Introduction
Ensure your JavaScript functions fulfill their stipulated tasks effectively by transitioning from a void return to a promise return in function arguments, increasing the precision and reliability of asynchronously processed data.
Quick Summary
When working with asynchronous operations, there may be scenarios when a Promise is returned in a function argument where a void return was expected. This often results from misunderstandings of how Promises function in TypeScript and can lead to unexpected behaviors. To help decipher this concept, let’s examine the differences between void functions and Promise-returning functions in more detail:
html
Return Type | Description |
---|---|
Void | A function declared with void does not return a value. It simply performs an operation without giving any information on its completion. |
Promise | A function returning a Promise indicates an operation that completes in the future. The Promise object represents a possible future value or eventual completion (or throwing) of an asynchronous operation. |
Upon taking a close look at the table highlights, we see the stark contrast between void and Promise-returning functions. Attempting to employ a Promise in place of a void return type is fundamentally inappropriate due to the synchronous nature of void against the inevitable asynchronous aspect of Promise.
In TypeScript, it’s common to see a warning along these lines when a function expecting a void return type receives a Promise instead. The TS compiler expects that the provided function won’t return anything (`void`), but your function does return a Promise. This message is TypeScript’s humble way of enforcing you to handle Promises properly.
For instance, we might have a scenario where we mistakingly use a Promise in a function that should return `void`:
function executeAction(action: () => void) {
action();
}
executeAction(async () => {
return await doSomeAsyncOperation();
});
In such case, we need to ensure that the `executeAction` function correctly supports Promise-returning functions:
xxxxxxxxxx
function executeAction(action: () => void | Promise) {
if (action() instanceof Promise) {
(action() as Promise).then(() => console.log('Async action completed'));
} else {
action();
console.log('Sync action completed');
}
}
executeAction(async () => {
return await doSomeAsyncOperation();
});
By augmenting our `executeAction` function above, it can now safely handle both synchronous and asynchronous operations.
As JavaScript creator, Brendan Eich once said, “Always bet on JavaScript,” highlighting the language’s resilience and adaptability. Despite seeing seemingly complex error messages, remember that with an understanding of core concepts and mechanisms behind these asynchronous operations, TypeScript provides precise tools for managing async tasks in a more predictable way.
Including the checking for promise return within your primary function will aid in circumventing situations where a Promise is incorrectly returned where a `void` type was expected. Understanding the distinctions between synchronous and asynchronous contexts empowers developers to efficiently manage their codebase by using appropriate function types in TypeScript.
Understanding the Concept of Void Return in Functions
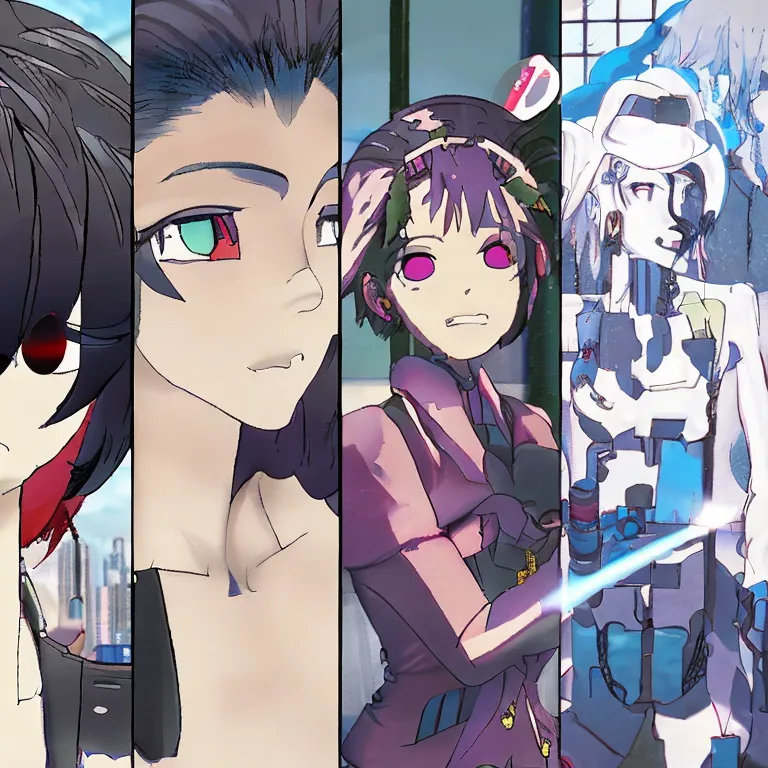
The “void” keyword in TypeScript is used specifically to denote that a function does not return any value. That being said, there can be certain complexities when it comes to asynchronous operations such as Promises. It becomes particularly convoluted when you try to utilize Promise as a returned value in a function where a void return was expected.
