Introduction
To circumvent the error ‘Cannot Use Import Statement Outside Modules,’ ensure you’re using a version of Node.js supportive of ES6 Syntax, or employ a Bundler like Webpack or a compiler such as Babel. This can help solve your semantic predicaments in JavaScript and promote effective coding practices.
Quick Summary
Problem | Cannot use import statement outside module |
---|---|
Identification | Error message displays when trying to use ‘import’ to load a JavaScript (ES6) module in a Node.js script |
Cause | Node.js by default treats JavaScript files as commonJS modules. The ‘import’ keyword is only available for ES6 modules. |
Solution | Using the ‘.mjs’ extension, Using esm library, Configuring package.json file with type: module |
The problem “Cannot use import statement outside modules” arises when you attempt to load an ECMAScript6 (ES6) module through the ‘import’ keyword within a Node.js script. By default, Node.js treats all JavaScript files as commonJS modules that do not support the ‘import’ keyword.
Though appearing as a roadblock, the modern JavaScript ecosystem has engineered multiple ways to combat this issue. One of these measures involves using the ‘.mjs’ extension for files that contain ES6 modules. This way, Node.js gets indicated that these are ES6 modules and can implement ‘import’ accordingly.
There is also an option to utilize third-party libraries such as ‘esm’ that enable Node.js to understand ‘import’ commands. You can simply install and configure it within your project which then can run ES6 modules flawlessly.
Alternatively, modifying the ‘package.json’ file in your project can be a viable solution to rectify the issue. Adding the “type”:”module” line to package.json instructs Node.js to treat .js files as ES6 Modules.
As a
"type": "module"
line in your package.json, it promises a seamless experience by treating all .js files in your project as ES6 modules, therefore allowing use of the import keyword.
These mechanisms help you maneuver through the modern JavaScript ecosystem while maintaining optimal interoperability with traditional systems. In the words of Brendan Eich, the creator of JavaScript, “Always bet on JS.” It is a testament to the adaptable nature of JavaScript and its ability to evolve and fit into varying contexts.
Unraveling the ‘Cannot Use Import Statement Outside Modules’ Error
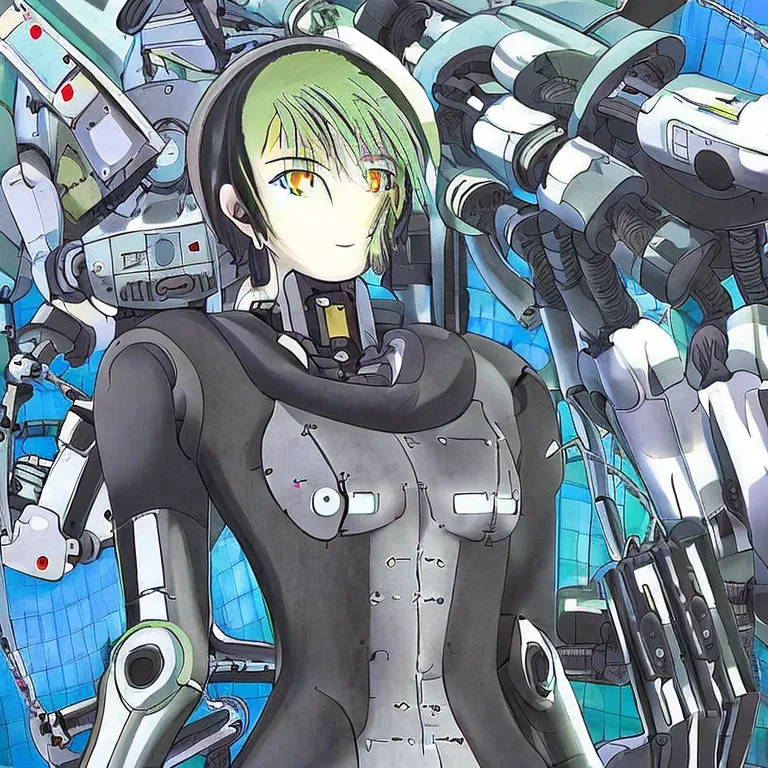
Delving into the intricacies of the ‘Cannot Use Import Statement Outside Modules’ error exposes underlying compatibility issues between TypeScript and JavaScript modules. Dissecting this error requires an understanding of how ECMAScript (ES6) modules work, as well as their incompatibility with CommonJS modules.
