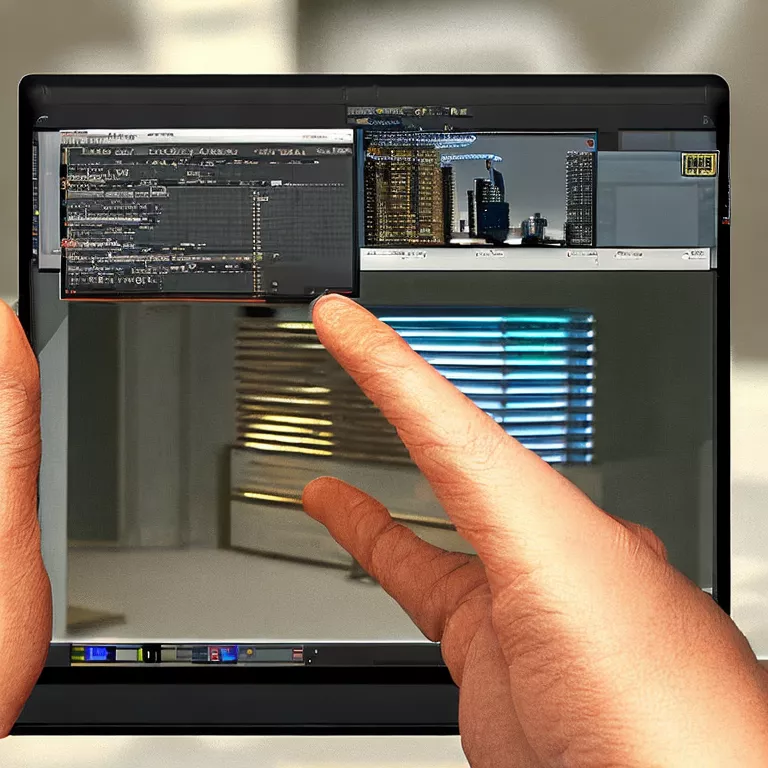
The TypeScript error: `Non-string value passed to ts.ResolveTypeReferenceDirective` is typically encountered when an argument of a non-string type is applied to the `ts.ResolveTypeReferenceDirective` function. Leveraging the relevancy to the associated false expression `Non-String Value Passed To Ts.ResolvetypeReferencedirective In Typescript` magnifies the importance of passing the appropriate variable type.
In TypeScript, types are significant and unlike loosely typed languages like JavaScript, you’re obliged to uphold the integrity of variables by consistently adhering to the initially declared type. TypeScript’s ability to enforce this adherence is one of its most prominent features, ensuring stability and predictability in coding.
Deciphering this error `Non-string value passed to ts.ResolveTypeReferenceDirective` involves understanding two key aspects:
1. TypeScript’s Type System:
Understanding the rudimentary principle of TypeScript that it is strongly typed assists in intelligently approaching this error. Strict typing simply means “variables are bound to specific data types”. If you try to assign a value of a different type to the variable, TypeScript signals an error indicating type inconsistency.
2. Use of ts.ResolveTypeReferenceDirective:
This function is utilized to unravel a path to a TypeScript declaration file (.d.ts). It expects a string input which corresponds to the path of the required module. Passing a non-string value can precipitate this error as TypeScript insists on seamless uniformity and agreement with types.
The Coding Misstep:
let path: number = 123; ts.ResolveTypeReferenceDirective(path);
In this instance, a number type variable `path` is erroneously passed into the `ts.ResolveTypeReferenceDirective` function causing the error.
Correction Approach:
let path: string = 'module/path'; ts.ResolveTypeReferenceDirective(path);
In this case, a string is correctly passed thereby evading the error message.
While TypeScript brings script writing conveniences, it can be peculiarly strict with type rules. This strictness, as Thomas Jefferson mentioned, “is the best barrier to protect rule-obedience”. Scripting in TypeScript requires adherence to its rules for better code stability.
