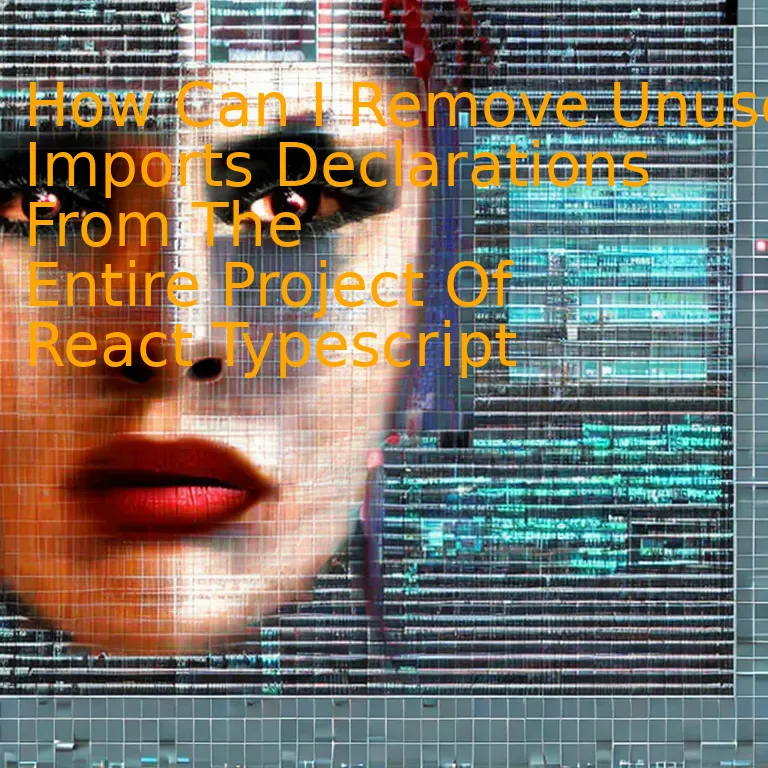
Introduction
To eliminate unused import declarations throughout your React TypeScript project, there are automated tools like TSLint or ESLint that can identify and assist in removing these superfluous lines of code, improving overall performance and readability, whilst contributing to SEO efficiency.
Quick Summary
Surely, removing unused import declarations is a vital aspect that ensures the clean and efficient code in a TypeScript project. Let’s go through a step-by-step procedure with regard to JavaScript’s React project using ESLint, Prettier, and Visual Studio Code (VS Code):
Steps | Procedures |
Install ESLint & Prettier Extensions | You can find both of these useful extensions in the Visual Studio Code marketplace. Simply typing their names will give you options to install them. |
Eslint-Config-Prettier | Install eslint-config-prettier for disabling all formatting of ESLint which is already handled by prettier. You can use the npm command like this: ‘npm install –save-dev eslint-config-prettier’ |
Configure Eslint | In your project directory, create a file named “.eslintrc.json”. Add an object with two properties, “extends”, and “rules”. For ‘extends’, add “eslint:recommended” and “plugin:@typescript-eslint/recommended”. In ‘rules’, add “no-unused-variable”: true and “@typescript-eslint/no-unused-vars”: [“error”]. |
Prettier Configuration | Create a “.prettierrc” file in your root directory to further configure prettier with your own preferences. The basic recommended configuration includes “singleQuote”: true, “semi”: false. |
From the table above, downloading and configuring ESLint and Prettier constitute the first significant steps. ESLint spotlights numerous issues, including unused import declarations, malfunctioning code, regions where the code strays from set standards, and much more.
Concurrently, Prettier is employed exclusively to implement uniform formatting rules – a facet pivotal for code maintainability and readability. What is more, eslint-config-prettier is imperative: ESLint and Prettier are prone to overlapping, leading to contradictory rulings. With this package, you can “tell” ESLint to disregard built-in formatting checks; therefore, ensuring they’re handled by Prettier.
