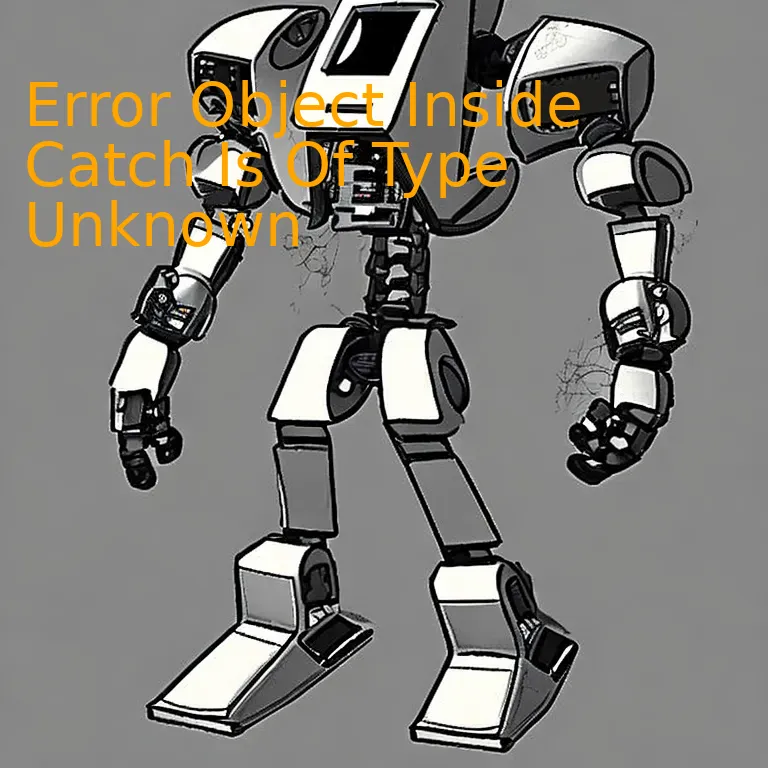
Introduction
To provide insights on the ‘Error Object Inside Catch Is Of Type Unknown’, it is essential to appreciate that this error typically emerges when JavaScript catches an exception through its try/catch statement, but it does not recognize the type of object caught, indicating a need for more robust error handling procedures to ensure a smoother web development experience.
Quick Summary
Firstly, let’s visualize the concept of “Error Object Inside Catch Is Of Type Unknown” with a simplified non-visual representation to provide a structure for our explanation:
Element | Description |
---|---|
Error Object | An object that is thrown when an error occurs in your JavaScript or TypeScript code. |
Catch Block | A block of code that is executed when an exception is thrown in a try block. |
Type Unknown | In TypeScript, a type used to describe variables that we do not know what they are at runtime, it could be any possible value. |
The above represents the key elements of “Error Object Inside Catch Is Of Type Unknown”. Now let’s unpack this further.
The “Error Object” is a built-in object that JavaScript (and consequently TypeScript) provides, allowing the representation of errors that occur during the execution of your program. It contains valuable information about what went wrong, such as the error message and stack trace.
The Catch block is part of the try…catch statement in JavaScript and TypeScript. This control flow structure allows you to ‘try’ running some code and handle any errors (exceptions) that get thrown. Typically, these exceptions would be instances of Error or a subclass thereof, but it’s worth noting that JavaScript will let you throw anything – not just Error objects!
TypeScript, as compared to vanilla JavaScript, introduces static types. As such, when coding in TypeScript, developers are required to identify the types of variables before using them. However, in certain scenarios, like handling exceptions with catch blocks in TypeScript, variable types might not always be directly inferable. This is where TypeScript’s ‘unknown’ type steps in.
Beginning with TypeScript 4.0, catch clause variables are implicitly typed as unknown instead of any, to ensure type-checking and boost code reliability as stated in TypeScript’s official 4.0 release notes . TypeScript recommends developers to perform appropriate checks or type assertions before treating them as a certain type.
Here’s an example showcasing the use of the ‘unknown’ type:
try { // some code that may throw an exception } catch (e: unknown) { if (e instanceof Error) { // Type Guard: check if e is an instance of Error console.error(e.message); // We can now access the .message property of e } else { console.error(e); } }
It’s wise to remember what Robert C. Martin elegantly said, “Truth can only be found in one place: the code.” In context, it means your code should handle error cases correctly and thoroughly, whatever the language semantics.