A typical error message might look something like this:
Error [ERR_REQUIRE_ESM]: Must use import to load ES Module: /path/to/module.js require() of ES modules is not supported. require() of /path/to/module.js from /path/to/app.js is an ES module file as it is a .js file whose nearest parent package.json contains "type": "module" which defines all .js files in that package scope as ES modules. Instead rename module.js to end in .cjs, change the requiring code to use import(), or remove "type": "module" from /path/to/package.json.
The root cause stems from Node.js treating JavaScript files as CommonJS modules by default. As a result, the
import
statement, which is specific to ES6 modules, triggers this error when utilized outside these modules.
Deciphering The Error
– Node.js treats JavaScript files as a CommonJS module by default
– ES6 introduced the
import
syntax for importing modules
– The Catch: JS interpreted by Node.js isn’t bundled the same way a front-end development operation would bundle it in a browser environment
– Considering this, when
import
is used in a script running directly in Node.js, a runtime error ‘cannot use import statement outside a module’ appears
So how can we solve this problem? Let’s explore a few possible ways:
Solution 1: Renaming The File Extension
One immediate solution is to change your file extension from
.js
to
.mjs
, denoting it as an ES module. Node.js takes cues from the extension and treats it accordingly:
// Rename 'app.js' to 'app.mjs'
Solution 2: Setting Type As Module In Package.json
An alternative is to set “type”: “module” in your package.json. This allows the usage of ECMAScript imports and exports syntax within .js files:
{ "type": "module" }
Solution 3: Using ES6 Import Syntax Babelify Your Code
A third option is front-end development tools like Babel, which compile JavaScript down into a backward-compatible version for current and older browsers or environments. These tools allow using cutting-edge features (like import/export) without worrying about compatibility issues. First, install Babel:
$ npx babel --plugins @babel/plugin-transform-modules-commonjs script1.js --out-file script1-compiled.js
Remember the words of John Sonmez, a renowned software developer and author, “No technology is worth learning that doesn’t actually activate the breakthrough you’re trying to achieve.” Understanding and addressing this error effectively can facilitate the seamless integration of TypeScript code into an existing JavaScript environment.
Analyzing Root Causes of ‘Cannot Use Import Statement Outside Modules’ in JavaScript
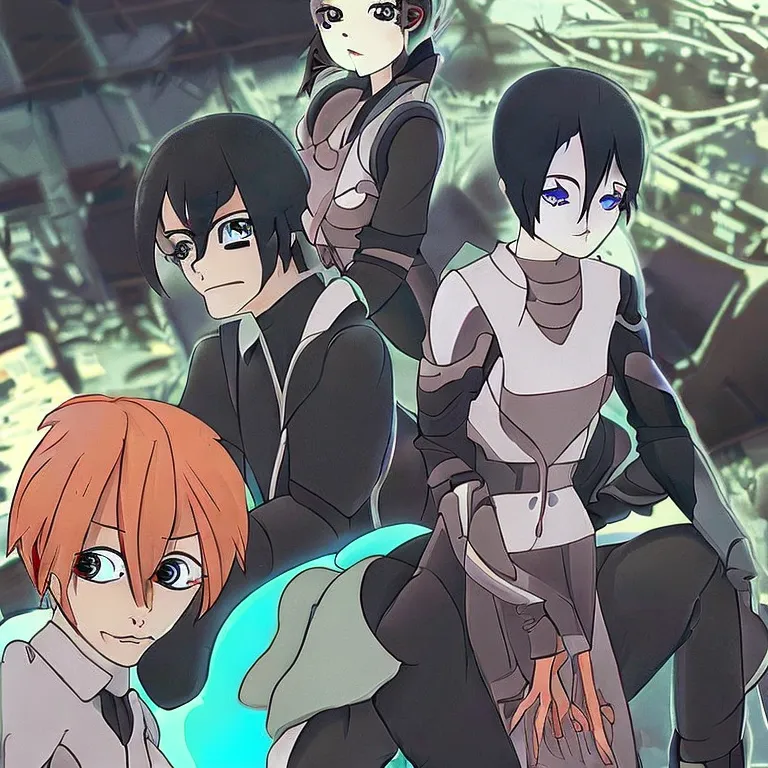
The ‘Cannot Use Import Statement Outside Modules’ error in JavaScript originates due to the inherent nature of ECMAScript modules (ESM), which are part of modern JavaScript and have several specifications, one of them being that they only function within module context.
