Introduction
In troubleshooting complex coding scenarios, it is crucial to grasp the cause of a common error known as TypeError: Converting circular structure to JSON, which typically stems from an object with the constructor ClientRequest, displaying its intricacy within the realms of JavaScript programming.
Quick Summary
An intricate and frequent specialist’s pitfall in JavaScript, or rather its variant TypeScript, is the occurrence of the dreaded “TypeError: Converting circular structure to JSON” error. This fatal eventuality occurs when an attempt is made to transform a circular data structure, meaning one that refers back to itself, into the universally recognized JSON format.
TypeError | Implication | Strategy |
---|---|---|
“Converting circular structure to JSON” | A circular reference within an object that can’t be converted into JSON format. | Identify the circular structure so as to break the cyclic dependency. |
Example: Object with constructor ‘ClientRequest’ | An HTTP request in a Node.js environment attempting serialisation vertically down the prototype chain. | Construct objects with decoupled entity relationships thus limiting exposure to serialization process. |
To further illustrate this, consider the following TypeScript example:
class ClientRequest {
clientReq: any;
constructor() {
this.clientReq = this; // creating a circular reference
}
}
let req = new ClientRequest();
let json = JSON.stringify(req); // throws TypeError: Converting circular structure to JSON
Above, we have a `ClientRequest` class which assigns a reference to itself in its constructor, creating a circular structure. When an attempt is made to convert an instance of this class to JSON via `JSON.stringify()`, the “TypeError: Converting circular structure to JSON” error is thrown.
Alleviation of this predicament can be achieved by avoiding the creation of circular structures, or by handling serialization of these structures manually. A manual approach generally implements a ‘toJSON()’ method on the problematic structure (an implicit hint for `JSON.stringify()`), as JavaScript does not inherently have an inbuilt way to handle these references.
According to Douglas Crockford, “The good thing about JSON is that it has no features,” yet programming languages and environments like TypeScript and Node.js leverage their features such as recursive object literals and prototypes, thus inadvertently guiding us towards this particular quagmire. The “TypeError: Converting circular structure to JSON” issue is just one example where JS developers need to remember that although JSON syntax is derived from JavaScript, they are not the same.
Understanding TypeError: Converting Circular Structure to JSON
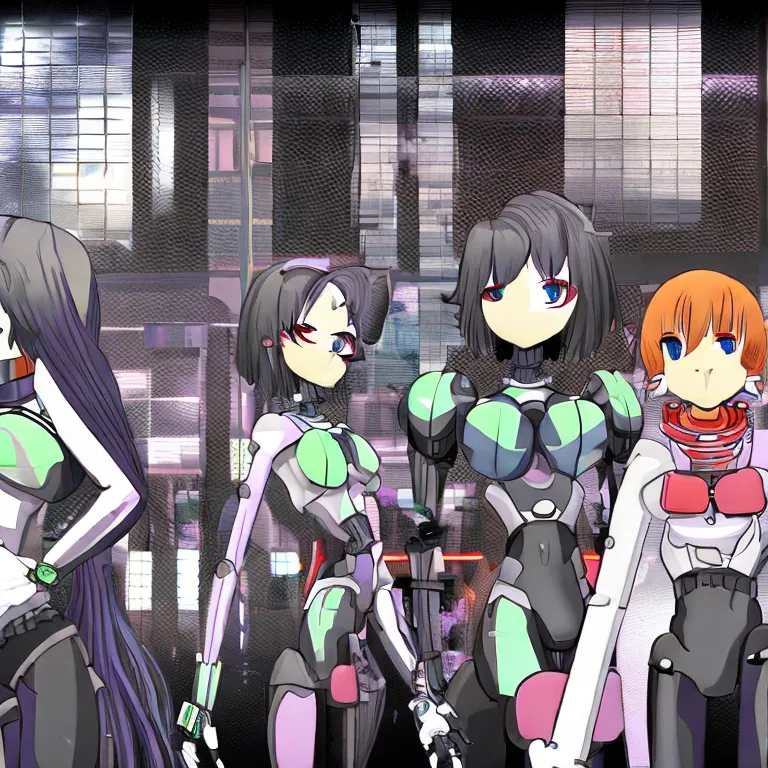
The TypeError: “Converting circular structure to JSON” is a frequent and ubiquitous error encountered when dealing with JavaScript, which, by extension, affects TypeScript as well since the latter is a superset of the former. This issue becomes particularly manifest especially when we delve into complex data structures or network requests, specificially in areas where we are dealing with an object constructed by ClientRequest.
