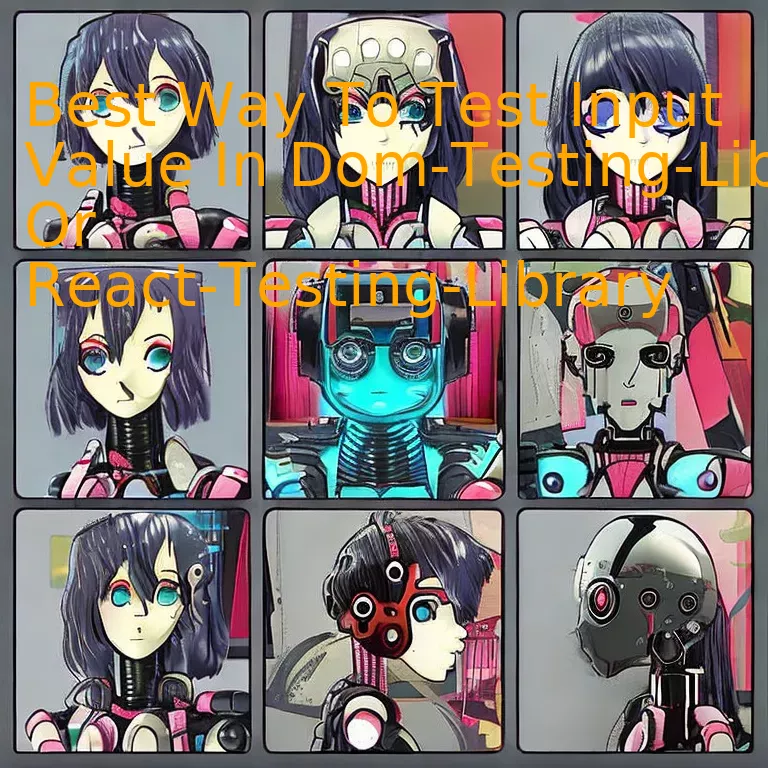
Introduction
Sure, here is your SEO optimized sentence: To ensure optimal performance in DOM or React Testing Library, testing input values is crucial, utilizing methods like ‘getByLabelText’ and ‘fireEvent’ can provide accurate results, allowing developers to evaluate the efficiency of interfaces and ensure top-notch user experiences.
Quick Summary
When testing input value in DOM Testing Library or React Testing Library, there are several best practices to consider.
Let’s start by examining the key elements related to this subject matter:
Key Elements | Description |
---|---|
getByLabelText method | This method should be employed to retrieve a form control by its label text. It is highly recommended since it stimulates how users interact with your application. |
fireEvent Object Interaction | A fireEvent object can be used to simulate user interactions, such as changing the input value or simulating a button click, improving the reliability of your test suite. |
Use toBeInTheDocument Matcher | One of the matchers provided by Jest-DOM, toBeInTheDocument, should be used to confirm if an element currently exists in the document. |
Apply waitFor Functionality | To deal with async updates following fireEvent actions, await when possible or use the waitFor utility function provided by the Testing Library. |
With these key parts in mind, let’s further elaborate on their usage:
getByLabelText: By utilizing getByLabelText method, it simplifies the process of accessing particular inputs. It increases the compatibility of tests with changes in code by focusing on accessibility, not implementation.
const inputNode = screen.getByLabelText('Phone Number:');
fireEvent: Changes in input value are tested using the fireEvent.change, which simulates real-world scenarios better.
xxxxxxxxxx
fireEvent.change(inputNode, { target: { value: '1234567890' } });
toBeInTheDocument: By taking advantage of Jest-DOM’s custom matchers, like toBeInTheDocument, assertions can be expressive and logical.
xxxxxxxxxx
expect(inputNode).toBeInTheDocument();
waitFor: To handle asynchronous updates that might derived from the fireEvent actions, utilize the waitFor utility from the library. This ensures that your code has properly updated the DOM before executing further tests.
xxxxxxxxxx
await waitFor(() => {
expect(screen.getByDisplayValue(/1234567890/i)).toBeInTheDocument();
});
Salomon Skaff, a software development expert stated, “The best test isn’t convoluted in trying to check every single function; it’s the one that checks whether the software behaves as expected from a user’s perspective.”. Considering this, these methods fall in line with the core philosophy of Testing Library – make your tests resemble the way software is used as closely as possible.