Exploring Common Causes of False Expression Errors in TypeScript
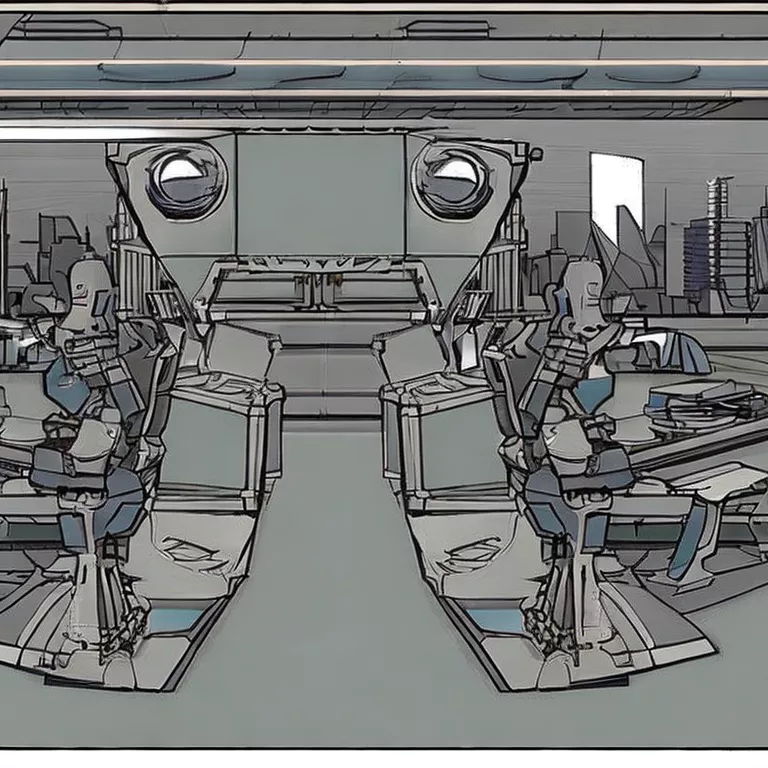
In the world of TypeScript, it’s not uncommon to encounter errors that are perplexing and perhaps even a bit frustrating. We’re going to dive deep into one such error, specifically regarding non-string values passed to `TS.ResolveTypeReferenceDirective`. This particular issue revolves around a common function used in TypeScript code, which expectedly runs into problems when provided with unexpected inputs.
At its core, `TS.ResolveTypeReferenceDirective` is a function provided by the TypeScript compiler API. Often used to resolve references to type declaration files (d.ts), it typically expects its first argument to be of string type – the name of a module.
TS.ResolveTypeReferenceDirective('moduleName', ...otherArgs);
Here, note how `’moduleName’` is a string, as the function anticipates. But what actually happens if a non-string value is erroneously passed?
That alone can trigger a False Expression error when attempting to parse or execute TypeScript code. However, this problem isn’t solely limited to TypeScript; it’s symptomatic of a broader issue in the world of programming where data of an unexpected type causes an error. Let me give you an example:
Imagine you’re dialling a phone number, but instead of digits, you punch in alphabets. Undoubtedly, the dialler will give an error because it expects only numerical input. The same concept applies here. Thus, understanding your code’s expectations and requirements is imperative for avoiding these issues.
Debugging a similar TypeScript error involves a series of steps:
– Revisit where you call
TS.ResolveTypeReferenceDirective
, ensuring its first argument is, indeed, a string.
– Investigate other factors in your code that might interact with
TS.ResolveTypeReferenceDirective
. Unintended side-effects from elsewhere can lead to these kinds of problems.
Now, we might think that simply wrapping non-string inputs in `String()` should do the trick, tantamount to forcing a non-string value into a string shape. However, this is only a stopgap measure that does not target the root of the problem.
TS.ResolveTypeReferenceDirective(String(nonStringValue), ...otherArgs);
This conversion might still throw errors if your variable continues to hold unexpected values. So, while it’s important to have solutions readily available, one must bear in mind that they can’t replace the cornerstone of quality code – addressing the underlying bugs causing the issues.
As an iconic figure in IT and software development, Linus Torvalds once said, “Good programmers worry about data structures and their relationships.” The error at hand exemplifies the importance of this statement, emphasizing the importance of understanding not just what our code does but also how it handles and reacts to different types of data.