As its name indicates, the error arises from the presence of circular reference within our code. Circular reference, put simply, happens in scenarios where two or more objects refer back to each other, thereby leading to an infinite loop during the conversion process. The standard method that JavaScript (and by extension, TypeScript) uses to convert objects into a string representation is
xxxxxxxxxx
JSON.stringify()
. However, this method encounters a hurdle when it comes across circular references, and thus, triggers the TypeError: “Converting circular structure to JSON”.
“If you’ve seen the ‘Converting circular structure to JSON’ error for the first time, don’t panic. It’s not as cryptic as it appears. It simply means your code’s structure goes around in circles.” – Addy Osmani
Remember the error message specifically mentioned starting at ‘Object with Constructor ClientRequest’? That provides us some insight into the origin of the problem.
ClientRequest is a core part of Node.js which is used when there’s a necessity for making HTTP requests. The error suggests that there’s a circular reference inside a ClientRequest object. To map out a clear idea, let’s consider an example:
A hypothetical code implementing ClientRequest:
xxxxxxxxxx
let http = require('http');
let req = http.request(options);
console.log(JSON.stringify(req));
When the script attempts to turn the ‘req’ object (instance of ClientRequest) into a JSON string, it throws the said error because ‘req’ has some properties that are circularly referenced.
So, how do we remedy this situation? A common approach is to write a function with a replacer argument for
xxxxxxxxxx
JSON.stringify()
, which manipulates the stringification process by letting you decide which values should be included or replaced in the stringify result. This function allows us to avoid adding any structure carrying a circular reference in the JSON output.
An illustration would be:
xxxxxxxxxx
function safeStringify(obj) {
let cache = [];
return JSON.stringify(obj, (key, value) => {
if (typeof value === 'object' && value !== null) {
if (cache.indexOf(value) !== -1) {
return;
}
cache.push(value);
}
return value;
});
}
console.log(safeStringify(req));
This solution ensures that we handle circular structures cautiously when converting them into JSON within a TypeScript file.
While this error is not uncommon, given the nuanced structure of working with HTTP requests textually through JavaScript and TypeScript, understanding it will certainly elevate your coding capabilities. It’s about recognizing these patterns and learning how to navigate them that ultimately leads to proficient debugging and more elegant codebases.
For an in-depth exploration on the subject, consult this detailed guide at [MDN Web Docs](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Errors/Cyclic_object_value).