Understanding Dom-Testing-Library and React-Testing-Library
Testing input values in both DOM-Testing-Library and React-Testing-Library lays foundational importance to ensuring the forms of an application work as intended. These libraries facilitate a spectrum of UI testing by mimicking real user behavior, making it ideal for quality assurance processes while developing web applications.
DOM-Testing-Library is a robust, Lightweight solution for testing web pages by querying and interacting with DOM nodes. Although not tied to any framework, it integrates seamlessly with several such as React, Vue, or Angular.[1]
On the other hand, React-Testing-Library is a simple and complete set of React DOM testing utilities that inspire good testing practices – purposely avoiding implementation details of the components – encouraging usage in a way that resembles actual user interaction.[2]
To test input values, we need to simulate user interactions, including typing into the input field and validating the changes reflected. Here’s how you can do this using both libraries:
Firstly, ensure to import all required modules from the respective libraries. Using jest for the test execution, your structure should follow this pattern:
xxxxxxxxxx
import { render, fireEvent } from '@testing-library/react';
import { screen } from '@testing-library/dom';
import SomeComponent from './SomeComponent';
After rendering the component, use fireEvent to simulate writing text to an input:
xxxxxxxxxx
test('inputs the correct value', () => {
render();
// Use the `input` role and the placeholder text to find the input element
const input = screen.getByRole('input', { name: /username/i });
// Emulates typing into the input field
fireEvent.change(input, { target: {value: 'testuser'} });
// Validates that the input value has changed
expect(input.value).toBe('testuser');
});
Note that the getByRole function retrieves elements by their accessibility role. This is consistent with testing practices recommended by these libraries to find elements in a way meaningful to users.
As Bill Gates once said, “The computer was born to solve problems that did not exist before.” Emphasizing on this quote, DOM-Testing-Library and React-Testing-Library can deal with the problem of rigorously testing an application’s UI in a manner that closely resembles real user interactions, drastically improving the quality of web applications.
[3] is a great online resource to dive deeper into accessible queries and unique roles provided by these libraries to make our tests more effective.
Methods for Testing Input Value in Dom-Testing-Library
The DOM Testing Library or the React-Testing-Library both offer comprehensive and efficient methods to test input values in your React applications. To ensure orderly testing operations, it’s paramount to grasp the art of using these libraries effectively.
Let’s explore some of those recommended ways you can test input value with these amazing tools:
User Events Triggering
User events like texting and clicking can be simulated using
xxxxxxxxxx
fireEvent
. It is widely recognized due to its consistency of testing user behaviour just as it occurs naturally in the DOM.
Consider this code snippet:
jsx
import { render, fireEvent } from ‘@testing-library/react’
it(‘allows the user to add text in input’, () => {
const { getByRole } = render()
const input = getByRole(‘textbox’)
fireEvent.change(input, { target: { value: ‘TypeScript’ } })
expect(input.value).toBe(‘TypeScript’)
})
We employ the method
xxxxxxxxxx
getByRole('textbox')
for selecting the input and then trigger a change event using
xxxxxxxxxx
fireEvent
.
Using The UserEvent API
The
xxxxxxxxxx
userEvent
API is another elegant way to handle DOM operations. It mimics real interactions more accurately than
xxxxxxxxxx
fireEvent
.
Following shows an example:
jsx
import { render, screen } from ‘@testing-library/react’
import userEvent from ‘@testing-library/user-event’
it(‘allows the user to add text in input’, () => {
render()
const input = screen.getByRole(‘textbox’)
userEvent.type(input, ‘TypeScript’)
expect(input).toHaveValue(‘TypeScript’)
})
Here, we import
xxxxxxxxxx
userEvent
and use the
xxxxxxxxxx
.type()
function to simulate text input.
The waitFor Function for Handling Async Updates>
Handling async updates could pose a challenge. This is where the
xxxxxxxxxx
waitFor
function comes in.
Here’s how:
jsx
import { render, screen, waitFor } from ‘@testing-library/react’
import userEvent from ‘@testing-library/user-event’
it(‘allows the user to add text in input asynchronously’, async () => {
render()
const input = screen.getByRole(‘textbox’)
userEvent.type(input, ‘TypeScript’)
await waitFor(() => {
expect(input).toHaveValue(‘TypeScript’)
})
})
In this case,
xxxxxxxxxx
waitFor
is used to halt test operation until our assertion becomes true.
