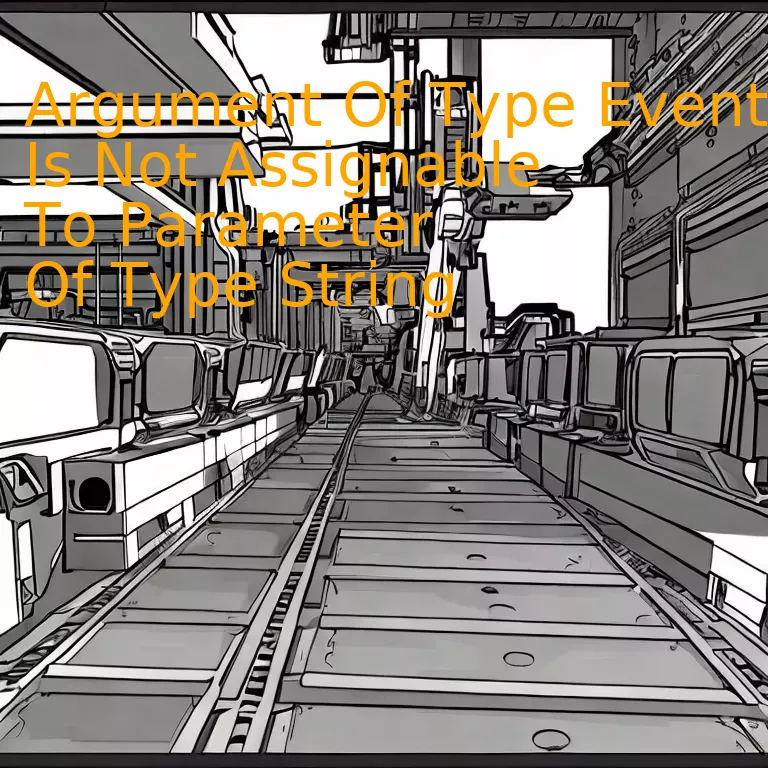
Introduction
Discover solutions concerning the assertion “Argument Of Type Event Is Not Assignable To Parameter Of Type String,” a common error that tends to arise in programming where an event type and a string type are mismatched, often causing confusion for developers but can be efficiently resolved with proper knowledge and code adjustment.
Quick Summary
Exploring the Complication: Argument Of Type ‘Event’ Is Not Assignable To Parameter Of Type ‘String’
Concepts | Details |
---|---|
TypeScript’s strong typing system | The TypeScript language incorporates a robust type system which aids in code quality and understandability. This feature assists in catching errors during the writing phase rather than in runtime, thereby enhancing the debugging process. |
Kinds of Types | In TypeScript, types such as String, Number, Boolean, Event among others are predefined. Specifically focusing on String and Event types which are at the center of our discussion. |
Mismatch between Event and String | An Event object cannot be used where a String is expected as they are two different types with different properties and methods inherent. Trying to assign one to the other causes a compile-time error, which in this case is ‘`Event`’ is not assignable to parameter of type ‘`String`’.” |
When we delve into TypeScript, we encounter its robust typing system which enhances code quality and understandability. This feature catches errors while coding, instead of during runtime, making troubleshooting more streamlined and effective.
In TypeScript, there are predefined types such as String, Number, Boolean, Event and many others. Two types pertinent to our discussion are String and Event. A string in TypeScript essentially holds a set of characters (text), just like in JavaScript. The String type offers various methods and properties inherent to it.
On the contrary, an Event is an object that represents an occurrence, typically user interactions like mouse clicks or key presses. The Event type accommodates properties and methods that provide information about the event.
A critical point to consider is that you can’t use one type where another is expected. Specifically, an Event object cannot be used where a String is expected. This is because the Event and String are two entirely different types with unique properties and methods. Trying to assign one to the other leads to a compile-time error stating
Argument of type 'Event' is not assignable to parameter of type 'String'
.
This implies that somewhere in your TypeScript code there’s an argument of type Event being passed into a function or method which expects a parameter of type String which is causing this error. It’s crucial to correctly match types when working with a strongly-typed language like TypeScript.
Misconception | Solution |
---|---|
xxxxxxxxxx Argument of type 'Event' is not assignable to parameter of type 'String' |
The best approach to fix this issue would generally involve investigating the piece of code where this error is occurring and rectifying the inaccurate argument or incorrect expectation by adjusting the Type from Event to String or vice versa depending on the business requirement. |
To deal with such scenarios, it’s advisable to have a clear picture of the function’s/task’s intent. Once the use-case is understood, it becomes easier to judge whether altering the expected type from String to Event would solve the issue or if the input’s type from Event to String should be changed.