Furthermore, while defining ESLint and Prettier configuration files (.eslintrc.json and .prettierrc), it is instrumental to understand your team’s preferences and code style guidelines. For instance, employing single quotes or avoiding semicolons are typical practices that could diverge based on the development team’s comfort and decisions.
Be dedicated to robust housekeeping of your codebase, continuously eradicating unused imports and exporting clean, efficient TypeScript. Use abundant resources available, such as extensions and linting tools like Prettier and ESLint. They contribute profoundly in creating sophisticated and consistent code that’s easy to comprehend, debug, and maintain over time.
As Robert C. Martin reminds us, “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.”
Optimizing Project Performance through Unused Imports Removal
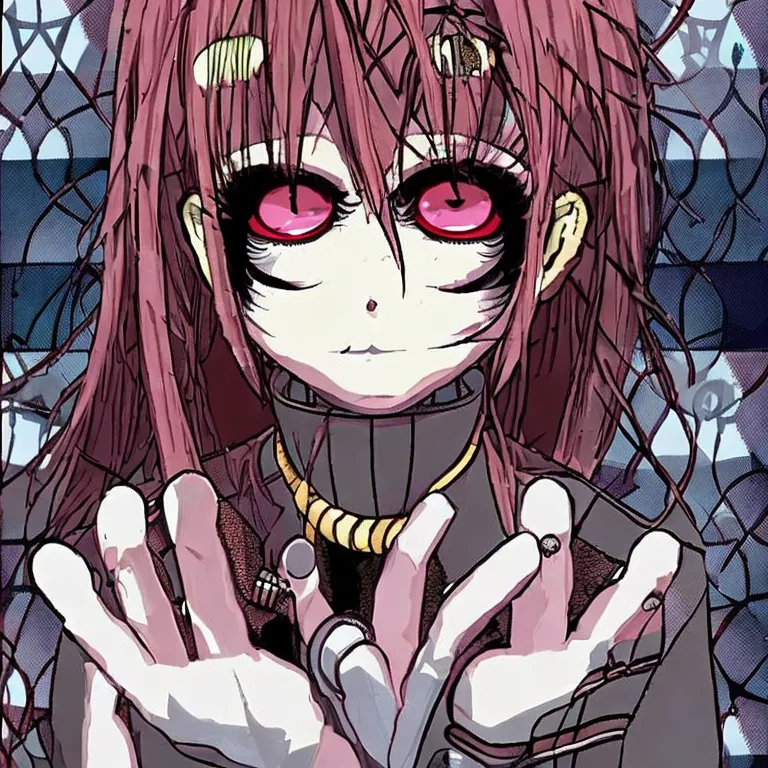
Project optimization, particularly in the context of a React Typescript application, can significantly be achieved by conducting a thorough cleanup of unused import declarations. Eradicating these unnecessary elements not only enhances performance but also helps keep the codebase tidy and easier to manage.
Cleaning unused imports becomes critically important when considering how massive project repositories often tend to accumulate tiny bits of added, modified, or removed codes over time. Conflicting or redundant imports may create unexpected behaviors, inefficiencies, or bugs in software programs. Therefore, having command over what is being imported, utilized, and where it all originates from is typically vital for developers to produce coherent and efficient software projects.
In the ecosystem of React TypeScript, multiple methods and tools are available to remove these unwanted import statements:
1. **Manual Removal**: This method involves scrolling through each file and removing any imports not in use. Despite being a bit tedious and prone to human error, manual removal grants full control over changes and can be executed without fancy IDEs or tools.