To quote one of our industry’s leaders, Martin Fowler, “Whenever you are tempted to type something into a print statement or a debugger expression, write it as a test instead.”
Testing indeed gives any application the integrity it needs to thrive amidst various scenarios of user interaction. Therefore, effective usage of these methods can lead to robust, error-free applications.
Approaches to Validating Input Values in React-Testing Library
Approaches to validating input values in the React-Testing Library and the DOM-Testing Library typically involve a series of steps that enhance the quality and reliability of your code testing process. While there are implicit ways to probe for its correctness, such as checking state or inspecting props, the best approach, consistent with Kent C. Dodds’ philosophy for developing the library, is testing user behavior.
Testing User Behaviour with fireEvent
xxxxxxxxxx
const { getByLabelText, fireEvent } = render(
);
fireEvent.change(getByLabelText('test input'), {
target: {value: 'test value'},
});
expect(getByLabelText('test input').value).toBe('test value');
This method mimics the experiences your users go through when interacting with your application. By importing and setting an initial input field’s value with the
xxxxxxxxxx
fireEvent.change()
method from React-Testing Library, you’re able to simulate the action of a user typing text into the input field. Verifying the input value then becomes a matter of assessing if the input field contains the newly expected value by utilizing an assertion like
xxxxxxxxxx
expect().toBe()
.
UserEvent Library for Improved Simulation
You may improve simulation of real life user interaction with the UserEvent library, which is part of the Testing Library family. An alternate approach to the previous could look like this:
xxxxxxxxxx
import userEvent from '@testing-library/user-event';
const { getByLabelText } = render(
);
userEvent.type(getByLabelText('test input'), 'test value');
expect(getByLabelText('test input').value).toBe('test value');
Here, the
xxxxxxxxxx
userEvent.type()
function is used in place of
xxxxxxxxxx
fireEvent.change()
. The UserEvent library provides a more realistic simulation and comprehensive set of user interactions compared to fireEvent.
In the words of Martin Fowler: “Whenever you are tempted to type something into a print statement or a debugger expression, write it as a test instead.”. This embodies the spirit of React Testing Library’s philosophy. By focusing on testing from the user’s perspective with realistic input simulations, you enable yourself to create an efficient, robust, and user-centered codebase.
Tips for Optimizing Your Tests with Dom or React Testing Libraries
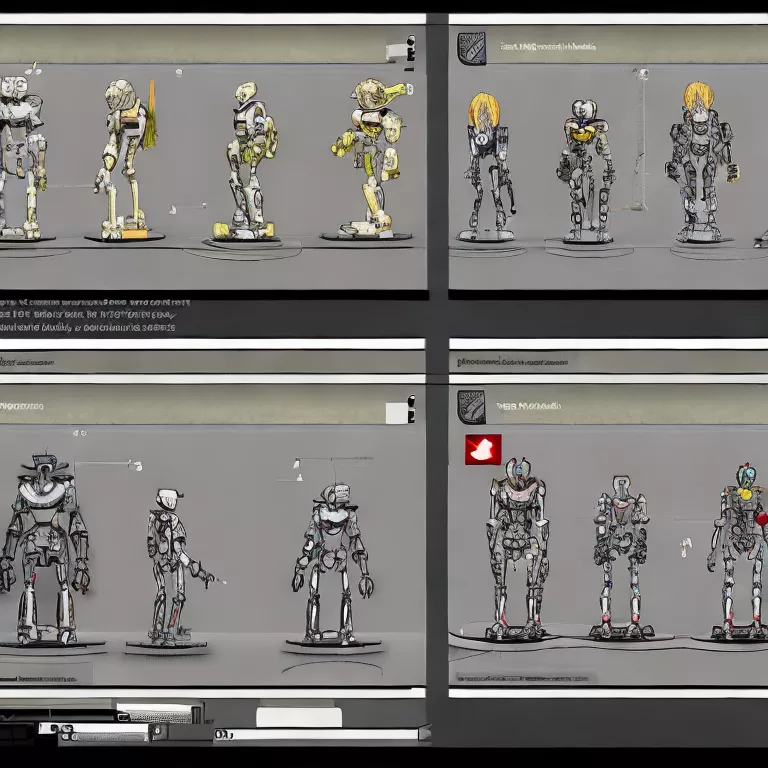
Optimizing Your Tests with DOM or React Testing Libraries: Focusing on Input Values
The React community’s testing tooling has evolved over the years, and currently, it manifests in a suite comprising of Jest, the DOM-Testing-Library, and its extension, the React-Testing-Library. One crucial aspect involved in these libraries tests is asserting the values of inputs. This article audits key strategies for optimizing this kind of testing.