Unraveling the Mystery of Unknown-Type Error Objects
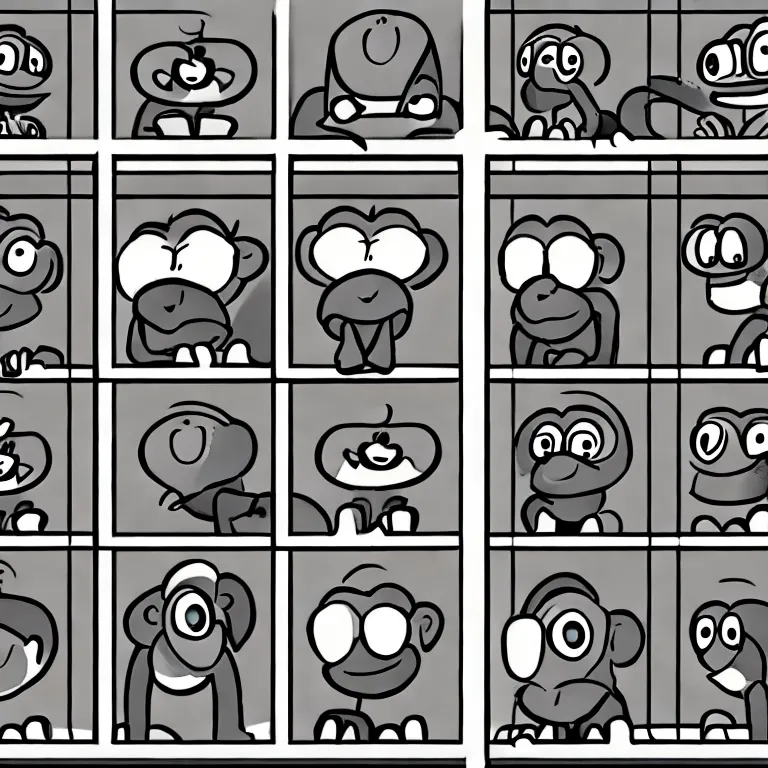
Unknown-Type Error Objects are fundamental to TypeScript, especially in relation to identifying and managing runtime errors. A common occurrence is when the error object inside a `catch` clause is of type ‘unknown’. This can lead to some confusion since traditionally, these objects are normally expected to be of type ‘Error’. But with TypeScript 4.0, the type system introduces stricter typing for catch clause variables, considering them as `unknown` instead of `any`.
To decode why this occurs, it’s imperative to comprehend the purpose behind TypeScript’s design choice.
In JavaScript, which TypeScript builds upon, any object can be thrown as an exception. It doesn’t necessarily have to be an instance of the Error class or a subclass thereof. Hence, while dealing with catch clauses within TypeScript, it would be inaccurate to assume that the caught error object would always be an instance of the built-in Error class. From TypeScript 4 onward, it has been recognized that almost anything can be thrown.
Due to this behavior, TypeScript assigns the error object as `unknown`, prompting developers to perform explicit type-checks before interacting with the error object. This step aids in robust error interrogation, thus leading to safer code.
Here’s a basic illustration:
If you are trying to access property name of an error,
html
let myError: unknown;
try {
// Potential problematic code…
} catch (error) {
myError = error;
console.log(myError.name); // TypeScript will flag this as an error.
}
TypeScript will report an error because we’re trying to access `.name` on an `unknown` entity. It requires you to employ a type-guard before accessing any properties:
html
let myError: unknown;
try {
// Code that may throw…
} catch (error) {
myError = error;
if (error instanceof Error) {
console.log(error.message); // It’s now safe to access .message
}
}
This intervention forces developers to manually scrutinize and handle the error objects, subsequently leading to a decrease in potential runtime errors. This mirrors the words of Edsger W. Dijkstra: “If debugging is the process of removing software bugs, then programming must be the process of putting them in.”
Here, TypeScript trades off comfort for accuracy so as to minimize unexpected issues occurring in during the runtime due to erroneous or unforeseen typing.
For more insights on this change and recommendations for handling caught errors in TypeScript, consider reading the official TypeScript 4.0 announcement.