Here is a brief code snippet detailing this:
// File: calc.js export const add = (a, b) => a + b;
// File: app.js import { add } from './calc.js'; console.log(add(2, 3)); // Output: 5
In this example, encountering the error might cause confusion if the environment or system isn’t set up to work with ESM, leading to the thrown error ‘Cannot use import statement outside modules’.
Here are some primary root causes:
• TypeScript Compiler Configuration: TypeScript includes an in-built compiler that transpiles TypeScript code into JavaScript by evaluating the ‘module’ within your tsconfig.json file. This can inadvertently result in CommonJS module syntax’s usage should the configuration for “module” be set as “CommonJS”, thereby causing the error as it doesn’t support the ‘import/export’ statements intrinsic to ESMs.
• Node.js Version: You might be running a version of Node.js that doesn’t support ECMAScript modules natively. Although Node.js versions 14 and above provide stable support for ECMAScript modules, lower versions originally lacked or provided experimental support before 13, leading to the aforementioned error.
• File Extensions: It may also come down to the particular file extension you’re using. Node.js typically assumes that .js files are CommonJS modules. Hence, attempting to use the ‘import’ statement in such a case can lead to the error ‘Cannot use import statement outside a module’. Such scenarios commonly occur if you misconfigure Babel with TypeScript.
Consider this relevant quote on dealing with coding issues like this, from Grace Hopper, an American computer scientist:
“One accurate measurement is worth a thousand expert opinions.”
In essence, solving issues such as these often requires detailed understanding, investigation and testing – your best tools being patience and perseverance.
Reference links:
Delving into Solutions: Fixing ‘Cannot Use Import Statement Outside Modules’ Problem
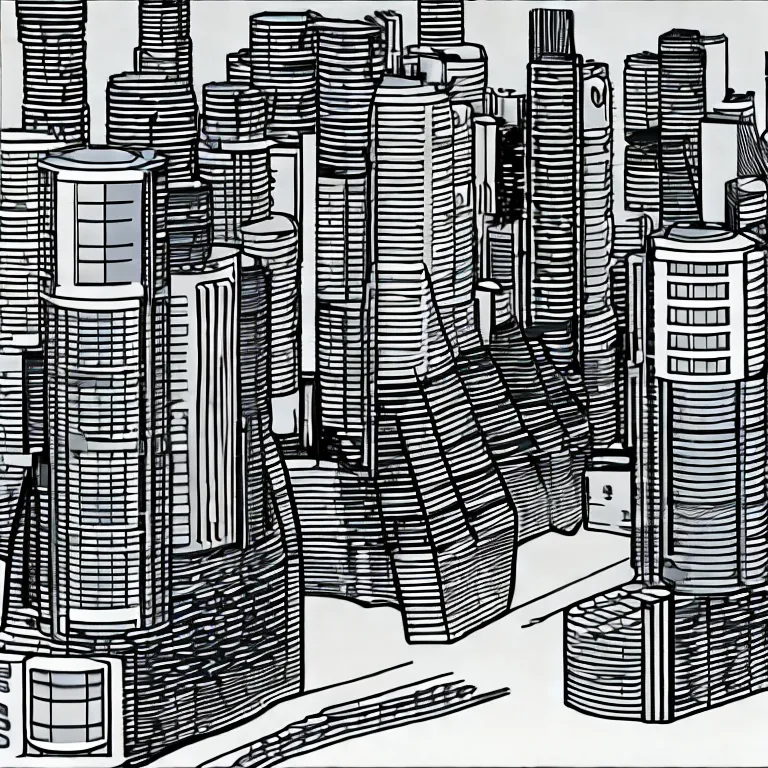
When faced with the “Cannot Use Import Statement Outside Modules” error in your TypeScript journey, you’re likely to feel a dash of panic. Relax, because this issue is entirely fixable. This notorious error springs to action when ES6 import statements are employed outside a module.