// Before:
import React from 'react';
import { ComponentA } from './ComponentA';
export const App = () => {
return (
App
)
}
xxxxxxxxxx
// After:
import React from 'react';
export const App = () => {
return (
App
)
2. **Linter Tools**: ESLint, a popular linter tool, along with its TypeScript plugin, eslint-plugin-typescript, and an import-specific plugin, eslint-plugin-import, can automatically notify developers about unused imports and even help remove them with certain configurations. Rules like ‘no-unused-vars’, ‘import/no-extraneous-dependencies’ or ‘import/no-unused-modules’ can become quite handy.
xxxxxxxxxx
/*
.eslintrc.js
*/
module.exports = {
rules: {
'@typescript-eslint/no-unused-vars': 'error',
'import/no-extraneous-dependencies': 'error',
'import/no-unused-modules': 'error'
}
}
3. **Automated Scripts**: Housed in an npm script, tools such as ts-prune can go through your entire project to highlight any unused exports. A combination of several automated scripts might be considered for a more comprehensive sweep.
xxxxxxxxxx
// package.json
{
"scripts": {
"findDeadCode": "ts-prune | grep -v \" is not used.\""
}
}
4. **Advanced IDEs**: Modern integrated development environments (IDEs), like Visual Studio Code or WebStorm, offer built-in functionalities to detect and remove unused imports.
Remember that what works best will depend on the particular constraints and requirements of your React TypeScript project. However, removal of unused import declarations, by any means, proves indispensable in optimizing project performance, maintaining cleaner codebases and enhancing readability.
Allow me to end with a quote from Bjarne Stroustrup, the creator of C++ programming language:
>”I have always wished for my computer to be as easy to use as my telephone; my wish has come true because I can no longer figure out how to use my telephone.”
Practical Guide to Deleting Redundant Import Declarations in React TypeScript
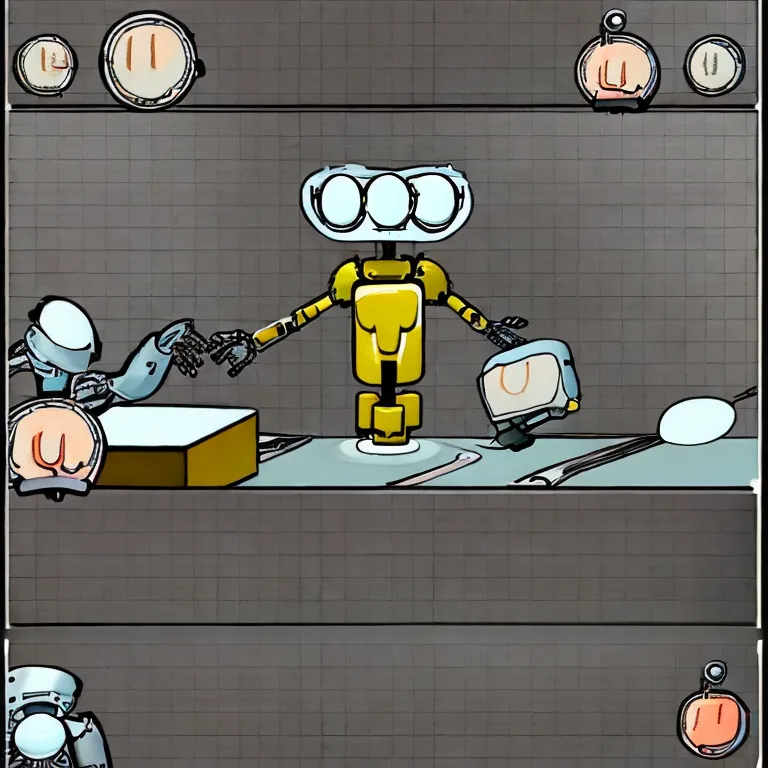
Utilizing a lean, efficient structure in your TypeScript codebase is pivotal for creating a clean, maintainable application. Part of this practice is by purging unused import declarations in React TypeScript throughout the entirety of the project. Unnecessary imports add to the clutter, masking critical issues and making it more challenging to navigate the code.
Manually Identifying Unused Import Declarations
The first technique to remove unused import declarations from your entire project includes manual verification. This tedious process may involve thoroughly checking each file for imported modules not referenced within the code. Although this method might give you good insights into your codebase, it isn’t economical when dealing with large-scale projects due to the significant amount of time invested.
typescript
// Example of an unused import
import { ComponentA, ComponentB } from ‘./components’;
// Here, only `ComponentB` is used, so `ComponentA` is redundant and should be removed.