[Tom Preston-Werner](https://twitter.com/mojombo), co-founder of GitHub eloquently puts it:
“When I’m working on a problem, I never think about beauty. I think about how to solve the problem. But when I have finished, if the solution is not beautiful, I know it is wrong.” This quote stresses the importance of devising effective and accurate solutions in programming, which resonate with solving type errors in TypeScript as well.”
Understanding Argument Types: Event vs String in Coding
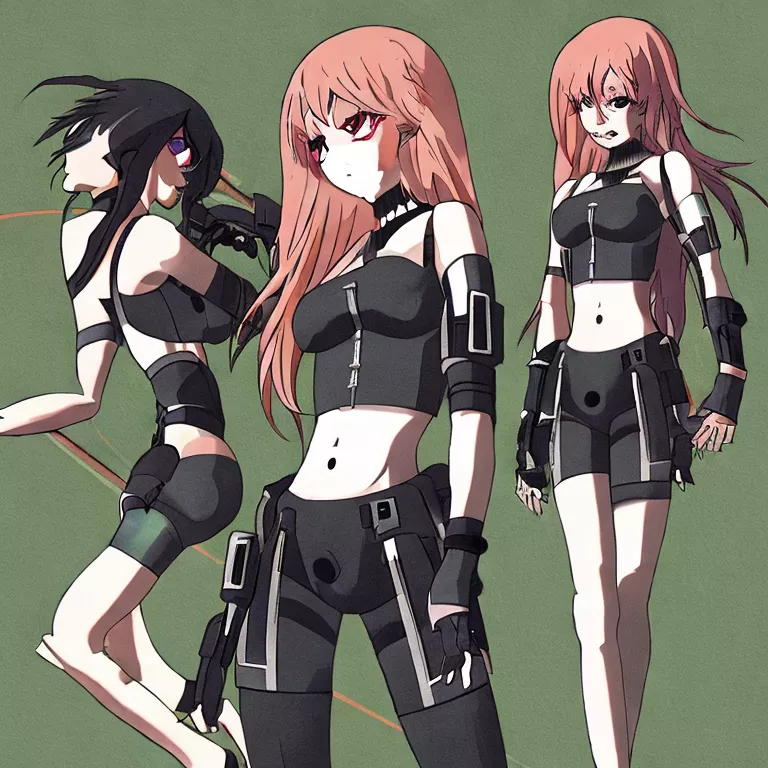
The question at hand involves understanding argument types, specifically `Event` vs `String`, in the coding world. Particular emphasis is laid on the error “Argument of type `Event` is not assignable to parameter of type `String`”. This generally happens when you are passing an event object where a string is expected.
Broadly speaking, each function in TypeScript can accept certain types of arguments. Ensuring correct data types is essential; many common errors arise from misusing or misunderstanding them. Let’s dive deeper into these two argument types: `Event` and `String`.
What are Event and String Argument Types?
An
xxxxxxxxxx
Event
object represents an event which takes place in the DOM (Document Object Model). The Event object contains information about the event, including the event’s type and the element which triggered the event. You’re most likely to come across this during scenarios involving user interaction with your application.
xxxxxxxxxx
button.addEventListener('click', (event) => {
console.log(event.target);
});
On the other hand, a
xxxxxxxxxx
String
represents textual data. It is one of the fundamental datatypes used universally in almost every programming language. Strings have a vast arsenal of methods for manipulation such as `length`, `substring`, and more.
xxxxxxxxxx
let message: string = "Hello, World!";
The Conflict between Event and String
In TypeScript, strict type checking ensures that parameters passed to a function match the declared parameter type for the function. When you receive the error – “Argument of type ‘Event’ is not assignable to parameter of type ‘String'”, TypeScript is basically saying that you are passing an ‘Event’ object to a function that is expecting a ‘String’.
Imagine a situation like below:
xxxxxxxxxx
function greet(message: string) {
console.log(`Hello ${message}`);
}
button.addEventListener('click', (event) => {
greet(event); // Throws error
});
The welcome message function `greet` anticipates a string parameter. However, an event is being passed instead, causing the compiler to flag an error.
Resolving the Error
To solve this, you should pass the appropriate data type. If your function expects a string, ensure a string is what it gets. In the example above, if you wanted to pass some text in event trigger, it may look something like this:
xxxxxxxxxx
button.addEventListener('click', (event) => {
greet("from click event"); // Resolves error
});
As Frederick P. Brooks Jr., a renowned software engineer and computer scientist once said, “The programmer, like the poet, works only slightly removed from pure thought-stuff. He builds his castles in the air, from air, creating by exertion of the imagination.” Recognizing the difference between ‘Event’ and ‘String’ argument types and how to navigate associated nuances signifies an important stride in structured and sound coding.(source) Understanding these differences helps build your conceptual castles more robustly and effectively.