Let us break down the concept:
xxxxxxxxxx
function exampleA(): void {
console.log('Hello');
}
In the code above,
xxxxxxxxxx
exampleA
is a classic instance of a void function. It performs a task (logging ‘Hello’ in this case) and does not return anything.
However, issues can arise if a function like the one below is used:
xxxxxxxxxx
function exampleB(): void {
new Promise((resolve, reject) => { // Asynchronous Task
setTimeout(() => resolve('Doing something'), 5000);
})
}
Here, we have a Promise inside a function that is supposed to be a void type. However, Promises are asynchronous constructs and the fact they don’t actually adhere to the ‘void’ constraint makes this confusing. This is because a Promise inherently represents a completion or failure of an asynchronous operation, and might eventually translate to a value in the future.
Typically, functions are marked as ‘async’ when they are doing asynchronous tasks and these return a Promise implicitly. In order to meet the void return type expectation while also making the asynchronous nature of the function clear, you could use await inside of an async function, which gives your function the ability to perform synchronous actions after the Promise has resolved.
xxxxxxxxxx
async function exampleC(): Promise {
await new Promise((resolve, reject) => { // Asynchronous Task
setTimeout(() => resolve('Doing something'), 5000);
})
}
Here,
xxxxxxxxxx
exampleC()
function is explicitly marked as async and its return type is
xxxxxxxxxx
Promise
. It makes use of `await` to ensure that the Promise resolves before the function continues execution.
As Google’s developer advocate, Jake Archibald said, “The ‘async’ keyword turns a method into an asynchronous method. This means you can use the await keyword within,” this snippet perfectly encapsulates how we are actually making the function’s behavior more transparent. More About Async Function (source).
In conclusion, while injecting Promises in functions with expected void returns can be problematic due to their asynchronous nature, using async-await syntax helps cater to both – performing asynchronous tasks and honoring the void constraint.
Delving into Promise: Its Functionality and Implications
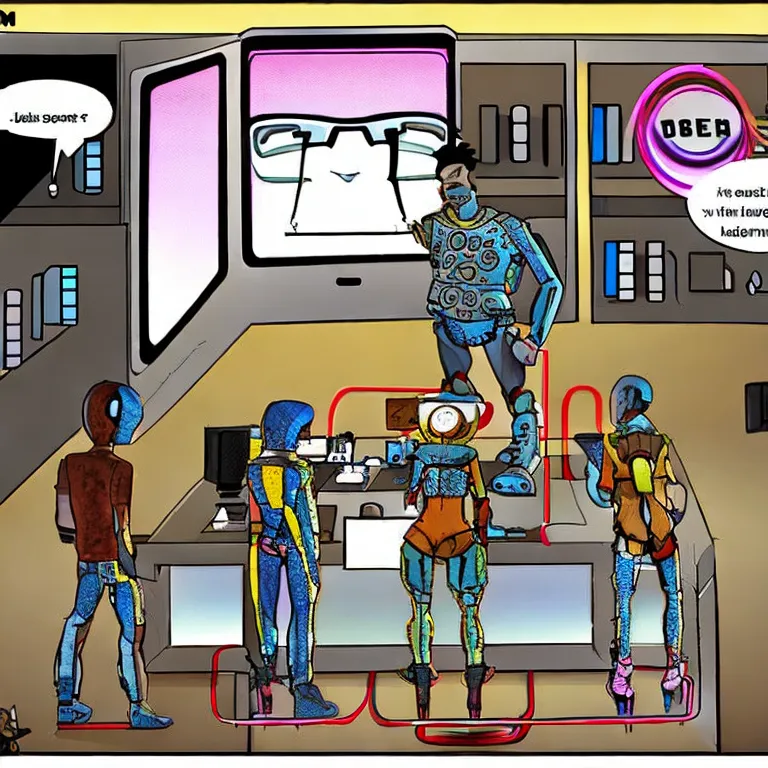
Promises in TypeScript offer a powerful way to deal with asynchronous operations, offering both readability through clear handling of results and errors, and more robust, predictable code. When effectively utilized, they allow programs to handle time-consuming tasks in a way that doesn’t block the rest of the program from executing, therefore vastly improving performance.
