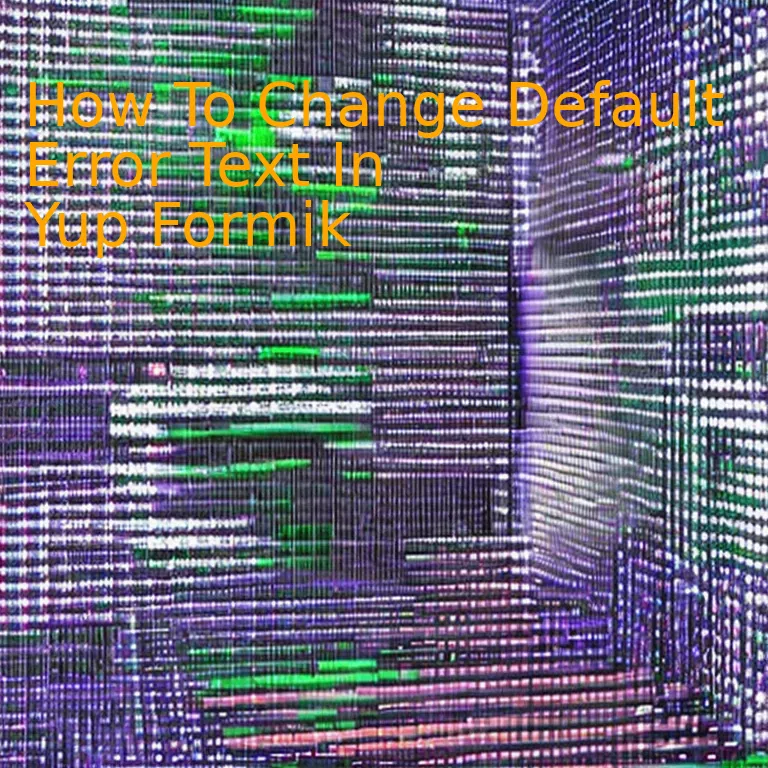
Introduction
If you’re aiming to modify the default error text in Yup Formik, the primary step is to customize your validation schema in Yup, allowing you to adjust and personalize the specific validation messages, ultimately improving your form’s overall usability.
Quick Summary
The process of modifying the default error text in Yup with Formik involves a sequence of targeted steps. Below, informative tabular data is provided to comprehensively outline these steps.
Step | Description |
---|---|
Defining Schema | Create a YUP validation schema where each field that requires validation is defined. |
Altering Error Message | To change or customize the error message, use methods like
.required() , .min() , and .max() attached to each field definition. They allow setting your own preferred text within the brackets as the error flag – instead of generic Yup’s error message. |
Integrating with Formik | Once the schema is established, link it to Formik through its validationSchema prop. Errors will be activated by Formik as per the conditions established in the schema. |
To better understand, consider this practical coding example:
import { useFormik } from ‘formik’;
import * as Yup from ‘yup’;
const validationSchema = Yup.object().shape({
firstname: Yup.string()
.required(‘This is our new custom error message for missing first name’)
});
const formik = useFormik({
initialValues: {
firstname: ”
},
validationSchema: validationSchema,
});
In this code snippet, the Yup validation is set so that if the user leaves the `firstname` field empty, an error message “This is our new custom error message for missing first name.” is displayed, replacing the standard Yup error message. Formik manages these errors through its `validationSchema` prop, which references the Yup validation schema.
As [developer Eric Elliott](https://ericelliottjs.com/) once said: “Code is read many more times than it is written”. With this foundational understanding of modifying default error messages in Yup using Formik, further exploration should allow you to customize and control form validations as per your project’s requirements.