Decoding the Error: ‘Argument Of Type Event Is Not Assignable To Parameter Of Type String’
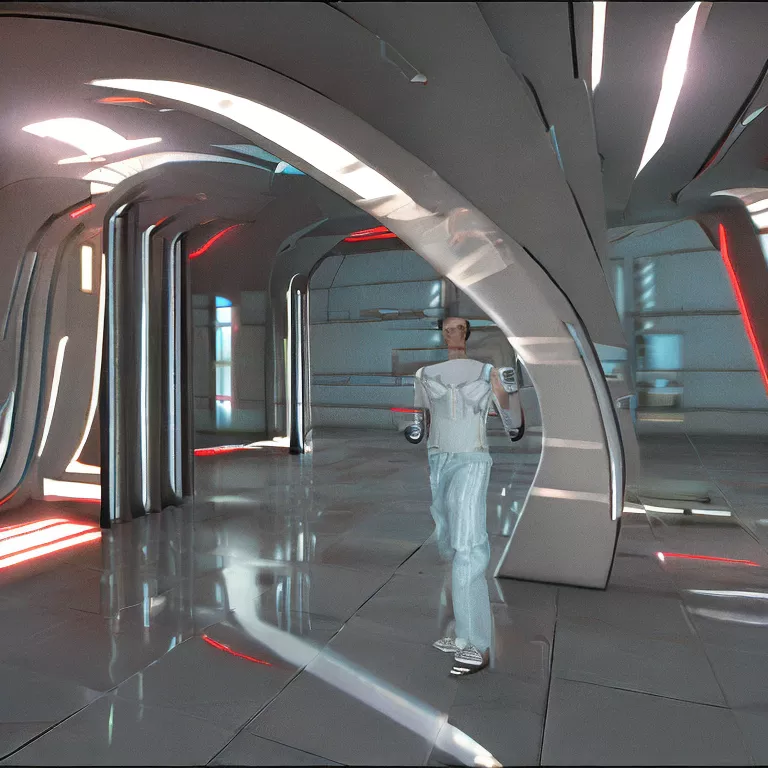
The TypeScript error ‘Argument of type Event is not assignable to parameter of type String’ is something you might encounter at one point or another during development. This error typically arises when trying to utilize event-related functionality in TypeScript.
This issue originates from static types in TypeScript. Static types are a feature where the type (number, string, object etc.) of a variable is established at compile time and it cannot be changed throughout its lifespan. The forward compatibility, scalability, and predictability of static types have made them a core part of TypeScript.source
When it comes to handling events in TypeScript, the error ensues when you try to assign a wrong type value to an expected different type. In our case, the error ‘Argument of type Event is not assignable to parameter of type String’ indicates that we’re trying to use an object of ‘Event’ type as a ‘String’ which is statically wrong in TypeScript.
To further illustrate this scenario, let’s take this code snippet:
xxxxxxxxxx
function handleInputChange(e: string) {
console.log(e);
}
myInput.addEventListener('input', handleInputChange)
In the code above, `handleInputChange` is explicitly saying that it accepts a string for argument `e`. However, `addEventListener` function calls the `handleInputChange` with an Event object instead of a string, hence the mismatch.