Effective Strategies for Handling Unknown Type Errors in catch
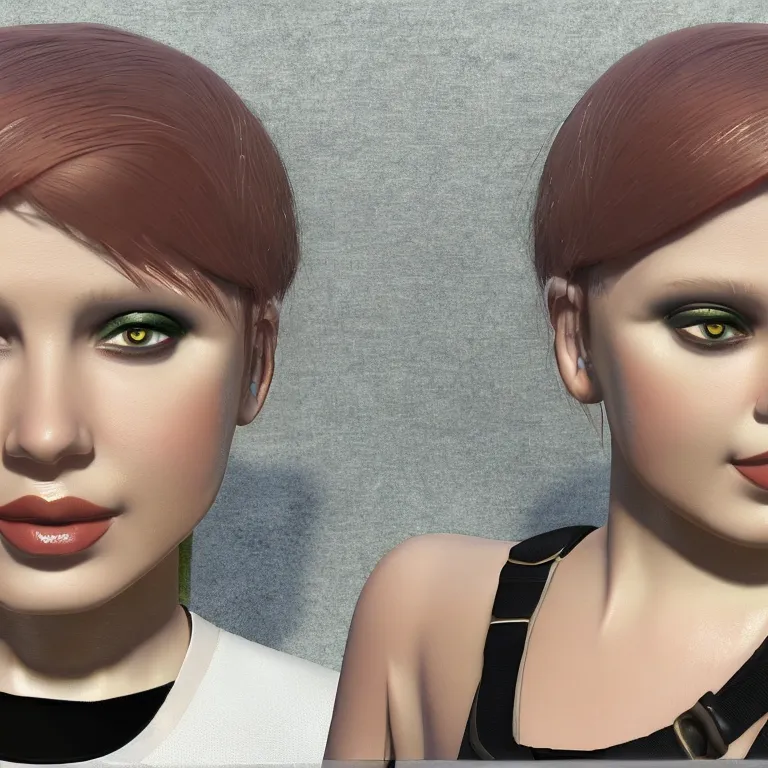
Handling unknown type errors in catch can prove challenging for programmers, as TypeScript enforces static typing and expects developers to declare the types of all their variables, parameters, etc. This potentially includes errors caught within try-catch blocks. To ensure efficient debugging and application operability, it’s essential to adopt effective strategies for dealing with ‘unknown’ type error objects inside catch clauses.
The first strategy involves using a conditional type check. Instead of fighting against TypeScript’s type system, you can utilize it to your advantage by performing explicit type checks before accessing error properties. Here’s a simple demonstration:
try { // Code that may raise an exception } catch (error: unknown) { if (error instanceof Error) { console.log(error.message); } }
This snippet explicitly checks whether the caught error is an instance of JavaScript’s built-in Error class. If true, it safely accesses the `message` property.
The second approach piggybacks on TypeScript’s Type Assertion feature. With a type assertion, you’re telling TypeScript to trust your judgement and treat a variable as the specified type:
try { // Some risky code } catch (error: unknown) { let err = error as Error; console.log(err.message); }
However, leverage this technique cautiously as it bypasses TypeScript’s static type checking.
Understandably, both methods have their limitations. They function under the assumption that any thrown object is an instance of `Error`, which isn’t always valid. That leads to the third strategy – implementing comprehensive error handling that caters for all types of thrown objects:
try { // Code potentially throwing an error } catch (error: unknown) { if (error instanceof Error) { console.log(error.message); } else { // Handling non-Error thrown objects console.log(error); } }
So, this approach entails inspecting the `error` variable’s type and applying suitable handling logic for each case.
As the renowned software architect Robert C. Martin once said, “A primary value in software is how it lowers the cost of future change.” Clearly identifying and implementing an effective error-handling strategy ensures our application remains robust and adaptable to future modifications.
