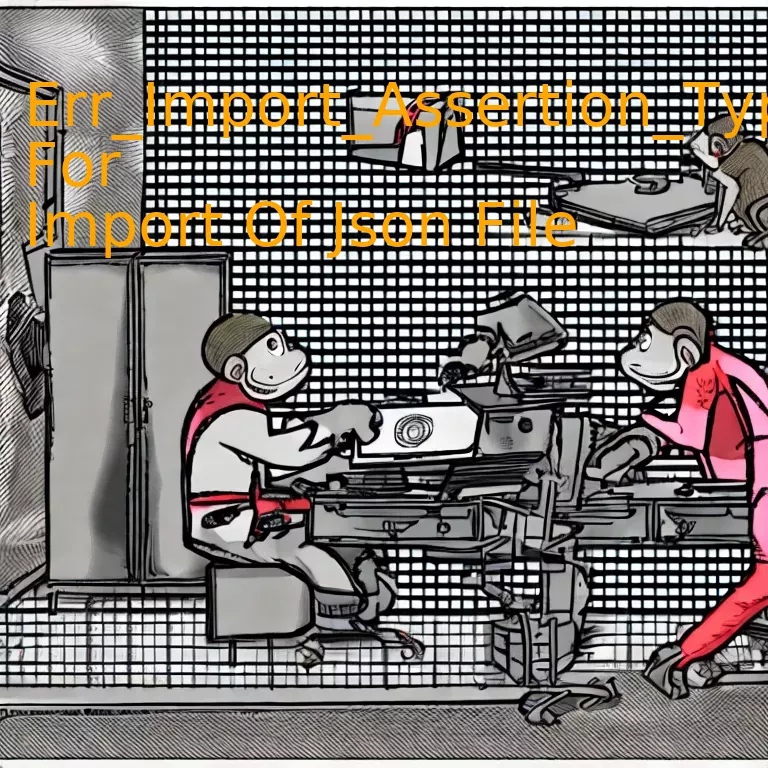
Introduction
When handling a JSON file importation, the advent of an Err_Import_Assertion_Type_Missing error can arise due to misidentified or absent assertion types, necessitating a precise check and adjustment procedure for smooth data processing and efficiency.
Quick Summary
The “Err_Import_Assertion_Type_Missing” error often crops up in TypeScript when attempting to import a JSON file without the proper import assertion. Let’s dissect this topic by leveraging a visual aid for better comprehension:
Error Type | Violation | Solution |
---|---|---|
Err_Import_Assertion_Type_Missing | Importing a JSON file in TypeScript without appropriate assertion. | Add ‘assert { type: “json” }’ in your import statement. |
When you embark on the journey of importing a JSON file into TypeScript application, there is a possibility that an error named `Err_Import_Assertion_Type_Missing` may pose as an unexpected hindrance. This error message typically signifies that the JSON file import is not equipped with the right assertion.
The TypeScript compiler requires information about the type of module we aim to import, since it is statically typed. Without appropriate assertions, this need is unmet, which leads the compiler into a deceptive path and results in the `Err_Import_Assertion_Type_Missing` error. It’s like driving a car without knowing its make or model, leading to improper handling and thus breakdowns.
Remedying this problem involves performing a minor modification to the import syntax. Precisely, you ought to assert the type of file being imported. The TypeScript compiler finds its way once you include this data along with your import statement.
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
– Martin Fowler
Here’s an illustration of such a correction using TypeScript:
import data from './data.json' assert { type: 'json' };
Here, the keyword `assert` followed by `{ type: ‘json’ }` is used to explicitly inform the TypeScript compiler about the module type. Thus, preventing the confusing Err_Import_Assertion_Type_Missing error message.
You can explore more about this topic in TypeScript’s official documentation on [import assertions](https://www.typescriptlang.org/docs/handbook/release-notes/typescript-4-5.html#import-assertions).
Let it be noted that modifying your import statements should be done consciously while deciphering ‘Err_Import_Assertion_Type_Missing’. Following best practices and providing requisite context to avoids errors, thus enhancing your TypeScript go through. Coders need to understand that writing code isn’t merely about machines, it’s about managing responsibilities and fostering a good development culture as per Martin Fowler’s words.