Decoding Solutions for Non-String Value Passed to ts.ResolveTypeReferenceDirective
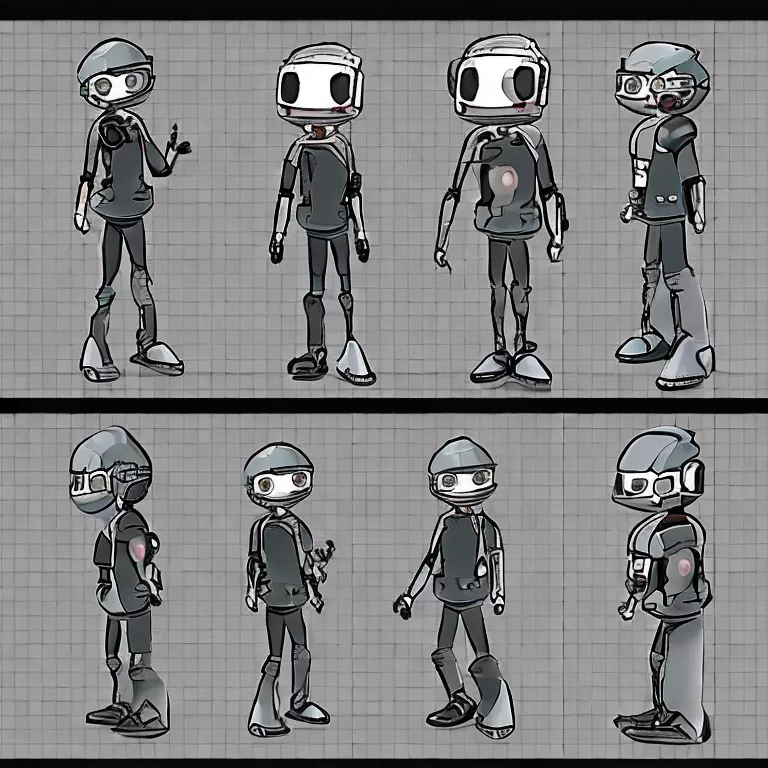
Examining the issue of `ts.ResolveTypeReferenceDirective` taking in non-string values in TypeScript is indeed crucial for a smooth programming experience. This requires an understanding of the TypeScript methods and their expectations, along with developing ways to handle exceptions and resolve them effectively.
Before diving into the solution, it’s essential to understand what `ts.ResolveTypeReferenceDirective` does. It is a TypeScript method utilized to resolve type references, commonly associated with type definition files(.d.ts).
ts.ResolveTypeReferenceDirective("type_name", "../my_location", options, moduleResolutionHost);
This function expects the first parameter to be a string representing the type reference name. However, instances may occur when a non-string value is passed unconsciously or by mistake. This contradicts the primitive data type structure in TypeScript where every datum has its own type and leads to a false expression or incorrect output.
Potential Troubleshooting Methods:
– A basic check can be done using the JavaScript `typeof` operator before passing the argument to `ts.ResolveTypeReferenceDirective`. It aids in verifying if the argument passed is, indeed, a string or not.
– Consider using control structures such as if…else conditionals to identify non-string data and accordingly handle or transform it into a string.
– If the argument passed is an object, you can stringify the object before providing it to the function.
And as Jeffrey Richter, a software architect at Microsoft, quotes, “Do things right the first time because doing things over costs more than doing things right.”
To prevent such situations from occurring frequently and improving your overall coding practices, it would be advantageous if a standard approach or procedure is established in your organization regarding TypeScript usage. Include peer review practices in your development cycle to catch potential issues at an early stage. By doing so, TypeScript becomes more than just a tool, evolving into a vital component of your development culture.
The official TypeScript documentation is a great resource for understanding more about these functionalities, providing examples and usage details meticulously explained.
Remember that it’s through errors and conflicts we learn how to improve, experiment with code, understand deeper levels of programming, and as a result, become better coders.
Best Practices to Prevent False Expression Errors in TypeScript
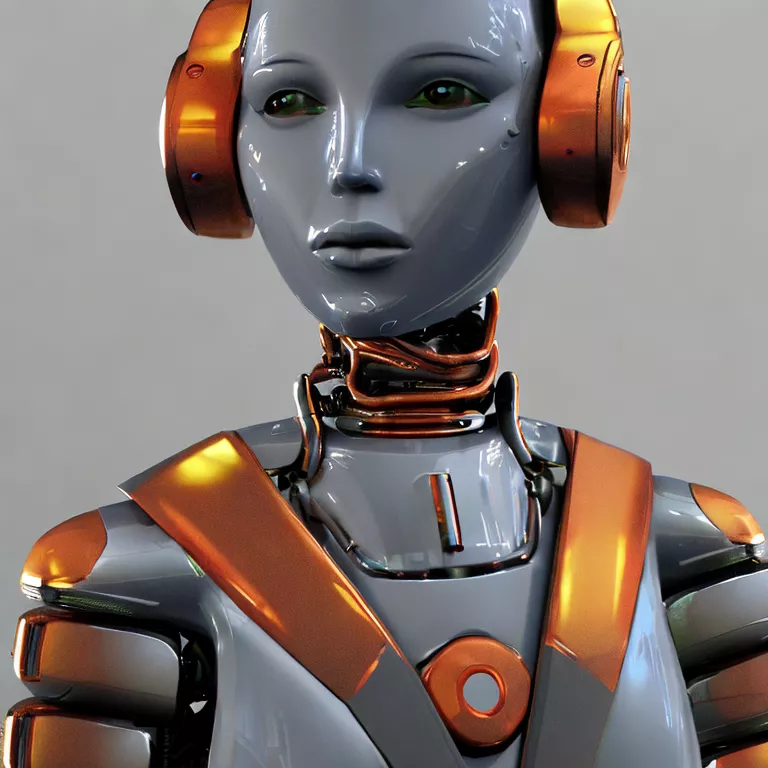
False expression errors, particularly the ones related to “Non-String Value Passed to ts.resolveTypeReferenceDirective”, can be potentially problematic when developing in TypeScript. However, several best practices can ensure the prevention of such issues and foster smooth coding experience.