To rectify this issue, here are potential solutions:
-**Change the Parameter Type**: Reframe your method or function to receive an object of type ‘Event’ instead of a ‘string’. A good example would be like:
xxxxxxxxxx
function handleInputChange(e: Event) {
console.log(e);
}
-**Transform the Event Object into the Desired Type**: You could extract the desired ‘string’ property from the ‘Event’ object before passing it to the function:
xxxxxxxxxx
myInput.addEventListener('input', (e) => handleInputChange(e.target.value))
In this modified code snippet, `e.target.value` is a string that represents the input’s current value. Now, we’re aligning with TypeScript’s expectation and the ‘string’ type is what is being sent to `handleInputChange`
As Bill Gates once said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” Don’t forget that writing clean, error-free TypeScript may not necessarily be the easiest path, but it leads us towards creating code that is more predictable, easier to debug, and reliable in operation.source
Solutions to Fixing the Mismatch Between Event and String Parameters
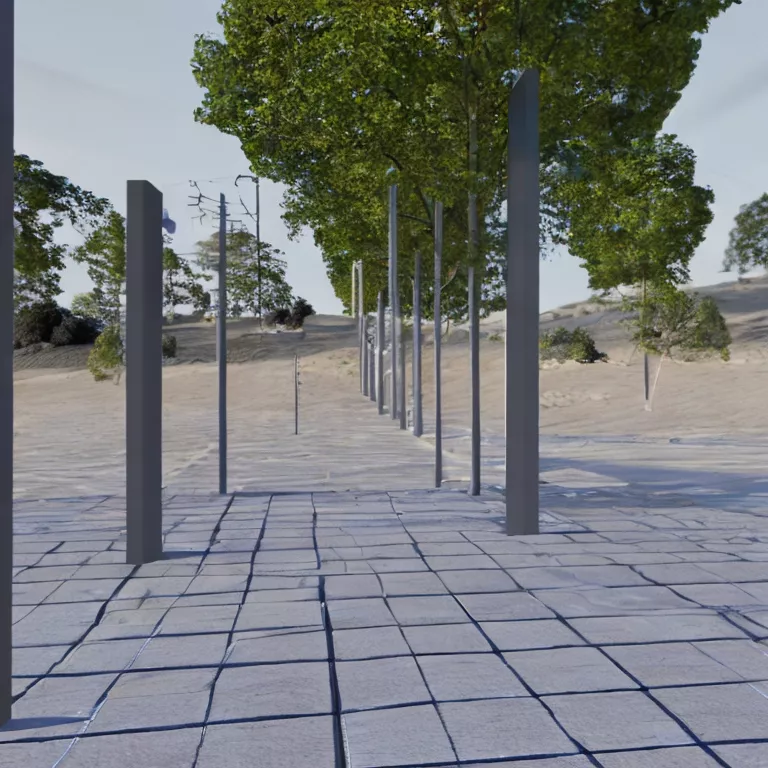
The issue of the mismatch between Event and String parameters is one that often crops up in TypeScript development, predominantly due to TypeScript’s rigorous type checking. This incompatibility problem usually arises when developers inadvertently use an Event object where a string parameter is expected.
In TypeScript, each variable or function parameter needs to match a specific type. That means you cannot assign an ‘Event’ to a ‘string’, as these are completely different data types. Strictly speaking, ‘Event’ is an object with multiple properties used to describe an event—a button click for example—while a ‘String’ is a sequence of characters.
Let’s address this issue from the perspective of a typical compiler error message that might prompt you to investigate this argument mismatch: “Argument of type ‘Event’ is not assignable to parameter of type ‘string’.” Here we see that the TypeScript compiler checks the types assigned to values and parameters, thus ensuring the consistency of the codebase. It can raise such an issue when there’s an unexpected assignment of incompatible types.
Here are a few key points and solutions to resolving this kind of type mismatch:
• Check your function signatures: Confirm that you’re passing the correct argument types that match your function’s signature. If a function expects a string, but receives an event, the “Argument of type ‘Event’ is not assignable” error will be triggered. Redefine your function to expect an Event type if you intend to pass an Event object.
xxxxxxxxxx
function eventName(event: Event) {
// handle the event here
}
• Access the appropriate properties: If you need to transform your Event into a string representation, perhaps to log it for debugging purposes, access the suitable attribute within the Event object. For instance, `event.type` gives the string representation of the Event type.
xxxxxxxxxx
function handleEvent(event: Event) {
let eventTypeString: string = event.type;
console.log(eventTypeString);
}
• Type Assertion: In special scenarios, TypeScript allows `Type assertion` to override the type-checking process albeit with caution needed not to misuse it. This can be a helpful method in situations where you are confident of the type that a value should have.
xxxxxxxxxx
let e: any = yourEventObj;
let str: string = (e);
JavaScript and Typescript guru, Douglas Crockford, once expressed his thoughts on typing systems in programming: “Types are an essential element of good programming style. They give the reader better insight into your intent, while giving the compiler more information to help trap common errors.”
It’s important to remember that, in TypeScript, these strict typings are there to make our code safer and more predictable. It accurately identifies potential runtime problems during compile-time, hence the need to ensure that we work with rather than against the type checking system.