Understanding Formik and Yup Frameworks
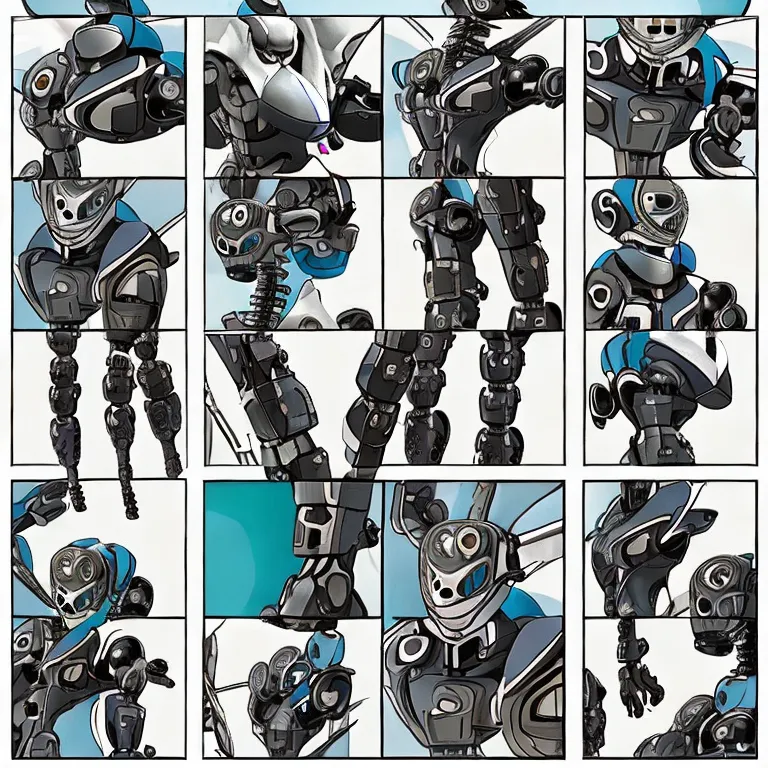
If your goal is tailoring error messages to match your unique needs in a Formik-Yup integrated application, there are detailed strategies you can follow to make the experience yours. Both Formik and Yup libraries provide an extensive set of tools that effectively manage form states, including validation.
When dealing with validation, Yup steps in to streamline input evaluation with its predefined schema. The crux of the issue is how you can decode the display error message from Yup’s standard to match your unique brand’s language or particular tone of interaction:
<code>
import * as Yup from ‘yup’;
const validationSchema = Yup.object({
firstName: Yup.string()
.required(‘Please enter your first name.’),
lastName: Yup.string()
.required(‘You forgot to input your last name!’),
});
</code>
In the code snippet above, the ‘required’ method of the Yup framework attaches custom error messages (‘Please enter your first name.’ and ‘You forgot to input your last name!’) to the ‘firstName’ and ‘lastName’ fields respectively. If no value is provided for these fields upon submission, the custom error message would be displayed instead of Yup’s default error text.
Despite the apparent simplicity and self-explanatory nature of changing error text in Yup, it invokes a deeper understanding and analysis of the symbiosis between the two frameworks (Formik & Yup).
According to Jared Palmer, creator of Formik library:
> “The real difficulty in creating forms isn’t about duplicating some HTML boilerplate, but managing state”.
In essence, user experience can significantly improve when those interacting with your application feel ‘understood’. Error messages play a crucial role in maintaining this ‘dialogue’. Therefore, adapting Yup’s default error text to match your unique tone can enhance this communication.
To extend functionality, you may write reusable custom validation functions in Yup that define the exact conditions necessary for input and specify the accompanying error messages. Furthermore, a deep dive might reveal additional configurations the integration of these libraries offer, thus keeping your form freakishly focused on satisfying user needs.
