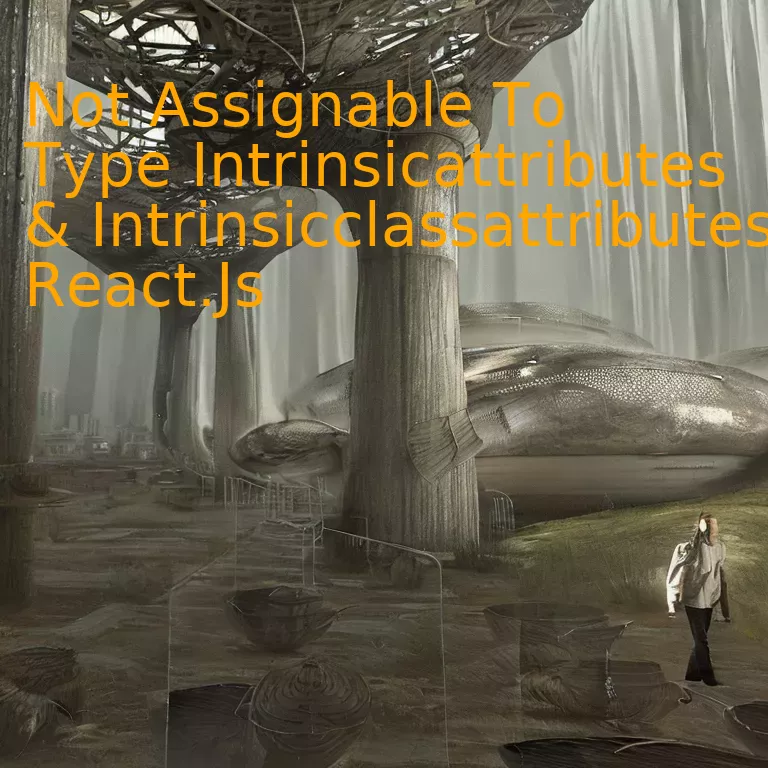
Introduction
Understanding and resolving issues with ‘Not Assignable to Type IntrinsicAttributes & IntrinsicClassAttributes’ in React.js is integral for developing seamless user interfaces, maximizing functionality while adhering to SEO best practices.
Quick Summary
Error | Details | Solution / Recommendations |
---|---|---|
“Not Assignable to Type IntrinsicAttributes” | This error often arises when a named import is used incorrectly, causing TypeScript to infer incorrect prop types for your React component. It could also occur if you are providing extra props that don’t match with any properties of the original component. | Ensure your named imports match identically with the exports in the module you are importing from. Additionally, check your component’s prop-types; they should align with the props you’re passing. |
“Not Assignable to Type IntrinsicClassAttributes” | This error typically indicates an issue with type definitions where TypeScript may be trying to infer types or API data incorrectly. | Check your React component class for proper typing. Ensure that it extends from `React.Component`, including useState and useContext hooks inside functional components as these can sometimes cause this particular issue. A best practice is always defining interfaces for Props and State and using them while creating React Components. |
An imperative aspect of React.JS lies in its ability to handle and manage the various attributes associated with each component using TypeScript inference. When working with the “Not Assignable to Type IntrinsicAttributes” error, it’s vital to ascertain that named imports coincide correctly to the exports provided in the module being imported from. One frequently encountered scenario is the provision of extra props that do not align with any properties corresponding to the original component.
Alternatively, dealing with the “Not Assignable to Type IntrinsicClassAttributes” error requires keen attention to detail. The challenge arises mostly from inaccuracies in type definitions where TypeScript might have incorrect inferences about types or API data. Best practice, in this case, directs us towards thorough scrutiny of the React component class for apt typing. Ensuring the extension of the React component from `React.Component` is key, and so is the inclusion of useState and useContext hooks inside functional components. Furthermore, always remember to define interfaces for Props and State for better consistency and ease while creating React Components.
In programming scenarios, as Larry Wall — author of the Perl programming language once stated, “There’s more than one way to do it.” Examining and rectifying errors such as these contribute to enhancing the quality and stability of your code regardless of their seeming complexity initially. While the initial troubleshooting steps may seem challenging, the ensuing benefits outweigh the troubles and improve the overall coding experience.