“The ‘guts’ of Promises cannot be directly observed in JavaScript. They are only indirectly observed through their influence upon JavaScript execution.” – Jake Archibald
In the specific case where a Promise is returned in a function argument where a void return was expected, it indirectly implies an attempt to perform some asynchronous operation within the function. However, TypeScript strictly enforces type safety and expects the function to have no return value. Hence, this situation becomes a bit tricky and calls for careful evaluation and planning of your code’s setup.
typescript
function doSomething(callback: () => void) {
callback();
}
doSomething(() => {
return new Promise(resolve => setTimeout(resolve, 1000));
});
In the above example, TypeScript will raise a warning indicating that we’re providing a function that returns a Promise (which is something) when our `doSomething` function expects a function that returns void, i.e., nothing.
There are few ways to handle this predicament:
1. Change Function Signature
You can modify your function signature to explicitly state it will return a Promise. This approach works best when it’s feasible to harness the asynchronous operation where originally a synchronous execution was planned.
2. Immediately Invoked Function Expression
Wrap Promise inside an Immediately Invoked Function Expression. It allows the promise to be executed, but the result isn’t returned.
typescript
doSomething(() => {
(async () => {
return new Promise(resolve => setTimeout(resolve, 1000));
})();
});
3. Explicitly ignore the Promise
This approach is only recommended if you don’t care at all about when or even if the Promise settles, and just want to start the process and forget about it.
typescript
doSomething(() => {
new Promise(resolve => setTimeout(resolve, 1000));
return;
});
While Promises facilitate asynchronous operations in a more readable and predictable format, understanding the type-safety intricacies is crucial. Structuring the code correctly will avoid the pitfalls associated with returning a Promise when a void was expected. Understanding the nature of these powerful tools can help developers structure their code for optimal performance and maintainability.
For further information, I recommend this insightful post by [Dr. Axel Rauschmayer on Promise](https://exploringjs.com/es6/ch_promises.html#_our-first-example-httpgeturl).
Remember, “Programs must be written for people to read, and only incidentally for machines to execute.” – Harold Abelson. So let’s write the code that is both functionally correct and easy-to-read.
Transforming Void Return to Promise in Function Arguments
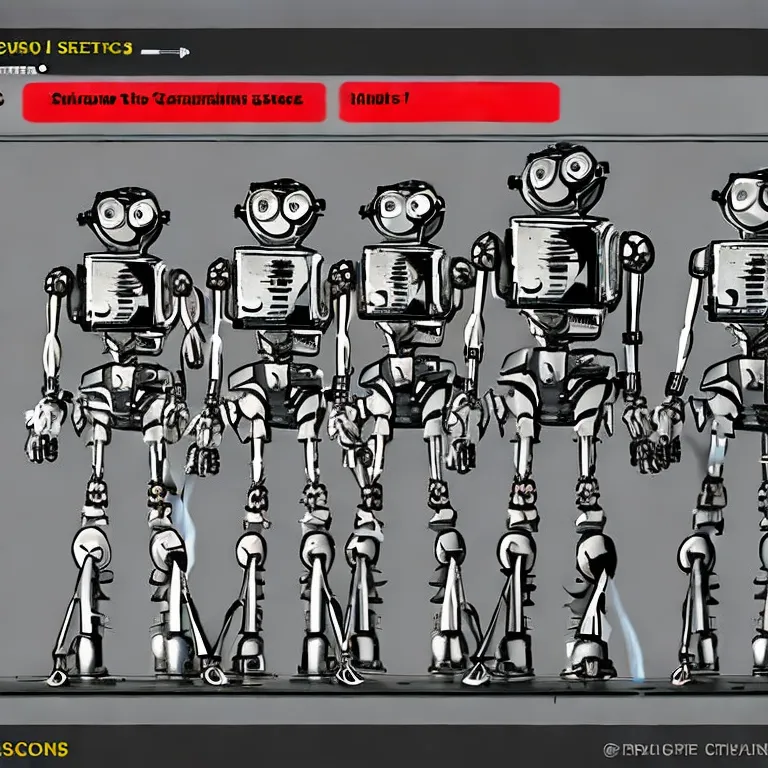
In TypeScript, a language that is built on top of JavaScript by adding static type definitions, you sometimes encounter scenarios where you have a function that expects an argument to be a function returning void. However, you might want this function to support Promises as well. Promises in TypeScript and JavaScript are constructs used for handling asynchronous operations.