Diagnosing the Issue: Exploring Circular Objects in JavaScript
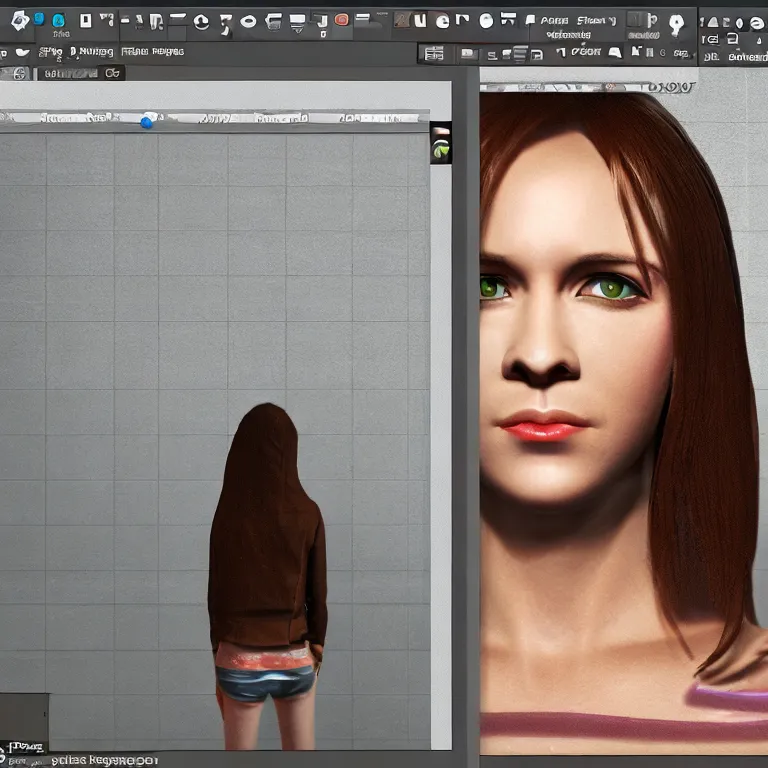
When dealing with the TypeError: Converting circular structure to JSON issue, it’s essential to understand that this problem is commonplace in JavaScript and TypeScript languages. This error typically arises when attempting to stringify an object that has a circular reference.
Let’s explore what a circular reference is:
A circular reference occurs when an object in JavaScript contains a property which points back to the same object. This can be illustrated as:
xxxxxxxxxx
const myObject = {};
myObject.myProperty = myObject;
Essentially, this creates an infinite loop, where “myObject.myProperty” points to “myObject”, and vice versa. Now, if you were to attempt converting this object into a JSON string using `JSON.stringify()`, it would raise an error as the conversion process leads into an endless loop, causing a stack overflow.
xxxxxxxxxx
console.log(JSON.stringify(myObject)); // TypeError: Converting circular structure to JSON
In TypeScript, especially within a Node.js environment, objects such as the ClientRequest instance are likely to have circular structures due to inherent configurations for handling HTTP requests. Then, attempting to convert these instances would result in the error message ‘TypeError: Converting circular structure to JSON –> starting at object with constructor ‘ClientRequest’.
To resolve this type of error, consider using special libraries such as [flatted](https://www.npmjs.com/package/flatted), which handle circular references during JSON stringification.
Alternatively, employ the second parameter of JSON.stringify(): a function (replacer) that alters the behavior of the stringification process.
Mentioned by Douglas Crockford, creator of JSON:
“By default, all enumerable own properties of an object are selected. The use of a replacer function selects properties based on more flexible criteria.”
Here is how it works:
xxxxxxxxxx
function replacer(key, value) {
if (value === myObject) {
return; // if value is a reference to myObject, don't convert it
}
return value;
}
console.log(JSON.stringify(myObject, replacer)); // "{}"
In this example, the replacer function avoids stringifying the circular property, thereby preventing the error.
Debugging in TypeScript and JavaScript can often be tricky and understanding these pitfalls well is crucial for effective problem resolution. Working with circular objects is no different – patience, practice, and use of appropriate tools or functions will help ensure smooth work with circular objects.