To untangle this convoluted situation, comprehension about two distinct types of `module` syntax provided by JavaScript is pivotal: CommonJS and ES modules.
- CommonJS: The traditional way of managing modules in Node.js. Here, the ‘require’ keyword is exclusively used for importing modules.
- ES Modules: This has more advanced capabilities. It capitalizes on an ‘import/ export’ syntax more native to the JS ecosystem.
Now, let’s jump into systematically addressing the problem at hand.
Solution 1: Make Use of Require Instead of Import
The most straightforward method to get around this problem is replacing `import` with `require`.
For instance, here’s how you transform an ES module:
import myModule from './myModule'
Into a CommonJS module:
const myModule = require('./myModule');
However, it’s important to consider that the module exporting must also be translated to CommonJS style, using “module.exports”.
Solution 2: Modify Package.json
Implementing “type”: “module” in package.json declares all .js files as ES modules. You can proceed to use the import statement freely given these predetermined conditions.
It looks something like this:
{ "type": "module", }
Solution 3: Rename Files to .mjs
This is another way to tell Node.js that a file is using an ES module. Once a file’s extension is .mjs, Node.js will know that it’s an ES module and handle it accordingly.
The choice between these solutions remains with the developer. It depends on your codebase, the version of Node.js you’re working with, how open you are to refactoring, and compatibility requirements1.
As Brendan Eich, creator of JavaScript, once profoundly stated, “Always bet on JavaScript”, regardless of the hurdles one encounters during its usage.
How Babel Plays a Role in Resolving ‘Cannot Use Import Statement Outside Modules’ Issue
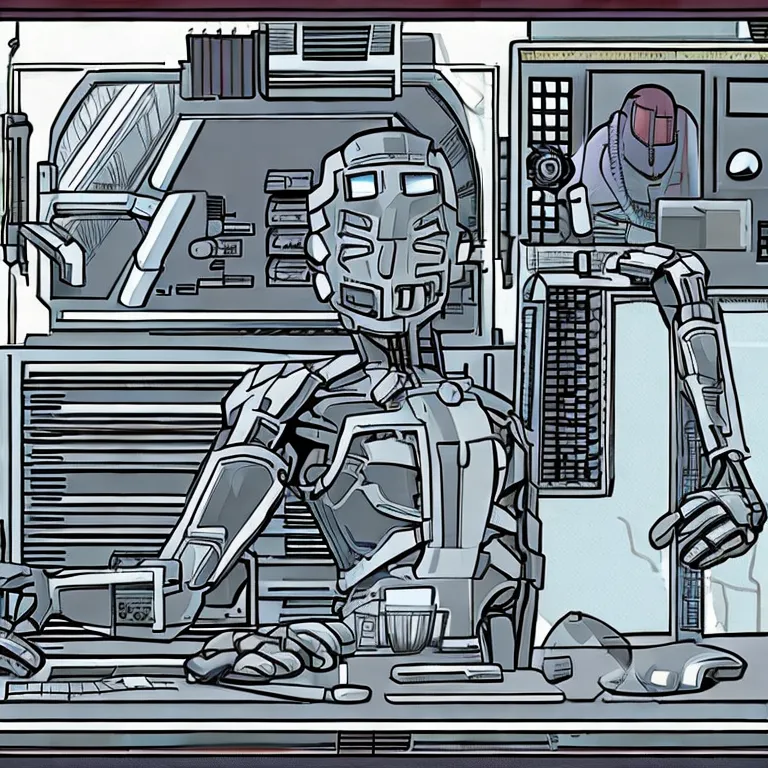
The error “Cannot Use Import Statement Outside Modules” usually occurs when a piece of JavaScript is utilizing ES6 import notation without the environment supporting it. Historically, JavaScript lacked any official module syntax, a feature that ES6 (or ECMAScript 2015) eventually included. Babel comes to the rescue in such instances, being a tool typically used to convert ECMAScript 2015+ code into a backwards compatible version for older JavaScript engines that don’t have the capabilities of understanding this latest version.