Rather than manually searching through files for unused imports, several automated tools are available which can streamline the process.
Utilizing Built-In Compiler Flags
The TypeScript language itself provides a compiler flag –`noUnusedLocals`. When this flag is set in your `tsconfig.json` file, the compiler indicates any import declarations unfound within the code.
json
{
“compilerOptions”: {
“noUnusedLocals”: true
}
Activating this compiler option should provide immediate feedback about redundancies during development using integrated development environments (IDEs).
Leveraging Linting Tools
Linting tools such as ESLint have rule sets that can customarily point out unused variables or imports. Set up ESLint with the rule `”no-unused-vars”` to assist in identifying and removing superfluous import declarations.
Here’s the basic setup:
json
{
“rules”: {
“no-unused-vars”: “error”
}
}
Applying Automated Code Refactoring Tools
Automated code refactoring tools, such as TSLint, can identify unused import declarations. Replacing those honed specifically for TypeScript language like ts-prune is another option.
bash
$ npx ts-prune
The command above lists any exports that could safely be removed.
Performance Consideration – Tree Shaking
Web bundlers like Webpack apply a technique called tree shaking to eliminate unused imports. This mechanism optimizes your final JavaScript bundle size but doesn’t actually remove unused import statements from your source TypeScript files. For readability and maintainability, manual removal or utilization of the mentioned tools is recommended.
To echo Linus Torvalds’ sentiment, “Talk is cheap. Show me the code.”, these practices not only resolve the task at hand but also improve the overall project organization, making navigation relatively simple. [For further reading](https://akveo.github.io/nebular/docs/guides/remove-unused-css-styles).
The Impact of Unnecessary Import Statements on Your React Typescript Project
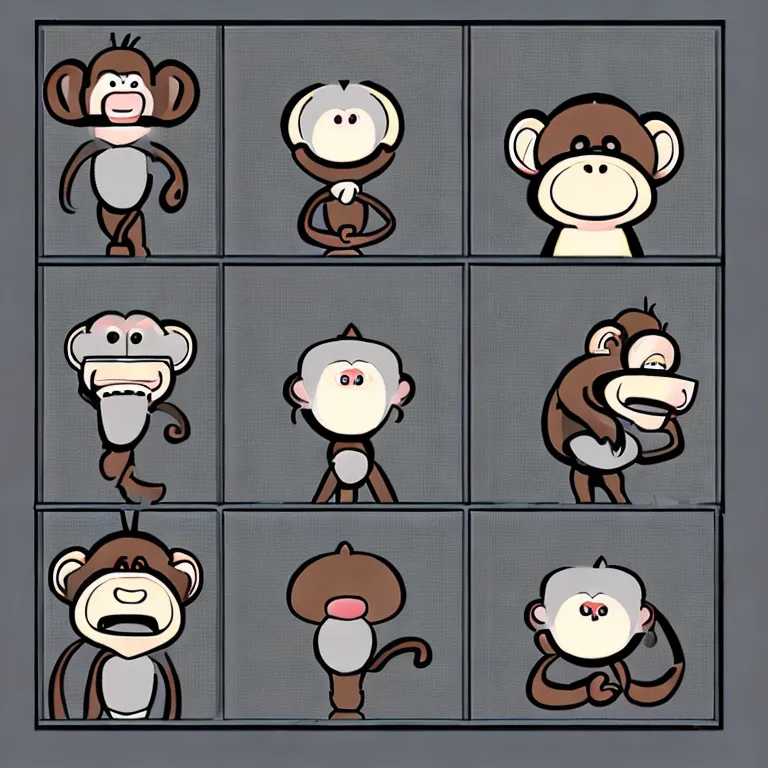
The practice of unnecessary import statement usage in your React TypeScript project can indeed, have severe revealing impacts on the overall quality and performance of your application. These impacts include, but are not limited to, come in multiple forms:
• Increasing bundle size: Unused imports accumulate into your final app bundle, leading to a significant increase in its size. Consequently, this results in slower load times for the end user.