Delving Deeper into ClientRequest Object and its Complex Structures
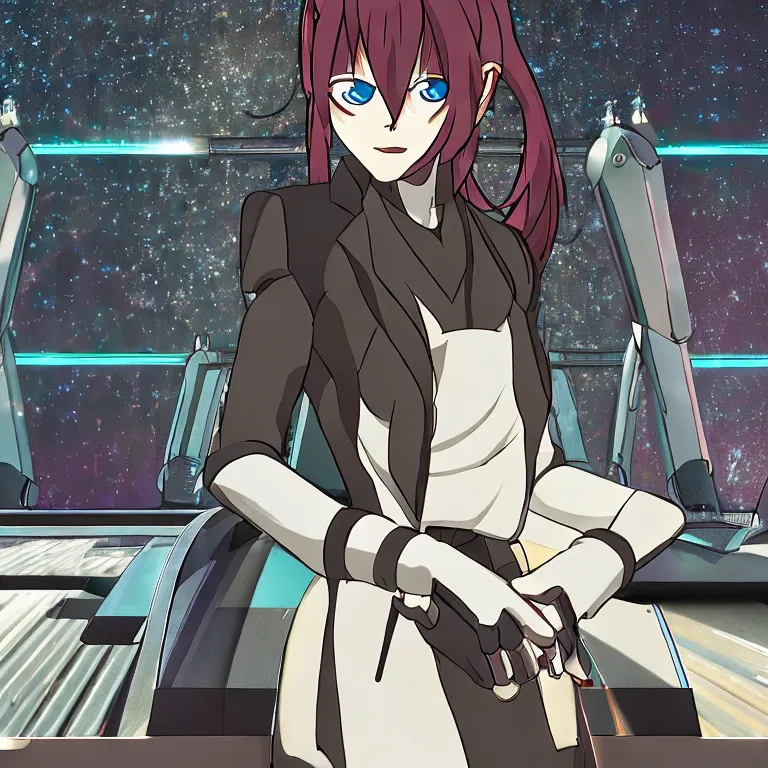
The
xxxxxxxxxx
TypeError: Converting circular structure to JSON
issue often occurs when you attempt to stringify a complex JavaScript object which contains cyclic references, also known as circular structures. The Node.js
xxxxxxxxxx
ClientRequest
object, due to its complex structure and interconnections with various network-related entities, qualifies as this type of object.
Understanding ClientRequest and Circular Structures
A ClientRequest instance is a highly intricate entity in the HTTP module of Node.js. It’s used to craft HTTP client requests. Its intertwined nature comes from its many properties linking it to other objects such as
xxxxxxxxxx
Socket
,
xxxxxxxxxx
IncomingMessage
, etc.
The phenomenon of circular structures has its roots in the object-oriented paradigm of JavaScript where an object can reference other objects through properties, creating a possibility for loops. For example:
xxxxxxxxxx
const objA = {};
const objB = { A: objA };
objA.B = objB; // objA now has a property B which points to objB, which has a property A pointing back to objA
This property referencing creates a loop (circle), hence the name “circular structure”.
The JSON Problem With Circular Structures
When it comes to the JSON.stringify() method, there’s a hiccup – it cannot handle circular structures. This is primarily because JSON, being a data interchange format, is not expected to contain functions or object references, thus making it ill-suited to accommodate circular structures. Trying to stringify a ClientRequest object, or any other object with inherent circularity, will result in the
xxxxxxxxxx
TypeError: Converting circular structure to JSON
error.
Solving The TypeError
There are various ways to resolve or bypass this issue:
- Util-modules: Use utility modules like ‘util'(*source*) which provides a method inspect() that can handle circular references.
- Custom toJSON method: Implement a custom toJSON()(*source*) method in your object’s prototype. It overrides the default behavior of JSON.stringify().
- Circular-JSON libraries: There are libraries such as ‘circular-json'(*source*) tailored to handle circular structures.
Do recall, prevention is better than cure. Hence, when working with complex objects like ClientRequest, it may be wise to design them in a way that avoids circularity where possible.
As Steve McConnell, noted software engineer and author said, “Good code is its own best documentation.” Further external help on preventing circular structures can be found here.