Babel executes this function by transpiling your ES6 Imports and Exports to CommonJS which is, until now, the most popular JavaScript module system available in Node.js. Here’s a mini explanation:
– ES6 Code
import express from "express";
– After Babel Transpilation
var express = require("express");
Here, Babel steps are essential to resolving the aforementioned issue. To begin transpiling your ES6 imports and exports to CommonJS –
1. Install Babel and its essentials dependencies:
npm install --save-dev @babel/core @babel/cli @babel/preset-env
npm install --save @babel/polyfill
2. Create a Babel configuration file (.babelrc) in the root directory and include the preset ‘@babel/preset-env’, which allows usage of the most recent JavaScript without needing to micromanage which syntax transforms are presently necessary.
{ "presets": ["@babel/preset-env"] }
3. Instruct Babel to transpile your application via npm by adding a build script to your package.json:
"scripts": { "build": "babel src -d dist" }
Then, command the code using—
npm run build
Jeff Atwood, the co-founder of Stack Overflow, has rightfully said- “Writing code from scratch is a waste of human resource.” So rather than trying to battle against language limitations ourselves, we can utilize tools like Babel to handle these issues for us effectively.”
Conclusion
Relating to the era of modern JavaScript and TypeScript development, one often encounters the conundrum – ‘Cannot Use Import Statement Outside Modules’. Oftentimes, it results from an attempt to use the import statement in a Node.js environment, where CommonJS modules are the default and ES6 module syntax isn’t directly applicable. While Babel and Webpack are popular solutions for those craving TypeScript and ES6 functionalities, an understanding of what lies beneath, simplifies our approach towards resolving the issue.
• In essence, JavaScript utilizes two primary types of modules: ES6 modules (utilize the import keyword) and CommonJS modules (use the require function for imports). Different environments like browsers support ES6 modules, while Node.js prefers CommonJS. Encountering “Cannot Use Import Statement Outside Modules” essentially means you’re trying to use an import statement in a script that’s expected to be a CommonJS module by your environment.
• Traditionally, Node.js didn’t support ES6 modules, causing this discrepancy and leading developers into this territory. However, as per current standings, Node.js does indeed support ES6 Modules since version 13.2, provided files have an ‘.mjs’ extension or the package.json contains “type”: “module”.
An example of how to use import suggests:
html
import express from ‘express’;
• Migration paths aren’t always straightforward, considering interoperability between classic scripts that use
require
and ES modules using
import
. A practical solution is configuring Babel to transpile ES6 into code understandable by Node.js.
• Enterprise scalability necessitates leveraging automation tools. Efficiently integrating compiling tools into your project scaffolding like Webpack or Babel eradicates the perplexity associated with such syntactic idiosyncrasies.
Bill Gates once said – “The first rule of any technology used in business is that automation applied to an efficient operation will magnify the efficiency.” This quote certainly resonates here; as developers, our continuous endeavor should be to magnify our efficiency, minimize complexities, and streamline our codebase, eventually augmenting the technology we utilize.
To wrap it up, the error ‘Cannot Use Import Statement Outside Modules’ brings to light a fascinating spectrum of JavaScript modernization. As developers navigate this landscape, comprehending differences between CommonJS and ES6 modules, integrating transpilation tools like Babel or Webpack can vastly streamline our developmental pipeline.
Explore more about these topics: ES6 Modules, Webpack, Babel