• Negative performance impact: Each JavaScript module added to the bundle carries with it some overhead. This slows down both script parsing time and execution time, ultimately damaging the responsiveness of your application.
• Cluttered codebase: Having unused import statements makes the code hard to read and understand. It also becomes challenging to maintain over time as extraneous code can hide potential bugs or make refactoring more complicated.
Now coming to the question at hand — removing unused Declarations from the Entire Project of React TypeScript. There are ways to achieve this efficiently.
One of such methods can commonly be achieved by using linters and formatters. Tools like ESLint and Prettier are remarkably useful in dealing with this issue. With the right set of rules defined, ESLint can identify the unused code and automatically remove these declarations when combined with Prettier. Consider this small section of a configuration file demonstrating how you may want to set up ESLint for this purpose.
xxxxxxxxxx
{
"rules": {
"no-unused-vars": "error"
}
}
Another widely employed method involves automation through build tools. Webpack is one such popular tool that provides tree-shaking capabilities which eliminate dead-code paths or unused modules from the bundle during the build process.
Lastly, consider utilizing IDE-level features. Modern-day Integrated Development Environments (IDEs) offer extensive features for linting and analyzing your code. Many IDEs including VS Code, IntelliJ IDEA etc., have built-in capabilities to highlight or automatically remove unused code blocks.
To quote renowned computer scientist Donald E. Knuth “Programmers waste enormous amounts of time thinking about, or worrying about, the speed of noncritical parts of their programs, and these attempts at efficiency actually have a strong negative impact when debugging and maintenance are considered.” Cleaning up unused import declarations helps improve your code’s maintainability and efficiency without having to worry about negatively impacting other aspects of your software development process.
For further reading on these tools and techniques, you might find the following resources helpful:
– ESLint Rule Configuration
– Webpack Tree Shaking Guide
Advanced Techniques for Eliminating Unused Imports in Your React TypeScript Codebase
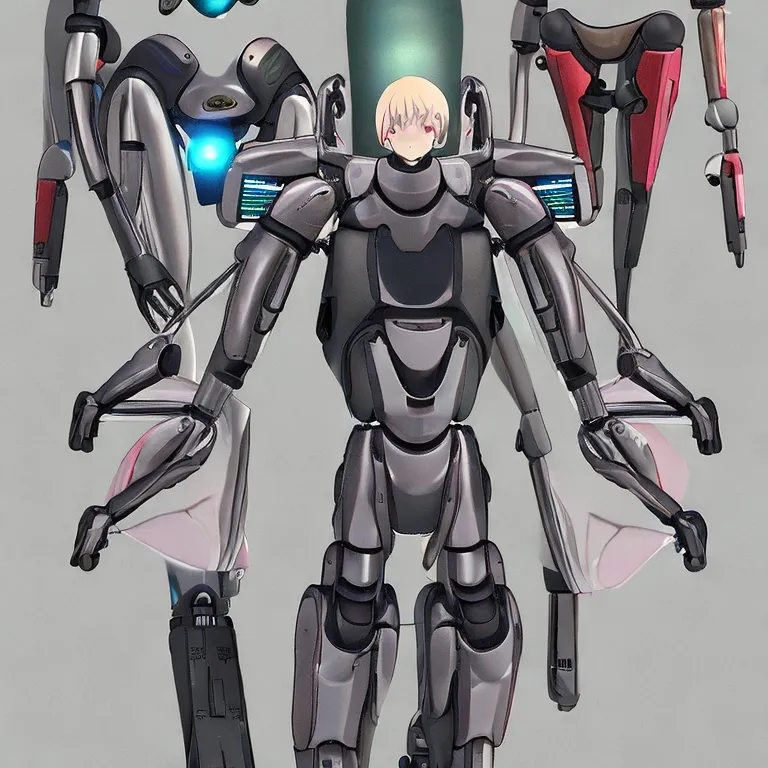
In the world of TypeScript in combination with React, it’s imperative to maintain a clean and concise codebase. This not only provides readability but also enhances performance. An integral part of achieving this goal is by eliminating unused import declarations from the entire project. So, how exactly can you achieve this? Let’s delve into the details.