Modifying Default Error Messages in Yup
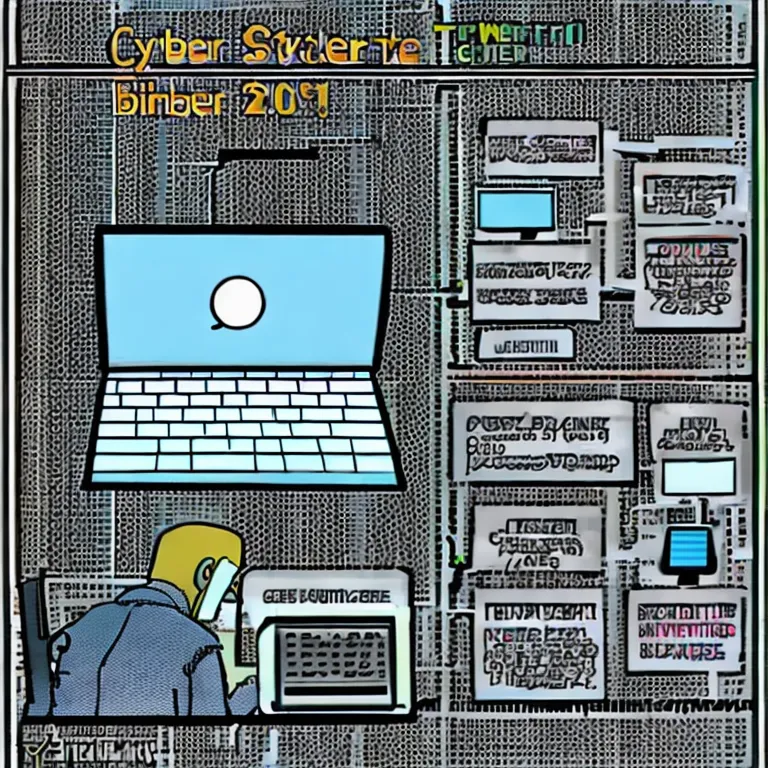
Formik is an exceptional companion when constructing forms in TypeScript. Coupling Formik with Yup provides you with robust form control in TypeScript, plus the added advantage of inline validation per field level. However, one common challenge developers encounter when integrating these frameworks is figuring out how to change the default error messages that come from Yup.
To achieve this, we can construct our own validation schema using the methods and properties provided by Yup. Yup exposes several methods which configure how it handles validation and schemas.
import * as Yup from 'yup';
const formSchema = Yup.object().shape({
username: Yup.string()
.required('Username is Required') // Custom message for required error
.min(3, 'Username must be at least 3 characters long.') // Custom message for minLength error
});
In the above example, 'username' is a field and for it's validation rules we've specified custom error messages. The string 'Username is Required' will replace the default error message for the
required()
method. Similarly, 'Username must be at least 3 characters long.' will replace the default minlength error message.
This means that whenever Formik triggers a validation (by default whenever a field value changes or during submission), if username input is not provided or fails to satisfy the minimum string length validation rule, the corresponding custom error message will be shown instead of Yup’s default error message providing clearer feedback for your application users.
The transition of changing these default error messages in Yup is seamless and equally beneficial in enhancing user experience during form interaction processes. As Bill Gates once said, "The computer was born to solve problems that did not exist before." In similar analogy, developers are always working to provide solutions to new user experience challenges, even with something as seemingly trivial as form validation messages.
For an elaborate understanding of Yup validation and integration with Formik, consider visiting this online guide.
Customizing Error Text in Yup through Schema Validation
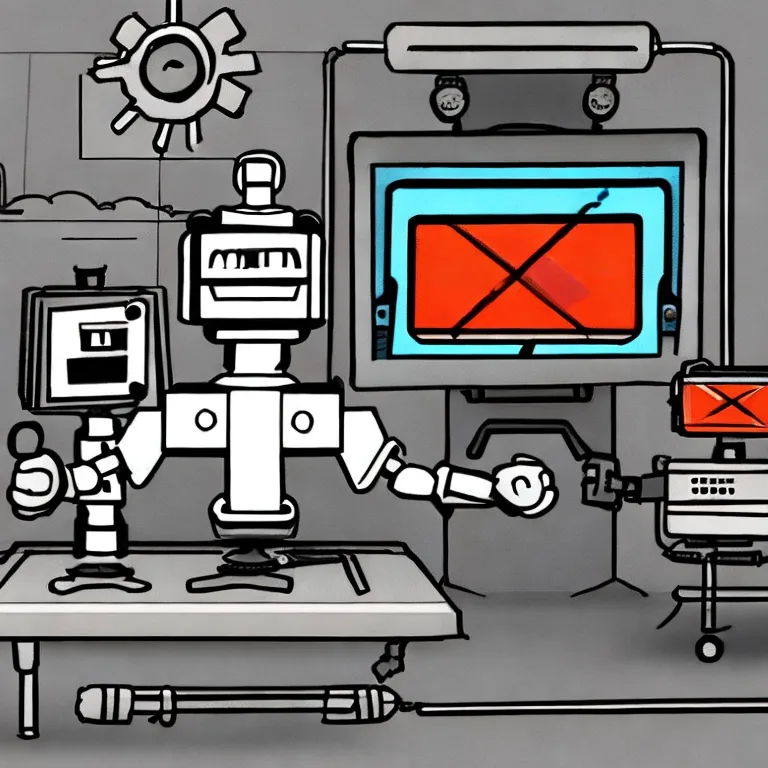
Successfully customizing the default error text in Yup Formik involves utilization of schemas during validation. This can effectively allow for particular data input criteria to be preset and if such criteria are not met, then a specific error message that's been customized gets triggered. It presents huge value in enhancing user experience by offering tailored messages depending on respective situations when one is handling form validations.