First, let’s take a look at a normal function declaration with a void return type:
xxxxxxxxxx
function process(callback: () => void) {
callback();
}
This `process` function accepts a `callback` argument which is expected to be a function that returns void. Invoking `process` would resemble something like:
xxxxxxxxxx
process(() => {
console.log('Task completed');
});
Yet sometimes, it becomes necessary to accommodate for asynchronous operations, in which case it may be beneficial to allow the callback argument to return a Promise as well:
xxxxxxxxxx
function process(callback: () => void | Promise) {
const result = callback();
if (result instanceof Promise) {
result.then(() => {
console.log('Asynchronous task completed');
});
}
}
This updated `process` function accepts a `callback` argument which can either be a traditional synchronous function returning void or alternatively an asynchronous function returning a Promise<void>. If the callback function returns a Promise, we wait for it to resolve before logging “Asynchronous task completed”.
An invocation of `process` with asynchronous functionality could then look something like:
xxxxxxxxxx
process(async () => {
await new Promise(resolve => setTimeout(resolve, 1000));
console.log('Async task completed');
});
To paraphrase Robert C. Martin, a software engineer and author known for his work on Agile principles, “the goal [of refactoring] is to make the code more flexible, easier to maintain, and shareable”, which aligns perfectly with this approach to handling synchronicity within function arguments in TypeScript.
References
Case Study: Unexpected Promises from a Presumed Void
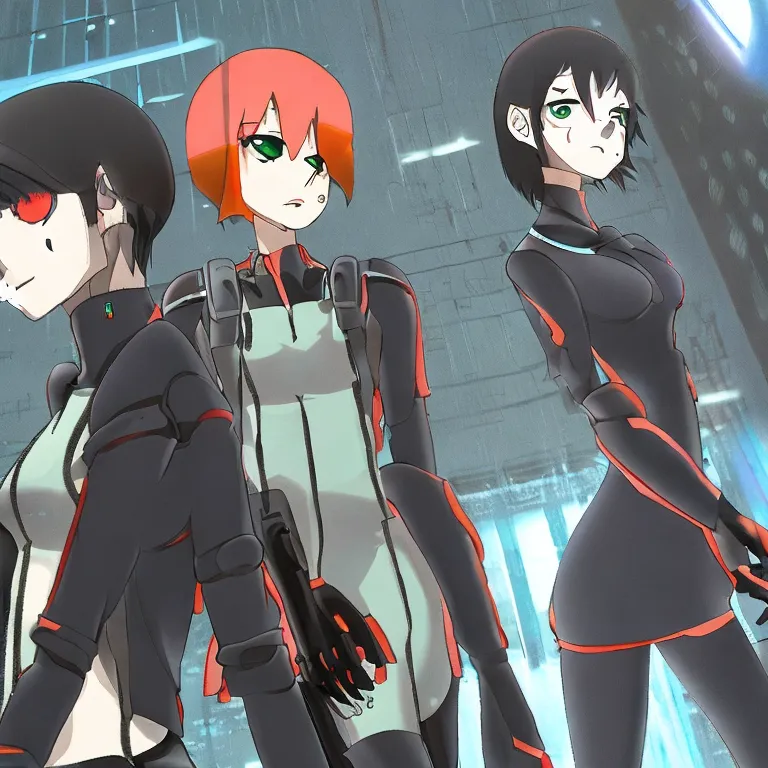
Delving into the intricacies of TypeScript, it is hard to overlook an essential aspect – the handling of Promises when a Void type seems probable. Fascinatingly, this issue becomes more pronounced while dealing with function arguments, where a TypeScript developer might anticipate a Void return but, to everyone’s astonishment, comes across an unexpected Promise.