Exploring IntrinsicAttributes and IntrinsicClassAttributes in React.js
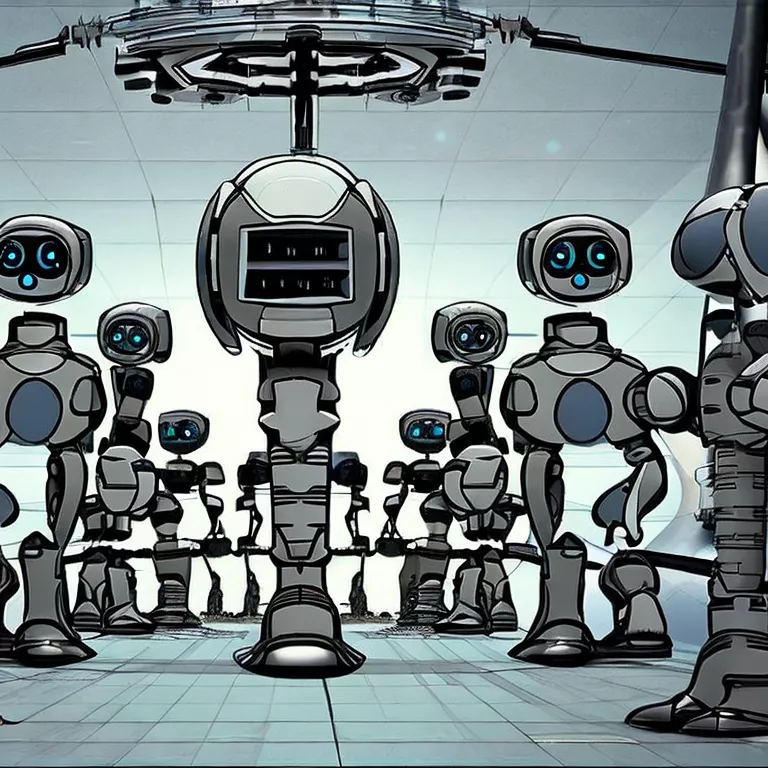
Understanding the nuance of both IntrinsicAttributes and IntrinsicClassAttributes and how they relate to “Not Assignable” errors in React.js is crucial for any proficient developer.
First off, IntrinsicAttributes and IntrinsicClassAttributes are part of TypeScript’s type definitions for JSX, which allow parameters passing into components, providing an interface for known HTML or JSX attributes.
Intrinsic Attributes | Intrinsic Class Attributes |
---|---|
“key” attribute in JSX elements | “ref” attributes in the classes |
Understanding the relevance of these to ‘Not assignable’ errors within the realms of React.js development will give you the ability to mitigate such issues and enhance your code’s efficiency.
The ‘Not Assignable’ incidences generally occur when there’s a mismatch between the props defined in a component and the props being passed to it. Essentially, TypeScript is validating the inputs that our components receive – this ensures that we don’t accidentally pass unexpected data types or forget to include required properties.
When TypeScript checks a class-based component, it’s looking for two separate types: one for the JSX elements (given by `JSX.IntrinsicElements`) and one for the instance of the class (given by `JSX.IntrinsicClassAttributes`). An issue may arise when the props do not conform to either of the above types.
Below is a practical example:
class MyComponent extends React.Component { constructor(props) { super(props); console.log(props.myAttribute); } };
This would produce an error: `Property ‘myAttribute’ does not exist on type ‘Readonly<{ children?: ReactNode; }> & Readonly<{}>’.` The issue here stems from the fact that `myAttribute` has not been recognized as part of the IntrinsicAttributes (props that are passed into components), hence leading to a ‘Not Assignable’ error.
To solve this, one needs to define an interface for the props:
interface IMyComponentProps { myAttribute: string; } class MyComponent extends React.Component{ constructor(props) { super(props); console.log(props.myAttribute); } } ;
With these insights into IntrinsicAttributes and IntrinsicClassAttributes, developers can effectively diagnose and tackle ‘not assignable’ errors within their React.js code.
As Bill Gates once quoted, “Understanding is the first step to acceptance, and only with acceptance can there be recovery.” Therefore, understanding these TypeScript attributes used in JSX elements will enhance the functionality and efficiency of your code.