Practical Examples & Use-Cases of Corrected ‘Not Assignable’ Errors
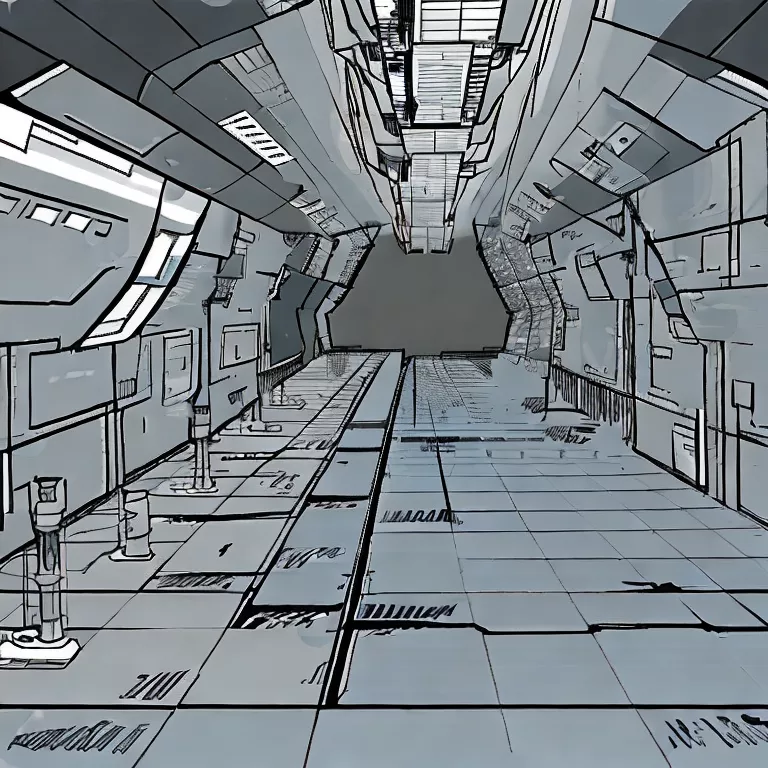
Successful code development in TypeScript includes constant vigilance over type-checking errors, one of which is the ‘Not Assignable’ error. This error occurs when an argument of one type gets erroneously assigned to a parameter of different data-type. A common instance encountered by many developers is the message “Argument of type ‘Event’ is not assignable to parameter of type ‘String'”.
To address and decode this error, it’s essential to start with understanding what this error signifies. This error creeps up when there’s inconsistency between the parameter type expected by a function and the argument that is being passed. In this case, the function expects a string but sees an Event Object.
For example, consider a function intended to handle form input:
xxxxxxxxxx
function handleInput(value: string) {
console.log(value);
}
A developer might try to call this function in response to an ‘onChange’ event from an input, as follows:
xxxxxxxxxx
handleInput(event)} />
At this point, TypeScript will flag an error, stating “Argument of type ‘Event’ is not assignable to parameter of type ‘String'”.
Why does this happen? Well, the dataset enclosed within the ‘onChange’ event is an EventObject, not a String. However, our ‘handleInput’ function only accepts strings.
To rectify this issue, you need to reach into that event object and extract the target value. Here’s how to do it:
xxxxxxxxxx
handleInput(event.target.value)} />
Now `handleInput` accepts `event.target.value`, a field on the Event object which is indeed of type string.
There are numerous other times when developers will run into a similar ‘Not Assignable’ situation – working with third party libraries, connecting to APIs, handling UI events. The fundamental approach to resolving it remains the same – always ensure compatibility between data types your function expects and those it actually receives.
As renowned software engineer Robert C. Martin quotes, “The way of the software samurai is through understanding and masterful control of the type system at his disposal.” Matching data-types accurately can be initially daunting but with consistent practice, your comfort levels accelerate and errors like ‘Not Assignable’ become a thing of the past.
Conclusion
Solving the Problem
The discrepancy of data types could be resolved by invoking the correct properties from the ‘Event’ object to extract the target value as a string or by revamping the method to ensure compatibility with an ‘Event’.
Below is an illustrative coding snippet displaying how the target value can be extracted.
xxxxxxxxxx
// Incorrect
let input: HTMLInputElement = document.querySelector('#input-id');
input.addEventListener('change', updateValue);
function updateValue(value: string) { console.log(value); }
// Correct
let input: HTMLInputElement = document.querySelector('#input-id');
input.addEventListener('change', (event:Event) => {
updateValue(event.target.value);
});
function updateValue(value: string) { console.log(value); }
When an event triggers in JavaScript, an event object is automatically created. This event object contains information about the event, including the target which is the element that triggered the event. In our modified example, the ‘updateValue’ function receives the target value from the event as a string, which aligns with the expected value thus solving the problem.
As Bill Gates once said, “I choose a lazy person to do a hard job. Because a lazy person will find an easy way to do it.” Interestingly, Gates’ quote resonates when looking at this TypeScript issue. Utilizing the inherent structures and tools within programming languages, such as TypeScript’s event listeners in this scenario streamlines coding efforts by simplifying processes.