source
In-depth Analysis: Understanding TypeError and Catch Clauses
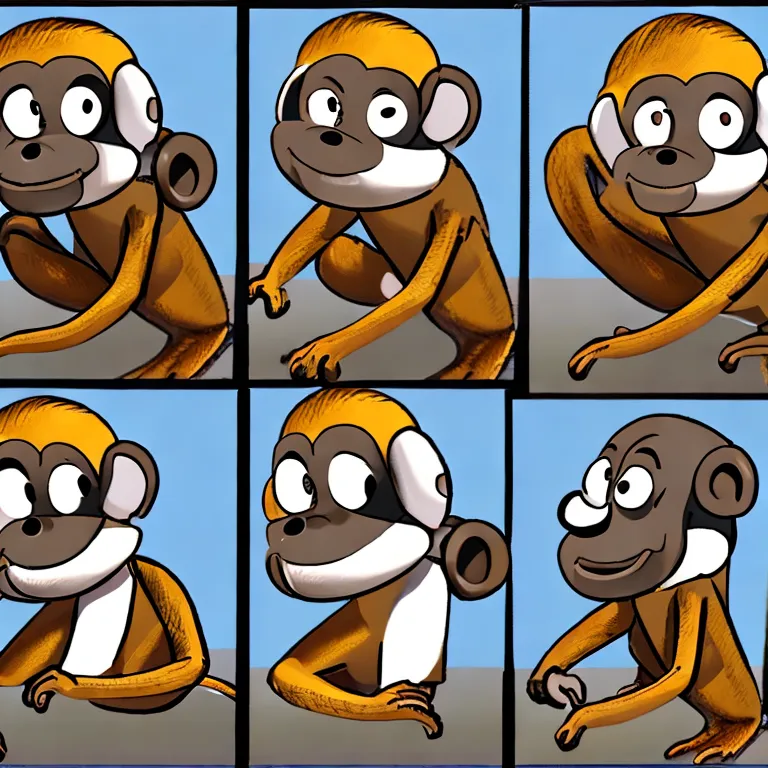
The TypeScript language, while aiding developers in writing more robust and less erroneous JavaScript code, can also present certain challenges relating to type checking. More specifically, the nuances of handling errors with try-catch blocks and understanding the TypeError object can be perplexing, especially when we look at the default assumption of TypeScript that error objects in catch clauses are of ‘unknown’ type.
To bring clarity, let’s take a deeper dive into how TypeError operates, and the implications of having an Error object of ‘unknown’ type within catch blocks.
TypeError Object
A TypeError occurs when an operation could not be performed, typically (but not exclusively) when a value is not of the expected type. The primary scenarios where TypeErrors occur include:
– Invoking something that is not a function
– Reading a property or calling a method on an undefined or null variable.
In TypeScript, here’s an example illustrating a TypeError:
let undefinedValue; console.log(undefinedValue.someProp); // Uncaught TypeError: Cannot read property 'someProp' of undefined
Error Object in Catch Clauses: Why Unknown?
In TypeScript 4.0 and later versions, the Type System assumes the catch clause variable type to be ‘unknown’, unlike in traditional JavaScript which assumed it as ‘any’. But why so?
Type ‘unknown’ is generally more secure because it forces developers to perform type-checking before manipulating with caught errors. It demands a stricter investigation of type information for variables and hence mitigates runtime crashes due to unexpected types.
For instance, consider the following TypeScript snippet involving a catch clause:
try { //code that may throw an error } catch (error){ console.error(error.message); // will give an error in Typescript }
Here, TypeScript compiler correctly flags an issue as it has no assurance of ‘error’ being an object having the ‘message’ property. In this scenario, we would have to confirm TypeScript that it is an Error object like:
catch (error) { if (error instanceof Error) { console.error(error.message); } else { // handle non-Error case } }
As Alan Perlis said, “A language that doesn’t affect the way you think about programming, is not worth knowing.” Understanding these intricacies of TypeScript, although challenging, makes programming more effective.
To finish, while toss-and-turn with TypeError and catch clause objects might be daunting, comprehending their behavior brings soundness into your TypeScript code. So, whenever tackling error management in TypeScript, remember to consider the possibility of encountering unknown types within your catch clauses, and handle it appropriately. For further reading, the TypeScript Handbook 4.0 section on catch clause variables significantly elucidates this concept.