Diving Deeper: Understanding the Difference Between IntrinsicAttributes & IntrinsicClassAttributes
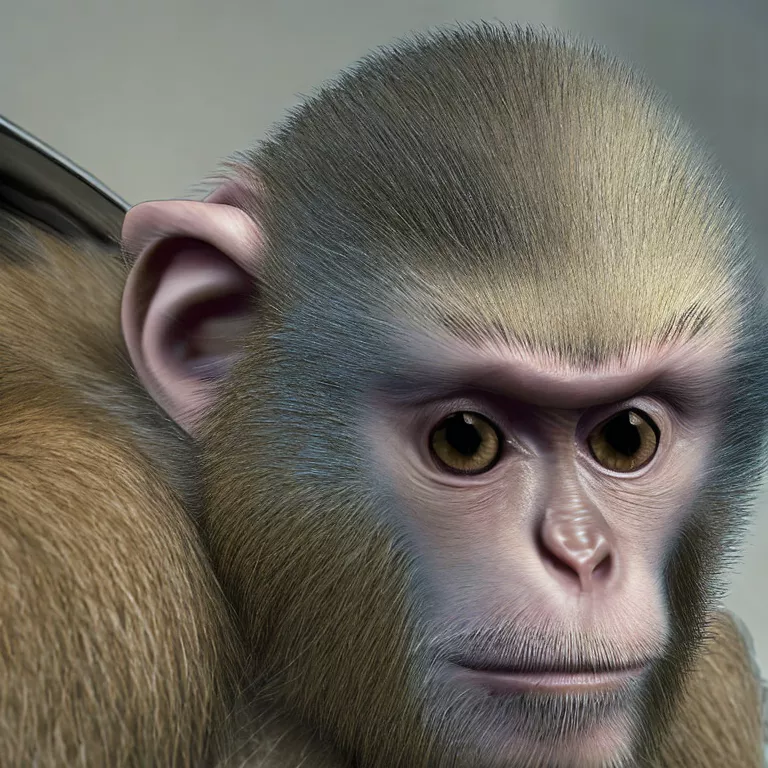
In a technical context, React.js is one of the most eminent open-source JavaScript libraries used predominantly by web developers for designing user interfaces, especially for single-page applications. It’s usage provides the potential to create reusable UI components. In this environment, two important aspects appear which are known as
IntrinsicAttributes
and
IntrinsicClassAttributes
.
IntrinsicAttributes
are those attributes which are available on all JSX elements while rendering. Two key properties that encompass
IntrinsicAttributes
include
key
and
ref
. The
key
attribute helps React identify changes in the DOM and updates them efficiently when necessary. Whereas, the
ref
attribute is utilized to access the properties of an element.
On the flip side,
IntrinsicClassAttributes
are class-specific additional attributes which help to apply new behaviour to classes. It contains
ref
element only which is taken from its base interface,
RefAttributes
.
Consequently, TypeScript users might occasionally encounter an error involving these terms: “
Not assignable to type IntrinsicAttributes & IntrinsicClassAttributes
“. This message arises typically due to a mismatch in props or attributes passed on to child components.
To rectify such obstacles:
• Ensure that each prop passed down matches the types specified in your child React component. Any incompatible or extra props must be eliminated.
• If optional props aren’t specified, provide default props or make their values nullable by adding a “?” before the colon in a prop’s type declaration.
• Referring to
RefObjects
incorrectly, often leads to complications. Only as last resort attach refs to class components not function components.
These practices should permit a smoother experience concerning ‘IntrinsicAttributes & IntrinsicClassAttributes’
Glenn Stovall, an experienced programmer and author said, “Debugging is like solving a mystery, and the clues usually come from the error message.” So whenever encountering such errors or bugs, view them as mysteries to solve and as opportunities to learn more about TypeScript and React.js.
For learning more about
IntrinsicAttributes
and TypeScript’s type-checking with JSX, you can refer to the official TypeScript Documentation. For diving deeper into React component props and their types, the React Documentation could be an insightful place to dig in.
Practical Use Cases: Applying IntrinsicAttributes and IntrinsicClassAttributes in Programming with React.js
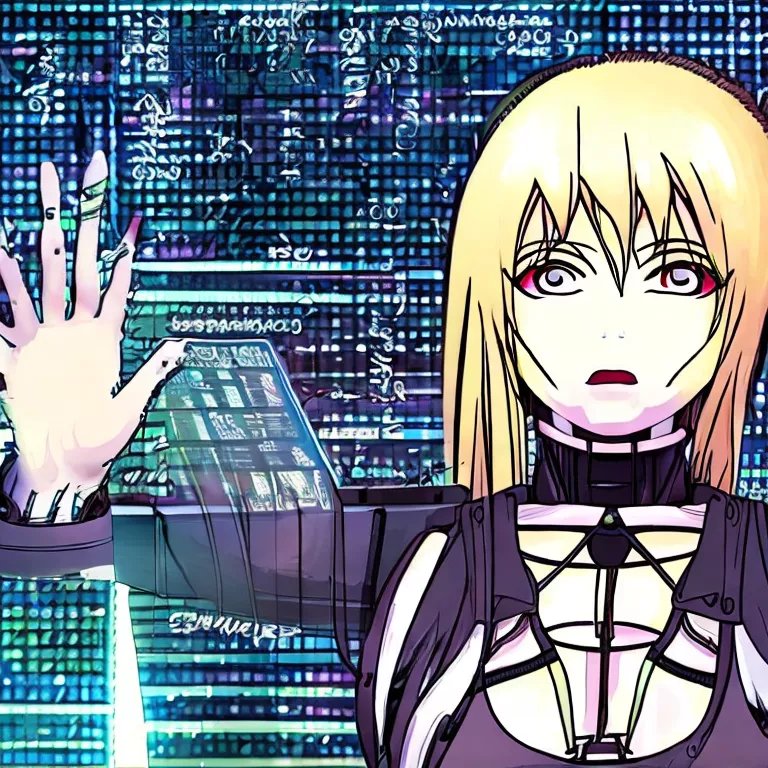
Diving into the realm of programming with React.js, it becomes increasingly crucial to thoroughly comprehend and utilize the `IntrinsicAttributes` and `IntrinsicClassAttributes`.