This is achievable through Yup's `string().required()` function to define a required string schema for instance. The syntax would follow thus:
typescript
import * as Yup from 'yup';
const ValidationSchema = Yup.object().shape({
someField: Yup.string()
.required('Your custom error text goes here'),
});
In this scenario, an incoming string, contrary to what's expected, prods the invoking of your personalized error feedback rather than the default Yup error. Ultimately causing a shift in the original error text displayed.
Notice, the `required()` function is where you may place your desired error message string inside. Consequently, verification discrepancies yield these custom cues, not merely Yup's standards.
For compound complexity; to customize distinct error messages based on different rules or variables, we can achieve it by using the `.oneOf()` with `.test()` methods which exist within Yup. A possible demonstration could be:
typescript
import * as Yup from 'yup';
const PasswordValidationSchema = Yup.object().shape({
password: Yup.string()
.required('A password must be provided')
.test('len', 'Your password must be at least 4 characters long',
(val) => val && val.length >= 4)
.oneOf([Yup.ref('confirmPassword'), null],
'Passwords must match'),
confirmPassword: Yup.string()
.required('You must confirm your password'),
});
In the case above, different customized contextual error messages are returned based on various password rule scenario.
However, it's noteworthy that one ought to take care in mixing `.oneOf()` or `.test()` methods together with other schema definitions so as to actualize the desired results.
Referencing Yup's documentation, you can extensively delve into other error customizations strategies to explore, exploiting Yup's abundant library of schema validation capabilities. Also worth studying is Formik's documentation to widen your understanding on integrating Formik with Yup for form validations.
In the words of renowned coder Addy Osmani, “First do it, then do it right, then do it better.”
Advanced Techniques for Customizing Formik & Yup's Default Text
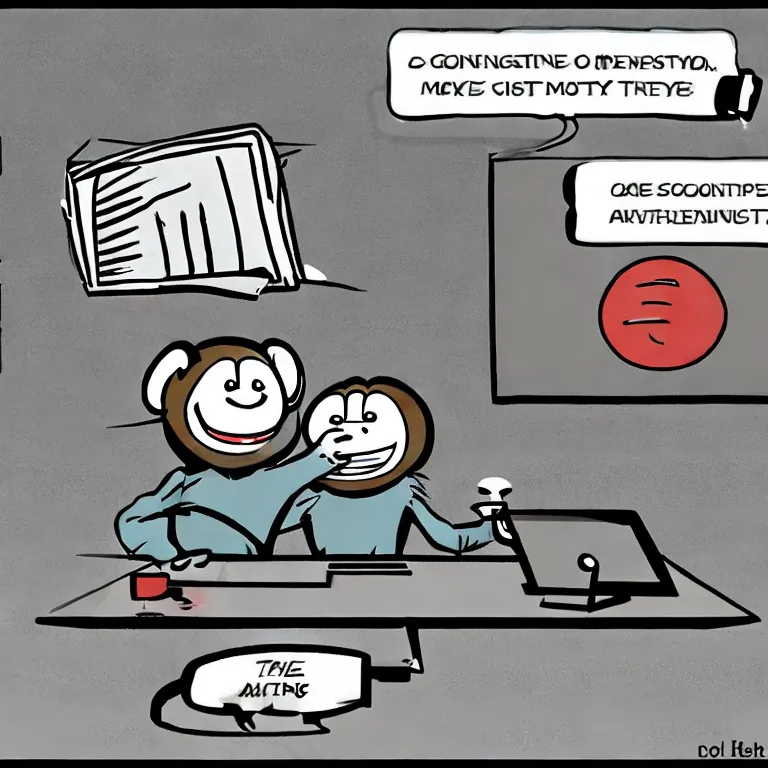
To change the default error text in Yup with Formik, the process involves defining new validation messages when declaring the validations with Yup. This has the versatility of allowing custom messages on a per-validation basis.