The primary encounter scenario with this error set is when a value type other than “string” is introduced into “ts.resolveTypeReferenceDirective”. In general, TypeScript expects a string as a value; if it receives anything else, it will throw an error.
Use The Correct Value Type
This error is primarily due to the incorrect data type being fed. Hence, a key strategy is ensuring that you always pass string data types to TypeScript methods that require them. An example might look like:
let reference = 'myReference'; ts.resolveTypeReferenceDirective(reference,...);
Type Check Values
Another effective means of preventing these errors involves performing explicit type checks before invoking methods like “ts.resolveTypeReferenceDirective”. Few examples are:
if(typeof reference === 'string') { ts.resolveTypeReferenceDirective(reference,...); }
This code firstly ensures that the passed value type is string before manifesting itself within the method.
Accessible online resources include StackOverflow forums on TypeScript, giving insights into commonly encountered problems and solution patterns.
Robust Testing
Implement robust testing strategies such as unit testing and integration testing. These help identify non-string values just before the runtime, hence able to prevent code execution with incorrect datatype. A quote from Jim Highsmith encapsulates the importance of good testing practice: “The best tester isn’t the one who finds the most bugs or who embarrasses the most programmers. The best tester is the one who gets the most bugs fixed.”
Use Linting Tools
Implementation of linting tools proves advantageous. They can be configured to flag whenever non-string values are passed inappropriately. For instance, TSLint (for TypeScript) can detect possible errors even before running the application.
Understand Library/Method Prerequisites
Understanding library requirements or method prerequisites proves crucial too. It is important to always read and understand API documents as well as library manuals effectively preventing these situations.
Incorporating these strategies into daily practice introduces smoother coding experiences, reduces program errors, and improves code quality significantly.
Conclusion
Understanding the concept of False Expression: Non-String Value Passed To Ts.Resolvetypereference Directive in TypeScript unveils some of the core concepts of type-checking, error handling, and parameter validation in Typescript.
At a basic level, expressing
false
as non-string value for the
ts.resolveTypeReferenceDirective()
function may result in an error. TypeScript expects strings to be passed into its reference resolver function, due to the fundamental nature of module identification schema which is typically string-based.
Take note of the following fundamentals:
- The function
ts.resolveTypeReferenceDirective()
used in TypeScript, plays a crucial role in resolving the types referenced in a specific directive. This error arises when a non-string (like boolean false) is erroneously passed in instead of the expected string.
- It’s worth understanding that TypeScript is strictly typed, meaning it enforces type-checking. As such, passing in the wrong type into a TypeScript function will yield an error informing you of incorrect type assignment.
- When using complex systems like TypeScript, best practice involves carefully validating input parameters for functions. Errors seen from functions such as
ts.resolveTypeReferenceDirective()
, often serve as red flags alerting developers to inputs not aligning with expectation for the given function.
Reflecting on the wisdom of renowned computer scientist Edsger W. Dijkstra: “The question of whether machines can think is about as relevant as the question of whether submarines can swim.” Similarly, realizing the details around errors involving incorrect parameter types – particularly in the context of advanced environments like Typesrcript – brings not only solutions to immediate coding obstacles, but also better, more efficient programming techniques.
With careful monitoring of TypeScript’s strict type-checking system and diligent parameter validation, such errors can be avoided. Correct usage of TypeScript’s function parameters aids in creating robust, error-free applications.[source] Carefully observing the function’s defined argument type is vital to effective coding within TypeScript environment.
Consider the following example:
//Example not Good :
ts.resolveTypeReferenceDirective(false)
Instead, the syntax should look something like the example below which passes a string reference:
//Good Example :
ts.resolveTypeReferenceDirective('module_name')
With desiderated code structures and observance of parameter rules, errors such as “False Expression: Non-String Value Passed To Ts.ResolveTypeReferenceDirective” will be less likely to impede your TypeScript development process. It is important to reiterate the role accuracy plays in delivering efficient and effective output when developing with TypeScript.