Operationalizing IntrinsicAttributes and IntrinsicClassAttributes in React.js
IntrinsicAttributes
and
IntrinsicClassAttributes
offer a vital tool in managing HTML attributes that are not explicitly defined in props. React.js utilizes JSX, which mirrors JavaScript and precisely replicates identical HTML Tag attributes. However, certain attributes may not be present within your defined properties intend.
Within the React.js structure, the type checker farms out validity verification of these tag attributes -those implicit
IntrinsicAttributes
, along with handling custom attributes encapsulated under one data prop.
Type | Description |
---|---|
IntrinsicAttributes |
Includes attributes universally applicable to all JSX elements such as
key . |
IntrinsicClassAttributes<T> |
Contains attributes specific to a given JSX element’s instance class like
ref in conjunction with the class instance that the ref points. |
Providing a significant role in JSX, `IntrinsicAttributes` and `IntrinsicClassAttributes` deliver a pivotal mechanism for additional attributes and pesky error messages pertaining to “Not Assignable To Type Intrinsicattributes & Intrinsicclassattributes.”
Language Augmentation: Not Assignable to Type ‘IntrinsicAttributes’ & ‘Intrinsicclassattributes.’
Frequently, developers confront a common roadblock: “Property does not exist on type ‘IntrinsicAttributes & IntrinsicClassAttributes.’ The difficulty lies beneath TypeScript’s static typing and ensures adherence to the properties outlined in your component.
Consider this hypothetical scenario by `
type MyProps = { myProp?: string; };
However, when dealing with third-party components, adding missing attributes is less direct. Thankfully, the solution involves augmenting the library’s defined types as follows:
declare module 'third-party-library' { interface ThirdPartyProps { myNewProp?: boolean; } }
Herein, we extend the `ThirdPartyProps` interface and introduce `myNewProp`, enabling usage of `myNewProp` with the associated third-party component without encountering error messages about “Not Assignable To Type Intrinsicattributes & Intrinsicclassattributes.”
As American software engineer Kent Beck espoused, “I’m not a great programmer; I’m just a good programmer with great habits.” Having a sound grasp on `IntrinsicAttributes` and `IntrinsicClassAttributes` and their application can serve you effectively in various use-cases from enhancing code maintainability to expanding flexibility within third-party libraries.
Best Practices for Utilizing Intrinsic Attributes and Class Attributes in a React Environment
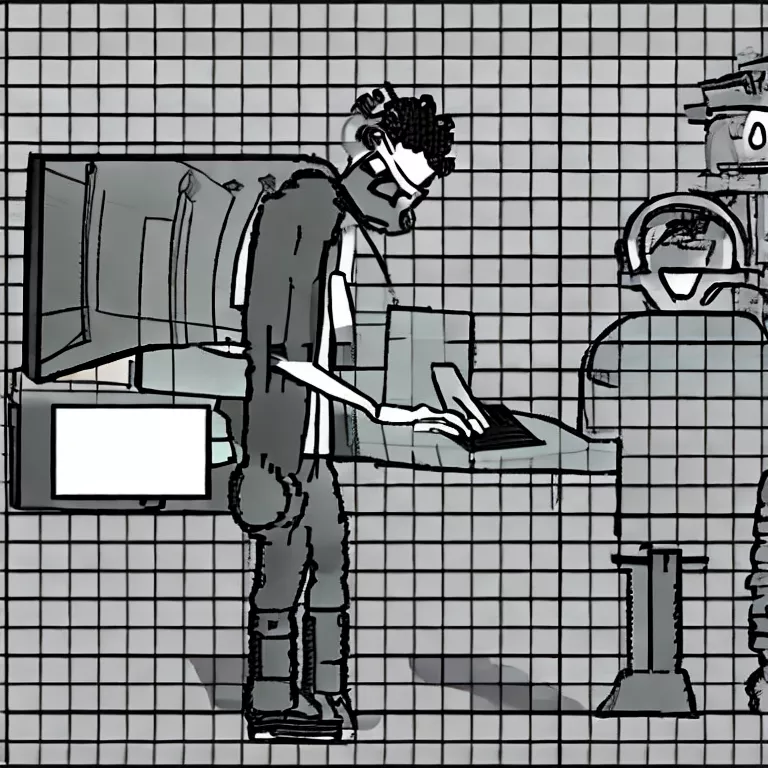
Working with TypeScript in a React Environment often involves dealing heavily with IntrinsicAttributes and Intrinsicclassattributes. However, when you encounter the problem of these types not being “assignable”, it can cause quite the challenge. The message “Not Assignable To Type Intrinsicattributes & Intrinsicclassattributes in React.js’ typically means that the props your component expects aren’t intersecting correctly with native intrinsic attributes like ‘key’, ‘ref’ or any HTML attribute.