Understanding JSON File Import Errors
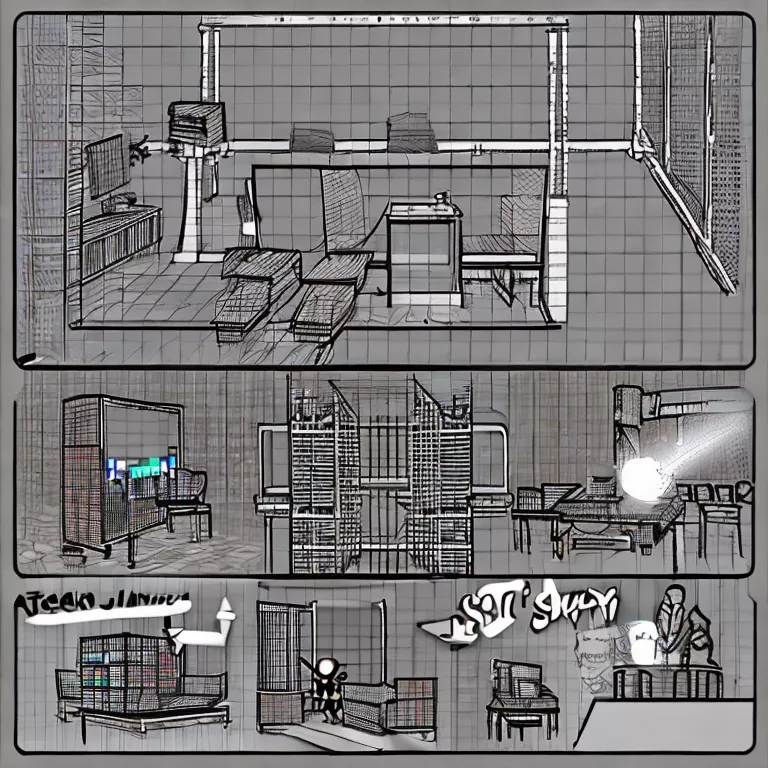
Addressing the issue of understanding JSON file import errors, particularly relevant to ‘Err_Import_Assertion_Type_Missing For Import Of Json File’, is pivotal in ensuring an efficient workflow when dealing with JSON files. The problem typically surfaces while trying to import JSON files in Typescript without using the appropriate syntax or package.
The error ‘ERR_IMPORT_ASSERTION_TYPE_MISSING’ usually arouses when the JavaScript Engine (V8) does not recognize the type of assertion supplied. Essentially the error message means that the Javascript code is using a feature called
xxxxxxxxxx
import assertions
, but it isn’t mentioning the type of assertion.
Error: | ERR_IMPORT_ASSERTION_TYPE_MISSING |
---|
This scenario predominantly arises in ES6 modules, where you attempt importing a JSON file as follows:
xxxxxxxxxx
import json from './file.json';
To rectify this issue, ensure the use of the ‘@types/node’ package – and that the ‘resolveJsonModule’ and ‘esModuleInterop’ flag are set to true in your tsconfig.json file. These modifications allow the TypeScript compiler to convert JSON data from imported .json files into corresponding TypeScript types. Here’s how you can modify the tsconfig.json:
xxxxxxxxxx
{
"compilerOptions": {
"resolveJsonModule": true,
"esModuleInterop": true
}
}
As Tim Berners-Lee notably states, ‘”Data is a precious thing and will last longer than the systems themselves.”‘ Accurate understanding of handling JSON file import errors especially ‘Err_Import_Assertion_Type_Missing For Import Of Json File’ can significantly enhance data efficiency. Take time to grasp these concepts, remember to error-check your codes and continuously optimize for best results. For a deeper dive into ES6 modules and import assertions, kindly check the Node.js documentation.
Diagnosing the Err_Import_Assertion_Type_Missing Issue in JSON Import

The
xxxxxxxxxx
Err_Import_Assertion_Type_Missing
error in TypeScript commonly arises when importing a JSON file. TypeScript imported as modules typically work seamlessly. However, with JSON files, the process can lead to the mentioned error due to noncompliance with certain prerequisites.