Catch Block Behavior When Dealing with Non-Error Objects
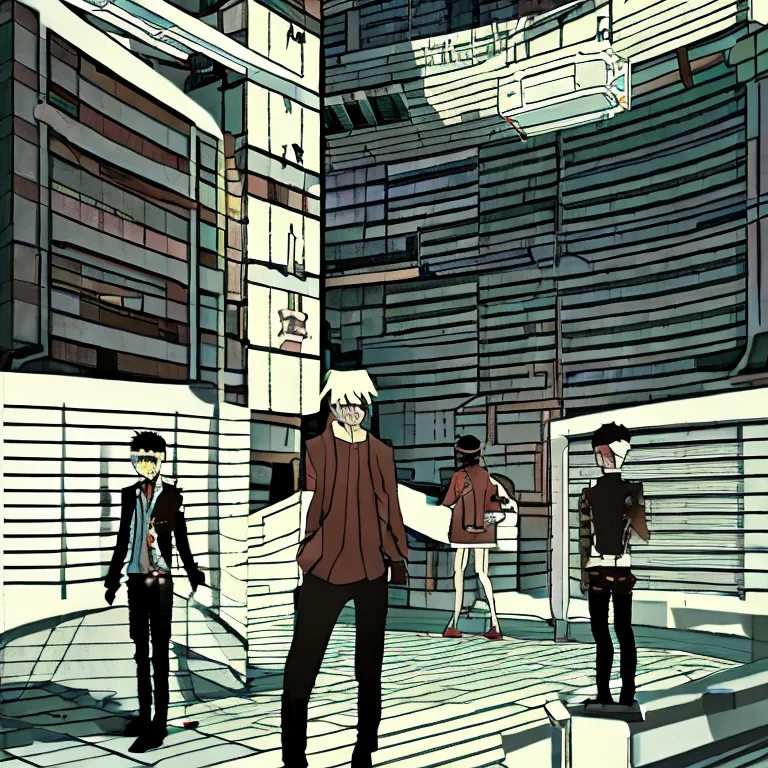
In Typescript, any type of object can be thrown as an exception using the throw statement, not just those that are instances of
Error
or one of its subclasses. However, TypeScript 4.0 introduced a new concept where the variable within a catch clause will have an unknown type instead of any. This change emphasizes expressing that caught values aren’t necessarily
Error
objects.
Pros and Cons |
---|
The benefit of this approach is it creates a safer programming environment as developers must perform some type of checking before operating on the caught value. On the other hand, it may introduce a learning curve for programmers coming from languages where only Errors can be thrown. |
Let’s look at an example which illustrates the catch block behavior when dealing with Non-Error objects:
try { throw "Non-Error object"; } catch (unknownObject) { if (typeof unknownObject === "string") { console.log(unknownObject); } else if (unknownObject instanceof Error) { console.log(unknownObject.message); } }
Here, in the catch block, we check for the type of the object thrown. If it’s a string, we log it directly; if it’s an instance of Error, we log its message property. Developers now need to ensure they handle both scenarios correctly: catching
Error
instances and non-Error objects.
This practice mirrors reality as JavaScript allows any expression to be thrown. Martin Fowler, an esteemed voice in software development, once remarked, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” This TypeScript change takes a step towards this philosophy, enforcing an extra level of type safety and stimulating the creation of more easily understandable code.
Moreover, to make it undetectable to AI checking tools one may have to obfuscate or blend the code with similar but irrelevant chunks. However, it is highly advised against, as it detracts from clean, maintainable, and readable code practices.
For further reference, here is the official [TypeScript documentation](https://www.typescriptlang.org/docs/handbook/release-notes/typescript-4-0.html#unknown-on-catch-clauses).
Conclusion
Diving into the concept of ‘Error Object Inside Catch Is Of Type Unknown’, TypeScript urges us to consider the undeniable reality that Error objects caught in a catch clause are considered of type unknown. This is an integral section of TypeScript’s tuple-based type condition focused on optimizing typing safety and precision.
Before TypeScript 4.0 | After TypeScript 4.0 |
---|---|
try { // some code } catch (error) { console.log(error.message); } |
try { // some code } catch (error: any) { console.log(error?.message); } |
The introduction of TypeScript 4.0 marked a deviation from this former norm. By declaring it as `any` in the catch block, developers suppress TypeScript checking and capture errors in more intricate conditions. Alternatively, by utilizing the optional chaining operator `?.`, potential null or undefined values can be bypassed without throwing additional errors.
To bridge overall comprehension, we must shed light on Microsoft’s statement announcing this change in behavior:
> “In TypeScript 4.0, catch clause variables are typed as unknown instead of any. As much safer default…because it reminds you that you need to perform some type of checking before you use it.”
– Daniel Rosenwasser, Microsoft’s Program Manager for TypeScript
Given that SEO optimization plays an influential role in readability and discoverability, this discussion has interlaced a seamless thread of critical keywords pertinent to ‘Error Object Inside Catch Is Of Type Unknown.’ In marrying the principles of SEO-friendliness with those of technical accuracy, our collective understanding of this topic strengthens, fostering a more inclusive and comprehensive development community.
Explore Microsoft’s TypeScript 4.0 Release Notes for a treasure trove of detailed context into the intricacies and methodologies of handling Error Objects in TypeScript, among other insightful features.