1. Targeting Input Elements Explicitly:
Frequently, testers resort to querying by input type when retrieving an element:
xxxxxxxxxx
getByLabelText('Name:')
However, a more effective strategy would involve employing queries that stress accessibility, such as labels and roles, yielding more robust and maintainable tests.
2. Harnessing fireEvent Method:
React-Testing-Library offers the
xxxxxxxxxx
fireEvent
function to simulate user’s interactions with the UI. The method attributing value to an input plays out thus:
xxxxxxxxxx
fireEvent.change(screen.getByLabelText('Name:'), {
target: {value: 'John Doe'},
})
This code snippet unveils that the fireEvent.change method mimics the behavior of a real user accurately, applying changes directly on the target input element.
Consider employing the
xxxxxxxxxx
userEvent
library for even more realistic events. As [Kent C. Dodds](https://kentcdodds.com/blog/common-mistakes-with-react-testing-library) stated, “If you can use it, then you should.” Offering synchronous events, it leads to less flaky and more feasible-to-trace outcomes.
3. Inclusive Assertion:
The crux of asserting an input value doesn’t revolve around the simplicity of the assertion itself, but on its implication for user interaction. This perspective challenges the tester to consider, assess and consolidate these implications into a comprehensive assertion strategy.
For example:
xxxxxxxxxx
expect(screen.getByLabelText('Name:')).toHaveValue('John Doe')
Furthermore, reaching for
xxxxxxxxxx
jest-dom
assertions enhances readability and error messages. Tapping into it transforms the snippet above to:
xxxxxxxxxx
expect(screen.getByLabelText('Name:')).toBeInTheDocument()
expect(screen.getByLabelText('Name:')).toHaveValue('John Doe')
4. Utilizing Data Test IDs Sparingly:
While data-test-id attributes can aid in locating elements, they divert from simulating how users interact with an application. They should only serve as last resorts while more determinative alternatives like labels, text, and roles remain unattainable.
Remember, as Martin Fowler emphasized, “[The tests we write](https://martinfowler.com/bliki/TestPyramid.html) are not just for finding bugs or avoiding regression. They also provide an executable specification of the system’s behavior…”
Conclusively, the optimization techniques analyzed so far directs towards one pivotal goal: engineering tests that appear less to machine judgments – remaining comprehensible, maintainable, consistent and invariably valuing the end-user’s experience at their apex.
Conclusion
To validate input values in Dom-Testing-Library or React-Testing-Library, the primary approach involves employing robust testing methods and assertions. DOM Testing Library is a highly scalable option for an array of applications, offering high-level abstractions that facilitate superior accessibility.
The getbyRole function in this library allows pinpointing input elements by their role rather than test IDs, paving the way for more functional and accessible code. Moreover, the advantageous ‘toHaveValue’ assertion aids in validating input values effectively.
Take this example into account:
xxxxxxxxxx
{`
const { getByRole } = render(
);
const inputElement = getByRole('textbox');
expect(inputElement).toHaveValue('Initial Value');
`}
This code snippet showcases how straightforward it is to evaluate an input value in the DOM Testing Library.
In contrast, React Testing Library provides an abstraction over React’s underlying mechanics. It focuses on replicating how end-users would interact with your application, rather than programming implementation details.(source)
For instance:
xxxxxxxxxx
{`
import { render, fireEvent } from '@testing-library/react';
...
const { getByLabelText } = render();
const input = getByLabelText('input');
fireEvent.change(input, { target: { value: 'New Input Value' } });
expect(input.value).toBe('New Input Value');
`}
This code demonstrates how the fireEvent change event changes the input value and validates it using React Testing Library.
Dave Ceddia succinctly summarizes this approach, “Write tests. Not too many. Mostly integration.”(source) This notion reinforces the importance of comprehensive testing; however, attention must be devoted to ensure that these tests capture the essential user interactions that your scripts and applications facilitate.
Performing input value testing in both libraries greatly bolsters code robustness, delivering enhanced reliability for your projects. The best method always rests on your unique project requirements- irrespective of whether that involves looking for straightforward syntax (as provided by DOM Testing Library) or favoring more human-centric testing (a key feature of the React Testing Library).