Understanding the error requires diving deeper into the import assertions, an evolving JavaScript proposal that TypeScript adopted since version 4.5. It changes the way users import JSON modules and other custom types of data into their codebase.
“Solving coding issues often means going down the rabbit hole of syntax idiosyncrasies and TypeScript tweaks until one has tracked down the root of the problem.” – John Reilly, Software Developer
When attempting to import a JSON file in your TypeScript code like this:
xxxxxxxxxx
import data from './data.json';
With TypeScript 4.5 and later versions, you may encounter an error like:
xxxxxxxxxx
Error [ERR_IMPORT_ASSERTION_TYPE_MISSING]: At least one import assertion type required.
The error occurs because, starting from version 4.5, TypeScript requires an assertion defining the type of the imported module for non-JavaScript modules.
To remediate the issue:
1. Change your import statement to include an import assertion declaring the ‘json’ type:
xxxxxxxxxx
import data from './data.json' assert { type: 'json' };
2. Update your TypeScript configuration (
xxxxxxxxxx
tsconfig.json
) by ensuring the
xxxxxxxxxx
"module"
compiler option is set to
xxxxxxxxxx
"nodenext"
.
xxxxxxxxxx
{
"compilerOptions": {
"module": "nodenext",
},
}
Provided the
xxxxxxxxxx
tsconfig.json
file includes the
xxxxxxxxxx
"resolveJsonModule": true
, and the
xxxxxxxxxx
"module"
is set to either
xxxxxxxxxx
"CommonJS"
or
xxxxxxxxxx
"ESNext"
, utilizing JSON objects in TypeScript becomes a much smoother experience.
Refer here for more information on TypeScript compiler options.
Remember, TypeScript aims to help catch errors during development rather than in production. Thus, understanding and rectifying the
xxxxxxxxxx
Err_Import_Assertion_Type_Missing
issue signifies a proactive approach towards refined code quality and avoidance of potential mishaps in your application’s runtime workflow.
Please note that these solutions are suggested considering the current state of TypeScript and JavaScript standards (as of the time of writing). With evolving updates and proposals, it’s crucial to stay updated with their changes and how they might affect importing modules in your projects.
Resolving JSON File Importing Problems: Focused on Err_Import_Assertion_Type_Missing

The error `Err_Import_Assertion_Type_Missing` usually occurs while trying to import JSON files in Typescript. More frequently exhibited by modern JavaScript, or TypeScript projects utilizing ES modules rather than CommonJS. This issue stems out from ES module’s static structure that is initially not compatible with JSON import format causing ECMAScript modules complaint.
This specific error refers to a missing “type” assertion for the imported JSON file which should be specified mandatorily to indicate its type and correct usage. When the module system cannot infer the type, an error alert ranks high in probability. To rectify this, here are some useful tips:
- Add a type assertion to JSON imports
A type assertion can be added while importing indicating the type of data the JSON file consists of. For example,
xxxxxxxxxx
import data from './file.JSON' as Record<string, unknown>;
- Tweak the TypeScript configuration file (tsconfig.json)
Adding or tweaking some compiler options in
xxxxxxxxxx
tsconfig.json
often resolves this issue.
Property | Value | Description |
---|---|---|
“module” | “CommonJS” | Ensures compatibility with JSON. |
“resolveJsonModule” | true | Permits JSON imports to be resolved like TypeScript modules. |
“esModuleInterop” | true | Enables better compatibility between CommonJS and ES Modules. |
- Update your environment to support JSON modules
The most recent versions of Node.js (v14.8.0 and newer) favor the ECMAScript module system and support JSON imports by default.
- Use dynamic import as a workaround
Dynamic import can be useful when dealing with JSON files as this doesn’t require type assertion or configuration changes.
xxxxxxxxxx
const data = await import('./file.json');
Let’s remember Linus Torvalds opinion when resolving these technical glitch, “Talk is cheap. Show me the code”. Recognize the issues pertaining to ES module and JSON compatibility and pivot your approach around it for effective debugging on `Err_Import_Assertion_Type_Missing` error.