In order define this scenario better, let’s take a case study of a function `
xxxxxxxxxx
foo()
` which takes another function `
xxxxxxxxxx
bar()
` as an argument.
xxxxxxxxxx
type BarType = () => void;
function foo(bar: BarType) {
bar();
}
In the code snippet above, we have defined that `
xxxxxxxxxx
bar
` should be a function that does not return anything (`void`). However, in some instances, we might be passing functions like below:
xxxxxxxxxx
foo(() => {
return new Promise((resolve) => {
setTimeout(() => {
resolve("done");
}, 1000);
});
});
Our function `
xxxxxxxxxx
foo
` would still execute this without any errors in JavaScript, even though we are returning a Promise instead of `void`. This happens because JavaScript is dynamically typed and doesn’t enforce type checking at compile time. In TypeScript, however, this would result in a compile-time error because the types do not match as assumed.
The primary takeaway here doesn’t just revolve around TypeScript’s flexibility. It also brings the importance for developers to adhere strictly to data types. [_Douglas Crockford_, Software Developer and Author of “JavaScript: The Good Parts”](https://en.wikipedia.org/wiki/Douglas_Crockford), once noted that _”Programming is not about typing… it’s about thinking.”_ Hence, critical reasoning around proper utilization of Void and Promises is crucial to minimize unforeseen coding anomalies.
The observer pattern commonly implemented in JavaScript using Promises, might not be the most suitable approach when TypeScript language offers a stricter type checking and safer coding paradigm.
In similar light, it’s imperative to proactively anticipate such edge cases, especially while dealing with asynchronous operations that return Promises in place of void. Through this, TypeScript empowers developers navigate unexpected routes with enhanced precision, reducing the likelihood of runtime errors sneakily popping up from a seemingly harmless Void.
Conclusion
Promise returned where a void was expected is often an issue developers encounter when dealing with asynchronous programming in TypeScript. When a function argument yields a Promise, it signals unresolved asynchronous computation, which ‘void’ return type does not cater to. Instead, ‘void’ implies that no notable output will be produced by the function, therefore providing neither value nor Promise.
- Understanding the paradox: The contradiction appears when a function is designed to return void, but instead, an unresolved Promise is supplied. This essentially signifies that an operation’s outcome is being waited upon, conflicting with the initial void assurance. Therefore, having a clear understanding of the operating mode of Promises and async/await constructs becomes crucial.
- Error diagnosis: This error typically surfaces during compile check with Typescript. It instructs the developer that a function marked as
xxxxxxxxxx
void
cannot contain a return statement that emits a Promise object.
- Hone solutions: Addressing this requires refining functions to ensure it either returns a Promise if working asynchronously or makes it intrinsically synchronous so that it can dutifully return void without contradiction. For instance:
xxxxxxxxxx
// Asynchronous function returning Promise
async function foo(): Promise<void> {
await someAsyncOperation();
}
// Synchronous function returning Void
function bar(): void {
someSyncOperation();
}
For developing effective and maintainable code, one must strike a balance between robust async/await and applicable types in functions. Recall that the famed British computer scientist Tony Hoare once quipped, “There are two ways to write code: write code so simple there are obviously no mistakes in it, or write code so complex that there are no obvious mistakes in it.” Let that be a gentle reminder as we work to resolve the paradox of unexpected Promise returns in what should be a void function.
Learn more about functions in TypeScript here.