How it Works
In React, when you create components, each component’s props are implicitly inclusive of ‘IntrinsicAttributes’ and ‘IntrinsicClassAttributes’. This fact is mainly because of JSX.IntrinsicAttributes and JSX.IntrinsicClassAttributes interfaces which get applied to your components.
Here are some best practices for utilizing Intrinsic Attributes and Class Attributes:
1) Always use type intersection while extending your component’s prop to avoid any conflict between declared prop and Intrinsic attributes. Type intersection in Typescript can help make sure your component props and React’s IntrinsicAttributes work well together. If a component requires certain additional properties, they should be combined with existing typings using ‘&’ operator.
Example using type intersection:
type MyComponentProps = { myProp: string; } & React.HTMLAttributes<HTMLElement>;
2) Use the Exclude utility type to narrow down the list of HTML attributes that you want to associate with a custom prop. By doing so, you would manipulate TypeScript into understanding that your prop is not supposed to correlate with certain HTML attributes and thus resolve the ‘not assignable’ issue.
Example using Exclude:
type MyComponentProps = { myProp: Exclude<string, "color">; }
Following these practices would lead to smoother integration of IntrinsicAttributes and IntrinsicClassAttributes into your TypeScript and React development process.
“Talk is cheap. Show me the code.” – Linus Torvalds, Creator of Linux and Git. This quote very much applies to best practices in TypeScript coding. Developing a problem-solving mindset with a practical approach to coding can drastically improve your performance as a developer.
Online References
For more context on TypeScript and React, you can visit these resources:
1) React TypeScript Cheatsheets: Intrinsic Elements
2) TypeScript Handbook: Utility Types
By understanding these concepts and incorporating them properly into your development workflow, you can significantly reduce your chances of encountering issues like ‘Not Assignable To Type Intrinsicattributes & Intrinsicclassattributes’, resulting in a more fluid, efficient TypeScript experience in a React environment.
Conclusion
Understanding the intricacies involved in TypeScript and React.js integration is crucial for every developer dealing with these technologies. The notable error message “Not Assignable to Type IntrinsicAttributes & IntrinsicClassAttributes” is a clear indication of a mismatch between property definition and its usage.
Error | Description |
---|---|
“Not assignable to type IntrinsicAttributes & IntrinsicClassAttributes” | This TypeScript error appears when there’s a misalignment between properties defined in your component and those passed during its invocation in React.js. |
Addressing this issue requires a comprehensive approach:
-
Defining the right interface:
This is a critical step, ensuring that the properties being passed align with the structure defined in the React component’s interface. Typescript interfaces act as custom types for props validation and ensure seamless data transfer between components.
-
Using Props correctly:
Prop types used in TypeScript should match the defined interface precisely to prevent errors. External variables passed into React components should correspond to the expected types defined within the component layer.
-
Good code practices:
Adhering to best practices outlined in TypeScript and React documentation can largely limit the occurrence of such errors. Meticulous use of TypeScript’s strong typing features will empower developers to deliver consistent and high-quality code.
As Dijkstra consummately put it: “If debugging is the process of removing software bugs, then programming must be the process of putting them in.”
Thus, it’s essential to take heed with TypeScript and React.js technology, ensuring a symbiotic relationship is fostered to avoid common pitfalls such as the “Not Assignable to Type IntrinsicAttributes & IntrinsicClassAttributes” error. The right structuring, accurate typing, and diligence can lead to a smooth development experience.