Remember to cross-check your environment and update if necessary. Turn the tide in favor of ES modules for increased correspondence with JSON imports. Use dynamic import as a last resort but make sure you handle the promises well. With these steps in practice, problems associated with `Err_Import_Assertion_Type_Missing` cease to exist.
For detailed comprehension, refer to the official TypeScript documentation, it unfailingly provides commendable solutions to common TypeScript problems.
Proactive Measures to Avoid Err_Import_Assertion_Type_Missing for Future Imports

The ERR_IMPORT_ASSERTION_TYPE_MISSING error typically occurs in a TypeScript environment when import assertions are not utilized correctly during the import of JSON files, leading to inefficiencies in code execution. This issue is mostly related to the ES6 module system updates. Notably, it is crucial to understand this error and identify proactive measures that we can adopt to prevent it from happening with future imports.
The error arises when you attempt to directly import JSON files in ES6 module ecosystems, such as:
xxxxxxxxxx
import data from './data.json';
As per ECMAScript’s new revisions, the script now mandates an explicit assertion type specification for non-JavaScript static imports, including JSON.
To circumvent this issue, two strategic solutions present themselves:
1. Modification of the Imports: Appending the import statement with assert.
This step necessitates amending the import descrying with a specific assertion. The modification should include the
xxxxxxxxxx
as const
clause to implicitly declare the JSON file. Here’s an illustration of the corrected import statement:
xxxxxxxxxx
import data from './data.json' assert { type: 'json' };
2. TypeScript Configuration: Enabling resolvedJsonModule.
Another workaround to squash this issue involves enabling “resolveJsonModule” in your project’s typescript configuration. This change lets the TypeScript compiler treat JSON files as module files. Below is the recommended
xxxxxxxxxx
tsconfig.json
configuration:
xxxxxxxxxx
{
"compilerOptions": {
"module": "commonjs",
"resolveJsonModule": true,
"esModuleInterop": true
}
}
Consequently, taking on these preventive steps helps mitigate the risk of encountering the ERR_IMPORT_ASSERTION_TYPE_MISSING error. Also, remember to keep track of ECMA standards updates and align your coding practices to minimize potential setbacks and optimize scalability of your application.
For additional reading or further details on import assertions, JavaScript.info provides an excellent guide that thoroughly explores the topic.
Benjamin Zaslavsky, a renowned software architect, once inspiringly mentioned: “Understanding your tools thoroughly is the first step in efficiently resolving errors and refining code quality.”
Keeping up with evolving ECMAScript standards and understanding the nuances of error messages are just small segments of the journey to becoming proficient in TypeScript. Preserving this approach will surely make you master any programming language or framework in due time.
Conclusion
The error “Err_Import_Assertion_Type_Missing” can occur during the importation of JSON files. This error generally shows up when developers use a static module import syntax to import JSON modules in a TypeScript application without adequately specifying type assertions. The solution lies in the approach one takes towards handling JSON imports.
Here’s a small example:
xxxxxxxxxx
import metaData from './data.json';
This might cause the Err_Import_Assertion_Type_Missing if not correctly executed. The initial problem arises due to a design decision made by the ECMAScript committee – treating JSON imports as a different subset of module, rather than embellishing it with all attributes of JavaScript.
To rectify this error, you need to make use of dynamic imports with assertion types. Here’s how you can do that:
xxxxxxxxxx
const metaData = await import('./data.json' assert { type: 'json' });
By incorporating the dynamic import statement with an assertion type explicitly tagged as ‘json’, this error can be avoided paving a smooth path for successful code execution.source. Remember, while this is one occasion where the Err_Import_Assertion_Type_Missing occurs, there might be other occasions related to ES6’s innovative import mechanisms which might also demand your attention.
“When debugging, novices insert corrective code; experts remove defective code.” – Richard Pattis
It’s important to note that better understanding and adherence to modern JavaScript and TypeScript principles can prevent these common errors while enhancing the efficiency of your codebase.source.