Effective Solutions to Solve “Converting Circular Structure To JSON” Errors
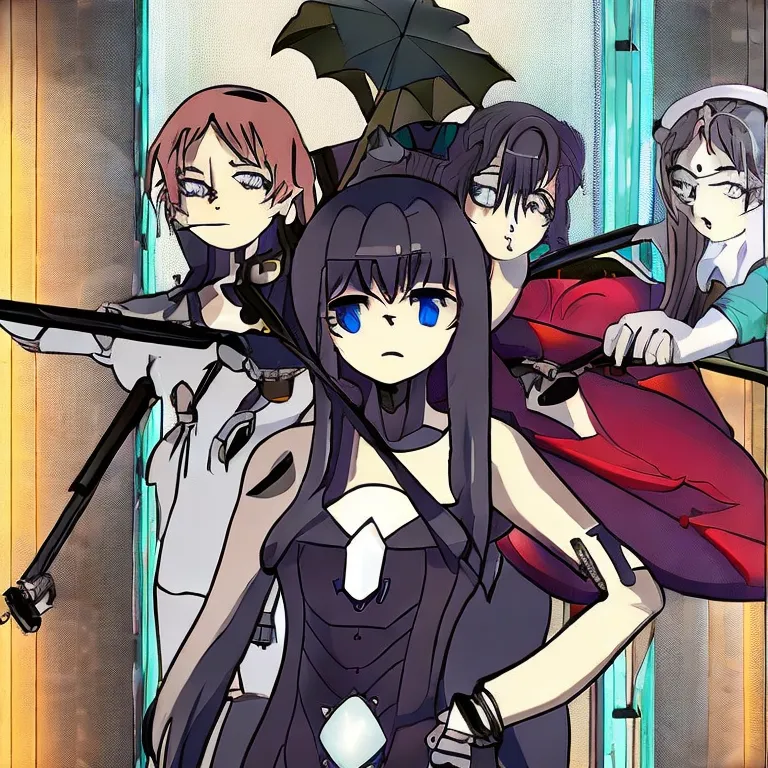
Overcoming the “TypeError: Converting circular structure to JSON” error, precisely within the context of starting at object with constructor ‘ClientRequest’, can be a considerable obstacle for TypeScript developers. This issue generally stems from trying to stringify an object that possesses recursive, circular dependencies.
Identifying the Root Cause
JSON.stringify() does not perform well when faced with objects that include circular references. This type of reference implies that a property of the object points back to the object itself or another property which eventually leads back to the original object.
The ‘ClientRequest’ constructor is utilized in the HTTP client module of Node.js, frequently prone to this issue owing to its verbose and complex structure filled with such circular properties.
Alternatives for Managing These Issues
There are efficient methods to manage this challenge:
– Utilize a Replacer Function in JSON.stringify():
xxxxxxxxxx
let cache = new Set();
JSON.stringify(obj, (key, value) => {
if(typeof value === 'object' && value !== null) {
if(cache.has(value)) {
// Duplicate reference found
return; // Avoids circular structure
}
// Store value in our set
cache.add(value);
}
return value;
});
cache.clear();
This code uses Set to keep a record of all unique items that have been stringified already, thus preventing duplication of entries during conversion.
– Employ a specialized library: Libraries such as JSON.prune or json-stringify-safe specifically handle circular JSON scenarios efficiently.
Severing Ties with Circular References
If your use case permits, you could implement ways to reduce or eliminate the circular references while structuring your code. This might involve re-planning how data is structured or using non-circular data structures.
As prolific computer scientist Edsger W. Dijkstra reportedly once said, “Simplicity is a great virtue… One eventually finds the simplest solutions to complex problems.”
Understanding the nature of circular JSON errors and implementing robust methods to detect, manage, and prevent them can help TypeScript developers maintain efficient, readable, and reliable code.
Conclusion
Encountering the
xxxxxxxxxx
TypeError: Converting circular structure to JSON --> starting at object with constructor 'ClientRequest'
issue typically highlights a structural hiccup in data representation when dealing with JavaScript Object Notation (JSON). This is fundamentally caused by JavaScript’s incapacity to convert a circular reference into JSON format, given JSON cannot intuitively handle cyclic structures. This means that certain types of self-referencing objects such as the infamous “ClientRequest” constructor instances present an inherent conflict when conversion to JSON is attempted.
There are specifically designed strategies for managing this pitfall:
– Implementing a custom
xxxxxxxxxx
toJSON
method is one possibility. The concept behind this strategy is that it redefines how objects get serialized, thereby effectively circumventing the circularity problem.