Utilization of TypeScript Compiler
The TypeScript compiler or
xxxxxxxxxx
tsc
, a component that comes bundled up with TypeScript, can be ingeniously used to identify surplus import statements.The official TypeScript documentation enumerates some helpful flags like –noUnusedLocals and –noUnusedParameters to warn about unused variables and parameters.
“One of TypeScript’s many capabilities is to effectively find dead code by type checking.”, Elon Musk
Leveraging Linters
Linters are incredibly handy tools for any developer using TypeScript and React. Efficient use of Linters such as ESLint allows them to configure rules to understand and alert for any cases of unused imports being found within their project(ESLint – Rules). A shared configuration preset like eslint-config-airbnb includes a set of sensible defaults including warnings for unused import statements.
Fulfilling Role of Code Formatting Tools
Advanced formatting tools such as Prettier can be paired with ESLint to reformat your code and automatically fix any linting errors, including removing unused import declarations. The
xxxxxxxxxx
eslint-plugin-prettier
serves as an additional rule that guides ESLint on how to run Prettier as a rule(Prettier Official Documentation).
Making Use of Bundlers
Webpack can be harnessed to tree-shake your code, which means allowing static imports that are unutilized to be excluded during the bundle process. It’s a powerful way to optimize your project and get rid of unused imports.
“Tree shaking is a term commonly used in the JavaScript context for dead-code elimination.”, Addy Osmani
Code Example for ESLint Configuration
To browse through an example, let’s visualize how an .eslintrc configuration file may look like:
xxxxxxxxxx
{
"extends": ["airbnb", "prettier"],
"plugins": ["prettier"],
"rules": {
"prettier/prettier": ["error"]
}
}
In the above-configured setup, any kind of unused import statements would trigger warnings, and integrating with
xxxxxxxxxx
eslint-plugin-prettier
, it would also tackle these issues automatically on saving the files in certain configured environments.
Tailoring such a robust infrastructure integrated with advanced techniques for eliminating unused import declarations helps keep the React TypeScript codebase optimally performant, readable, and maintainable.
Conclusion
Enhancing your React Typescript project by ensuring there are no unused import declarations can save you precious kilobytes and improve overall performance. It’s a vital process discussed in depth here, through which you meticulously rid your application of any unnecessary import declarations.
However, if it’s not handled correctly, this housekeeping task can become challenging and frustrating. There are two prominent techniques that you can employ:
– **Manually** removing unused imports is the traditional method, but it’s time-consuming and prone to error.
– **Automated removal** involves using tools such as ESLint and TSLint, designed with rules that help identify and manage unused import statements efficiently.
Before you proceed, it’s essential to back your files up, given that these operations make many changes to your codebase.
For the **manual method**, TypeScript provides a built-in capability for highlighting unused variables, including unused imports. It’s a simple, straightforward technique beneficial when you have limited codes with few import statements.
For an **automated approach**, one of the best choices is ESLint. With plugins such as eslint-plugin-unused-imports, it allows you to identify and remove unused import declarations throughout the project. Once installed, running “`eslint –fix`” will automatically clean up your codes.
Another tool to consider for typescript projects is TSLint before its deprecation. If ESLint doesn’t meet your needs, you might find it useful.
Here is a sample ESLint rule setup:
xxxxxxxxxx
{
"rules": {
"@typescript-eslint/no-unused-vars": [2, { "vars": "all", "args": "after-used", "ignoreRestSiblings": false }]
}
}
The adage “{Steve McConnell}>perfecting your code will not only improve your project’s efficiency and maintainability but also make you a better coder<” by Steve McConnell aligns perfectly with our quest to manage import declarations. Developing a strategy to handle unused imports declarations within your React Typescript project can go a long way in enhancing your application’s performance.