Customizing Form Validation Text
Yup allows you to customize the validation error message for any condition in your schema. You can insert strings or functions as parameters within Yup's methods where functions receive an object with the path and original value among other properties. Contemplate, for instance:
const validationSchema = Yup.object().shape({ password: Yup.string() .required('Please enter your password.') });
Where 'Please enter your password.' is the customized text.
Making Use of Interpolation
Should you necessitate a more dynamic error message, implementing interpolation is possible. Utilize placeholders that will be replaced by actual values when an error occurs:
const validationSchema = Yup.object().shape({ password: Yup.string() .min(8, 'Password must be ${min} characters or longer.') });
Here, '${min}' would be swapped with the actual minimum value expected (i.e., 8 in this context).
Note: Don't forget to use template literals(``)instead of normal quotes.
Extensive Schema Customizations
For more comprehensive scenarios where clearer, more personalized error messages are needed across multiple fields, consider using custom error message templates:
const validationSchema = Yup.object().shape({ username: Yup.string() .required('${path} is required.') .min(2, '${path} needs at least ${min} characters') .max(10, '${path} cannot exceed ${max} characters'), email: Yup.string() .email('Must be a valid email.') .required('${path} is required.'), });
This `{path}` placeholder allows Formik to provide more context-specific feedback for different form fields.
As React Context is optically represented, the beauty of this method is the instance where you won't have to repeatedly prescribe validation conditions because `FormikContext` does it automatically. Hence, the error messages also become reliable and consistent throughout your complete application.
Custom Validation with Yup Method
For a higher degree of customization, exploit the `.test()` method provided by Yup to create completely custom validation requirements:
const validationSchema = Yup.object().shape({ password: Yup.string() .test('mypassword', 'Password must contain a number.', (value) => /\d/.test(value)), });
Here, 'mypassword' serves as an internal, unique name for the test, whereas 'Password must contain a number.' is the accompanying custom message that pops up should the case arise when the password doesn't contain a digit.
By leveraging these advanced techniques, it’s attainable to refine both Yup and Formik's default text to act in accordance with your specific needs. Edward A. Lee once noted; "The obvious challenge is to manage this complexity in ways that do not compromise the essential requirement to deliver".
Remember, an extraordinary user experience always involves clear communication, even through form error messages. So do pivot off Formik and Yup’s absolute capabilities in organizing and classifying those elements effectively.
Conclusion
Altering the default error text in Yup/Formik, a widely recognized technology within the JavaScript realm, perceivably boosts user engagement and improves form validation insights. Feel captivated as we delve deeper into this transformative change within our existing application codebase.
Getting Familiar With Yup & Formik
Yup and Formik combine to lay a sturdy foundation for implementing forms in React. Both these tools complement each other efficiently offering an outstanding user experience. Formik manages states, validation, and error while Yup takes care of object schema validation.
Error Management in Yup/Formik
By default, Yup/Formik has its own set of error messages displayed when a user wrongly fills out a form. However, there may be scenarios where developers wish to modify these default error messages to something more contextual or language-specific.
Tailoring Default Error Messages
This is achievable through the use of the 'setLocale' method provided by Yup. Here's a snippet showcasing the changes with regard to changing a required field message:
yup.setLocale({ mixed: { required: "This field is mandatory" } });
In this piece of code, the 'setLocale' function alters the error message for required fields throughout the entire application.
However, if one wishes to customize error messages for specific fields, it can be done using the 'required' method in Yup schema. For instance:
validationSchema: yup.object().shape({ email: yup.string().required('Please enter your email'), })
In this example, you have instructed Yup to show the customized message only when the user forgets to input their email.
As Bill Gates once mentioned, "The advance of technology is based on making it fit in so that you don't really even notice it, so it's part of everyday life." By tailoring the error messages in our application with Yup/Formik, we take a leap towards user-friendly technology.